mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-15 04:18:01 +00:00
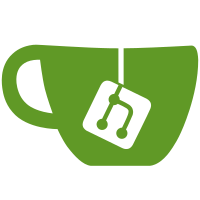
* fix(zitadelctl): implement takedown command * fix(zitadelctl): correct destroy flow * fix(zitadelctl): correct backup commands to read crds beforehand * fix: add of destroyfile * fix: clean for userlist * fix: change backup and restore to crdb native * fix: timeout for delete pvc for cockroachdb * fix: corrected unit tests * fix: add ignored file for scale * fix: correct handling of gitops in backup command * feat: add s3 backup kind * fix: backuplist for s3 and timeout for pv deletion * fix(database): fix nil pointer with binary version * fix(database): cleanup of errors which cam with merging of the s3 logic * fix: correct unit tests * fix: cleanup monitor output Co-authored-by: Elio Bischof <eliobischof@gmail.com> * fix: backup imagepullpolixy to ifnotpresent Co-authored-by: Elio Bischof <eliobischof@gmail.com>
66 lines
2.2 KiB
Go
66 lines
2.2 KiB
Go
package s3
|
|
|
|
import (
|
|
"fmt"
|
|
|
|
"github.com/caos/orbos/pkg/secret"
|
|
"github.com/caos/orbos/pkg/tree"
|
|
)
|
|
|
|
type DesiredV0 struct {
|
|
Common *tree.Common `yaml:",inline"`
|
|
Spec *Spec
|
|
}
|
|
|
|
type Spec struct {
|
|
Verbose bool
|
|
Cron string `yaml:"cron,omitempty"`
|
|
Bucket string `yaml:"bucket,omitempty"`
|
|
Endpoint string `yaml:"endpoint,omitempty"`
|
|
Region string `yaml:"region,omitempty"`
|
|
AccessKeyID *secret.Secret `yaml:"accessKeyID,omitempty"`
|
|
ExistingAccessKeyID *secret.Existing `yaml:"existingAccessKeyID,omitempty"`
|
|
SecretAccessKey *secret.Secret `yaml:"secretAccessKey,omitempty"`
|
|
ExistingSecretAccessKey *secret.Existing `yaml:"existingSecretAccessKey,omitempty"`
|
|
SessionToken *secret.Secret `yaml:"sessionToken,omitempty"`
|
|
ExistingSessionToken *secret.Existing `yaml:"existingSessionToken,omitempty"`
|
|
}
|
|
|
|
func (s *Spec) IsZero() bool {
|
|
if ((s.AccessKeyID == nil || s.AccessKeyID.IsZero()) && (s.ExistingAccessKeyID == nil || s.ExistingAccessKeyID.IsZero())) &&
|
|
((s.SecretAccessKey == nil || s.SecretAccessKey.IsZero()) && (s.ExistingSecretAccessKey == nil || s.ExistingSecretAccessKey.IsZero())) &&
|
|
((s.SessionToken == nil || s.SessionToken.IsZero()) && (s.ExistingSessionToken == nil || s.ExistingSessionToken.IsZero())) &&
|
|
!s.Verbose &&
|
|
s.Bucket == "" &&
|
|
s.Cron == "" &&
|
|
s.Endpoint == "" &&
|
|
s.Region == "" {
|
|
return true
|
|
}
|
|
return false
|
|
}
|
|
|
|
func ParseDesiredV0(desiredTree *tree.Tree) (*DesiredV0, error) {
|
|
desiredKind := &DesiredV0{
|
|
Common: desiredTree.Common,
|
|
Spec: &Spec{},
|
|
}
|
|
|
|
if err := desiredTree.Original.Decode(desiredKind); err != nil {
|
|
return nil, fmt.Errorf("parsing desired state failed: %s", err)
|
|
}
|
|
|
|
return desiredKind, nil
|
|
}
|
|
|
|
func (d *DesiredV0) validateSecrets() error {
|
|
if err := secret.ValidateSecret(d.Spec.AccessKeyID, d.Spec.ExistingAccessKeyID); err != nil {
|
|
return fmt.Errorf("validating access key id failed: %w", err)
|
|
}
|
|
if err := secret.ValidateSecret(d.Spec.SecretAccessKey, d.Spec.ExistingSecretAccessKey); err != nil {
|
|
return fmt.Errorf("validating secret access key failed: %w", err)
|
|
}
|
|
|
|
return nil
|
|
}
|