mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
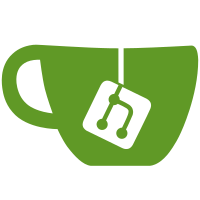
This pr upgrades oidc to v3 . Function signature changes have been migrated as well. Specifically there are more client calls that take a context now. Where feasable a context is added to those calls. Where a context is not (easily) available context.TODO() is used as a reminder for when it does. Related to #6619
46 lines
1.6 KiB
Go
46 lines
1.6 KiB
Go
package gitlab
|
|
|
|
import (
|
|
openid "github.com/zitadel/oidc/v3/pkg/oidc"
|
|
|
|
"github.com/zitadel/zitadel/internal/idp"
|
|
"github.com/zitadel/zitadel/internal/idp/providers/oidc"
|
|
)
|
|
|
|
const (
|
|
issuer = "https://gitlab.com"
|
|
name = "GitLab"
|
|
)
|
|
|
|
var _ idp.Provider = (*Provider)(nil)
|
|
|
|
// Provider is the [idp.Provider] implementation for Gitlab
|
|
type Provider struct {
|
|
*oidc.Provider
|
|
}
|
|
|
|
// New creates a GitLab.com provider using the [oidc.Provider] (OIDC generic provider)
|
|
func New(clientID, clientSecret, redirectURI string, scopes []string, options ...oidc.ProviderOpts) (*Provider, error) {
|
|
return NewCustomIssuer(name, issuer, clientID, clientSecret, redirectURI, scopes, options...)
|
|
}
|
|
|
|
// NewCustomIssuer creates a GitLab provider using the [oidc.Provider] (OIDC generic provider)
|
|
// with a custom issuer for self-managed instances
|
|
func NewCustomIssuer(name, issuer, clientID, clientSecret, redirectURI string, scopes []string, options ...oidc.ProviderOpts) (*Provider, error) {
|
|
if len(scopes) == 0 {
|
|
// the OIDC provider would set `openid profile email phone` as default scope,
|
|
// but since gitlab does not handle unknown scopes correctly (phone) and returns an error,
|
|
// we will just set a separate default list
|
|
scopes = []string{openid.ScopeOpenID, openid.ScopeProfile, openid.ScopeEmail}
|
|
}
|
|
// gitlab is currently not able to handle the prompt `select_account`:
|
|
// https://gitlab.com/gitlab-org/gitlab/-/issues/377368
|
|
rp, err := oidc.New(name, issuer, clientID, clientSecret, redirectURI, scopes, oidc.DefaultMapper, options...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return &Provider{
|
|
Provider: rp,
|
|
}, nil
|
|
}
|