mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
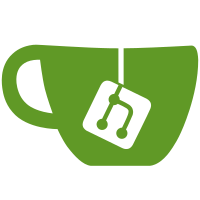
* fix(oidcsettings): corrected projection, unittests and added the add endpoint * fix(oidcsettings): corrected default handling and instance setup * fix: set oidc settings correctly in console * cleanup * e2e test * improve e2e test * lint e2e Co-authored-by: Livio Spring <livio.a@gmail.com> Co-authored-by: Fabi <38692350+hifabienne@users.noreply.github.com>
40 lines
1.2 KiB
Go
40 lines
1.2 KiB
Go
package admin
|
|
|
|
import (
|
|
"context"
|
|
|
|
"github.com/zitadel/zitadel/internal/api/authz"
|
|
"github.com/zitadel/zitadel/internal/api/grpc/object"
|
|
admin_pb "github.com/zitadel/zitadel/pkg/grpc/admin"
|
|
)
|
|
|
|
func (s *Server) GetOIDCSettings(ctx context.Context, _ *admin_pb.GetOIDCSettingsRequest) (*admin_pb.GetOIDCSettingsResponse, error) {
|
|
result, err := s.query.OIDCSettingsByAggID(ctx, authz.GetInstance(ctx).InstanceID())
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return &admin_pb.GetOIDCSettingsResponse{
|
|
Settings: OIDCSettingsToPb(result),
|
|
}, nil
|
|
}
|
|
|
|
func (s *Server) AddOIDCSettings(ctx context.Context, req *admin_pb.AddOIDCSettingsRequest) (*admin_pb.AddOIDCSettingsResponse, error) {
|
|
result, err := s.command.AddOIDCSettings(ctx, AddOIDCConfigToConfig(req))
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return &admin_pb.AddOIDCSettingsResponse{
|
|
Details: object.DomainToChangeDetailsPb(result),
|
|
}, nil
|
|
}
|
|
|
|
func (s *Server) UpdateOIDCSettings(ctx context.Context, req *admin_pb.UpdateOIDCSettingsRequest) (*admin_pb.UpdateOIDCSettingsResponse, error) {
|
|
result, err := s.command.ChangeOIDCSettings(ctx, UpdateOIDCConfigToConfig(req))
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return &admin_pb.UpdateOIDCSettingsResponse{
|
|
Details: object.DomainToChangeDetailsPb(result),
|
|
}, nil
|
|
}
|