mirror of
https://github.com/zitadel/zitadel.git
synced 2025-07-11 23:38:31 +00:00
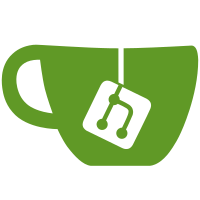
# Which Problems Are Solved We identified the need of caching. Currently we have a number of places where we use different ways of caching, like go maps or LRU. We might also want shared chaches in the future, like Redis-based or in special SQL tables. # How the Problems Are Solved Define a generic Cache interface which allows different implementations. - A noop implementation is provided and enabled as. - An implementation using go maps is provided - disabled in defaults.yaml - enabled in integration tests - Authz middleware instance objects are cached using the interface. # Additional Changes - Enabled integration test command raceflag - Fix a race condition in the limits integration test client - Fix a number of flaky integration tests. (Because zitadel is super fast now!) 🎸 🚀 # Additional Context Related to https://github.com/zitadel/zitadel/issues/8648
79 lines
2.2 KiB
Go
79 lines
2.2 KiB
Go
// Code generated by "enumer -type instanceIndex"; DO NOT EDIT.
|
|
|
|
package query
|
|
|
|
import (
|
|
"fmt"
|
|
"strings"
|
|
)
|
|
|
|
const _instanceIndexName = "instanceIndexByIDinstanceIndexByHost"
|
|
|
|
var _instanceIndexIndex = [...]uint8{0, 17, 36}
|
|
|
|
const _instanceIndexLowerName = "instanceindexbyidinstanceindexbyhost"
|
|
|
|
func (i instanceIndex) String() string {
|
|
if i < 0 || i >= instanceIndex(len(_instanceIndexIndex)-1) {
|
|
return fmt.Sprintf("instanceIndex(%d)", i)
|
|
}
|
|
return _instanceIndexName[_instanceIndexIndex[i]:_instanceIndexIndex[i+1]]
|
|
}
|
|
|
|
// An "invalid array index" compiler error signifies that the constant values have changed.
|
|
// Re-run the stringer command to generate them again.
|
|
func _instanceIndexNoOp() {
|
|
var x [1]struct{}
|
|
_ = x[instanceIndexByID-(0)]
|
|
_ = x[instanceIndexByHost-(1)]
|
|
}
|
|
|
|
var _instanceIndexValues = []instanceIndex{instanceIndexByID, instanceIndexByHost}
|
|
|
|
var _instanceIndexNameToValueMap = map[string]instanceIndex{
|
|
_instanceIndexName[0:17]: instanceIndexByID,
|
|
_instanceIndexLowerName[0:17]: instanceIndexByID,
|
|
_instanceIndexName[17:36]: instanceIndexByHost,
|
|
_instanceIndexLowerName[17:36]: instanceIndexByHost,
|
|
}
|
|
|
|
var _instanceIndexNames = []string{
|
|
_instanceIndexName[0:17],
|
|
_instanceIndexName[17:36],
|
|
}
|
|
|
|
// instanceIndexString retrieves an enum value from the enum constants string name.
|
|
// Throws an error if the param is not part of the enum.
|
|
func instanceIndexString(s string) (instanceIndex, error) {
|
|
if val, ok := _instanceIndexNameToValueMap[s]; ok {
|
|
return val, nil
|
|
}
|
|
|
|
if val, ok := _instanceIndexNameToValueMap[strings.ToLower(s)]; ok {
|
|
return val, nil
|
|
}
|
|
return 0, fmt.Errorf("%s does not belong to instanceIndex values", s)
|
|
}
|
|
|
|
// instanceIndexValues returns all values of the enum
|
|
func instanceIndexValues() []instanceIndex {
|
|
return _instanceIndexValues
|
|
}
|
|
|
|
// instanceIndexStrings returns a slice of all String values of the enum
|
|
func instanceIndexStrings() []string {
|
|
strs := make([]string, len(_instanceIndexNames))
|
|
copy(strs, _instanceIndexNames)
|
|
return strs
|
|
}
|
|
|
|
// IsAinstanceIndex returns "true" if the value is listed in the enum definition. "false" otherwise
|
|
func (i instanceIndex) IsAinstanceIndex() bool {
|
|
for _, v := range _instanceIndexValues {
|
|
if i == v {
|
|
return true
|
|
}
|
|
}
|
|
return false
|
|
}
|