mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
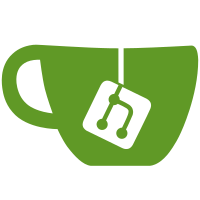
* add token exchange feature flag * allow setting reason and actor to access tokens * impersonation * set token types and scopes in response * upgrade oidc to working draft state * fix tests * audience and scope validation * id toke and jwt as input * return id tokens * add grant type token exchange to app config * add integration tests * check and deny actors in api calls * fix instance setting tests by triggering projection on write and cleanup * insert sleep statements again * solve linting issues * add translations * pin oidc v3.15.0 * resolve comments, add event translation * fix refreshtoken test * use ValidateAuthReqScopes from oidc * apparently the linter can't make up its mind * persist actor thru refresh tokens and check in tests * remove unneeded triggers
189 lines
5.1 KiB
Go
189 lines
5.1 KiB
Go
package user
|
|
|
|
import (
|
|
"context"
|
|
"time"
|
|
|
|
"github.com/zitadel/zitadel/internal/domain"
|
|
"github.com/zitadel/zitadel/internal/eventstore"
|
|
"github.com/zitadel/zitadel/internal/zerrors"
|
|
)
|
|
|
|
const (
|
|
refreshTokenEventPrefix = humanEventPrefix + "refresh.token."
|
|
HumanRefreshTokenAddedType = refreshTokenEventPrefix + "added"
|
|
HumanRefreshTokenRenewedType = refreshTokenEventPrefix + "renewed"
|
|
HumanRefreshTokenRemovedType = refreshTokenEventPrefix + "removed"
|
|
)
|
|
|
|
type HumanRefreshTokenAddedEvent struct {
|
|
eventstore.BaseEvent `json:"-"`
|
|
|
|
TokenID string `json:"tokenId"`
|
|
ClientID string `json:"clientId"`
|
|
UserAgentID string `json:"userAgentId"`
|
|
Audience []string `json:"audience"`
|
|
Scopes []string `json:"scopes"`
|
|
AuthMethodsReferences []string `json:"authMethodReferences"`
|
|
AuthTime time.Time `json:"authTime"`
|
|
IdleExpiration time.Duration `json:"idleExpiration"`
|
|
Expiration time.Duration `json:"expiration"`
|
|
PreferredLanguage string `json:"preferredLanguage"`
|
|
Actor *domain.TokenActor `json:"actor,omitempty"`
|
|
}
|
|
|
|
func (e *HumanRefreshTokenAddedEvent) Payload() interface{} {
|
|
return e
|
|
}
|
|
|
|
func (e *HumanRefreshTokenAddedEvent) UniqueConstraints() []*eventstore.UniqueConstraint {
|
|
return nil
|
|
}
|
|
|
|
func (e *HumanRefreshTokenAddedEvent) Assets() []*eventstore.Asset {
|
|
return nil
|
|
}
|
|
|
|
func NewHumanRefreshTokenAddedEvent(
|
|
ctx context.Context,
|
|
aggregate *eventstore.Aggregate,
|
|
tokenID,
|
|
clientID,
|
|
userAgentID,
|
|
preferredLanguage string,
|
|
audience,
|
|
scopes,
|
|
authMethodsReferences []string,
|
|
authTime time.Time,
|
|
idleExpiration,
|
|
expiration time.Duration,
|
|
actor *domain.TokenActor,
|
|
) *HumanRefreshTokenAddedEvent {
|
|
return &HumanRefreshTokenAddedEvent{
|
|
BaseEvent: *eventstore.NewBaseEventForPush(
|
|
ctx,
|
|
aggregate,
|
|
HumanRefreshTokenAddedType,
|
|
),
|
|
TokenID: tokenID,
|
|
ClientID: clientID,
|
|
UserAgentID: userAgentID,
|
|
Audience: audience,
|
|
Scopes: scopes,
|
|
AuthMethodsReferences: authMethodsReferences,
|
|
AuthTime: authTime,
|
|
IdleExpiration: idleExpiration,
|
|
Expiration: expiration,
|
|
PreferredLanguage: preferredLanguage,
|
|
Actor: actor,
|
|
}
|
|
}
|
|
|
|
func HumanRefreshTokenAddedEventMapper(event eventstore.Event) (eventstore.Event, error) {
|
|
refreshTokenAdded := &HumanRefreshTokenAddedEvent{
|
|
BaseEvent: *eventstore.BaseEventFromRepo(event),
|
|
}
|
|
err := event.Unmarshal(refreshTokenAdded)
|
|
if err != nil {
|
|
return nil, zerrors.ThrowInternal(err, "USER-DGr14", "unable to unmarshal refresh token added")
|
|
}
|
|
|
|
return refreshTokenAdded, nil
|
|
}
|
|
|
|
type HumanRefreshTokenRenewedEvent struct {
|
|
eventstore.BaseEvent `json:"-"`
|
|
|
|
TokenID string `json:"tokenId"`
|
|
RefreshToken string `json:"refreshToken"`
|
|
IdleExpiration time.Duration `json:"idleExpiration"`
|
|
}
|
|
|
|
func (e *HumanRefreshTokenRenewedEvent) Payload() interface{} {
|
|
return e
|
|
}
|
|
|
|
func (e *HumanRefreshTokenRenewedEvent) UniqueConstraints() []*eventstore.UniqueConstraint {
|
|
return nil
|
|
}
|
|
|
|
func (e *HumanRefreshTokenRenewedEvent) Assets() []*eventstore.Asset {
|
|
return nil
|
|
}
|
|
|
|
func NewHumanRefreshTokenRenewedEvent(
|
|
ctx context.Context,
|
|
aggregate *eventstore.Aggregate,
|
|
tokenID,
|
|
refreshToken string,
|
|
idleExpiration time.Duration,
|
|
) *HumanRefreshTokenRenewedEvent {
|
|
return &HumanRefreshTokenRenewedEvent{
|
|
BaseEvent: *eventstore.NewBaseEventForPush(
|
|
ctx,
|
|
aggregate,
|
|
HumanRefreshTokenRenewedType,
|
|
),
|
|
TokenID: tokenID,
|
|
IdleExpiration: idleExpiration,
|
|
RefreshToken: refreshToken,
|
|
}
|
|
}
|
|
|
|
func HumanRefreshTokenRenewedEventEventMapper(event eventstore.Event) (eventstore.Event, error) {
|
|
tokenAdded := &HumanRefreshTokenRenewedEvent{
|
|
BaseEvent: *eventstore.BaseEventFromRepo(event),
|
|
}
|
|
err := event.Unmarshal(tokenAdded)
|
|
if err != nil {
|
|
return nil, zerrors.ThrowInternal(err, "USER-GBt21", "unable to unmarshal refresh token renewed")
|
|
}
|
|
|
|
return tokenAdded, nil
|
|
}
|
|
|
|
type HumanRefreshTokenRemovedEvent struct {
|
|
eventstore.BaseEvent `json:"-"`
|
|
|
|
TokenID string `json:"tokenId"`
|
|
}
|
|
|
|
func (e *HumanRefreshTokenRemovedEvent) Payload() interface{} {
|
|
return e
|
|
}
|
|
|
|
func (e *HumanRefreshTokenRemovedEvent) UniqueConstraints() []*eventstore.UniqueConstraint {
|
|
return nil
|
|
}
|
|
|
|
func (e *HumanRefreshTokenRemovedEvent) Assets() []*eventstore.Asset {
|
|
return nil
|
|
}
|
|
|
|
func NewHumanRefreshTokenRemovedEvent(
|
|
ctx context.Context,
|
|
aggregate *eventstore.Aggregate,
|
|
tokenID string,
|
|
) *HumanRefreshTokenRemovedEvent {
|
|
return &HumanRefreshTokenRemovedEvent{
|
|
BaseEvent: *eventstore.NewBaseEventForPush(
|
|
ctx,
|
|
aggregate,
|
|
HumanRefreshTokenRemovedType,
|
|
),
|
|
TokenID: tokenID,
|
|
}
|
|
}
|
|
|
|
func HumanRefreshTokenRemovedEventEventMapper(event eventstore.Event) (eventstore.Event, error) {
|
|
tokenAdded := &HumanRefreshTokenRemovedEvent{
|
|
BaseEvent: *eventstore.BaseEventFromRepo(event),
|
|
}
|
|
err := event.Unmarshal(tokenAdded)
|
|
if err != nil {
|
|
return nil, zerrors.ThrowInternal(err, "USER-Dggs2", "unable to unmarshal refresh token removed")
|
|
}
|
|
|
|
return tokenAdded, nil
|
|
}
|