mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 02:54:20 +00:00
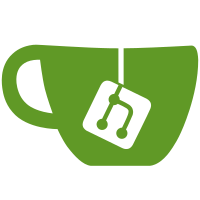
* project quota added * project quota removed * add periods table * make log record generic * accumulate usage * query usage * count action run seconds * fix filter in ReportQuotaUsage * fix existing tests * fix logstore tests * fix typo * fix: add quota unit tests command side * fix: add quota unit tests command side * fix: add quota unit tests command side * move notifications into debouncer and improve limit querying * cleanup * comment * fix: add quota unit tests command side * fix remaining quota usage query * implement InmemLogStorage * cleanup and linting * improve test * fix: add quota unit tests command side * fix: add quota unit tests command side * fix: add quota unit tests command side * fix: add quota unit tests command side * action notifications and fixes for notifications query * revert console prefix * fix: add quota unit tests command side * fix: add quota integration tests * improve accountable requests * improve accountable requests * fix: add quota integration tests * fix: add quota integration tests * fix: add quota integration tests * comment * remove ability to store logs in db and other changes requested from review * changes requested from review * changes requested from review * Update internal/api/http/middleware/access_interceptor.go Co-authored-by: Silvan <silvan.reusser@gmail.com> * tests: fix quotas integration tests * improve incrementUsageStatement * linting * fix: delete e2e tests as intergation tests cover functionality * Update internal/api/http/middleware/access_interceptor.go Co-authored-by: Silvan <silvan.reusser@gmail.com> * backup * fix conflict * create rc * create prerelease * remove issue release labeling * fix tracing --------- Co-authored-by: Livio Spring <livio.a@gmail.com> Co-authored-by: Stefan Benz <stefan@caos.ch> Co-authored-by: adlerhurst <silvan.reusser@gmail.com>
63 lines
1.8 KiB
Go
63 lines
1.8 KiB
Go
package command
|
|
|
|
import (
|
|
"context"
|
|
|
|
"github.com/zitadel/zitadel/internal/eventstore"
|
|
"github.com/zitadel/zitadel/internal/repository/quota"
|
|
"github.com/zitadel/zitadel/internal/telemetry/tracing"
|
|
)
|
|
|
|
// ReportQuotaUsage writes a slice of *quota.NotificationDueEvent directly to the eventstore
|
|
func (c *Commands) ReportQuotaUsage(ctx context.Context, dueNotifications []*quota.NotificationDueEvent) (err error) {
|
|
ctx, span := tracing.NewSpan(ctx)
|
|
defer func() { span.EndWithError(err) }()
|
|
|
|
cmds := make([]eventstore.Command, 0, len(dueNotifications))
|
|
for _, notification := range dueNotifications {
|
|
ctxFilter, spanFilter := tracing.NewNamedSpan(ctx, "filterNotificationDueEvents")
|
|
events, errFilter := c.eventstore.Filter(
|
|
ctxFilter,
|
|
eventstore.NewSearchQueryBuilder(eventstore.ColumnsEvent).
|
|
InstanceID(notification.Aggregate().InstanceID).
|
|
AddQuery().
|
|
AggregateTypes(quota.AggregateType).
|
|
AggregateIDs(notification.Aggregate().ID).
|
|
EventTypes(quota.NotificationDueEventType).
|
|
EventData(map[string]interface{}{
|
|
"id": notification.ID,
|
|
"periodStart": notification.PeriodStart,
|
|
"threshold": notification.Threshold,
|
|
}).Builder(),
|
|
)
|
|
spanFilter.EndWithError(errFilter)
|
|
if errFilter != nil {
|
|
return errFilter
|
|
}
|
|
if len(events) > 0 {
|
|
continue
|
|
}
|
|
cmds = append(cmds, notification)
|
|
}
|
|
if len(cmds) == 0 {
|
|
return nil
|
|
}
|
|
ctxPush, spanPush := tracing.NewNamedSpan(ctx, "pushNotificationDueEvents")
|
|
_, errPush := c.eventstore.Push(ctxPush, cmds...)
|
|
spanPush.EndWithError(errPush)
|
|
return errPush
|
|
}
|
|
|
|
func (c *Commands) UsageNotificationSent(ctx context.Context, dueEvent *quota.NotificationDueEvent) error {
|
|
id, err := c.idGenerator.Next()
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
_, err = c.eventstore.Push(
|
|
ctx,
|
|
quota.NewNotifiedEvent(ctx, id, dueEvent),
|
|
)
|
|
return err
|
|
}
|