mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
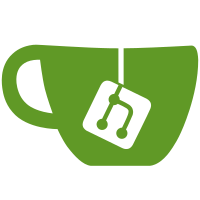
* feat: jwt idp * feat: command side * feat: add tests * fill idp views with jwt idps and return apis * add jwtEndpoint to jwt idp * begin jwt request handling * merge * handle jwt idp * cleanup * fixes * autoregister * get token from specific header name * error handling * fix texts * handle renderExternalNotFoundOption Co-authored-by: fabi <fabienne.gerschwiler@gmail.com>
84 lines
2.1 KiB
Go
84 lines
2.1 KiB
Go
package model
|
|
|
|
import (
|
|
"github.com/caos/zitadel/internal/crypto"
|
|
"github.com/caos/zitadel/internal/domain"
|
|
caos_errors "github.com/caos/zitadel/internal/errors"
|
|
|
|
"time"
|
|
)
|
|
|
|
type IDPConfigView struct {
|
|
AggregateID string
|
|
IDPConfigID string
|
|
Name string
|
|
StylingType IDPStylingType
|
|
AutoRegister bool
|
|
State IDPConfigState
|
|
CreationDate time.Time
|
|
ChangeDate time.Time
|
|
Sequence uint64
|
|
IDPProviderType IDPProviderType
|
|
|
|
IsOIDC bool
|
|
OIDCClientID string
|
|
OIDCClientSecret *crypto.CryptoValue
|
|
OIDCIssuer string
|
|
OIDCScopes []string
|
|
OIDCIDPDisplayNameMapping OIDCMappingField
|
|
OIDCUsernameMapping OIDCMappingField
|
|
OAuthAuthorizationEndpoint string
|
|
OAuthTokenEndpoint string
|
|
JWTEndpoint string
|
|
JWTIssuer string
|
|
JWTKeysEndpoint string
|
|
JWTHeaderName string
|
|
}
|
|
|
|
type IDPConfigSearchRequest struct {
|
|
Offset uint64
|
|
Limit uint64
|
|
SortingColumn IDPConfigSearchKey
|
|
Asc bool
|
|
Queries []*IDPConfigSearchQuery
|
|
}
|
|
|
|
type IDPConfigSearchKey int32
|
|
|
|
const (
|
|
IDPConfigSearchKeyUnspecified IDPConfigSearchKey = iota
|
|
IDPConfigSearchKeyName
|
|
IDPConfigSearchKeyAggregateID
|
|
IDPConfigSearchKeyIdpConfigID
|
|
IDPConfigSearchKeyIdpProviderType
|
|
)
|
|
|
|
type IDPConfigSearchQuery struct {
|
|
Key IDPConfigSearchKey
|
|
Method domain.SearchMethod
|
|
Value interface{}
|
|
}
|
|
|
|
type IDPConfigSearchResponse struct {
|
|
Offset uint64
|
|
Limit uint64
|
|
TotalResult uint64
|
|
Result []*IDPConfigView
|
|
Sequence uint64
|
|
Timestamp time.Time
|
|
}
|
|
|
|
func (r *IDPConfigSearchRequest) EnsureLimit(limit uint64) error {
|
|
if r.Limit > limit {
|
|
return caos_errors.ThrowInvalidArgument(nil, "SEARCH-Mv9sd", "Errors.Limit.ExceedsDefault")
|
|
}
|
|
if r.Limit == 0 {
|
|
r.Limit = limit
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (r *IDPConfigSearchRequest) AppendMyOrgQuery(orgID, iamID string) {
|
|
r.Queries = append(r.Queries, &IDPConfigSearchQuery{Key: IDPConfigSearchKeyAggregateID, Method: domain.SearchMethodIsOneOf, Value: []string{orgID, iamID}})
|
|
}
|