mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
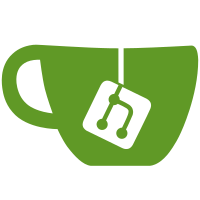
* fix: writesecret with --gitops doesn't need a kubeconfig * fix: writesecret with --gitops doesn't need a kubeconfig * fix: don't print error if writing secret succeeded
115 lines
2.7 KiB
Go
115 lines
2.7 KiB
Go
package cmds
|
|
|
|
import (
|
|
"errors"
|
|
"fmt"
|
|
"io/ioutil"
|
|
"os"
|
|
|
|
"github.com/caos/orbos/pkg/kubernetes/cli"
|
|
|
|
"github.com/caos/orbos/pkg/secret"
|
|
"github.com/caos/zitadel/operator/secrets"
|
|
"github.com/spf13/cobra"
|
|
)
|
|
|
|
func WriteSecretCommand(getRv GetRootValues) *cobra.Command {
|
|
|
|
var (
|
|
value string
|
|
file string
|
|
stdin bool
|
|
cmd = &cobra.Command{
|
|
Use: "writesecret [path]",
|
|
Short: "Encrypt a secret and push it to the repository",
|
|
Long: "Encrypt a secret and push it to the repository.\nIf no path is provided, a secret can interactively be chosen from a list of all possible secrets",
|
|
Args: cobra.MaximumNArgs(1),
|
|
Example: `zitadelctl writesecret database.bucket.serviceaccountjson.encrypted --file ~/googlecloudstoragesa.json
|
|
zitadelctl writesecret database.bucket.serviceaccountjson.encrypted --value "$(cat ~/googlecloudstoragesa.json)"
|
|
cat ~/googlecloudstoragesa.json | zitadelctl writesecret database.bucket.serviceaccountjson.encrypted --stdin`,
|
|
}
|
|
)
|
|
|
|
flags := cmd.Flags()
|
|
flags.StringVar(&value, "value", "", "Secret value to encrypt")
|
|
flags.StringVarP(&file, "file", "s", "", "File containing the value to encrypt")
|
|
flags.BoolVar(&stdin, "stdin", false, "Value to encrypt is read from standard input")
|
|
|
|
cmd.RunE = func(cmd *cobra.Command, args []string) (err error) {
|
|
|
|
path := ""
|
|
if len(args) > 0 {
|
|
path = args[0]
|
|
}
|
|
|
|
rv := getRv("writesecret", map[string]interface{}{"path": path, "value": value != "", "file": file, "stdin": stdin}, "")
|
|
defer func() {
|
|
err = rv.ErrFunc(err)
|
|
}()
|
|
|
|
monitor := rv.Monitor
|
|
orbConfig := rv.OrbConfig
|
|
gitClient := rv.GitClient
|
|
|
|
s, err := key(value, file, stdin)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
k8sClient, err := cli.Client(monitor, orbConfig, gitClient, rv.Kubeconfig, rv.Gitops, true)
|
|
if err != nil && !rv.Gitops {
|
|
return err
|
|
}
|
|
err = nil
|
|
|
|
return secret.Write(
|
|
monitor,
|
|
k8sClient,
|
|
path,
|
|
s,
|
|
"zitadelctl",
|
|
fmt.Sprintf(rv.Version),
|
|
secrets.GetAllSecretsFunc(monitor, path != "", rv.Gitops, gitClient, k8sClient, orbConfig),
|
|
secrets.PushFunc(monitor, rv.Gitops, gitClient, k8sClient),
|
|
)
|
|
}
|
|
return cmd
|
|
}
|
|
|
|
func key(value string, file string, stdin bool) (string, error) {
|
|
|
|
channels := 0
|
|
if value != "" {
|
|
channels++
|
|
}
|
|
if file != "" {
|
|
channels++
|
|
}
|
|
if stdin {
|
|
channels++
|
|
}
|
|
|
|
if channels != 1 {
|
|
return "", errors.New("Key must be provided eighter by value or by file path or by standard input")
|
|
}
|
|
|
|
if value != "" {
|
|
return value, nil
|
|
}
|
|
|
|
readFunc := func() ([]byte, error) {
|
|
return ioutil.ReadFile(file)
|
|
}
|
|
if stdin {
|
|
readFunc = func() ([]byte, error) {
|
|
return ioutil.ReadAll(os.Stdin)
|
|
}
|
|
}
|
|
|
|
key, err := readFunc()
|
|
if err != nil {
|
|
panic(err)
|
|
}
|
|
return string(key), err
|
|
}
|