mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 19:14:23 +00:00
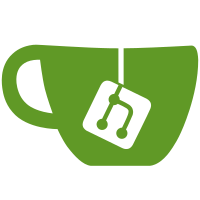
* feat: call webhooks at least once * self review * feat: improve notification observability * feat: add notification tracing * test(e2e): test at-least-once webhook delivery * fix webhook notifications * dedicated quota notifications handler * fix linting * fix e2e test * wait less in e2e test * fix: don't ignore failed events in handlers * fix: don't ignore failed events in handlers * faster requeues * question * fix retries * fix retries * retry * don't instance ids query * revert handler_projection * statements can be nil * cleanup * make unit tests pass * add comments * add comments * lint * spool only active instances * feat(config): handle inactive instances * customizable HandleInactiveInstances * call inactive instances quota webhooks * test: handling with and w/o inactive instances * omit retrying noop statements * docs: describe projection options * enable global handling of inactive instances * self review * requeue quota notifications every 5m * remove caos_errors reference * fix comment styles * make handlers package flat * fix linting * fix repeating quota notifications * test with more usage * debug log channel init failures
53 lines
1.4 KiB
Go
53 lines
1.4 KiB
Go
package fs
|
|
|
|
import (
|
|
"fmt"
|
|
"os"
|
|
"path/filepath"
|
|
"sort"
|
|
"strings"
|
|
"time"
|
|
|
|
"github.com/k3a/html2text"
|
|
"github.com/zitadel/logging"
|
|
|
|
"github.com/zitadel/zitadel/internal/errors"
|
|
"github.com/zitadel/zitadel/internal/notification/channels"
|
|
"github.com/zitadel/zitadel/internal/notification/messages"
|
|
)
|
|
|
|
func InitFSChannel(config Config) (channels.NotificationChannel, error) {
|
|
if err := os.MkdirAll(config.Path, os.ModePerm); err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
logging.Debug("successfully initialized filesystem email and sms channel")
|
|
|
|
return channels.HandleMessageFunc(func(message channels.Message) error {
|
|
|
|
fileName := fmt.Sprintf("%d_", time.Now().Unix())
|
|
content, err := message.GetContent()
|
|
if err != nil {
|
|
return err
|
|
}
|
|
switch msg := message.(type) {
|
|
case *messages.Email:
|
|
recipients := make([]string, len(msg.Recipients))
|
|
copy(recipients, msg.Recipients)
|
|
sort.Strings(recipients)
|
|
fileName = fileName + "mail_to_" + strings.Join(recipients, "_") + ".html"
|
|
if config.Compact {
|
|
content = html2text.HTML2Text(content)
|
|
}
|
|
case *messages.SMS:
|
|
fileName = fileName + "sms_to_" + msg.RecipientPhoneNumber + ".txt"
|
|
case *messages.JSON:
|
|
fileName = "message.json"
|
|
default:
|
|
return errors.ThrowUnimplementedf(nil, "NOTIF-6f9a1", "filesystem provider doesn't support message type %T", message)
|
|
}
|
|
|
|
return os.WriteFile(filepath.Join(config.Path, fileName), []byte(content), 0666)
|
|
}), nil
|
|
}
|