mirror of
https://github.com/zitadel/zitadel.git
synced 2025-07-02 04:59:18 +00:00
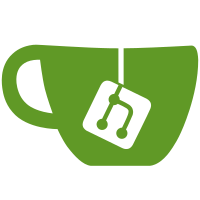
* feat(crypto): use passwap for machine and app secrets * fix command package tests * add hash generator command test * naming convention, fix query tests * rename PasswordHasher and cleanup start commands * add reducer tests * fix intergration tests, cleanup old config * add app secret unit tests * solve setup panics * fix push of updated events * add missing event translations * update documentation * solve linter errors * remove nolint:SA1019 as it doesn't seem to help anyway * add nolint to deprecated filter usage * update users migration version * remove unused ClientSecret from APIConfigChangedEvent --------- Co-authored-by: Livio Spring <livio.a@gmail.com>
72 lines
3.2 KiB
Go
72 lines
3.2 KiB
Go
package command
|
|
|
|
import (
|
|
"context"
|
|
"io"
|
|
|
|
"github.com/zitadel/zitadel/internal/api/authz"
|
|
"github.com/zitadel/zitadel/internal/domain"
|
|
"github.com/zitadel/zitadel/internal/repository/user"
|
|
"github.com/zitadel/zitadel/internal/zerrors"
|
|
)
|
|
|
|
// RequestPasswordReset generates a code
|
|
// and triggers a notification e-mail with the default confirmation URL format.
|
|
func (c *Commands) RequestPasswordReset(ctx context.Context, userID string) (*domain.ObjectDetails, *string, error) {
|
|
return c.requestPasswordReset(ctx, userID, false, "", domain.NotificationTypeEmail)
|
|
}
|
|
|
|
// RequestPasswordResetURLTemplate generates a code
|
|
// and triggers a notification e-mail with the confirmation URL rendered from the passed urlTmpl.
|
|
// urlTmpl must be a valid [tmpl.Template].
|
|
func (c *Commands) RequestPasswordResetURLTemplate(ctx context.Context, userID, urlTmpl string, notificationType domain.NotificationType) (*domain.ObjectDetails, *string, error) {
|
|
if err := domain.RenderConfirmURLTemplate(io.Discard, urlTmpl, userID, "code", "orgID"); err != nil {
|
|
return nil, nil, err
|
|
}
|
|
return c.requestPasswordReset(ctx, userID, false, urlTmpl, notificationType)
|
|
}
|
|
|
|
// RequestPasswordResetReturnCode generates a code and does not send a notification email.
|
|
// The generated plain text code will be returned.
|
|
func (c *Commands) RequestPasswordResetReturnCode(ctx context.Context, userID string) (*domain.ObjectDetails, *string, error) {
|
|
return c.requestPasswordReset(ctx, userID, true, "", 0)
|
|
}
|
|
|
|
// requestPasswordReset creates a code for a password change.
|
|
// returnCode controls if the plain text version of the code will be set in the return object.
|
|
// When the plain text code is returned, no notification e-mail will be sent to the user.
|
|
// urlTmpl allows changing the target URL that is used by the e-mail and should be a validated Go template, if used.
|
|
func (c *Commands) requestPasswordReset(ctx context.Context, userID string, returnCode bool, urlTmpl string, notificationType domain.NotificationType) (_ *domain.ObjectDetails, plainCode *string, err error) {
|
|
if userID == "" {
|
|
return nil, nil, zerrors.ThrowInvalidArgument(nil, "COMMAND-SAFdda", "Errors.User.IDMissing")
|
|
}
|
|
model, err := c.getHumanWriteModelByID(ctx, userID, "")
|
|
if err != nil {
|
|
return nil, nil, err
|
|
}
|
|
if !model.UserState.Exists() {
|
|
return nil, nil, zerrors.ThrowNotFound(nil, "COMMAND-SAF4f", "Errors.User.NotFound")
|
|
}
|
|
if model.UserState == domain.UserStateInitial {
|
|
return nil, nil, zerrors.ThrowPreconditionFailed(nil, "COMMAND-Sfe4g", "Errors.User.NotInitialised")
|
|
}
|
|
if authz.GetCtxData(ctx).UserID != userID {
|
|
if err = c.checkPermission(ctx, domain.PermissionUserWrite, model.ResourceOwner, userID); err != nil {
|
|
return nil, nil, err
|
|
}
|
|
}
|
|
code, err := c.newEncryptedCode(ctx, c.eventstore.Filter, domain.SecretGeneratorTypePasswordResetCode, c.userEncryption) //nolint:staticcheck
|
|
if err != nil {
|
|
return nil, nil, err
|
|
}
|
|
cmd := user.NewHumanPasswordCodeAddedEventV2(ctx, UserAggregateFromWriteModel(&model.WriteModel), code.Crypted, code.Expiry, notificationType, urlTmpl, returnCode)
|
|
|
|
if returnCode {
|
|
plainCode = &code.Plain
|
|
}
|
|
if err = c.pushAppendAndReduce(ctx, model, cmd); err != nil {
|
|
return nil, nil, err
|
|
}
|
|
return writeModelToObjectDetails(&model.WriteModel), plainCode, nil
|
|
}
|