mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
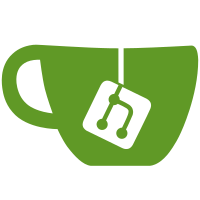
* at least registration prompt works * in memory test for login * buttons to start webauthn process * begin eventstore impl * begin eventstore impl * serialize into bytes * fix: u2f, passwordless types * fix for localhost * fix script * fix: u2f, passwordless types * fix: add u2f * fix: verify u2f * fix: session data in event store * fix: u2f credentials in eventstore * fix: webauthn pkg handles business models * feat: tests * feat: append events * fix: test * fix: check only ready webauthn creds * fix: move u2f methods to authrepo * frontend improvements * fix return * feat: add passwordless * feat: add passwordless * improve ui / error handling * separate call for login * fix login * js * feat: u2f login methods * feat: remove unused session id * feat: error handling * feat: error handling * feat: refactor user eventstore * feat: finish webauthn * feat: u2f and passwordlss in auth.proto * u2f step * passwordless step * cleanup js * EndpointPasswordLessLogin * migration * update mfaChecked test * next step test * token name * cleanup * attribute * passwordless as tokens * remove sms as otp type * add "user" to amr for webauthn * error handling * fixes * fix tests * naming * naming * fixes * session handler * i18n * error handling in login * Update internal/ui/login/static/i18n/de.yaml Co-authored-by: Fabi <38692350+fgerschwiler@users.noreply.github.com> * Update internal/ui/login/static/i18n/en.yaml Co-authored-by: Fabi <38692350+fgerschwiler@users.noreply.github.com> * improvements * merge fixes * fixes * fixes Co-authored-by: Fabiennne <fabienne.gerschwiler@gmail.com> Co-authored-by: Fabi <38692350+fgerschwiler@users.noreply.github.com>
264 lines
5.2 KiB
Go
264 lines
5.2 KiB
Go
package model
|
|
|
|
import (
|
|
"net"
|
|
"reflect"
|
|
"testing"
|
|
)
|
|
|
|
func TestAuthRequest_IsValid(t *testing.T) {
|
|
type fields struct {
|
|
ID string
|
|
AgentID string
|
|
BrowserInfo *BrowserInfo
|
|
ApplicationID string
|
|
CallbackURI string
|
|
Request Request
|
|
}
|
|
tests := []struct {
|
|
name string
|
|
fields fields
|
|
want bool
|
|
}{
|
|
{
|
|
"missing id, false",
|
|
fields{},
|
|
false,
|
|
},
|
|
{
|
|
"missing agent id, false",
|
|
fields{
|
|
ID: "id",
|
|
},
|
|
false,
|
|
},
|
|
{
|
|
"missing browser info, false",
|
|
fields{
|
|
ID: "id",
|
|
AgentID: "agentID",
|
|
},
|
|
false,
|
|
},
|
|
{
|
|
"browser info invalid, false",
|
|
fields{
|
|
ID: "id",
|
|
AgentID: "agentID",
|
|
BrowserInfo: &BrowserInfo{},
|
|
},
|
|
false,
|
|
},
|
|
{
|
|
"missing application id, false",
|
|
fields{
|
|
ID: "id",
|
|
AgentID: "agentID",
|
|
BrowserInfo: &BrowserInfo{
|
|
UserAgent: "user agent",
|
|
AcceptLanguage: "accept language",
|
|
RemoteIP: net.IPv4(29, 4, 20, 19),
|
|
},
|
|
},
|
|
false,
|
|
},
|
|
{
|
|
"missing callback uri, false",
|
|
fields{
|
|
ID: "id",
|
|
AgentID: "agentID",
|
|
BrowserInfo: &BrowserInfo{
|
|
UserAgent: "user agent",
|
|
AcceptLanguage: "accept language",
|
|
RemoteIP: net.IPv4(29, 4, 20, 19),
|
|
},
|
|
ApplicationID: "appID",
|
|
},
|
|
false,
|
|
},
|
|
{
|
|
"missing request, false",
|
|
fields{
|
|
ID: "id",
|
|
AgentID: "agentID",
|
|
BrowserInfo: &BrowserInfo{
|
|
UserAgent: "user agent",
|
|
AcceptLanguage: "accept language",
|
|
RemoteIP: net.IPv4(29, 4, 20, 19),
|
|
},
|
|
ApplicationID: "appID",
|
|
CallbackURI: "schema://callback",
|
|
},
|
|
false,
|
|
},
|
|
{
|
|
"request invalid, false",
|
|
fields{
|
|
ID: "id",
|
|
AgentID: "agentID",
|
|
BrowserInfo: &BrowserInfo{
|
|
UserAgent: "user agent",
|
|
AcceptLanguage: "accept language",
|
|
RemoteIP: net.IPv4(29, 4, 20, 19),
|
|
},
|
|
ApplicationID: "appID",
|
|
CallbackURI: "schema://callback",
|
|
Request: &AuthRequestOIDC{},
|
|
},
|
|
false,
|
|
},
|
|
{
|
|
"valid auth request, true",
|
|
fields{
|
|
ID: "id",
|
|
AgentID: "agentID",
|
|
BrowserInfo: &BrowserInfo{
|
|
UserAgent: "user agent",
|
|
AcceptLanguage: "accept language",
|
|
RemoteIP: net.IPv4(29, 4, 20, 19),
|
|
},
|
|
ApplicationID: "appID",
|
|
CallbackURI: "schema://callback",
|
|
Request: &AuthRequestOIDC{
|
|
Scopes: []string{"openid"},
|
|
CodeChallenge: &OIDCCodeChallenge{
|
|
Challenge: "challenge",
|
|
Method: CodeChallengeMethodS256,
|
|
},
|
|
},
|
|
},
|
|
true,
|
|
},
|
|
}
|
|
for _, tt := range tests {
|
|
t.Run(tt.name, func(t *testing.T) {
|
|
a := &AuthRequest{
|
|
ID: tt.fields.ID,
|
|
AgentID: tt.fields.AgentID,
|
|
BrowserInfo: tt.fields.BrowserInfo,
|
|
ApplicationID: tt.fields.ApplicationID,
|
|
CallbackURI: tt.fields.CallbackURI,
|
|
Request: tt.fields.Request,
|
|
}
|
|
if got := a.IsValid(); got != tt.want {
|
|
t.Errorf("IsValid() = %v, want %v", got, tt.want)
|
|
}
|
|
})
|
|
}
|
|
}
|
|
|
|
func TestAuthRequest_MFALevel(t *testing.T) {
|
|
type fields struct {
|
|
Prompt Prompt
|
|
PossibleLOAs []LevelOfAssurance
|
|
}
|
|
tests := []struct {
|
|
name string
|
|
fields fields
|
|
want MFALevel
|
|
}{
|
|
//PLANNED: Add / replace test cases when LOA is set
|
|
{"-1",
|
|
fields{},
|
|
-1,
|
|
},
|
|
}
|
|
for _, tt := range tests {
|
|
t.Run(tt.name, func(t *testing.T) {
|
|
a := &AuthRequest{
|
|
Prompt: tt.fields.Prompt,
|
|
PossibleLOAs: tt.fields.PossibleLOAs,
|
|
}
|
|
if got := a.MFALevel(); got != tt.want {
|
|
t.Errorf("MFALevel() = %v, want %v", got, tt.want)
|
|
}
|
|
})
|
|
}
|
|
}
|
|
|
|
func TestAuthRequest_WithCurrentInfo(t *testing.T) {
|
|
type fields struct {
|
|
ID string
|
|
AgentID string
|
|
BrowserInfo *BrowserInfo
|
|
}
|
|
type args struct {
|
|
info *BrowserInfo
|
|
}
|
|
tests := []struct {
|
|
name string
|
|
fields fields
|
|
args args
|
|
want *AuthRequest
|
|
}{
|
|
{
|
|
"unchanged",
|
|
fields{
|
|
ID: "id",
|
|
AgentID: "agentID",
|
|
BrowserInfo: &BrowserInfo{
|
|
UserAgent: "ua",
|
|
AcceptLanguage: "de",
|
|
RemoteIP: net.IPv4(29, 4, 20, 19),
|
|
},
|
|
},
|
|
args{
|
|
&BrowserInfo{
|
|
UserAgent: "ua",
|
|
AcceptLanguage: "de",
|
|
RemoteIP: net.IPv4(29, 4, 20, 19),
|
|
},
|
|
},
|
|
&AuthRequest{
|
|
ID: "id",
|
|
AgentID: "agentID",
|
|
BrowserInfo: &BrowserInfo{
|
|
UserAgent: "ua",
|
|
AcceptLanguage: "de",
|
|
RemoteIP: net.IPv4(29, 4, 20, 19),
|
|
},
|
|
},
|
|
},
|
|
{
|
|
"changed",
|
|
fields{
|
|
ID: "id",
|
|
AgentID: "agentID",
|
|
BrowserInfo: &BrowserInfo{
|
|
UserAgent: "ua",
|
|
AcceptLanguage: "de",
|
|
RemoteIP: net.IPv4(29, 4, 20, 19),
|
|
},
|
|
},
|
|
args{
|
|
&BrowserInfo{
|
|
UserAgent: "ua",
|
|
AcceptLanguage: "de",
|
|
RemoteIP: net.IPv4(16, 12, 20, 19),
|
|
},
|
|
},
|
|
&AuthRequest{
|
|
ID: "id",
|
|
AgentID: "agentID",
|
|
BrowserInfo: &BrowserInfo{
|
|
UserAgent: "ua",
|
|
AcceptLanguage: "de",
|
|
RemoteIP: net.IPv4(16, 12, 20, 19),
|
|
},
|
|
},
|
|
},
|
|
}
|
|
for _, tt := range tests {
|
|
t.Run(tt.name, func(t *testing.T) {
|
|
a := &AuthRequest{
|
|
ID: tt.fields.ID,
|
|
AgentID: tt.fields.AgentID,
|
|
BrowserInfo: tt.fields.BrowserInfo,
|
|
}
|
|
if got := a.WithCurrentInfo(tt.args.info); !reflect.DeepEqual(got, tt.want) {
|
|
t.Errorf("WithCurrentInfo() = %v, want %v", got, tt.want)
|
|
}
|
|
})
|
|
}
|
|
}
|