mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
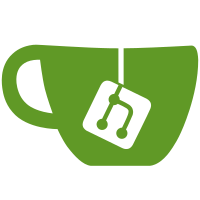
* feat: first implementation for saml sp * fix: add command side instance and org for saml provider * fix: add query side instance and org for saml provider * fix: request handling in event and retrieval of finished intent * fix: add review changes and integration tests * fix: add integration tests for saml idp * fix: correct unit tests with review changes * fix: add saml session unit test * fix: add saml session unit test * fix: add saml session unit test * fix: changes from review * fix: changes from review * fix: proto build error * fix: proto build error * fix: proto build error * fix: proto require metadata oneof * fix: login with saml provider * fix: integration test for saml assertion * lint client.go * fix json tag * fix: linting * fix import * fix: linting * fix saml idp query * fix: linting * lint: try all issues * revert linting config * fix: add regenerate endpoints * fix: translations * fix mk.yaml * ignore acs path for user agent cookie * fix: add AuthFromProvider test for saml * fix: integration test for saml retrieve information --------- Co-authored-by: Livio Spring <livio.a@gmail.com>
69 lines
1.7 KiB
Go
69 lines
1.7 KiB
Go
package gitlab
|
|
|
|
import (
|
|
"context"
|
|
"testing"
|
|
|
|
"github.com/stretchr/testify/assert"
|
|
"github.com/stretchr/testify/require"
|
|
|
|
"github.com/zitadel/zitadel/internal/idp"
|
|
"github.com/zitadel/zitadel/internal/idp/providers/oidc"
|
|
)
|
|
|
|
func TestProvider_BeginAuth(t *testing.T) {
|
|
type fields struct {
|
|
clientID string
|
|
clientSecret string
|
|
redirectURI string
|
|
scopes []string
|
|
opts []oidc.ProviderOpts
|
|
}
|
|
tests := []struct {
|
|
name string
|
|
fields fields
|
|
want idp.Session
|
|
}{
|
|
{
|
|
name: "successful auth",
|
|
fields: fields{
|
|
clientID: "clientID",
|
|
clientSecret: "clientSecret",
|
|
redirectURI: "redirectURI",
|
|
scopes: []string{"openid"},
|
|
},
|
|
want: &oidc.Session{
|
|
AuthURL: "https://gitlab.com/oauth/authorize?client_id=clientID&redirect_uri=redirectURI&response_type=code&scope=openid&state=testState",
|
|
},
|
|
},
|
|
{
|
|
name: "successful auth default scopes",
|
|
fields: fields{
|
|
clientID: "clientID",
|
|
clientSecret: "clientSecret",
|
|
redirectURI: "redirectURI",
|
|
},
|
|
want: &oidc.Session{
|
|
AuthURL: "https://gitlab.com/oauth/authorize?client_id=clientID&redirect_uri=redirectURI&response_type=code&scope=openid+profile+email&state=testState",
|
|
},
|
|
},
|
|
}
|
|
for _, tt := range tests {
|
|
t.Run(tt.name, func(t *testing.T) {
|
|
a := assert.New(t)
|
|
r := require.New(t)
|
|
|
|
provider, err := New(tt.fields.clientID, tt.fields.clientSecret, tt.fields.redirectURI, tt.fields.scopes, tt.fields.opts...)
|
|
r.NoError(err)
|
|
ctx := context.Background()
|
|
session, err := provider.BeginAuth(ctx, "testState")
|
|
r.NoError(err)
|
|
|
|
wantHeaders, wantContent := tt.want.GetAuth(ctx)
|
|
gotHeaders, gotContent := session.GetAuth(ctx)
|
|
a.Equal(wantHeaders, gotHeaders)
|
|
a.Equal(wantContent, gotContent)
|
|
})
|
|
}
|
|
}
|