mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 19:14:23 +00:00
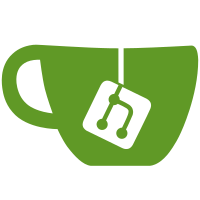
* feat(command): remove org * refactor: imports, unused code, error handling * reduce org removed in action * add org deletion to projections * add org removal to projections * add org removal to projections * org removed projection * lint import * projections * fix: table names in tests * fix: table names in tests * logging * add org state * fix(domain): add Owner removed to object details * feat(ListQuery): add with owner removed * fix(org-delete): add bool to functions to select with owner removed * fix(org-delete): add bools to user grants with events to determine if dependencies lost owner * fix(org-delete): add unit tests for owner removed and org removed events * fix(org-delete): add handling of org remove for grants and members * fix(org-delete): correction of unit tests for owner removed * fix(org-delete): update projections, unit tests and get functions * fix(org-delete): add change date to authnkeys and owner removed to org metadata * fix(org-delete): include owner removed for login names * fix(org-delete): some column fixes in projections and build for queries with owner removed * indexes * fix(org-delete): include review changes * fix(org-delete): change user projection name after merge * fix(org-delete): include review changes for project grant where no project owner is necessary * fix(org-delete): include auth and adminapi tables with owner removed information * fix(org-delete): cleanup username and orgdomain uniqueconstraints when org is removed * fix(org-delete): add permissions for org.remove * remove unnecessary unique constraints * fix column order in primary keys * fix(org-delete): include review changes * fix(org-delete): add owner removed indexes and chang setup step to create tables * fix(org-delete): move PK order of instance_id and change added user_grant from review * fix(org-delete): no params for prepareUserQuery * change to step 6 * merge main * fix(org-delete): OldUserName rename to private * fix linting * cleanup * fix: remove org test * create prerelease * chore: delete org-delete as prerelease Co-authored-by: Stefan Benz <stefan@caos.ch> Co-authored-by: Livio Spring <livio.a@gmail.com> Co-authored-by: Fabi <38692350+hifabienne@users.noreply.github.com> Co-authored-by: Stefan Benz <46600784+stebenz@users.noreply.github.com>
195 lines
6.7 KiB
Go
195 lines
6.7 KiB
Go
package model
|
|
|
|
import (
|
|
"encoding/json"
|
|
"time"
|
|
|
|
"github.com/zitadel/logging"
|
|
|
|
"github.com/zitadel/zitadel/internal/domain"
|
|
caos_errs "github.com/zitadel/zitadel/internal/errors"
|
|
"github.com/zitadel/zitadel/internal/eventstore"
|
|
"github.com/zitadel/zitadel/internal/eventstore/v1/models"
|
|
"github.com/zitadel/zitadel/internal/repository/instance"
|
|
"github.com/zitadel/zitadel/internal/repository/org"
|
|
)
|
|
|
|
const (
|
|
LabelPolicyKeyAggregateID = "aggregate_id"
|
|
LabelPolicyKeyState = "label_policy_state"
|
|
LabelPolicyKeyInstanceID = "instance_id"
|
|
LabelPolicyKeyOwnerRemoved = "owner_removed"
|
|
)
|
|
|
|
type LabelPolicyView struct {
|
|
AggregateID string `json:"-" gorm:"column:aggregate_id;primary_key"`
|
|
State int32 `json:"-" gorm:"column:label_policy_state;primary_key"`
|
|
CreationDate time.Time `json:"-" gorm:"column:creation_date"`
|
|
ChangeDate time.Time `json:"-" gorm:"column:change_date"`
|
|
|
|
PrimaryColor string `json:"primaryColor" gorm:"column:primary_color"`
|
|
BackgroundColor string `json:"backgroundColor" gorm:"column:background_color"`
|
|
WarnColor string `json:"warnColor" gorm:"column:warn_color"`
|
|
FontColor string `json:"fontColor" gorm:"column:font_color"`
|
|
PrimaryColorDark string `json:"primaryColorDark" gorm:"column:primary_color_dark"`
|
|
BackgroundColorDark string `json:"backgroundColorDark" gorm:"column:background_color_dark"`
|
|
WarnColorDark string `json:"warnColorDark" gorm:"column:warn_color_dark"`
|
|
FontColorDark string `json:"fontColorDark" gorm:"column:font_color_dark"`
|
|
LogoURL string `json:"-" gorm:"column:logo_url"`
|
|
IconURL string `json:"-" gorm:"column:icon_url"`
|
|
LogoDarkURL string `json:"-" gorm:"column:logo_dark_url"`
|
|
IconDarkURL string `json:"-" gorm:"column:icon_dark_url"`
|
|
FontURL string `json:"-" gorm:"column:font_url"`
|
|
HideLoginNameSuffix bool `json:"hideLoginNameSuffix" gorm:"column:hide_login_name_suffix"`
|
|
ErrorMsgPopup bool `json:"errorMsgPopup" gorm:"column:err_msg_popup"`
|
|
DisableWatermark bool `json:"disableWatermark" gorm:"column:disable_watermark"`
|
|
Default bool `json:"-" gorm:"-"`
|
|
|
|
Sequence uint64 `json:"-" gorm:"column:sequence"`
|
|
InstanceID string `json:"instanceID" gorm:"column:instance_id;primary_key"`
|
|
}
|
|
|
|
type AssetView struct {
|
|
AssetURL string `json:"storeKey"`
|
|
}
|
|
|
|
func (p *LabelPolicyView) ToDomain() *domain.LabelPolicy {
|
|
return &domain.LabelPolicy{
|
|
ObjectRoot: models.ObjectRoot{
|
|
AggregateID: p.AggregateID,
|
|
CreationDate: p.CreationDate,
|
|
ChangeDate: p.ChangeDate,
|
|
Sequence: p.Sequence,
|
|
},
|
|
Default: p.Default,
|
|
PrimaryColor: p.PrimaryColor,
|
|
BackgroundColor: p.BackgroundColor,
|
|
WarnColor: p.WarnColor,
|
|
FontColor: p.FontColor,
|
|
LogoURL: p.LogoURL,
|
|
IconURL: p.IconURL,
|
|
|
|
PrimaryColorDark: p.PrimaryColorDark,
|
|
BackgroundColorDark: p.BackgroundColorDark,
|
|
WarnColorDark: p.WarnColorDark,
|
|
FontColorDark: p.FontColorDark,
|
|
LogoDarkURL: p.LogoDarkURL,
|
|
IconDarkURL: p.IconDarkURL,
|
|
Font: p.FontURL,
|
|
|
|
HideLoginNameSuffix: p.HideLoginNameSuffix,
|
|
ErrorMsgPopup: p.ErrorMsgPopup,
|
|
DisableWatermark: p.DisableWatermark,
|
|
}
|
|
}
|
|
|
|
func (i *LabelPolicyView) AppendEvent(event *models.Event) (err error) {
|
|
asset := &AssetView{}
|
|
i.Sequence = event.Sequence
|
|
i.ChangeDate = event.CreationDate
|
|
switch eventstore.EventType(event.Type) {
|
|
case instance.LabelPolicyAddedEventType,
|
|
org.LabelPolicyAddedEventType:
|
|
i.setRootData(event)
|
|
i.CreationDate = event.CreationDate
|
|
i.State = int32(domain.LabelPolicyStatePreview)
|
|
err = i.SetData(event)
|
|
case instance.LabelPolicyChangedEventType,
|
|
org.LabelPolicyChangedEventType:
|
|
err = i.SetData(event)
|
|
i.State = int32(domain.LabelPolicyStatePreview)
|
|
case instance.LabelPolicyLogoAddedEventType,
|
|
org.LabelPolicyLogoAddedEventType:
|
|
err = asset.SetData(event)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
i.LogoURL = asset.AssetURL
|
|
i.State = int32(domain.LabelPolicyStatePreview)
|
|
case instance.LabelPolicyLogoRemovedEventType,
|
|
org.LabelPolicyLogoRemovedEventType:
|
|
i.LogoURL = ""
|
|
i.State = int32(domain.LabelPolicyStatePreview)
|
|
case instance.LabelPolicyIconAddedEventType,
|
|
org.LabelPolicyIconAddedEventType:
|
|
err = asset.SetData(event)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
i.IconURL = asset.AssetURL
|
|
i.State = int32(domain.LabelPolicyStatePreview)
|
|
case instance.LabelPolicyIconRemovedEventType,
|
|
org.LabelPolicyIconRemovedEventType:
|
|
i.IconURL = ""
|
|
case instance.LabelPolicyLogoDarkAddedEventType,
|
|
org.LabelPolicyLogoDarkAddedEventType:
|
|
err = asset.SetData(event)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
i.LogoDarkURL = asset.AssetURL
|
|
i.State = int32(domain.LabelPolicyStatePreview)
|
|
case instance.LabelPolicyLogoDarkRemovedEventType,
|
|
org.LabelPolicyLogoDarkRemovedEventType:
|
|
i.LogoDarkURL = ""
|
|
i.State = int32(domain.LabelPolicyStatePreview)
|
|
case instance.LabelPolicyIconDarkAddedEventType,
|
|
org.LabelPolicyIconDarkAddedEventType:
|
|
err = asset.SetData(event)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
i.IconDarkURL = asset.AssetURL
|
|
i.State = int32(domain.LabelPolicyStatePreview)
|
|
case instance.LabelPolicyIconDarkRemovedEventType,
|
|
org.LabelPolicyIconDarkRemovedEventType:
|
|
i.IconDarkURL = ""
|
|
i.State = int32(domain.LabelPolicyStatePreview)
|
|
case instance.LabelPolicyFontAddedEventType,
|
|
org.LabelPolicyFontAddedEventType:
|
|
err = asset.SetData(event)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
i.FontURL = asset.AssetURL
|
|
i.State = int32(domain.LabelPolicyStatePreview)
|
|
case instance.LabelPolicyFontRemovedEventType,
|
|
org.LabelPolicyFontRemovedEventType:
|
|
i.FontURL = ""
|
|
i.State = int32(domain.LabelPolicyStatePreview)
|
|
case instance.LabelPolicyActivatedEventType,
|
|
org.LabelPolicyActivatedEventType:
|
|
i.State = int32(domain.LabelPolicyStateActive)
|
|
case instance.LabelPolicyAssetsRemovedEventType,
|
|
org.LabelPolicyAssetsRemovedEventType:
|
|
i.LogoURL = ""
|
|
i.IconURL = ""
|
|
i.LogoDarkURL = ""
|
|
i.IconDarkURL = ""
|
|
i.FontURL = ""
|
|
i.State = int32(domain.LabelPolicyStatePreview)
|
|
}
|
|
return err
|
|
}
|
|
|
|
func (r *LabelPolicyView) setRootData(event *models.Event) {
|
|
r.AggregateID = event.AggregateID
|
|
r.InstanceID = event.InstanceID
|
|
}
|
|
|
|
func (r *LabelPolicyView) SetData(event *models.Event) error {
|
|
if err := json.Unmarshal(event.Data, r); err != nil {
|
|
logging.Log("MODEL-Flp9C").WithError(err).Error("could not unmarshal event data")
|
|
return caos_errs.ThrowInternal(err, "MODEL-Hs8uf", "Could not unmarshal data")
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (r *AssetView) SetData(event *models.Event) error {
|
|
if err := json.Unmarshal(event.Data, r); err != nil {
|
|
logging.Log("MODEL-Ms8f2").WithError(err).Error("could not unmarshal event data")
|
|
return caos_errs.ThrowInternal(err, "MODEL-Hs8uf", "Could not unmarshal data")
|
|
}
|
|
return nil
|
|
}
|