mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-19 14:27:32 +00:00
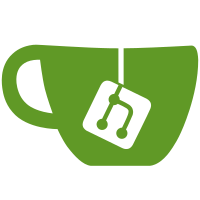
* fix(zitadelctl): implement takedown command * fix(zitadelctl): correct destroy flow * fix(zitadelctl): correct backup commands to read crds beforehand * fix: add of destroyfile * fix: clean for userlist * fix: change backup and restore to crdb native * fix: timeout for delete pvc for cockroachdb * fix: corrected unit tests * fix: add ignored file for scale * fix: correct handling of gitops in backup command * feat: add s3 backup kind * fix: backuplist for s3 and timeout for pv deletion * fix(database): fix nil pointer with binary version * fix(database): cleanup of errors which cam with merging of the s3 logic * fix: correct unit tests * fix: cleanup monitor output Co-authored-by: Elio Bischof <eliobischof@gmail.com> * fix: backup imagepullpolixy to ifnotpresent Co-authored-by: Elio Bischof <eliobischof@gmail.com>
61 lines
1.5 KiB
Go
61 lines
1.5 KiB
Go
package statefulset
|
|
|
|
import (
|
|
"github.com/caos/orbos/mntr"
|
|
"github.com/caos/orbos/pkg/kubernetes"
|
|
"github.com/caos/orbos/pkg/kubernetes/resources"
|
|
"github.com/caos/orbos/pkg/labels"
|
|
macherrs "k8s.io/apimachinery/pkg/api/errors"
|
|
"strings"
|
|
"time"
|
|
)
|
|
|
|
func CleanPVCs(
|
|
monitor mntr.Monitor,
|
|
namespace string,
|
|
sfsSelectable *labels.Selectable,
|
|
replicaCount int,
|
|
) resources.QueryFunc {
|
|
name := sfsSelectable.Name()
|
|
return func(k8sClient kubernetes.ClientInt) (resources.EnsureFunc, error) {
|
|
pvcs, err := k8sClient.ListPersistentVolumeClaims(namespace)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
internalPvcs := []string{}
|
|
for _, pvc := range pvcs.Items {
|
|
if strings.HasPrefix(pvc.Name, datadirInternal+"-"+name) {
|
|
internalPvcs = append(internalPvcs, pvc.Name)
|
|
}
|
|
}
|
|
return func(k8sClient kubernetes.ClientInt) error {
|
|
noSFS := false
|
|
monitor.Info("Scale down statefulset")
|
|
if err := k8sClient.ScaleStatefulset(namespace, name, 0); err != nil {
|
|
if macherrs.IsNotFound(err) {
|
|
noSFS = true
|
|
} else {
|
|
return err
|
|
}
|
|
}
|
|
time.Sleep(2 * time.Second)
|
|
|
|
monitor.Info("Delete persistent volume claims")
|
|
for _, pvcName := range internalPvcs {
|
|
if err := k8sClient.DeletePersistentVolumeClaim(namespace, pvcName, cleanTimeout); err != nil {
|
|
return err
|
|
}
|
|
}
|
|
time.Sleep(2 * time.Second)
|
|
|
|
if !noSFS {
|
|
monitor.Info("Scale up statefulset")
|
|
if err := k8sClient.ScaleStatefulset(namespace, name, replicaCount); err != nil {
|
|
return err
|
|
}
|
|
}
|
|
return nil
|
|
}, nil
|
|
}
|
|
}
|