mirror of
https://github.com/zitadel/zitadel.git
synced 2025-03-01 15:07:24 +00:00
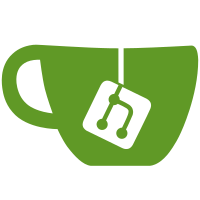
# Which Problems Are Solved - SCIM user metadata mapping keys have differing case styles. # How the Problems Are Solved - key casing style is unified to strict camelCase # Additional Context Part of #8140 Although this is technically a breaking change, it is considered acceptable because the SCIM feature is still in the preview stage and not fully implemented yet. Co-authored-by: Stefan Benz <46600784+stebenz@users.noreply.github.com>
100 lines
2.9 KiB
Go
100 lines
2.9 KiB
Go
package metadata
|
|
|
|
import (
|
|
"context"
|
|
"strings"
|
|
|
|
"github.com/zitadel/zitadel/internal/query"
|
|
)
|
|
|
|
type Key string
|
|
type ScopedKey string
|
|
|
|
const (
|
|
externalIdProvisioningDomainPlaceholder = "{provisioningDomain}"
|
|
|
|
KeyPrefix = "urn:zitadel:scim:"
|
|
KeyProvisioningDomain Key = KeyPrefix + "provisioningDomain"
|
|
|
|
KeyExternalId Key = KeyPrefix + "externalId"
|
|
keyScopedExternalIdTemplate = KeyPrefix + externalIdProvisioningDomainPlaceholder + ":externalId"
|
|
KeyMiddleName Key = KeyPrefix + "name.middleName"
|
|
KeyHonorificPrefix Key = KeyPrefix + "name.honorificPrefix"
|
|
KeyHonorificSuffix Key = KeyPrefix + "name.honorificSuffix"
|
|
KeyProfileUrl Key = KeyPrefix + "profileUrl"
|
|
KeyTitle Key = KeyPrefix + "title"
|
|
KeyLocale Key = KeyPrefix + "locale"
|
|
KeyTimezone Key = KeyPrefix + "timezone"
|
|
KeyIms Key = KeyPrefix + "ims"
|
|
KeyPhotos Key = KeyPrefix + "photos"
|
|
KeyAddresses Key = KeyPrefix + "addresses"
|
|
KeyEntitlements Key = KeyPrefix + "entitlements"
|
|
KeyRoles Key = KeyPrefix + "roles"
|
|
)
|
|
|
|
var (
|
|
ScimUserRelevantMetadataKeys = []Key{
|
|
KeyExternalId,
|
|
KeyMiddleName,
|
|
KeyHonorificPrefix,
|
|
KeyHonorificSuffix,
|
|
KeyProfileUrl,
|
|
KeyTitle,
|
|
KeyLocale,
|
|
KeyTimezone,
|
|
KeyIms,
|
|
KeyPhotos,
|
|
KeyAddresses,
|
|
KeyEntitlements,
|
|
KeyRoles,
|
|
}
|
|
|
|
AttributePathToMetadataKeys = map[string][]Key{
|
|
"externalid": {KeyExternalId},
|
|
"name": {KeyMiddleName, KeyHonorificPrefix, KeyHonorificSuffix},
|
|
"name.middlename": {KeyMiddleName},
|
|
"name.honorificprefix": {KeyHonorificPrefix},
|
|
"name.honorificsuffix": {KeyHonorificSuffix},
|
|
"profileurl": {KeyProfileUrl},
|
|
"title": {KeyTitle},
|
|
"locale": {KeyLocale},
|
|
"timezone": {KeyTimezone},
|
|
"ims": {KeyIms},
|
|
"photos": {KeyPhotos},
|
|
"addresses": {KeyAddresses},
|
|
"entitlements": {KeyEntitlements},
|
|
"roles": {KeyRoles},
|
|
}
|
|
)
|
|
|
|
func ScopeExternalIdKey(provisioningDomain string) ScopedKey {
|
|
return ScopedKey(strings.Replace(keyScopedExternalIdTemplate, externalIdProvisioningDomainPlaceholder, provisioningDomain, 1))
|
|
}
|
|
|
|
func ScopeKey(ctx context.Context, key Key) ScopedKey {
|
|
// only the externalID is scoped
|
|
if key == KeyExternalId {
|
|
return GetScimContextData(ctx).ExternalIDScopedMetadataKey
|
|
}
|
|
|
|
return ScopedKey(key)
|
|
}
|
|
|
|
func MapToScopedKeyMap(md map[string][]byte) map[ScopedKey][]byte {
|
|
result := make(map[ScopedKey][]byte, len(md))
|
|
for k, v := range md {
|
|
result[ScopedKey(k)] = v
|
|
}
|
|
|
|
return result
|
|
}
|
|
|
|
func MapListToScopedKeyMap(metadataList []*query.UserMetadata) map[ScopedKey][]byte {
|
|
metadataMap := make(map[ScopedKey][]byte, len(metadataList))
|
|
for _, entry := range metadataList {
|
|
metadataMap[ScopedKey(entry.Key)] = entry.Value
|
|
}
|
|
|
|
return metadataMap
|
|
}
|