mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
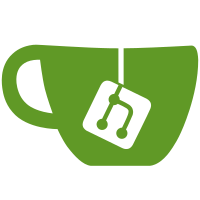
* feat: add mfa to login policy * feat: add mfa to login policy * feat: add mfa to login policy * feat: add mfa to login policy * feat: add mfa to login policy on org * feat: add mfa to login policy on org * feat: append events on policy views * feat: iam login policy mfa definition * feat: login policies on orgs * feat: configured mfas in login process * feat: configured mfas in login process * Update internal/ui/login/static/i18n/en.yaml Co-authored-by: Livio Amstutz <livio.a@gmail.com> * fix: rename software and hardware mfas * fix: pr requests * fix user mfa * fix: test * fix: oidc version * fix: oidc version * fix: proto gen Co-authored-by: Livio Amstutz <livio.a@gmail.com> Co-authored-by: Max Peintner <max@caos.ch>
6096 lines
148 KiB
Go
6096 lines
148 KiB
Go
// Code generated by protoc-gen-validate. DO NOT EDIT.
|
|
// source: admin.proto
|
|
|
|
package admin
|
|
|
|
import (
|
|
"bytes"
|
|
"errors"
|
|
"fmt"
|
|
"net"
|
|
"net/mail"
|
|
"net/url"
|
|
"regexp"
|
|
"strings"
|
|
"time"
|
|
"unicode/utf8"
|
|
|
|
"github.com/golang/protobuf/ptypes"
|
|
)
|
|
|
|
// ensure the imports are used
|
|
var (
|
|
_ = bytes.MinRead
|
|
_ = errors.New("")
|
|
_ = fmt.Print
|
|
_ = utf8.UTFMax
|
|
_ = (*regexp.Regexp)(nil)
|
|
_ = (*strings.Reader)(nil)
|
|
_ = net.IPv4len
|
|
_ = time.Duration(0)
|
|
_ = (*url.URL)(nil)
|
|
_ = (*mail.Address)(nil)
|
|
_ = ptypes.DynamicAny{}
|
|
)
|
|
|
|
// define the regex for a UUID once up-front
|
|
var _admin_uuidPattern = regexp.MustCompile("^[0-9a-fA-F]{8}-[0-9a-fA-F]{4}-[0-9a-fA-F]{4}-[0-9a-fA-F]{4}-[0-9a-fA-F]{12}$")
|
|
|
|
// Validate checks the field values on OrgID with the rules defined in the
|
|
// proto definition for this message. If any rules are violated, an error is returned.
|
|
func (m *OrgID) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetId()) < 1 {
|
|
return OrgIDValidationError{
|
|
field: "Id",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// OrgIDValidationError is the validation error returned by OrgID.Validate if
|
|
// the designated constraints aren't met.
|
|
type OrgIDValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e OrgIDValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e OrgIDValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e OrgIDValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e OrgIDValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e OrgIDValidationError) ErrorName() string { return "OrgIDValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e OrgIDValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sOrgID.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = OrgIDValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = OrgIDValidationError{}
|
|
|
|
// Validate checks the field values on UniqueOrgRequest with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *UniqueOrgRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetName()) < 1 {
|
|
return UniqueOrgRequestValidationError{
|
|
field: "Name",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetDomain()) < 1 {
|
|
return UniqueOrgRequestValidationError{
|
|
field: "Domain",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// UniqueOrgRequestValidationError is the validation error returned by
|
|
// UniqueOrgRequest.Validate if the designated constraints aren't met.
|
|
type UniqueOrgRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UniqueOrgRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UniqueOrgRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UniqueOrgRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UniqueOrgRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UniqueOrgRequestValidationError) ErrorName() string { return "UniqueOrgRequestValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UniqueOrgRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUniqueOrgRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UniqueOrgRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UniqueOrgRequestValidationError{}
|
|
|
|
// Validate checks the field values on UniqueOrgResponse with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *UniqueOrgResponse) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for IsUnique
|
|
|
|
return nil
|
|
}
|
|
|
|
// UniqueOrgResponseValidationError is the validation error returned by
|
|
// UniqueOrgResponse.Validate if the designated constraints aren't met.
|
|
type UniqueOrgResponseValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UniqueOrgResponseValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UniqueOrgResponseValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UniqueOrgResponseValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UniqueOrgResponseValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UniqueOrgResponseValidationError) ErrorName() string {
|
|
return "UniqueOrgResponseValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UniqueOrgResponseValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUniqueOrgResponse.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UniqueOrgResponseValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UniqueOrgResponseValidationError{}
|
|
|
|
// Validate checks the field values on Org with the rules defined in the proto
|
|
// definition for this message. If any rules are violated, an error is returned.
|
|
func (m *Org) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Id
|
|
|
|
// no validation rules for State
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return OrgValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return OrgValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for Name
|
|
|
|
// no validation rules for Domain
|
|
|
|
return nil
|
|
}
|
|
|
|
// OrgValidationError is the validation error returned by Org.Validate if the
|
|
// designated constraints aren't met.
|
|
type OrgValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e OrgValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e OrgValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e OrgValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e OrgValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e OrgValidationError) ErrorName() string { return "OrgValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e OrgValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sOrg.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = OrgValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = OrgValidationError{}
|
|
|
|
// Validate checks the field values on OrgSearchRequest with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *OrgSearchRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
if _, ok := _OrgSearchRequest_SortingColumn_NotInLookup[m.GetSortingColumn()]; ok {
|
|
return OrgSearchRequestValidationError{
|
|
field: "SortingColumn",
|
|
reason: "value must not be in list [0]",
|
|
}
|
|
}
|
|
|
|
// no validation rules for Asc
|
|
|
|
for idx, item := range m.GetQueries() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return OrgSearchRequestValidationError{
|
|
field: fmt.Sprintf("Queries[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// OrgSearchRequestValidationError is the validation error returned by
|
|
// OrgSearchRequest.Validate if the designated constraints aren't met.
|
|
type OrgSearchRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e OrgSearchRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e OrgSearchRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e OrgSearchRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e OrgSearchRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e OrgSearchRequestValidationError) ErrorName() string { return "OrgSearchRequestValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e OrgSearchRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sOrgSearchRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = OrgSearchRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = OrgSearchRequestValidationError{}
|
|
|
|
var _OrgSearchRequest_SortingColumn_NotInLookup = map[OrgSearchKey]struct{}{
|
|
0: {},
|
|
}
|
|
|
|
// Validate checks the field values on OrgSearchQuery with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *OrgSearchQuery) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if _, ok := _OrgSearchQuery_Key_NotInLookup[m.GetKey()]; ok {
|
|
return OrgSearchQueryValidationError{
|
|
field: "Key",
|
|
reason: "value must not be in list [0]",
|
|
}
|
|
}
|
|
|
|
// no validation rules for Method
|
|
|
|
// no validation rules for Value
|
|
|
|
return nil
|
|
}
|
|
|
|
// OrgSearchQueryValidationError is the validation error returned by
|
|
// OrgSearchQuery.Validate if the designated constraints aren't met.
|
|
type OrgSearchQueryValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e OrgSearchQueryValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e OrgSearchQueryValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e OrgSearchQueryValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e OrgSearchQueryValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e OrgSearchQueryValidationError) ErrorName() string { return "OrgSearchQueryValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e OrgSearchQueryValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sOrgSearchQuery.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = OrgSearchQueryValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = OrgSearchQueryValidationError{}
|
|
|
|
var _OrgSearchQuery_Key_NotInLookup = map[OrgSearchKey]struct{}{
|
|
0: {},
|
|
}
|
|
|
|
// Validate checks the field values on OrgSearchResponse with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *OrgSearchResponse) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
// no validation rules for TotalResult
|
|
|
|
for idx, item := range m.GetResult() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return OrgSearchResponseValidationError{
|
|
field: fmt.Sprintf("Result[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
// no validation rules for ProcessedSequence
|
|
|
|
if v, ok := interface{}(m.GetViewTimestamp()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return OrgSearchResponseValidationError{
|
|
field: "ViewTimestamp",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// OrgSearchResponseValidationError is the validation error returned by
|
|
// OrgSearchResponse.Validate if the designated constraints aren't met.
|
|
type OrgSearchResponseValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e OrgSearchResponseValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e OrgSearchResponseValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e OrgSearchResponseValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e OrgSearchResponseValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e OrgSearchResponseValidationError) ErrorName() string {
|
|
return "OrgSearchResponseValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e OrgSearchResponseValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sOrgSearchResponse.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = OrgSearchResponseValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = OrgSearchResponseValidationError{}
|
|
|
|
// Validate checks the field values on OrgSetUpRequest with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *OrgSetUpRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if m.GetOrg() == nil {
|
|
return OrgSetUpRequestValidationError{
|
|
field: "Org",
|
|
reason: "value is required",
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetOrg()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return OrgSetUpRequestValidationError{
|
|
field: "Org",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if m.GetUser() == nil {
|
|
return OrgSetUpRequestValidationError{
|
|
field: "User",
|
|
reason: "value is required",
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetUser()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return OrgSetUpRequestValidationError{
|
|
field: "User",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// OrgSetUpRequestValidationError is the validation error returned by
|
|
// OrgSetUpRequest.Validate if the designated constraints aren't met.
|
|
type OrgSetUpRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e OrgSetUpRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e OrgSetUpRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e OrgSetUpRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e OrgSetUpRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e OrgSetUpRequestValidationError) ErrorName() string { return "OrgSetUpRequestValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e OrgSetUpRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sOrgSetUpRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = OrgSetUpRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = OrgSetUpRequestValidationError{}
|
|
|
|
// Validate checks the field values on OrgSetUpResponse with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *OrgSetUpResponse) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetOrg()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return OrgSetUpResponseValidationError{
|
|
field: "Org",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetUser()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return OrgSetUpResponseValidationError{
|
|
field: "User",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// OrgSetUpResponseValidationError is the validation error returned by
|
|
// OrgSetUpResponse.Validate if the designated constraints aren't met.
|
|
type OrgSetUpResponseValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e OrgSetUpResponseValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e OrgSetUpResponseValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e OrgSetUpResponseValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e OrgSetUpResponseValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e OrgSetUpResponseValidationError) ErrorName() string { return "OrgSetUpResponseValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e OrgSetUpResponseValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sOrgSetUpResponse.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = OrgSetUpResponseValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = OrgSetUpResponseValidationError{}
|
|
|
|
// Validate checks the field values on CreateUserRequest with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *CreateUserRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if !_CreateUserRequest_UserName_Pattern.MatchString(m.GetUserName()) {
|
|
return CreateUserRequestValidationError{
|
|
field: "UserName",
|
|
reason: "value does not match regex pattern \"^[^[:space:]]{1,200}$\"",
|
|
}
|
|
}
|
|
|
|
switch m.User.(type) {
|
|
|
|
case *CreateUserRequest_Human:
|
|
|
|
if v, ok := interface{}(m.GetHuman()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return CreateUserRequestValidationError{
|
|
field: "Human",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
case *CreateUserRequest_Machine:
|
|
|
|
if v, ok := interface{}(m.GetMachine()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return CreateUserRequestValidationError{
|
|
field: "Machine",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
default:
|
|
return CreateUserRequestValidationError{
|
|
field: "User",
|
|
reason: "value is required",
|
|
}
|
|
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// CreateUserRequestValidationError is the validation error returned by
|
|
// CreateUserRequest.Validate if the designated constraints aren't met.
|
|
type CreateUserRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e CreateUserRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e CreateUserRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e CreateUserRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e CreateUserRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e CreateUserRequestValidationError) ErrorName() string {
|
|
return "CreateUserRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e CreateUserRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sCreateUserRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = CreateUserRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = CreateUserRequestValidationError{}
|
|
|
|
var _CreateUserRequest_UserName_Pattern = regexp.MustCompile("^[^[:space:]]{1,200}$")
|
|
|
|
// Validate checks the field values on CreateHumanRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *CreateHumanRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if l := utf8.RuneCountInString(m.GetFirstName()); l < 1 || l > 200 {
|
|
return CreateHumanRequestValidationError{
|
|
field: "FirstName",
|
|
reason: "value length must be between 1 and 200 runes, inclusive",
|
|
}
|
|
}
|
|
|
|
if l := utf8.RuneCountInString(m.GetLastName()); l < 1 || l > 200 {
|
|
return CreateHumanRequestValidationError{
|
|
field: "LastName",
|
|
reason: "value length must be between 1 and 200 runes, inclusive",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetNickName()) > 200 {
|
|
return CreateHumanRequestValidationError{
|
|
field: "NickName",
|
|
reason: "value length must be at most 200 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetPreferredLanguage()) > 200 {
|
|
return CreateHumanRequestValidationError{
|
|
field: "PreferredLanguage",
|
|
reason: "value length must be at most 200 runes",
|
|
}
|
|
}
|
|
|
|
// no validation rules for Gender
|
|
|
|
if l := utf8.RuneCountInString(m.GetEmail()); l < 1 || l > 200 {
|
|
return CreateHumanRequestValidationError{
|
|
field: "Email",
|
|
reason: "value length must be between 1 and 200 runes, inclusive",
|
|
}
|
|
}
|
|
|
|
if err := m._validateEmail(m.GetEmail()); err != nil {
|
|
return CreateHumanRequestValidationError{
|
|
field: "Email",
|
|
reason: "value must be a valid email address",
|
|
cause: err,
|
|
}
|
|
}
|
|
|
|
// no validation rules for IsEmailVerified
|
|
|
|
if utf8.RuneCountInString(m.GetPhone()) > 20 {
|
|
return CreateHumanRequestValidationError{
|
|
field: "Phone",
|
|
reason: "value length must be at most 20 runes",
|
|
}
|
|
}
|
|
|
|
// no validation rules for IsPhoneVerified
|
|
|
|
if utf8.RuneCountInString(m.GetCountry()) > 200 {
|
|
return CreateHumanRequestValidationError{
|
|
field: "Country",
|
|
reason: "value length must be at most 200 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetLocality()) > 200 {
|
|
return CreateHumanRequestValidationError{
|
|
field: "Locality",
|
|
reason: "value length must be at most 200 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetPostalCode()) > 200 {
|
|
return CreateHumanRequestValidationError{
|
|
field: "PostalCode",
|
|
reason: "value length must be at most 200 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetRegion()) > 200 {
|
|
return CreateHumanRequestValidationError{
|
|
field: "Region",
|
|
reason: "value length must be at most 200 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetStreetAddress()) > 200 {
|
|
return CreateHumanRequestValidationError{
|
|
field: "StreetAddress",
|
|
reason: "value length must be at most 200 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetPassword()) > 72 {
|
|
return CreateHumanRequestValidationError{
|
|
field: "Password",
|
|
reason: "value length must be at most 72 runes",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
func (m *CreateHumanRequest) _validateHostname(host string) error {
|
|
s := strings.ToLower(strings.TrimSuffix(host, "."))
|
|
|
|
if len(host) > 253 {
|
|
return errors.New("hostname cannot exceed 253 characters")
|
|
}
|
|
|
|
for _, part := range strings.Split(s, ".") {
|
|
if l := len(part); l == 0 || l > 63 {
|
|
return errors.New("hostname part must be non-empty and cannot exceed 63 characters")
|
|
}
|
|
|
|
if part[0] == '-' {
|
|
return errors.New("hostname parts cannot begin with hyphens")
|
|
}
|
|
|
|
if part[len(part)-1] == '-' {
|
|
return errors.New("hostname parts cannot end with hyphens")
|
|
}
|
|
|
|
for _, r := range part {
|
|
if (r < 'a' || r > 'z') && (r < '0' || r > '9') && r != '-' {
|
|
return fmt.Errorf("hostname parts can only contain alphanumeric characters or hyphens, got %q", string(r))
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
func (m *CreateHumanRequest) _validateEmail(addr string) error {
|
|
a, err := mail.ParseAddress(addr)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
addr = a.Address
|
|
|
|
if len(addr) > 254 {
|
|
return errors.New("email addresses cannot exceed 254 characters")
|
|
}
|
|
|
|
parts := strings.SplitN(addr, "@", 2)
|
|
|
|
if len(parts[0]) > 64 {
|
|
return errors.New("email address local phrase cannot exceed 64 characters")
|
|
}
|
|
|
|
return m._validateHostname(parts[1])
|
|
}
|
|
|
|
// CreateHumanRequestValidationError is the validation error returned by
|
|
// CreateHumanRequest.Validate if the designated constraints aren't met.
|
|
type CreateHumanRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e CreateHumanRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e CreateHumanRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e CreateHumanRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e CreateHumanRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e CreateHumanRequestValidationError) ErrorName() string {
|
|
return "CreateHumanRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e CreateHumanRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sCreateHumanRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = CreateHumanRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = CreateHumanRequestValidationError{}
|
|
|
|
// Validate checks the field values on CreateMachineRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *CreateMachineRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if l := utf8.RuneCountInString(m.GetName()); l < 1 || l > 200 {
|
|
return CreateMachineRequestValidationError{
|
|
field: "Name",
|
|
reason: "value length must be between 1 and 200 runes, inclusive",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetDescription()) > 500 {
|
|
return CreateMachineRequestValidationError{
|
|
field: "Description",
|
|
reason: "value length must be at most 500 runes",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// CreateMachineRequestValidationError is the validation error returned by
|
|
// CreateMachineRequest.Validate if the designated constraints aren't met.
|
|
type CreateMachineRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e CreateMachineRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e CreateMachineRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e CreateMachineRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e CreateMachineRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e CreateMachineRequestValidationError) ErrorName() string {
|
|
return "CreateMachineRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e CreateMachineRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sCreateMachineRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = CreateMachineRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = CreateMachineRequestValidationError{}
|
|
|
|
// Validate checks the field values on UserResponse with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *UserResponse) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Id
|
|
|
|
// no validation rules for State
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserResponseValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserResponseValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for Sequence
|
|
|
|
// no validation rules for UserName
|
|
|
|
switch m.User.(type) {
|
|
|
|
case *UserResponse_Human:
|
|
|
|
if v, ok := interface{}(m.GetHuman()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserResponseValidationError{
|
|
field: "Human",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
case *UserResponse_Machine:
|
|
|
|
if v, ok := interface{}(m.GetMachine()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserResponseValidationError{
|
|
field: "Machine",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
default:
|
|
return UserResponseValidationError{
|
|
field: "User",
|
|
reason: "value is required",
|
|
}
|
|
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// UserResponseValidationError is the validation error returned by
|
|
// UserResponse.Validate if the designated constraints aren't met.
|
|
type UserResponseValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UserResponseValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UserResponseValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UserResponseValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UserResponseValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UserResponseValidationError) ErrorName() string { return "UserResponseValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UserResponseValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUserResponse.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UserResponseValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UserResponseValidationError{}
|
|
|
|
// Validate checks the field values on HumanResponse with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *HumanResponse) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for FirstName
|
|
|
|
// no validation rules for LastName
|
|
|
|
// no validation rules for DisplayName
|
|
|
|
// no validation rules for NickName
|
|
|
|
// no validation rules for PreferredLanguage
|
|
|
|
// no validation rules for Gender
|
|
|
|
// no validation rules for Email
|
|
|
|
// no validation rules for IsEmailVerified
|
|
|
|
// no validation rules for Phone
|
|
|
|
// no validation rules for IsPhoneVerified
|
|
|
|
// no validation rules for Country
|
|
|
|
// no validation rules for Locality
|
|
|
|
// no validation rules for PostalCode
|
|
|
|
// no validation rules for Region
|
|
|
|
// no validation rules for StreetAddress
|
|
|
|
return nil
|
|
}
|
|
|
|
// HumanResponseValidationError is the validation error returned by
|
|
// HumanResponse.Validate if the designated constraints aren't met.
|
|
type HumanResponseValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e HumanResponseValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e HumanResponseValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e HumanResponseValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e HumanResponseValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e HumanResponseValidationError) ErrorName() string { return "HumanResponseValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e HumanResponseValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sHumanResponse.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = HumanResponseValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = HumanResponseValidationError{}
|
|
|
|
// Validate checks the field values on MachineResponse with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *MachineResponse) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Name
|
|
|
|
// no validation rules for Description
|
|
|
|
for idx, item := range m.GetKeys() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return MachineResponseValidationError{
|
|
field: fmt.Sprintf("Keys[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// MachineResponseValidationError is the validation error returned by
|
|
// MachineResponse.Validate if the designated constraints aren't met.
|
|
type MachineResponseValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e MachineResponseValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e MachineResponseValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e MachineResponseValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e MachineResponseValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e MachineResponseValidationError) ErrorName() string { return "MachineResponseValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e MachineResponseValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sMachineResponse.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = MachineResponseValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = MachineResponseValidationError{}
|
|
|
|
// Validate checks the field values on MachineKeyResponse with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *MachineKeyResponse) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Id
|
|
|
|
// no validation rules for Type
|
|
|
|
// no validation rules for Sequence
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return MachineKeyResponseValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetExpirationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return MachineKeyResponseValidationError{
|
|
field: "ExpirationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// MachineKeyResponseValidationError is the validation error returned by
|
|
// MachineKeyResponse.Validate if the designated constraints aren't met.
|
|
type MachineKeyResponseValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e MachineKeyResponseValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e MachineKeyResponseValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e MachineKeyResponseValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e MachineKeyResponseValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e MachineKeyResponseValidationError) ErrorName() string {
|
|
return "MachineKeyResponseValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e MachineKeyResponseValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sMachineKeyResponse.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = MachineKeyResponseValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = MachineKeyResponseValidationError{}
|
|
|
|
// Validate checks the field values on CreateOrgRequest with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *CreateOrgRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetName()) < 1 {
|
|
return CreateOrgRequestValidationError{
|
|
field: "Name",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
// no validation rules for Domain
|
|
|
|
return nil
|
|
}
|
|
|
|
// CreateOrgRequestValidationError is the validation error returned by
|
|
// CreateOrgRequest.Validate if the designated constraints aren't met.
|
|
type CreateOrgRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e CreateOrgRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e CreateOrgRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e CreateOrgRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e CreateOrgRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e CreateOrgRequestValidationError) ErrorName() string { return "CreateOrgRequestValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e CreateOrgRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sCreateOrgRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = CreateOrgRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = CreateOrgRequestValidationError{}
|
|
|
|
// Validate checks the field values on OrgIamPolicy with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *OrgIamPolicy) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for OrgId
|
|
|
|
// no validation rules for UserLoginMustBeDomain
|
|
|
|
// no validation rules for Default
|
|
|
|
// no validation rules for Sequence
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return OrgIamPolicyValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return OrgIamPolicyValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// OrgIamPolicyValidationError is the validation error returned by
|
|
// OrgIamPolicy.Validate if the designated constraints aren't met.
|
|
type OrgIamPolicyValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e OrgIamPolicyValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e OrgIamPolicyValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e OrgIamPolicyValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e OrgIamPolicyValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e OrgIamPolicyValidationError) ErrorName() string { return "OrgIamPolicyValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e OrgIamPolicyValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sOrgIamPolicy.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = OrgIamPolicyValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = OrgIamPolicyValidationError{}
|
|
|
|
// Validate checks the field values on OrgIamPolicyView with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *OrgIamPolicyView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for OrgId
|
|
|
|
// no validation rules for UserLoginMustBeDomain
|
|
|
|
// no validation rules for Default
|
|
|
|
// no validation rules for Sequence
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return OrgIamPolicyViewValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return OrgIamPolicyViewValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// OrgIamPolicyViewValidationError is the validation error returned by
|
|
// OrgIamPolicyView.Validate if the designated constraints aren't met.
|
|
type OrgIamPolicyViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e OrgIamPolicyViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e OrgIamPolicyViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e OrgIamPolicyViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e OrgIamPolicyViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e OrgIamPolicyViewValidationError) ErrorName() string { return "OrgIamPolicyViewValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e OrgIamPolicyViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sOrgIamPolicyView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = OrgIamPolicyViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = OrgIamPolicyViewValidationError{}
|
|
|
|
// Validate checks the field values on OrgIamPolicyRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *OrgIamPolicyRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetOrgId()) < 1 {
|
|
return OrgIamPolicyRequestValidationError{
|
|
field: "OrgId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
// no validation rules for Description
|
|
|
|
// no validation rules for UserLoginMustBeDomain
|
|
|
|
return nil
|
|
}
|
|
|
|
// OrgIamPolicyRequestValidationError is the validation error returned by
|
|
// OrgIamPolicyRequest.Validate if the designated constraints aren't met.
|
|
type OrgIamPolicyRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e OrgIamPolicyRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e OrgIamPolicyRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e OrgIamPolicyRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e OrgIamPolicyRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e OrgIamPolicyRequestValidationError) ErrorName() string {
|
|
return "OrgIamPolicyRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e OrgIamPolicyRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sOrgIamPolicyRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = OrgIamPolicyRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = OrgIamPolicyRequestValidationError{}
|
|
|
|
// Validate checks the field values on OrgIamPolicyID with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *OrgIamPolicyID) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetOrgId()) < 1 {
|
|
return OrgIamPolicyIDValidationError{
|
|
field: "OrgId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// OrgIamPolicyIDValidationError is the validation error returned by
|
|
// OrgIamPolicyID.Validate if the designated constraints aren't met.
|
|
type OrgIamPolicyIDValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e OrgIamPolicyIDValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e OrgIamPolicyIDValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e OrgIamPolicyIDValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e OrgIamPolicyIDValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e OrgIamPolicyIDValidationError) ErrorName() string { return "OrgIamPolicyIDValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e OrgIamPolicyIDValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sOrgIamPolicyID.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = OrgIamPolicyIDValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = OrgIamPolicyIDValidationError{}
|
|
|
|
// Validate checks the field values on IamMemberRoles with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *IamMemberRoles) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// IamMemberRolesValidationError is the validation error returned by
|
|
// IamMemberRoles.Validate if the designated constraints aren't met.
|
|
type IamMemberRolesValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e IamMemberRolesValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e IamMemberRolesValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e IamMemberRolesValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e IamMemberRolesValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e IamMemberRolesValidationError) ErrorName() string { return "IamMemberRolesValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e IamMemberRolesValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sIamMemberRoles.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = IamMemberRolesValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = IamMemberRolesValidationError{}
|
|
|
|
// Validate checks the field values on IamMember with the rules defined in the
|
|
// proto definition for this message. If any rules are violated, an error is returned.
|
|
func (m *IamMember) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for UserId
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return IamMemberValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return IamMemberValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for Sequence
|
|
|
|
return nil
|
|
}
|
|
|
|
// IamMemberValidationError is the validation error returned by
|
|
// IamMember.Validate if the designated constraints aren't met.
|
|
type IamMemberValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e IamMemberValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e IamMemberValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e IamMemberValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e IamMemberValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e IamMemberValidationError) ErrorName() string { return "IamMemberValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e IamMemberValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sIamMember.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = IamMemberValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = IamMemberValidationError{}
|
|
|
|
// Validate checks the field values on AddIamMemberRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *AddIamMemberRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetUserId()) < 1 {
|
|
return AddIamMemberRequestValidationError{
|
|
field: "UserId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// AddIamMemberRequestValidationError is the validation error returned by
|
|
// AddIamMemberRequest.Validate if the designated constraints aren't met.
|
|
type AddIamMemberRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e AddIamMemberRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e AddIamMemberRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e AddIamMemberRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e AddIamMemberRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e AddIamMemberRequestValidationError) ErrorName() string {
|
|
return "AddIamMemberRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e AddIamMemberRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sAddIamMemberRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = AddIamMemberRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = AddIamMemberRequestValidationError{}
|
|
|
|
// Validate checks the field values on ChangeIamMemberRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *ChangeIamMemberRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetUserId()) < 1 {
|
|
return ChangeIamMemberRequestValidationError{
|
|
field: "UserId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// ChangeIamMemberRequestValidationError is the validation error returned by
|
|
// ChangeIamMemberRequest.Validate if the designated constraints aren't met.
|
|
type ChangeIamMemberRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ChangeIamMemberRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ChangeIamMemberRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ChangeIamMemberRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ChangeIamMemberRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ChangeIamMemberRequestValidationError) ErrorName() string {
|
|
return "ChangeIamMemberRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ChangeIamMemberRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sChangeIamMemberRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ChangeIamMemberRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ChangeIamMemberRequestValidationError{}
|
|
|
|
// Validate checks the field values on RemoveIamMemberRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *RemoveIamMemberRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetUserId()) < 1 {
|
|
return RemoveIamMemberRequestValidationError{
|
|
field: "UserId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// RemoveIamMemberRequestValidationError is the validation error returned by
|
|
// RemoveIamMemberRequest.Validate if the designated constraints aren't met.
|
|
type RemoveIamMemberRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e RemoveIamMemberRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e RemoveIamMemberRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e RemoveIamMemberRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e RemoveIamMemberRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e RemoveIamMemberRequestValidationError) ErrorName() string {
|
|
return "RemoveIamMemberRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e RemoveIamMemberRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sRemoveIamMemberRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = RemoveIamMemberRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = RemoveIamMemberRequestValidationError{}
|
|
|
|
// Validate checks the field values on IamMemberSearchResponse with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *IamMemberSearchResponse) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
// no validation rules for TotalResult
|
|
|
|
for idx, item := range m.GetResult() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return IamMemberSearchResponseValidationError{
|
|
field: fmt.Sprintf("Result[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
// no validation rules for ProcessedSequence
|
|
|
|
if v, ok := interface{}(m.GetViewTimestamp()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return IamMemberSearchResponseValidationError{
|
|
field: "ViewTimestamp",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// IamMemberSearchResponseValidationError is the validation error returned by
|
|
// IamMemberSearchResponse.Validate if the designated constraints aren't met.
|
|
type IamMemberSearchResponseValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e IamMemberSearchResponseValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e IamMemberSearchResponseValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e IamMemberSearchResponseValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e IamMemberSearchResponseValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e IamMemberSearchResponseValidationError) ErrorName() string {
|
|
return "IamMemberSearchResponseValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e IamMemberSearchResponseValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sIamMemberSearchResponse.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = IamMemberSearchResponseValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = IamMemberSearchResponseValidationError{}
|
|
|
|
// Validate checks the field values on IamMemberView with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *IamMemberView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for UserId
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return IamMemberViewValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return IamMemberViewValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for Sequence
|
|
|
|
// no validation rules for UserName
|
|
|
|
// no validation rules for Email
|
|
|
|
// no validation rules for FirstName
|
|
|
|
// no validation rules for LastName
|
|
|
|
// no validation rules for DisplayName
|
|
|
|
return nil
|
|
}
|
|
|
|
// IamMemberViewValidationError is the validation error returned by
|
|
// IamMemberView.Validate if the designated constraints aren't met.
|
|
type IamMemberViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e IamMemberViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e IamMemberViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e IamMemberViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e IamMemberViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e IamMemberViewValidationError) ErrorName() string { return "IamMemberViewValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e IamMemberViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sIamMemberView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = IamMemberViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = IamMemberViewValidationError{}
|
|
|
|
// Validate checks the field values on IamMemberSearchRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *IamMemberSearchRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
for idx, item := range m.GetQueries() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return IamMemberSearchRequestValidationError{
|
|
field: fmt.Sprintf("Queries[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// IamMemberSearchRequestValidationError is the validation error returned by
|
|
// IamMemberSearchRequest.Validate if the designated constraints aren't met.
|
|
type IamMemberSearchRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e IamMemberSearchRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e IamMemberSearchRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e IamMemberSearchRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e IamMemberSearchRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e IamMemberSearchRequestValidationError) ErrorName() string {
|
|
return "IamMemberSearchRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e IamMemberSearchRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sIamMemberSearchRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = IamMemberSearchRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = IamMemberSearchRequestValidationError{}
|
|
|
|
// Validate checks the field values on IamMemberSearchQuery with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *IamMemberSearchQuery) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if _, ok := _IamMemberSearchQuery_Key_NotInLookup[m.GetKey()]; ok {
|
|
return IamMemberSearchQueryValidationError{
|
|
field: "Key",
|
|
reason: "value must not be in list [0]",
|
|
}
|
|
}
|
|
|
|
// no validation rules for Method
|
|
|
|
// no validation rules for Value
|
|
|
|
return nil
|
|
}
|
|
|
|
// IamMemberSearchQueryValidationError is the validation error returned by
|
|
// IamMemberSearchQuery.Validate if the designated constraints aren't met.
|
|
type IamMemberSearchQueryValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e IamMemberSearchQueryValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e IamMemberSearchQueryValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e IamMemberSearchQueryValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e IamMemberSearchQueryValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e IamMemberSearchQueryValidationError) ErrorName() string {
|
|
return "IamMemberSearchQueryValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e IamMemberSearchQueryValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sIamMemberSearchQuery.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = IamMemberSearchQueryValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = IamMemberSearchQueryValidationError{}
|
|
|
|
var _IamMemberSearchQuery_Key_NotInLookup = map[IamMemberSearchKey]struct{}{
|
|
0: {},
|
|
}
|
|
|
|
// Validate checks the field values on FailedEventID with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *FailedEventID) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetDatabase()) < 1 {
|
|
return FailedEventIDValidationError{
|
|
field: "Database",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetViewName()) < 1 {
|
|
return FailedEventIDValidationError{
|
|
field: "ViewName",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
// no validation rules for FailedSequence
|
|
|
|
return nil
|
|
}
|
|
|
|
// FailedEventIDValidationError is the validation error returned by
|
|
// FailedEventID.Validate if the designated constraints aren't met.
|
|
type FailedEventIDValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e FailedEventIDValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e FailedEventIDValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e FailedEventIDValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e FailedEventIDValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e FailedEventIDValidationError) ErrorName() string { return "FailedEventIDValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e FailedEventIDValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sFailedEventID.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = FailedEventIDValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = FailedEventIDValidationError{}
|
|
|
|
// Validate checks the field values on FailedEvents with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *FailedEvents) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
for idx, item := range m.GetFailedEvents() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return FailedEventsValidationError{
|
|
field: fmt.Sprintf("FailedEvents[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// FailedEventsValidationError is the validation error returned by
|
|
// FailedEvents.Validate if the designated constraints aren't met.
|
|
type FailedEventsValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e FailedEventsValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e FailedEventsValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e FailedEventsValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e FailedEventsValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e FailedEventsValidationError) ErrorName() string { return "FailedEventsValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e FailedEventsValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sFailedEvents.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = FailedEventsValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = FailedEventsValidationError{}
|
|
|
|
// Validate checks the field values on FailedEvent with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *FailedEvent) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Database
|
|
|
|
// no validation rules for ViewName
|
|
|
|
// no validation rules for FailedSequence
|
|
|
|
// no validation rules for FailureCount
|
|
|
|
// no validation rules for ErrorMessage
|
|
|
|
return nil
|
|
}
|
|
|
|
// FailedEventValidationError is the validation error returned by
|
|
// FailedEvent.Validate if the designated constraints aren't met.
|
|
type FailedEventValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e FailedEventValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e FailedEventValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e FailedEventValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e FailedEventValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e FailedEventValidationError) ErrorName() string { return "FailedEventValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e FailedEventValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sFailedEvent.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = FailedEventValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = FailedEventValidationError{}
|
|
|
|
// Validate checks the field values on ViewID with the rules defined in the
|
|
// proto definition for this message. If any rules are violated, an error is returned.
|
|
func (m *ViewID) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetDatabase()) < 1 {
|
|
return ViewIDValidationError{
|
|
field: "Database",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetViewName()) < 1 {
|
|
return ViewIDValidationError{
|
|
field: "ViewName",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// ViewIDValidationError is the validation error returned by ViewID.Validate if
|
|
// the designated constraints aren't met.
|
|
type ViewIDValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ViewIDValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ViewIDValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ViewIDValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ViewIDValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ViewIDValidationError) ErrorName() string { return "ViewIDValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ViewIDValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sViewID.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ViewIDValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ViewIDValidationError{}
|
|
|
|
// Validate checks the field values on Views with the rules defined in the
|
|
// proto definition for this message. If any rules are violated, an error is returned.
|
|
func (m *Views) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
for idx, item := range m.GetViews() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ViewsValidationError{
|
|
field: fmt.Sprintf("Views[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// ViewsValidationError is the validation error returned by Views.Validate if
|
|
// the designated constraints aren't met.
|
|
type ViewsValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ViewsValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ViewsValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ViewsValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ViewsValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ViewsValidationError) ErrorName() string { return "ViewsValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ViewsValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sViews.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ViewsValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ViewsValidationError{}
|
|
|
|
// Validate checks the field values on View with the rules defined in the proto
|
|
// definition for this message. If any rules are violated, an error is returned.
|
|
func (m *View) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Database
|
|
|
|
// no validation rules for ViewName
|
|
|
|
// no validation rules for ProcessedSequence
|
|
|
|
if v, ok := interface{}(m.GetViewTimestamp()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ViewValidationError{
|
|
field: "ViewTimestamp",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// ViewValidationError is the validation error returned by View.Validate if the
|
|
// designated constraints aren't met.
|
|
type ViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ViewValidationError) ErrorName() string { return "ViewValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ViewValidationError{}
|
|
|
|
// Validate checks the field values on IdpID with the rules defined in the
|
|
// proto definition for this message. If any rules are violated, an error is returned.
|
|
func (m *IdpID) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetId()) < 1 {
|
|
return IdpIDValidationError{
|
|
field: "Id",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// IdpIDValidationError is the validation error returned by IdpID.Validate if
|
|
// the designated constraints aren't met.
|
|
type IdpIDValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e IdpIDValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e IdpIDValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e IdpIDValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e IdpIDValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e IdpIDValidationError) ErrorName() string { return "IdpIDValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e IdpIDValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sIdpID.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = IdpIDValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = IdpIDValidationError{}
|
|
|
|
// Validate checks the field values on Idp with the rules defined in the proto
|
|
// definition for this message. If any rules are violated, an error is returned.
|
|
func (m *Idp) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Id
|
|
|
|
// no validation rules for State
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return IdpValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return IdpValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for Name
|
|
|
|
// no validation rules for StylingType
|
|
|
|
// no validation rules for Sequence
|
|
|
|
switch m.IdpConfig.(type) {
|
|
|
|
case *Idp_OidcConfig:
|
|
|
|
if v, ok := interface{}(m.GetOidcConfig()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return IdpValidationError{
|
|
field: "OidcConfig",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// IdpValidationError is the validation error returned by Idp.Validate if the
|
|
// designated constraints aren't met.
|
|
type IdpValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e IdpValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e IdpValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e IdpValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e IdpValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e IdpValidationError) ErrorName() string { return "IdpValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e IdpValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sIdp.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = IdpValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = IdpValidationError{}
|
|
|
|
// Validate checks the field values on IdpUpdate with the rules defined in the
|
|
// proto definition for this message. If any rules are violated, an error is returned.
|
|
func (m *IdpUpdate) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetId()) < 1 {
|
|
return IdpUpdateValidationError{
|
|
field: "Id",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
// no validation rules for Name
|
|
|
|
// no validation rules for StylingType
|
|
|
|
return nil
|
|
}
|
|
|
|
// IdpUpdateValidationError is the validation error returned by
|
|
// IdpUpdate.Validate if the designated constraints aren't met.
|
|
type IdpUpdateValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e IdpUpdateValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e IdpUpdateValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e IdpUpdateValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e IdpUpdateValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e IdpUpdateValidationError) ErrorName() string { return "IdpUpdateValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e IdpUpdateValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sIdpUpdate.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = IdpUpdateValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = IdpUpdateValidationError{}
|
|
|
|
// Validate checks the field values on OidcIdpConfig with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *OidcIdpConfig) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for ClientId
|
|
|
|
// no validation rules for ClientSecret
|
|
|
|
// no validation rules for Issuer
|
|
|
|
return nil
|
|
}
|
|
|
|
// OidcIdpConfigValidationError is the validation error returned by
|
|
// OidcIdpConfig.Validate if the designated constraints aren't met.
|
|
type OidcIdpConfigValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e OidcIdpConfigValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e OidcIdpConfigValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e OidcIdpConfigValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e OidcIdpConfigValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e OidcIdpConfigValidationError) ErrorName() string { return "OidcIdpConfigValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e OidcIdpConfigValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sOidcIdpConfig.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = OidcIdpConfigValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = OidcIdpConfigValidationError{}
|
|
|
|
// Validate checks the field values on OidcIdpConfigCreate with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *OidcIdpConfigCreate) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if l := utf8.RuneCountInString(m.GetName()); l < 1 || l > 200 {
|
|
return OidcIdpConfigCreateValidationError{
|
|
field: "Name",
|
|
reason: "value length must be between 1 and 200 runes, inclusive",
|
|
}
|
|
}
|
|
|
|
// no validation rules for StylingType
|
|
|
|
if l := utf8.RuneCountInString(m.GetClientId()); l < 1 || l > 200 {
|
|
return OidcIdpConfigCreateValidationError{
|
|
field: "ClientId",
|
|
reason: "value length must be between 1 and 200 runes, inclusive",
|
|
}
|
|
}
|
|
|
|
if l := utf8.RuneCountInString(m.GetClientSecret()); l < 1 || l > 200 {
|
|
return OidcIdpConfigCreateValidationError{
|
|
field: "ClientSecret",
|
|
reason: "value length must be between 1 and 200 runes, inclusive",
|
|
}
|
|
}
|
|
|
|
if l := utf8.RuneCountInString(m.GetIssuer()); l < 1 || l > 200 {
|
|
return OidcIdpConfigCreateValidationError{
|
|
field: "Issuer",
|
|
reason: "value length must be between 1 and 200 runes, inclusive",
|
|
}
|
|
}
|
|
|
|
// no validation rules for IdpDisplayNameMapping
|
|
|
|
// no validation rules for UsernameMapping
|
|
|
|
return nil
|
|
}
|
|
|
|
// OidcIdpConfigCreateValidationError is the validation error returned by
|
|
// OidcIdpConfigCreate.Validate if the designated constraints aren't met.
|
|
type OidcIdpConfigCreateValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e OidcIdpConfigCreateValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e OidcIdpConfigCreateValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e OidcIdpConfigCreateValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e OidcIdpConfigCreateValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e OidcIdpConfigCreateValidationError) ErrorName() string {
|
|
return "OidcIdpConfigCreateValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e OidcIdpConfigCreateValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sOidcIdpConfigCreate.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = OidcIdpConfigCreateValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = OidcIdpConfigCreateValidationError{}
|
|
|
|
// Validate checks the field values on OidcIdpConfigUpdate with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *OidcIdpConfigUpdate) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetIdpId()) < 1 {
|
|
return OidcIdpConfigUpdateValidationError{
|
|
field: "IdpId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
if l := utf8.RuneCountInString(m.GetClientId()); l < 1 || l > 200 {
|
|
return OidcIdpConfigUpdateValidationError{
|
|
field: "ClientId",
|
|
reason: "value length must be between 1 and 200 runes, inclusive",
|
|
}
|
|
}
|
|
|
|
// no validation rules for ClientSecret
|
|
|
|
if l := utf8.RuneCountInString(m.GetIssuer()); l < 1 || l > 200 {
|
|
return OidcIdpConfigUpdateValidationError{
|
|
field: "Issuer",
|
|
reason: "value length must be between 1 and 200 runes, inclusive",
|
|
}
|
|
}
|
|
|
|
// no validation rules for IdpDisplayNameMapping
|
|
|
|
// no validation rules for UsernameMapping
|
|
|
|
return nil
|
|
}
|
|
|
|
// OidcIdpConfigUpdateValidationError is the validation error returned by
|
|
// OidcIdpConfigUpdate.Validate if the designated constraints aren't met.
|
|
type OidcIdpConfigUpdateValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e OidcIdpConfigUpdateValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e OidcIdpConfigUpdateValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e OidcIdpConfigUpdateValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e OidcIdpConfigUpdateValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e OidcIdpConfigUpdateValidationError) ErrorName() string {
|
|
return "OidcIdpConfigUpdateValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e OidcIdpConfigUpdateValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sOidcIdpConfigUpdate.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = OidcIdpConfigUpdateValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = OidcIdpConfigUpdateValidationError{}
|
|
|
|
// Validate checks the field values on IdpSearchResponse with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *IdpSearchResponse) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
// no validation rules for TotalResult
|
|
|
|
for idx, item := range m.GetResult() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return IdpSearchResponseValidationError{
|
|
field: fmt.Sprintf("Result[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
// no validation rules for ProcessedSequence
|
|
|
|
if v, ok := interface{}(m.GetViewTimestamp()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return IdpSearchResponseValidationError{
|
|
field: "ViewTimestamp",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// IdpSearchResponseValidationError is the validation error returned by
|
|
// IdpSearchResponse.Validate if the designated constraints aren't met.
|
|
type IdpSearchResponseValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e IdpSearchResponseValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e IdpSearchResponseValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e IdpSearchResponseValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e IdpSearchResponseValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e IdpSearchResponseValidationError) ErrorName() string {
|
|
return "IdpSearchResponseValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e IdpSearchResponseValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sIdpSearchResponse.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = IdpSearchResponseValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = IdpSearchResponseValidationError{}
|
|
|
|
// Validate checks the field values on IdpView with the rules defined in the
|
|
// proto definition for this message. If any rules are violated, an error is returned.
|
|
func (m *IdpView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Id
|
|
|
|
// no validation rules for State
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return IdpViewValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return IdpViewValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for Name
|
|
|
|
// no validation rules for StylingType
|
|
|
|
// no validation rules for Sequence
|
|
|
|
switch m.IdpConfigView.(type) {
|
|
|
|
case *IdpView_OidcConfig:
|
|
|
|
if v, ok := interface{}(m.GetOidcConfig()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return IdpViewValidationError{
|
|
field: "OidcConfig",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// IdpViewValidationError is the validation error returned by IdpView.Validate
|
|
// if the designated constraints aren't met.
|
|
type IdpViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e IdpViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e IdpViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e IdpViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e IdpViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e IdpViewValidationError) ErrorName() string { return "IdpViewValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e IdpViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sIdpView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = IdpViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = IdpViewValidationError{}
|
|
|
|
// Validate checks the field values on OidcIdpConfigView with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *OidcIdpConfigView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for ClientId
|
|
|
|
// no validation rules for Issuer
|
|
|
|
// no validation rules for IdpDisplayNameMapping
|
|
|
|
// no validation rules for UsernameMapping
|
|
|
|
return nil
|
|
}
|
|
|
|
// OidcIdpConfigViewValidationError is the validation error returned by
|
|
// OidcIdpConfigView.Validate if the designated constraints aren't met.
|
|
type OidcIdpConfigViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e OidcIdpConfigViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e OidcIdpConfigViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e OidcIdpConfigViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e OidcIdpConfigViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e OidcIdpConfigViewValidationError) ErrorName() string {
|
|
return "OidcIdpConfigViewValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e OidcIdpConfigViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sOidcIdpConfigView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = OidcIdpConfigViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = OidcIdpConfigViewValidationError{}
|
|
|
|
// Validate checks the field values on IdpSearchRequest with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *IdpSearchRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
for idx, item := range m.GetQueries() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return IdpSearchRequestValidationError{
|
|
field: fmt.Sprintf("Queries[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// IdpSearchRequestValidationError is the validation error returned by
|
|
// IdpSearchRequest.Validate if the designated constraints aren't met.
|
|
type IdpSearchRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e IdpSearchRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e IdpSearchRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e IdpSearchRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e IdpSearchRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e IdpSearchRequestValidationError) ErrorName() string { return "IdpSearchRequestValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e IdpSearchRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sIdpSearchRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = IdpSearchRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = IdpSearchRequestValidationError{}
|
|
|
|
// Validate checks the field values on IdpSearchQuery with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *IdpSearchQuery) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if _, ok := _IdpSearchQuery_Key_NotInLookup[m.GetKey()]; ok {
|
|
return IdpSearchQueryValidationError{
|
|
field: "Key",
|
|
reason: "value must not be in list [0]",
|
|
}
|
|
}
|
|
|
|
// no validation rules for Method
|
|
|
|
// no validation rules for Value
|
|
|
|
return nil
|
|
}
|
|
|
|
// IdpSearchQueryValidationError is the validation error returned by
|
|
// IdpSearchQuery.Validate if the designated constraints aren't met.
|
|
type IdpSearchQueryValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e IdpSearchQueryValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e IdpSearchQueryValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e IdpSearchQueryValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e IdpSearchQueryValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e IdpSearchQueryValidationError) ErrorName() string { return "IdpSearchQueryValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e IdpSearchQueryValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sIdpSearchQuery.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = IdpSearchQueryValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = IdpSearchQueryValidationError{}
|
|
|
|
var _IdpSearchQuery_Key_NotInLookup = map[IdpSearchKey]struct{}{
|
|
0: {},
|
|
}
|
|
|
|
// Validate checks the field values on DefaultLabelPolicy with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *DefaultLabelPolicy) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for PrimaryColor
|
|
|
|
// no validation rules for SecondaryColor
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return DefaultLabelPolicyValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return DefaultLabelPolicyValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// DefaultLabelPolicyValidationError is the validation error returned by
|
|
// DefaultLabelPolicy.Validate if the designated constraints aren't met.
|
|
type DefaultLabelPolicyValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e DefaultLabelPolicyValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e DefaultLabelPolicyValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e DefaultLabelPolicyValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e DefaultLabelPolicyValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e DefaultLabelPolicyValidationError) ErrorName() string {
|
|
return "DefaultLabelPolicyValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e DefaultLabelPolicyValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sDefaultLabelPolicy.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = DefaultLabelPolicyValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = DefaultLabelPolicyValidationError{}
|
|
|
|
// Validate checks the field values on DefaultLabelPolicyUpdate with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *DefaultLabelPolicyUpdate) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for PrimaryColor
|
|
|
|
// no validation rules for SecondaryColor
|
|
|
|
return nil
|
|
}
|
|
|
|
// DefaultLabelPolicyUpdateValidationError is the validation error returned by
|
|
// DefaultLabelPolicyUpdate.Validate if the designated constraints aren't met.
|
|
type DefaultLabelPolicyUpdateValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e DefaultLabelPolicyUpdateValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e DefaultLabelPolicyUpdateValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e DefaultLabelPolicyUpdateValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e DefaultLabelPolicyUpdateValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e DefaultLabelPolicyUpdateValidationError) ErrorName() string {
|
|
return "DefaultLabelPolicyUpdateValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e DefaultLabelPolicyUpdateValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sDefaultLabelPolicyUpdate.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = DefaultLabelPolicyUpdateValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = DefaultLabelPolicyUpdateValidationError{}
|
|
|
|
// Validate checks the field values on DefaultLabelPolicyView with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *DefaultLabelPolicyView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for PrimaryColor
|
|
|
|
// no validation rules for SecondaryColor
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return DefaultLabelPolicyViewValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return DefaultLabelPolicyViewValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// DefaultLabelPolicyViewValidationError is the validation error returned by
|
|
// DefaultLabelPolicyView.Validate if the designated constraints aren't met.
|
|
type DefaultLabelPolicyViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e DefaultLabelPolicyViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e DefaultLabelPolicyViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e DefaultLabelPolicyViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e DefaultLabelPolicyViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e DefaultLabelPolicyViewValidationError) ErrorName() string {
|
|
return "DefaultLabelPolicyViewValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e DefaultLabelPolicyViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sDefaultLabelPolicyView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = DefaultLabelPolicyViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = DefaultLabelPolicyViewValidationError{}
|
|
|
|
// Validate checks the field values on DefaultLoginPolicy with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *DefaultLoginPolicy) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for AllowUsernamePassword
|
|
|
|
// no validation rules for AllowRegister
|
|
|
|
// no validation rules for AllowExternalIdp
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return DefaultLoginPolicyValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return DefaultLoginPolicyValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for ForceMfa
|
|
|
|
return nil
|
|
}
|
|
|
|
// DefaultLoginPolicyValidationError is the validation error returned by
|
|
// DefaultLoginPolicy.Validate if the designated constraints aren't met.
|
|
type DefaultLoginPolicyValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e DefaultLoginPolicyValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e DefaultLoginPolicyValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e DefaultLoginPolicyValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e DefaultLoginPolicyValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e DefaultLoginPolicyValidationError) ErrorName() string {
|
|
return "DefaultLoginPolicyValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e DefaultLoginPolicyValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sDefaultLoginPolicy.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = DefaultLoginPolicyValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = DefaultLoginPolicyValidationError{}
|
|
|
|
// Validate checks the field values on DefaultLoginPolicyRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *DefaultLoginPolicyRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for AllowUsernamePassword
|
|
|
|
// no validation rules for AllowRegister
|
|
|
|
// no validation rules for AllowExternalIdp
|
|
|
|
// no validation rules for ForceMfa
|
|
|
|
return nil
|
|
}
|
|
|
|
// DefaultLoginPolicyRequestValidationError is the validation error returned by
|
|
// DefaultLoginPolicyRequest.Validate if the designated constraints aren't met.
|
|
type DefaultLoginPolicyRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e DefaultLoginPolicyRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e DefaultLoginPolicyRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e DefaultLoginPolicyRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e DefaultLoginPolicyRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e DefaultLoginPolicyRequestValidationError) ErrorName() string {
|
|
return "DefaultLoginPolicyRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e DefaultLoginPolicyRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sDefaultLoginPolicyRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = DefaultLoginPolicyRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = DefaultLoginPolicyRequestValidationError{}
|
|
|
|
// Validate checks the field values on IdpProviderID with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *IdpProviderID) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetIdpConfigId()) < 1 {
|
|
return IdpProviderIDValidationError{
|
|
field: "IdpConfigId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// IdpProviderIDValidationError is the validation error returned by
|
|
// IdpProviderID.Validate if the designated constraints aren't met.
|
|
type IdpProviderIDValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e IdpProviderIDValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e IdpProviderIDValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e IdpProviderIDValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e IdpProviderIDValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e IdpProviderIDValidationError) ErrorName() string { return "IdpProviderIDValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e IdpProviderIDValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sIdpProviderID.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = IdpProviderIDValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = IdpProviderIDValidationError{}
|
|
|
|
// Validate checks the field values on DefaultLoginPolicyView with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *DefaultLoginPolicyView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for AllowUsernamePassword
|
|
|
|
// no validation rules for AllowRegister
|
|
|
|
// no validation rules for AllowExternalIdp
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return DefaultLoginPolicyViewValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return DefaultLoginPolicyViewValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for ForceMfa
|
|
|
|
return nil
|
|
}
|
|
|
|
// DefaultLoginPolicyViewValidationError is the validation error returned by
|
|
// DefaultLoginPolicyView.Validate if the designated constraints aren't met.
|
|
type DefaultLoginPolicyViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e DefaultLoginPolicyViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e DefaultLoginPolicyViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e DefaultLoginPolicyViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e DefaultLoginPolicyViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e DefaultLoginPolicyViewValidationError) ErrorName() string {
|
|
return "DefaultLoginPolicyViewValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e DefaultLoginPolicyViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sDefaultLoginPolicyView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = DefaultLoginPolicyViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = DefaultLoginPolicyViewValidationError{}
|
|
|
|
// Validate checks the field values on IdpProviderView with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *IdpProviderView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for IdpConfigId
|
|
|
|
// no validation rules for Name
|
|
|
|
// no validation rules for Type
|
|
|
|
return nil
|
|
}
|
|
|
|
// IdpProviderViewValidationError is the validation error returned by
|
|
// IdpProviderView.Validate if the designated constraints aren't met.
|
|
type IdpProviderViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e IdpProviderViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e IdpProviderViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e IdpProviderViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e IdpProviderViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e IdpProviderViewValidationError) ErrorName() string { return "IdpProviderViewValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e IdpProviderViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sIdpProviderView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = IdpProviderViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = IdpProviderViewValidationError{}
|
|
|
|
// Validate checks the field values on IdpProviderSearchResponse with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *IdpProviderSearchResponse) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
// no validation rules for TotalResult
|
|
|
|
for idx, item := range m.GetResult() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return IdpProviderSearchResponseValidationError{
|
|
field: fmt.Sprintf("Result[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
// no validation rules for ProcessedSequence
|
|
|
|
if v, ok := interface{}(m.GetViewTimestamp()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return IdpProviderSearchResponseValidationError{
|
|
field: "ViewTimestamp",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// IdpProviderSearchResponseValidationError is the validation error returned by
|
|
// IdpProviderSearchResponse.Validate if the designated constraints aren't met.
|
|
type IdpProviderSearchResponseValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e IdpProviderSearchResponseValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e IdpProviderSearchResponseValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e IdpProviderSearchResponseValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e IdpProviderSearchResponseValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e IdpProviderSearchResponseValidationError) ErrorName() string {
|
|
return "IdpProviderSearchResponseValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e IdpProviderSearchResponseValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sIdpProviderSearchResponse.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = IdpProviderSearchResponseValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = IdpProviderSearchResponseValidationError{}
|
|
|
|
// Validate checks the field values on IdpProviderSearchRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *IdpProviderSearchRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
return nil
|
|
}
|
|
|
|
// IdpProviderSearchRequestValidationError is the validation error returned by
|
|
// IdpProviderSearchRequest.Validate if the designated constraints aren't met.
|
|
type IdpProviderSearchRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e IdpProviderSearchRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e IdpProviderSearchRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e IdpProviderSearchRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e IdpProviderSearchRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e IdpProviderSearchRequestValidationError) ErrorName() string {
|
|
return "IdpProviderSearchRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e IdpProviderSearchRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sIdpProviderSearchRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = IdpProviderSearchRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = IdpProviderSearchRequestValidationError{}
|
|
|
|
// Validate checks the field values on SecondFactorsResult with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *SecondFactorsResult) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// SecondFactorsResultValidationError is the validation error returned by
|
|
// SecondFactorsResult.Validate if the designated constraints aren't met.
|
|
type SecondFactorsResultValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e SecondFactorsResultValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e SecondFactorsResultValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e SecondFactorsResultValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e SecondFactorsResultValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e SecondFactorsResultValidationError) ErrorName() string {
|
|
return "SecondFactorsResultValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e SecondFactorsResultValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sSecondFactorsResult.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = SecondFactorsResultValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = SecondFactorsResultValidationError{}
|
|
|
|
// Validate checks the field values on SecondFactor with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *SecondFactor) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for SecondFactor
|
|
|
|
return nil
|
|
}
|
|
|
|
// SecondFactorValidationError is the validation error returned by
|
|
// SecondFactor.Validate if the designated constraints aren't met.
|
|
type SecondFactorValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e SecondFactorValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e SecondFactorValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e SecondFactorValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e SecondFactorValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e SecondFactorValidationError) ErrorName() string { return "SecondFactorValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e SecondFactorValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sSecondFactor.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = SecondFactorValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = SecondFactorValidationError{}
|
|
|
|
// Validate checks the field values on MultiFactorsResult with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *MultiFactorsResult) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// MultiFactorsResultValidationError is the validation error returned by
|
|
// MultiFactorsResult.Validate if the designated constraints aren't met.
|
|
type MultiFactorsResultValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e MultiFactorsResultValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e MultiFactorsResultValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e MultiFactorsResultValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e MultiFactorsResultValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e MultiFactorsResultValidationError) ErrorName() string {
|
|
return "MultiFactorsResultValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e MultiFactorsResultValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sMultiFactorsResult.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = MultiFactorsResultValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = MultiFactorsResultValidationError{}
|
|
|
|
// Validate checks the field values on MultiFactor with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *MultiFactor) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for MultiFactor
|
|
|
|
return nil
|
|
}
|
|
|
|
// MultiFactorValidationError is the validation error returned by
|
|
// MultiFactor.Validate if the designated constraints aren't met.
|
|
type MultiFactorValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e MultiFactorValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e MultiFactorValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e MultiFactorValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e MultiFactorValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e MultiFactorValidationError) ErrorName() string { return "MultiFactorValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e MultiFactorValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sMultiFactor.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = MultiFactorValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = MultiFactorValidationError{}
|
|
|
|
// Validate checks the field values on DefaultPasswordComplexityPolicy with the
|
|
// rules defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *DefaultPasswordComplexityPolicy) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for MinLength
|
|
|
|
// no validation rules for HasUppercase
|
|
|
|
// no validation rules for HasLowercase
|
|
|
|
// no validation rules for HasNumber
|
|
|
|
// no validation rules for HasSymbol
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return DefaultPasswordComplexityPolicyValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return DefaultPasswordComplexityPolicyValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// DefaultPasswordComplexityPolicyValidationError is the validation error
|
|
// returned by DefaultPasswordComplexityPolicy.Validate if the designated
|
|
// constraints aren't met.
|
|
type DefaultPasswordComplexityPolicyValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e DefaultPasswordComplexityPolicyValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e DefaultPasswordComplexityPolicyValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e DefaultPasswordComplexityPolicyValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e DefaultPasswordComplexityPolicyValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e DefaultPasswordComplexityPolicyValidationError) ErrorName() string {
|
|
return "DefaultPasswordComplexityPolicyValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e DefaultPasswordComplexityPolicyValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sDefaultPasswordComplexityPolicy.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = DefaultPasswordComplexityPolicyValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = DefaultPasswordComplexityPolicyValidationError{}
|
|
|
|
// Validate checks the field values on DefaultPasswordComplexityPolicyRequest
|
|
// with the rules defined in the proto definition for this message. If any
|
|
// rules are violated, an error is returned.
|
|
func (m *DefaultPasswordComplexityPolicyRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for MinLength
|
|
|
|
// no validation rules for HasUppercase
|
|
|
|
// no validation rules for HasLowercase
|
|
|
|
// no validation rules for HasNumber
|
|
|
|
// no validation rules for HasSymbol
|
|
|
|
return nil
|
|
}
|
|
|
|
// DefaultPasswordComplexityPolicyRequestValidationError is the validation
|
|
// error returned by DefaultPasswordComplexityPolicyRequest.Validate if the
|
|
// designated constraints aren't met.
|
|
type DefaultPasswordComplexityPolicyRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e DefaultPasswordComplexityPolicyRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e DefaultPasswordComplexityPolicyRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e DefaultPasswordComplexityPolicyRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e DefaultPasswordComplexityPolicyRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e DefaultPasswordComplexityPolicyRequestValidationError) ErrorName() string {
|
|
return "DefaultPasswordComplexityPolicyRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e DefaultPasswordComplexityPolicyRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sDefaultPasswordComplexityPolicyRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = DefaultPasswordComplexityPolicyRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = DefaultPasswordComplexityPolicyRequestValidationError{}
|
|
|
|
// Validate checks the field values on DefaultPasswordComplexityPolicyView with
|
|
// the rules defined in the proto definition for this message. If any rules
|
|
// are violated, an error is returned.
|
|
func (m *DefaultPasswordComplexityPolicyView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for MinLength
|
|
|
|
// no validation rules for HasUppercase
|
|
|
|
// no validation rules for HasLowercase
|
|
|
|
// no validation rules for HasNumber
|
|
|
|
// no validation rules for HasSymbol
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return DefaultPasswordComplexityPolicyViewValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return DefaultPasswordComplexityPolicyViewValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// DefaultPasswordComplexityPolicyViewValidationError is the validation error
|
|
// returned by DefaultPasswordComplexityPolicyView.Validate if the designated
|
|
// constraints aren't met.
|
|
type DefaultPasswordComplexityPolicyViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e DefaultPasswordComplexityPolicyViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e DefaultPasswordComplexityPolicyViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e DefaultPasswordComplexityPolicyViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e DefaultPasswordComplexityPolicyViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e DefaultPasswordComplexityPolicyViewValidationError) ErrorName() string {
|
|
return "DefaultPasswordComplexityPolicyViewValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e DefaultPasswordComplexityPolicyViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sDefaultPasswordComplexityPolicyView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = DefaultPasswordComplexityPolicyViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = DefaultPasswordComplexityPolicyViewValidationError{}
|
|
|
|
// Validate checks the field values on DefaultPasswordAgePolicy with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *DefaultPasswordAgePolicy) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for MaxAgeDays
|
|
|
|
// no validation rules for ExpireWarnDays
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return DefaultPasswordAgePolicyValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return DefaultPasswordAgePolicyValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// DefaultPasswordAgePolicyValidationError is the validation error returned by
|
|
// DefaultPasswordAgePolicy.Validate if the designated constraints aren't met.
|
|
type DefaultPasswordAgePolicyValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e DefaultPasswordAgePolicyValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e DefaultPasswordAgePolicyValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e DefaultPasswordAgePolicyValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e DefaultPasswordAgePolicyValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e DefaultPasswordAgePolicyValidationError) ErrorName() string {
|
|
return "DefaultPasswordAgePolicyValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e DefaultPasswordAgePolicyValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sDefaultPasswordAgePolicy.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = DefaultPasswordAgePolicyValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = DefaultPasswordAgePolicyValidationError{}
|
|
|
|
// Validate checks the field values on DefaultPasswordAgePolicyRequest with the
|
|
// rules defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *DefaultPasswordAgePolicyRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for MaxAgeDays
|
|
|
|
// no validation rules for ExpireWarnDays
|
|
|
|
return nil
|
|
}
|
|
|
|
// DefaultPasswordAgePolicyRequestValidationError is the validation error
|
|
// returned by DefaultPasswordAgePolicyRequest.Validate if the designated
|
|
// constraints aren't met.
|
|
type DefaultPasswordAgePolicyRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e DefaultPasswordAgePolicyRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e DefaultPasswordAgePolicyRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e DefaultPasswordAgePolicyRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e DefaultPasswordAgePolicyRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e DefaultPasswordAgePolicyRequestValidationError) ErrorName() string {
|
|
return "DefaultPasswordAgePolicyRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e DefaultPasswordAgePolicyRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sDefaultPasswordAgePolicyRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = DefaultPasswordAgePolicyRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = DefaultPasswordAgePolicyRequestValidationError{}
|
|
|
|
// Validate checks the field values on DefaultPasswordAgePolicyView with the
|
|
// rules defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *DefaultPasswordAgePolicyView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for MaxAgeDays
|
|
|
|
// no validation rules for ExpireWarnDays
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return DefaultPasswordAgePolicyViewValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return DefaultPasswordAgePolicyViewValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// DefaultPasswordAgePolicyViewValidationError is the validation error returned
|
|
// by DefaultPasswordAgePolicyView.Validate if the designated constraints
|
|
// aren't met.
|
|
type DefaultPasswordAgePolicyViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e DefaultPasswordAgePolicyViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e DefaultPasswordAgePolicyViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e DefaultPasswordAgePolicyViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e DefaultPasswordAgePolicyViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e DefaultPasswordAgePolicyViewValidationError) ErrorName() string {
|
|
return "DefaultPasswordAgePolicyViewValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e DefaultPasswordAgePolicyViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sDefaultPasswordAgePolicyView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = DefaultPasswordAgePolicyViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = DefaultPasswordAgePolicyViewValidationError{}
|
|
|
|
// Validate checks the field values on DefaultPasswordLockoutPolicy with the
|
|
// rules defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *DefaultPasswordLockoutPolicy) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for MaxAttempts
|
|
|
|
// no validation rules for ShowLockoutFailure
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return DefaultPasswordLockoutPolicyValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return DefaultPasswordLockoutPolicyValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// DefaultPasswordLockoutPolicyValidationError is the validation error returned
|
|
// by DefaultPasswordLockoutPolicy.Validate if the designated constraints
|
|
// aren't met.
|
|
type DefaultPasswordLockoutPolicyValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e DefaultPasswordLockoutPolicyValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e DefaultPasswordLockoutPolicyValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e DefaultPasswordLockoutPolicyValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e DefaultPasswordLockoutPolicyValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e DefaultPasswordLockoutPolicyValidationError) ErrorName() string {
|
|
return "DefaultPasswordLockoutPolicyValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e DefaultPasswordLockoutPolicyValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sDefaultPasswordLockoutPolicy.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = DefaultPasswordLockoutPolicyValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = DefaultPasswordLockoutPolicyValidationError{}
|
|
|
|
// Validate checks the field values on DefaultPasswordLockoutPolicyRequest with
|
|
// the rules defined in the proto definition for this message. If any rules
|
|
// are violated, an error is returned.
|
|
func (m *DefaultPasswordLockoutPolicyRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for MaxAttempts
|
|
|
|
// no validation rules for ShowLockoutFailure
|
|
|
|
return nil
|
|
}
|
|
|
|
// DefaultPasswordLockoutPolicyRequestValidationError is the validation error
|
|
// returned by DefaultPasswordLockoutPolicyRequest.Validate if the designated
|
|
// constraints aren't met.
|
|
type DefaultPasswordLockoutPolicyRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e DefaultPasswordLockoutPolicyRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e DefaultPasswordLockoutPolicyRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e DefaultPasswordLockoutPolicyRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e DefaultPasswordLockoutPolicyRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e DefaultPasswordLockoutPolicyRequestValidationError) ErrorName() string {
|
|
return "DefaultPasswordLockoutPolicyRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e DefaultPasswordLockoutPolicyRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sDefaultPasswordLockoutPolicyRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = DefaultPasswordLockoutPolicyRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = DefaultPasswordLockoutPolicyRequestValidationError{}
|
|
|
|
// Validate checks the field values on DefaultPasswordLockoutPolicyView with
|
|
// the rules defined in the proto definition for this message. If any rules
|
|
// are violated, an error is returned.
|
|
func (m *DefaultPasswordLockoutPolicyView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for MaxAttempts
|
|
|
|
// no validation rules for ShowLockoutFailure
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return DefaultPasswordLockoutPolicyViewValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return DefaultPasswordLockoutPolicyViewValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// DefaultPasswordLockoutPolicyViewValidationError is the validation error
|
|
// returned by DefaultPasswordLockoutPolicyView.Validate if the designated
|
|
// constraints aren't met.
|
|
type DefaultPasswordLockoutPolicyViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e DefaultPasswordLockoutPolicyViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e DefaultPasswordLockoutPolicyViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e DefaultPasswordLockoutPolicyViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e DefaultPasswordLockoutPolicyViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e DefaultPasswordLockoutPolicyViewValidationError) ErrorName() string {
|
|
return "DefaultPasswordLockoutPolicyViewValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e DefaultPasswordLockoutPolicyViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sDefaultPasswordLockoutPolicyView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = DefaultPasswordLockoutPolicyViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = DefaultPasswordLockoutPolicyViewValidationError{}
|