mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-16 21:08:00 +00:00
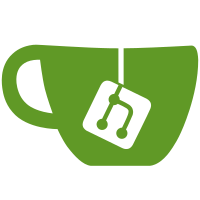
# Which Problems Are Solved Migration of milestones failed on our QA due to the new milestone Type enum being 0-indexed. The valid range was 0 till 5, inclusive. While on the previous zitadel version this was 1 till 6, inclusive. # How the Problems Are Solved Offset the first constant with `1`. # Additional Changes - none # Additional Context Introduced in https://github.com/zitadel/zitadel/pull/8788
140 lines
3.1 KiB
Go
140 lines
3.1 KiB
Go
package milestone
|
|
|
|
import (
|
|
"context"
|
|
"time"
|
|
|
|
"github.com/zitadel/zitadel/internal/eventstore"
|
|
)
|
|
|
|
//go:generate enumer -type Type -json -linecomment -transform=snake
|
|
type Type int
|
|
|
|
const (
|
|
InstanceCreated Type = iota + 1
|
|
AuthenticationSucceededOnInstance
|
|
ProjectCreated
|
|
ApplicationCreated
|
|
AuthenticationSucceededOnApplication
|
|
InstanceDeleted
|
|
)
|
|
|
|
const (
|
|
eventTypePrefix = "milestone."
|
|
ReachedEventType = eventTypePrefix + "reached"
|
|
PushedEventType = eventTypePrefix + "pushed"
|
|
)
|
|
|
|
type ReachedEvent struct {
|
|
*eventstore.BaseEvent `json:"-"`
|
|
MilestoneType Type `json:"type"`
|
|
ReachedDate *time.Time `json:"reachedDate,omitempty"` // Defaults to [eventstore.BaseEvent.Creation] when empty
|
|
}
|
|
|
|
// Payload implements eventstore.Command.
|
|
func (e *ReachedEvent) Payload() any {
|
|
return e
|
|
}
|
|
|
|
func (e *ReachedEvent) UniqueConstraints() []*eventstore.UniqueConstraint {
|
|
return nil
|
|
}
|
|
|
|
func (e *ReachedEvent) SetBaseEvent(b *eventstore.BaseEvent) {
|
|
e.BaseEvent = b
|
|
}
|
|
|
|
func (e *ReachedEvent) GetReachedDate() time.Time {
|
|
if e.ReachedDate != nil {
|
|
return *e.ReachedDate
|
|
}
|
|
return e.Creation
|
|
}
|
|
|
|
func NewReachedEvent(
|
|
ctx context.Context,
|
|
aggregate *Aggregate,
|
|
typ Type,
|
|
) *ReachedEvent {
|
|
return NewReachedEventWithDate(ctx, aggregate, typ, nil)
|
|
}
|
|
|
|
// NewReachedEventWithDate creates a [ReachedEvent] with a fixed Reached Date.
|
|
func NewReachedEventWithDate(
|
|
ctx context.Context,
|
|
aggregate *Aggregate,
|
|
typ Type,
|
|
reachedDate *time.Time,
|
|
) *ReachedEvent {
|
|
return &ReachedEvent{
|
|
BaseEvent: eventstore.NewBaseEventForPush(
|
|
ctx,
|
|
&aggregate.Aggregate,
|
|
ReachedEventType,
|
|
),
|
|
MilestoneType: typ,
|
|
ReachedDate: reachedDate,
|
|
}
|
|
}
|
|
|
|
type PushedEvent struct {
|
|
*eventstore.BaseEvent `json:"-"`
|
|
MilestoneType Type `json:"type"`
|
|
ExternalDomain string `json:"externalDomain"`
|
|
PrimaryDomain string `json:"primaryDomain"`
|
|
Endpoints []string `json:"endpoints"`
|
|
PushedDate *time.Time `json:"pushedDate,omitempty"` // Defaults to [eventstore.BaseEvent.Creation] when empty
|
|
}
|
|
|
|
// Payload implements eventstore.Command.
|
|
func (p *PushedEvent) Payload() any {
|
|
return p
|
|
}
|
|
|
|
func (p *PushedEvent) UniqueConstraints() []*eventstore.UniqueConstraint {
|
|
return nil
|
|
}
|
|
|
|
func (p *PushedEvent) SetBaseEvent(b *eventstore.BaseEvent) {
|
|
p.BaseEvent = b
|
|
}
|
|
|
|
func (e *PushedEvent) GetPushedDate() time.Time {
|
|
if e.PushedDate != nil {
|
|
return *e.PushedDate
|
|
}
|
|
return e.Creation
|
|
}
|
|
|
|
func NewPushedEvent(
|
|
ctx context.Context,
|
|
aggregate *Aggregate,
|
|
typ Type,
|
|
endpoints []string,
|
|
externalDomain string,
|
|
) *PushedEvent {
|
|
return NewPushedEventWithDate(ctx, aggregate, typ, endpoints, externalDomain, nil)
|
|
}
|
|
|
|
// NewPushedEventWithDate creates a [PushedEvent] with a fixed Pushed Date.
|
|
func NewPushedEventWithDate(
|
|
ctx context.Context,
|
|
aggregate *Aggregate,
|
|
typ Type,
|
|
endpoints []string,
|
|
externalDomain string,
|
|
pushedDate *time.Time,
|
|
) *PushedEvent {
|
|
return &PushedEvent{
|
|
BaseEvent: eventstore.NewBaseEventForPush(
|
|
ctx,
|
|
&aggregate.Aggregate,
|
|
PushedEventType,
|
|
),
|
|
MilestoneType: typ,
|
|
Endpoints: endpoints,
|
|
ExternalDomain: externalDomain,
|
|
PushedDate: pushedDate,
|
|
}
|
|
}
|