mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 19:14:23 +00:00
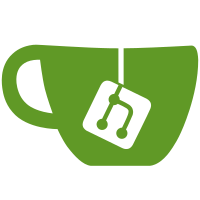
This implementation increases parallel write capabilities of the eventstore. Please have a look at the technical advisories: [05](https://zitadel.com/docs/support/advisory/a10005) and [06](https://zitadel.com/docs/support/advisory/a10006). The implementation of eventstore.push is rewritten and stored events are migrated to a new table `eventstore.events2`. If you are using cockroach: make sure that the database user of ZITADEL has `VIEWACTIVITY` grant. This is used to query events.
49 lines
1.4 KiB
Go
49 lines
1.4 KiB
Go
package senders
|
|
|
|
import (
|
|
"context"
|
|
|
|
"github.com/zitadel/logging"
|
|
"github.com/zitadel/zitadel/internal/api/authz"
|
|
"github.com/zitadel/zitadel/internal/notification/channels"
|
|
"github.com/zitadel/zitadel/internal/notification/channels/fs"
|
|
"github.com/zitadel/zitadel/internal/notification/channels/instrumenting"
|
|
"github.com/zitadel/zitadel/internal/notification/channels/log"
|
|
"github.com/zitadel/zitadel/internal/notification/channels/webhook"
|
|
)
|
|
|
|
const webhookSpanName = "webhook.NotificationChannel"
|
|
|
|
func WebhookChannels(
|
|
ctx context.Context,
|
|
webhookConfig webhook.Config,
|
|
getFileSystemProvider func(ctx context.Context) (*fs.Config, error),
|
|
getLogProvider func(ctx context.Context) (*log.Config, error),
|
|
successMetricName,
|
|
failureMetricName string,
|
|
) (*Chain, error) {
|
|
if err := webhookConfig.Validate(); err != nil {
|
|
return nil, err
|
|
}
|
|
channels := make([]channels.NotificationChannel, 0, 3)
|
|
webhookChannel, err := webhook.InitChannel(ctx, webhookConfig)
|
|
logging.WithFields(
|
|
"instance", authz.GetInstance(ctx).InstanceID(),
|
|
"callurl", webhookConfig.CallURL,
|
|
).OnError(err).Debug("initializing JSON channel failed")
|
|
if err == nil {
|
|
channels = append(
|
|
channels,
|
|
instrumenting.Wrap(
|
|
ctx,
|
|
webhookChannel,
|
|
webhookSpanName,
|
|
successMetricName,
|
|
failureMetricName,
|
|
),
|
|
)
|
|
}
|
|
channels = append(channels, debugChannels(ctx, getFileSystemProvider, getLogProvider)...)
|
|
return ChainChannels(channels...), nil
|
|
}
|