mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
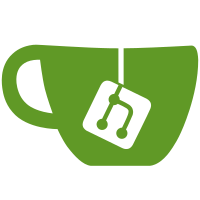
* fix: move eventstore pkgs * fix: move eventstore pkgs * fix: remove v2 view * fix: remove v2 view
58 lines
1.7 KiB
Go
58 lines
1.7 KiB
Go
package model
|
|
|
|
import (
|
|
caos_errs "github.com/caos/zitadel/internal/errors"
|
|
"github.com/caos/zitadel/internal/eventstore/v1/models"
|
|
"regexp"
|
|
)
|
|
|
|
var (
|
|
hasStringLowerCase = regexp.MustCompile(`[a-z]`).MatchString
|
|
hasStringUpperCase = regexp.MustCompile(`[A-Z]`).MatchString
|
|
hasNumber = regexp.MustCompile(`[0-9]`).MatchString
|
|
hasSymbol = regexp.MustCompile(`[^A-Za-z0-9]`).MatchString
|
|
)
|
|
|
|
type PasswordComplexityPolicy struct {
|
|
models.ObjectRoot
|
|
|
|
State PolicyState
|
|
MinLength uint64
|
|
HasLowercase bool
|
|
HasUppercase bool
|
|
HasNumber bool
|
|
HasSymbol bool
|
|
|
|
Default bool
|
|
}
|
|
|
|
func (p *PasswordComplexityPolicy) IsValid() error {
|
|
if p.MinLength == 0 || p.MinLength > 72 {
|
|
return caos_errs.ThrowInvalidArgument(nil, "MODEL-Lsp0e", "Errors.User.PasswordComplexityPolicy.MinLengthNotAllowed")
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (p *PasswordComplexityPolicy) Check(password string) error {
|
|
if p.MinLength != 0 && uint64(len(password)) < p.MinLength {
|
|
return caos_errs.ThrowInvalidArgument(nil, "MODEL-HuJf6", "Errors.User.PasswordComplexityPolicy.MinLength")
|
|
}
|
|
|
|
if p.HasLowercase && !hasStringLowerCase(password) {
|
|
return caos_errs.ThrowInvalidArgument(nil, "MODEL-co3Xw", "Errors.User.PasswordComplexityPolicy.HasLower")
|
|
}
|
|
|
|
if p.HasUppercase && !hasStringUpperCase(password) {
|
|
return caos_errs.ThrowInvalidArgument(nil, "MODEL-VoaRj", "Errors.User.PasswordComplexityPolicy.HasUpper")
|
|
}
|
|
|
|
if p.HasNumber && !hasNumber(password) {
|
|
return caos_errs.ThrowInvalidArgument(nil, "MODEL-ZBv4H", "Errors.User.PasswordComplexityPolicy.HasNumber")
|
|
}
|
|
|
|
if p.HasSymbol && !hasSymbol(password) {
|
|
return caos_errs.ThrowInvalidArgument(nil, "MODEL-ZDLwA", "Errors.User.PasswordComplexityPolicy.HasSymbol")
|
|
}
|
|
return nil
|
|
}
|