mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 19:14:23 +00:00
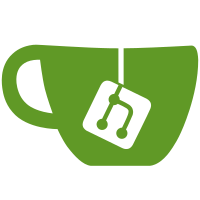
Add functionality to configure the access token type on the service accounts to provide the oidc library with the necessary information to create the right type of access token.
34 lines
1021 B
Go
34 lines
1021 B
Go
package oidc
|
|
|
|
import (
|
|
"context"
|
|
|
|
"github.com/zitadel/oidc/v2/pkg/oidc"
|
|
"github.com/zitadel/oidc/v2/pkg/op"
|
|
|
|
"github.com/zitadel/zitadel/internal/domain"
|
|
"github.com/zitadel/zitadel/internal/errors"
|
|
)
|
|
|
|
func (o *OPStorage) JWTProfileTokenType(ctx context.Context, request op.TokenRequest) (op.AccessTokenType, error) {
|
|
mapJWTProfileScopesToAudience(ctx, request)
|
|
user, err := o.query.GetUserByID(ctx, false, request.GetSubject(), false)
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
// the user should always be a machine, but let's just be sure
|
|
if user.Machine == nil {
|
|
return 0, errors.ThrowInvalidArgument(nil, "OIDC-jk26S", "invalid client type")
|
|
}
|
|
return accessTokenTypeToOIDC(user.Machine.AccessTokenType), nil
|
|
}
|
|
|
|
func mapJWTProfileScopesToAudience(ctx context.Context, request op.TokenRequest) {
|
|
// the request should always be a JWTTokenRequest, but let's make sure
|
|
jwt, ok := request.(*oidc.JWTTokenRequest)
|
|
if !ok {
|
|
return
|
|
}
|
|
jwt.Audience = domain.AddAudScopeToAudience(ctx, jwt.Audience, jwt.Scopes)
|
|
}
|