mirror of
https://github.com/zitadel/zitadel.git
synced 2025-04-06 13:15:45 +00:00
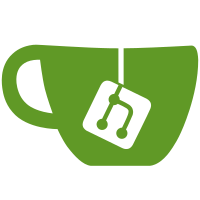
* feat: default custom message text * feat: org custom message text * feat: org custom message text * feat: custom messages query side * feat: default messages * feat: message text user fields * feat: check for inactive user * feat: fix send password reset * feat: fix custom org text * feat: add variables to docs * feat: custom text tests * feat: fix notifications * feat: add custom text feature * feat: add custom text feature * feat: feature in custom message texts * feat: add custom text feature in frontend * feat: merge main * feat: feature tests * feat: change phone message in setup * fix: remove unused code, add event translation * fix: merge main and fix problems * fix: english translation file * fix: migration versions * fix: setup * fix: custom login text * feat: add all possible custom texts for login * feat: iam login texts * feat: org login texts * feat: protos * fix: custom text in admin api * fix: add success login text * fix: docs * fix: add custom login texts to management api * fix: add sub messages to custom login texts * fix: setup custom texts * feat: get org login texts * feat: get org login texts * feat: handler in adminapi * feat: handlers in auth and admin * feat: render login texts * feat: custom login text * feat: add all login text keys * feat: handle correct login texts * feat: custom login texts in command side * feat: custom login texts in command side * feat: fix yaml file * feat: merge master and add confirmation text * feat: fix html * feat: read default login texts * feat: get default text files * feat: get custom texts org * feat: tests * feat: change translator handling * fix translator from authReq * feat: change h1 on login screens * feat: add custom login text for remove * feat: add custom login text for remove * feat: cache translation files * feat: cache translation files * feat: zitadel user in env var * feat: add registration user description * feat: better func naming * feat: tests * feat: add mutex to read file * feat: add mutex to read file * fix mutex for accessing translation map * fix: translation key Co-authored-by: Livio Amstutz <livio.a@gmail.com>
137 lines
4.2 KiB
Go
137 lines
4.2 KiB
Go
package handler
|
|
|
|
import (
|
|
"github.com/caos/zitadel/internal/domain"
|
|
"net/http"
|
|
|
|
http_mw "github.com/caos/zitadel/internal/api/http/middleware"
|
|
"github.com/caos/zitadel/internal/errors"
|
|
)
|
|
|
|
const (
|
|
queryInitPWCode = "code"
|
|
queryInitPWUserID = "userID"
|
|
|
|
tmplInitPassword = "initpassword"
|
|
tmplInitPasswordDone = "initpassworddone"
|
|
)
|
|
|
|
type initPasswordFormData struct {
|
|
Code string `schema:"code"`
|
|
Password string `schema:"password"`
|
|
PasswordConfirm string `schema:"passwordconfirm"`
|
|
UserID string `schema:"userID"`
|
|
Resend bool `schema:"resend"`
|
|
}
|
|
|
|
type initPasswordData struct {
|
|
baseData
|
|
profileData
|
|
Code string
|
|
UserID string
|
|
PasswordPolicyDescription string
|
|
MinLength uint64
|
|
HasUppercase string
|
|
HasLowercase string
|
|
HasNumber string
|
|
HasSymbol string
|
|
}
|
|
|
|
func (l *Login) handleInitPassword(w http.ResponseWriter, r *http.Request) {
|
|
userID := r.FormValue(queryInitPWUserID)
|
|
code := r.FormValue(queryInitPWCode)
|
|
l.renderInitPassword(w, r, nil, userID, code, nil)
|
|
}
|
|
|
|
func (l *Login) handleInitPasswordCheck(w http.ResponseWriter, r *http.Request) {
|
|
data := new(initPasswordFormData)
|
|
authReq, err := l.getAuthRequestAndParseData(r, data)
|
|
if err != nil {
|
|
l.renderError(w, r, authReq, err)
|
|
return
|
|
}
|
|
|
|
if data.Resend {
|
|
l.resendPasswordSet(w, r, authReq)
|
|
return
|
|
}
|
|
l.checkPWCode(w, r, authReq, data, nil)
|
|
}
|
|
|
|
func (l *Login) checkPWCode(w http.ResponseWriter, r *http.Request, authReq *domain.AuthRequest, data *initPasswordFormData, err error) {
|
|
if data.Password != data.PasswordConfirm {
|
|
err := errors.ThrowInvalidArgument(nil, "VIEW-KaGue", "Errors.User.Password.ConfirmationWrong")
|
|
l.renderInitPassword(w, r, authReq, data.UserID, data.Code, err)
|
|
return
|
|
}
|
|
userOrg := ""
|
|
if authReq != nil {
|
|
userOrg = authReq.UserOrgID
|
|
}
|
|
userAgentID, _ := http_mw.UserAgentIDFromCtx(r.Context())
|
|
err = l.command.SetPasswordWithVerifyCode(setContext(r.Context(), userOrg), userOrg, data.UserID, data.Code, data.Password, userAgentID)
|
|
if err != nil {
|
|
l.renderInitPassword(w, r, authReq, data.UserID, "", err)
|
|
return
|
|
}
|
|
l.renderInitPasswordDone(w, r, authReq)
|
|
}
|
|
|
|
func (l *Login) resendPasswordSet(w http.ResponseWriter, r *http.Request, authReq *domain.AuthRequest) {
|
|
if authReq == nil {
|
|
l.renderError(w, r, nil, errors.ThrowInternal(nil, "LOGIN-8sn7s", "Errors.AuthRequest.NotFound"))
|
|
return
|
|
}
|
|
userOrg := login
|
|
if authReq != nil {
|
|
userOrg = authReq.UserOrgID
|
|
}
|
|
user, err := l.authRepo.UserByLoginName(setContext(r.Context(), userOrg), authReq.LoginName)
|
|
if err != nil {
|
|
l.renderInitPassword(w, r, authReq, authReq.UserID, "", err)
|
|
return
|
|
}
|
|
_, err = l.command.RequestSetPassword(setContext(r.Context(), userOrg), user.ID, user.ResourceOwner, domain.NotificationTypeEmail)
|
|
l.renderInitPassword(w, r, authReq, authReq.UserID, "", err)
|
|
}
|
|
|
|
func (l *Login) renderInitPassword(w http.ResponseWriter, r *http.Request, authReq *domain.AuthRequest, userID, code string, err error) {
|
|
var errID, errMessage string
|
|
if err != nil {
|
|
errID, errMessage = l.getErrorMessage(r, err)
|
|
}
|
|
if userID == "" && authReq != nil {
|
|
userID = authReq.UserID
|
|
}
|
|
data := initPasswordData{
|
|
baseData: l.getBaseData(r, authReq, "Init Password", errID, errMessage),
|
|
profileData: l.getProfileData(authReq),
|
|
UserID: userID,
|
|
Code: code,
|
|
}
|
|
policy, description, _ := l.getPasswordComplexityPolicyByUserID(r, authReq, userID)
|
|
if policy != nil {
|
|
data.PasswordPolicyDescription = description
|
|
data.MinLength = policy.MinLength
|
|
if policy.HasUppercase {
|
|
data.HasUppercase = UpperCaseRegex
|
|
}
|
|
if policy.HasLowercase {
|
|
data.HasLowercase = LowerCaseRegex
|
|
}
|
|
if policy.HasSymbol {
|
|
data.HasSymbol = SymbolRegex
|
|
}
|
|
if policy.HasNumber {
|
|
data.HasNumber = NumberRegex
|
|
}
|
|
}
|
|
translator := l.getTranslator(authReq)
|
|
l.renderer.RenderTemplate(w, r, translator, l.renderer.Templates[tmplInitPassword], data, nil)
|
|
}
|
|
|
|
func (l *Login) renderInitPasswordDone(w http.ResponseWriter, r *http.Request, authReq *domain.AuthRequest) {
|
|
data := l.getUserData(r, authReq, "Password Init Done", "", "")
|
|
l.renderer.RenderTemplate(w, r, l.getTranslator(authReq), l.renderer.Templates[tmplInitPasswordDone], data, nil)
|
|
}
|