mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
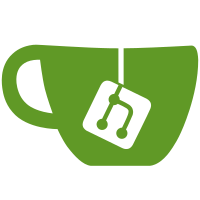
* Label Policy added * save * chore: update docs action * Save * Save * Get colors from DB * Variables inserted * Get images from global directory. * Add tests * Add tests * Corrections from mergerequest * Corrections from mergerequest * Test corrected. * Added colors to all notifications. * Added colors to Corrected text and formatting.all notifications. * Spelling error corrected. * fix: tests * Merge Branch corrected. * Step6 added * Corrections from mergerequest * fix: generate management * Formatted texts. * fix: migrations Co-authored-by: Florian Forster <florian@caos.ch> Co-authored-by: adlerhurst <silvan.reusser@gmail.com> Co-authored-by: Fabiennne <fabienne.gerschwiler@gmail.com>
4910 lines
183 KiB
Go
4910 lines
183 KiB
Go
// Code generated by protoc-gen-go. DO NOT EDIT.
|
|
// source: auth.proto
|
|
|
|
package auth
|
|
|
|
import (
|
|
context "context"
|
|
fmt "fmt"
|
|
_ "github.com/caos/zitadel/internal/protoc/protoc-gen-authoption/authoption"
|
|
message "github.com/caos/zitadel/pkg/grpc/message"
|
|
_ "github.com/envoyproxy/protoc-gen-validate/validate"
|
|
proto "github.com/golang/protobuf/proto"
|
|
empty "github.com/golang/protobuf/ptypes/empty"
|
|
_struct "github.com/golang/protobuf/ptypes/struct"
|
|
timestamp "github.com/golang/protobuf/ptypes/timestamp"
|
|
_ "github.com/grpc-ecosystem/grpc-gateway/protoc-gen-swagger/options"
|
|
_ "google.golang.org/genproto/googleapis/api/annotations"
|
|
grpc "google.golang.org/grpc"
|
|
codes "google.golang.org/grpc/codes"
|
|
status "google.golang.org/grpc/status"
|
|
math "math"
|
|
)
|
|
|
|
// Reference imports to suppress errors if they are not otherwise used.
|
|
var _ = proto.Marshal
|
|
var _ = fmt.Errorf
|
|
var _ = math.Inf
|
|
|
|
// This is a compile-time assertion to ensure that this generated file
|
|
// is compatible with the proto package it is being compiled against.
|
|
// A compilation error at this line likely means your copy of the
|
|
// proto package needs to be updated.
|
|
const _ = proto.ProtoPackageIsVersion3 // please upgrade the proto package
|
|
|
|
type UserSessionState int32
|
|
|
|
const (
|
|
UserSessionState_USERSESSIONSTATE_UNSPECIFIED UserSessionState = 0
|
|
UserSessionState_USERSESSIONSTATE_ACTIVE UserSessionState = 1
|
|
UserSessionState_USERSESSIONSTATE_TERMINATED UserSessionState = 2
|
|
)
|
|
|
|
var UserSessionState_name = map[int32]string{
|
|
0: "USERSESSIONSTATE_UNSPECIFIED",
|
|
1: "USERSESSIONSTATE_ACTIVE",
|
|
2: "USERSESSIONSTATE_TERMINATED",
|
|
}
|
|
|
|
var UserSessionState_value = map[string]int32{
|
|
"USERSESSIONSTATE_UNSPECIFIED": 0,
|
|
"USERSESSIONSTATE_ACTIVE": 1,
|
|
"USERSESSIONSTATE_TERMINATED": 2,
|
|
}
|
|
|
|
func (x UserSessionState) String() string {
|
|
return proto.EnumName(UserSessionState_name, int32(x))
|
|
}
|
|
|
|
func (UserSessionState) EnumDescriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{0}
|
|
}
|
|
|
|
type MachineKeyType int32
|
|
|
|
const (
|
|
MachineKeyType_MACHINEKEY_UNSPECIFIED MachineKeyType = 0
|
|
MachineKeyType_MACHINEKEY_JSON MachineKeyType = 1
|
|
)
|
|
|
|
var MachineKeyType_name = map[int32]string{
|
|
0: "MACHINEKEY_UNSPECIFIED",
|
|
1: "MACHINEKEY_JSON",
|
|
}
|
|
|
|
var MachineKeyType_value = map[string]int32{
|
|
"MACHINEKEY_UNSPECIFIED": 0,
|
|
"MACHINEKEY_JSON": 1,
|
|
}
|
|
|
|
func (x MachineKeyType) String() string {
|
|
return proto.EnumName(MachineKeyType_name, int32(x))
|
|
}
|
|
|
|
func (MachineKeyType) EnumDescriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{1}
|
|
}
|
|
|
|
type UserState int32
|
|
|
|
const (
|
|
UserState_USERSTATE_UNSPECIFIED UserState = 0
|
|
UserState_USERSTATE_ACTIVE UserState = 1
|
|
UserState_USERSTATE_INACTIVE UserState = 2
|
|
UserState_USERSTATE_DELETED UserState = 3
|
|
UserState_USERSTATE_LOCKED UserState = 4
|
|
UserState_USERSTATE_SUSPEND UserState = 5
|
|
UserState_USERSTATE_INITIAL UserState = 6
|
|
)
|
|
|
|
var UserState_name = map[int32]string{
|
|
0: "USERSTATE_UNSPECIFIED",
|
|
1: "USERSTATE_ACTIVE",
|
|
2: "USERSTATE_INACTIVE",
|
|
3: "USERSTATE_DELETED",
|
|
4: "USERSTATE_LOCKED",
|
|
5: "USERSTATE_SUSPEND",
|
|
6: "USERSTATE_INITIAL",
|
|
}
|
|
|
|
var UserState_value = map[string]int32{
|
|
"USERSTATE_UNSPECIFIED": 0,
|
|
"USERSTATE_ACTIVE": 1,
|
|
"USERSTATE_INACTIVE": 2,
|
|
"USERSTATE_DELETED": 3,
|
|
"USERSTATE_LOCKED": 4,
|
|
"USERSTATE_SUSPEND": 5,
|
|
"USERSTATE_INITIAL": 6,
|
|
}
|
|
|
|
func (x UserState) String() string {
|
|
return proto.EnumName(UserState_name, int32(x))
|
|
}
|
|
|
|
func (UserState) EnumDescriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{2}
|
|
}
|
|
|
|
type Gender int32
|
|
|
|
const (
|
|
Gender_GENDER_UNSPECIFIED Gender = 0
|
|
Gender_GENDER_FEMALE Gender = 1
|
|
Gender_GENDER_MALE Gender = 2
|
|
Gender_GENDER_DIVERSE Gender = 3
|
|
)
|
|
|
|
var Gender_name = map[int32]string{
|
|
0: "GENDER_UNSPECIFIED",
|
|
1: "GENDER_FEMALE",
|
|
2: "GENDER_MALE",
|
|
3: "GENDER_DIVERSE",
|
|
}
|
|
|
|
var Gender_value = map[string]int32{
|
|
"GENDER_UNSPECIFIED": 0,
|
|
"GENDER_FEMALE": 1,
|
|
"GENDER_MALE": 2,
|
|
"GENDER_DIVERSE": 3,
|
|
}
|
|
|
|
func (x Gender) String() string {
|
|
return proto.EnumName(Gender_name, int32(x))
|
|
}
|
|
|
|
func (Gender) EnumDescriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{3}
|
|
}
|
|
|
|
type MfaType int32
|
|
|
|
const (
|
|
MfaType_MFATYPE_UNSPECIFIED MfaType = 0
|
|
MfaType_MFATYPE_SMS MfaType = 1
|
|
MfaType_MFATYPE_OTP MfaType = 2
|
|
)
|
|
|
|
var MfaType_name = map[int32]string{
|
|
0: "MFATYPE_UNSPECIFIED",
|
|
1: "MFATYPE_SMS",
|
|
2: "MFATYPE_OTP",
|
|
}
|
|
|
|
var MfaType_value = map[string]int32{
|
|
"MFATYPE_UNSPECIFIED": 0,
|
|
"MFATYPE_SMS": 1,
|
|
"MFATYPE_OTP": 2,
|
|
}
|
|
|
|
func (x MfaType) String() string {
|
|
return proto.EnumName(MfaType_name, int32(x))
|
|
}
|
|
|
|
func (MfaType) EnumDescriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{4}
|
|
}
|
|
|
|
type MFAState int32
|
|
|
|
const (
|
|
MFAState_MFASTATE_UNSPECIFIED MFAState = 0
|
|
MFAState_MFASTATE_NOT_READY MFAState = 1
|
|
MFAState_MFASTATE_READY MFAState = 2
|
|
MFAState_MFASTATE_REMOVED MFAState = 3
|
|
)
|
|
|
|
var MFAState_name = map[int32]string{
|
|
0: "MFASTATE_UNSPECIFIED",
|
|
1: "MFASTATE_NOT_READY",
|
|
2: "MFASTATE_READY",
|
|
3: "MFASTATE_REMOVED",
|
|
}
|
|
|
|
var MFAState_value = map[string]int32{
|
|
"MFASTATE_UNSPECIFIED": 0,
|
|
"MFASTATE_NOT_READY": 1,
|
|
"MFASTATE_READY": 2,
|
|
"MFASTATE_REMOVED": 3,
|
|
}
|
|
|
|
func (x MFAState) String() string {
|
|
return proto.EnumName(MFAState_name, int32(x))
|
|
}
|
|
|
|
func (MFAState) EnumDescriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{5}
|
|
}
|
|
|
|
type UserGrantSearchKey int32
|
|
|
|
const (
|
|
UserGrantSearchKey_UserGrantSearchKey_UNKNOWN UserGrantSearchKey = 0
|
|
UserGrantSearchKey_UserGrantSearchKey_ORG_ID UserGrantSearchKey = 1
|
|
UserGrantSearchKey_UserGrantSearchKey_PROJECT_ID UserGrantSearchKey = 2
|
|
)
|
|
|
|
var UserGrantSearchKey_name = map[int32]string{
|
|
0: "UserGrantSearchKey_UNKNOWN",
|
|
1: "UserGrantSearchKey_ORG_ID",
|
|
2: "UserGrantSearchKey_PROJECT_ID",
|
|
}
|
|
|
|
var UserGrantSearchKey_value = map[string]int32{
|
|
"UserGrantSearchKey_UNKNOWN": 0,
|
|
"UserGrantSearchKey_ORG_ID": 1,
|
|
"UserGrantSearchKey_PROJECT_ID": 2,
|
|
}
|
|
|
|
func (x UserGrantSearchKey) String() string {
|
|
return proto.EnumName(UserGrantSearchKey_name, int32(x))
|
|
}
|
|
|
|
func (UserGrantSearchKey) EnumDescriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{6}
|
|
}
|
|
|
|
type MyProjectOrgSearchKey int32
|
|
|
|
const (
|
|
MyProjectOrgSearchKey_MYPROJECTORGSEARCHKEY_UNSPECIFIED MyProjectOrgSearchKey = 0
|
|
MyProjectOrgSearchKey_MYPROJECTORGSEARCHKEY_ORG_NAME MyProjectOrgSearchKey = 1
|
|
)
|
|
|
|
var MyProjectOrgSearchKey_name = map[int32]string{
|
|
0: "MYPROJECTORGSEARCHKEY_UNSPECIFIED",
|
|
1: "MYPROJECTORGSEARCHKEY_ORG_NAME",
|
|
}
|
|
|
|
var MyProjectOrgSearchKey_value = map[string]int32{
|
|
"MYPROJECTORGSEARCHKEY_UNSPECIFIED": 0,
|
|
"MYPROJECTORGSEARCHKEY_ORG_NAME": 1,
|
|
}
|
|
|
|
func (x MyProjectOrgSearchKey) String() string {
|
|
return proto.EnumName(MyProjectOrgSearchKey_name, int32(x))
|
|
}
|
|
|
|
func (MyProjectOrgSearchKey) EnumDescriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{7}
|
|
}
|
|
|
|
type SearchMethod int32
|
|
|
|
const (
|
|
SearchMethod_SEARCHMETHOD_EQUALS SearchMethod = 0
|
|
SearchMethod_SEARCHMETHOD_STARTS_WITH SearchMethod = 1
|
|
SearchMethod_SEARCHMETHOD_CONTAINS SearchMethod = 2
|
|
SearchMethod_SEARCHMETHOD_EQUALS_IGNORE_CASE SearchMethod = 3
|
|
SearchMethod_SEARCHMETHOD_STARTS_WITH_IGNORE_CASE SearchMethod = 4
|
|
SearchMethod_SEARCHMETHOD_CONTAINS_IGNORE_CASE SearchMethod = 5
|
|
)
|
|
|
|
var SearchMethod_name = map[int32]string{
|
|
0: "SEARCHMETHOD_EQUALS",
|
|
1: "SEARCHMETHOD_STARTS_WITH",
|
|
2: "SEARCHMETHOD_CONTAINS",
|
|
3: "SEARCHMETHOD_EQUALS_IGNORE_CASE",
|
|
4: "SEARCHMETHOD_STARTS_WITH_IGNORE_CASE",
|
|
5: "SEARCHMETHOD_CONTAINS_IGNORE_CASE",
|
|
}
|
|
|
|
var SearchMethod_value = map[string]int32{
|
|
"SEARCHMETHOD_EQUALS": 0,
|
|
"SEARCHMETHOD_STARTS_WITH": 1,
|
|
"SEARCHMETHOD_CONTAINS": 2,
|
|
"SEARCHMETHOD_EQUALS_IGNORE_CASE": 3,
|
|
"SEARCHMETHOD_STARTS_WITH_IGNORE_CASE": 4,
|
|
"SEARCHMETHOD_CONTAINS_IGNORE_CASE": 5,
|
|
}
|
|
|
|
func (x SearchMethod) String() string {
|
|
return proto.EnumName(SearchMethod_name, int32(x))
|
|
}
|
|
|
|
func (SearchMethod) EnumDescriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{8}
|
|
}
|
|
|
|
type UserSessionViews struct {
|
|
UserSessions []*UserSessionView `protobuf:"bytes,1,rep,name=user_sessions,json=userSessions,proto3" json:"user_sessions,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *UserSessionViews) Reset() { *m = UserSessionViews{} }
|
|
func (m *UserSessionViews) String() string { return proto.CompactTextString(m) }
|
|
func (*UserSessionViews) ProtoMessage() {}
|
|
func (*UserSessionViews) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{0}
|
|
}
|
|
|
|
func (m *UserSessionViews) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_UserSessionViews.Unmarshal(m, b)
|
|
}
|
|
func (m *UserSessionViews) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_UserSessionViews.Marshal(b, m, deterministic)
|
|
}
|
|
func (m *UserSessionViews) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_UserSessionViews.Merge(m, src)
|
|
}
|
|
func (m *UserSessionViews) XXX_Size() int {
|
|
return xxx_messageInfo_UserSessionViews.Size(m)
|
|
}
|
|
func (m *UserSessionViews) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_UserSessionViews.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_UserSessionViews proto.InternalMessageInfo
|
|
|
|
func (m *UserSessionViews) GetUserSessions() []*UserSessionView {
|
|
if m != nil {
|
|
return m.UserSessions
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type UserSessionView struct {
|
|
Id string `protobuf:"bytes,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
AgentId string `protobuf:"bytes,2,opt,name=agent_id,json=agentId,proto3" json:"agent_id,omitempty"`
|
|
AuthState UserSessionState `protobuf:"varint,3,opt,name=auth_state,json=authState,proto3,enum=caos.zitadel.auth.api.v1.UserSessionState" json:"auth_state,omitempty"`
|
|
UserId string `protobuf:"bytes,4,opt,name=user_id,json=userId,proto3" json:"user_id,omitempty"`
|
|
UserName string `protobuf:"bytes,5,opt,name=user_name,json=userName,proto3" json:"user_name,omitempty"`
|
|
Sequence uint64 `protobuf:"varint,6,opt,name=sequence,proto3" json:"sequence,omitempty"`
|
|
LoginName string `protobuf:"bytes,7,opt,name=login_name,json=loginName,proto3" json:"login_name,omitempty"`
|
|
DisplayName string `protobuf:"bytes,8,opt,name=display_name,json=displayName,proto3" json:"display_name,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *UserSessionView) Reset() { *m = UserSessionView{} }
|
|
func (m *UserSessionView) String() string { return proto.CompactTextString(m) }
|
|
func (*UserSessionView) ProtoMessage() {}
|
|
func (*UserSessionView) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{1}
|
|
}
|
|
|
|
func (m *UserSessionView) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_UserSessionView.Unmarshal(m, b)
|
|
}
|
|
func (m *UserSessionView) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_UserSessionView.Marshal(b, m, deterministic)
|
|
}
|
|
func (m *UserSessionView) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_UserSessionView.Merge(m, src)
|
|
}
|
|
func (m *UserSessionView) XXX_Size() int {
|
|
return xxx_messageInfo_UserSessionView.Size(m)
|
|
}
|
|
func (m *UserSessionView) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_UserSessionView.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_UserSessionView proto.InternalMessageInfo
|
|
|
|
func (m *UserSessionView) GetId() string {
|
|
if m != nil {
|
|
return m.Id
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UserSessionView) GetAgentId() string {
|
|
if m != nil {
|
|
return m.AgentId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UserSessionView) GetAuthState() UserSessionState {
|
|
if m != nil {
|
|
return m.AuthState
|
|
}
|
|
return UserSessionState_USERSESSIONSTATE_UNSPECIFIED
|
|
}
|
|
|
|
func (m *UserSessionView) GetUserId() string {
|
|
if m != nil {
|
|
return m.UserId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UserSessionView) GetUserName() string {
|
|
if m != nil {
|
|
return m.UserName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UserSessionView) GetSequence() uint64 {
|
|
if m != nil {
|
|
return m.Sequence
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (m *UserSessionView) GetLoginName() string {
|
|
if m != nil {
|
|
return m.LoginName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UserSessionView) GetDisplayName() string {
|
|
if m != nil {
|
|
return m.DisplayName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type UserView struct {
|
|
Id string `protobuf:"bytes,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
State UserState `protobuf:"varint,2,opt,name=state,proto3,enum=caos.zitadel.auth.api.v1.UserState" json:"state,omitempty"`
|
|
CreationDate *timestamp.Timestamp `protobuf:"bytes,3,opt,name=creation_date,json=creationDate,proto3" json:"creation_date,omitempty"`
|
|
ChangeDate *timestamp.Timestamp `protobuf:"bytes,4,opt,name=change_date,json=changeDate,proto3" json:"change_date,omitempty"`
|
|
Sequence uint64 `protobuf:"varint,5,opt,name=sequence,proto3" json:"sequence,omitempty"`
|
|
LoginNames []string `protobuf:"bytes,6,rep,name=login_names,json=loginNames,proto3" json:"login_names,omitempty"`
|
|
PreferredLoginName string `protobuf:"bytes,7,opt,name=preferred_login_name,json=preferredLoginName,proto3" json:"preferred_login_name,omitempty"`
|
|
LastLogin *timestamp.Timestamp `protobuf:"bytes,8,opt,name=last_login,json=lastLogin,proto3" json:"last_login,omitempty"`
|
|
ResourceOwner string `protobuf:"bytes,9,opt,name=resource_owner,json=resourceOwner,proto3" json:"resource_owner,omitempty"`
|
|
UserName string `protobuf:"bytes,10,opt,name=user_name,json=userName,proto3" json:"user_name,omitempty"`
|
|
// Types that are valid to be assigned to User:
|
|
// *UserView_Human
|
|
// *UserView_Machine
|
|
User isUserView_User `protobuf_oneof:"user"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *UserView) Reset() { *m = UserView{} }
|
|
func (m *UserView) String() string { return proto.CompactTextString(m) }
|
|
func (*UserView) ProtoMessage() {}
|
|
func (*UserView) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{2}
|
|
}
|
|
|
|
func (m *UserView) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_UserView.Unmarshal(m, b)
|
|
}
|
|
func (m *UserView) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_UserView.Marshal(b, m, deterministic)
|
|
}
|
|
func (m *UserView) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_UserView.Merge(m, src)
|
|
}
|
|
func (m *UserView) XXX_Size() int {
|
|
return xxx_messageInfo_UserView.Size(m)
|
|
}
|
|
func (m *UserView) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_UserView.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_UserView proto.InternalMessageInfo
|
|
|
|
func (m *UserView) GetId() string {
|
|
if m != nil {
|
|
return m.Id
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UserView) GetState() UserState {
|
|
if m != nil {
|
|
return m.State
|
|
}
|
|
return UserState_USERSTATE_UNSPECIFIED
|
|
}
|
|
|
|
func (m *UserView) GetCreationDate() *timestamp.Timestamp {
|
|
if m != nil {
|
|
return m.CreationDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (m *UserView) GetChangeDate() *timestamp.Timestamp {
|
|
if m != nil {
|
|
return m.ChangeDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (m *UserView) GetSequence() uint64 {
|
|
if m != nil {
|
|
return m.Sequence
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (m *UserView) GetLoginNames() []string {
|
|
if m != nil {
|
|
return m.LoginNames
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (m *UserView) GetPreferredLoginName() string {
|
|
if m != nil {
|
|
return m.PreferredLoginName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UserView) GetLastLogin() *timestamp.Timestamp {
|
|
if m != nil {
|
|
return m.LastLogin
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (m *UserView) GetResourceOwner() string {
|
|
if m != nil {
|
|
return m.ResourceOwner
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UserView) GetUserName() string {
|
|
if m != nil {
|
|
return m.UserName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type isUserView_User interface {
|
|
isUserView_User()
|
|
}
|
|
|
|
type UserView_Human struct {
|
|
Human *HumanView `protobuf:"bytes,11,opt,name=human,proto3,oneof"`
|
|
}
|
|
|
|
type UserView_Machine struct {
|
|
Machine *MachineView `protobuf:"bytes,12,opt,name=machine,proto3,oneof"`
|
|
}
|
|
|
|
func (*UserView_Human) isUserView_User() {}
|
|
|
|
func (*UserView_Machine) isUserView_User() {}
|
|
|
|
func (m *UserView) GetUser() isUserView_User {
|
|
if m != nil {
|
|
return m.User
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (m *UserView) GetHuman() *HumanView {
|
|
if x, ok := m.GetUser().(*UserView_Human); ok {
|
|
return x.Human
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (m *UserView) GetMachine() *MachineView {
|
|
if x, ok := m.GetUser().(*UserView_Machine); ok {
|
|
return x.Machine
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// XXX_OneofWrappers is for the internal use of the proto package.
|
|
func (*UserView) XXX_OneofWrappers() []interface{} {
|
|
return []interface{}{
|
|
(*UserView_Human)(nil),
|
|
(*UserView_Machine)(nil),
|
|
}
|
|
}
|
|
|
|
type MachineView struct {
|
|
LastKeyAdded *timestamp.Timestamp `protobuf:"bytes,1,opt,name=last_key_added,json=lastKeyAdded,proto3" json:"last_key_added,omitempty"`
|
|
Name string `protobuf:"bytes,2,opt,name=name,proto3" json:"name,omitempty"`
|
|
Description string `protobuf:"bytes,3,opt,name=description,proto3" json:"description,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *MachineView) Reset() { *m = MachineView{} }
|
|
func (m *MachineView) String() string { return proto.CompactTextString(m) }
|
|
func (*MachineView) ProtoMessage() {}
|
|
func (*MachineView) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{3}
|
|
}
|
|
|
|
func (m *MachineView) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_MachineView.Unmarshal(m, b)
|
|
}
|
|
func (m *MachineView) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_MachineView.Marshal(b, m, deterministic)
|
|
}
|
|
func (m *MachineView) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_MachineView.Merge(m, src)
|
|
}
|
|
func (m *MachineView) XXX_Size() int {
|
|
return xxx_messageInfo_MachineView.Size(m)
|
|
}
|
|
func (m *MachineView) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_MachineView.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_MachineView proto.InternalMessageInfo
|
|
|
|
func (m *MachineView) GetLastKeyAdded() *timestamp.Timestamp {
|
|
if m != nil {
|
|
return m.LastKeyAdded
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (m *MachineView) GetName() string {
|
|
if m != nil {
|
|
return m.Name
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *MachineView) GetDescription() string {
|
|
if m != nil {
|
|
return m.Description
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type MachineKeyView struct {
|
|
Id string `protobuf:"bytes,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
Type MachineKeyType `protobuf:"varint,2,opt,name=type,proto3,enum=caos.zitadel.auth.api.v1.MachineKeyType" json:"type,omitempty"`
|
|
Sequence uint64 `protobuf:"varint,3,opt,name=sequence,proto3" json:"sequence,omitempty"`
|
|
CreationDate *timestamp.Timestamp `protobuf:"bytes,4,opt,name=creation_date,json=creationDate,proto3" json:"creation_date,omitempty"`
|
|
ExpirationDate *timestamp.Timestamp `protobuf:"bytes,5,opt,name=expiration_date,json=expirationDate,proto3" json:"expiration_date,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *MachineKeyView) Reset() { *m = MachineKeyView{} }
|
|
func (m *MachineKeyView) String() string { return proto.CompactTextString(m) }
|
|
func (*MachineKeyView) ProtoMessage() {}
|
|
func (*MachineKeyView) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{4}
|
|
}
|
|
|
|
func (m *MachineKeyView) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_MachineKeyView.Unmarshal(m, b)
|
|
}
|
|
func (m *MachineKeyView) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_MachineKeyView.Marshal(b, m, deterministic)
|
|
}
|
|
func (m *MachineKeyView) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_MachineKeyView.Merge(m, src)
|
|
}
|
|
func (m *MachineKeyView) XXX_Size() int {
|
|
return xxx_messageInfo_MachineKeyView.Size(m)
|
|
}
|
|
func (m *MachineKeyView) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_MachineKeyView.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_MachineKeyView proto.InternalMessageInfo
|
|
|
|
func (m *MachineKeyView) GetId() string {
|
|
if m != nil {
|
|
return m.Id
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *MachineKeyView) GetType() MachineKeyType {
|
|
if m != nil {
|
|
return m.Type
|
|
}
|
|
return MachineKeyType_MACHINEKEY_UNSPECIFIED
|
|
}
|
|
|
|
func (m *MachineKeyView) GetSequence() uint64 {
|
|
if m != nil {
|
|
return m.Sequence
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (m *MachineKeyView) GetCreationDate() *timestamp.Timestamp {
|
|
if m != nil {
|
|
return m.CreationDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (m *MachineKeyView) GetExpirationDate() *timestamp.Timestamp {
|
|
if m != nil {
|
|
return m.ExpirationDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type HumanView struct {
|
|
PasswordChanged *timestamp.Timestamp `protobuf:"bytes,1,opt,name=password_changed,json=passwordChanged,proto3" json:"password_changed,omitempty"`
|
|
FirstName string `protobuf:"bytes,2,opt,name=first_name,json=firstName,proto3" json:"first_name,omitempty"`
|
|
LastName string `protobuf:"bytes,3,opt,name=last_name,json=lastName,proto3" json:"last_name,omitempty"`
|
|
DisplayName string `protobuf:"bytes,4,opt,name=display_name,json=displayName,proto3" json:"display_name,omitempty"`
|
|
NickName string `protobuf:"bytes,5,opt,name=nick_name,json=nickName,proto3" json:"nick_name,omitempty"`
|
|
PreferredLanguage string `protobuf:"bytes,6,opt,name=preferred_language,json=preferredLanguage,proto3" json:"preferred_language,omitempty"`
|
|
Gender Gender `protobuf:"varint,7,opt,name=gender,proto3,enum=caos.zitadel.auth.api.v1.Gender" json:"gender,omitempty"`
|
|
Email string `protobuf:"bytes,8,opt,name=email,proto3" json:"email,omitempty"`
|
|
IsEmailVerified bool `protobuf:"varint,9,opt,name=is_email_verified,json=isEmailVerified,proto3" json:"is_email_verified,omitempty"`
|
|
Phone string `protobuf:"bytes,10,opt,name=phone,proto3" json:"phone,omitempty"`
|
|
IsPhoneVerified bool `protobuf:"varint,11,opt,name=is_phone_verified,json=isPhoneVerified,proto3" json:"is_phone_verified,omitempty"`
|
|
Country string `protobuf:"bytes,12,opt,name=country,proto3" json:"country,omitempty"`
|
|
Locality string `protobuf:"bytes,13,opt,name=locality,proto3" json:"locality,omitempty"`
|
|
PostalCode string `protobuf:"bytes,14,opt,name=postal_code,json=postalCode,proto3" json:"postal_code,omitempty"`
|
|
Region string `protobuf:"bytes,15,opt,name=region,proto3" json:"region,omitempty"`
|
|
StreetAddress string `protobuf:"bytes,16,opt,name=street_address,json=streetAddress,proto3" json:"street_address,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *HumanView) Reset() { *m = HumanView{} }
|
|
func (m *HumanView) String() string { return proto.CompactTextString(m) }
|
|
func (*HumanView) ProtoMessage() {}
|
|
func (*HumanView) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{5}
|
|
}
|
|
|
|
func (m *HumanView) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_HumanView.Unmarshal(m, b)
|
|
}
|
|
func (m *HumanView) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_HumanView.Marshal(b, m, deterministic)
|
|
}
|
|
func (m *HumanView) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_HumanView.Merge(m, src)
|
|
}
|
|
func (m *HumanView) XXX_Size() int {
|
|
return xxx_messageInfo_HumanView.Size(m)
|
|
}
|
|
func (m *HumanView) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_HumanView.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_HumanView proto.InternalMessageInfo
|
|
|
|
func (m *HumanView) GetPasswordChanged() *timestamp.Timestamp {
|
|
if m != nil {
|
|
return m.PasswordChanged
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (m *HumanView) GetFirstName() string {
|
|
if m != nil {
|
|
return m.FirstName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *HumanView) GetLastName() string {
|
|
if m != nil {
|
|
return m.LastName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *HumanView) GetDisplayName() string {
|
|
if m != nil {
|
|
return m.DisplayName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *HumanView) GetNickName() string {
|
|
if m != nil {
|
|
return m.NickName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *HumanView) GetPreferredLanguage() string {
|
|
if m != nil {
|
|
return m.PreferredLanguage
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *HumanView) GetGender() Gender {
|
|
if m != nil {
|
|
return m.Gender
|
|
}
|
|
return Gender_GENDER_UNSPECIFIED
|
|
}
|
|
|
|
func (m *HumanView) GetEmail() string {
|
|
if m != nil {
|
|
return m.Email
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *HumanView) GetIsEmailVerified() bool {
|
|
if m != nil {
|
|
return m.IsEmailVerified
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (m *HumanView) GetPhone() string {
|
|
if m != nil {
|
|
return m.Phone
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *HumanView) GetIsPhoneVerified() bool {
|
|
if m != nil {
|
|
return m.IsPhoneVerified
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (m *HumanView) GetCountry() string {
|
|
if m != nil {
|
|
return m.Country
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *HumanView) GetLocality() string {
|
|
if m != nil {
|
|
return m.Locality
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *HumanView) GetPostalCode() string {
|
|
if m != nil {
|
|
return m.PostalCode
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *HumanView) GetRegion() string {
|
|
if m != nil {
|
|
return m.Region
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *HumanView) GetStreetAddress() string {
|
|
if m != nil {
|
|
return m.StreetAddress
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type UserProfile struct {
|
|
Id string `protobuf:"bytes,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
FirstName string `protobuf:"bytes,2,opt,name=first_name,json=firstName,proto3" json:"first_name,omitempty"`
|
|
LastName string `protobuf:"bytes,3,opt,name=last_name,json=lastName,proto3" json:"last_name,omitempty"`
|
|
NickName string `protobuf:"bytes,4,opt,name=nick_name,json=nickName,proto3" json:"nick_name,omitempty"`
|
|
DisplayName string `protobuf:"bytes,5,opt,name=display_name,json=displayName,proto3" json:"display_name,omitempty"`
|
|
PreferredLanguage string `protobuf:"bytes,6,opt,name=preferred_language,json=preferredLanguage,proto3" json:"preferred_language,omitempty"`
|
|
Gender Gender `protobuf:"varint,7,opt,name=gender,proto3,enum=caos.zitadel.auth.api.v1.Gender" json:"gender,omitempty"`
|
|
Sequence uint64 `protobuf:"varint,8,opt,name=sequence,proto3" json:"sequence,omitempty"`
|
|
CreationDate *timestamp.Timestamp `protobuf:"bytes,9,opt,name=creation_date,json=creationDate,proto3" json:"creation_date,omitempty"`
|
|
ChangeDate *timestamp.Timestamp `protobuf:"bytes,10,opt,name=change_date,json=changeDate,proto3" json:"change_date,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *UserProfile) Reset() { *m = UserProfile{} }
|
|
func (m *UserProfile) String() string { return proto.CompactTextString(m) }
|
|
func (*UserProfile) ProtoMessage() {}
|
|
func (*UserProfile) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{6}
|
|
}
|
|
|
|
func (m *UserProfile) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_UserProfile.Unmarshal(m, b)
|
|
}
|
|
func (m *UserProfile) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_UserProfile.Marshal(b, m, deterministic)
|
|
}
|
|
func (m *UserProfile) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_UserProfile.Merge(m, src)
|
|
}
|
|
func (m *UserProfile) XXX_Size() int {
|
|
return xxx_messageInfo_UserProfile.Size(m)
|
|
}
|
|
func (m *UserProfile) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_UserProfile.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_UserProfile proto.InternalMessageInfo
|
|
|
|
func (m *UserProfile) GetId() string {
|
|
if m != nil {
|
|
return m.Id
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UserProfile) GetFirstName() string {
|
|
if m != nil {
|
|
return m.FirstName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UserProfile) GetLastName() string {
|
|
if m != nil {
|
|
return m.LastName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UserProfile) GetNickName() string {
|
|
if m != nil {
|
|
return m.NickName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UserProfile) GetDisplayName() string {
|
|
if m != nil {
|
|
return m.DisplayName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UserProfile) GetPreferredLanguage() string {
|
|
if m != nil {
|
|
return m.PreferredLanguage
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UserProfile) GetGender() Gender {
|
|
if m != nil {
|
|
return m.Gender
|
|
}
|
|
return Gender_GENDER_UNSPECIFIED
|
|
}
|
|
|
|
func (m *UserProfile) GetSequence() uint64 {
|
|
if m != nil {
|
|
return m.Sequence
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (m *UserProfile) GetCreationDate() *timestamp.Timestamp {
|
|
if m != nil {
|
|
return m.CreationDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (m *UserProfile) GetChangeDate() *timestamp.Timestamp {
|
|
if m != nil {
|
|
return m.ChangeDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type UserProfileView struct {
|
|
Id string `protobuf:"bytes,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
FirstName string `protobuf:"bytes,2,opt,name=first_name,json=firstName,proto3" json:"first_name,omitempty"`
|
|
LastName string `protobuf:"bytes,3,opt,name=last_name,json=lastName,proto3" json:"last_name,omitempty"`
|
|
NickName string `protobuf:"bytes,4,opt,name=nick_name,json=nickName,proto3" json:"nick_name,omitempty"`
|
|
DisplayName string `protobuf:"bytes,5,opt,name=display_name,json=displayName,proto3" json:"display_name,omitempty"`
|
|
PreferredLanguage string `protobuf:"bytes,6,opt,name=preferred_language,json=preferredLanguage,proto3" json:"preferred_language,omitempty"`
|
|
Gender Gender `protobuf:"varint,7,opt,name=gender,proto3,enum=caos.zitadel.auth.api.v1.Gender" json:"gender,omitempty"`
|
|
Sequence uint64 `protobuf:"varint,8,opt,name=sequence,proto3" json:"sequence,omitempty"`
|
|
CreationDate *timestamp.Timestamp `protobuf:"bytes,9,opt,name=creation_date,json=creationDate,proto3" json:"creation_date,omitempty"`
|
|
ChangeDate *timestamp.Timestamp `protobuf:"bytes,10,opt,name=change_date,json=changeDate,proto3" json:"change_date,omitempty"`
|
|
LoginNames []string `protobuf:"bytes,11,rep,name=login_names,json=loginNames,proto3" json:"login_names,omitempty"`
|
|
PreferredLoginName string `protobuf:"bytes,12,opt,name=preferred_login_name,json=preferredLoginName,proto3" json:"preferred_login_name,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *UserProfileView) Reset() { *m = UserProfileView{} }
|
|
func (m *UserProfileView) String() string { return proto.CompactTextString(m) }
|
|
func (*UserProfileView) ProtoMessage() {}
|
|
func (*UserProfileView) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{7}
|
|
}
|
|
|
|
func (m *UserProfileView) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_UserProfileView.Unmarshal(m, b)
|
|
}
|
|
func (m *UserProfileView) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_UserProfileView.Marshal(b, m, deterministic)
|
|
}
|
|
func (m *UserProfileView) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_UserProfileView.Merge(m, src)
|
|
}
|
|
func (m *UserProfileView) XXX_Size() int {
|
|
return xxx_messageInfo_UserProfileView.Size(m)
|
|
}
|
|
func (m *UserProfileView) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_UserProfileView.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_UserProfileView proto.InternalMessageInfo
|
|
|
|
func (m *UserProfileView) GetId() string {
|
|
if m != nil {
|
|
return m.Id
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UserProfileView) GetFirstName() string {
|
|
if m != nil {
|
|
return m.FirstName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UserProfileView) GetLastName() string {
|
|
if m != nil {
|
|
return m.LastName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UserProfileView) GetNickName() string {
|
|
if m != nil {
|
|
return m.NickName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UserProfileView) GetDisplayName() string {
|
|
if m != nil {
|
|
return m.DisplayName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UserProfileView) GetPreferredLanguage() string {
|
|
if m != nil {
|
|
return m.PreferredLanguage
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UserProfileView) GetGender() Gender {
|
|
if m != nil {
|
|
return m.Gender
|
|
}
|
|
return Gender_GENDER_UNSPECIFIED
|
|
}
|
|
|
|
func (m *UserProfileView) GetSequence() uint64 {
|
|
if m != nil {
|
|
return m.Sequence
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (m *UserProfileView) GetCreationDate() *timestamp.Timestamp {
|
|
if m != nil {
|
|
return m.CreationDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (m *UserProfileView) GetChangeDate() *timestamp.Timestamp {
|
|
if m != nil {
|
|
return m.ChangeDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (m *UserProfileView) GetLoginNames() []string {
|
|
if m != nil {
|
|
return m.LoginNames
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (m *UserProfileView) GetPreferredLoginName() string {
|
|
if m != nil {
|
|
return m.PreferredLoginName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type UpdateUserProfileRequest struct {
|
|
FirstName string `protobuf:"bytes,1,opt,name=first_name,json=firstName,proto3" json:"first_name,omitempty"`
|
|
LastName string `protobuf:"bytes,2,opt,name=last_name,json=lastName,proto3" json:"last_name,omitempty"`
|
|
NickName string `protobuf:"bytes,3,opt,name=nick_name,json=nickName,proto3" json:"nick_name,omitempty"`
|
|
PreferredLanguage string `protobuf:"bytes,4,opt,name=preferred_language,json=preferredLanguage,proto3" json:"preferred_language,omitempty"`
|
|
Gender Gender `protobuf:"varint,5,opt,name=gender,proto3,enum=caos.zitadel.auth.api.v1.Gender" json:"gender,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *UpdateUserProfileRequest) Reset() { *m = UpdateUserProfileRequest{} }
|
|
func (m *UpdateUserProfileRequest) String() string { return proto.CompactTextString(m) }
|
|
func (*UpdateUserProfileRequest) ProtoMessage() {}
|
|
func (*UpdateUserProfileRequest) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{8}
|
|
}
|
|
|
|
func (m *UpdateUserProfileRequest) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_UpdateUserProfileRequest.Unmarshal(m, b)
|
|
}
|
|
func (m *UpdateUserProfileRequest) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_UpdateUserProfileRequest.Marshal(b, m, deterministic)
|
|
}
|
|
func (m *UpdateUserProfileRequest) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_UpdateUserProfileRequest.Merge(m, src)
|
|
}
|
|
func (m *UpdateUserProfileRequest) XXX_Size() int {
|
|
return xxx_messageInfo_UpdateUserProfileRequest.Size(m)
|
|
}
|
|
func (m *UpdateUserProfileRequest) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_UpdateUserProfileRequest.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_UpdateUserProfileRequest proto.InternalMessageInfo
|
|
|
|
func (m *UpdateUserProfileRequest) GetFirstName() string {
|
|
if m != nil {
|
|
return m.FirstName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UpdateUserProfileRequest) GetLastName() string {
|
|
if m != nil {
|
|
return m.LastName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UpdateUserProfileRequest) GetNickName() string {
|
|
if m != nil {
|
|
return m.NickName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UpdateUserProfileRequest) GetPreferredLanguage() string {
|
|
if m != nil {
|
|
return m.PreferredLanguage
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UpdateUserProfileRequest) GetGender() Gender {
|
|
if m != nil {
|
|
return m.Gender
|
|
}
|
|
return Gender_GENDER_UNSPECIFIED
|
|
}
|
|
|
|
type ChangeUserNameRequest struct {
|
|
UserName string `protobuf:"bytes,1,opt,name=user_name,json=userName,proto3" json:"user_name,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *ChangeUserNameRequest) Reset() { *m = ChangeUserNameRequest{} }
|
|
func (m *ChangeUserNameRequest) String() string { return proto.CompactTextString(m) }
|
|
func (*ChangeUserNameRequest) ProtoMessage() {}
|
|
func (*ChangeUserNameRequest) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{9}
|
|
}
|
|
|
|
func (m *ChangeUserNameRequest) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_ChangeUserNameRequest.Unmarshal(m, b)
|
|
}
|
|
func (m *ChangeUserNameRequest) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_ChangeUserNameRequest.Marshal(b, m, deterministic)
|
|
}
|
|
func (m *ChangeUserNameRequest) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_ChangeUserNameRequest.Merge(m, src)
|
|
}
|
|
func (m *ChangeUserNameRequest) XXX_Size() int {
|
|
return xxx_messageInfo_ChangeUserNameRequest.Size(m)
|
|
}
|
|
func (m *ChangeUserNameRequest) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_ChangeUserNameRequest.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_ChangeUserNameRequest proto.InternalMessageInfo
|
|
|
|
func (m *ChangeUserNameRequest) GetUserName() string {
|
|
if m != nil {
|
|
return m.UserName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type UserEmail struct {
|
|
Id string `protobuf:"bytes,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
Email string `protobuf:"bytes,2,opt,name=email,proto3" json:"email,omitempty"`
|
|
IsEmailVerified bool `protobuf:"varint,3,opt,name=isEmailVerified,proto3" json:"isEmailVerified,omitempty"`
|
|
Sequence uint64 `protobuf:"varint,4,opt,name=sequence,proto3" json:"sequence,omitempty"`
|
|
CreationDate *timestamp.Timestamp `protobuf:"bytes,5,opt,name=creation_date,json=creationDate,proto3" json:"creation_date,omitempty"`
|
|
ChangeDate *timestamp.Timestamp `protobuf:"bytes,6,opt,name=change_date,json=changeDate,proto3" json:"change_date,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *UserEmail) Reset() { *m = UserEmail{} }
|
|
func (m *UserEmail) String() string { return proto.CompactTextString(m) }
|
|
func (*UserEmail) ProtoMessage() {}
|
|
func (*UserEmail) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{10}
|
|
}
|
|
|
|
func (m *UserEmail) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_UserEmail.Unmarshal(m, b)
|
|
}
|
|
func (m *UserEmail) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_UserEmail.Marshal(b, m, deterministic)
|
|
}
|
|
func (m *UserEmail) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_UserEmail.Merge(m, src)
|
|
}
|
|
func (m *UserEmail) XXX_Size() int {
|
|
return xxx_messageInfo_UserEmail.Size(m)
|
|
}
|
|
func (m *UserEmail) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_UserEmail.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_UserEmail proto.InternalMessageInfo
|
|
|
|
func (m *UserEmail) GetId() string {
|
|
if m != nil {
|
|
return m.Id
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UserEmail) GetEmail() string {
|
|
if m != nil {
|
|
return m.Email
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UserEmail) GetIsEmailVerified() bool {
|
|
if m != nil {
|
|
return m.IsEmailVerified
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (m *UserEmail) GetSequence() uint64 {
|
|
if m != nil {
|
|
return m.Sequence
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (m *UserEmail) GetCreationDate() *timestamp.Timestamp {
|
|
if m != nil {
|
|
return m.CreationDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (m *UserEmail) GetChangeDate() *timestamp.Timestamp {
|
|
if m != nil {
|
|
return m.ChangeDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type UserEmailView struct {
|
|
Id string `protobuf:"bytes,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
Email string `protobuf:"bytes,2,opt,name=email,proto3" json:"email,omitempty"`
|
|
IsEmailVerified bool `protobuf:"varint,3,opt,name=isEmailVerified,proto3" json:"isEmailVerified,omitempty"`
|
|
Sequence uint64 `protobuf:"varint,4,opt,name=sequence,proto3" json:"sequence,omitempty"`
|
|
CreationDate *timestamp.Timestamp `protobuf:"bytes,5,opt,name=creation_date,json=creationDate,proto3" json:"creation_date,omitempty"`
|
|
ChangeDate *timestamp.Timestamp `protobuf:"bytes,6,opt,name=change_date,json=changeDate,proto3" json:"change_date,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *UserEmailView) Reset() { *m = UserEmailView{} }
|
|
func (m *UserEmailView) String() string { return proto.CompactTextString(m) }
|
|
func (*UserEmailView) ProtoMessage() {}
|
|
func (*UserEmailView) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{11}
|
|
}
|
|
|
|
func (m *UserEmailView) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_UserEmailView.Unmarshal(m, b)
|
|
}
|
|
func (m *UserEmailView) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_UserEmailView.Marshal(b, m, deterministic)
|
|
}
|
|
func (m *UserEmailView) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_UserEmailView.Merge(m, src)
|
|
}
|
|
func (m *UserEmailView) XXX_Size() int {
|
|
return xxx_messageInfo_UserEmailView.Size(m)
|
|
}
|
|
func (m *UserEmailView) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_UserEmailView.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_UserEmailView proto.InternalMessageInfo
|
|
|
|
func (m *UserEmailView) GetId() string {
|
|
if m != nil {
|
|
return m.Id
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UserEmailView) GetEmail() string {
|
|
if m != nil {
|
|
return m.Email
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UserEmailView) GetIsEmailVerified() bool {
|
|
if m != nil {
|
|
return m.IsEmailVerified
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (m *UserEmailView) GetSequence() uint64 {
|
|
if m != nil {
|
|
return m.Sequence
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (m *UserEmailView) GetCreationDate() *timestamp.Timestamp {
|
|
if m != nil {
|
|
return m.CreationDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (m *UserEmailView) GetChangeDate() *timestamp.Timestamp {
|
|
if m != nil {
|
|
return m.ChangeDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type VerifyMyUserEmailRequest struct {
|
|
Code string `protobuf:"bytes,1,opt,name=code,proto3" json:"code,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *VerifyMyUserEmailRequest) Reset() { *m = VerifyMyUserEmailRequest{} }
|
|
func (m *VerifyMyUserEmailRequest) String() string { return proto.CompactTextString(m) }
|
|
func (*VerifyMyUserEmailRequest) ProtoMessage() {}
|
|
func (*VerifyMyUserEmailRequest) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{12}
|
|
}
|
|
|
|
func (m *VerifyMyUserEmailRequest) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_VerifyMyUserEmailRequest.Unmarshal(m, b)
|
|
}
|
|
func (m *VerifyMyUserEmailRequest) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_VerifyMyUserEmailRequest.Marshal(b, m, deterministic)
|
|
}
|
|
func (m *VerifyMyUserEmailRequest) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_VerifyMyUserEmailRequest.Merge(m, src)
|
|
}
|
|
func (m *VerifyMyUserEmailRequest) XXX_Size() int {
|
|
return xxx_messageInfo_VerifyMyUserEmailRequest.Size(m)
|
|
}
|
|
func (m *VerifyMyUserEmailRequest) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_VerifyMyUserEmailRequest.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_VerifyMyUserEmailRequest proto.InternalMessageInfo
|
|
|
|
func (m *VerifyMyUserEmailRequest) GetCode() string {
|
|
if m != nil {
|
|
return m.Code
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type UpdateUserEmailRequest struct {
|
|
Email string `protobuf:"bytes,1,opt,name=email,proto3" json:"email,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *UpdateUserEmailRequest) Reset() { *m = UpdateUserEmailRequest{} }
|
|
func (m *UpdateUserEmailRequest) String() string { return proto.CompactTextString(m) }
|
|
func (*UpdateUserEmailRequest) ProtoMessage() {}
|
|
func (*UpdateUserEmailRequest) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{13}
|
|
}
|
|
|
|
func (m *UpdateUserEmailRequest) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_UpdateUserEmailRequest.Unmarshal(m, b)
|
|
}
|
|
func (m *UpdateUserEmailRequest) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_UpdateUserEmailRequest.Marshal(b, m, deterministic)
|
|
}
|
|
func (m *UpdateUserEmailRequest) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_UpdateUserEmailRequest.Merge(m, src)
|
|
}
|
|
func (m *UpdateUserEmailRequest) XXX_Size() int {
|
|
return xxx_messageInfo_UpdateUserEmailRequest.Size(m)
|
|
}
|
|
func (m *UpdateUserEmailRequest) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_UpdateUserEmailRequest.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_UpdateUserEmailRequest proto.InternalMessageInfo
|
|
|
|
func (m *UpdateUserEmailRequest) GetEmail() string {
|
|
if m != nil {
|
|
return m.Email
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type UserPhone struct {
|
|
Id string `protobuf:"bytes,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
Phone string `protobuf:"bytes,2,opt,name=phone,proto3" json:"phone,omitempty"`
|
|
IsPhoneVerified bool `protobuf:"varint,3,opt,name=is_phone_verified,json=isPhoneVerified,proto3" json:"is_phone_verified,omitempty"`
|
|
Sequence uint64 `protobuf:"varint,4,opt,name=sequence,proto3" json:"sequence,omitempty"`
|
|
CreationDate *timestamp.Timestamp `protobuf:"bytes,5,opt,name=creation_date,json=creationDate,proto3" json:"creation_date,omitempty"`
|
|
ChangeDate *timestamp.Timestamp `protobuf:"bytes,6,opt,name=change_date,json=changeDate,proto3" json:"change_date,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *UserPhone) Reset() { *m = UserPhone{} }
|
|
func (m *UserPhone) String() string { return proto.CompactTextString(m) }
|
|
func (*UserPhone) ProtoMessage() {}
|
|
func (*UserPhone) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{14}
|
|
}
|
|
|
|
func (m *UserPhone) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_UserPhone.Unmarshal(m, b)
|
|
}
|
|
func (m *UserPhone) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_UserPhone.Marshal(b, m, deterministic)
|
|
}
|
|
func (m *UserPhone) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_UserPhone.Merge(m, src)
|
|
}
|
|
func (m *UserPhone) XXX_Size() int {
|
|
return xxx_messageInfo_UserPhone.Size(m)
|
|
}
|
|
func (m *UserPhone) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_UserPhone.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_UserPhone proto.InternalMessageInfo
|
|
|
|
func (m *UserPhone) GetId() string {
|
|
if m != nil {
|
|
return m.Id
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UserPhone) GetPhone() string {
|
|
if m != nil {
|
|
return m.Phone
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UserPhone) GetIsPhoneVerified() bool {
|
|
if m != nil {
|
|
return m.IsPhoneVerified
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (m *UserPhone) GetSequence() uint64 {
|
|
if m != nil {
|
|
return m.Sequence
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (m *UserPhone) GetCreationDate() *timestamp.Timestamp {
|
|
if m != nil {
|
|
return m.CreationDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (m *UserPhone) GetChangeDate() *timestamp.Timestamp {
|
|
if m != nil {
|
|
return m.ChangeDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type UserPhoneView struct {
|
|
Id string `protobuf:"bytes,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
Phone string `protobuf:"bytes,2,opt,name=phone,proto3" json:"phone,omitempty"`
|
|
IsPhoneVerified bool `protobuf:"varint,3,opt,name=is_phone_verified,json=isPhoneVerified,proto3" json:"is_phone_verified,omitempty"`
|
|
Sequence uint64 `protobuf:"varint,4,opt,name=sequence,proto3" json:"sequence,omitempty"`
|
|
CreationDate *timestamp.Timestamp `protobuf:"bytes,5,opt,name=creation_date,json=creationDate,proto3" json:"creation_date,omitempty"`
|
|
ChangeDate *timestamp.Timestamp `protobuf:"bytes,6,opt,name=change_date,json=changeDate,proto3" json:"change_date,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *UserPhoneView) Reset() { *m = UserPhoneView{} }
|
|
func (m *UserPhoneView) String() string { return proto.CompactTextString(m) }
|
|
func (*UserPhoneView) ProtoMessage() {}
|
|
func (*UserPhoneView) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{15}
|
|
}
|
|
|
|
func (m *UserPhoneView) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_UserPhoneView.Unmarshal(m, b)
|
|
}
|
|
func (m *UserPhoneView) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_UserPhoneView.Marshal(b, m, deterministic)
|
|
}
|
|
func (m *UserPhoneView) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_UserPhoneView.Merge(m, src)
|
|
}
|
|
func (m *UserPhoneView) XXX_Size() int {
|
|
return xxx_messageInfo_UserPhoneView.Size(m)
|
|
}
|
|
func (m *UserPhoneView) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_UserPhoneView.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_UserPhoneView proto.InternalMessageInfo
|
|
|
|
func (m *UserPhoneView) GetId() string {
|
|
if m != nil {
|
|
return m.Id
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UserPhoneView) GetPhone() string {
|
|
if m != nil {
|
|
return m.Phone
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UserPhoneView) GetIsPhoneVerified() bool {
|
|
if m != nil {
|
|
return m.IsPhoneVerified
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (m *UserPhoneView) GetSequence() uint64 {
|
|
if m != nil {
|
|
return m.Sequence
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (m *UserPhoneView) GetCreationDate() *timestamp.Timestamp {
|
|
if m != nil {
|
|
return m.CreationDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (m *UserPhoneView) GetChangeDate() *timestamp.Timestamp {
|
|
if m != nil {
|
|
return m.ChangeDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type UpdateUserPhoneRequest struct {
|
|
Phone string `protobuf:"bytes,1,opt,name=phone,proto3" json:"phone,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *UpdateUserPhoneRequest) Reset() { *m = UpdateUserPhoneRequest{} }
|
|
func (m *UpdateUserPhoneRequest) String() string { return proto.CompactTextString(m) }
|
|
func (*UpdateUserPhoneRequest) ProtoMessage() {}
|
|
func (*UpdateUserPhoneRequest) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{16}
|
|
}
|
|
|
|
func (m *UpdateUserPhoneRequest) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_UpdateUserPhoneRequest.Unmarshal(m, b)
|
|
}
|
|
func (m *UpdateUserPhoneRequest) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_UpdateUserPhoneRequest.Marshal(b, m, deterministic)
|
|
}
|
|
func (m *UpdateUserPhoneRequest) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_UpdateUserPhoneRequest.Merge(m, src)
|
|
}
|
|
func (m *UpdateUserPhoneRequest) XXX_Size() int {
|
|
return xxx_messageInfo_UpdateUserPhoneRequest.Size(m)
|
|
}
|
|
func (m *UpdateUserPhoneRequest) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_UpdateUserPhoneRequest.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_UpdateUserPhoneRequest proto.InternalMessageInfo
|
|
|
|
func (m *UpdateUserPhoneRequest) GetPhone() string {
|
|
if m != nil {
|
|
return m.Phone
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type VerifyUserPhoneRequest struct {
|
|
Code string `protobuf:"bytes,1,opt,name=code,proto3" json:"code,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *VerifyUserPhoneRequest) Reset() { *m = VerifyUserPhoneRequest{} }
|
|
func (m *VerifyUserPhoneRequest) String() string { return proto.CompactTextString(m) }
|
|
func (*VerifyUserPhoneRequest) ProtoMessage() {}
|
|
func (*VerifyUserPhoneRequest) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{17}
|
|
}
|
|
|
|
func (m *VerifyUserPhoneRequest) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_VerifyUserPhoneRequest.Unmarshal(m, b)
|
|
}
|
|
func (m *VerifyUserPhoneRequest) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_VerifyUserPhoneRequest.Marshal(b, m, deterministic)
|
|
}
|
|
func (m *VerifyUserPhoneRequest) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_VerifyUserPhoneRequest.Merge(m, src)
|
|
}
|
|
func (m *VerifyUserPhoneRequest) XXX_Size() int {
|
|
return xxx_messageInfo_VerifyUserPhoneRequest.Size(m)
|
|
}
|
|
func (m *VerifyUserPhoneRequest) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_VerifyUserPhoneRequest.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_VerifyUserPhoneRequest proto.InternalMessageInfo
|
|
|
|
func (m *VerifyUserPhoneRequest) GetCode() string {
|
|
if m != nil {
|
|
return m.Code
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type UserAddress struct {
|
|
Id string `protobuf:"bytes,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
Country string `protobuf:"bytes,2,opt,name=country,proto3" json:"country,omitempty"`
|
|
Locality string `protobuf:"bytes,3,opt,name=locality,proto3" json:"locality,omitempty"`
|
|
PostalCode string `protobuf:"bytes,4,opt,name=postal_code,json=postalCode,proto3" json:"postal_code,omitempty"`
|
|
Region string `protobuf:"bytes,5,opt,name=region,proto3" json:"region,omitempty"`
|
|
StreetAddress string `protobuf:"bytes,6,opt,name=street_address,json=streetAddress,proto3" json:"street_address,omitempty"`
|
|
Sequence uint64 `protobuf:"varint,7,opt,name=sequence,proto3" json:"sequence,omitempty"`
|
|
CreationDate *timestamp.Timestamp `protobuf:"bytes,8,opt,name=creation_date,json=creationDate,proto3" json:"creation_date,omitempty"`
|
|
ChangeDate *timestamp.Timestamp `protobuf:"bytes,9,opt,name=change_date,json=changeDate,proto3" json:"change_date,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *UserAddress) Reset() { *m = UserAddress{} }
|
|
func (m *UserAddress) String() string { return proto.CompactTextString(m) }
|
|
func (*UserAddress) ProtoMessage() {}
|
|
func (*UserAddress) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{18}
|
|
}
|
|
|
|
func (m *UserAddress) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_UserAddress.Unmarshal(m, b)
|
|
}
|
|
func (m *UserAddress) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_UserAddress.Marshal(b, m, deterministic)
|
|
}
|
|
func (m *UserAddress) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_UserAddress.Merge(m, src)
|
|
}
|
|
func (m *UserAddress) XXX_Size() int {
|
|
return xxx_messageInfo_UserAddress.Size(m)
|
|
}
|
|
func (m *UserAddress) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_UserAddress.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_UserAddress proto.InternalMessageInfo
|
|
|
|
func (m *UserAddress) GetId() string {
|
|
if m != nil {
|
|
return m.Id
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UserAddress) GetCountry() string {
|
|
if m != nil {
|
|
return m.Country
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UserAddress) GetLocality() string {
|
|
if m != nil {
|
|
return m.Locality
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UserAddress) GetPostalCode() string {
|
|
if m != nil {
|
|
return m.PostalCode
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UserAddress) GetRegion() string {
|
|
if m != nil {
|
|
return m.Region
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UserAddress) GetStreetAddress() string {
|
|
if m != nil {
|
|
return m.StreetAddress
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UserAddress) GetSequence() uint64 {
|
|
if m != nil {
|
|
return m.Sequence
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (m *UserAddress) GetCreationDate() *timestamp.Timestamp {
|
|
if m != nil {
|
|
return m.CreationDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (m *UserAddress) GetChangeDate() *timestamp.Timestamp {
|
|
if m != nil {
|
|
return m.ChangeDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type UserAddressView struct {
|
|
Id string `protobuf:"bytes,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
Country string `protobuf:"bytes,2,opt,name=country,proto3" json:"country,omitempty"`
|
|
Locality string `protobuf:"bytes,3,opt,name=locality,proto3" json:"locality,omitempty"`
|
|
PostalCode string `protobuf:"bytes,4,opt,name=postal_code,json=postalCode,proto3" json:"postal_code,omitempty"`
|
|
Region string `protobuf:"bytes,5,opt,name=region,proto3" json:"region,omitempty"`
|
|
StreetAddress string `protobuf:"bytes,6,opt,name=street_address,json=streetAddress,proto3" json:"street_address,omitempty"`
|
|
Sequence uint64 `protobuf:"varint,7,opt,name=sequence,proto3" json:"sequence,omitempty"`
|
|
CreationDate *timestamp.Timestamp `protobuf:"bytes,8,opt,name=creation_date,json=creationDate,proto3" json:"creation_date,omitempty"`
|
|
ChangeDate *timestamp.Timestamp `protobuf:"bytes,9,opt,name=change_date,json=changeDate,proto3" json:"change_date,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *UserAddressView) Reset() { *m = UserAddressView{} }
|
|
func (m *UserAddressView) String() string { return proto.CompactTextString(m) }
|
|
func (*UserAddressView) ProtoMessage() {}
|
|
func (*UserAddressView) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{19}
|
|
}
|
|
|
|
func (m *UserAddressView) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_UserAddressView.Unmarshal(m, b)
|
|
}
|
|
func (m *UserAddressView) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_UserAddressView.Marshal(b, m, deterministic)
|
|
}
|
|
func (m *UserAddressView) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_UserAddressView.Merge(m, src)
|
|
}
|
|
func (m *UserAddressView) XXX_Size() int {
|
|
return xxx_messageInfo_UserAddressView.Size(m)
|
|
}
|
|
func (m *UserAddressView) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_UserAddressView.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_UserAddressView proto.InternalMessageInfo
|
|
|
|
func (m *UserAddressView) GetId() string {
|
|
if m != nil {
|
|
return m.Id
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UserAddressView) GetCountry() string {
|
|
if m != nil {
|
|
return m.Country
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UserAddressView) GetLocality() string {
|
|
if m != nil {
|
|
return m.Locality
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UserAddressView) GetPostalCode() string {
|
|
if m != nil {
|
|
return m.PostalCode
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UserAddressView) GetRegion() string {
|
|
if m != nil {
|
|
return m.Region
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UserAddressView) GetStreetAddress() string {
|
|
if m != nil {
|
|
return m.StreetAddress
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UserAddressView) GetSequence() uint64 {
|
|
if m != nil {
|
|
return m.Sequence
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (m *UserAddressView) GetCreationDate() *timestamp.Timestamp {
|
|
if m != nil {
|
|
return m.CreationDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (m *UserAddressView) GetChangeDate() *timestamp.Timestamp {
|
|
if m != nil {
|
|
return m.ChangeDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type UpdateUserAddressRequest struct {
|
|
Country string `protobuf:"bytes,1,opt,name=country,proto3" json:"country,omitempty"`
|
|
Locality string `protobuf:"bytes,2,opt,name=locality,proto3" json:"locality,omitempty"`
|
|
PostalCode string `protobuf:"bytes,3,opt,name=postal_code,json=postalCode,proto3" json:"postal_code,omitempty"`
|
|
Region string `protobuf:"bytes,4,opt,name=region,proto3" json:"region,omitempty"`
|
|
StreetAddress string `protobuf:"bytes,5,opt,name=street_address,json=streetAddress,proto3" json:"street_address,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *UpdateUserAddressRequest) Reset() { *m = UpdateUserAddressRequest{} }
|
|
func (m *UpdateUserAddressRequest) String() string { return proto.CompactTextString(m) }
|
|
func (*UpdateUserAddressRequest) ProtoMessage() {}
|
|
func (*UpdateUserAddressRequest) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{20}
|
|
}
|
|
|
|
func (m *UpdateUserAddressRequest) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_UpdateUserAddressRequest.Unmarshal(m, b)
|
|
}
|
|
func (m *UpdateUserAddressRequest) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_UpdateUserAddressRequest.Marshal(b, m, deterministic)
|
|
}
|
|
func (m *UpdateUserAddressRequest) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_UpdateUserAddressRequest.Merge(m, src)
|
|
}
|
|
func (m *UpdateUserAddressRequest) XXX_Size() int {
|
|
return xxx_messageInfo_UpdateUserAddressRequest.Size(m)
|
|
}
|
|
func (m *UpdateUserAddressRequest) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_UpdateUserAddressRequest.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_UpdateUserAddressRequest proto.InternalMessageInfo
|
|
|
|
func (m *UpdateUserAddressRequest) GetCountry() string {
|
|
if m != nil {
|
|
return m.Country
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UpdateUserAddressRequest) GetLocality() string {
|
|
if m != nil {
|
|
return m.Locality
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UpdateUserAddressRequest) GetPostalCode() string {
|
|
if m != nil {
|
|
return m.PostalCode
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UpdateUserAddressRequest) GetRegion() string {
|
|
if m != nil {
|
|
return m.Region
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UpdateUserAddressRequest) GetStreetAddress() string {
|
|
if m != nil {
|
|
return m.StreetAddress
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type PasswordChange struct {
|
|
OldPassword string `protobuf:"bytes,1,opt,name=old_password,json=oldPassword,proto3" json:"old_password,omitempty"`
|
|
NewPassword string `protobuf:"bytes,2,opt,name=new_password,json=newPassword,proto3" json:"new_password,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *PasswordChange) Reset() { *m = PasswordChange{} }
|
|
func (m *PasswordChange) String() string { return proto.CompactTextString(m) }
|
|
func (*PasswordChange) ProtoMessage() {}
|
|
func (*PasswordChange) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{21}
|
|
}
|
|
|
|
func (m *PasswordChange) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_PasswordChange.Unmarshal(m, b)
|
|
}
|
|
func (m *PasswordChange) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_PasswordChange.Marshal(b, m, deterministic)
|
|
}
|
|
func (m *PasswordChange) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_PasswordChange.Merge(m, src)
|
|
}
|
|
func (m *PasswordChange) XXX_Size() int {
|
|
return xxx_messageInfo_PasswordChange.Size(m)
|
|
}
|
|
func (m *PasswordChange) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_PasswordChange.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_PasswordChange proto.InternalMessageInfo
|
|
|
|
func (m *PasswordChange) GetOldPassword() string {
|
|
if m != nil {
|
|
return m.OldPassword
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *PasswordChange) GetNewPassword() string {
|
|
if m != nil {
|
|
return m.NewPassword
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type VerifyMfaOtp struct {
|
|
Code string `protobuf:"bytes,1,opt,name=code,proto3" json:"code,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *VerifyMfaOtp) Reset() { *m = VerifyMfaOtp{} }
|
|
func (m *VerifyMfaOtp) String() string { return proto.CompactTextString(m) }
|
|
func (*VerifyMfaOtp) ProtoMessage() {}
|
|
func (*VerifyMfaOtp) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{22}
|
|
}
|
|
|
|
func (m *VerifyMfaOtp) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_VerifyMfaOtp.Unmarshal(m, b)
|
|
}
|
|
func (m *VerifyMfaOtp) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_VerifyMfaOtp.Marshal(b, m, deterministic)
|
|
}
|
|
func (m *VerifyMfaOtp) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_VerifyMfaOtp.Merge(m, src)
|
|
}
|
|
func (m *VerifyMfaOtp) XXX_Size() int {
|
|
return xxx_messageInfo_VerifyMfaOtp.Size(m)
|
|
}
|
|
func (m *VerifyMfaOtp) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_VerifyMfaOtp.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_VerifyMfaOtp proto.InternalMessageInfo
|
|
|
|
func (m *VerifyMfaOtp) GetCode() string {
|
|
if m != nil {
|
|
return m.Code
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type MultiFactors struct {
|
|
Mfas []*MultiFactor `protobuf:"bytes,1,rep,name=mfas,proto3" json:"mfas,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *MultiFactors) Reset() { *m = MultiFactors{} }
|
|
func (m *MultiFactors) String() string { return proto.CompactTextString(m) }
|
|
func (*MultiFactors) ProtoMessage() {}
|
|
func (*MultiFactors) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{23}
|
|
}
|
|
|
|
func (m *MultiFactors) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_MultiFactors.Unmarshal(m, b)
|
|
}
|
|
func (m *MultiFactors) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_MultiFactors.Marshal(b, m, deterministic)
|
|
}
|
|
func (m *MultiFactors) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_MultiFactors.Merge(m, src)
|
|
}
|
|
func (m *MultiFactors) XXX_Size() int {
|
|
return xxx_messageInfo_MultiFactors.Size(m)
|
|
}
|
|
func (m *MultiFactors) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_MultiFactors.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_MultiFactors proto.InternalMessageInfo
|
|
|
|
func (m *MultiFactors) GetMfas() []*MultiFactor {
|
|
if m != nil {
|
|
return m.Mfas
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type MultiFactor struct {
|
|
Type MfaType `protobuf:"varint,1,opt,name=type,proto3,enum=caos.zitadel.auth.api.v1.MfaType" json:"type,omitempty"`
|
|
State MFAState `protobuf:"varint,2,opt,name=state,proto3,enum=caos.zitadel.auth.api.v1.MFAState" json:"state,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *MultiFactor) Reset() { *m = MultiFactor{} }
|
|
func (m *MultiFactor) String() string { return proto.CompactTextString(m) }
|
|
func (*MultiFactor) ProtoMessage() {}
|
|
func (*MultiFactor) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{24}
|
|
}
|
|
|
|
func (m *MultiFactor) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_MultiFactor.Unmarshal(m, b)
|
|
}
|
|
func (m *MultiFactor) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_MultiFactor.Marshal(b, m, deterministic)
|
|
}
|
|
func (m *MultiFactor) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_MultiFactor.Merge(m, src)
|
|
}
|
|
func (m *MultiFactor) XXX_Size() int {
|
|
return xxx_messageInfo_MultiFactor.Size(m)
|
|
}
|
|
func (m *MultiFactor) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_MultiFactor.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_MultiFactor proto.InternalMessageInfo
|
|
|
|
func (m *MultiFactor) GetType() MfaType {
|
|
if m != nil {
|
|
return m.Type
|
|
}
|
|
return MfaType_MFATYPE_UNSPECIFIED
|
|
}
|
|
|
|
func (m *MultiFactor) GetState() MFAState {
|
|
if m != nil {
|
|
return m.State
|
|
}
|
|
return MFAState_MFASTATE_UNSPECIFIED
|
|
}
|
|
|
|
type MfaOtpResponse struct {
|
|
UserId string `protobuf:"bytes,1,opt,name=user_id,json=userId,proto3" json:"user_id,omitempty"`
|
|
Url string `protobuf:"bytes,2,opt,name=url,proto3" json:"url,omitempty"`
|
|
Secret string `protobuf:"bytes,3,opt,name=secret,proto3" json:"secret,omitempty"`
|
|
State MFAState `protobuf:"varint,4,opt,name=state,proto3,enum=caos.zitadel.auth.api.v1.MFAState" json:"state,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *MfaOtpResponse) Reset() { *m = MfaOtpResponse{} }
|
|
func (m *MfaOtpResponse) String() string { return proto.CompactTextString(m) }
|
|
func (*MfaOtpResponse) ProtoMessage() {}
|
|
func (*MfaOtpResponse) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{25}
|
|
}
|
|
|
|
func (m *MfaOtpResponse) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_MfaOtpResponse.Unmarshal(m, b)
|
|
}
|
|
func (m *MfaOtpResponse) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_MfaOtpResponse.Marshal(b, m, deterministic)
|
|
}
|
|
func (m *MfaOtpResponse) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_MfaOtpResponse.Merge(m, src)
|
|
}
|
|
func (m *MfaOtpResponse) XXX_Size() int {
|
|
return xxx_messageInfo_MfaOtpResponse.Size(m)
|
|
}
|
|
func (m *MfaOtpResponse) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_MfaOtpResponse.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_MfaOtpResponse proto.InternalMessageInfo
|
|
|
|
func (m *MfaOtpResponse) GetUserId() string {
|
|
if m != nil {
|
|
return m.UserId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *MfaOtpResponse) GetUrl() string {
|
|
if m != nil {
|
|
return m.Url
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *MfaOtpResponse) GetSecret() string {
|
|
if m != nil {
|
|
return m.Secret
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *MfaOtpResponse) GetState() MFAState {
|
|
if m != nil {
|
|
return m.State
|
|
}
|
|
return MFAState_MFASTATE_UNSPECIFIED
|
|
}
|
|
|
|
type UserGrantSearchRequest struct {
|
|
Offset uint64 `protobuf:"varint,1,opt,name=offset,proto3" json:"offset,omitempty"`
|
|
Limit uint64 `protobuf:"varint,2,opt,name=limit,proto3" json:"limit,omitempty"`
|
|
SortingColumn UserGrantSearchKey `protobuf:"varint,3,opt,name=sorting_column,json=sortingColumn,proto3,enum=caos.zitadel.auth.api.v1.UserGrantSearchKey" json:"sorting_column,omitempty"`
|
|
Asc bool `protobuf:"varint,4,opt,name=asc,proto3" json:"asc,omitempty"`
|
|
Queries []*UserGrantSearchQuery `protobuf:"bytes,5,rep,name=queries,proto3" json:"queries,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *UserGrantSearchRequest) Reset() { *m = UserGrantSearchRequest{} }
|
|
func (m *UserGrantSearchRequest) String() string { return proto.CompactTextString(m) }
|
|
func (*UserGrantSearchRequest) ProtoMessage() {}
|
|
func (*UserGrantSearchRequest) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{26}
|
|
}
|
|
|
|
func (m *UserGrantSearchRequest) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_UserGrantSearchRequest.Unmarshal(m, b)
|
|
}
|
|
func (m *UserGrantSearchRequest) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_UserGrantSearchRequest.Marshal(b, m, deterministic)
|
|
}
|
|
func (m *UserGrantSearchRequest) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_UserGrantSearchRequest.Merge(m, src)
|
|
}
|
|
func (m *UserGrantSearchRequest) XXX_Size() int {
|
|
return xxx_messageInfo_UserGrantSearchRequest.Size(m)
|
|
}
|
|
func (m *UserGrantSearchRequest) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_UserGrantSearchRequest.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_UserGrantSearchRequest proto.InternalMessageInfo
|
|
|
|
func (m *UserGrantSearchRequest) GetOffset() uint64 {
|
|
if m != nil {
|
|
return m.Offset
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (m *UserGrantSearchRequest) GetLimit() uint64 {
|
|
if m != nil {
|
|
return m.Limit
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (m *UserGrantSearchRequest) GetSortingColumn() UserGrantSearchKey {
|
|
if m != nil {
|
|
return m.SortingColumn
|
|
}
|
|
return UserGrantSearchKey_UserGrantSearchKey_UNKNOWN
|
|
}
|
|
|
|
func (m *UserGrantSearchRequest) GetAsc() bool {
|
|
if m != nil {
|
|
return m.Asc
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (m *UserGrantSearchRequest) GetQueries() []*UserGrantSearchQuery {
|
|
if m != nil {
|
|
return m.Queries
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type UserGrantSearchQuery struct {
|
|
Key UserGrantSearchKey `protobuf:"varint,1,opt,name=key,proto3,enum=caos.zitadel.auth.api.v1.UserGrantSearchKey" json:"key,omitempty"`
|
|
Method SearchMethod `protobuf:"varint,2,opt,name=method,proto3,enum=caos.zitadel.auth.api.v1.SearchMethod" json:"method,omitempty"`
|
|
Value string `protobuf:"bytes,3,opt,name=value,proto3" json:"value,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *UserGrantSearchQuery) Reset() { *m = UserGrantSearchQuery{} }
|
|
func (m *UserGrantSearchQuery) String() string { return proto.CompactTextString(m) }
|
|
func (*UserGrantSearchQuery) ProtoMessage() {}
|
|
func (*UserGrantSearchQuery) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{27}
|
|
}
|
|
|
|
func (m *UserGrantSearchQuery) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_UserGrantSearchQuery.Unmarshal(m, b)
|
|
}
|
|
func (m *UserGrantSearchQuery) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_UserGrantSearchQuery.Marshal(b, m, deterministic)
|
|
}
|
|
func (m *UserGrantSearchQuery) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_UserGrantSearchQuery.Merge(m, src)
|
|
}
|
|
func (m *UserGrantSearchQuery) XXX_Size() int {
|
|
return xxx_messageInfo_UserGrantSearchQuery.Size(m)
|
|
}
|
|
func (m *UserGrantSearchQuery) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_UserGrantSearchQuery.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_UserGrantSearchQuery proto.InternalMessageInfo
|
|
|
|
func (m *UserGrantSearchQuery) GetKey() UserGrantSearchKey {
|
|
if m != nil {
|
|
return m.Key
|
|
}
|
|
return UserGrantSearchKey_UserGrantSearchKey_UNKNOWN
|
|
}
|
|
|
|
func (m *UserGrantSearchQuery) GetMethod() SearchMethod {
|
|
if m != nil {
|
|
return m.Method
|
|
}
|
|
return SearchMethod_SEARCHMETHOD_EQUALS
|
|
}
|
|
|
|
func (m *UserGrantSearchQuery) GetValue() string {
|
|
if m != nil {
|
|
return m.Value
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type UserGrantSearchResponse struct {
|
|
Offset uint64 `protobuf:"varint,1,opt,name=offset,proto3" json:"offset,omitempty"`
|
|
Limit uint64 `protobuf:"varint,2,opt,name=limit,proto3" json:"limit,omitempty"`
|
|
TotalResult uint64 `protobuf:"varint,3,opt,name=total_result,json=totalResult,proto3" json:"total_result,omitempty"`
|
|
Result []*UserGrantView `protobuf:"bytes,4,rep,name=result,proto3" json:"result,omitempty"`
|
|
ProcessedSequence uint64 `protobuf:"varint,5,opt,name=processed_sequence,json=processedSequence,proto3" json:"processed_sequence,omitempty"`
|
|
ViewTimestamp *timestamp.Timestamp `protobuf:"bytes,6,opt,name=view_timestamp,json=viewTimestamp,proto3" json:"view_timestamp,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *UserGrantSearchResponse) Reset() { *m = UserGrantSearchResponse{} }
|
|
func (m *UserGrantSearchResponse) String() string { return proto.CompactTextString(m) }
|
|
func (*UserGrantSearchResponse) ProtoMessage() {}
|
|
func (*UserGrantSearchResponse) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{28}
|
|
}
|
|
|
|
func (m *UserGrantSearchResponse) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_UserGrantSearchResponse.Unmarshal(m, b)
|
|
}
|
|
func (m *UserGrantSearchResponse) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_UserGrantSearchResponse.Marshal(b, m, deterministic)
|
|
}
|
|
func (m *UserGrantSearchResponse) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_UserGrantSearchResponse.Merge(m, src)
|
|
}
|
|
func (m *UserGrantSearchResponse) XXX_Size() int {
|
|
return xxx_messageInfo_UserGrantSearchResponse.Size(m)
|
|
}
|
|
func (m *UserGrantSearchResponse) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_UserGrantSearchResponse.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_UserGrantSearchResponse proto.InternalMessageInfo
|
|
|
|
func (m *UserGrantSearchResponse) GetOffset() uint64 {
|
|
if m != nil {
|
|
return m.Offset
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (m *UserGrantSearchResponse) GetLimit() uint64 {
|
|
if m != nil {
|
|
return m.Limit
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (m *UserGrantSearchResponse) GetTotalResult() uint64 {
|
|
if m != nil {
|
|
return m.TotalResult
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (m *UserGrantSearchResponse) GetResult() []*UserGrantView {
|
|
if m != nil {
|
|
return m.Result
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (m *UserGrantSearchResponse) GetProcessedSequence() uint64 {
|
|
if m != nil {
|
|
return m.ProcessedSequence
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (m *UserGrantSearchResponse) GetViewTimestamp() *timestamp.Timestamp {
|
|
if m != nil {
|
|
return m.ViewTimestamp
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type UserGrantView struct {
|
|
OrgId string `protobuf:"bytes,1,opt,name=OrgId,proto3" json:"OrgId,omitempty"`
|
|
ProjectId string `protobuf:"bytes,2,opt,name=ProjectId,proto3" json:"ProjectId,omitempty"`
|
|
UserId string `protobuf:"bytes,3,opt,name=UserId,proto3" json:"UserId,omitempty"`
|
|
Roles []string `protobuf:"bytes,4,rep,name=Roles,proto3" json:"Roles,omitempty"`
|
|
OrgName string `protobuf:"bytes,5,opt,name=OrgName,proto3" json:"OrgName,omitempty"`
|
|
GrantId string `protobuf:"bytes,6,opt,name=GrantId,proto3" json:"GrantId,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *UserGrantView) Reset() { *m = UserGrantView{} }
|
|
func (m *UserGrantView) String() string { return proto.CompactTextString(m) }
|
|
func (*UserGrantView) ProtoMessage() {}
|
|
func (*UserGrantView) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{29}
|
|
}
|
|
|
|
func (m *UserGrantView) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_UserGrantView.Unmarshal(m, b)
|
|
}
|
|
func (m *UserGrantView) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_UserGrantView.Marshal(b, m, deterministic)
|
|
}
|
|
func (m *UserGrantView) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_UserGrantView.Merge(m, src)
|
|
}
|
|
func (m *UserGrantView) XXX_Size() int {
|
|
return xxx_messageInfo_UserGrantView.Size(m)
|
|
}
|
|
func (m *UserGrantView) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_UserGrantView.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_UserGrantView proto.InternalMessageInfo
|
|
|
|
func (m *UserGrantView) GetOrgId() string {
|
|
if m != nil {
|
|
return m.OrgId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UserGrantView) GetProjectId() string {
|
|
if m != nil {
|
|
return m.ProjectId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UserGrantView) GetUserId() string {
|
|
if m != nil {
|
|
return m.UserId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UserGrantView) GetRoles() []string {
|
|
if m != nil {
|
|
return m.Roles
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (m *UserGrantView) GetOrgName() string {
|
|
if m != nil {
|
|
return m.OrgName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UserGrantView) GetGrantId() string {
|
|
if m != nil {
|
|
return m.GrantId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type MyProjectOrgSearchRequest struct {
|
|
Offset uint64 `protobuf:"varint,1,opt,name=offset,proto3" json:"offset,omitempty"`
|
|
Limit uint64 `protobuf:"varint,2,opt,name=limit,proto3" json:"limit,omitempty"`
|
|
Asc bool `protobuf:"varint,4,opt,name=asc,proto3" json:"asc,omitempty"`
|
|
Queries []*MyProjectOrgSearchQuery `protobuf:"bytes,5,rep,name=queries,proto3" json:"queries,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *MyProjectOrgSearchRequest) Reset() { *m = MyProjectOrgSearchRequest{} }
|
|
func (m *MyProjectOrgSearchRequest) String() string { return proto.CompactTextString(m) }
|
|
func (*MyProjectOrgSearchRequest) ProtoMessage() {}
|
|
func (*MyProjectOrgSearchRequest) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{30}
|
|
}
|
|
|
|
func (m *MyProjectOrgSearchRequest) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_MyProjectOrgSearchRequest.Unmarshal(m, b)
|
|
}
|
|
func (m *MyProjectOrgSearchRequest) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_MyProjectOrgSearchRequest.Marshal(b, m, deterministic)
|
|
}
|
|
func (m *MyProjectOrgSearchRequest) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_MyProjectOrgSearchRequest.Merge(m, src)
|
|
}
|
|
func (m *MyProjectOrgSearchRequest) XXX_Size() int {
|
|
return xxx_messageInfo_MyProjectOrgSearchRequest.Size(m)
|
|
}
|
|
func (m *MyProjectOrgSearchRequest) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_MyProjectOrgSearchRequest.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_MyProjectOrgSearchRequest proto.InternalMessageInfo
|
|
|
|
func (m *MyProjectOrgSearchRequest) GetOffset() uint64 {
|
|
if m != nil {
|
|
return m.Offset
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (m *MyProjectOrgSearchRequest) GetLimit() uint64 {
|
|
if m != nil {
|
|
return m.Limit
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (m *MyProjectOrgSearchRequest) GetAsc() bool {
|
|
if m != nil {
|
|
return m.Asc
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (m *MyProjectOrgSearchRequest) GetQueries() []*MyProjectOrgSearchQuery {
|
|
if m != nil {
|
|
return m.Queries
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type MyProjectOrgSearchQuery struct {
|
|
Key MyProjectOrgSearchKey `protobuf:"varint,1,opt,name=key,proto3,enum=caos.zitadel.auth.api.v1.MyProjectOrgSearchKey" json:"key,omitempty"`
|
|
Method SearchMethod `protobuf:"varint,2,opt,name=method,proto3,enum=caos.zitadel.auth.api.v1.SearchMethod" json:"method,omitempty"`
|
|
Value string `protobuf:"bytes,3,opt,name=value,proto3" json:"value,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *MyProjectOrgSearchQuery) Reset() { *m = MyProjectOrgSearchQuery{} }
|
|
func (m *MyProjectOrgSearchQuery) String() string { return proto.CompactTextString(m) }
|
|
func (*MyProjectOrgSearchQuery) ProtoMessage() {}
|
|
func (*MyProjectOrgSearchQuery) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{31}
|
|
}
|
|
|
|
func (m *MyProjectOrgSearchQuery) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_MyProjectOrgSearchQuery.Unmarshal(m, b)
|
|
}
|
|
func (m *MyProjectOrgSearchQuery) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_MyProjectOrgSearchQuery.Marshal(b, m, deterministic)
|
|
}
|
|
func (m *MyProjectOrgSearchQuery) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_MyProjectOrgSearchQuery.Merge(m, src)
|
|
}
|
|
func (m *MyProjectOrgSearchQuery) XXX_Size() int {
|
|
return xxx_messageInfo_MyProjectOrgSearchQuery.Size(m)
|
|
}
|
|
func (m *MyProjectOrgSearchQuery) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_MyProjectOrgSearchQuery.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_MyProjectOrgSearchQuery proto.InternalMessageInfo
|
|
|
|
func (m *MyProjectOrgSearchQuery) GetKey() MyProjectOrgSearchKey {
|
|
if m != nil {
|
|
return m.Key
|
|
}
|
|
return MyProjectOrgSearchKey_MYPROJECTORGSEARCHKEY_UNSPECIFIED
|
|
}
|
|
|
|
func (m *MyProjectOrgSearchQuery) GetMethod() SearchMethod {
|
|
if m != nil {
|
|
return m.Method
|
|
}
|
|
return SearchMethod_SEARCHMETHOD_EQUALS
|
|
}
|
|
|
|
func (m *MyProjectOrgSearchQuery) GetValue() string {
|
|
if m != nil {
|
|
return m.Value
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type MyProjectOrgSearchResponse struct {
|
|
Offset uint64 `protobuf:"varint,1,opt,name=offset,proto3" json:"offset,omitempty"`
|
|
Limit uint64 `protobuf:"varint,2,opt,name=limit,proto3" json:"limit,omitempty"`
|
|
TotalResult uint64 `protobuf:"varint,3,opt,name=total_result,json=totalResult,proto3" json:"total_result,omitempty"`
|
|
Result []*Org `protobuf:"bytes,4,rep,name=result,proto3" json:"result,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *MyProjectOrgSearchResponse) Reset() { *m = MyProjectOrgSearchResponse{} }
|
|
func (m *MyProjectOrgSearchResponse) String() string { return proto.CompactTextString(m) }
|
|
func (*MyProjectOrgSearchResponse) ProtoMessage() {}
|
|
func (*MyProjectOrgSearchResponse) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{32}
|
|
}
|
|
|
|
func (m *MyProjectOrgSearchResponse) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_MyProjectOrgSearchResponse.Unmarshal(m, b)
|
|
}
|
|
func (m *MyProjectOrgSearchResponse) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_MyProjectOrgSearchResponse.Marshal(b, m, deterministic)
|
|
}
|
|
func (m *MyProjectOrgSearchResponse) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_MyProjectOrgSearchResponse.Merge(m, src)
|
|
}
|
|
func (m *MyProjectOrgSearchResponse) XXX_Size() int {
|
|
return xxx_messageInfo_MyProjectOrgSearchResponse.Size(m)
|
|
}
|
|
func (m *MyProjectOrgSearchResponse) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_MyProjectOrgSearchResponse.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_MyProjectOrgSearchResponse proto.InternalMessageInfo
|
|
|
|
func (m *MyProjectOrgSearchResponse) GetOffset() uint64 {
|
|
if m != nil {
|
|
return m.Offset
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (m *MyProjectOrgSearchResponse) GetLimit() uint64 {
|
|
if m != nil {
|
|
return m.Limit
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (m *MyProjectOrgSearchResponse) GetTotalResult() uint64 {
|
|
if m != nil {
|
|
return m.TotalResult
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (m *MyProjectOrgSearchResponse) GetResult() []*Org {
|
|
if m != nil {
|
|
return m.Result
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type Org struct {
|
|
Id string `protobuf:"bytes,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
Name string `protobuf:"bytes,2,opt,name=name,proto3" json:"name,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *Org) Reset() { *m = Org{} }
|
|
func (m *Org) String() string { return proto.CompactTextString(m) }
|
|
func (*Org) ProtoMessage() {}
|
|
func (*Org) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{33}
|
|
}
|
|
|
|
func (m *Org) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_Org.Unmarshal(m, b)
|
|
}
|
|
func (m *Org) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_Org.Marshal(b, m, deterministic)
|
|
}
|
|
func (m *Org) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_Org.Merge(m, src)
|
|
}
|
|
func (m *Org) XXX_Size() int {
|
|
return xxx_messageInfo_Org.Size(m)
|
|
}
|
|
func (m *Org) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_Org.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_Org proto.InternalMessageInfo
|
|
|
|
func (m *Org) GetId() string {
|
|
if m != nil {
|
|
return m.Id
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *Org) GetName() string {
|
|
if m != nil {
|
|
return m.Name
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type MyPermissions struct {
|
|
Permissions []string `protobuf:"bytes,1,rep,name=permissions,proto3" json:"permissions,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *MyPermissions) Reset() { *m = MyPermissions{} }
|
|
func (m *MyPermissions) String() string { return proto.CompactTextString(m) }
|
|
func (*MyPermissions) ProtoMessage() {}
|
|
func (*MyPermissions) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{34}
|
|
}
|
|
|
|
func (m *MyPermissions) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_MyPermissions.Unmarshal(m, b)
|
|
}
|
|
func (m *MyPermissions) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_MyPermissions.Marshal(b, m, deterministic)
|
|
}
|
|
func (m *MyPermissions) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_MyPermissions.Merge(m, src)
|
|
}
|
|
func (m *MyPermissions) XXX_Size() int {
|
|
return xxx_messageInfo_MyPermissions.Size(m)
|
|
}
|
|
func (m *MyPermissions) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_MyPermissions.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_MyPermissions proto.InternalMessageInfo
|
|
|
|
func (m *MyPermissions) GetPermissions() []string {
|
|
if m != nil {
|
|
return m.Permissions
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type ChangesRequest struct {
|
|
Limit uint64 `protobuf:"varint,1,opt,name=limit,proto3" json:"limit,omitempty"`
|
|
SequenceOffset uint64 `protobuf:"varint,2,opt,name=sequence_offset,json=sequenceOffset,proto3" json:"sequence_offset,omitempty"`
|
|
Asc bool `protobuf:"varint,3,opt,name=asc,proto3" json:"asc,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *ChangesRequest) Reset() { *m = ChangesRequest{} }
|
|
func (m *ChangesRequest) String() string { return proto.CompactTextString(m) }
|
|
func (*ChangesRequest) ProtoMessage() {}
|
|
func (*ChangesRequest) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{35}
|
|
}
|
|
|
|
func (m *ChangesRequest) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_ChangesRequest.Unmarshal(m, b)
|
|
}
|
|
func (m *ChangesRequest) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_ChangesRequest.Marshal(b, m, deterministic)
|
|
}
|
|
func (m *ChangesRequest) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_ChangesRequest.Merge(m, src)
|
|
}
|
|
func (m *ChangesRequest) XXX_Size() int {
|
|
return xxx_messageInfo_ChangesRequest.Size(m)
|
|
}
|
|
func (m *ChangesRequest) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_ChangesRequest.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_ChangesRequest proto.InternalMessageInfo
|
|
|
|
func (m *ChangesRequest) GetLimit() uint64 {
|
|
if m != nil {
|
|
return m.Limit
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (m *ChangesRequest) GetSequenceOffset() uint64 {
|
|
if m != nil {
|
|
return m.SequenceOffset
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (m *ChangesRequest) GetAsc() bool {
|
|
if m != nil {
|
|
return m.Asc
|
|
}
|
|
return false
|
|
}
|
|
|
|
type Changes struct {
|
|
Changes []*Change `protobuf:"bytes,1,rep,name=changes,proto3" json:"changes,omitempty"`
|
|
Offset uint64 `protobuf:"varint,2,opt,name=offset,proto3" json:"offset,omitempty"`
|
|
Limit uint64 `protobuf:"varint,3,opt,name=limit,proto3" json:"limit,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *Changes) Reset() { *m = Changes{} }
|
|
func (m *Changes) String() string { return proto.CompactTextString(m) }
|
|
func (*Changes) ProtoMessage() {}
|
|
func (*Changes) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{36}
|
|
}
|
|
|
|
func (m *Changes) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_Changes.Unmarshal(m, b)
|
|
}
|
|
func (m *Changes) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_Changes.Marshal(b, m, deterministic)
|
|
}
|
|
func (m *Changes) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_Changes.Merge(m, src)
|
|
}
|
|
func (m *Changes) XXX_Size() int {
|
|
return xxx_messageInfo_Changes.Size(m)
|
|
}
|
|
func (m *Changes) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_Changes.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_Changes proto.InternalMessageInfo
|
|
|
|
func (m *Changes) GetChanges() []*Change {
|
|
if m != nil {
|
|
return m.Changes
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (m *Changes) GetOffset() uint64 {
|
|
if m != nil {
|
|
return m.Offset
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (m *Changes) GetLimit() uint64 {
|
|
if m != nil {
|
|
return m.Limit
|
|
}
|
|
return 0
|
|
}
|
|
|
|
type Change struct {
|
|
ChangeDate *timestamp.Timestamp `protobuf:"bytes,1,opt,name=change_date,json=changeDate,proto3" json:"change_date,omitempty"`
|
|
EventType *message.LocalizedMessage `protobuf:"bytes,2,opt,name=event_type,json=eventType,proto3" json:"event_type,omitempty"`
|
|
Sequence uint64 `protobuf:"varint,3,opt,name=sequence,proto3" json:"sequence,omitempty"`
|
|
EditorId string `protobuf:"bytes,4,opt,name=editor_id,json=editorId,proto3" json:"editor_id,omitempty"`
|
|
Editor string `protobuf:"bytes,5,opt,name=editor,proto3" json:"editor,omitempty"`
|
|
Data *_struct.Struct `protobuf:"bytes,6,opt,name=data,proto3" json:"data,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *Change) Reset() { *m = Change{} }
|
|
func (m *Change) String() string { return proto.CompactTextString(m) }
|
|
func (*Change) ProtoMessage() {}
|
|
func (*Change) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{37}
|
|
}
|
|
|
|
func (m *Change) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_Change.Unmarshal(m, b)
|
|
}
|
|
func (m *Change) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_Change.Marshal(b, m, deterministic)
|
|
}
|
|
func (m *Change) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_Change.Merge(m, src)
|
|
}
|
|
func (m *Change) XXX_Size() int {
|
|
return xxx_messageInfo_Change.Size(m)
|
|
}
|
|
func (m *Change) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_Change.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_Change proto.InternalMessageInfo
|
|
|
|
func (m *Change) GetChangeDate() *timestamp.Timestamp {
|
|
if m != nil {
|
|
return m.ChangeDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (m *Change) GetEventType() *message.LocalizedMessage {
|
|
if m != nil {
|
|
return m.EventType
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (m *Change) GetSequence() uint64 {
|
|
if m != nil {
|
|
return m.Sequence
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (m *Change) GetEditorId() string {
|
|
if m != nil {
|
|
return m.EditorId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *Change) GetEditor() string {
|
|
if m != nil {
|
|
return m.Editor
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *Change) GetData() *_struct.Struct {
|
|
if m != nil {
|
|
return m.Data
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type PasswordComplexityPolicy struct {
|
|
Id string `protobuf:"bytes,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
Description string `protobuf:"bytes,2,opt,name=description,proto3" json:"description,omitempty"`
|
|
CreationDate *timestamp.Timestamp `protobuf:"bytes,3,opt,name=creation_date,json=creationDate,proto3" json:"creation_date,omitempty"`
|
|
ChangeDate *timestamp.Timestamp `protobuf:"bytes,4,opt,name=change_date,json=changeDate,proto3" json:"change_date,omitempty"`
|
|
MinLength uint64 `protobuf:"varint,5,opt,name=min_length,json=minLength,proto3" json:"min_length,omitempty"`
|
|
HasLowercase bool `protobuf:"varint,6,opt,name=has_lowercase,json=hasLowercase,proto3" json:"has_lowercase,omitempty"`
|
|
HasUppercase bool `protobuf:"varint,7,opt,name=has_uppercase,json=hasUppercase,proto3" json:"has_uppercase,omitempty"`
|
|
HasNumber bool `protobuf:"varint,8,opt,name=has_number,json=hasNumber,proto3" json:"has_number,omitempty"`
|
|
HasSymbol bool `protobuf:"varint,9,opt,name=has_symbol,json=hasSymbol,proto3" json:"has_symbol,omitempty"`
|
|
Sequence uint64 `protobuf:"varint,10,opt,name=sequence,proto3" json:"sequence,omitempty"`
|
|
IsDefault bool `protobuf:"varint,11,opt,name=is_default,json=isDefault,proto3" json:"is_default,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *PasswordComplexityPolicy) Reset() { *m = PasswordComplexityPolicy{} }
|
|
func (m *PasswordComplexityPolicy) String() string { return proto.CompactTextString(m) }
|
|
func (*PasswordComplexityPolicy) ProtoMessage() {}
|
|
func (*PasswordComplexityPolicy) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{38}
|
|
}
|
|
|
|
func (m *PasswordComplexityPolicy) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_PasswordComplexityPolicy.Unmarshal(m, b)
|
|
}
|
|
func (m *PasswordComplexityPolicy) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_PasswordComplexityPolicy.Marshal(b, m, deterministic)
|
|
}
|
|
func (m *PasswordComplexityPolicy) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_PasswordComplexityPolicy.Merge(m, src)
|
|
}
|
|
func (m *PasswordComplexityPolicy) XXX_Size() int {
|
|
return xxx_messageInfo_PasswordComplexityPolicy.Size(m)
|
|
}
|
|
func (m *PasswordComplexityPolicy) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_PasswordComplexityPolicy.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_PasswordComplexityPolicy proto.InternalMessageInfo
|
|
|
|
func (m *PasswordComplexityPolicy) GetId() string {
|
|
if m != nil {
|
|
return m.Id
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *PasswordComplexityPolicy) GetDescription() string {
|
|
if m != nil {
|
|
return m.Description
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *PasswordComplexityPolicy) GetCreationDate() *timestamp.Timestamp {
|
|
if m != nil {
|
|
return m.CreationDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (m *PasswordComplexityPolicy) GetChangeDate() *timestamp.Timestamp {
|
|
if m != nil {
|
|
return m.ChangeDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (m *PasswordComplexityPolicy) GetMinLength() uint64 {
|
|
if m != nil {
|
|
return m.MinLength
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (m *PasswordComplexityPolicy) GetHasLowercase() bool {
|
|
if m != nil {
|
|
return m.HasLowercase
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (m *PasswordComplexityPolicy) GetHasUppercase() bool {
|
|
if m != nil {
|
|
return m.HasUppercase
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (m *PasswordComplexityPolicy) GetHasNumber() bool {
|
|
if m != nil {
|
|
return m.HasNumber
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (m *PasswordComplexityPolicy) GetHasSymbol() bool {
|
|
if m != nil {
|
|
return m.HasSymbol
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (m *PasswordComplexityPolicy) GetSequence() uint64 {
|
|
if m != nil {
|
|
return m.Sequence
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (m *PasswordComplexityPolicy) GetIsDefault() bool {
|
|
if m != nil {
|
|
return m.IsDefault
|
|
}
|
|
return false
|
|
}
|
|
|
|
type ExternalIDPResponse struct {
|
|
IdpConfigId string `protobuf:"bytes,1,opt,name=idp_config_id,json=idpConfigId,proto3" json:"idp_config_id,omitempty"`
|
|
UserId string `protobuf:"bytes,2,opt,name=user_id,json=userId,proto3" json:"user_id,omitempty"`
|
|
DisplayName string `protobuf:"bytes,3,opt,name=display_name,json=displayName,proto3" json:"display_name,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *ExternalIDPResponse) Reset() { *m = ExternalIDPResponse{} }
|
|
func (m *ExternalIDPResponse) String() string { return proto.CompactTextString(m) }
|
|
func (*ExternalIDPResponse) ProtoMessage() {}
|
|
func (*ExternalIDPResponse) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{39}
|
|
}
|
|
|
|
func (m *ExternalIDPResponse) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_ExternalIDPResponse.Unmarshal(m, b)
|
|
}
|
|
func (m *ExternalIDPResponse) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_ExternalIDPResponse.Marshal(b, m, deterministic)
|
|
}
|
|
func (m *ExternalIDPResponse) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_ExternalIDPResponse.Merge(m, src)
|
|
}
|
|
func (m *ExternalIDPResponse) XXX_Size() int {
|
|
return xxx_messageInfo_ExternalIDPResponse.Size(m)
|
|
}
|
|
func (m *ExternalIDPResponse) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_ExternalIDPResponse.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_ExternalIDPResponse proto.InternalMessageInfo
|
|
|
|
func (m *ExternalIDPResponse) GetIdpConfigId() string {
|
|
if m != nil {
|
|
return m.IdpConfigId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *ExternalIDPResponse) GetUserId() string {
|
|
if m != nil {
|
|
return m.UserId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *ExternalIDPResponse) GetDisplayName() string {
|
|
if m != nil {
|
|
return m.DisplayName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type ExternalIDPRemoveRequest struct {
|
|
IdpConfigId string `protobuf:"bytes,1,opt,name=idp_config_id,json=idpConfigId,proto3" json:"idp_config_id,omitempty"`
|
|
ExternalUserId string `protobuf:"bytes,2,opt,name=external_user_id,json=externalUserId,proto3" json:"external_user_id,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *ExternalIDPRemoveRequest) Reset() { *m = ExternalIDPRemoveRequest{} }
|
|
func (m *ExternalIDPRemoveRequest) String() string { return proto.CompactTextString(m) }
|
|
func (*ExternalIDPRemoveRequest) ProtoMessage() {}
|
|
func (*ExternalIDPRemoveRequest) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{40}
|
|
}
|
|
|
|
func (m *ExternalIDPRemoveRequest) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_ExternalIDPRemoveRequest.Unmarshal(m, b)
|
|
}
|
|
func (m *ExternalIDPRemoveRequest) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_ExternalIDPRemoveRequest.Marshal(b, m, deterministic)
|
|
}
|
|
func (m *ExternalIDPRemoveRequest) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_ExternalIDPRemoveRequest.Merge(m, src)
|
|
}
|
|
func (m *ExternalIDPRemoveRequest) XXX_Size() int {
|
|
return xxx_messageInfo_ExternalIDPRemoveRequest.Size(m)
|
|
}
|
|
func (m *ExternalIDPRemoveRequest) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_ExternalIDPRemoveRequest.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_ExternalIDPRemoveRequest proto.InternalMessageInfo
|
|
|
|
func (m *ExternalIDPRemoveRequest) GetIdpConfigId() string {
|
|
if m != nil {
|
|
return m.IdpConfigId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *ExternalIDPRemoveRequest) GetExternalUserId() string {
|
|
if m != nil {
|
|
return m.ExternalUserId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type ExternalIDPSearchRequest struct {
|
|
Offset uint64 `protobuf:"varint,1,opt,name=offset,proto3" json:"offset,omitempty"`
|
|
Limit uint64 `protobuf:"varint,2,opt,name=limit,proto3" json:"limit,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *ExternalIDPSearchRequest) Reset() { *m = ExternalIDPSearchRequest{} }
|
|
func (m *ExternalIDPSearchRequest) String() string { return proto.CompactTextString(m) }
|
|
func (*ExternalIDPSearchRequest) ProtoMessage() {}
|
|
func (*ExternalIDPSearchRequest) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{41}
|
|
}
|
|
|
|
func (m *ExternalIDPSearchRequest) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_ExternalIDPSearchRequest.Unmarshal(m, b)
|
|
}
|
|
func (m *ExternalIDPSearchRequest) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_ExternalIDPSearchRequest.Marshal(b, m, deterministic)
|
|
}
|
|
func (m *ExternalIDPSearchRequest) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_ExternalIDPSearchRequest.Merge(m, src)
|
|
}
|
|
func (m *ExternalIDPSearchRequest) XXX_Size() int {
|
|
return xxx_messageInfo_ExternalIDPSearchRequest.Size(m)
|
|
}
|
|
func (m *ExternalIDPSearchRequest) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_ExternalIDPSearchRequest.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_ExternalIDPSearchRequest proto.InternalMessageInfo
|
|
|
|
func (m *ExternalIDPSearchRequest) GetOffset() uint64 {
|
|
if m != nil {
|
|
return m.Offset
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (m *ExternalIDPSearchRequest) GetLimit() uint64 {
|
|
if m != nil {
|
|
return m.Limit
|
|
}
|
|
return 0
|
|
}
|
|
|
|
type ExternalIDPSearchResponse struct {
|
|
Offset uint64 `protobuf:"varint,1,opt,name=offset,proto3" json:"offset,omitempty"`
|
|
Limit uint64 `protobuf:"varint,2,opt,name=limit,proto3" json:"limit,omitempty"`
|
|
TotalResult uint64 `protobuf:"varint,3,opt,name=total_result,json=totalResult,proto3" json:"total_result,omitempty"`
|
|
Result []*ExternalIDPView `protobuf:"bytes,4,rep,name=result,proto3" json:"result,omitempty"`
|
|
ProcessedSequence uint64 `protobuf:"varint,5,opt,name=processed_sequence,json=processedSequence,proto3" json:"processed_sequence,omitempty"`
|
|
ViewTimestamp *timestamp.Timestamp `protobuf:"bytes,6,opt,name=view_timestamp,json=viewTimestamp,proto3" json:"view_timestamp,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *ExternalIDPSearchResponse) Reset() { *m = ExternalIDPSearchResponse{} }
|
|
func (m *ExternalIDPSearchResponse) String() string { return proto.CompactTextString(m) }
|
|
func (*ExternalIDPSearchResponse) ProtoMessage() {}
|
|
func (*ExternalIDPSearchResponse) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{42}
|
|
}
|
|
|
|
func (m *ExternalIDPSearchResponse) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_ExternalIDPSearchResponse.Unmarshal(m, b)
|
|
}
|
|
func (m *ExternalIDPSearchResponse) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_ExternalIDPSearchResponse.Marshal(b, m, deterministic)
|
|
}
|
|
func (m *ExternalIDPSearchResponse) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_ExternalIDPSearchResponse.Merge(m, src)
|
|
}
|
|
func (m *ExternalIDPSearchResponse) XXX_Size() int {
|
|
return xxx_messageInfo_ExternalIDPSearchResponse.Size(m)
|
|
}
|
|
func (m *ExternalIDPSearchResponse) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_ExternalIDPSearchResponse.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_ExternalIDPSearchResponse proto.InternalMessageInfo
|
|
|
|
func (m *ExternalIDPSearchResponse) GetOffset() uint64 {
|
|
if m != nil {
|
|
return m.Offset
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (m *ExternalIDPSearchResponse) GetLimit() uint64 {
|
|
if m != nil {
|
|
return m.Limit
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (m *ExternalIDPSearchResponse) GetTotalResult() uint64 {
|
|
if m != nil {
|
|
return m.TotalResult
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (m *ExternalIDPSearchResponse) GetResult() []*ExternalIDPView {
|
|
if m != nil {
|
|
return m.Result
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (m *ExternalIDPSearchResponse) GetProcessedSequence() uint64 {
|
|
if m != nil {
|
|
return m.ProcessedSequence
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (m *ExternalIDPSearchResponse) GetViewTimestamp() *timestamp.Timestamp {
|
|
if m != nil {
|
|
return m.ViewTimestamp
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type ExternalIDPView struct {
|
|
UserId string `protobuf:"bytes,1,opt,name=user_id,json=userId,proto3" json:"user_id,omitempty"`
|
|
IdpConfigId string `protobuf:"bytes,2,opt,name=idp_config_id,json=idpConfigId,proto3" json:"idp_config_id,omitempty"`
|
|
ExternalUserId string `protobuf:"bytes,3,opt,name=external_user_id,json=externalUserId,proto3" json:"external_user_id,omitempty"`
|
|
IdpName string `protobuf:"bytes,4,opt,name=idp_name,json=idpName,proto3" json:"idp_name,omitempty"`
|
|
ExternalUserDisplayName string `protobuf:"bytes,5,opt,name=external_user_display_name,json=externalUserDisplayName,proto3" json:"external_user_display_name,omitempty"`
|
|
CreationDate *timestamp.Timestamp `protobuf:"bytes,6,opt,name=creation_date,json=creationDate,proto3" json:"creation_date,omitempty"`
|
|
ChangeDate *timestamp.Timestamp `protobuf:"bytes,7,opt,name=change_date,json=changeDate,proto3" json:"change_date,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *ExternalIDPView) Reset() { *m = ExternalIDPView{} }
|
|
func (m *ExternalIDPView) String() string { return proto.CompactTextString(m) }
|
|
func (*ExternalIDPView) ProtoMessage() {}
|
|
func (*ExternalIDPView) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_8bbd6f3875b0e874, []int{43}
|
|
}
|
|
|
|
func (m *ExternalIDPView) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_ExternalIDPView.Unmarshal(m, b)
|
|
}
|
|
func (m *ExternalIDPView) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_ExternalIDPView.Marshal(b, m, deterministic)
|
|
}
|
|
func (m *ExternalIDPView) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_ExternalIDPView.Merge(m, src)
|
|
}
|
|
func (m *ExternalIDPView) XXX_Size() int {
|
|
return xxx_messageInfo_ExternalIDPView.Size(m)
|
|
}
|
|
func (m *ExternalIDPView) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_ExternalIDPView.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_ExternalIDPView proto.InternalMessageInfo
|
|
|
|
func (m *ExternalIDPView) GetUserId() string {
|
|
if m != nil {
|
|
return m.UserId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *ExternalIDPView) GetIdpConfigId() string {
|
|
if m != nil {
|
|
return m.IdpConfigId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *ExternalIDPView) GetExternalUserId() string {
|
|
if m != nil {
|
|
return m.ExternalUserId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *ExternalIDPView) GetIdpName() string {
|
|
if m != nil {
|
|
return m.IdpName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *ExternalIDPView) GetExternalUserDisplayName() string {
|
|
if m != nil {
|
|
return m.ExternalUserDisplayName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *ExternalIDPView) GetCreationDate() *timestamp.Timestamp {
|
|
if m != nil {
|
|
return m.CreationDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (m *ExternalIDPView) GetChangeDate() *timestamp.Timestamp {
|
|
if m != nil {
|
|
return m.ChangeDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func init() {
|
|
proto.RegisterEnum("caos.zitadel.auth.api.v1.UserSessionState", UserSessionState_name, UserSessionState_value)
|
|
proto.RegisterEnum("caos.zitadel.auth.api.v1.MachineKeyType", MachineKeyType_name, MachineKeyType_value)
|
|
proto.RegisterEnum("caos.zitadel.auth.api.v1.UserState", UserState_name, UserState_value)
|
|
proto.RegisterEnum("caos.zitadel.auth.api.v1.Gender", Gender_name, Gender_value)
|
|
proto.RegisterEnum("caos.zitadel.auth.api.v1.MfaType", MfaType_name, MfaType_value)
|
|
proto.RegisterEnum("caos.zitadel.auth.api.v1.MFAState", MFAState_name, MFAState_value)
|
|
proto.RegisterEnum("caos.zitadel.auth.api.v1.UserGrantSearchKey", UserGrantSearchKey_name, UserGrantSearchKey_value)
|
|
proto.RegisterEnum("caos.zitadel.auth.api.v1.MyProjectOrgSearchKey", MyProjectOrgSearchKey_name, MyProjectOrgSearchKey_value)
|
|
proto.RegisterEnum("caos.zitadel.auth.api.v1.SearchMethod", SearchMethod_name, SearchMethod_value)
|
|
proto.RegisterType((*UserSessionViews)(nil), "caos.zitadel.auth.api.v1.UserSessionViews")
|
|
proto.RegisterType((*UserSessionView)(nil), "caos.zitadel.auth.api.v1.UserSessionView")
|
|
proto.RegisterType((*UserView)(nil), "caos.zitadel.auth.api.v1.UserView")
|
|
proto.RegisterType((*MachineView)(nil), "caos.zitadel.auth.api.v1.MachineView")
|
|
proto.RegisterType((*MachineKeyView)(nil), "caos.zitadel.auth.api.v1.MachineKeyView")
|
|
proto.RegisterType((*HumanView)(nil), "caos.zitadel.auth.api.v1.HumanView")
|
|
proto.RegisterType((*UserProfile)(nil), "caos.zitadel.auth.api.v1.UserProfile")
|
|
proto.RegisterType((*UserProfileView)(nil), "caos.zitadel.auth.api.v1.UserProfileView")
|
|
proto.RegisterType((*UpdateUserProfileRequest)(nil), "caos.zitadel.auth.api.v1.UpdateUserProfileRequest")
|
|
proto.RegisterType((*ChangeUserNameRequest)(nil), "caos.zitadel.auth.api.v1.ChangeUserNameRequest")
|
|
proto.RegisterType((*UserEmail)(nil), "caos.zitadel.auth.api.v1.UserEmail")
|
|
proto.RegisterType((*UserEmailView)(nil), "caos.zitadel.auth.api.v1.UserEmailView")
|
|
proto.RegisterType((*VerifyMyUserEmailRequest)(nil), "caos.zitadel.auth.api.v1.VerifyMyUserEmailRequest")
|
|
proto.RegisterType((*UpdateUserEmailRequest)(nil), "caos.zitadel.auth.api.v1.UpdateUserEmailRequest")
|
|
proto.RegisterType((*UserPhone)(nil), "caos.zitadel.auth.api.v1.UserPhone")
|
|
proto.RegisterType((*UserPhoneView)(nil), "caos.zitadel.auth.api.v1.UserPhoneView")
|
|
proto.RegisterType((*UpdateUserPhoneRequest)(nil), "caos.zitadel.auth.api.v1.UpdateUserPhoneRequest")
|
|
proto.RegisterType((*VerifyUserPhoneRequest)(nil), "caos.zitadel.auth.api.v1.VerifyUserPhoneRequest")
|
|
proto.RegisterType((*UserAddress)(nil), "caos.zitadel.auth.api.v1.UserAddress")
|
|
proto.RegisterType((*UserAddressView)(nil), "caos.zitadel.auth.api.v1.UserAddressView")
|
|
proto.RegisterType((*UpdateUserAddressRequest)(nil), "caos.zitadel.auth.api.v1.UpdateUserAddressRequest")
|
|
proto.RegisterType((*PasswordChange)(nil), "caos.zitadel.auth.api.v1.PasswordChange")
|
|
proto.RegisterType((*VerifyMfaOtp)(nil), "caos.zitadel.auth.api.v1.VerifyMfaOtp")
|
|
proto.RegisterType((*MultiFactors)(nil), "caos.zitadel.auth.api.v1.MultiFactors")
|
|
proto.RegisterType((*MultiFactor)(nil), "caos.zitadel.auth.api.v1.MultiFactor")
|
|
proto.RegisterType((*MfaOtpResponse)(nil), "caos.zitadel.auth.api.v1.MfaOtpResponse")
|
|
proto.RegisterType((*UserGrantSearchRequest)(nil), "caos.zitadel.auth.api.v1.UserGrantSearchRequest")
|
|
proto.RegisterType((*UserGrantSearchQuery)(nil), "caos.zitadel.auth.api.v1.UserGrantSearchQuery")
|
|
proto.RegisterType((*UserGrantSearchResponse)(nil), "caos.zitadel.auth.api.v1.UserGrantSearchResponse")
|
|
proto.RegisterType((*UserGrantView)(nil), "caos.zitadel.auth.api.v1.UserGrantView")
|
|
proto.RegisterType((*MyProjectOrgSearchRequest)(nil), "caos.zitadel.auth.api.v1.MyProjectOrgSearchRequest")
|
|
proto.RegisterType((*MyProjectOrgSearchQuery)(nil), "caos.zitadel.auth.api.v1.MyProjectOrgSearchQuery")
|
|
proto.RegisterType((*MyProjectOrgSearchResponse)(nil), "caos.zitadel.auth.api.v1.MyProjectOrgSearchResponse")
|
|
proto.RegisterType((*Org)(nil), "caos.zitadel.auth.api.v1.Org")
|
|
proto.RegisterType((*MyPermissions)(nil), "caos.zitadel.auth.api.v1.MyPermissions")
|
|
proto.RegisterType((*ChangesRequest)(nil), "caos.zitadel.auth.api.v1.ChangesRequest")
|
|
proto.RegisterType((*Changes)(nil), "caos.zitadel.auth.api.v1.Changes")
|
|
proto.RegisterType((*Change)(nil), "caos.zitadel.auth.api.v1.Change")
|
|
proto.RegisterType((*PasswordComplexityPolicy)(nil), "caos.zitadel.auth.api.v1.PasswordComplexityPolicy")
|
|
proto.RegisterType((*ExternalIDPResponse)(nil), "caos.zitadel.auth.api.v1.ExternalIDPResponse")
|
|
proto.RegisterType((*ExternalIDPRemoveRequest)(nil), "caos.zitadel.auth.api.v1.ExternalIDPRemoveRequest")
|
|
proto.RegisterType((*ExternalIDPSearchRequest)(nil), "caos.zitadel.auth.api.v1.ExternalIDPSearchRequest")
|
|
proto.RegisterType((*ExternalIDPSearchResponse)(nil), "caos.zitadel.auth.api.v1.ExternalIDPSearchResponse")
|
|
proto.RegisterType((*ExternalIDPView)(nil), "caos.zitadel.auth.api.v1.ExternalIDPView")
|
|
}
|
|
|
|
func init() { proto.RegisterFile("auth.proto", fileDescriptor_8bbd6f3875b0e874) }
|
|
|
|
var fileDescriptor_8bbd6f3875b0e874 = []byte{
|
|
// 3922 bytes of a gzipped FileDescriptorProto
|
|
0x1f, 0x8b, 0x08, 0x00, 0x00, 0x00, 0x00, 0x00, 0x02, 0xff, 0xec, 0x5a, 0xdd, 0x6f, 0x23, 0x59,
|
|
0x56, 0xef, 0x2a, 0x3b, 0x89, 0x7d, 0x9c, 0x38, 0xce, 0xed, 0xee, 0xc4, 0xed, 0xf4, 0x47, 0xba,
|
|
0x7a, 0x7a, 0x27, 0xed, 0xed, 0x89, 0xbb, 0x33, 0x3b, 0xd2, 0x74, 0xcf, 0x8a, 0xc5, 0x49, 0xdc,
|
|
0x1d, 0x6f, 0x62, 0x3b, 0x5b, 0x76, 0x7a, 0x99, 0x5d, 0xcd, 0x5a, 0xd5, 0xae, 0x1b, 0xbb, 0x76,
|
|
0xec, 0xaa, 0xda, 0xaa, 0x72, 0x7a, 0x3c, 0xa3, 0xe1, 0x63, 0x10, 0xab, 0x05, 0x69, 0x41, 0x2c,
|
|
0x08, 0x09, 0x01, 0x0f, 0x2b, 0x01, 0xe2, 0x01, 0xc1, 0x03, 0x02, 0x1e, 0x80, 0x77, 0x5e, 0x58,
|
|
0x69, 0x85, 0xc4, 0x1f, 0x80, 0xf8, 0x0f, 0x10, 0x02, 0x9a, 0x17, 0x74, 0x3f, 0xaa, 0x5c, 0x1f,
|
|
0x2e, 0xdb, 0x99, 0x66, 0x76, 0xd0, 0x6a, 0x9f, 0xec, 0x7b, 0xee, 0x39, 0xe7, 0x9e, 0x7b, 0xce,
|
|
0xef, 0xde, 0x7b, 0xee, 0x3d, 0x05, 0xa0, 0x0c, 0x9d, 0xde, 0x8e, 0x69, 0x19, 0x8e, 0x81, 0xf2,
|
|
0x1d, 0xc5, 0xb0, 0x77, 0x3e, 0xd4, 0x1c, 0x45, 0xc5, 0xfd, 0x1d, 0xda, 0xa1, 0x98, 0xda, 0xce,
|
|
0xf9, 0xc3, 0xc2, 0xf5, 0xae, 0x61, 0x74, 0xfb, 0xb8, 0xa4, 0x98, 0x5a, 0x49, 0xd1, 0x75, 0xc3,
|
|
0x51, 0x1c, 0xcd, 0xd0, 0x6d, 0x26, 0x57, 0xd8, 0xe4, 0xbd, 0xb4, 0xf5, 0x7c, 0x78, 0x56, 0xc2,
|
|
0x03, 0xd3, 0x19, 0xf1, 0xce, 0xeb, 0xe1, 0x4e, 0xdb, 0xb1, 0x86, 0x1d, 0x87, 0xf7, 0xde, 0x0a,
|
|
0xf7, 0x3a, 0xda, 0x00, 0xdb, 0x8e, 0x32, 0x30, 0x39, 0xc3, 0xc6, 0xb9, 0xd2, 0xd7, 0x54, 0xc5,
|
|
0xc1, 0x25, 0xf7, 0x0f, 0xef, 0xb8, 0x4f, 0x7f, 0x3a, 0x6f, 0x74, 0xb1, 0xfe, 0x86, 0xfd, 0x42,
|
|
0xe9, 0x76, 0xb1, 0x55, 0x32, 0x4c, 0x6a, 0xd6, 0x04, 0x13, 0xf3, 0x64, 0x36, 0xac, 0xdb, 0xe5,
|
|
0xe2, 0x3d, 0x97, 0xe9, 0x4f, 0x69, 0x80, 0x6d, 0x5b, 0xe9, 0x72, 0xe5, 0xd2, 0x73, 0xc8, 0x9d,
|
|
0xda, 0xd8, 0x6a, 0x62, 0xdb, 0xd6, 0x0c, 0xfd, 0x99, 0x86, 0x5f, 0xd8, 0xa8, 0x0e, 0x2b, 0x43,
|
|
0x1b, 0x5b, 0x6d, 0x9b, 0x11, 0xed, 0xbc, 0xb0, 0x95, 0xd8, 0xce, 0xec, 0xde, 0xdb, 0x89, 0xf3,
|
|
0xda, 0x4e, 0x48, 0x85, 0xbc, 0x3c, 0x1c, 0x13, 0x6c, 0xe9, 0x0f, 0x44, 0x58, 0x0d, 0x71, 0xa0,
|
|
0x2c, 0x88, 0x9a, 0x9a, 0x17, 0xb6, 0x84, 0xed, 0xb4, 0x2c, 0x6a, 0x2a, 0xba, 0x06, 0x29, 0xa5,
|
|
0x8b, 0x75, 0xa7, 0xad, 0xa9, 0x79, 0x91, 0x52, 0x97, 0x68, 0xbb, 0xaa, 0xa2, 0x2a, 0x0b, 0x5d,
|
|
0xdb, 0x76, 0x14, 0x07, 0xe7, 0x13, 0x5b, 0xc2, 0x76, 0x76, 0xb7, 0x38, 0x97, 0x2d, 0x4d, 0x22,
|
|
0x21, 0xa7, 0x49, 0x2f, 0xfd, 0x8b, 0x36, 0x60, 0x89, 0xce, 0x4c, 0x53, 0xf3, 0x49, 0x3a, 0xc8,
|
|
0x22, 0x69, 0x56, 0x55, 0xb4, 0x09, 0x69, 0xda, 0xa1, 0x2b, 0x03, 0x9c, 0x5f, 0xa0, 0x5d, 0x29,
|
|
0x42, 0xa8, 0x2b, 0x03, 0x8c, 0x0a, 0x90, 0xb2, 0xf1, 0x77, 0x86, 0x58, 0xef, 0xe0, 0xfc, 0xe2,
|
|
0x96, 0xb0, 0x9d, 0x94, 0xbd, 0x36, 0xba, 0x01, 0xd0, 0x37, 0xba, 0x9a, 0xce, 0x24, 0x97, 0xa8,
|
|
0x64, 0x9a, 0x52, 0xa8, 0xe8, 0x6d, 0x58, 0x56, 0x35, 0xdb, 0xec, 0x2b, 0x23, 0xc6, 0x90, 0xa2,
|
|
0x0c, 0x19, 0x4e, 0x23, 0x2c, 0xd2, 0x8f, 0x92, 0x90, 0x22, 0x36, 0x4f, 0x74, 0xcb, 0x23, 0x58,
|
|
0x60, 0xd3, 0x16, 0xe9, 0xb4, 0xef, 0xcc, 0x98, 0x36, 0x9d, 0x2f, 0x93, 0x40, 0x5f, 0x81, 0x95,
|
|
0x8e, 0x85, 0x29, 0x36, 0xda, 0xaa, 0xeb, 0xb9, 0xcc, 0x6e, 0x61, 0x87, 0x01, 0x71, 0xc7, 0x05,
|
|
0xe2, 0x4e, 0xcb, 0x05, 0xa2, 0xbc, 0xec, 0x0a, 0x1c, 0x10, 0x05, 0xef, 0x40, 0xa6, 0xd3, 0x53,
|
|
0xf4, 0x2e, 0x66, 0xe2, 0xc9, 0x99, 0xe2, 0xc0, 0xd8, 0xa9, 0xb0, 0xdf, 0x67, 0x0b, 0x21, 0x9f,
|
|
0xdd, 0x82, 0xcc, 0xd8, 0x67, 0x76, 0x7e, 0x71, 0x2b, 0xb1, 0x9d, 0x96, 0xc1, 0x73, 0x9a, 0x8d,
|
|
0x1e, 0xc0, 0x15, 0xd3, 0xc2, 0x67, 0xd8, 0xb2, 0xb0, 0xda, 0x8e, 0xb8, 0x17, 0x79, 0x7d, 0xc7,
|
|
0x9e, 0x9f, 0x1f, 0x01, 0xf4, 0x15, 0xdb, 0x61, 0xcc, 0xd4, 0xcb, 0xd3, 0x4d, 0x4d, 0x13, 0x6e,
|
|
0x2a, 0x8e, 0xee, 0x42, 0xd6, 0xc2, 0xb6, 0x31, 0xb4, 0x3a, 0xb8, 0x6d, 0xbc, 0xd0, 0xb1, 0x95,
|
|
0x4f, 0xd3, 0x61, 0x56, 0x5c, 0x6a, 0x83, 0x10, 0x83, 0x08, 0x81, 0x10, 0x42, 0xde, 0x81, 0x85,
|
|
0xde, 0x70, 0xa0, 0xe8, 0xf9, 0x0c, 0x1d, 0x79, 0x4a, 0x98, 0x0e, 0x09, 0x1b, 0x09, 0xf5, 0xe1,
|
|
0x25, 0x99, 0xc9, 0xa0, 0x32, 0x2c, 0x0d, 0x94, 0x4e, 0x4f, 0xd3, 0x71, 0x7e, 0x99, 0x8a, 0xdf,
|
|
0x8d, 0x17, 0xaf, 0x31, 0x46, 0xae, 0xc0, 0x95, 0xdb, 0xcb, 0x40, 0x92, 0xd8, 0x82, 0x12, 0xff,
|
|
0xb5, 0x27, 0x48, 0xbf, 0x26, 0x40, 0xc6, 0xc7, 0x87, 0x7e, 0x1e, 0xb2, 0xd4, 0x37, 0xef, 0xe3,
|
|
0x51, 0x5b, 0x51, 0x55, 0xcc, 0xf0, 0x35, 0x03, 0x09, 0x44, 0xe2, 0x08, 0x8f, 0xca, 0x84, 0x1f,
|
|
0x21, 0x48, 0xd2, 0x69, 0xb3, 0x85, 0x49, 0xff, 0xa3, 0x2d, 0xc8, 0xa8, 0xd8, 0xee, 0x58, 0x1a,
|
|
0xdd, 0x63, 0x28, 0xb8, 0x08, 0xb0, 0xc7, 0x24, 0xe9, 0x97, 0x45, 0xc8, 0x72, 0x3b, 0x8e, 0xf0,
|
|
0x68, 0x22, 0xbc, 0xbf, 0x0c, 0x49, 0x67, 0x64, 0xba, 0xe8, 0xde, 0x9e, 0x39, 0xef, 0x23, 0x3c,
|
|
0x6a, 0x8d, 0x4c, 0x2c, 0x53, 0xa9, 0x00, 0xc6, 0x12, 0x21, 0x8c, 0x45, 0xd0, 0x9f, 0xbc, 0x20,
|
|
0xfa, 0xf7, 0x61, 0x15, 0x7f, 0x60, 0x6a, 0x96, 0x4f, 0xc5, 0xc2, 0x4c, 0x15, 0xd9, 0xb1, 0x08,
|
|
0x51, 0x22, 0xfd, 0x38, 0x09, 0x69, 0x2f, 0xe2, 0xa8, 0x02, 0x39, 0x53, 0xb1, 0xed, 0x17, 0x86,
|
|
0xa5, 0xb6, 0xd9, 0x52, 0x99, 0x27, 0x14, 0xab, 0xae, 0xcc, 0x3e, 0x13, 0x21, 0x5b, 0xce, 0x99,
|
|
0x66, 0xd9, 0x4e, 0xdb, 0x17, 0x93, 0x34, 0xa5, 0x50, 0x2c, 0x6e, 0x02, 0x05, 0x37, 0xeb, 0x65,
|
|
0x61, 0x49, 0x11, 0xc2, 0xc4, 0xfd, 0x28, 0x19, 0xd9, 0x8f, 0x88, 0xbc, 0xae, 0x75, 0xde, 0x0f,
|
|
0x6c, 0x85, 0x84, 0x40, 0x3b, 0xdf, 0x00, 0xe4, 0x5b, 0x99, 0x8a, 0xde, 0x1d, 0x2a, 0x5d, 0xb6,
|
|
0x29, 0xa6, 0xe5, 0xb5, 0xf1, 0xba, 0xe4, 0x1d, 0xe8, 0x6d, 0x58, 0xec, 0x62, 0x5d, 0xc5, 0x16,
|
|
0x5d, 0xba, 0xd9, 0xdd, 0xad, 0xf8, 0x08, 0x3f, 0xa5, 0x7c, 0x32, 0xe7, 0x47, 0x57, 0x60, 0x01,
|
|
0x0f, 0x14, 0xad, 0xcf, 0x77, 0x4c, 0xd6, 0x40, 0x45, 0x58, 0xd3, 0xec, 0x36, 0xfd, 0xdf, 0x3e,
|
|
0xc7, 0x96, 0x76, 0xa6, 0x61, 0x95, 0x2e, 0xd7, 0x94, 0xbc, 0xaa, 0xd9, 0x15, 0x42, 0x7f, 0xc6,
|
|
0xc9, 0x44, 0x83, 0xd9, 0x33, 0x74, 0x77, 0xb1, 0xb2, 0x06, 0xd7, 0x40, 0xff, 0x8f, 0x35, 0x64,
|
|
0x5c, 0x0d, 0x27, 0x84, 0xee, 0x69, 0xc8, 0xc3, 0x52, 0xc7, 0x18, 0xea, 0x8e, 0x35, 0xa2, 0x0b,
|
|
0x33, 0x2d, 0xbb, 0x4d, 0x82, 0xbc, 0xbe, 0xd1, 0x51, 0xfa, 0x9a, 0x33, 0xca, 0xaf, 0x70, 0x17,
|
|
0xf3, 0x36, 0xd9, 0xdd, 0x4c, 0xc3, 0x76, 0x94, 0x7e, 0xbb, 0x63, 0xa8, 0x38, 0x9f, 0xa5, 0xdd,
|
|
0xc0, 0x48, 0xfb, 0x86, 0x8a, 0xd1, 0x3a, 0x2c, 0x5a, 0xb8, 0x4b, 0x16, 0xcd, 0x2a, 0x3b, 0x83,
|
|
0x58, 0x8b, 0x6c, 0x44, 0xb6, 0x63, 0x61, 0xec, 0x90, 0x55, 0x6a, 0x61, 0xdb, 0xce, 0xe7, 0xd8,
|
|
0x46, 0xc4, 0xa8, 0x65, 0x46, 0x94, 0xfe, 0x30, 0x01, 0x19, 0xb2, 0xd9, 0x9f, 0x58, 0xc6, 0x99,
|
|
0xd6, 0xc7, 0x91, 0x35, 0xf5, 0x2a, 0xf0, 0x08, 0xc4, 0x3e, 0x19, 0x8a, 0x7d, 0x18, 0x3b, 0x0b,
|
|
0x51, 0xec, 0xfc, 0xc4, 0xe0, 0xe1, 0x5f, 0xfa, 0xa9, 0x59, 0x4b, 0x3f, 0xfd, 0x6a, 0x07, 0x1f,
|
|
0x5c, 0xe4, 0xe0, 0x93, 0xfe, 0x3d, 0xc1, 0x92, 0x1d, 0x1e, 0x9e, 0x89, 0xdb, 0xde, 0xcf, 0x42,
|
|
0xf4, 0x79, 0x86, 0x28, 0x9c, 0x7f, 0x64, 0xe6, 0xce, 0x3f, 0x96, 0xe3, 0xf2, 0x0f, 0xe9, 0x37,
|
|
0x44, 0xc8, 0x9f, 0x9a, 0xc4, 0x16, 0x5f, 0xec, 0x65, 0x32, 0x5d, 0xdb, 0x41, 0xf7, 0x02, 0xe1,
|
|
0xa6, 0x30, 0xd8, 0x83, 0x97, 0x7b, 0x4b, 0xd6, 0x42, 0x4e, 0xc8, 0xff, 0xa3, 0xe0, 0x0f, 0xfd,
|
|
0xeb, 0xfe, 0xd0, 0x8b, 0x11, 0xce, 0x31, 0x0c, 0xee, 0xfa, 0x61, 0x40, 0x31, 0xb2, 0x97, 0x7a,
|
|
0xb9, 0xb7, 0x60, 0x25, 0x28, 0x9b, 0x07, 0x88, 0x47, 0x13, 0xa3, 0x9d, 0x8c, 0x28, 0x9e, 0x1a,
|
|
0xf9, 0x85, 0x8b, 0x45, 0x5e, 0x92, 0xe1, 0x2a, 0x3b, 0xab, 0x4e, 0x79, 0x7e, 0xe4, 0x3a, 0xe2,
|
|
0x91, 0x3f, 0x87, 0x62, 0x7e, 0xb8, 0xfe, 0x72, 0xef, 0x9a, 0xb5, 0xb1, 0x7b, 0xf5, 0x5b, 0xdf,
|
|
0xfc, 0xd6, 0x37, 0x1f, 0xdb, 0xa6, 0xd2, 0xc1, 0x8f, 0xdf, 0x7b, 0xef, 0xa3, 0x87, 0xf7, 0x77,
|
|
0x1f, 0x3c, 0xf8, 0xf8, 0xb5, 0x71, 0x86, 0x25, 0xfd, 0x87, 0x00, 0x69, 0xa2, 0x8e, 0xee, 0xf1,
|
|
0x91, 0x05, 0xe5, 0x9d, 0x16, 0xa2, 0xff, 0xb4, 0xd8, 0x86, 0xf0, 0xa1, 0x40, 0x3d, 0x35, 0xe1,
|
|
0xac, 0xf0, 0x63, 0x35, 0x39, 0x0b, 0xab, 0x0b, 0xaf, 0x86, 0xd5, 0xc5, 0x0b, 0x6d, 0x27, 0xff,
|
|
0x2d, 0xc0, 0x8a, 0x37, 0xef, 0x89, 0x9b, 0xc9, 0x4f, 0xef, 0xdc, 0x1f, 0x43, 0x9e, 0x5a, 0x39,
|
|
0xaa, 0x8d, 0x3c, 0x17, 0xb8, 0x50, 0xba, 0x09, 0x49, 0x7a, 0xbc, 0x46, 0x57, 0x13, 0xa5, 0x4b,
|
|
0x8f, 0x61, 0x7d, 0xbc, 0x1e, 0x03, 0x92, 0x5b, 0xae, 0xbf, 0xa2, 0xa2, 0xac, 0x43, 0xfa, 0x4f,
|
|
0x8e, 0x35, 0x9a, 0x0d, 0x4c, 0xf2, 0x37, 0xcb, 0x2b, 0xc4, 0x99, 0x79, 0x45, 0x62, 0x72, 0x5e,
|
|
0xf1, 0xff, 0xd7, 0xe3, 0xff, 0xc3, 0xd1, 0xc6, 0xec, 0x8d, 0x41, 0xdb, 0x4f, 0xed, 0xec, 0x1f,
|
|
0xf9, 0x31, 0x43, 0x8d, 0x76, 0x31, 0x73, 0xcb, 0x9d, 0x35, 0xc3, 0x4c, 0xfa, 0xe5, 0xde, 0xa2,
|
|
0x95, 0xcc, 0x09, 0xf9, 0x2b, 0xdc, 0x01, 0xd2, 0xdb, 0xb0, 0xce, 0xa0, 0x1a, 0x11, 0x9d, 0x05,
|
|
0xd4, 0x7f, 0x12, 0x59, 0x3a, 0xc7, 0xd3, 0xbb, 0x88, 0xc3, 0x7d, 0x49, 0xa8, 0x18, 0x9f, 0x84,
|
|
0x26, 0xa6, 0x27, 0xa1, 0xc9, 0x29, 0x49, 0xe8, 0xc2, 0x8c, 0x24, 0x74, 0x71, 0x42, 0x12, 0x1a,
|
|
0x08, 0xe2, 0xd2, 0xac, 0x20, 0xa6, 0x5e, 0x2d, 0x88, 0xe9, 0x0b, 0x05, 0xf1, 0xc7, 0xfc, 0xb1,
|
|
0x89, 0x5b, 0x3a, 0x11, 0xc4, 0x3f, 0xf3, 0xe9, 0xc5, 0x7c, 0xfa, 0xaf, 0x82, 0x3f, 0xbb, 0xe1,
|
|
0xf6, 0xba, 0x00, 0x97, 0xc6, 0xce, 0x14, 0x42, 0x79, 0x88, 0xe7, 0xd6, 0xd7, 0x7c, 0x6e, 0x15,
|
|
0xc3, 0xc9, 0x8a, 0xe7, 0xe0, 0x7b, 0x41, 0x07, 0x87, 0xb3, 0x1a, 0xbf, 0xab, 0xb7, 0x3c, 0x57,
|
|
0x27, 0x43, 0x5c, 0xae, 0xd3, 0x4b, 0x11, 0xa7, 0x2f, 0x84, 0x38, 0x43, 0xf7, 0xaa, 0x3e, 0x64,
|
|
0x4f, 0x02, 0x37, 0x6d, 0x74, 0x1f, 0x96, 0x8d, 0xbe, 0xda, 0x76, 0xef, 0xdf, 0xe1, 0xc5, 0x7f,
|
|
0x28, 0x67, 0x8c, 0xbe, 0xea, 0xca, 0x10, 0x6e, 0x1d, 0xbf, 0x18, 0x73, 0x8b, 0x11, 0x6e, 0x1d,
|
|
0xbf, 0x70, 0xb9, 0xa5, 0x2f, 0xc2, 0x32, 0x3f, 0xdb, 0xce, 0x94, 0x86, 0x63, 0xa2, 0xcd, 0xc0,
|
|
0x36, 0xb1, 0xf4, 0x72, 0x2f, 0x69, 0x89, 0x39, 0x77, 0x8f, 0xa8, 0xc2, 0x72, 0x6d, 0xd8, 0x77,
|
|
0xb4, 0x27, 0x4a, 0xc7, 0x31, 0x2c, 0x1b, 0x3d, 0x82, 0xe4, 0xe0, 0x4c, 0x71, 0xdf, 0x65, 0xa7,
|
|
0x3d, 0x17, 0x8d, 0xa5, 0x64, 0x2a, 0x22, 0xfd, 0x22, 0x64, 0x7c, 0x44, 0xf4, 0x16, 0x7f, 0x80,
|
|
0x11, 0x68, 0x8a, 0x77, 0x7b, 0x8a, 0xa6, 0x33, 0xc5, 0xf7, 0xf2, 0xf2, 0x76, 0xf0, 0x59, 0x52,
|
|
0x9a, 0x22, 0xf7, 0xa4, 0xec, 0x7f, 0x95, 0x94, 0xbe, 0x2f, 0x40, 0x96, 0x4d, 0x59, 0xc6, 0xb6,
|
|
0x69, 0xe8, 0x76, 0xe0, 0x51, 0x56, 0x08, 0x3c, 0xca, 0xe6, 0x20, 0x31, 0xb4, 0xdc, 0xbc, 0x86,
|
|
0xfc, 0x25, 0x2b, 0xcc, 0xc6, 0x1d, 0x0b, 0x3b, 0x7c, 0x71, 0xf2, 0xd6, 0xd8, 0x9e, 0xe4, 0x45,
|
|
0xed, 0xf9, 0x15, 0x11, 0xd6, 0x09, 0xa8, 0x9f, 0x5a, 0x8a, 0xee, 0x34, 0xb1, 0x62, 0x75, 0x7a,
|
|
0x2e, 0xb0, 0xd7, 0x61, 0xd1, 0x38, 0x3b, 0xb3, 0xb1, 0x43, 0xcd, 0x4a, 0xca, 0xbc, 0x45, 0x8e,
|
|
0xc0, 0xbe, 0x36, 0xd0, 0x1c, 0x6a, 0x58, 0x52, 0x66, 0x0d, 0xf4, 0x1e, 0x64, 0x6d, 0xc3, 0x72,
|
|
0x34, 0xbd, 0xdb, 0xee, 0x18, 0xfd, 0xe1, 0x40, 0xe7, 0x2f, 0xd5, 0xf7, 0xa7, 0x3f, 0xd9, 0xfa,
|
|
0xc6, 0x3d, 0xc2, 0x23, 0x8a, 0xce, 0x4f, 0x04, 0x71, 0xeb, 0x92, 0xbc, 0xc2, 0xb5, 0xed, 0x53,
|
|
0x65, 0xc4, 0x17, 0x8a, 0xdd, 0xa1, 0xf3, 0x4b, 0xc9, 0xe4, 0x2f, 0x3a, 0x84, 0xa5, 0xef, 0x0c,
|
|
0xb1, 0xa5, 0x61, 0x82, 0x6c, 0x82, 0x83, 0x9d, 0xb9, 0x47, 0xfa, 0xda, 0x10, 0x5b, 0x23, 0xd9,
|
|
0x15, 0x97, 0xfe, 0x46, 0x80, 0x2b, 0x93, 0x38, 0xd0, 0x21, 0x24, 0xde, 0xc7, 0x23, 0x0e, 0x8e,
|
|
0x4f, 0x3b, 0x11, 0xa2, 0x02, 0xfd, 0x1c, 0x2c, 0x0e, 0xb0, 0xd3, 0x33, 0x54, 0x8e, 0x98, 0x2f,
|
|
0xc4, 0x2b, 0x63, 0x3a, 0x6a, 0x94, 0x5b, 0xe6, 0x52, 0xc4, 0xe7, 0xe7, 0x4a, 0x7f, 0xe8, 0x5e,
|
|
0x87, 0x59, 0x43, 0xfa, 0x23, 0x11, 0x36, 0x22, 0xc1, 0xe3, 0xa8, 0xba, 0x58, 0xf4, 0x6e, 0xc3,
|
|
0xb2, 0x63, 0x90, 0x9d, 0xc7, 0xc2, 0xf6, 0xb0, 0xef, 0xf0, 0xe7, 0xc4, 0x0c, 0xa5, 0xc9, 0x94,
|
|
0x84, 0xbe, 0x42, 0xb6, 0x1c, 0xda, 0x99, 0xa4, 0xee, 0x7e, 0x7d, 0x0e, 0x7f, 0xd0, 0x62, 0x08,
|
|
0x17, 0x63, 0x37, 0x6f, 0xa3, 0x83, 0x6d, 0x1b, 0xab, 0xed, 0xd0, 0xe3, 0xf8, 0x9a, 0xd7, 0xd3,
|
|
0x74, 0xb7, 0xfc, 0x32, 0x64, 0xcf, 0x35, 0xfc, 0xa2, 0xed, 0xd5, 0x89, 0xe6, 0xc8, 0x66, 0x56,
|
|
0x88, 0x84, 0xd7, 0x94, 0xfe, 0x84, 0xa7, 0x73, 0x9e, 0x2d, 0x64, 0xf6, 0x0d, 0xab, 0x5b, 0x75,
|
|
0x57, 0x1a, 0x6b, 0xa0, 0xeb, 0x90, 0x3e, 0xb1, 0x8c, 0x6f, 0xe3, 0x8e, 0x53, 0x75, 0xab, 0x2f,
|
|
0x63, 0x02, 0xf1, 0xe4, 0x29, 0x5d, 0x90, 0xee, 0xa2, 0x63, 0x2d, 0xa2, 0x4b, 0x36, 0xfa, 0xd8,
|
|
0xa6, 0xfe, 0x48, 0xcb, 0xac, 0x41, 0xce, 0xd6, 0x86, 0xd5, 0xad, 0x8f, 0x5f, 0x1f, 0xdc, 0x26,
|
|
0xe9, 0xa1, 0x86, 0x54, 0x55, 0x7e, 0xfe, 0xb9, 0x4d, 0xe9, 0xcf, 0x04, 0xb8, 0x56, 0x1b, 0xf1,
|
|
0x11, 0x1b, 0x56, 0xf7, 0x55, 0xd6, 0x61, 0x74, 0xa1, 0x1c, 0x85, 0x17, 0xca, 0xc3, 0x29, 0xdb,
|
|
0x43, 0xc4, 0x8a, 0xd0, 0x5a, 0xf9, 0x7b, 0x01, 0x36, 0x62, 0x98, 0xd0, 0x91, 0x7f, 0xb9, 0x94,
|
|
0x2e, 0x32, 0xc8, 0x4f, 0x6c, 0xc5, 0xfc, 0xb1, 0x00, 0x85, 0x49, 0x9e, 0xfe, 0xac, 0x16, 0xcd,
|
|
0x5b, 0xa1, 0x45, 0x73, 0x23, 0x7e, 0x16, 0x0d, 0xab, 0xeb, 0x2e, 0x15, 0xe9, 0x1e, 0x24, 0x1a,
|
|
0x56, 0x37, 0x92, 0xb7, 0x4d, 0xa8, 0x43, 0x48, 0x0f, 0x61, 0xa5, 0x36, 0x3a, 0xc1, 0xd6, 0x40,
|
|
0x63, 0xd5, 0x46, 0xb4, 0x05, 0x19, 0x73, 0xdc, 0xa4, 0x67, 0x64, 0x5a, 0xf6, 0x93, 0x24, 0x05,
|
|
0xb2, 0xec, 0x84, 0xf7, 0x72, 0x18, 0x6f, 0x7e, 0x82, 0x7f, 0x7e, 0xaf, 0xc3, 0xaa, 0xbb, 0x4c,
|
|
0xdb, 0xdc, 0x2d, 0x6c, 0xfe, 0x59, 0x97, 0xdc, 0x60, 0xee, 0xe1, 0x98, 0x4b, 0x78, 0x98, 0x93,
|
|
0x6c, 0x58, 0xe2, 0x43, 0xa0, 0xc7, 0xb0, 0xc4, 0x52, 0x29, 0xf7, 0xbc, 0x9e, 0xf2, 0x90, 0xc2,
|
|
0x64, 0x64, 0x57, 0xc0, 0x17, 0x0f, 0x71, 0x72, 0x3c, 0x12, 0x3e, 0x7b, 0xa5, 0x5f, 0x17, 0x61,
|
|
0x91, 0xa7, 0x2e, 0xa1, 0x74, 0x4f, 0xb8, 0xd0, 0xfb, 0xd8, 0x01, 0x00, 0x3e, 0xc7, 0xba, 0xd3,
|
|
0xf6, 0x6a, 0x33, 0xd1, 0x24, 0x83, 0xd9, 0x7b, 0x4c, 0x53, 0xb7, 0x0f, 0xb1, 0x5a, 0x63, 0x45,
|
|
0x65, 0x39, 0x4d, 0x05, 0x5b, 0xb3, 0xaa, 0x33, 0x9b, 0x90, 0xc6, 0xaa, 0xe6, 0x18, 0xbe, 0x4a,
|
|
0x6c, 0x8a, 0x11, 0xd8, 0x7e, 0xc3, 0xfe, 0xbb, 0x69, 0x34, 0x6b, 0xa1, 0x2f, 0x42, 0x52, 0x55,
|
|
0x1c, 0x85, 0x6f, 0x83, 0x1b, 0x91, 0xc9, 0x34, 0x69, 0xb9, 0x5d, 0xa6, 0x4c, 0xd2, 0x9f, 0x27,
|
|
0x20, 0xef, 0xa5, 0x73, 0xc6, 0xc0, 0xec, 0xe3, 0x0f, 0x34, 0x67, 0x74, 0x62, 0xf4, 0xb5, 0xce,
|
|
0x28, 0x82, 0xab, 0x50, 0x2d, 0x4b, 0x8c, 0xd4, 0xb2, 0x3e, 0xe7, 0x62, 0xea, 0x0d, 0x80, 0x81,
|
|
0xa6, 0xb7, 0xfb, 0x58, 0xef, 0x3a, 0x3d, 0x7e, 0x62, 0xa4, 0x07, 0x9a, 0x7e, 0x4c, 0x09, 0xe8,
|
|
0x0e, 0xac, 0xf4, 0x14, 0xbb, 0xdd, 0x37, 0x5e, 0x60, 0xab, 0xa3, 0xd8, 0xec, 0xda, 0x9b, 0x92,
|
|
0x97, 0x7b, 0x8a, 0x7d, 0xec, 0xd2, 0x5c, 0xa6, 0xa1, 0x69, 0x72, 0xa6, 0x25, 0x8f, 0xe9, 0xd4,
|
|
0xa5, 0x91, 0x81, 0x08, 0x93, 0x3e, 0x1c, 0x3c, 0xc7, 0x16, 0xbd, 0x63, 0xa4, 0xe4, 0x74, 0x4f,
|
|
0xb1, 0xeb, 0x94, 0xe0, 0x76, 0xdb, 0xa3, 0xc1, 0x73, 0xa3, 0xcf, 0xeb, 0x2e, 0xa4, 0xbb, 0x49,
|
|
0x09, 0x81, 0x88, 0x43, 0xb4, 0x4e, 0xae, 0xd9, 0x6d, 0x15, 0x9f, 0x29, 0x64, 0x33, 0x60, 0x05,
|
|
0x97, 0xb4, 0x66, 0x1f, 0x30, 0x82, 0x34, 0x84, 0xcb, 0x95, 0x0f, 0x1c, 0x6c, 0xe9, 0x4a, 0xbf,
|
|
0x7a, 0x70, 0xe2, 0xed, 0x47, 0x12, 0xac, 0x68, 0xaa, 0xd9, 0xee, 0x18, 0xfa, 0x99, 0xd6, 0x1d,
|
|
0x27, 0x88, 0x19, 0x4d, 0x35, 0xf7, 0x29, 0xad, 0xaa, 0xfa, 0xd3, 0x47, 0x31, 0x90, 0x3e, 0x86,
|
|
0x1f, 0xc3, 0x13, 0xd1, 0xda, 0x7b, 0x0f, 0xf2, 0x81, 0x61, 0x07, 0xc6, 0x39, 0x1e, 0xdf, 0x6b,
|
|
0x66, 0x8f, 0xbd, 0x0d, 0x39, 0xcc, 0xe5, 0xdb, 0x41, 0x23, 0xb2, 0x2e, 0x9d, 0x1d, 0x96, 0xd2,
|
|
0x61, 0x60, 0xa4, 0x57, 0x38, 0xe0, 0xa4, 0x1f, 0x8a, 0x70, 0x6d, 0x82, 0xaa, 0xcf, 0x6a, 0x07,
|
|
0x2f, 0x87, 0x76, 0xf0, 0x29, 0x5f, 0x81, 0xf8, 0xac, 0xfa, 0x9c, 0x13, 0x9f, 0x1f, 0x89, 0xb0,
|
|
0x1a, 0xb2, 0x26, 0xfe, 0x9a, 0x11, 0x09, 0xb4, 0x38, 0x5f, 0xa0, 0x13, 0x93, 0x02, 0x8d, 0xae,
|
|
0x41, 0x8a, 0x68, 0xf3, 0x95, 0x67, 0x96, 0x34, 0xd5, 0xe4, 0x5f, 0x09, 0x14, 0x82, 0x4a, 0x26,
|
|
0xd4, 0x6a, 0x36, 0xfc, 0xea, 0x0e, 0x7c, 0x75, 0x9b, 0xc8, 0x0e, 0xb4, 0xf8, 0x6a, 0x3b, 0xd0,
|
|
0xd2, 0x45, 0x76, 0xa0, 0xa2, 0x15, 0xf8, 0x4c, 0x88, 0x7d, 0x4c, 0xb3, 0x05, 0xd7, 0x4f, 0x9b,
|
|
0x15, 0xb9, 0x59, 0x69, 0x36, 0xab, 0x8d, 0x7a, 0xb3, 0x55, 0x6e, 0x55, 0xda, 0xa7, 0xf5, 0xe6,
|
|
0x49, 0x65, 0xbf, 0xfa, 0xa4, 0x5a, 0x39, 0xc8, 0x5d, 0x42, 0x9b, 0xb0, 0x11, 0xe1, 0x28, 0xef,
|
|
0xb7, 0xaa, 0xcf, 0x2a, 0x39, 0x01, 0xdd, 0x82, 0xcd, 0x48, 0x67, 0xab, 0x22, 0xd7, 0xaa, 0xf5,
|
|
0x72, 0xab, 0x72, 0x90, 0x13, 0x8b, 0x65, 0xff, 0xe7, 0x03, 0xfc, 0x48, 0x59, 0xaf, 0x95, 0xf7,
|
|
0x0f, 0xab, 0xf5, 0xca, 0x51, 0xe5, 0xdd, 0xd0, 0x58, 0x97, 0x61, 0xd5, 0xd7, 0xf7, 0xd5, 0x66,
|
|
0xa3, 0x9e, 0x13, 0x8a, 0x7f, 0xc9, 0x5f, 0x72, 0x99, 0xc1, 0xd7, 0xe0, 0x2a, 0x1d, 0x71, 0x82,
|
|
0xa5, 0x57, 0x20, 0x37, 0xee, 0xf2, 0x4c, 0x5c, 0x07, 0x34, 0xa6, 0x56, 0xeb, 0x9c, 0x2e, 0xa2,
|
|
0xab, 0xb0, 0x36, 0xa6, 0x1f, 0x54, 0x8e, 0x2b, 0xc4, 0xe0, 0x44, 0x50, 0xc9, 0x71, 0x63, 0xff,
|
|
0xa8, 0x72, 0x90, 0x4b, 0x06, 0x99, 0x9b, 0xa7, 0xcd, 0x93, 0x4a, 0xfd, 0x20, 0xb7, 0x10, 0x24,
|
|
0x57, 0xeb, 0xd5, 0x56, 0xb5, 0x7c, 0x9c, 0x5b, 0x2c, 0xfe, 0x02, 0x2c, 0xb2, 0x6a, 0x0a, 0x19,
|
|
0xfc, 0x69, 0xa5, 0x7e, 0x50, 0x91, 0x43, 0xa6, 0xae, 0xc1, 0x0a, 0xa7, 0x3f, 0xa9, 0xd4, 0xca,
|
|
0xc7, 0xc4, 0xce, 0x55, 0xc8, 0x70, 0x12, 0x25, 0x88, 0x08, 0x41, 0x96, 0x13, 0x0e, 0xaa, 0xcf,
|
|
0x88, 0x8f, 0x73, 0x89, 0xe2, 0x01, 0x2c, 0xf1, 0x4b, 0x3c, 0xda, 0x80, 0xcb, 0xb5, 0x27, 0xe5,
|
|
0xd6, 0xbb, 0x27, 0x61, 0x37, 0xac, 0x42, 0xc6, 0xed, 0x68, 0xd6, 0x9a, 0x4c, 0xb3, 0x4b, 0x68,
|
|
0xb4, 0x4e, 0x72, 0x62, 0xf1, 0x0c, 0x52, 0xee, 0x15, 0x1a, 0xe5, 0xe1, 0x0a, 0xf9, 0x3f, 0xc1,
|
|
0x9d, 0xeb, 0x80, 0xbc, 0x9e, 0x7a, 0xa3, 0xd5, 0x96, 0x2b, 0xe5, 0x83, 0x77, 0x73, 0x02, 0xb1,
|
|
0xcb, 0xa3, 0x33, 0x9a, 0x48, 0xbc, 0xe6, 0xa3, 0xd5, 0x1a, 0xcf, 0x88, 0x2f, 0x8b, 0xe7, 0x80,
|
|
0xa2, 0xb7, 0x4a, 0x74, 0x13, 0x0a, 0x51, 0x6a, 0xfb, 0xb4, 0x7e, 0x54, 0x6f, 0x7c, 0xbd, 0x9e,
|
|
0xbb, 0x84, 0x6e, 0xc0, 0xb5, 0x09, 0xfd, 0x0d, 0xf9, 0x69, 0xbb, 0x7a, 0x90, 0x13, 0xd0, 0x6d,
|
|
0xb8, 0x31, 0xa1, 0xfb, 0x44, 0x6e, 0x7c, 0xb5, 0xb2, 0xdf, 0x22, 0x2c, 0x62, 0xf1, 0x39, 0x5c,
|
|
0x9d, 0x98, 0x9e, 0xa3, 0xbb, 0x70, 0xbb, 0xf6, 0x2e, 0x67, 0x6d, 0xc8, 0x4f, 0x9b, 0x95, 0xb2,
|
|
0xbc, 0x7f, 0x18, 0x85, 0xa1, 0x04, 0x37, 0x27, 0xb3, 0x11, 0x23, 0xea, 0xe5, 0x5a, 0x25, 0x27,
|
|
0x14, 0xff, 0x45, 0x80, 0x65, 0x7f, 0xce, 0x4e, 0xe2, 0xc1, 0x18, 0x6b, 0x95, 0xd6, 0x61, 0xe3,
|
|
0xa0, 0x5d, 0xf9, 0xda, 0x69, 0xf9, 0xb8, 0x99, 0xbb, 0x84, 0xae, 0x43, 0x3e, 0xd0, 0xd1, 0x6c,
|
|
0x95, 0xe5, 0x56, 0xb3, 0xfd, 0xf5, 0x6a, 0xeb, 0x30, 0x27, 0x10, 0x3c, 0x07, 0x7a, 0xf7, 0x1b,
|
|
0xf5, 0x56, 0xb9, 0x5a, 0x6f, 0xe6, 0x44, 0x74, 0x07, 0x6e, 0x4d, 0xd0, 0xd8, 0xae, 0x3e, 0xad,
|
|
0x37, 0xe4, 0x4a, 0x7b, 0xbf, 0x4c, 0x10, 0x81, 0xb6, 0xe1, 0xb5, 0x38, 0xed, 0x01, 0xce, 0x24,
|
|
0x99, 0xfc, 0xc4, 0x91, 0x02, 0x6c, 0x0b, 0xbb, 0xbf, 0x7d, 0x07, 0x32, 0xe5, 0xa1, 0xd3, 0x6b,
|
|
0x62, 0xeb, 0x5c, 0xeb, 0x60, 0x72, 0xf3, 0x3a, 0xc4, 0x4a, 0xdf, 0xe9, 0x7d, 0x88, 0xd6, 0x23,
|
|
0x1b, 0x4d, 0x65, 0x60, 0x3a, 0xa3, 0x42, 0x0c, 0x5d, 0xca, 0x7d, 0xf2, 0xcf, 0xff, 0xf6, 0x3b,
|
|
0x22, 0xa0, 0x54, 0xa9, 0xc7, 0x35, 0x3c, 0x85, 0x05, 0x19, 0x2b, 0xea, 0xe8, 0xc2, 0xaa, 0xb2,
|
|
0x54, 0x55, 0x0a, 0x2d, 0x96, 0x2c, 0x2a, 0x5f, 0x87, 0xd4, 0x33, 0xfe, 0x85, 0x65, 0xac, 0xae,
|
|
0xb8, 0xec, 0x52, 0x5a, 0xa3, 0xca, 0x32, 0x28, 0xed, 0x7d, 0xa5, 0x89, 0xbe, 0x2b, 0xc0, 0xda,
|
|
0x53, 0xec, 0xb0, 0x22, 0x95, 0xfb, 0xd1, 0x63, 0xac, 0xe6, 0xe2, 0xdc, 0x5f, 0x51, 0xda, 0xd2,
|
|
0x1b, 0x9f, 0xfc, 0x75, 0x7e, 0x15, 0x56, 0x08, 0x0f, 0xd6, 0x1d, 0xad, 0xa3, 0x38, 0x58, 0xa5,
|
|
0xe3, 0x5f, 0x41, 0xa8, 0x44, 0x4e, 0x10, 0xbb, 0x34, 0xc0, 0x25, 0xf7, 0x33, 0x4d, 0x34, 0x80,
|
|
0xb4, 0x67, 0x47, 0xec, 0xf8, 0xd2, 0xf4, 0xf1, 0xc9, 0xc0, 0xd2, 0x6b, 0x71, 0xe3, 0x92, 0x79,
|
|
0xbb, 0xe3, 0xa2, 0x5f, 0x15, 0x20, 0xe7, 0x8d, 0xe7, 0x7e, 0x8d, 0x12, 0x37, 0xec, 0x8c, 0x8f,
|
|
0x47, 0x7d, 0x5f, 0x4b, 0x48, 0xf7, 0xe3, 0x46, 0xbf, 0x8c, 0xd6, 0xc6, 0xb3, 0x36, 0xf9, 0x80,
|
|
0x3f, 0x14, 0xe0, 0x32, 0x7b, 0x9b, 0x0e, 0x1a, 0xb2, 0x3b, 0x65, 0xc0, 0x98, 0x42, 0x7d, 0xe1,
|
|
0xee, 0x5c, 0x46, 0x4a, 0xa5, 0x38, 0x03, 0xd7, 0x0b, 0x51, 0x03, 0x1f, 0x0b, 0x45, 0xf4, 0x7d,
|
|
0x01, 0x72, 0xec, 0x62, 0xc6, 0x6c, 0xa4, 0x07, 0x7a, 0x69, 0xd6, 0x35, 0x30, 0x54, 0x3d, 0x8f,
|
|
0xc5, 0xf7, 0x83, 0x38, 0x73, 0x36, 0x0a, 0x3e, 0x94, 0x90, 0x3f, 0x24, 0xcf, 0x20, 0xf6, 0x7c,
|
|
0x0c, 0x59, 0x2f, 0x70, 0xac, 0xa0, 0x1e, 0x17, 0xb6, 0x19, 0x8f, 0x5c, 0x5e, 0x55, 0x5a, 0x2a,
|
|
0xc6, 0x19, 0xb1, 0x86, 0x56, 0xc7, 0x46, 0xb0, 0xda, 0xf4, 0xef, 0x0b, 0xb0, 0xe6, 0x77, 0x07,
|
|
0x33, 0xe1, 0xc1, 0x3c, 0x01, 0xf3, 0x57, 0x72, 0x0b, 0x77, 0xe6, 0x30, 0x6e, 0xca, 0x1a, 0x2a,
|
|
0x84, 0x0d, 0x23, 0xae, 0xf9, 0x3d, 0x01, 0xd6, 0x22, 0x45, 0xe7, 0x69, 0x60, 0x8a, 0xab, 0x50,
|
|
0xc7, 0x86, 0xeb, 0xad, 0x38, 0x83, 0xae, 0x4b, 0x1b, 0x21, 0x83, 0x4a, 0xac, 0x98, 0x3a, 0x22,
|
|
0x86, 0xfd, 0x40, 0x80, 0x1b, 0x32, 0xb6, 0xb1, 0xae, 0xd6, 0x46, 0xbe, 0x02, 0x7e, 0x87, 0x26,
|
|
0x78, 0xb5, 0x69, 0x31, 0x8c, 0x33, 0xa4, 0x1c, 0x67, 0xc8, 0xb6, 0x74, 0x27, 0x62, 0x88, 0x45,
|
|
0x87, 0x3e, 0xf7, 0x8d, 0x19, 0x06, 0x12, 0xab, 0x96, 0x7f, 0x4a, 0x20, 0x79, 0x05, 0xe7, 0x39,
|
|
0x81, 0xc4, 0xca, 0xce, 0x61, 0x20, 0x31, 0x13, 0xe6, 0x02, 0x92, 0xbf, 0x46, 0x3b, 0x0b, 0x48,
|
|
0x94, 0x77, 0x4e, 0x20, 0x51, 0xc3, 0x88, 0x6b, 0x0c, 0x58, 0x63, 0xf7, 0xc9, 0x79, 0xbc, 0x13,
|
|
0x17, 0xa2, 0x78, 0x67, 0x14, 0x23, 0xce, 0xf8, 0xdd, 0x10, 0x72, 0x67, 0x3a, 0x63, 0x72, 0xc1,
|
|
0xfa, 0x15, 0x71, 0x4b, 0x6d, 0x89, 0xc3, 0xad, 0xef, 0x43, 0x00, 0x86, 0x21, 0x56, 0x36, 0xfd,
|
|
0x4c, 0x70, 0xcb, 0x0d, 0x99, 0x8c, 0xdb, 0xc0, 0xd1, 0xe5, 0xd6, 0x5f, 0x3f, 0xe5, 0xd1, 0xe5,
|
|
0x2b, 0x34, 0xcf, 0x79, 0x74, 0xf1, 0x82, 0x24, 0x3d, 0x16, 0x3c, 0x2b, 0xdc, 0xe7, 0xc2, 0xed,
|
|
0x59, 0xc7, 0x82, 0xfb, 0x68, 0x59, 0xb8, 0x3d, 0x93, 0x73, 0x4e, 0x7b, 0xdc, 0xd7, 0xc6, 0xf0,
|
|
0x51, 0xea, 0x3a, 0x66, 0xae, 0xa3, 0x34, 0x58, 0x15, 0x9e, 0x75, 0x94, 0xba, 0x45, 0xd7, 0xf9,
|
|
0x8e, 0x52, 0xee, 0x30, 0x12, 0xb9, 0x73, 0x9e, 0xe3, 0xd4, 0xce, 0x94, 0xf8, 0x88, 0x7d, 0x61,
|
|
0xae, 0x8a, 0xa8, 0x2d, 0xdd, 0x8b, 0x1b, 0x3d, 0x87, 0xb2, 0xe3, 0xd1, 0x07, 0x64, 0xa8, 0xdf,
|
|
0xf2, 0x1d, 0xe1, 0x5e, 0xc9, 0x77, 0x4a, 0xac, 0x82, 0xa5, 0xe4, 0x58, 0x2c, 0x3f, 0x8a, 0xb3,
|
|
0x60, 0xab, 0xb0, 0xe9, 0xc3, 0x32, 0x57, 0x66, 0x97, 0xf8, 0x77, 0xe3, 0xc4, 0x13, 0x7f, 0x2a,
|
|
0xc0, 0x0d, 0xea, 0x8a, 0xd8, 0x67, 0xce, 0x38, 0xf7, 0xec, 0xce, 0x61, 0x76, 0x48, 0xd7, 0x14,
|
|
0x43, 0xd1, 0xcd, 0x92, 0x49, 0x78, 0x34, 0x6c, 0xfb, 0x0c, 0xed, 0x78, 0x0a, 0xd0, 0x3f, 0x08,
|
|
0x70, 0x85, 0x5f, 0x77, 0x46, 0xbe, 0x47, 0x99, 0xa9, 0xb8, 0x8a, 0x7b, 0x2b, 0x2b, 0xbc, 0x79,
|
|
0x21, 0x19, 0xf6, 0x28, 0x26, 0x7d, 0x39, 0xce, 0xf8, 0x3b, 0xd2, 0x4d, 0xdf, 0x49, 0xc7, 0xe5,
|
|
0x35, 0xd5, 0xb4, 0x4b, 0x6d, 0x9b, 0xaa, 0x20, 0x8e, 0xfe, 0x5b, 0x01, 0x2e, 0xbb, 0x5b, 0xb9,
|
|
0x6f, 0x8c, 0x39, 0xcd, 0x0f, 0x3c, 0x2a, 0xc6, 0xe2, 0xa0, 0x15, 0x67, 0xe1, 0x3b, 0xc5, 0x47,
|
|
0x31, 0x16, 0x7e, 0x14, 0x78, 0xb4, 0xfa, 0xb8, 0xf4, 0x51, 0xf8, 0x81, 0xea, 0x63, 0xf4, 0x4b,
|
|
0x90, 0x2e, 0xab, 0x6a, 0xed, 0x4c, 0x69, 0xb4, 0x4e, 0x62, 0xd1, 0xb0, 0x3d, 0xb5, 0xe8, 0xef,
|
|
0x2b, 0xd4, 0x4f, 0x49, 0x34, 0x25, 0x14, 0x5c, 0x2e, 0x25, 0xc3, 0x31, 0x89, 0xeb, 0xbe, 0x27,
|
|
0xf8, 0x3f, 0x73, 0x68, 0x9d, 0xa0, 0x2f, 0xcc, 0x4c, 0xa4, 0xe8, 0x90, 0xb1, 0x7e, 0x7a, 0x3b,
|
|
0xce, 0x84, 0x5b, 0x85, 0x42, 0xd4, 0x04, 0xff, 0x39, 0x34, 0x80, 0x65, 0x1e, 0xc4, 0xe9, 0xee,
|
|
0x88, 0x1b, 0x39, 0xfe, 0xf8, 0x2f, 0x4e, 0x98, 0x3c, 0xfa, 0x0b, 0x01, 0xd6, 0x5c, 0xd0, 0x7b,
|
|
0x6f, 0x0e, 0x53, 0x53, 0x93, 0x89, 0x1f, 0x21, 0x14, 0x1e, 0x5e, 0x40, 0x82, 0x87, 0xe9, 0x4b,
|
|
0x71, 0x96, 0x6e, 0x4a, 0xeb, 0xd4, 0xd2, 0x2e, 0x11, 0xa2, 0xe6, 0xfa, 0x50, 0xfe, 0x77, 0x02,
|
|
0x5c, 0x76, 0x0d, 0x1e, 0xbf, 0x7f, 0xd8, 0xe8, 0xcd, 0x8b, 0xd4, 0x31, 0x5d, 0xab, 0xbf, 0x74,
|
|
0x31, 0x21, 0x6e, 0x78, 0xfc, 0x1e, 0x23, 0x6d, 0x96, 0xba, 0x7d, 0xe3, 0xb9, 0xd2, 0x27, 0xb7,
|
|
0x2a, 0x22, 0x6c, 0x58, 0xdd, 0xc0, 0x1a, 0xfd, 0x4d, 0x01, 0x36, 0xe8, 0x66, 0xf8, 0x0d, 0x36,
|
|
0xa4, 0xbf, 0x20, 0xf8, 0x29, 0x52, 0xd2, 0x40, 0x45, 0x51, 0xda, 0x8d, 0xb3, 0xeb, 0x1a, 0xda,
|
|
0x28, 0xf9, 0x0a, 0x8b, 0x25, 0xae, 0x8a, 0x5c, 0x8e, 0xbf, 0xeb, 0x1a, 0xc4, 0x67, 0xfb, 0x7f,
|
|
0x6a, 0xd0, 0xd4, 0x1c, 0xd9, 0x6f, 0xd0, 0x00, 0xef, 0xfd, 0x95, 0xf0, 0x83, 0xf2, 0xf7, 0x04,
|
|
0xf4, 0x0e, 0xa4, 0xca, 0x43, 0xa7, 0xb7, 0x55, 0x3e, 0xa9, 0x4a, 0x45, 0xb4, 0xdd, 0x73, 0x1c,
|
|
0xd3, 0x7e, 0x5c, 0x2a, 0x75, 0x35, 0xa7, 0x37, 0x7c, 0xbe, 0xd3, 0x31, 0x06, 0x25, 0x32, 0xb6,
|
|
0x37, 0x03, 0xf3, 0xfd, 0x6e, 0x89, 0xa8, 0xdf, 0x4d, 0x3c, 0xd8, 0x79, 0x58, 0x14, 0xc4, 0xdd,
|
|
0x9c, 0x62, 0x9a, 0x7d, 0x9e, 0x41, 0x95, 0xbe, 0x6d, 0x1b, 0x7a, 0x90, 0xd2, 0xb5, 0xcc, 0xce,
|
|
0xe3, 0x08, 0xcf, 0xe3, 0x08, 0xcf, 0x37, 0xee, 0x4e, 0x1b, 0x91, 0x70, 0xd0, 0x61, 0x9f, 0x2f,
|
|
0x52, 0xd7, 0xbc, 0xf9, 0xbf, 0x01, 0x00, 0x00, 0xff, 0xff, 0xf6, 0x2f, 0x40, 0xc5, 0xb5, 0x3b,
|
|
0x00, 0x00,
|
|
}
|
|
|
|
// Reference imports to suppress errors if they are not otherwise used.
|
|
var _ context.Context
|
|
var _ grpc.ClientConn
|
|
|
|
// This is a compile-time assertion to ensure that this generated file
|
|
// is compatible with the grpc package it is being compiled against.
|
|
const _ = grpc.SupportPackageIsVersion4
|
|
|
|
// AuthServiceClient is the client API for AuthService service.
|
|
//
|
|
// For semantics around ctx use and closing/ending streaming RPCs, please refer to https://godoc.org/google.golang.org/grpc#ClientConn.NewStream.
|
|
type AuthServiceClient interface {
|
|
// Readiness
|
|
Healthz(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*empty.Empty, error)
|
|
Ready(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*empty.Empty, error)
|
|
Validate(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*_struct.Struct, error)
|
|
// Authorization
|
|
GetMyUserSessions(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*UserSessionViews, error)
|
|
//User
|
|
GetMyUser(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*UserView, error)
|
|
GetMyUserProfile(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*UserProfileView, error)
|
|
UpdateMyUserProfile(ctx context.Context, in *UpdateUserProfileRequest, opts ...grpc.CallOption) (*UserProfile, error)
|
|
ChangeMyUserName(ctx context.Context, in *ChangeUserNameRequest, opts ...grpc.CallOption) (*empty.Empty, error)
|
|
GetMyUserEmail(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*UserEmailView, error)
|
|
ChangeMyUserEmail(ctx context.Context, in *UpdateUserEmailRequest, opts ...grpc.CallOption) (*UserEmail, error)
|
|
VerifyMyUserEmail(ctx context.Context, in *VerifyMyUserEmailRequest, opts ...grpc.CallOption) (*empty.Empty, error)
|
|
ResendMyEmailVerificationMail(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*empty.Empty, error)
|
|
GetMyUserPhone(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*UserPhoneView, error)
|
|
ChangeMyUserPhone(ctx context.Context, in *UpdateUserPhoneRequest, opts ...grpc.CallOption) (*UserPhone, error)
|
|
RemoveMyUserPhone(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*empty.Empty, error)
|
|
VerifyMyUserPhone(ctx context.Context, in *VerifyUserPhoneRequest, opts ...grpc.CallOption) (*empty.Empty, error)
|
|
ResendMyPhoneVerificationCode(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*empty.Empty, error)
|
|
GetMyUserAddress(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*UserAddressView, error)
|
|
GetMyUserChanges(ctx context.Context, in *ChangesRequest, opts ...grpc.CallOption) (*Changes, error)
|
|
UpdateMyUserAddress(ctx context.Context, in *UpdateUserAddressRequest, opts ...grpc.CallOption) (*UserAddress, error)
|
|
GetMyMfas(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*MultiFactors, error)
|
|
//Password
|
|
ChangeMyPassword(ctx context.Context, in *PasswordChange, opts ...grpc.CallOption) (*empty.Empty, error)
|
|
GetMyPasswordComplexityPolicy(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*PasswordComplexityPolicy, error)
|
|
//ExternalIDP
|
|
SearchMyExternalIDPs(ctx context.Context, in *ExternalIDPSearchRequest, opts ...grpc.CallOption) (*ExternalIDPSearchResponse, error)
|
|
RemoveMyExternalIDP(ctx context.Context, in *ExternalIDPRemoveRequest, opts ...grpc.CallOption) (*empty.Empty, error)
|
|
// MFA
|
|
AddMfaOTP(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*MfaOtpResponse, error)
|
|
VerifyMfaOTP(ctx context.Context, in *VerifyMfaOtp, opts ...grpc.CallOption) (*empty.Empty, error)
|
|
RemoveMfaOTP(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*empty.Empty, error)
|
|
SearchMyUserGrant(ctx context.Context, in *UserGrantSearchRequest, opts ...grpc.CallOption) (*UserGrantSearchResponse, error)
|
|
SearchMyProjectOrgs(ctx context.Context, in *MyProjectOrgSearchRequest, opts ...grpc.CallOption) (*MyProjectOrgSearchResponse, error)
|
|
//Permission
|
|
GetMyZitadelPermissions(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*MyPermissions, error)
|
|
GetMyProjectPermissions(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*MyPermissions, error)
|
|
}
|
|
|
|
type authServiceClient struct {
|
|
cc *grpc.ClientConn
|
|
}
|
|
|
|
func NewAuthServiceClient(cc *grpc.ClientConn) AuthServiceClient {
|
|
return &authServiceClient{cc}
|
|
}
|
|
|
|
func (c *authServiceClient) Healthz(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*empty.Empty, error) {
|
|
out := new(empty.Empty)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/Healthz", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) Ready(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*empty.Empty, error) {
|
|
out := new(empty.Empty)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/Ready", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) Validate(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*_struct.Struct, error) {
|
|
out := new(_struct.Struct)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/Validate", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) GetMyUserSessions(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*UserSessionViews, error) {
|
|
out := new(UserSessionViews)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/GetMyUserSessions", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) GetMyUser(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*UserView, error) {
|
|
out := new(UserView)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/GetMyUser", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) GetMyUserProfile(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*UserProfileView, error) {
|
|
out := new(UserProfileView)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/GetMyUserProfile", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) UpdateMyUserProfile(ctx context.Context, in *UpdateUserProfileRequest, opts ...grpc.CallOption) (*UserProfile, error) {
|
|
out := new(UserProfile)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/UpdateMyUserProfile", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) ChangeMyUserName(ctx context.Context, in *ChangeUserNameRequest, opts ...grpc.CallOption) (*empty.Empty, error) {
|
|
out := new(empty.Empty)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/ChangeMyUserName", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) GetMyUserEmail(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*UserEmailView, error) {
|
|
out := new(UserEmailView)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/GetMyUserEmail", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) ChangeMyUserEmail(ctx context.Context, in *UpdateUserEmailRequest, opts ...grpc.CallOption) (*UserEmail, error) {
|
|
out := new(UserEmail)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/ChangeMyUserEmail", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) VerifyMyUserEmail(ctx context.Context, in *VerifyMyUserEmailRequest, opts ...grpc.CallOption) (*empty.Empty, error) {
|
|
out := new(empty.Empty)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/VerifyMyUserEmail", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) ResendMyEmailVerificationMail(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*empty.Empty, error) {
|
|
out := new(empty.Empty)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/ResendMyEmailVerificationMail", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) GetMyUserPhone(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*UserPhoneView, error) {
|
|
out := new(UserPhoneView)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/GetMyUserPhone", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) ChangeMyUserPhone(ctx context.Context, in *UpdateUserPhoneRequest, opts ...grpc.CallOption) (*UserPhone, error) {
|
|
out := new(UserPhone)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/ChangeMyUserPhone", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) RemoveMyUserPhone(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*empty.Empty, error) {
|
|
out := new(empty.Empty)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/RemoveMyUserPhone", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) VerifyMyUserPhone(ctx context.Context, in *VerifyUserPhoneRequest, opts ...grpc.CallOption) (*empty.Empty, error) {
|
|
out := new(empty.Empty)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/VerifyMyUserPhone", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) ResendMyPhoneVerificationCode(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*empty.Empty, error) {
|
|
out := new(empty.Empty)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/ResendMyPhoneVerificationCode", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) GetMyUserAddress(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*UserAddressView, error) {
|
|
out := new(UserAddressView)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/GetMyUserAddress", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) GetMyUserChanges(ctx context.Context, in *ChangesRequest, opts ...grpc.CallOption) (*Changes, error) {
|
|
out := new(Changes)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/GetMyUserChanges", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) UpdateMyUserAddress(ctx context.Context, in *UpdateUserAddressRequest, opts ...grpc.CallOption) (*UserAddress, error) {
|
|
out := new(UserAddress)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/UpdateMyUserAddress", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) GetMyMfas(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*MultiFactors, error) {
|
|
out := new(MultiFactors)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/GetMyMfas", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) ChangeMyPassword(ctx context.Context, in *PasswordChange, opts ...grpc.CallOption) (*empty.Empty, error) {
|
|
out := new(empty.Empty)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/ChangeMyPassword", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) GetMyPasswordComplexityPolicy(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*PasswordComplexityPolicy, error) {
|
|
out := new(PasswordComplexityPolicy)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/GetMyPasswordComplexityPolicy", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) SearchMyExternalIDPs(ctx context.Context, in *ExternalIDPSearchRequest, opts ...grpc.CallOption) (*ExternalIDPSearchResponse, error) {
|
|
out := new(ExternalIDPSearchResponse)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/SearchMyExternalIDPs", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) RemoveMyExternalIDP(ctx context.Context, in *ExternalIDPRemoveRequest, opts ...grpc.CallOption) (*empty.Empty, error) {
|
|
out := new(empty.Empty)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/RemoveMyExternalIDP", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) AddMfaOTP(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*MfaOtpResponse, error) {
|
|
out := new(MfaOtpResponse)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/AddMfaOTP", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) VerifyMfaOTP(ctx context.Context, in *VerifyMfaOtp, opts ...grpc.CallOption) (*empty.Empty, error) {
|
|
out := new(empty.Empty)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/VerifyMfaOTP", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) RemoveMfaOTP(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*empty.Empty, error) {
|
|
out := new(empty.Empty)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/RemoveMfaOTP", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) SearchMyUserGrant(ctx context.Context, in *UserGrantSearchRequest, opts ...grpc.CallOption) (*UserGrantSearchResponse, error) {
|
|
out := new(UserGrantSearchResponse)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/SearchMyUserGrant", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) SearchMyProjectOrgs(ctx context.Context, in *MyProjectOrgSearchRequest, opts ...grpc.CallOption) (*MyProjectOrgSearchResponse, error) {
|
|
out := new(MyProjectOrgSearchResponse)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/SearchMyProjectOrgs", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) GetMyZitadelPermissions(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*MyPermissions, error) {
|
|
out := new(MyPermissions)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/GetMyZitadelPermissions", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) GetMyProjectPermissions(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*MyPermissions, error) {
|
|
out := new(MyPermissions)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/GetMyProjectPermissions", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
// AuthServiceServer is the server API for AuthService service.
|
|
type AuthServiceServer interface {
|
|
// Readiness
|
|
Healthz(context.Context, *empty.Empty) (*empty.Empty, error)
|
|
Ready(context.Context, *empty.Empty) (*empty.Empty, error)
|
|
Validate(context.Context, *empty.Empty) (*_struct.Struct, error)
|
|
// Authorization
|
|
GetMyUserSessions(context.Context, *empty.Empty) (*UserSessionViews, error)
|
|
//User
|
|
GetMyUser(context.Context, *empty.Empty) (*UserView, error)
|
|
GetMyUserProfile(context.Context, *empty.Empty) (*UserProfileView, error)
|
|
UpdateMyUserProfile(context.Context, *UpdateUserProfileRequest) (*UserProfile, error)
|
|
ChangeMyUserName(context.Context, *ChangeUserNameRequest) (*empty.Empty, error)
|
|
GetMyUserEmail(context.Context, *empty.Empty) (*UserEmailView, error)
|
|
ChangeMyUserEmail(context.Context, *UpdateUserEmailRequest) (*UserEmail, error)
|
|
VerifyMyUserEmail(context.Context, *VerifyMyUserEmailRequest) (*empty.Empty, error)
|
|
ResendMyEmailVerificationMail(context.Context, *empty.Empty) (*empty.Empty, error)
|
|
GetMyUserPhone(context.Context, *empty.Empty) (*UserPhoneView, error)
|
|
ChangeMyUserPhone(context.Context, *UpdateUserPhoneRequest) (*UserPhone, error)
|
|
RemoveMyUserPhone(context.Context, *empty.Empty) (*empty.Empty, error)
|
|
VerifyMyUserPhone(context.Context, *VerifyUserPhoneRequest) (*empty.Empty, error)
|
|
ResendMyPhoneVerificationCode(context.Context, *empty.Empty) (*empty.Empty, error)
|
|
GetMyUserAddress(context.Context, *empty.Empty) (*UserAddressView, error)
|
|
GetMyUserChanges(context.Context, *ChangesRequest) (*Changes, error)
|
|
UpdateMyUserAddress(context.Context, *UpdateUserAddressRequest) (*UserAddress, error)
|
|
GetMyMfas(context.Context, *empty.Empty) (*MultiFactors, error)
|
|
//Password
|
|
ChangeMyPassword(context.Context, *PasswordChange) (*empty.Empty, error)
|
|
GetMyPasswordComplexityPolicy(context.Context, *empty.Empty) (*PasswordComplexityPolicy, error)
|
|
//ExternalIDP
|
|
SearchMyExternalIDPs(context.Context, *ExternalIDPSearchRequest) (*ExternalIDPSearchResponse, error)
|
|
RemoveMyExternalIDP(context.Context, *ExternalIDPRemoveRequest) (*empty.Empty, error)
|
|
// MFA
|
|
AddMfaOTP(context.Context, *empty.Empty) (*MfaOtpResponse, error)
|
|
VerifyMfaOTP(context.Context, *VerifyMfaOtp) (*empty.Empty, error)
|
|
RemoveMfaOTP(context.Context, *empty.Empty) (*empty.Empty, error)
|
|
SearchMyUserGrant(context.Context, *UserGrantSearchRequest) (*UserGrantSearchResponse, error)
|
|
SearchMyProjectOrgs(context.Context, *MyProjectOrgSearchRequest) (*MyProjectOrgSearchResponse, error)
|
|
//Permission
|
|
GetMyZitadelPermissions(context.Context, *empty.Empty) (*MyPermissions, error)
|
|
GetMyProjectPermissions(context.Context, *empty.Empty) (*MyPermissions, error)
|
|
}
|
|
|
|
// UnimplementedAuthServiceServer can be embedded to have forward compatible implementations.
|
|
type UnimplementedAuthServiceServer struct {
|
|
}
|
|
|
|
func (*UnimplementedAuthServiceServer) Healthz(ctx context.Context, req *empty.Empty) (*empty.Empty, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method Healthz not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) Ready(ctx context.Context, req *empty.Empty) (*empty.Empty, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method Ready not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) Validate(ctx context.Context, req *empty.Empty) (*_struct.Struct, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method Validate not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) GetMyUserSessions(ctx context.Context, req *empty.Empty) (*UserSessionViews, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method GetMyUserSessions not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) GetMyUser(ctx context.Context, req *empty.Empty) (*UserView, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method GetMyUser not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) GetMyUserProfile(ctx context.Context, req *empty.Empty) (*UserProfileView, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method GetMyUserProfile not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) UpdateMyUserProfile(ctx context.Context, req *UpdateUserProfileRequest) (*UserProfile, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method UpdateMyUserProfile not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) ChangeMyUserName(ctx context.Context, req *ChangeUserNameRequest) (*empty.Empty, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method ChangeMyUserName not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) GetMyUserEmail(ctx context.Context, req *empty.Empty) (*UserEmailView, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method GetMyUserEmail not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) ChangeMyUserEmail(ctx context.Context, req *UpdateUserEmailRequest) (*UserEmail, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method ChangeMyUserEmail not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) VerifyMyUserEmail(ctx context.Context, req *VerifyMyUserEmailRequest) (*empty.Empty, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method VerifyMyUserEmail not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) ResendMyEmailVerificationMail(ctx context.Context, req *empty.Empty) (*empty.Empty, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method ResendMyEmailVerificationMail not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) GetMyUserPhone(ctx context.Context, req *empty.Empty) (*UserPhoneView, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method GetMyUserPhone not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) ChangeMyUserPhone(ctx context.Context, req *UpdateUserPhoneRequest) (*UserPhone, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method ChangeMyUserPhone not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) RemoveMyUserPhone(ctx context.Context, req *empty.Empty) (*empty.Empty, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method RemoveMyUserPhone not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) VerifyMyUserPhone(ctx context.Context, req *VerifyUserPhoneRequest) (*empty.Empty, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method VerifyMyUserPhone not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) ResendMyPhoneVerificationCode(ctx context.Context, req *empty.Empty) (*empty.Empty, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method ResendMyPhoneVerificationCode not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) GetMyUserAddress(ctx context.Context, req *empty.Empty) (*UserAddressView, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method GetMyUserAddress not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) GetMyUserChanges(ctx context.Context, req *ChangesRequest) (*Changes, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method GetMyUserChanges not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) UpdateMyUserAddress(ctx context.Context, req *UpdateUserAddressRequest) (*UserAddress, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method UpdateMyUserAddress not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) GetMyMfas(ctx context.Context, req *empty.Empty) (*MultiFactors, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method GetMyMfas not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) ChangeMyPassword(ctx context.Context, req *PasswordChange) (*empty.Empty, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method ChangeMyPassword not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) GetMyPasswordComplexityPolicy(ctx context.Context, req *empty.Empty) (*PasswordComplexityPolicy, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method GetMyPasswordComplexityPolicy not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) SearchMyExternalIDPs(ctx context.Context, req *ExternalIDPSearchRequest) (*ExternalIDPSearchResponse, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method SearchMyExternalIDPs not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) RemoveMyExternalIDP(ctx context.Context, req *ExternalIDPRemoveRequest) (*empty.Empty, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method RemoveMyExternalIDP not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) AddMfaOTP(ctx context.Context, req *empty.Empty) (*MfaOtpResponse, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method AddMfaOTP not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) VerifyMfaOTP(ctx context.Context, req *VerifyMfaOtp) (*empty.Empty, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method VerifyMfaOTP not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) RemoveMfaOTP(ctx context.Context, req *empty.Empty) (*empty.Empty, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method RemoveMfaOTP not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) SearchMyUserGrant(ctx context.Context, req *UserGrantSearchRequest) (*UserGrantSearchResponse, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method SearchMyUserGrant not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) SearchMyProjectOrgs(ctx context.Context, req *MyProjectOrgSearchRequest) (*MyProjectOrgSearchResponse, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method SearchMyProjectOrgs not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) GetMyZitadelPermissions(ctx context.Context, req *empty.Empty) (*MyPermissions, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method GetMyZitadelPermissions not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) GetMyProjectPermissions(ctx context.Context, req *empty.Empty) (*MyPermissions, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method GetMyProjectPermissions not implemented")
|
|
}
|
|
|
|
func RegisterAuthServiceServer(s *grpc.Server, srv AuthServiceServer) {
|
|
s.RegisterService(&_AuthService_serviceDesc, srv)
|
|
}
|
|
|
|
func _AuthService_Healthz_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(empty.Empty)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).Healthz(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/Healthz",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).Healthz(ctx, req.(*empty.Empty))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_Ready_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(empty.Empty)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).Ready(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/Ready",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).Ready(ctx, req.(*empty.Empty))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_Validate_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(empty.Empty)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).Validate(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/Validate",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).Validate(ctx, req.(*empty.Empty))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_GetMyUserSessions_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(empty.Empty)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).GetMyUserSessions(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/GetMyUserSessions",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).GetMyUserSessions(ctx, req.(*empty.Empty))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_GetMyUser_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(empty.Empty)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).GetMyUser(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/GetMyUser",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).GetMyUser(ctx, req.(*empty.Empty))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_GetMyUserProfile_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(empty.Empty)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).GetMyUserProfile(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/GetMyUserProfile",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).GetMyUserProfile(ctx, req.(*empty.Empty))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_UpdateMyUserProfile_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(UpdateUserProfileRequest)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).UpdateMyUserProfile(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/UpdateMyUserProfile",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).UpdateMyUserProfile(ctx, req.(*UpdateUserProfileRequest))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_ChangeMyUserName_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(ChangeUserNameRequest)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).ChangeMyUserName(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/ChangeMyUserName",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).ChangeMyUserName(ctx, req.(*ChangeUserNameRequest))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_GetMyUserEmail_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(empty.Empty)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).GetMyUserEmail(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/GetMyUserEmail",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).GetMyUserEmail(ctx, req.(*empty.Empty))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_ChangeMyUserEmail_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(UpdateUserEmailRequest)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).ChangeMyUserEmail(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/ChangeMyUserEmail",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).ChangeMyUserEmail(ctx, req.(*UpdateUserEmailRequest))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_VerifyMyUserEmail_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(VerifyMyUserEmailRequest)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).VerifyMyUserEmail(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/VerifyMyUserEmail",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).VerifyMyUserEmail(ctx, req.(*VerifyMyUserEmailRequest))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_ResendMyEmailVerificationMail_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(empty.Empty)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).ResendMyEmailVerificationMail(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/ResendMyEmailVerificationMail",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).ResendMyEmailVerificationMail(ctx, req.(*empty.Empty))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_GetMyUserPhone_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(empty.Empty)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).GetMyUserPhone(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/GetMyUserPhone",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).GetMyUserPhone(ctx, req.(*empty.Empty))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_ChangeMyUserPhone_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(UpdateUserPhoneRequest)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).ChangeMyUserPhone(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/ChangeMyUserPhone",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).ChangeMyUserPhone(ctx, req.(*UpdateUserPhoneRequest))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_RemoveMyUserPhone_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(empty.Empty)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).RemoveMyUserPhone(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/RemoveMyUserPhone",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).RemoveMyUserPhone(ctx, req.(*empty.Empty))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_VerifyMyUserPhone_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(VerifyUserPhoneRequest)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).VerifyMyUserPhone(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/VerifyMyUserPhone",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).VerifyMyUserPhone(ctx, req.(*VerifyUserPhoneRequest))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_ResendMyPhoneVerificationCode_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(empty.Empty)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).ResendMyPhoneVerificationCode(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/ResendMyPhoneVerificationCode",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).ResendMyPhoneVerificationCode(ctx, req.(*empty.Empty))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_GetMyUserAddress_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(empty.Empty)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).GetMyUserAddress(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/GetMyUserAddress",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).GetMyUserAddress(ctx, req.(*empty.Empty))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_GetMyUserChanges_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(ChangesRequest)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).GetMyUserChanges(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/GetMyUserChanges",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).GetMyUserChanges(ctx, req.(*ChangesRequest))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_UpdateMyUserAddress_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(UpdateUserAddressRequest)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).UpdateMyUserAddress(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/UpdateMyUserAddress",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).UpdateMyUserAddress(ctx, req.(*UpdateUserAddressRequest))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_GetMyMfas_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(empty.Empty)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).GetMyMfas(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/GetMyMfas",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).GetMyMfas(ctx, req.(*empty.Empty))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_ChangeMyPassword_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(PasswordChange)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).ChangeMyPassword(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/ChangeMyPassword",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).ChangeMyPassword(ctx, req.(*PasswordChange))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_GetMyPasswordComplexityPolicy_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(empty.Empty)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).GetMyPasswordComplexityPolicy(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/GetMyPasswordComplexityPolicy",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).GetMyPasswordComplexityPolicy(ctx, req.(*empty.Empty))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_SearchMyExternalIDPs_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(ExternalIDPSearchRequest)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).SearchMyExternalIDPs(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/SearchMyExternalIDPs",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).SearchMyExternalIDPs(ctx, req.(*ExternalIDPSearchRequest))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_RemoveMyExternalIDP_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(ExternalIDPRemoveRequest)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).RemoveMyExternalIDP(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/RemoveMyExternalIDP",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).RemoveMyExternalIDP(ctx, req.(*ExternalIDPRemoveRequest))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_AddMfaOTP_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(empty.Empty)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).AddMfaOTP(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/AddMfaOTP",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).AddMfaOTP(ctx, req.(*empty.Empty))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_VerifyMfaOTP_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(VerifyMfaOtp)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).VerifyMfaOTP(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/VerifyMfaOTP",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).VerifyMfaOTP(ctx, req.(*VerifyMfaOtp))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_RemoveMfaOTP_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(empty.Empty)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).RemoveMfaOTP(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/RemoveMfaOTP",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).RemoveMfaOTP(ctx, req.(*empty.Empty))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_SearchMyUserGrant_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(UserGrantSearchRequest)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).SearchMyUserGrant(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/SearchMyUserGrant",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).SearchMyUserGrant(ctx, req.(*UserGrantSearchRequest))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_SearchMyProjectOrgs_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(MyProjectOrgSearchRequest)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).SearchMyProjectOrgs(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/SearchMyProjectOrgs",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).SearchMyProjectOrgs(ctx, req.(*MyProjectOrgSearchRequest))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_GetMyZitadelPermissions_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(empty.Empty)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).GetMyZitadelPermissions(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/GetMyZitadelPermissions",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).GetMyZitadelPermissions(ctx, req.(*empty.Empty))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_GetMyProjectPermissions_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(empty.Empty)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).GetMyProjectPermissions(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/GetMyProjectPermissions",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).GetMyProjectPermissions(ctx, req.(*empty.Empty))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
var _AuthService_serviceDesc = grpc.ServiceDesc{
|
|
ServiceName: "caos.zitadel.auth.api.v1.AuthService",
|
|
HandlerType: (*AuthServiceServer)(nil),
|
|
Methods: []grpc.MethodDesc{
|
|
{
|
|
MethodName: "Healthz",
|
|
Handler: _AuthService_Healthz_Handler,
|
|
},
|
|
{
|
|
MethodName: "Ready",
|
|
Handler: _AuthService_Ready_Handler,
|
|
},
|
|
{
|
|
MethodName: "Validate",
|
|
Handler: _AuthService_Validate_Handler,
|
|
},
|
|
{
|
|
MethodName: "GetMyUserSessions",
|
|
Handler: _AuthService_GetMyUserSessions_Handler,
|
|
},
|
|
{
|
|
MethodName: "GetMyUser",
|
|
Handler: _AuthService_GetMyUser_Handler,
|
|
},
|
|
{
|
|
MethodName: "GetMyUserProfile",
|
|
Handler: _AuthService_GetMyUserProfile_Handler,
|
|
},
|
|
{
|
|
MethodName: "UpdateMyUserProfile",
|
|
Handler: _AuthService_UpdateMyUserProfile_Handler,
|
|
},
|
|
{
|
|
MethodName: "ChangeMyUserName",
|
|
Handler: _AuthService_ChangeMyUserName_Handler,
|
|
},
|
|
{
|
|
MethodName: "GetMyUserEmail",
|
|
Handler: _AuthService_GetMyUserEmail_Handler,
|
|
},
|
|
{
|
|
MethodName: "ChangeMyUserEmail",
|
|
Handler: _AuthService_ChangeMyUserEmail_Handler,
|
|
},
|
|
{
|
|
MethodName: "VerifyMyUserEmail",
|
|
Handler: _AuthService_VerifyMyUserEmail_Handler,
|
|
},
|
|
{
|
|
MethodName: "ResendMyEmailVerificationMail",
|
|
Handler: _AuthService_ResendMyEmailVerificationMail_Handler,
|
|
},
|
|
{
|
|
MethodName: "GetMyUserPhone",
|
|
Handler: _AuthService_GetMyUserPhone_Handler,
|
|
},
|
|
{
|
|
MethodName: "ChangeMyUserPhone",
|
|
Handler: _AuthService_ChangeMyUserPhone_Handler,
|
|
},
|
|
{
|
|
MethodName: "RemoveMyUserPhone",
|
|
Handler: _AuthService_RemoveMyUserPhone_Handler,
|
|
},
|
|
{
|
|
MethodName: "VerifyMyUserPhone",
|
|
Handler: _AuthService_VerifyMyUserPhone_Handler,
|
|
},
|
|
{
|
|
MethodName: "ResendMyPhoneVerificationCode",
|
|
Handler: _AuthService_ResendMyPhoneVerificationCode_Handler,
|
|
},
|
|
{
|
|
MethodName: "GetMyUserAddress",
|
|
Handler: _AuthService_GetMyUserAddress_Handler,
|
|
},
|
|
{
|
|
MethodName: "GetMyUserChanges",
|
|
Handler: _AuthService_GetMyUserChanges_Handler,
|
|
},
|
|
{
|
|
MethodName: "UpdateMyUserAddress",
|
|
Handler: _AuthService_UpdateMyUserAddress_Handler,
|
|
},
|
|
{
|
|
MethodName: "GetMyMfas",
|
|
Handler: _AuthService_GetMyMfas_Handler,
|
|
},
|
|
{
|
|
MethodName: "ChangeMyPassword",
|
|
Handler: _AuthService_ChangeMyPassword_Handler,
|
|
},
|
|
{
|
|
MethodName: "GetMyPasswordComplexityPolicy",
|
|
Handler: _AuthService_GetMyPasswordComplexityPolicy_Handler,
|
|
},
|
|
{
|
|
MethodName: "SearchMyExternalIDPs",
|
|
Handler: _AuthService_SearchMyExternalIDPs_Handler,
|
|
},
|
|
{
|
|
MethodName: "RemoveMyExternalIDP",
|
|
Handler: _AuthService_RemoveMyExternalIDP_Handler,
|
|
},
|
|
{
|
|
MethodName: "AddMfaOTP",
|
|
Handler: _AuthService_AddMfaOTP_Handler,
|
|
},
|
|
{
|
|
MethodName: "VerifyMfaOTP",
|
|
Handler: _AuthService_VerifyMfaOTP_Handler,
|
|
},
|
|
{
|
|
MethodName: "RemoveMfaOTP",
|
|
Handler: _AuthService_RemoveMfaOTP_Handler,
|
|
},
|
|
{
|
|
MethodName: "SearchMyUserGrant",
|
|
Handler: _AuthService_SearchMyUserGrant_Handler,
|
|
},
|
|
{
|
|
MethodName: "SearchMyProjectOrgs",
|
|
Handler: _AuthService_SearchMyProjectOrgs_Handler,
|
|
},
|
|
{
|
|
MethodName: "GetMyZitadelPermissions",
|
|
Handler: _AuthService_GetMyZitadelPermissions_Handler,
|
|
},
|
|
{
|
|
MethodName: "GetMyProjectPermissions",
|
|
Handler: _AuthService_GetMyProjectPermissions_Handler,
|
|
},
|
|
},
|
|
Streams: []grpc.StreamDesc{},
|
|
Metadata: "auth.proto",
|
|
}
|