mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
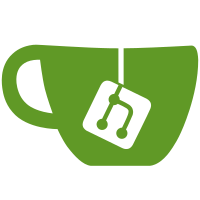
This implementation increases parallel write capabilities of the eventstore. Please have a look at the technical advisories: [05](https://zitadel.com/docs/support/advisory/a10005) and [06](https://zitadel.com/docs/support/advisory/a10006). The implementation of eventstore.push is rewritten and stored events are migrated to a new table `eventstore.events2`. If you are using cockroach: make sure that the database user of ZITADEL has `VIEWACTIVITY` grant. This is used to query events.
136 lines
3.4 KiB
Go
136 lines
3.4 KiB
Go
package policy
|
|
|
|
import (
|
|
"github.com/zitadel/zitadel/internal/errors"
|
|
"github.com/zitadel/zitadel/internal/eventstore"
|
|
)
|
|
|
|
const (
|
|
LockoutPolicyAddedEventType = "policy.lockout.added"
|
|
LockoutPolicyChangedEventType = "policy.lockout.changed"
|
|
LockoutPolicyRemovedEventType = "policy.lockout.removed"
|
|
)
|
|
|
|
type LockoutPolicyAddedEvent struct {
|
|
eventstore.BaseEvent `json:"-"`
|
|
|
|
MaxPasswordAttempts uint64 `json:"maxPasswordAttempts,omitempty"`
|
|
ShowLockOutFailures bool `json:"showLockOutFailures,omitempty"`
|
|
}
|
|
|
|
func (e *LockoutPolicyAddedEvent) Payload() interface{} {
|
|
return e
|
|
}
|
|
|
|
func (e *LockoutPolicyAddedEvent) UniqueConstraints() []*eventstore.UniqueConstraint {
|
|
return nil
|
|
}
|
|
|
|
func NewLockoutPolicyAddedEvent(
|
|
base *eventstore.BaseEvent,
|
|
maxAttempts uint64,
|
|
showLockOutFailures bool,
|
|
) *LockoutPolicyAddedEvent {
|
|
|
|
return &LockoutPolicyAddedEvent{
|
|
BaseEvent: *base,
|
|
MaxPasswordAttempts: maxAttempts,
|
|
ShowLockOutFailures: showLockOutFailures,
|
|
}
|
|
}
|
|
|
|
func LockoutPolicyAddedEventMapper(event eventstore.Event) (eventstore.Event, error) {
|
|
e := &LockoutPolicyAddedEvent{
|
|
BaseEvent: *eventstore.BaseEventFromRepo(event),
|
|
}
|
|
|
|
err := event.Unmarshal(e)
|
|
if err != nil {
|
|
return nil, errors.ThrowInternal(err, "POLIC-8XiVd", "unable to unmarshal policy")
|
|
}
|
|
|
|
return e, nil
|
|
}
|
|
|
|
type LockoutPolicyChangedEvent struct {
|
|
eventstore.BaseEvent `json:"-"`
|
|
|
|
MaxPasswordAttempts *uint64 `json:"maxPasswordAttempts,omitempty"`
|
|
ShowLockOutFailures *bool `json:"showLockOutFailures,omitempty"`
|
|
}
|
|
|
|
func (e *LockoutPolicyChangedEvent) Payload() interface{} {
|
|
return e
|
|
}
|
|
|
|
func (e *LockoutPolicyChangedEvent) UniqueConstraints() []*eventstore.UniqueConstraint {
|
|
return nil
|
|
}
|
|
|
|
func NewLockoutPolicyChangedEvent(
|
|
base *eventstore.BaseEvent,
|
|
changes []LockoutPolicyChanges,
|
|
) (*LockoutPolicyChangedEvent, error) {
|
|
if len(changes) == 0 {
|
|
return nil, errors.ThrowPreconditionFailed(nil, "POLICY-sdgh6", "Errors.NoChangesFound")
|
|
}
|
|
changeEvent := &LockoutPolicyChangedEvent{
|
|
BaseEvent: *base,
|
|
}
|
|
for _, change := range changes {
|
|
change(changeEvent)
|
|
}
|
|
return changeEvent, nil
|
|
}
|
|
|
|
type LockoutPolicyChanges func(*LockoutPolicyChangedEvent)
|
|
|
|
func ChangeMaxAttempts(maxAttempts uint64) func(*LockoutPolicyChangedEvent) {
|
|
return func(e *LockoutPolicyChangedEvent) {
|
|
e.MaxPasswordAttempts = &maxAttempts
|
|
}
|
|
}
|
|
|
|
func ChangeShowLockOutFailures(showLockOutFailures bool) func(*LockoutPolicyChangedEvent) {
|
|
return func(e *LockoutPolicyChangedEvent) {
|
|
e.ShowLockOutFailures = &showLockOutFailures
|
|
}
|
|
}
|
|
|
|
func LockoutPolicyChangedEventMapper(event eventstore.Event) (eventstore.Event, error) {
|
|
e := &LockoutPolicyChangedEvent{
|
|
BaseEvent: *eventstore.BaseEventFromRepo(event),
|
|
}
|
|
|
|
err := event.Unmarshal(e)
|
|
if err != nil {
|
|
return nil, errors.ThrowInternal(err, "POLIC-lWGRc", "unable to unmarshal policy")
|
|
}
|
|
|
|
return e, nil
|
|
}
|
|
|
|
type LockoutPolicyRemovedEvent struct {
|
|
eventstore.BaseEvent `json:"-"`
|
|
}
|
|
|
|
func (e *LockoutPolicyRemovedEvent) Payload() interface{} {
|
|
return nil
|
|
}
|
|
|
|
func (e *LockoutPolicyRemovedEvent) UniqueConstraints() []*eventstore.UniqueConstraint {
|
|
return nil
|
|
}
|
|
|
|
func NewLockoutPolicyRemovedEvent(base *eventstore.BaseEvent) *LockoutPolicyRemovedEvent {
|
|
return &LockoutPolicyRemovedEvent{
|
|
BaseEvent: *base,
|
|
}
|
|
}
|
|
|
|
func LockoutPolicyRemovedEventMapper(event eventstore.Event) (eventstore.Event, error) {
|
|
return &LockoutPolicyRemovedEvent{
|
|
BaseEvent: *eventstore.BaseEventFromRepo(event),
|
|
}, nil
|
|
}
|