mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
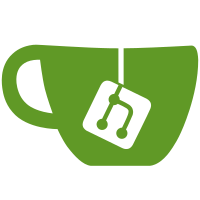
* backup new protoc plugin * backup * session * backup * initial implementation * change to specific events * implement tests * cleanup * refactor: use new protoc plugin for api v2 * change package * simplify code * cleanup * cleanup * fix merge * start queries * fix tests * improve returned values * add token to projection * tests * test db map * update query * permission checks * fix tests and linting * rework token creation * i18n * refactor token check and fix tests * session to PB test * request to query tests * cleanup proto * test user check * add comment * simplify database map type * Update docs/docs/guides/integrate/access-zitadel-system-api.md Co-authored-by: Tim Möhlmann <tim+github@zitadel.com> * fix test * cleanup * docs --------- Co-authored-by: Tim Möhlmann <tim+github@zitadel.com>
96 lines
1.9 KiB
Go
96 lines
1.9 KiB
Go
package database
|
|
|
|
import (
|
|
"database/sql/driver"
|
|
"encoding/json"
|
|
|
|
"github.com/jackc/pgtype"
|
|
)
|
|
|
|
type StringArray []string
|
|
|
|
// Scan implements the [database/sql.Scanner] interface.
|
|
func (s *StringArray) Scan(src any) error {
|
|
array := new(pgtype.TextArray)
|
|
if err := array.Scan(src); err != nil {
|
|
return err
|
|
}
|
|
if err := array.AssignTo(s); err != nil {
|
|
return err
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// Value implements the [database/sql/driver.Valuer] interface.
|
|
func (s StringArray) Value() (driver.Value, error) {
|
|
if len(s) == 0 {
|
|
return nil, nil
|
|
}
|
|
|
|
array := pgtype.TextArray{}
|
|
if err := array.Set(s); err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
return array.Value()
|
|
}
|
|
|
|
type enumField interface {
|
|
~int8 | ~uint8 | ~int16 | ~uint16 | ~int32 | ~uint32
|
|
}
|
|
|
|
type EnumArray[F enumField] []F
|
|
|
|
// Scan implements the [database/sql.Scanner] interface.
|
|
func (s *EnumArray[F]) Scan(src any) error {
|
|
array := new(pgtype.Int2Array)
|
|
if err := array.Scan(src); err != nil {
|
|
return err
|
|
}
|
|
ints := make([]int32, 0, len(array.Elements))
|
|
if err := array.AssignTo(&ints); err != nil {
|
|
return err
|
|
}
|
|
*s = make([]F, len(ints))
|
|
for i, a := range ints {
|
|
(*s)[i] = F(a)
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// Value implements the [database/sql/driver.Valuer] interface.
|
|
func (s EnumArray[F]) Value() (driver.Value, error) {
|
|
if len(s) == 0 {
|
|
return nil, nil
|
|
}
|
|
|
|
array := pgtype.Int2Array{}
|
|
if err := array.Set(s); err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
return array.Value()
|
|
}
|
|
|
|
type Map[V any] map[string]V
|
|
|
|
// Scan implements the [database/sql.Scanner] interface.
|
|
func (m *Map[V]) Scan(src any) error {
|
|
bytea := new(pgtype.Bytea)
|
|
if err := bytea.Scan(src); err != nil {
|
|
return err
|
|
}
|
|
if len(bytea.Bytes) == 0 {
|
|
return nil
|
|
}
|
|
return json.Unmarshal(bytea.Bytes, &m)
|
|
}
|
|
|
|
// Value implements the [database/sql/driver.Valuer] interface.
|
|
func (m Map[V]) Value() (driver.Value, error) {
|
|
if len(m) == 0 {
|
|
return nil, nil
|
|
}
|
|
return json.Marshal(m)
|
|
}
|