mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
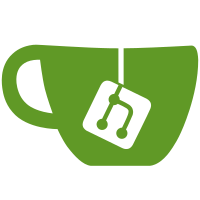
* fix: add prompt on oidc rp * fix: add prompt on oidc rp * fix: translation * fix: translation * fix: not existing login policy * fix: login policy * fix: identity provider detail * fix: idp update * fix: idps in login policy * fix: lint * fix: scss * fix: external idps on auth user detail * fix: idp create mapping fields * fix: remove idp provider * fix: angular lint * fix: login policy view * fix: translations
6863 lines
272 KiB
Go
6863 lines
272 KiB
Go
// Code generated by protoc-gen-go. DO NOT EDIT.
|
|
// versions:
|
|
// protoc-gen-go v1.25.0
|
|
// protoc v3.11.3
|
|
// source: auth.proto
|
|
|
|
package auth
|
|
|
|
import (
|
|
context "context"
|
|
_ "github.com/caos/zitadel/internal/protoc/protoc-gen-authoption/authoption"
|
|
message "github.com/caos/zitadel/pkg/grpc/message"
|
|
_ "github.com/envoyproxy/protoc-gen-validate/validate"
|
|
proto "github.com/golang/protobuf/proto"
|
|
empty "github.com/golang/protobuf/ptypes/empty"
|
|
_struct "github.com/golang/protobuf/ptypes/struct"
|
|
timestamp "github.com/golang/protobuf/ptypes/timestamp"
|
|
_ "github.com/grpc-ecosystem/grpc-gateway/protoc-gen-swagger/options"
|
|
_ "google.golang.org/genproto/googleapis/api/annotations"
|
|
grpc "google.golang.org/grpc"
|
|
codes "google.golang.org/grpc/codes"
|
|
status "google.golang.org/grpc/status"
|
|
protoreflect "google.golang.org/protobuf/reflect/protoreflect"
|
|
protoimpl "google.golang.org/protobuf/runtime/protoimpl"
|
|
reflect "reflect"
|
|
sync "sync"
|
|
)
|
|
|
|
const (
|
|
// Verify that this generated code is sufficiently up-to-date.
|
|
_ = protoimpl.EnforceVersion(20 - protoimpl.MinVersion)
|
|
// Verify that runtime/protoimpl is sufficiently up-to-date.
|
|
_ = protoimpl.EnforceVersion(protoimpl.MaxVersion - 20)
|
|
)
|
|
|
|
// This is a compile-time assertion that a sufficiently up-to-date version
|
|
// of the legacy proto package is being used.
|
|
const _ = proto.ProtoPackageIsVersion4
|
|
|
|
type UserSessionState int32
|
|
|
|
const (
|
|
UserSessionState_USERSESSIONSTATE_UNSPECIFIED UserSessionState = 0
|
|
UserSessionState_USERSESSIONSTATE_ACTIVE UserSessionState = 1
|
|
UserSessionState_USERSESSIONSTATE_TERMINATED UserSessionState = 2
|
|
)
|
|
|
|
// Enum value maps for UserSessionState.
|
|
var (
|
|
UserSessionState_name = map[int32]string{
|
|
0: "USERSESSIONSTATE_UNSPECIFIED",
|
|
1: "USERSESSIONSTATE_ACTIVE",
|
|
2: "USERSESSIONSTATE_TERMINATED",
|
|
}
|
|
UserSessionState_value = map[string]int32{
|
|
"USERSESSIONSTATE_UNSPECIFIED": 0,
|
|
"USERSESSIONSTATE_ACTIVE": 1,
|
|
"USERSESSIONSTATE_TERMINATED": 2,
|
|
}
|
|
)
|
|
|
|
func (x UserSessionState) Enum() *UserSessionState {
|
|
p := new(UserSessionState)
|
|
*p = x
|
|
return p
|
|
}
|
|
|
|
func (x UserSessionState) String() string {
|
|
return protoimpl.X.EnumStringOf(x.Descriptor(), protoreflect.EnumNumber(x))
|
|
}
|
|
|
|
func (UserSessionState) Descriptor() protoreflect.EnumDescriptor {
|
|
return file_auth_proto_enumTypes[0].Descriptor()
|
|
}
|
|
|
|
func (UserSessionState) Type() protoreflect.EnumType {
|
|
return &file_auth_proto_enumTypes[0]
|
|
}
|
|
|
|
func (x UserSessionState) Number() protoreflect.EnumNumber {
|
|
return protoreflect.EnumNumber(x)
|
|
}
|
|
|
|
// Deprecated: Use UserSessionState.Descriptor instead.
|
|
func (UserSessionState) EnumDescriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{0}
|
|
}
|
|
|
|
type MachineKeyType int32
|
|
|
|
const (
|
|
MachineKeyType_MACHINEKEY_UNSPECIFIED MachineKeyType = 0
|
|
MachineKeyType_MACHINEKEY_JSON MachineKeyType = 1
|
|
)
|
|
|
|
// Enum value maps for MachineKeyType.
|
|
var (
|
|
MachineKeyType_name = map[int32]string{
|
|
0: "MACHINEKEY_UNSPECIFIED",
|
|
1: "MACHINEKEY_JSON",
|
|
}
|
|
MachineKeyType_value = map[string]int32{
|
|
"MACHINEKEY_UNSPECIFIED": 0,
|
|
"MACHINEKEY_JSON": 1,
|
|
}
|
|
)
|
|
|
|
func (x MachineKeyType) Enum() *MachineKeyType {
|
|
p := new(MachineKeyType)
|
|
*p = x
|
|
return p
|
|
}
|
|
|
|
func (x MachineKeyType) String() string {
|
|
return protoimpl.X.EnumStringOf(x.Descriptor(), protoreflect.EnumNumber(x))
|
|
}
|
|
|
|
func (MachineKeyType) Descriptor() protoreflect.EnumDescriptor {
|
|
return file_auth_proto_enumTypes[1].Descriptor()
|
|
}
|
|
|
|
func (MachineKeyType) Type() protoreflect.EnumType {
|
|
return &file_auth_proto_enumTypes[1]
|
|
}
|
|
|
|
func (x MachineKeyType) Number() protoreflect.EnumNumber {
|
|
return protoreflect.EnumNumber(x)
|
|
}
|
|
|
|
// Deprecated: Use MachineKeyType.Descriptor instead.
|
|
func (MachineKeyType) EnumDescriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{1}
|
|
}
|
|
|
|
type UserState int32
|
|
|
|
const (
|
|
UserState_USERSTATE_UNSPECIFIED UserState = 0
|
|
UserState_USERSTATE_ACTIVE UserState = 1
|
|
UserState_USERSTATE_INACTIVE UserState = 2
|
|
UserState_USERSTATE_DELETED UserState = 3
|
|
UserState_USERSTATE_LOCKED UserState = 4
|
|
UserState_USERSTATE_SUSPEND UserState = 5
|
|
UserState_USERSTATE_INITIAL UserState = 6
|
|
)
|
|
|
|
// Enum value maps for UserState.
|
|
var (
|
|
UserState_name = map[int32]string{
|
|
0: "USERSTATE_UNSPECIFIED",
|
|
1: "USERSTATE_ACTIVE",
|
|
2: "USERSTATE_INACTIVE",
|
|
3: "USERSTATE_DELETED",
|
|
4: "USERSTATE_LOCKED",
|
|
5: "USERSTATE_SUSPEND",
|
|
6: "USERSTATE_INITIAL",
|
|
}
|
|
UserState_value = map[string]int32{
|
|
"USERSTATE_UNSPECIFIED": 0,
|
|
"USERSTATE_ACTIVE": 1,
|
|
"USERSTATE_INACTIVE": 2,
|
|
"USERSTATE_DELETED": 3,
|
|
"USERSTATE_LOCKED": 4,
|
|
"USERSTATE_SUSPEND": 5,
|
|
"USERSTATE_INITIAL": 6,
|
|
}
|
|
)
|
|
|
|
func (x UserState) Enum() *UserState {
|
|
p := new(UserState)
|
|
*p = x
|
|
return p
|
|
}
|
|
|
|
func (x UserState) String() string {
|
|
return protoimpl.X.EnumStringOf(x.Descriptor(), protoreflect.EnumNumber(x))
|
|
}
|
|
|
|
func (UserState) Descriptor() protoreflect.EnumDescriptor {
|
|
return file_auth_proto_enumTypes[2].Descriptor()
|
|
}
|
|
|
|
func (UserState) Type() protoreflect.EnumType {
|
|
return &file_auth_proto_enumTypes[2]
|
|
}
|
|
|
|
func (x UserState) Number() protoreflect.EnumNumber {
|
|
return protoreflect.EnumNumber(x)
|
|
}
|
|
|
|
// Deprecated: Use UserState.Descriptor instead.
|
|
func (UserState) EnumDescriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{2}
|
|
}
|
|
|
|
type Gender int32
|
|
|
|
const (
|
|
Gender_GENDER_UNSPECIFIED Gender = 0
|
|
Gender_GENDER_FEMALE Gender = 1
|
|
Gender_GENDER_MALE Gender = 2
|
|
Gender_GENDER_DIVERSE Gender = 3
|
|
)
|
|
|
|
// Enum value maps for Gender.
|
|
var (
|
|
Gender_name = map[int32]string{
|
|
0: "GENDER_UNSPECIFIED",
|
|
1: "GENDER_FEMALE",
|
|
2: "GENDER_MALE",
|
|
3: "GENDER_DIVERSE",
|
|
}
|
|
Gender_value = map[string]int32{
|
|
"GENDER_UNSPECIFIED": 0,
|
|
"GENDER_FEMALE": 1,
|
|
"GENDER_MALE": 2,
|
|
"GENDER_DIVERSE": 3,
|
|
}
|
|
)
|
|
|
|
func (x Gender) Enum() *Gender {
|
|
p := new(Gender)
|
|
*p = x
|
|
return p
|
|
}
|
|
|
|
func (x Gender) String() string {
|
|
return protoimpl.X.EnumStringOf(x.Descriptor(), protoreflect.EnumNumber(x))
|
|
}
|
|
|
|
func (Gender) Descriptor() protoreflect.EnumDescriptor {
|
|
return file_auth_proto_enumTypes[3].Descriptor()
|
|
}
|
|
|
|
func (Gender) Type() protoreflect.EnumType {
|
|
return &file_auth_proto_enumTypes[3]
|
|
}
|
|
|
|
func (x Gender) Number() protoreflect.EnumNumber {
|
|
return protoreflect.EnumNumber(x)
|
|
}
|
|
|
|
// Deprecated: Use Gender.Descriptor instead.
|
|
func (Gender) EnumDescriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{3}
|
|
}
|
|
|
|
type MfaType int32
|
|
|
|
const (
|
|
MfaType_MFATYPE_UNSPECIFIED MfaType = 0
|
|
MfaType_MFATYPE_SMS MfaType = 1
|
|
MfaType_MFATYPE_OTP MfaType = 2
|
|
)
|
|
|
|
// Enum value maps for MfaType.
|
|
var (
|
|
MfaType_name = map[int32]string{
|
|
0: "MFATYPE_UNSPECIFIED",
|
|
1: "MFATYPE_SMS",
|
|
2: "MFATYPE_OTP",
|
|
}
|
|
MfaType_value = map[string]int32{
|
|
"MFATYPE_UNSPECIFIED": 0,
|
|
"MFATYPE_SMS": 1,
|
|
"MFATYPE_OTP": 2,
|
|
}
|
|
)
|
|
|
|
func (x MfaType) Enum() *MfaType {
|
|
p := new(MfaType)
|
|
*p = x
|
|
return p
|
|
}
|
|
|
|
func (x MfaType) String() string {
|
|
return protoimpl.X.EnumStringOf(x.Descriptor(), protoreflect.EnumNumber(x))
|
|
}
|
|
|
|
func (MfaType) Descriptor() protoreflect.EnumDescriptor {
|
|
return file_auth_proto_enumTypes[4].Descriptor()
|
|
}
|
|
|
|
func (MfaType) Type() protoreflect.EnumType {
|
|
return &file_auth_proto_enumTypes[4]
|
|
}
|
|
|
|
func (x MfaType) Number() protoreflect.EnumNumber {
|
|
return protoreflect.EnumNumber(x)
|
|
}
|
|
|
|
// Deprecated: Use MfaType.Descriptor instead.
|
|
func (MfaType) EnumDescriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{4}
|
|
}
|
|
|
|
type MFAState int32
|
|
|
|
const (
|
|
MFAState_MFASTATE_UNSPECIFIED MFAState = 0
|
|
MFAState_MFASTATE_NOT_READY MFAState = 1
|
|
MFAState_MFASTATE_READY MFAState = 2
|
|
MFAState_MFASTATE_REMOVED MFAState = 3
|
|
)
|
|
|
|
// Enum value maps for MFAState.
|
|
var (
|
|
MFAState_name = map[int32]string{
|
|
0: "MFASTATE_UNSPECIFIED",
|
|
1: "MFASTATE_NOT_READY",
|
|
2: "MFASTATE_READY",
|
|
3: "MFASTATE_REMOVED",
|
|
}
|
|
MFAState_value = map[string]int32{
|
|
"MFASTATE_UNSPECIFIED": 0,
|
|
"MFASTATE_NOT_READY": 1,
|
|
"MFASTATE_READY": 2,
|
|
"MFASTATE_REMOVED": 3,
|
|
}
|
|
)
|
|
|
|
func (x MFAState) Enum() *MFAState {
|
|
p := new(MFAState)
|
|
*p = x
|
|
return p
|
|
}
|
|
|
|
func (x MFAState) String() string {
|
|
return protoimpl.X.EnumStringOf(x.Descriptor(), protoreflect.EnumNumber(x))
|
|
}
|
|
|
|
func (MFAState) Descriptor() protoreflect.EnumDescriptor {
|
|
return file_auth_proto_enumTypes[5].Descriptor()
|
|
}
|
|
|
|
func (MFAState) Type() protoreflect.EnumType {
|
|
return &file_auth_proto_enumTypes[5]
|
|
}
|
|
|
|
func (x MFAState) Number() protoreflect.EnumNumber {
|
|
return protoreflect.EnumNumber(x)
|
|
}
|
|
|
|
// Deprecated: Use MFAState.Descriptor instead.
|
|
func (MFAState) EnumDescriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{5}
|
|
}
|
|
|
|
type UserGrantSearchKey int32
|
|
|
|
const (
|
|
UserGrantSearchKey_UserGrantSearchKey_UNKNOWN UserGrantSearchKey = 0
|
|
UserGrantSearchKey_UserGrantSearchKey_ORG_ID UserGrantSearchKey = 1
|
|
UserGrantSearchKey_UserGrantSearchKey_PROJECT_ID UserGrantSearchKey = 2
|
|
)
|
|
|
|
// Enum value maps for UserGrantSearchKey.
|
|
var (
|
|
UserGrantSearchKey_name = map[int32]string{
|
|
0: "UserGrantSearchKey_UNKNOWN",
|
|
1: "UserGrantSearchKey_ORG_ID",
|
|
2: "UserGrantSearchKey_PROJECT_ID",
|
|
}
|
|
UserGrantSearchKey_value = map[string]int32{
|
|
"UserGrantSearchKey_UNKNOWN": 0,
|
|
"UserGrantSearchKey_ORG_ID": 1,
|
|
"UserGrantSearchKey_PROJECT_ID": 2,
|
|
}
|
|
)
|
|
|
|
func (x UserGrantSearchKey) Enum() *UserGrantSearchKey {
|
|
p := new(UserGrantSearchKey)
|
|
*p = x
|
|
return p
|
|
}
|
|
|
|
func (x UserGrantSearchKey) String() string {
|
|
return protoimpl.X.EnumStringOf(x.Descriptor(), protoreflect.EnumNumber(x))
|
|
}
|
|
|
|
func (UserGrantSearchKey) Descriptor() protoreflect.EnumDescriptor {
|
|
return file_auth_proto_enumTypes[6].Descriptor()
|
|
}
|
|
|
|
func (UserGrantSearchKey) Type() protoreflect.EnumType {
|
|
return &file_auth_proto_enumTypes[6]
|
|
}
|
|
|
|
func (x UserGrantSearchKey) Number() protoreflect.EnumNumber {
|
|
return protoreflect.EnumNumber(x)
|
|
}
|
|
|
|
// Deprecated: Use UserGrantSearchKey.Descriptor instead.
|
|
func (UserGrantSearchKey) EnumDescriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{6}
|
|
}
|
|
|
|
type MyProjectOrgSearchKey int32
|
|
|
|
const (
|
|
MyProjectOrgSearchKey_MYPROJECTORGSEARCHKEY_UNSPECIFIED MyProjectOrgSearchKey = 0
|
|
MyProjectOrgSearchKey_MYPROJECTORGSEARCHKEY_ORG_NAME MyProjectOrgSearchKey = 1
|
|
)
|
|
|
|
// Enum value maps for MyProjectOrgSearchKey.
|
|
var (
|
|
MyProjectOrgSearchKey_name = map[int32]string{
|
|
0: "MYPROJECTORGSEARCHKEY_UNSPECIFIED",
|
|
1: "MYPROJECTORGSEARCHKEY_ORG_NAME",
|
|
}
|
|
MyProjectOrgSearchKey_value = map[string]int32{
|
|
"MYPROJECTORGSEARCHKEY_UNSPECIFIED": 0,
|
|
"MYPROJECTORGSEARCHKEY_ORG_NAME": 1,
|
|
}
|
|
)
|
|
|
|
func (x MyProjectOrgSearchKey) Enum() *MyProjectOrgSearchKey {
|
|
p := new(MyProjectOrgSearchKey)
|
|
*p = x
|
|
return p
|
|
}
|
|
|
|
func (x MyProjectOrgSearchKey) String() string {
|
|
return protoimpl.X.EnumStringOf(x.Descriptor(), protoreflect.EnumNumber(x))
|
|
}
|
|
|
|
func (MyProjectOrgSearchKey) Descriptor() protoreflect.EnumDescriptor {
|
|
return file_auth_proto_enumTypes[7].Descriptor()
|
|
}
|
|
|
|
func (MyProjectOrgSearchKey) Type() protoreflect.EnumType {
|
|
return &file_auth_proto_enumTypes[7]
|
|
}
|
|
|
|
func (x MyProjectOrgSearchKey) Number() protoreflect.EnumNumber {
|
|
return protoreflect.EnumNumber(x)
|
|
}
|
|
|
|
// Deprecated: Use MyProjectOrgSearchKey.Descriptor instead.
|
|
func (MyProjectOrgSearchKey) EnumDescriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{7}
|
|
}
|
|
|
|
type SearchMethod int32
|
|
|
|
const (
|
|
SearchMethod_SEARCHMETHOD_EQUALS SearchMethod = 0
|
|
SearchMethod_SEARCHMETHOD_STARTS_WITH SearchMethod = 1
|
|
SearchMethod_SEARCHMETHOD_CONTAINS SearchMethod = 2
|
|
SearchMethod_SEARCHMETHOD_EQUALS_IGNORE_CASE SearchMethod = 3
|
|
SearchMethod_SEARCHMETHOD_STARTS_WITH_IGNORE_CASE SearchMethod = 4
|
|
SearchMethod_SEARCHMETHOD_CONTAINS_IGNORE_CASE SearchMethod = 5
|
|
)
|
|
|
|
// Enum value maps for SearchMethod.
|
|
var (
|
|
SearchMethod_name = map[int32]string{
|
|
0: "SEARCHMETHOD_EQUALS",
|
|
1: "SEARCHMETHOD_STARTS_WITH",
|
|
2: "SEARCHMETHOD_CONTAINS",
|
|
3: "SEARCHMETHOD_EQUALS_IGNORE_CASE",
|
|
4: "SEARCHMETHOD_STARTS_WITH_IGNORE_CASE",
|
|
5: "SEARCHMETHOD_CONTAINS_IGNORE_CASE",
|
|
}
|
|
SearchMethod_value = map[string]int32{
|
|
"SEARCHMETHOD_EQUALS": 0,
|
|
"SEARCHMETHOD_STARTS_WITH": 1,
|
|
"SEARCHMETHOD_CONTAINS": 2,
|
|
"SEARCHMETHOD_EQUALS_IGNORE_CASE": 3,
|
|
"SEARCHMETHOD_STARTS_WITH_IGNORE_CASE": 4,
|
|
"SEARCHMETHOD_CONTAINS_IGNORE_CASE": 5,
|
|
}
|
|
)
|
|
|
|
func (x SearchMethod) Enum() *SearchMethod {
|
|
p := new(SearchMethod)
|
|
*p = x
|
|
return p
|
|
}
|
|
|
|
func (x SearchMethod) String() string {
|
|
return protoimpl.X.EnumStringOf(x.Descriptor(), protoreflect.EnumNumber(x))
|
|
}
|
|
|
|
func (SearchMethod) Descriptor() protoreflect.EnumDescriptor {
|
|
return file_auth_proto_enumTypes[8].Descriptor()
|
|
}
|
|
|
|
func (SearchMethod) Type() protoreflect.EnumType {
|
|
return &file_auth_proto_enumTypes[8]
|
|
}
|
|
|
|
func (x SearchMethod) Number() protoreflect.EnumNumber {
|
|
return protoreflect.EnumNumber(x)
|
|
}
|
|
|
|
// Deprecated: Use SearchMethod.Descriptor instead.
|
|
func (SearchMethod) EnumDescriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{8}
|
|
}
|
|
|
|
type UserSessionViews struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
UserSessions []*UserSessionView `protobuf:"bytes,1,rep,name=user_sessions,json=userSessions,proto3" json:"user_sessions,omitempty"`
|
|
}
|
|
|
|
func (x *UserSessionViews) Reset() {
|
|
*x = UserSessionViews{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_proto_msgTypes[0]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *UserSessionViews) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*UserSessionViews) ProtoMessage() {}
|
|
|
|
func (x *UserSessionViews) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_proto_msgTypes[0]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use UserSessionViews.ProtoReflect.Descriptor instead.
|
|
func (*UserSessionViews) Descriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{0}
|
|
}
|
|
|
|
func (x *UserSessionViews) GetUserSessions() []*UserSessionView {
|
|
if x != nil {
|
|
return x.UserSessions
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type UserSessionView struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Id string `protobuf:"bytes,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
AgentId string `protobuf:"bytes,2,opt,name=agent_id,json=agentId,proto3" json:"agent_id,omitempty"`
|
|
AuthState UserSessionState `protobuf:"varint,3,opt,name=auth_state,json=authState,proto3,enum=caos.zitadel.auth.api.v1.UserSessionState" json:"auth_state,omitempty"`
|
|
UserId string `protobuf:"bytes,4,opt,name=user_id,json=userId,proto3" json:"user_id,omitempty"`
|
|
UserName string `protobuf:"bytes,5,opt,name=user_name,json=userName,proto3" json:"user_name,omitempty"`
|
|
Sequence uint64 `protobuf:"varint,6,opt,name=sequence,proto3" json:"sequence,omitempty"`
|
|
LoginName string `protobuf:"bytes,7,opt,name=login_name,json=loginName,proto3" json:"login_name,omitempty"`
|
|
DisplayName string `protobuf:"bytes,8,opt,name=display_name,json=displayName,proto3" json:"display_name,omitempty"`
|
|
}
|
|
|
|
func (x *UserSessionView) Reset() {
|
|
*x = UserSessionView{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_proto_msgTypes[1]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *UserSessionView) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*UserSessionView) ProtoMessage() {}
|
|
|
|
func (x *UserSessionView) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_proto_msgTypes[1]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use UserSessionView.ProtoReflect.Descriptor instead.
|
|
func (*UserSessionView) Descriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{1}
|
|
}
|
|
|
|
func (x *UserSessionView) GetId() string {
|
|
if x != nil {
|
|
return x.Id
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UserSessionView) GetAgentId() string {
|
|
if x != nil {
|
|
return x.AgentId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UserSessionView) GetAuthState() UserSessionState {
|
|
if x != nil {
|
|
return x.AuthState
|
|
}
|
|
return UserSessionState_USERSESSIONSTATE_UNSPECIFIED
|
|
}
|
|
|
|
func (x *UserSessionView) GetUserId() string {
|
|
if x != nil {
|
|
return x.UserId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UserSessionView) GetUserName() string {
|
|
if x != nil {
|
|
return x.UserName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UserSessionView) GetSequence() uint64 {
|
|
if x != nil {
|
|
return x.Sequence
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *UserSessionView) GetLoginName() string {
|
|
if x != nil {
|
|
return x.LoginName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UserSessionView) GetDisplayName() string {
|
|
if x != nil {
|
|
return x.DisplayName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type UserView struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Id string `protobuf:"bytes,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
State UserState `protobuf:"varint,2,opt,name=state,proto3,enum=caos.zitadel.auth.api.v1.UserState" json:"state,omitempty"`
|
|
CreationDate *timestamp.Timestamp `protobuf:"bytes,3,opt,name=creation_date,json=creationDate,proto3" json:"creation_date,omitempty"`
|
|
ChangeDate *timestamp.Timestamp `protobuf:"bytes,4,opt,name=change_date,json=changeDate,proto3" json:"change_date,omitempty"`
|
|
Sequence uint64 `protobuf:"varint,5,opt,name=sequence,proto3" json:"sequence,omitempty"`
|
|
LoginNames []string `protobuf:"bytes,6,rep,name=login_names,json=loginNames,proto3" json:"login_names,omitempty"`
|
|
PreferredLoginName string `protobuf:"bytes,7,opt,name=preferred_login_name,json=preferredLoginName,proto3" json:"preferred_login_name,omitempty"`
|
|
LastLogin *timestamp.Timestamp `protobuf:"bytes,8,opt,name=last_login,json=lastLogin,proto3" json:"last_login,omitempty"`
|
|
ResourceOwner string `protobuf:"bytes,9,opt,name=resource_owner,json=resourceOwner,proto3" json:"resource_owner,omitempty"`
|
|
UserName string `protobuf:"bytes,10,opt,name=user_name,json=userName,proto3" json:"user_name,omitempty"`
|
|
// Types that are assignable to User:
|
|
// *UserView_Human
|
|
// *UserView_Machine
|
|
User isUserView_User `protobuf_oneof:"user"`
|
|
}
|
|
|
|
func (x *UserView) Reset() {
|
|
*x = UserView{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_proto_msgTypes[2]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *UserView) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*UserView) ProtoMessage() {}
|
|
|
|
func (x *UserView) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_proto_msgTypes[2]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use UserView.ProtoReflect.Descriptor instead.
|
|
func (*UserView) Descriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{2}
|
|
}
|
|
|
|
func (x *UserView) GetId() string {
|
|
if x != nil {
|
|
return x.Id
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UserView) GetState() UserState {
|
|
if x != nil {
|
|
return x.State
|
|
}
|
|
return UserState_USERSTATE_UNSPECIFIED
|
|
}
|
|
|
|
func (x *UserView) GetCreationDate() *timestamp.Timestamp {
|
|
if x != nil {
|
|
return x.CreationDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *UserView) GetChangeDate() *timestamp.Timestamp {
|
|
if x != nil {
|
|
return x.ChangeDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *UserView) GetSequence() uint64 {
|
|
if x != nil {
|
|
return x.Sequence
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *UserView) GetLoginNames() []string {
|
|
if x != nil {
|
|
return x.LoginNames
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *UserView) GetPreferredLoginName() string {
|
|
if x != nil {
|
|
return x.PreferredLoginName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UserView) GetLastLogin() *timestamp.Timestamp {
|
|
if x != nil {
|
|
return x.LastLogin
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *UserView) GetResourceOwner() string {
|
|
if x != nil {
|
|
return x.ResourceOwner
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UserView) GetUserName() string {
|
|
if x != nil {
|
|
return x.UserName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UserView) GetUser() isUserView_User {
|
|
if m != nil {
|
|
return m.User
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *UserView) GetHuman() *HumanView {
|
|
if x, ok := x.GetUser().(*UserView_Human); ok {
|
|
return x.Human
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *UserView) GetMachine() *MachineView {
|
|
if x, ok := x.GetUser().(*UserView_Machine); ok {
|
|
return x.Machine
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type isUserView_User interface {
|
|
isUserView_User()
|
|
}
|
|
|
|
type UserView_Human struct {
|
|
Human *HumanView `protobuf:"bytes,11,opt,name=human,proto3,oneof"`
|
|
}
|
|
|
|
type UserView_Machine struct {
|
|
Machine *MachineView `protobuf:"bytes,12,opt,name=machine,proto3,oneof"`
|
|
}
|
|
|
|
func (*UserView_Human) isUserView_User() {}
|
|
|
|
func (*UserView_Machine) isUserView_User() {}
|
|
|
|
type MachineView struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
LastKeyAdded *timestamp.Timestamp `protobuf:"bytes,1,opt,name=last_key_added,json=lastKeyAdded,proto3" json:"last_key_added,omitempty"`
|
|
Name string `protobuf:"bytes,2,opt,name=name,proto3" json:"name,omitempty"`
|
|
Description string `protobuf:"bytes,3,opt,name=description,proto3" json:"description,omitempty"`
|
|
}
|
|
|
|
func (x *MachineView) Reset() {
|
|
*x = MachineView{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_proto_msgTypes[3]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *MachineView) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*MachineView) ProtoMessage() {}
|
|
|
|
func (x *MachineView) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_proto_msgTypes[3]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use MachineView.ProtoReflect.Descriptor instead.
|
|
func (*MachineView) Descriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{3}
|
|
}
|
|
|
|
func (x *MachineView) GetLastKeyAdded() *timestamp.Timestamp {
|
|
if x != nil {
|
|
return x.LastKeyAdded
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *MachineView) GetName() string {
|
|
if x != nil {
|
|
return x.Name
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *MachineView) GetDescription() string {
|
|
if x != nil {
|
|
return x.Description
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type MachineKeyView struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Id string `protobuf:"bytes,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
Type MachineKeyType `protobuf:"varint,2,opt,name=type,proto3,enum=caos.zitadel.auth.api.v1.MachineKeyType" json:"type,omitempty"`
|
|
Sequence uint64 `protobuf:"varint,3,opt,name=sequence,proto3" json:"sequence,omitempty"`
|
|
CreationDate *timestamp.Timestamp `protobuf:"bytes,4,opt,name=creation_date,json=creationDate,proto3" json:"creation_date,omitempty"`
|
|
ExpirationDate *timestamp.Timestamp `protobuf:"bytes,5,opt,name=expiration_date,json=expirationDate,proto3" json:"expiration_date,omitempty"`
|
|
}
|
|
|
|
func (x *MachineKeyView) Reset() {
|
|
*x = MachineKeyView{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_proto_msgTypes[4]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *MachineKeyView) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*MachineKeyView) ProtoMessage() {}
|
|
|
|
func (x *MachineKeyView) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_proto_msgTypes[4]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use MachineKeyView.ProtoReflect.Descriptor instead.
|
|
func (*MachineKeyView) Descriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{4}
|
|
}
|
|
|
|
func (x *MachineKeyView) GetId() string {
|
|
if x != nil {
|
|
return x.Id
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *MachineKeyView) GetType() MachineKeyType {
|
|
if x != nil {
|
|
return x.Type
|
|
}
|
|
return MachineKeyType_MACHINEKEY_UNSPECIFIED
|
|
}
|
|
|
|
func (x *MachineKeyView) GetSequence() uint64 {
|
|
if x != nil {
|
|
return x.Sequence
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *MachineKeyView) GetCreationDate() *timestamp.Timestamp {
|
|
if x != nil {
|
|
return x.CreationDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *MachineKeyView) GetExpirationDate() *timestamp.Timestamp {
|
|
if x != nil {
|
|
return x.ExpirationDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type HumanView struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
PasswordChanged *timestamp.Timestamp `protobuf:"bytes,1,opt,name=password_changed,json=passwordChanged,proto3" json:"password_changed,omitempty"`
|
|
FirstName string `protobuf:"bytes,2,opt,name=first_name,json=firstName,proto3" json:"first_name,omitempty"`
|
|
LastName string `protobuf:"bytes,3,opt,name=last_name,json=lastName,proto3" json:"last_name,omitempty"`
|
|
DisplayName string `protobuf:"bytes,4,opt,name=display_name,json=displayName,proto3" json:"display_name,omitempty"`
|
|
NickName string `protobuf:"bytes,5,opt,name=nick_name,json=nickName,proto3" json:"nick_name,omitempty"`
|
|
PreferredLanguage string `protobuf:"bytes,6,opt,name=preferred_language,json=preferredLanguage,proto3" json:"preferred_language,omitempty"`
|
|
Gender Gender `protobuf:"varint,7,opt,name=gender,proto3,enum=caos.zitadel.auth.api.v1.Gender" json:"gender,omitempty"`
|
|
Email string `protobuf:"bytes,8,opt,name=email,proto3" json:"email,omitempty"`
|
|
IsEmailVerified bool `protobuf:"varint,9,opt,name=is_email_verified,json=isEmailVerified,proto3" json:"is_email_verified,omitempty"`
|
|
Phone string `protobuf:"bytes,10,opt,name=phone,proto3" json:"phone,omitempty"`
|
|
IsPhoneVerified bool `protobuf:"varint,11,opt,name=is_phone_verified,json=isPhoneVerified,proto3" json:"is_phone_verified,omitempty"`
|
|
Country string `protobuf:"bytes,12,opt,name=country,proto3" json:"country,omitempty"`
|
|
Locality string `protobuf:"bytes,13,opt,name=locality,proto3" json:"locality,omitempty"`
|
|
PostalCode string `protobuf:"bytes,14,opt,name=postal_code,json=postalCode,proto3" json:"postal_code,omitempty"`
|
|
Region string `protobuf:"bytes,15,opt,name=region,proto3" json:"region,omitempty"`
|
|
StreetAddress string `protobuf:"bytes,16,opt,name=street_address,json=streetAddress,proto3" json:"street_address,omitempty"`
|
|
}
|
|
|
|
func (x *HumanView) Reset() {
|
|
*x = HumanView{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_proto_msgTypes[5]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *HumanView) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*HumanView) ProtoMessage() {}
|
|
|
|
func (x *HumanView) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_proto_msgTypes[5]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use HumanView.ProtoReflect.Descriptor instead.
|
|
func (*HumanView) Descriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{5}
|
|
}
|
|
|
|
func (x *HumanView) GetPasswordChanged() *timestamp.Timestamp {
|
|
if x != nil {
|
|
return x.PasswordChanged
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *HumanView) GetFirstName() string {
|
|
if x != nil {
|
|
return x.FirstName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *HumanView) GetLastName() string {
|
|
if x != nil {
|
|
return x.LastName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *HumanView) GetDisplayName() string {
|
|
if x != nil {
|
|
return x.DisplayName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *HumanView) GetNickName() string {
|
|
if x != nil {
|
|
return x.NickName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *HumanView) GetPreferredLanguage() string {
|
|
if x != nil {
|
|
return x.PreferredLanguage
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *HumanView) GetGender() Gender {
|
|
if x != nil {
|
|
return x.Gender
|
|
}
|
|
return Gender_GENDER_UNSPECIFIED
|
|
}
|
|
|
|
func (x *HumanView) GetEmail() string {
|
|
if x != nil {
|
|
return x.Email
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *HumanView) GetIsEmailVerified() bool {
|
|
if x != nil {
|
|
return x.IsEmailVerified
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *HumanView) GetPhone() string {
|
|
if x != nil {
|
|
return x.Phone
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *HumanView) GetIsPhoneVerified() bool {
|
|
if x != nil {
|
|
return x.IsPhoneVerified
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *HumanView) GetCountry() string {
|
|
if x != nil {
|
|
return x.Country
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *HumanView) GetLocality() string {
|
|
if x != nil {
|
|
return x.Locality
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *HumanView) GetPostalCode() string {
|
|
if x != nil {
|
|
return x.PostalCode
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *HumanView) GetRegion() string {
|
|
if x != nil {
|
|
return x.Region
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *HumanView) GetStreetAddress() string {
|
|
if x != nil {
|
|
return x.StreetAddress
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type UserProfile struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Id string `protobuf:"bytes,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
FirstName string `protobuf:"bytes,2,opt,name=first_name,json=firstName,proto3" json:"first_name,omitempty"`
|
|
LastName string `protobuf:"bytes,3,opt,name=last_name,json=lastName,proto3" json:"last_name,omitempty"`
|
|
NickName string `protobuf:"bytes,4,opt,name=nick_name,json=nickName,proto3" json:"nick_name,omitempty"`
|
|
DisplayName string `protobuf:"bytes,5,opt,name=display_name,json=displayName,proto3" json:"display_name,omitempty"`
|
|
PreferredLanguage string `protobuf:"bytes,6,opt,name=preferred_language,json=preferredLanguage,proto3" json:"preferred_language,omitempty"`
|
|
Gender Gender `protobuf:"varint,7,opt,name=gender,proto3,enum=caos.zitadel.auth.api.v1.Gender" json:"gender,omitempty"`
|
|
Sequence uint64 `protobuf:"varint,8,opt,name=sequence,proto3" json:"sequence,omitempty"`
|
|
CreationDate *timestamp.Timestamp `protobuf:"bytes,9,opt,name=creation_date,json=creationDate,proto3" json:"creation_date,omitempty"`
|
|
ChangeDate *timestamp.Timestamp `protobuf:"bytes,10,opt,name=change_date,json=changeDate,proto3" json:"change_date,omitempty"`
|
|
}
|
|
|
|
func (x *UserProfile) Reset() {
|
|
*x = UserProfile{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_proto_msgTypes[6]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *UserProfile) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*UserProfile) ProtoMessage() {}
|
|
|
|
func (x *UserProfile) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_proto_msgTypes[6]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use UserProfile.ProtoReflect.Descriptor instead.
|
|
func (*UserProfile) Descriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{6}
|
|
}
|
|
|
|
func (x *UserProfile) GetId() string {
|
|
if x != nil {
|
|
return x.Id
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UserProfile) GetFirstName() string {
|
|
if x != nil {
|
|
return x.FirstName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UserProfile) GetLastName() string {
|
|
if x != nil {
|
|
return x.LastName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UserProfile) GetNickName() string {
|
|
if x != nil {
|
|
return x.NickName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UserProfile) GetDisplayName() string {
|
|
if x != nil {
|
|
return x.DisplayName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UserProfile) GetPreferredLanguage() string {
|
|
if x != nil {
|
|
return x.PreferredLanguage
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UserProfile) GetGender() Gender {
|
|
if x != nil {
|
|
return x.Gender
|
|
}
|
|
return Gender_GENDER_UNSPECIFIED
|
|
}
|
|
|
|
func (x *UserProfile) GetSequence() uint64 {
|
|
if x != nil {
|
|
return x.Sequence
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *UserProfile) GetCreationDate() *timestamp.Timestamp {
|
|
if x != nil {
|
|
return x.CreationDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *UserProfile) GetChangeDate() *timestamp.Timestamp {
|
|
if x != nil {
|
|
return x.ChangeDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type UserProfileView struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Id string `protobuf:"bytes,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
FirstName string `protobuf:"bytes,2,opt,name=first_name,json=firstName,proto3" json:"first_name,omitempty"`
|
|
LastName string `protobuf:"bytes,3,opt,name=last_name,json=lastName,proto3" json:"last_name,omitempty"`
|
|
NickName string `protobuf:"bytes,4,opt,name=nick_name,json=nickName,proto3" json:"nick_name,omitempty"`
|
|
DisplayName string `protobuf:"bytes,5,opt,name=display_name,json=displayName,proto3" json:"display_name,omitempty"`
|
|
PreferredLanguage string `protobuf:"bytes,6,opt,name=preferred_language,json=preferredLanguage,proto3" json:"preferred_language,omitempty"`
|
|
Gender Gender `protobuf:"varint,7,opt,name=gender,proto3,enum=caos.zitadel.auth.api.v1.Gender" json:"gender,omitempty"`
|
|
Sequence uint64 `protobuf:"varint,8,opt,name=sequence,proto3" json:"sequence,omitempty"`
|
|
CreationDate *timestamp.Timestamp `protobuf:"bytes,9,opt,name=creation_date,json=creationDate,proto3" json:"creation_date,omitempty"`
|
|
ChangeDate *timestamp.Timestamp `protobuf:"bytes,10,opt,name=change_date,json=changeDate,proto3" json:"change_date,omitempty"`
|
|
LoginNames []string `protobuf:"bytes,11,rep,name=login_names,json=loginNames,proto3" json:"login_names,omitempty"`
|
|
PreferredLoginName string `protobuf:"bytes,12,opt,name=preferred_login_name,json=preferredLoginName,proto3" json:"preferred_login_name,omitempty"`
|
|
}
|
|
|
|
func (x *UserProfileView) Reset() {
|
|
*x = UserProfileView{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_proto_msgTypes[7]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *UserProfileView) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*UserProfileView) ProtoMessage() {}
|
|
|
|
func (x *UserProfileView) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_proto_msgTypes[7]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use UserProfileView.ProtoReflect.Descriptor instead.
|
|
func (*UserProfileView) Descriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{7}
|
|
}
|
|
|
|
func (x *UserProfileView) GetId() string {
|
|
if x != nil {
|
|
return x.Id
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UserProfileView) GetFirstName() string {
|
|
if x != nil {
|
|
return x.FirstName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UserProfileView) GetLastName() string {
|
|
if x != nil {
|
|
return x.LastName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UserProfileView) GetNickName() string {
|
|
if x != nil {
|
|
return x.NickName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UserProfileView) GetDisplayName() string {
|
|
if x != nil {
|
|
return x.DisplayName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UserProfileView) GetPreferredLanguage() string {
|
|
if x != nil {
|
|
return x.PreferredLanguage
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UserProfileView) GetGender() Gender {
|
|
if x != nil {
|
|
return x.Gender
|
|
}
|
|
return Gender_GENDER_UNSPECIFIED
|
|
}
|
|
|
|
func (x *UserProfileView) GetSequence() uint64 {
|
|
if x != nil {
|
|
return x.Sequence
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *UserProfileView) GetCreationDate() *timestamp.Timestamp {
|
|
if x != nil {
|
|
return x.CreationDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *UserProfileView) GetChangeDate() *timestamp.Timestamp {
|
|
if x != nil {
|
|
return x.ChangeDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *UserProfileView) GetLoginNames() []string {
|
|
if x != nil {
|
|
return x.LoginNames
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *UserProfileView) GetPreferredLoginName() string {
|
|
if x != nil {
|
|
return x.PreferredLoginName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type UpdateUserProfileRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
FirstName string `protobuf:"bytes,1,opt,name=first_name,json=firstName,proto3" json:"first_name,omitempty"`
|
|
LastName string `protobuf:"bytes,2,opt,name=last_name,json=lastName,proto3" json:"last_name,omitempty"`
|
|
NickName string `protobuf:"bytes,3,opt,name=nick_name,json=nickName,proto3" json:"nick_name,omitempty"`
|
|
PreferredLanguage string `protobuf:"bytes,4,opt,name=preferred_language,json=preferredLanguage,proto3" json:"preferred_language,omitempty"`
|
|
Gender Gender `protobuf:"varint,5,opt,name=gender,proto3,enum=caos.zitadel.auth.api.v1.Gender" json:"gender,omitempty"`
|
|
}
|
|
|
|
func (x *UpdateUserProfileRequest) Reset() {
|
|
*x = UpdateUserProfileRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_proto_msgTypes[8]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *UpdateUserProfileRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*UpdateUserProfileRequest) ProtoMessage() {}
|
|
|
|
func (x *UpdateUserProfileRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_proto_msgTypes[8]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use UpdateUserProfileRequest.ProtoReflect.Descriptor instead.
|
|
func (*UpdateUserProfileRequest) Descriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{8}
|
|
}
|
|
|
|
func (x *UpdateUserProfileRequest) GetFirstName() string {
|
|
if x != nil {
|
|
return x.FirstName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UpdateUserProfileRequest) GetLastName() string {
|
|
if x != nil {
|
|
return x.LastName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UpdateUserProfileRequest) GetNickName() string {
|
|
if x != nil {
|
|
return x.NickName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UpdateUserProfileRequest) GetPreferredLanguage() string {
|
|
if x != nil {
|
|
return x.PreferredLanguage
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UpdateUserProfileRequest) GetGender() Gender {
|
|
if x != nil {
|
|
return x.Gender
|
|
}
|
|
return Gender_GENDER_UNSPECIFIED
|
|
}
|
|
|
|
type ChangeUserNameRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
UserName string `protobuf:"bytes,1,opt,name=user_name,json=userName,proto3" json:"user_name,omitempty"`
|
|
}
|
|
|
|
func (x *ChangeUserNameRequest) Reset() {
|
|
*x = ChangeUserNameRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_proto_msgTypes[9]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *ChangeUserNameRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*ChangeUserNameRequest) ProtoMessage() {}
|
|
|
|
func (x *ChangeUserNameRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_proto_msgTypes[9]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use ChangeUserNameRequest.ProtoReflect.Descriptor instead.
|
|
func (*ChangeUserNameRequest) Descriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{9}
|
|
}
|
|
|
|
func (x *ChangeUserNameRequest) GetUserName() string {
|
|
if x != nil {
|
|
return x.UserName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type UserEmail struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Id string `protobuf:"bytes,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
Email string `protobuf:"bytes,2,opt,name=email,proto3" json:"email,omitempty"`
|
|
IsEmailVerified bool `protobuf:"varint,3,opt,name=isEmailVerified,proto3" json:"isEmailVerified,omitempty"`
|
|
Sequence uint64 `protobuf:"varint,4,opt,name=sequence,proto3" json:"sequence,omitempty"`
|
|
CreationDate *timestamp.Timestamp `protobuf:"bytes,5,opt,name=creation_date,json=creationDate,proto3" json:"creation_date,omitempty"`
|
|
ChangeDate *timestamp.Timestamp `protobuf:"bytes,6,opt,name=change_date,json=changeDate,proto3" json:"change_date,omitempty"`
|
|
}
|
|
|
|
func (x *UserEmail) Reset() {
|
|
*x = UserEmail{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_proto_msgTypes[10]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *UserEmail) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*UserEmail) ProtoMessage() {}
|
|
|
|
func (x *UserEmail) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_proto_msgTypes[10]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use UserEmail.ProtoReflect.Descriptor instead.
|
|
func (*UserEmail) Descriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{10}
|
|
}
|
|
|
|
func (x *UserEmail) GetId() string {
|
|
if x != nil {
|
|
return x.Id
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UserEmail) GetEmail() string {
|
|
if x != nil {
|
|
return x.Email
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UserEmail) GetIsEmailVerified() bool {
|
|
if x != nil {
|
|
return x.IsEmailVerified
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *UserEmail) GetSequence() uint64 {
|
|
if x != nil {
|
|
return x.Sequence
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *UserEmail) GetCreationDate() *timestamp.Timestamp {
|
|
if x != nil {
|
|
return x.CreationDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *UserEmail) GetChangeDate() *timestamp.Timestamp {
|
|
if x != nil {
|
|
return x.ChangeDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type UserEmailView struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Id string `protobuf:"bytes,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
Email string `protobuf:"bytes,2,opt,name=email,proto3" json:"email,omitempty"`
|
|
IsEmailVerified bool `protobuf:"varint,3,opt,name=isEmailVerified,proto3" json:"isEmailVerified,omitempty"`
|
|
Sequence uint64 `protobuf:"varint,4,opt,name=sequence,proto3" json:"sequence,omitempty"`
|
|
CreationDate *timestamp.Timestamp `protobuf:"bytes,5,opt,name=creation_date,json=creationDate,proto3" json:"creation_date,omitempty"`
|
|
ChangeDate *timestamp.Timestamp `protobuf:"bytes,6,opt,name=change_date,json=changeDate,proto3" json:"change_date,omitempty"`
|
|
}
|
|
|
|
func (x *UserEmailView) Reset() {
|
|
*x = UserEmailView{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_proto_msgTypes[11]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *UserEmailView) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*UserEmailView) ProtoMessage() {}
|
|
|
|
func (x *UserEmailView) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_proto_msgTypes[11]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use UserEmailView.ProtoReflect.Descriptor instead.
|
|
func (*UserEmailView) Descriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{11}
|
|
}
|
|
|
|
func (x *UserEmailView) GetId() string {
|
|
if x != nil {
|
|
return x.Id
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UserEmailView) GetEmail() string {
|
|
if x != nil {
|
|
return x.Email
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UserEmailView) GetIsEmailVerified() bool {
|
|
if x != nil {
|
|
return x.IsEmailVerified
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *UserEmailView) GetSequence() uint64 {
|
|
if x != nil {
|
|
return x.Sequence
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *UserEmailView) GetCreationDate() *timestamp.Timestamp {
|
|
if x != nil {
|
|
return x.CreationDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *UserEmailView) GetChangeDate() *timestamp.Timestamp {
|
|
if x != nil {
|
|
return x.ChangeDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type VerifyMyUserEmailRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Code string `protobuf:"bytes,1,opt,name=code,proto3" json:"code,omitempty"`
|
|
}
|
|
|
|
func (x *VerifyMyUserEmailRequest) Reset() {
|
|
*x = VerifyMyUserEmailRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_proto_msgTypes[12]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *VerifyMyUserEmailRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*VerifyMyUserEmailRequest) ProtoMessage() {}
|
|
|
|
func (x *VerifyMyUserEmailRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_proto_msgTypes[12]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use VerifyMyUserEmailRequest.ProtoReflect.Descriptor instead.
|
|
func (*VerifyMyUserEmailRequest) Descriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{12}
|
|
}
|
|
|
|
func (x *VerifyMyUserEmailRequest) GetCode() string {
|
|
if x != nil {
|
|
return x.Code
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type UpdateUserEmailRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Email string `protobuf:"bytes,1,opt,name=email,proto3" json:"email,omitempty"`
|
|
}
|
|
|
|
func (x *UpdateUserEmailRequest) Reset() {
|
|
*x = UpdateUserEmailRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_proto_msgTypes[13]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *UpdateUserEmailRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*UpdateUserEmailRequest) ProtoMessage() {}
|
|
|
|
func (x *UpdateUserEmailRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_proto_msgTypes[13]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use UpdateUserEmailRequest.ProtoReflect.Descriptor instead.
|
|
func (*UpdateUserEmailRequest) Descriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{13}
|
|
}
|
|
|
|
func (x *UpdateUserEmailRequest) GetEmail() string {
|
|
if x != nil {
|
|
return x.Email
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type UserPhone struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Id string `protobuf:"bytes,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
Phone string `protobuf:"bytes,2,opt,name=phone,proto3" json:"phone,omitempty"`
|
|
IsPhoneVerified bool `protobuf:"varint,3,opt,name=is_phone_verified,json=isPhoneVerified,proto3" json:"is_phone_verified,omitempty"`
|
|
Sequence uint64 `protobuf:"varint,4,opt,name=sequence,proto3" json:"sequence,omitempty"`
|
|
CreationDate *timestamp.Timestamp `protobuf:"bytes,5,opt,name=creation_date,json=creationDate,proto3" json:"creation_date,omitempty"`
|
|
ChangeDate *timestamp.Timestamp `protobuf:"bytes,6,opt,name=change_date,json=changeDate,proto3" json:"change_date,omitempty"`
|
|
}
|
|
|
|
func (x *UserPhone) Reset() {
|
|
*x = UserPhone{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_proto_msgTypes[14]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *UserPhone) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*UserPhone) ProtoMessage() {}
|
|
|
|
func (x *UserPhone) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_proto_msgTypes[14]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use UserPhone.ProtoReflect.Descriptor instead.
|
|
func (*UserPhone) Descriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{14}
|
|
}
|
|
|
|
func (x *UserPhone) GetId() string {
|
|
if x != nil {
|
|
return x.Id
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UserPhone) GetPhone() string {
|
|
if x != nil {
|
|
return x.Phone
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UserPhone) GetIsPhoneVerified() bool {
|
|
if x != nil {
|
|
return x.IsPhoneVerified
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *UserPhone) GetSequence() uint64 {
|
|
if x != nil {
|
|
return x.Sequence
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *UserPhone) GetCreationDate() *timestamp.Timestamp {
|
|
if x != nil {
|
|
return x.CreationDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *UserPhone) GetChangeDate() *timestamp.Timestamp {
|
|
if x != nil {
|
|
return x.ChangeDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type UserPhoneView struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Id string `protobuf:"bytes,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
Phone string `protobuf:"bytes,2,opt,name=phone,proto3" json:"phone,omitempty"`
|
|
IsPhoneVerified bool `protobuf:"varint,3,opt,name=is_phone_verified,json=isPhoneVerified,proto3" json:"is_phone_verified,omitempty"`
|
|
Sequence uint64 `protobuf:"varint,4,opt,name=sequence,proto3" json:"sequence,omitempty"`
|
|
CreationDate *timestamp.Timestamp `protobuf:"bytes,5,opt,name=creation_date,json=creationDate,proto3" json:"creation_date,omitempty"`
|
|
ChangeDate *timestamp.Timestamp `protobuf:"bytes,6,opt,name=change_date,json=changeDate,proto3" json:"change_date,omitempty"`
|
|
}
|
|
|
|
func (x *UserPhoneView) Reset() {
|
|
*x = UserPhoneView{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_proto_msgTypes[15]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *UserPhoneView) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*UserPhoneView) ProtoMessage() {}
|
|
|
|
func (x *UserPhoneView) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_proto_msgTypes[15]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use UserPhoneView.ProtoReflect.Descriptor instead.
|
|
func (*UserPhoneView) Descriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{15}
|
|
}
|
|
|
|
func (x *UserPhoneView) GetId() string {
|
|
if x != nil {
|
|
return x.Id
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UserPhoneView) GetPhone() string {
|
|
if x != nil {
|
|
return x.Phone
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UserPhoneView) GetIsPhoneVerified() bool {
|
|
if x != nil {
|
|
return x.IsPhoneVerified
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *UserPhoneView) GetSequence() uint64 {
|
|
if x != nil {
|
|
return x.Sequence
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *UserPhoneView) GetCreationDate() *timestamp.Timestamp {
|
|
if x != nil {
|
|
return x.CreationDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *UserPhoneView) GetChangeDate() *timestamp.Timestamp {
|
|
if x != nil {
|
|
return x.ChangeDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type UpdateUserPhoneRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Phone string `protobuf:"bytes,1,opt,name=phone,proto3" json:"phone,omitempty"`
|
|
}
|
|
|
|
func (x *UpdateUserPhoneRequest) Reset() {
|
|
*x = UpdateUserPhoneRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_proto_msgTypes[16]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *UpdateUserPhoneRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*UpdateUserPhoneRequest) ProtoMessage() {}
|
|
|
|
func (x *UpdateUserPhoneRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_proto_msgTypes[16]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use UpdateUserPhoneRequest.ProtoReflect.Descriptor instead.
|
|
func (*UpdateUserPhoneRequest) Descriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{16}
|
|
}
|
|
|
|
func (x *UpdateUserPhoneRequest) GetPhone() string {
|
|
if x != nil {
|
|
return x.Phone
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type VerifyUserPhoneRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Code string `protobuf:"bytes,1,opt,name=code,proto3" json:"code,omitempty"`
|
|
}
|
|
|
|
func (x *VerifyUserPhoneRequest) Reset() {
|
|
*x = VerifyUserPhoneRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_proto_msgTypes[17]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *VerifyUserPhoneRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*VerifyUserPhoneRequest) ProtoMessage() {}
|
|
|
|
func (x *VerifyUserPhoneRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_proto_msgTypes[17]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use VerifyUserPhoneRequest.ProtoReflect.Descriptor instead.
|
|
func (*VerifyUserPhoneRequest) Descriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{17}
|
|
}
|
|
|
|
func (x *VerifyUserPhoneRequest) GetCode() string {
|
|
if x != nil {
|
|
return x.Code
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type UserAddress struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Id string `protobuf:"bytes,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
Country string `protobuf:"bytes,2,opt,name=country,proto3" json:"country,omitempty"`
|
|
Locality string `protobuf:"bytes,3,opt,name=locality,proto3" json:"locality,omitempty"`
|
|
PostalCode string `protobuf:"bytes,4,opt,name=postal_code,json=postalCode,proto3" json:"postal_code,omitempty"`
|
|
Region string `protobuf:"bytes,5,opt,name=region,proto3" json:"region,omitempty"`
|
|
StreetAddress string `protobuf:"bytes,6,opt,name=street_address,json=streetAddress,proto3" json:"street_address,omitempty"`
|
|
Sequence uint64 `protobuf:"varint,7,opt,name=sequence,proto3" json:"sequence,omitempty"`
|
|
CreationDate *timestamp.Timestamp `protobuf:"bytes,8,opt,name=creation_date,json=creationDate,proto3" json:"creation_date,omitempty"`
|
|
ChangeDate *timestamp.Timestamp `protobuf:"bytes,9,opt,name=change_date,json=changeDate,proto3" json:"change_date,omitempty"`
|
|
}
|
|
|
|
func (x *UserAddress) Reset() {
|
|
*x = UserAddress{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_proto_msgTypes[18]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *UserAddress) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*UserAddress) ProtoMessage() {}
|
|
|
|
func (x *UserAddress) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_proto_msgTypes[18]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use UserAddress.ProtoReflect.Descriptor instead.
|
|
func (*UserAddress) Descriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{18}
|
|
}
|
|
|
|
func (x *UserAddress) GetId() string {
|
|
if x != nil {
|
|
return x.Id
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UserAddress) GetCountry() string {
|
|
if x != nil {
|
|
return x.Country
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UserAddress) GetLocality() string {
|
|
if x != nil {
|
|
return x.Locality
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UserAddress) GetPostalCode() string {
|
|
if x != nil {
|
|
return x.PostalCode
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UserAddress) GetRegion() string {
|
|
if x != nil {
|
|
return x.Region
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UserAddress) GetStreetAddress() string {
|
|
if x != nil {
|
|
return x.StreetAddress
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UserAddress) GetSequence() uint64 {
|
|
if x != nil {
|
|
return x.Sequence
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *UserAddress) GetCreationDate() *timestamp.Timestamp {
|
|
if x != nil {
|
|
return x.CreationDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *UserAddress) GetChangeDate() *timestamp.Timestamp {
|
|
if x != nil {
|
|
return x.ChangeDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type UserAddressView struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Id string `protobuf:"bytes,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
Country string `protobuf:"bytes,2,opt,name=country,proto3" json:"country,omitempty"`
|
|
Locality string `protobuf:"bytes,3,opt,name=locality,proto3" json:"locality,omitempty"`
|
|
PostalCode string `protobuf:"bytes,4,opt,name=postal_code,json=postalCode,proto3" json:"postal_code,omitempty"`
|
|
Region string `protobuf:"bytes,5,opt,name=region,proto3" json:"region,omitempty"`
|
|
StreetAddress string `protobuf:"bytes,6,opt,name=street_address,json=streetAddress,proto3" json:"street_address,omitempty"`
|
|
Sequence uint64 `protobuf:"varint,7,opt,name=sequence,proto3" json:"sequence,omitempty"`
|
|
CreationDate *timestamp.Timestamp `protobuf:"bytes,8,opt,name=creation_date,json=creationDate,proto3" json:"creation_date,omitempty"`
|
|
ChangeDate *timestamp.Timestamp `protobuf:"bytes,9,opt,name=change_date,json=changeDate,proto3" json:"change_date,omitempty"`
|
|
}
|
|
|
|
func (x *UserAddressView) Reset() {
|
|
*x = UserAddressView{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_proto_msgTypes[19]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *UserAddressView) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*UserAddressView) ProtoMessage() {}
|
|
|
|
func (x *UserAddressView) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_proto_msgTypes[19]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use UserAddressView.ProtoReflect.Descriptor instead.
|
|
func (*UserAddressView) Descriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{19}
|
|
}
|
|
|
|
func (x *UserAddressView) GetId() string {
|
|
if x != nil {
|
|
return x.Id
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UserAddressView) GetCountry() string {
|
|
if x != nil {
|
|
return x.Country
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UserAddressView) GetLocality() string {
|
|
if x != nil {
|
|
return x.Locality
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UserAddressView) GetPostalCode() string {
|
|
if x != nil {
|
|
return x.PostalCode
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UserAddressView) GetRegion() string {
|
|
if x != nil {
|
|
return x.Region
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UserAddressView) GetStreetAddress() string {
|
|
if x != nil {
|
|
return x.StreetAddress
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UserAddressView) GetSequence() uint64 {
|
|
if x != nil {
|
|
return x.Sequence
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *UserAddressView) GetCreationDate() *timestamp.Timestamp {
|
|
if x != nil {
|
|
return x.CreationDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *UserAddressView) GetChangeDate() *timestamp.Timestamp {
|
|
if x != nil {
|
|
return x.ChangeDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type UpdateUserAddressRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Country string `protobuf:"bytes,1,opt,name=country,proto3" json:"country,omitempty"`
|
|
Locality string `protobuf:"bytes,2,opt,name=locality,proto3" json:"locality,omitempty"`
|
|
PostalCode string `protobuf:"bytes,3,opt,name=postal_code,json=postalCode,proto3" json:"postal_code,omitempty"`
|
|
Region string `protobuf:"bytes,4,opt,name=region,proto3" json:"region,omitempty"`
|
|
StreetAddress string `protobuf:"bytes,5,opt,name=street_address,json=streetAddress,proto3" json:"street_address,omitempty"`
|
|
}
|
|
|
|
func (x *UpdateUserAddressRequest) Reset() {
|
|
*x = UpdateUserAddressRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_proto_msgTypes[20]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *UpdateUserAddressRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*UpdateUserAddressRequest) ProtoMessage() {}
|
|
|
|
func (x *UpdateUserAddressRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_proto_msgTypes[20]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use UpdateUserAddressRequest.ProtoReflect.Descriptor instead.
|
|
func (*UpdateUserAddressRequest) Descriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{20}
|
|
}
|
|
|
|
func (x *UpdateUserAddressRequest) GetCountry() string {
|
|
if x != nil {
|
|
return x.Country
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UpdateUserAddressRequest) GetLocality() string {
|
|
if x != nil {
|
|
return x.Locality
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UpdateUserAddressRequest) GetPostalCode() string {
|
|
if x != nil {
|
|
return x.PostalCode
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UpdateUserAddressRequest) GetRegion() string {
|
|
if x != nil {
|
|
return x.Region
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UpdateUserAddressRequest) GetStreetAddress() string {
|
|
if x != nil {
|
|
return x.StreetAddress
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type PasswordChange struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
OldPassword string `protobuf:"bytes,1,opt,name=old_password,json=oldPassword,proto3" json:"old_password,omitempty"`
|
|
NewPassword string `protobuf:"bytes,2,opt,name=new_password,json=newPassword,proto3" json:"new_password,omitempty"`
|
|
}
|
|
|
|
func (x *PasswordChange) Reset() {
|
|
*x = PasswordChange{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_proto_msgTypes[21]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *PasswordChange) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*PasswordChange) ProtoMessage() {}
|
|
|
|
func (x *PasswordChange) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_proto_msgTypes[21]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use PasswordChange.ProtoReflect.Descriptor instead.
|
|
func (*PasswordChange) Descriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{21}
|
|
}
|
|
|
|
func (x *PasswordChange) GetOldPassword() string {
|
|
if x != nil {
|
|
return x.OldPassword
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *PasswordChange) GetNewPassword() string {
|
|
if x != nil {
|
|
return x.NewPassword
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type VerifyMfaOtp struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Code string `protobuf:"bytes,1,opt,name=code,proto3" json:"code,omitempty"`
|
|
}
|
|
|
|
func (x *VerifyMfaOtp) Reset() {
|
|
*x = VerifyMfaOtp{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_proto_msgTypes[22]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *VerifyMfaOtp) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*VerifyMfaOtp) ProtoMessage() {}
|
|
|
|
func (x *VerifyMfaOtp) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_proto_msgTypes[22]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use VerifyMfaOtp.ProtoReflect.Descriptor instead.
|
|
func (*VerifyMfaOtp) Descriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{22}
|
|
}
|
|
|
|
func (x *VerifyMfaOtp) GetCode() string {
|
|
if x != nil {
|
|
return x.Code
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type MultiFactors struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Mfas []*MultiFactor `protobuf:"bytes,1,rep,name=mfas,proto3" json:"mfas,omitempty"`
|
|
}
|
|
|
|
func (x *MultiFactors) Reset() {
|
|
*x = MultiFactors{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_proto_msgTypes[23]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *MultiFactors) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*MultiFactors) ProtoMessage() {}
|
|
|
|
func (x *MultiFactors) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_proto_msgTypes[23]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use MultiFactors.ProtoReflect.Descriptor instead.
|
|
func (*MultiFactors) Descriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{23}
|
|
}
|
|
|
|
func (x *MultiFactors) GetMfas() []*MultiFactor {
|
|
if x != nil {
|
|
return x.Mfas
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type MultiFactor struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Type MfaType `protobuf:"varint,1,opt,name=type,proto3,enum=caos.zitadel.auth.api.v1.MfaType" json:"type,omitempty"`
|
|
State MFAState `protobuf:"varint,2,opt,name=state,proto3,enum=caos.zitadel.auth.api.v1.MFAState" json:"state,omitempty"`
|
|
}
|
|
|
|
func (x *MultiFactor) Reset() {
|
|
*x = MultiFactor{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_proto_msgTypes[24]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *MultiFactor) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*MultiFactor) ProtoMessage() {}
|
|
|
|
func (x *MultiFactor) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_proto_msgTypes[24]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use MultiFactor.ProtoReflect.Descriptor instead.
|
|
func (*MultiFactor) Descriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{24}
|
|
}
|
|
|
|
func (x *MultiFactor) GetType() MfaType {
|
|
if x != nil {
|
|
return x.Type
|
|
}
|
|
return MfaType_MFATYPE_UNSPECIFIED
|
|
}
|
|
|
|
func (x *MultiFactor) GetState() MFAState {
|
|
if x != nil {
|
|
return x.State
|
|
}
|
|
return MFAState_MFASTATE_UNSPECIFIED
|
|
}
|
|
|
|
type MfaOtpResponse struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
UserId string `protobuf:"bytes,1,opt,name=user_id,json=userId,proto3" json:"user_id,omitempty"`
|
|
Url string `protobuf:"bytes,2,opt,name=url,proto3" json:"url,omitempty"`
|
|
Secret string `protobuf:"bytes,3,opt,name=secret,proto3" json:"secret,omitempty"`
|
|
State MFAState `protobuf:"varint,4,opt,name=state,proto3,enum=caos.zitadel.auth.api.v1.MFAState" json:"state,omitempty"`
|
|
}
|
|
|
|
func (x *MfaOtpResponse) Reset() {
|
|
*x = MfaOtpResponse{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_proto_msgTypes[25]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *MfaOtpResponse) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*MfaOtpResponse) ProtoMessage() {}
|
|
|
|
func (x *MfaOtpResponse) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_proto_msgTypes[25]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use MfaOtpResponse.ProtoReflect.Descriptor instead.
|
|
func (*MfaOtpResponse) Descriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{25}
|
|
}
|
|
|
|
func (x *MfaOtpResponse) GetUserId() string {
|
|
if x != nil {
|
|
return x.UserId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *MfaOtpResponse) GetUrl() string {
|
|
if x != nil {
|
|
return x.Url
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *MfaOtpResponse) GetSecret() string {
|
|
if x != nil {
|
|
return x.Secret
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *MfaOtpResponse) GetState() MFAState {
|
|
if x != nil {
|
|
return x.State
|
|
}
|
|
return MFAState_MFASTATE_UNSPECIFIED
|
|
}
|
|
|
|
type UserGrantSearchRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Offset uint64 `protobuf:"varint,1,opt,name=offset,proto3" json:"offset,omitempty"`
|
|
Limit uint64 `protobuf:"varint,2,opt,name=limit,proto3" json:"limit,omitempty"`
|
|
SortingColumn UserGrantSearchKey `protobuf:"varint,3,opt,name=sorting_column,json=sortingColumn,proto3,enum=caos.zitadel.auth.api.v1.UserGrantSearchKey" json:"sorting_column,omitempty"`
|
|
Asc bool `protobuf:"varint,4,opt,name=asc,proto3" json:"asc,omitempty"`
|
|
Queries []*UserGrantSearchQuery `protobuf:"bytes,5,rep,name=queries,proto3" json:"queries,omitempty"`
|
|
}
|
|
|
|
func (x *UserGrantSearchRequest) Reset() {
|
|
*x = UserGrantSearchRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_proto_msgTypes[26]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *UserGrantSearchRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*UserGrantSearchRequest) ProtoMessage() {}
|
|
|
|
func (x *UserGrantSearchRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_proto_msgTypes[26]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use UserGrantSearchRequest.ProtoReflect.Descriptor instead.
|
|
func (*UserGrantSearchRequest) Descriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{26}
|
|
}
|
|
|
|
func (x *UserGrantSearchRequest) GetOffset() uint64 {
|
|
if x != nil {
|
|
return x.Offset
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *UserGrantSearchRequest) GetLimit() uint64 {
|
|
if x != nil {
|
|
return x.Limit
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *UserGrantSearchRequest) GetSortingColumn() UserGrantSearchKey {
|
|
if x != nil {
|
|
return x.SortingColumn
|
|
}
|
|
return UserGrantSearchKey_UserGrantSearchKey_UNKNOWN
|
|
}
|
|
|
|
func (x *UserGrantSearchRequest) GetAsc() bool {
|
|
if x != nil {
|
|
return x.Asc
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *UserGrantSearchRequest) GetQueries() []*UserGrantSearchQuery {
|
|
if x != nil {
|
|
return x.Queries
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type UserGrantSearchQuery struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Key UserGrantSearchKey `protobuf:"varint,1,opt,name=key,proto3,enum=caos.zitadel.auth.api.v1.UserGrantSearchKey" json:"key,omitempty"`
|
|
Method SearchMethod `protobuf:"varint,2,opt,name=method,proto3,enum=caos.zitadel.auth.api.v1.SearchMethod" json:"method,omitempty"`
|
|
Value string `protobuf:"bytes,3,opt,name=value,proto3" json:"value,omitempty"`
|
|
}
|
|
|
|
func (x *UserGrantSearchQuery) Reset() {
|
|
*x = UserGrantSearchQuery{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_proto_msgTypes[27]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *UserGrantSearchQuery) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*UserGrantSearchQuery) ProtoMessage() {}
|
|
|
|
func (x *UserGrantSearchQuery) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_proto_msgTypes[27]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use UserGrantSearchQuery.ProtoReflect.Descriptor instead.
|
|
func (*UserGrantSearchQuery) Descriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{27}
|
|
}
|
|
|
|
func (x *UserGrantSearchQuery) GetKey() UserGrantSearchKey {
|
|
if x != nil {
|
|
return x.Key
|
|
}
|
|
return UserGrantSearchKey_UserGrantSearchKey_UNKNOWN
|
|
}
|
|
|
|
func (x *UserGrantSearchQuery) GetMethod() SearchMethod {
|
|
if x != nil {
|
|
return x.Method
|
|
}
|
|
return SearchMethod_SEARCHMETHOD_EQUALS
|
|
}
|
|
|
|
func (x *UserGrantSearchQuery) GetValue() string {
|
|
if x != nil {
|
|
return x.Value
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type UserGrantSearchResponse struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Offset uint64 `protobuf:"varint,1,opt,name=offset,proto3" json:"offset,omitempty"`
|
|
Limit uint64 `protobuf:"varint,2,opt,name=limit,proto3" json:"limit,omitempty"`
|
|
TotalResult uint64 `protobuf:"varint,3,opt,name=total_result,json=totalResult,proto3" json:"total_result,omitempty"`
|
|
Result []*UserGrantView `protobuf:"bytes,4,rep,name=result,proto3" json:"result,omitempty"`
|
|
ProcessedSequence uint64 `protobuf:"varint,5,opt,name=processed_sequence,json=processedSequence,proto3" json:"processed_sequence,omitempty"`
|
|
ViewTimestamp *timestamp.Timestamp `protobuf:"bytes,6,opt,name=view_timestamp,json=viewTimestamp,proto3" json:"view_timestamp,omitempty"`
|
|
}
|
|
|
|
func (x *UserGrantSearchResponse) Reset() {
|
|
*x = UserGrantSearchResponse{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_proto_msgTypes[28]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *UserGrantSearchResponse) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*UserGrantSearchResponse) ProtoMessage() {}
|
|
|
|
func (x *UserGrantSearchResponse) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_proto_msgTypes[28]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use UserGrantSearchResponse.ProtoReflect.Descriptor instead.
|
|
func (*UserGrantSearchResponse) Descriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{28}
|
|
}
|
|
|
|
func (x *UserGrantSearchResponse) GetOffset() uint64 {
|
|
if x != nil {
|
|
return x.Offset
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *UserGrantSearchResponse) GetLimit() uint64 {
|
|
if x != nil {
|
|
return x.Limit
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *UserGrantSearchResponse) GetTotalResult() uint64 {
|
|
if x != nil {
|
|
return x.TotalResult
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *UserGrantSearchResponse) GetResult() []*UserGrantView {
|
|
if x != nil {
|
|
return x.Result
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *UserGrantSearchResponse) GetProcessedSequence() uint64 {
|
|
if x != nil {
|
|
return x.ProcessedSequence
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *UserGrantSearchResponse) GetViewTimestamp() *timestamp.Timestamp {
|
|
if x != nil {
|
|
return x.ViewTimestamp
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type UserGrantView struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
OrgId string `protobuf:"bytes,1,opt,name=OrgId,proto3" json:"OrgId,omitempty"`
|
|
ProjectId string `protobuf:"bytes,2,opt,name=ProjectId,proto3" json:"ProjectId,omitempty"`
|
|
UserId string `protobuf:"bytes,3,opt,name=UserId,proto3" json:"UserId,omitempty"`
|
|
Roles []string `protobuf:"bytes,4,rep,name=Roles,proto3" json:"Roles,omitempty"`
|
|
OrgName string `protobuf:"bytes,5,opt,name=OrgName,proto3" json:"OrgName,omitempty"`
|
|
GrantId string `protobuf:"bytes,6,opt,name=GrantId,proto3" json:"GrantId,omitempty"`
|
|
}
|
|
|
|
func (x *UserGrantView) Reset() {
|
|
*x = UserGrantView{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_proto_msgTypes[29]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *UserGrantView) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*UserGrantView) ProtoMessage() {}
|
|
|
|
func (x *UserGrantView) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_proto_msgTypes[29]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use UserGrantView.ProtoReflect.Descriptor instead.
|
|
func (*UserGrantView) Descriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{29}
|
|
}
|
|
|
|
func (x *UserGrantView) GetOrgId() string {
|
|
if x != nil {
|
|
return x.OrgId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UserGrantView) GetProjectId() string {
|
|
if x != nil {
|
|
return x.ProjectId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UserGrantView) GetUserId() string {
|
|
if x != nil {
|
|
return x.UserId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UserGrantView) GetRoles() []string {
|
|
if x != nil {
|
|
return x.Roles
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *UserGrantView) GetOrgName() string {
|
|
if x != nil {
|
|
return x.OrgName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UserGrantView) GetGrantId() string {
|
|
if x != nil {
|
|
return x.GrantId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type MyProjectOrgSearchRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Offset uint64 `protobuf:"varint,1,opt,name=offset,proto3" json:"offset,omitempty"`
|
|
Limit uint64 `protobuf:"varint,2,opt,name=limit,proto3" json:"limit,omitempty"`
|
|
Asc bool `protobuf:"varint,4,opt,name=asc,proto3" json:"asc,omitempty"`
|
|
Queries []*MyProjectOrgSearchQuery `protobuf:"bytes,5,rep,name=queries,proto3" json:"queries,omitempty"`
|
|
}
|
|
|
|
func (x *MyProjectOrgSearchRequest) Reset() {
|
|
*x = MyProjectOrgSearchRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_proto_msgTypes[30]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *MyProjectOrgSearchRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*MyProjectOrgSearchRequest) ProtoMessage() {}
|
|
|
|
func (x *MyProjectOrgSearchRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_proto_msgTypes[30]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use MyProjectOrgSearchRequest.ProtoReflect.Descriptor instead.
|
|
func (*MyProjectOrgSearchRequest) Descriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{30}
|
|
}
|
|
|
|
func (x *MyProjectOrgSearchRequest) GetOffset() uint64 {
|
|
if x != nil {
|
|
return x.Offset
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *MyProjectOrgSearchRequest) GetLimit() uint64 {
|
|
if x != nil {
|
|
return x.Limit
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *MyProjectOrgSearchRequest) GetAsc() bool {
|
|
if x != nil {
|
|
return x.Asc
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *MyProjectOrgSearchRequest) GetQueries() []*MyProjectOrgSearchQuery {
|
|
if x != nil {
|
|
return x.Queries
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type MyProjectOrgSearchQuery struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Key MyProjectOrgSearchKey `protobuf:"varint,1,opt,name=key,proto3,enum=caos.zitadel.auth.api.v1.MyProjectOrgSearchKey" json:"key,omitempty"`
|
|
Method SearchMethod `protobuf:"varint,2,opt,name=method,proto3,enum=caos.zitadel.auth.api.v1.SearchMethod" json:"method,omitempty"`
|
|
Value string `protobuf:"bytes,3,opt,name=value,proto3" json:"value,omitempty"`
|
|
}
|
|
|
|
func (x *MyProjectOrgSearchQuery) Reset() {
|
|
*x = MyProjectOrgSearchQuery{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_proto_msgTypes[31]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *MyProjectOrgSearchQuery) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*MyProjectOrgSearchQuery) ProtoMessage() {}
|
|
|
|
func (x *MyProjectOrgSearchQuery) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_proto_msgTypes[31]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use MyProjectOrgSearchQuery.ProtoReflect.Descriptor instead.
|
|
func (*MyProjectOrgSearchQuery) Descriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{31}
|
|
}
|
|
|
|
func (x *MyProjectOrgSearchQuery) GetKey() MyProjectOrgSearchKey {
|
|
if x != nil {
|
|
return x.Key
|
|
}
|
|
return MyProjectOrgSearchKey_MYPROJECTORGSEARCHKEY_UNSPECIFIED
|
|
}
|
|
|
|
func (x *MyProjectOrgSearchQuery) GetMethod() SearchMethod {
|
|
if x != nil {
|
|
return x.Method
|
|
}
|
|
return SearchMethod_SEARCHMETHOD_EQUALS
|
|
}
|
|
|
|
func (x *MyProjectOrgSearchQuery) GetValue() string {
|
|
if x != nil {
|
|
return x.Value
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type MyProjectOrgSearchResponse struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Offset uint64 `protobuf:"varint,1,opt,name=offset,proto3" json:"offset,omitempty"`
|
|
Limit uint64 `protobuf:"varint,2,opt,name=limit,proto3" json:"limit,omitempty"`
|
|
TotalResult uint64 `protobuf:"varint,3,opt,name=total_result,json=totalResult,proto3" json:"total_result,omitempty"`
|
|
Result []*Org `protobuf:"bytes,4,rep,name=result,proto3" json:"result,omitempty"`
|
|
}
|
|
|
|
func (x *MyProjectOrgSearchResponse) Reset() {
|
|
*x = MyProjectOrgSearchResponse{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_proto_msgTypes[32]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *MyProjectOrgSearchResponse) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*MyProjectOrgSearchResponse) ProtoMessage() {}
|
|
|
|
func (x *MyProjectOrgSearchResponse) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_proto_msgTypes[32]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use MyProjectOrgSearchResponse.ProtoReflect.Descriptor instead.
|
|
func (*MyProjectOrgSearchResponse) Descriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{32}
|
|
}
|
|
|
|
func (x *MyProjectOrgSearchResponse) GetOffset() uint64 {
|
|
if x != nil {
|
|
return x.Offset
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *MyProjectOrgSearchResponse) GetLimit() uint64 {
|
|
if x != nil {
|
|
return x.Limit
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *MyProjectOrgSearchResponse) GetTotalResult() uint64 {
|
|
if x != nil {
|
|
return x.TotalResult
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *MyProjectOrgSearchResponse) GetResult() []*Org {
|
|
if x != nil {
|
|
return x.Result
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type Org struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Id string `protobuf:"bytes,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
Name string `protobuf:"bytes,2,opt,name=name,proto3" json:"name,omitempty"`
|
|
}
|
|
|
|
func (x *Org) Reset() {
|
|
*x = Org{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_proto_msgTypes[33]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *Org) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*Org) ProtoMessage() {}
|
|
|
|
func (x *Org) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_proto_msgTypes[33]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use Org.ProtoReflect.Descriptor instead.
|
|
func (*Org) Descriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{33}
|
|
}
|
|
|
|
func (x *Org) GetId() string {
|
|
if x != nil {
|
|
return x.Id
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *Org) GetName() string {
|
|
if x != nil {
|
|
return x.Name
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type MyPermissions struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Permissions []string `protobuf:"bytes,1,rep,name=permissions,proto3" json:"permissions,omitempty"`
|
|
}
|
|
|
|
func (x *MyPermissions) Reset() {
|
|
*x = MyPermissions{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_proto_msgTypes[34]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *MyPermissions) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*MyPermissions) ProtoMessage() {}
|
|
|
|
func (x *MyPermissions) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_proto_msgTypes[34]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use MyPermissions.ProtoReflect.Descriptor instead.
|
|
func (*MyPermissions) Descriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{34}
|
|
}
|
|
|
|
func (x *MyPermissions) GetPermissions() []string {
|
|
if x != nil {
|
|
return x.Permissions
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type ChangesRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Limit uint64 `protobuf:"varint,1,opt,name=limit,proto3" json:"limit,omitempty"`
|
|
SequenceOffset uint64 `protobuf:"varint,2,opt,name=sequence_offset,json=sequenceOffset,proto3" json:"sequence_offset,omitempty"`
|
|
Asc bool `protobuf:"varint,3,opt,name=asc,proto3" json:"asc,omitempty"`
|
|
}
|
|
|
|
func (x *ChangesRequest) Reset() {
|
|
*x = ChangesRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_proto_msgTypes[35]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *ChangesRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*ChangesRequest) ProtoMessage() {}
|
|
|
|
func (x *ChangesRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_proto_msgTypes[35]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use ChangesRequest.ProtoReflect.Descriptor instead.
|
|
func (*ChangesRequest) Descriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{35}
|
|
}
|
|
|
|
func (x *ChangesRequest) GetLimit() uint64 {
|
|
if x != nil {
|
|
return x.Limit
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *ChangesRequest) GetSequenceOffset() uint64 {
|
|
if x != nil {
|
|
return x.SequenceOffset
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *ChangesRequest) GetAsc() bool {
|
|
if x != nil {
|
|
return x.Asc
|
|
}
|
|
return false
|
|
}
|
|
|
|
type Changes struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Changes []*Change `protobuf:"bytes,1,rep,name=changes,proto3" json:"changes,omitempty"`
|
|
Offset uint64 `protobuf:"varint,2,opt,name=offset,proto3" json:"offset,omitempty"`
|
|
Limit uint64 `protobuf:"varint,3,opt,name=limit,proto3" json:"limit,omitempty"`
|
|
}
|
|
|
|
func (x *Changes) Reset() {
|
|
*x = Changes{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_proto_msgTypes[36]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *Changes) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*Changes) ProtoMessage() {}
|
|
|
|
func (x *Changes) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_proto_msgTypes[36]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use Changes.ProtoReflect.Descriptor instead.
|
|
func (*Changes) Descriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{36}
|
|
}
|
|
|
|
func (x *Changes) GetChanges() []*Change {
|
|
if x != nil {
|
|
return x.Changes
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *Changes) GetOffset() uint64 {
|
|
if x != nil {
|
|
return x.Offset
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *Changes) GetLimit() uint64 {
|
|
if x != nil {
|
|
return x.Limit
|
|
}
|
|
return 0
|
|
}
|
|
|
|
type Change struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
ChangeDate *timestamp.Timestamp `protobuf:"bytes,1,opt,name=change_date,json=changeDate,proto3" json:"change_date,omitempty"`
|
|
EventType *message.LocalizedMessage `protobuf:"bytes,2,opt,name=event_type,json=eventType,proto3" json:"event_type,omitempty"`
|
|
Sequence uint64 `protobuf:"varint,3,opt,name=sequence,proto3" json:"sequence,omitempty"`
|
|
EditorId string `protobuf:"bytes,4,opt,name=editor_id,json=editorId,proto3" json:"editor_id,omitempty"`
|
|
Editor string `protobuf:"bytes,5,opt,name=editor,proto3" json:"editor,omitempty"`
|
|
Data *_struct.Struct `protobuf:"bytes,6,opt,name=data,proto3" json:"data,omitempty"`
|
|
}
|
|
|
|
func (x *Change) Reset() {
|
|
*x = Change{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_proto_msgTypes[37]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *Change) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*Change) ProtoMessage() {}
|
|
|
|
func (x *Change) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_proto_msgTypes[37]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use Change.ProtoReflect.Descriptor instead.
|
|
func (*Change) Descriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{37}
|
|
}
|
|
|
|
func (x *Change) GetChangeDate() *timestamp.Timestamp {
|
|
if x != nil {
|
|
return x.ChangeDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *Change) GetEventType() *message.LocalizedMessage {
|
|
if x != nil {
|
|
return x.EventType
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *Change) GetSequence() uint64 {
|
|
if x != nil {
|
|
return x.Sequence
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *Change) GetEditorId() string {
|
|
if x != nil {
|
|
return x.EditorId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *Change) GetEditor() string {
|
|
if x != nil {
|
|
return x.Editor
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *Change) GetData() *_struct.Struct {
|
|
if x != nil {
|
|
return x.Data
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type PasswordComplexityPolicy struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Id string `protobuf:"bytes,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
Description string `protobuf:"bytes,2,opt,name=description,proto3" json:"description,omitempty"`
|
|
CreationDate *timestamp.Timestamp `protobuf:"bytes,3,opt,name=creation_date,json=creationDate,proto3" json:"creation_date,omitempty"`
|
|
ChangeDate *timestamp.Timestamp `protobuf:"bytes,4,opt,name=change_date,json=changeDate,proto3" json:"change_date,omitempty"`
|
|
MinLength uint64 `protobuf:"varint,5,opt,name=min_length,json=minLength,proto3" json:"min_length,omitempty"`
|
|
HasLowercase bool `protobuf:"varint,6,opt,name=has_lowercase,json=hasLowercase,proto3" json:"has_lowercase,omitempty"`
|
|
HasUppercase bool `protobuf:"varint,7,opt,name=has_uppercase,json=hasUppercase,proto3" json:"has_uppercase,omitempty"`
|
|
HasNumber bool `protobuf:"varint,8,opt,name=has_number,json=hasNumber,proto3" json:"has_number,omitempty"`
|
|
HasSymbol bool `protobuf:"varint,9,opt,name=has_symbol,json=hasSymbol,proto3" json:"has_symbol,omitempty"`
|
|
Sequence uint64 `protobuf:"varint,10,opt,name=sequence,proto3" json:"sequence,omitempty"`
|
|
IsDefault bool `protobuf:"varint,11,opt,name=is_default,json=isDefault,proto3" json:"is_default,omitempty"`
|
|
}
|
|
|
|
func (x *PasswordComplexityPolicy) Reset() {
|
|
*x = PasswordComplexityPolicy{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_proto_msgTypes[38]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *PasswordComplexityPolicy) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*PasswordComplexityPolicy) ProtoMessage() {}
|
|
|
|
func (x *PasswordComplexityPolicy) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_proto_msgTypes[38]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use PasswordComplexityPolicy.ProtoReflect.Descriptor instead.
|
|
func (*PasswordComplexityPolicy) Descriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{38}
|
|
}
|
|
|
|
func (x *PasswordComplexityPolicy) GetId() string {
|
|
if x != nil {
|
|
return x.Id
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *PasswordComplexityPolicy) GetDescription() string {
|
|
if x != nil {
|
|
return x.Description
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *PasswordComplexityPolicy) GetCreationDate() *timestamp.Timestamp {
|
|
if x != nil {
|
|
return x.CreationDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *PasswordComplexityPolicy) GetChangeDate() *timestamp.Timestamp {
|
|
if x != nil {
|
|
return x.ChangeDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *PasswordComplexityPolicy) GetMinLength() uint64 {
|
|
if x != nil {
|
|
return x.MinLength
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *PasswordComplexityPolicy) GetHasLowercase() bool {
|
|
if x != nil {
|
|
return x.HasLowercase
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *PasswordComplexityPolicy) GetHasUppercase() bool {
|
|
if x != nil {
|
|
return x.HasUppercase
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *PasswordComplexityPolicy) GetHasNumber() bool {
|
|
if x != nil {
|
|
return x.HasNumber
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *PasswordComplexityPolicy) GetHasSymbol() bool {
|
|
if x != nil {
|
|
return x.HasSymbol
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *PasswordComplexityPolicy) GetSequence() uint64 {
|
|
if x != nil {
|
|
return x.Sequence
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *PasswordComplexityPolicy) GetIsDefault() bool {
|
|
if x != nil {
|
|
return x.IsDefault
|
|
}
|
|
return false
|
|
}
|
|
|
|
type ExternalIDPResponse struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
IdpConfigId string `protobuf:"bytes,1,opt,name=idp_config_id,json=idpConfigId,proto3" json:"idp_config_id,omitempty"`
|
|
UserId string `protobuf:"bytes,2,opt,name=user_id,json=userId,proto3" json:"user_id,omitempty"`
|
|
DisplayName string `protobuf:"bytes,3,opt,name=display_name,json=displayName,proto3" json:"display_name,omitempty"`
|
|
}
|
|
|
|
func (x *ExternalIDPResponse) Reset() {
|
|
*x = ExternalIDPResponse{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_proto_msgTypes[39]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *ExternalIDPResponse) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*ExternalIDPResponse) ProtoMessage() {}
|
|
|
|
func (x *ExternalIDPResponse) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_proto_msgTypes[39]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use ExternalIDPResponse.ProtoReflect.Descriptor instead.
|
|
func (*ExternalIDPResponse) Descriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{39}
|
|
}
|
|
|
|
func (x *ExternalIDPResponse) GetIdpConfigId() string {
|
|
if x != nil {
|
|
return x.IdpConfigId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *ExternalIDPResponse) GetUserId() string {
|
|
if x != nil {
|
|
return x.UserId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *ExternalIDPResponse) GetDisplayName() string {
|
|
if x != nil {
|
|
return x.DisplayName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type ExternalIDPRemoveRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
IdpConfigId string `protobuf:"bytes,1,opt,name=idp_config_id,json=idpConfigId,proto3" json:"idp_config_id,omitempty"`
|
|
ExternalUserId string `protobuf:"bytes,2,opt,name=external_user_id,json=externalUserId,proto3" json:"external_user_id,omitempty"`
|
|
}
|
|
|
|
func (x *ExternalIDPRemoveRequest) Reset() {
|
|
*x = ExternalIDPRemoveRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_proto_msgTypes[40]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *ExternalIDPRemoveRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*ExternalIDPRemoveRequest) ProtoMessage() {}
|
|
|
|
func (x *ExternalIDPRemoveRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_proto_msgTypes[40]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use ExternalIDPRemoveRequest.ProtoReflect.Descriptor instead.
|
|
func (*ExternalIDPRemoveRequest) Descriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{40}
|
|
}
|
|
|
|
func (x *ExternalIDPRemoveRequest) GetIdpConfigId() string {
|
|
if x != nil {
|
|
return x.IdpConfigId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *ExternalIDPRemoveRequest) GetExternalUserId() string {
|
|
if x != nil {
|
|
return x.ExternalUserId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type ExternalIDPSearchRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Offset uint64 `protobuf:"varint,1,opt,name=offset,proto3" json:"offset,omitempty"`
|
|
Limit uint64 `protobuf:"varint,2,opt,name=limit,proto3" json:"limit,omitempty"`
|
|
}
|
|
|
|
func (x *ExternalIDPSearchRequest) Reset() {
|
|
*x = ExternalIDPSearchRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_proto_msgTypes[41]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *ExternalIDPSearchRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*ExternalIDPSearchRequest) ProtoMessage() {}
|
|
|
|
func (x *ExternalIDPSearchRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_proto_msgTypes[41]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use ExternalIDPSearchRequest.ProtoReflect.Descriptor instead.
|
|
func (*ExternalIDPSearchRequest) Descriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{41}
|
|
}
|
|
|
|
func (x *ExternalIDPSearchRequest) GetOffset() uint64 {
|
|
if x != nil {
|
|
return x.Offset
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *ExternalIDPSearchRequest) GetLimit() uint64 {
|
|
if x != nil {
|
|
return x.Limit
|
|
}
|
|
return 0
|
|
}
|
|
|
|
type ExternalIDPSearchResponse struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Offset uint64 `protobuf:"varint,1,opt,name=offset,proto3" json:"offset,omitempty"`
|
|
Limit uint64 `protobuf:"varint,2,opt,name=limit,proto3" json:"limit,omitempty"`
|
|
TotalResult uint64 `protobuf:"varint,3,opt,name=total_result,json=totalResult,proto3" json:"total_result,omitempty"`
|
|
Result []*ExternalIDPView `protobuf:"bytes,4,rep,name=result,proto3" json:"result,omitempty"`
|
|
ProcessedSequence uint64 `protobuf:"varint,5,opt,name=processed_sequence,json=processedSequence,proto3" json:"processed_sequence,omitempty"`
|
|
ViewTimestamp *timestamp.Timestamp `protobuf:"bytes,6,opt,name=view_timestamp,json=viewTimestamp,proto3" json:"view_timestamp,omitempty"`
|
|
}
|
|
|
|
func (x *ExternalIDPSearchResponse) Reset() {
|
|
*x = ExternalIDPSearchResponse{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_proto_msgTypes[42]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *ExternalIDPSearchResponse) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*ExternalIDPSearchResponse) ProtoMessage() {}
|
|
|
|
func (x *ExternalIDPSearchResponse) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_proto_msgTypes[42]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use ExternalIDPSearchResponse.ProtoReflect.Descriptor instead.
|
|
func (*ExternalIDPSearchResponse) Descriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{42}
|
|
}
|
|
|
|
func (x *ExternalIDPSearchResponse) GetOffset() uint64 {
|
|
if x != nil {
|
|
return x.Offset
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *ExternalIDPSearchResponse) GetLimit() uint64 {
|
|
if x != nil {
|
|
return x.Limit
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *ExternalIDPSearchResponse) GetTotalResult() uint64 {
|
|
if x != nil {
|
|
return x.TotalResult
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *ExternalIDPSearchResponse) GetResult() []*ExternalIDPView {
|
|
if x != nil {
|
|
return x.Result
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *ExternalIDPSearchResponse) GetProcessedSequence() uint64 {
|
|
if x != nil {
|
|
return x.ProcessedSequence
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *ExternalIDPSearchResponse) GetViewTimestamp() *timestamp.Timestamp {
|
|
if x != nil {
|
|
return x.ViewTimestamp
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type ExternalIDPView struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
UserId string `protobuf:"bytes,1,opt,name=user_id,json=userId,proto3" json:"user_id,omitempty"`
|
|
IdpConfigId string `protobuf:"bytes,2,opt,name=idp_config_id,json=idpConfigId,proto3" json:"idp_config_id,omitempty"`
|
|
ExternalUserId string `protobuf:"bytes,3,opt,name=external_user_id,json=externalUserId,proto3" json:"external_user_id,omitempty"`
|
|
IdpName string `protobuf:"bytes,4,opt,name=idp_name,json=idpName,proto3" json:"idp_name,omitempty"`
|
|
ExternalUserDisplayName string `protobuf:"bytes,5,opt,name=external_user_display_name,json=externalUserDisplayName,proto3" json:"external_user_display_name,omitempty"`
|
|
CreationDate *timestamp.Timestamp `protobuf:"bytes,6,opt,name=creation_date,json=creationDate,proto3" json:"creation_date,omitempty"`
|
|
ChangeDate *timestamp.Timestamp `protobuf:"bytes,7,opt,name=change_date,json=changeDate,proto3" json:"change_date,omitempty"`
|
|
}
|
|
|
|
func (x *ExternalIDPView) Reset() {
|
|
*x = ExternalIDPView{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_proto_msgTypes[43]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *ExternalIDPView) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*ExternalIDPView) ProtoMessage() {}
|
|
|
|
func (x *ExternalIDPView) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_proto_msgTypes[43]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use ExternalIDPView.ProtoReflect.Descriptor instead.
|
|
func (*ExternalIDPView) Descriptor() ([]byte, []int) {
|
|
return file_auth_proto_rawDescGZIP(), []int{43}
|
|
}
|
|
|
|
func (x *ExternalIDPView) GetUserId() string {
|
|
if x != nil {
|
|
return x.UserId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *ExternalIDPView) GetIdpConfigId() string {
|
|
if x != nil {
|
|
return x.IdpConfigId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *ExternalIDPView) GetExternalUserId() string {
|
|
if x != nil {
|
|
return x.ExternalUserId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *ExternalIDPView) GetIdpName() string {
|
|
if x != nil {
|
|
return x.IdpName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *ExternalIDPView) GetExternalUserDisplayName() string {
|
|
if x != nil {
|
|
return x.ExternalUserDisplayName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *ExternalIDPView) GetCreationDate() *timestamp.Timestamp {
|
|
if x != nil {
|
|
return x.CreationDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *ExternalIDPView) GetChangeDate() *timestamp.Timestamp {
|
|
if x != nil {
|
|
return x.ChangeDate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
var File_auth_proto protoreflect.FileDescriptor
|
|
|
|
var file_auth_proto_rawDesc = []byte{
|
|
0x0a, 0x0a, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x12, 0x18, 0x63, 0x61,
|
|
0x6f, 0x73, 0x2e, 0x7a, 0x69, 0x74, 0x61, 0x64, 0x65, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e,
|
|
0x61, 0x70, 0x69, 0x2e, 0x76, 0x31, 0x1a, 0x1c, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2f, 0x61,
|
|
0x70, 0x69, 0x2f, 0x61, 0x6e, 0x6e, 0x6f, 0x74, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x2e, 0x70,
|
|
0x72, 0x6f, 0x74, 0x6f, 0x1a, 0x1b, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2f, 0x70, 0x72, 0x6f,
|
|
0x74, 0x6f, 0x62, 0x75, 0x66, 0x2f, 0x65, 0x6d, 0x70, 0x74, 0x79, 0x2e, 0x70, 0x72, 0x6f, 0x74,
|
|
0x6f, 0x1a, 0x1c, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2f, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62,
|
|
0x75, 0x66, 0x2f, 0x73, 0x74, 0x72, 0x75, 0x63, 0x74, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x1a,
|
|
0x1f, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2f, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66,
|
|
0x2f, 0x74, 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d, 0x70, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f,
|
|
0x1a, 0x17, 0x76, 0x61, 0x6c, 0x69, 0x64, 0x61, 0x74, 0x65, 0x2f, 0x76, 0x61, 0x6c, 0x69, 0x64,
|
|
0x61, 0x74, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x1a, 0x2c, 0x70, 0x72, 0x6f, 0x74, 0x6f,
|
|
0x63, 0x2d, 0x67, 0x65, 0x6e, 0x2d, 0x73, 0x77, 0x61, 0x67, 0x67, 0x65, 0x72, 0x2f, 0x6f, 0x70,
|
|
0x74, 0x69, 0x6f, 0x6e, 0x73, 0x2f, 0x61, 0x6e, 0x6e, 0x6f, 0x74, 0x61, 0x74, 0x69, 0x6f, 0x6e,
|
|
0x73, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x1a, 0x18, 0x61, 0x75, 0x74, 0x68, 0x6f, 0x70, 0x74,
|
|
0x69, 0x6f, 0x6e, 0x2f, 0x6f, 0x70, 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x2e, 0x70, 0x72, 0x6f, 0x74,
|
|
0x6f, 0x1a, 0x13, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x2f, 0x6d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65,
|
|
0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x22, 0x62, 0x0a, 0x10, 0x55, 0x73, 0x65, 0x72, 0x53, 0x65,
|
|
0x73, 0x73, 0x69, 0x6f, 0x6e, 0x56, 0x69, 0x65, 0x77, 0x73, 0x12, 0x4e, 0x0a, 0x0d, 0x75, 0x73,
|
|
0x65, 0x72, 0x5f, 0x73, 0x65, 0x73, 0x73, 0x69, 0x6f, 0x6e, 0x73, 0x18, 0x01, 0x20, 0x03, 0x28,
|
|
0x0b, 0x32, 0x29, 0x2e, 0x63, 0x61, 0x6f, 0x73, 0x2e, 0x7a, 0x69, 0x74, 0x61, 0x64, 0x65, 0x6c,
|
|
0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x61, 0x70, 0x69, 0x2e, 0x76, 0x31, 0x2e, 0x55, 0x73, 0x65,
|
|
0x72, 0x53, 0x65, 0x73, 0x73, 0x69, 0x6f, 0x6e, 0x56, 0x69, 0x65, 0x77, 0x52, 0x0c, 0x75, 0x73,
|
|
0x65, 0x72, 0x53, 0x65, 0x73, 0x73, 0x69, 0x6f, 0x6e, 0x73, 0x22, 0x9b, 0x02, 0x0a, 0x0f, 0x55,
|
|
0x73, 0x65, 0x72, 0x53, 0x65, 0x73, 0x73, 0x69, 0x6f, 0x6e, 0x56, 0x69, 0x65, 0x77, 0x12, 0x0e,
|
|
0x0a, 0x02, 0x69, 0x64, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x02, 0x69, 0x64, 0x12, 0x19,
|
|
0x0a, 0x08, 0x61, 0x67, 0x65, 0x6e, 0x74, 0x5f, 0x69, 0x64, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09,
|
|
0x52, 0x07, 0x61, 0x67, 0x65, 0x6e, 0x74, 0x49, 0x64, 0x12, 0x49, 0x0a, 0x0a, 0x61, 0x75, 0x74,
|
|
0x68, 0x5f, 0x73, 0x74, 0x61, 0x74, 0x65, 0x18, 0x03, 0x20, 0x01, 0x28, 0x0e, 0x32, 0x2a, 0x2e,
|
|
0x63, 0x61, 0x6f, 0x73, 0x2e, 0x7a, 0x69, 0x74, 0x61, 0x64, 0x65, 0x6c, 0x2e, 0x61, 0x75, 0x74,
|
|
0x68, 0x2e, 0x61, 0x70, 0x69, 0x2e, 0x76, 0x31, 0x2e, 0x55, 0x73, 0x65, 0x72, 0x53, 0x65, 0x73,
|
|
0x73, 0x69, 0x6f, 0x6e, 0x53, 0x74, 0x61, 0x74, 0x65, 0x52, 0x09, 0x61, 0x75, 0x74, 0x68, 0x53,
|
|
0x74, 0x61, 0x74, 0x65, 0x12, 0x17, 0x0a, 0x07, 0x75, 0x73, 0x65, 0x72, 0x5f, 0x69, 0x64, 0x18,
|
|
0x04, 0x20, 0x01, 0x28, 0x09, 0x52, 0x06, 0x75, 0x73, 0x65, 0x72, 0x49, 0x64, 0x12, 0x1b, 0x0a,
|
|
0x09, 0x75, 0x73, 0x65, 0x72, 0x5f, 0x6e, 0x61, 0x6d, 0x65, 0x18, 0x05, 0x20, 0x01, 0x28, 0x09,
|
|
0x52, 0x08, 0x75, 0x73, 0x65, 0x72, 0x4e, 0x61, 0x6d, 0x65, 0x12, 0x1a, 0x0a, 0x08, 0x73, 0x65,
|
|
0x71, 0x75, 0x65, 0x6e, 0x63, 0x65, 0x18, 0x06, 0x20, 0x01, 0x28, 0x04, 0x52, 0x08, 0x73, 0x65,
|
|
0x71, 0x75, 0x65, 0x6e, 0x63, 0x65, 0x12, 0x1d, 0x0a, 0x0a, 0x6c, 0x6f, 0x67, 0x69, 0x6e, 0x5f,
|
|
0x6e, 0x61, 0x6d, 0x65, 0x18, 0x07, 0x20, 0x01, 0x28, 0x09, 0x52, 0x09, 0x6c, 0x6f, 0x67, 0x69,
|
|
0x6e, 0x4e, 0x61, 0x6d, 0x65, 0x12, 0x21, 0x0a, 0x0c, 0x64, 0x69, 0x73, 0x70, 0x6c, 0x61, 0x79,
|
|
0x5f, 0x6e, 0x61, 0x6d, 0x65, 0x18, 0x08, 0x20, 0x01, 0x28, 0x09, 0x52, 0x0b, 0x64, 0x69, 0x73,
|
|
0x70, 0x6c, 0x61, 0x79, 0x4e, 0x61, 0x6d, 0x65, 0x22, 0xce, 0x04, 0x0a, 0x08, 0x55, 0x73, 0x65,
|
|
0x72, 0x56, 0x69, 0x65, 0x77, 0x12, 0x0e, 0x0a, 0x02, 0x69, 0x64, 0x18, 0x01, 0x20, 0x01, 0x28,
|
|
0x09, 0x52, 0x02, 0x69, 0x64, 0x12, 0x39, 0x0a, 0x05, 0x73, 0x74, 0x61, 0x74, 0x65, 0x18, 0x02,
|
|
0x20, 0x01, 0x28, 0x0e, 0x32, 0x23, 0x2e, 0x63, 0x61, 0x6f, 0x73, 0x2e, 0x7a, 0x69, 0x74, 0x61,
|
|
0x64, 0x65, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x61, 0x70, 0x69, 0x2e, 0x76, 0x31, 0x2e,
|
|
0x55, 0x73, 0x65, 0x72, 0x53, 0x74, 0x61, 0x74, 0x65, 0x52, 0x05, 0x73, 0x74, 0x61, 0x74, 0x65,
|
|
0x12, 0x3f, 0x0a, 0x0d, 0x63, 0x72, 0x65, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x5f, 0x64, 0x61, 0x74,
|
|
0x65, 0x18, 0x03, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1a, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65,
|
|
0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e, 0x54, 0x69, 0x6d, 0x65, 0x73, 0x74,
|
|
0x61, 0x6d, 0x70, 0x52, 0x0c, 0x63, 0x72, 0x65, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x44, 0x61, 0x74,
|
|
0x65, 0x12, 0x3b, 0x0a, 0x0b, 0x63, 0x68, 0x61, 0x6e, 0x67, 0x65, 0x5f, 0x64, 0x61, 0x74, 0x65,
|
|
0x18, 0x04, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1a, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e,
|
|
0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e, 0x54, 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61,
|
|
0x6d, 0x70, 0x52, 0x0a, 0x63, 0x68, 0x61, 0x6e, 0x67, 0x65, 0x44, 0x61, 0x74, 0x65, 0x12, 0x1a,
|
|
0x0a, 0x08, 0x73, 0x65, 0x71, 0x75, 0x65, 0x6e, 0x63, 0x65, 0x18, 0x05, 0x20, 0x01, 0x28, 0x04,
|
|
0x52, 0x08, 0x73, 0x65, 0x71, 0x75, 0x65, 0x6e, 0x63, 0x65, 0x12, 0x1f, 0x0a, 0x0b, 0x6c, 0x6f,
|
|
0x67, 0x69, 0x6e, 0x5f, 0x6e, 0x61, 0x6d, 0x65, 0x73, 0x18, 0x06, 0x20, 0x03, 0x28, 0x09, 0x52,
|
|
0x0a, 0x6c, 0x6f, 0x67, 0x69, 0x6e, 0x4e, 0x61, 0x6d, 0x65, 0x73, 0x12, 0x30, 0x0a, 0x14, 0x70,
|
|
0x72, 0x65, 0x66, 0x65, 0x72, 0x72, 0x65, 0x64, 0x5f, 0x6c, 0x6f, 0x67, 0x69, 0x6e, 0x5f, 0x6e,
|
|
0x61, 0x6d, 0x65, 0x18, 0x07, 0x20, 0x01, 0x28, 0x09, 0x52, 0x12, 0x70, 0x72, 0x65, 0x66, 0x65,
|
|
0x72, 0x72, 0x65, 0x64, 0x4c, 0x6f, 0x67, 0x69, 0x6e, 0x4e, 0x61, 0x6d, 0x65, 0x12, 0x39, 0x0a,
|
|
0x0a, 0x6c, 0x61, 0x73, 0x74, 0x5f, 0x6c, 0x6f, 0x67, 0x69, 0x6e, 0x18, 0x08, 0x20, 0x01, 0x28,
|
|
0x0b, 0x32, 0x1a, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f,
|
|
0x62, 0x75, 0x66, 0x2e, 0x54, 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d, 0x70, 0x52, 0x09, 0x6c,
|
|
0x61, 0x73, 0x74, 0x4c, 0x6f, 0x67, 0x69, 0x6e, 0x12, 0x25, 0x0a, 0x0e, 0x72, 0x65, 0x73, 0x6f,
|
|
0x75, 0x72, 0x63, 0x65, 0x5f, 0x6f, 0x77, 0x6e, 0x65, 0x72, 0x18, 0x09, 0x20, 0x01, 0x28, 0x09,
|
|
0x52, 0x0d, 0x72, 0x65, 0x73, 0x6f, 0x75, 0x72, 0x63, 0x65, 0x4f, 0x77, 0x6e, 0x65, 0x72, 0x12,
|
|
0x1b, 0x0a, 0x09, 0x75, 0x73, 0x65, 0x72, 0x5f, 0x6e, 0x61, 0x6d, 0x65, 0x18, 0x0a, 0x20, 0x01,
|
|
0x28, 0x09, 0x52, 0x08, 0x75, 0x73, 0x65, 0x72, 0x4e, 0x61, 0x6d, 0x65, 0x12, 0x3b, 0x0a, 0x05,
|
|
0x68, 0x75, 0x6d, 0x61, 0x6e, 0x18, 0x0b, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x23, 0x2e, 0x63, 0x61,
|
|
0x6f, 0x73, 0x2e, 0x7a, 0x69, 0x74, 0x61, 0x64, 0x65, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e,
|
|
0x61, 0x70, 0x69, 0x2e, 0x76, 0x31, 0x2e, 0x48, 0x75, 0x6d, 0x61, 0x6e, 0x56, 0x69, 0x65, 0x77,
|
|
0x48, 0x00, 0x52, 0x05, 0x68, 0x75, 0x6d, 0x61, 0x6e, 0x12, 0x41, 0x0a, 0x07, 0x6d, 0x61, 0x63,
|
|
0x68, 0x69, 0x6e, 0x65, 0x18, 0x0c, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x25, 0x2e, 0x63, 0x61, 0x6f,
|
|
0x73, 0x2e, 0x7a, 0x69, 0x74, 0x61, 0x64, 0x65, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x61,
|
|
0x70, 0x69, 0x2e, 0x76, 0x31, 0x2e, 0x4d, 0x61, 0x63, 0x68, 0x69, 0x6e, 0x65, 0x56, 0x69, 0x65,
|
|
0x77, 0x48, 0x00, 0x52, 0x07, 0x6d, 0x61, 0x63, 0x68, 0x69, 0x6e, 0x65, 0x42, 0x0b, 0x0a, 0x04,
|
|
0x75, 0x73, 0x65, 0x72, 0x12, 0x03, 0xf8, 0x42, 0x01, 0x22, 0x85, 0x01, 0x0a, 0x0b, 0x4d, 0x61,
|
|
0x63, 0x68, 0x69, 0x6e, 0x65, 0x56, 0x69, 0x65, 0x77, 0x12, 0x40, 0x0a, 0x0e, 0x6c, 0x61, 0x73,
|
|
0x74, 0x5f, 0x6b, 0x65, 0x79, 0x5f, 0x61, 0x64, 0x64, 0x65, 0x64, 0x18, 0x01, 0x20, 0x01, 0x28,
|
|
0x0b, 0x32, 0x1a, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f,
|
|
0x62, 0x75, 0x66, 0x2e, 0x54, 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d, 0x70, 0x52, 0x0c, 0x6c,
|
|
0x61, 0x73, 0x74, 0x4b, 0x65, 0x79, 0x41, 0x64, 0x64, 0x65, 0x64, 0x12, 0x12, 0x0a, 0x04, 0x6e,
|
|
0x61, 0x6d, 0x65, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52, 0x04, 0x6e, 0x61, 0x6d, 0x65, 0x12,
|
|
0x20, 0x0a, 0x0b, 0x64, 0x65, 0x73, 0x63, 0x72, 0x69, 0x70, 0x74, 0x69, 0x6f, 0x6e, 0x18, 0x03,
|
|
0x20, 0x01, 0x28, 0x09, 0x52, 0x0b, 0x64, 0x65, 0x73, 0x63, 0x72, 0x69, 0x70, 0x74, 0x69, 0x6f,
|
|
0x6e, 0x22, 0x80, 0x02, 0x0a, 0x0e, 0x4d, 0x61, 0x63, 0x68, 0x69, 0x6e, 0x65, 0x4b, 0x65, 0x79,
|
|
0x56, 0x69, 0x65, 0x77, 0x12, 0x0e, 0x0a, 0x02, 0x69, 0x64, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09,
|
|
0x52, 0x02, 0x69, 0x64, 0x12, 0x3c, 0x0a, 0x04, 0x74, 0x79, 0x70, 0x65, 0x18, 0x02, 0x20, 0x01,
|
|
0x28, 0x0e, 0x32, 0x28, 0x2e, 0x63, 0x61, 0x6f, 0x73, 0x2e, 0x7a, 0x69, 0x74, 0x61, 0x64, 0x65,
|
|
0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x61, 0x70, 0x69, 0x2e, 0x76, 0x31, 0x2e, 0x4d, 0x61,
|
|
0x63, 0x68, 0x69, 0x6e, 0x65, 0x4b, 0x65, 0x79, 0x54, 0x79, 0x70, 0x65, 0x52, 0x04, 0x74, 0x79,
|
|
0x70, 0x65, 0x12, 0x1a, 0x0a, 0x08, 0x73, 0x65, 0x71, 0x75, 0x65, 0x6e, 0x63, 0x65, 0x18, 0x03,
|
|
0x20, 0x01, 0x28, 0x04, 0x52, 0x08, 0x73, 0x65, 0x71, 0x75, 0x65, 0x6e, 0x63, 0x65, 0x12, 0x3f,
|
|
0x0a, 0x0d, 0x63, 0x72, 0x65, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x5f, 0x64, 0x61, 0x74, 0x65, 0x18,
|
|
0x04, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1a, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70,
|
|
0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e, 0x54, 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d,
|
|
0x70, 0x52, 0x0c, 0x63, 0x72, 0x65, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x44, 0x61, 0x74, 0x65, 0x12,
|
|
0x43, 0x0a, 0x0f, 0x65, 0x78, 0x70, 0x69, 0x72, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x5f, 0x64, 0x61,
|
|
0x74, 0x65, 0x18, 0x05, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1a, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c,
|
|
0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e, 0x54, 0x69, 0x6d, 0x65, 0x73,
|
|
0x74, 0x61, 0x6d, 0x70, 0x52, 0x0e, 0x65, 0x78, 0x70, 0x69, 0x72, 0x61, 0x74, 0x69, 0x6f, 0x6e,
|
|
0x44, 0x61, 0x74, 0x65, 0x22, 0xd1, 0x04, 0x0a, 0x09, 0x48, 0x75, 0x6d, 0x61, 0x6e, 0x56, 0x69,
|
|
0x65, 0x77, 0x12, 0x45, 0x0a, 0x10, 0x70, 0x61, 0x73, 0x73, 0x77, 0x6f, 0x72, 0x64, 0x5f, 0x63,
|
|
0x68, 0x61, 0x6e, 0x67, 0x65, 0x64, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1a, 0x2e, 0x67,
|
|
0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e, 0x54,
|
|
0x69, 0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d, 0x70, 0x52, 0x0f, 0x70, 0x61, 0x73, 0x73, 0x77, 0x6f,
|
|
0x72, 0x64, 0x43, 0x68, 0x61, 0x6e, 0x67, 0x65, 0x64, 0x12, 0x1d, 0x0a, 0x0a, 0x66, 0x69, 0x72,
|
|
0x73, 0x74, 0x5f, 0x6e, 0x61, 0x6d, 0x65, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52, 0x09, 0x66,
|
|
0x69, 0x72, 0x73, 0x74, 0x4e, 0x61, 0x6d, 0x65, 0x12, 0x1b, 0x0a, 0x09, 0x6c, 0x61, 0x73, 0x74,
|
|
0x5f, 0x6e, 0x61, 0x6d, 0x65, 0x18, 0x03, 0x20, 0x01, 0x28, 0x09, 0x52, 0x08, 0x6c, 0x61, 0x73,
|
|
0x74, 0x4e, 0x61, 0x6d, 0x65, 0x12, 0x21, 0x0a, 0x0c, 0x64, 0x69, 0x73, 0x70, 0x6c, 0x61, 0x79,
|
|
0x5f, 0x6e, 0x61, 0x6d, 0x65, 0x18, 0x04, 0x20, 0x01, 0x28, 0x09, 0x52, 0x0b, 0x64, 0x69, 0x73,
|
|
0x70, 0x6c, 0x61, 0x79, 0x4e, 0x61, 0x6d, 0x65, 0x12, 0x1b, 0x0a, 0x09, 0x6e, 0x69, 0x63, 0x6b,
|
|
0x5f, 0x6e, 0x61, 0x6d, 0x65, 0x18, 0x05, 0x20, 0x01, 0x28, 0x09, 0x52, 0x08, 0x6e, 0x69, 0x63,
|
|
0x6b, 0x4e, 0x61, 0x6d, 0x65, 0x12, 0x2d, 0x0a, 0x12, 0x70, 0x72, 0x65, 0x66, 0x65, 0x72, 0x72,
|
|
0x65, 0x64, 0x5f, 0x6c, 0x61, 0x6e, 0x67, 0x75, 0x61, 0x67, 0x65, 0x18, 0x06, 0x20, 0x01, 0x28,
|
|
0x09, 0x52, 0x11, 0x70, 0x72, 0x65, 0x66, 0x65, 0x72, 0x72, 0x65, 0x64, 0x4c, 0x61, 0x6e, 0x67,
|
|
0x75, 0x61, 0x67, 0x65, 0x12, 0x38, 0x0a, 0x06, 0x67, 0x65, 0x6e, 0x64, 0x65, 0x72, 0x18, 0x07,
|
|
0x20, 0x01, 0x28, 0x0e, 0x32, 0x20, 0x2e, 0x63, 0x61, 0x6f, 0x73, 0x2e, 0x7a, 0x69, 0x74, 0x61,
|
|
0x64, 0x65, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x61, 0x70, 0x69, 0x2e, 0x76, 0x31, 0x2e,
|
|
0x47, 0x65, 0x6e, 0x64, 0x65, 0x72, 0x52, 0x06, 0x67, 0x65, 0x6e, 0x64, 0x65, 0x72, 0x12, 0x14,
|
|
0x0a, 0x05, 0x65, 0x6d, 0x61, 0x69, 0x6c, 0x18, 0x08, 0x20, 0x01, 0x28, 0x09, 0x52, 0x05, 0x65,
|
|
0x6d, 0x61, 0x69, 0x6c, 0x12, 0x2a, 0x0a, 0x11, 0x69, 0x73, 0x5f, 0x65, 0x6d, 0x61, 0x69, 0x6c,
|
|
0x5f, 0x76, 0x65, 0x72, 0x69, 0x66, 0x69, 0x65, 0x64, 0x18, 0x09, 0x20, 0x01, 0x28, 0x08, 0x52,
|
|
0x0f, 0x69, 0x73, 0x45, 0x6d, 0x61, 0x69, 0x6c, 0x56, 0x65, 0x72, 0x69, 0x66, 0x69, 0x65, 0x64,
|
|
0x12, 0x14, 0x0a, 0x05, 0x70, 0x68, 0x6f, 0x6e, 0x65, 0x18, 0x0a, 0x20, 0x01, 0x28, 0x09, 0x52,
|
|
0x05, 0x70, 0x68, 0x6f, 0x6e, 0x65, 0x12, 0x2a, 0x0a, 0x11, 0x69, 0x73, 0x5f, 0x70, 0x68, 0x6f,
|
|
0x6e, 0x65, 0x5f, 0x76, 0x65, 0x72, 0x69, 0x66, 0x69, 0x65, 0x64, 0x18, 0x0b, 0x20, 0x01, 0x28,
|
|
0x08, 0x52, 0x0f, 0x69, 0x73, 0x50, 0x68, 0x6f, 0x6e, 0x65, 0x56, 0x65, 0x72, 0x69, 0x66, 0x69,
|
|
0x65, 0x64, 0x12, 0x18, 0x0a, 0x07, 0x63, 0x6f, 0x75, 0x6e, 0x74, 0x72, 0x79, 0x18, 0x0c, 0x20,
|
|
0x01, 0x28, 0x09, 0x52, 0x07, 0x63, 0x6f, 0x75, 0x6e, 0x74, 0x72, 0x79, 0x12, 0x1a, 0x0a, 0x08,
|
|
0x6c, 0x6f, 0x63, 0x61, 0x6c, 0x69, 0x74, 0x79, 0x18, 0x0d, 0x20, 0x01, 0x28, 0x09, 0x52, 0x08,
|
|
0x6c, 0x6f, 0x63, 0x61, 0x6c, 0x69, 0x74, 0x79, 0x12, 0x1f, 0x0a, 0x0b, 0x70, 0x6f, 0x73, 0x74,
|
|
0x61, 0x6c, 0x5f, 0x63, 0x6f, 0x64, 0x65, 0x18, 0x0e, 0x20, 0x01, 0x28, 0x09, 0x52, 0x0a, 0x70,
|
|
0x6f, 0x73, 0x74, 0x61, 0x6c, 0x43, 0x6f, 0x64, 0x65, 0x12, 0x16, 0x0a, 0x06, 0x72, 0x65, 0x67,
|
|
0x69, 0x6f, 0x6e, 0x18, 0x0f, 0x20, 0x01, 0x28, 0x09, 0x52, 0x06, 0x72, 0x65, 0x67, 0x69, 0x6f,
|
|
0x6e, 0x12, 0x25, 0x0a, 0x0e, 0x73, 0x74, 0x72, 0x65, 0x65, 0x74, 0x5f, 0x61, 0x64, 0x64, 0x72,
|
|
0x65, 0x73, 0x73, 0x18, 0x10, 0x20, 0x01, 0x28, 0x09, 0x52, 0x0d, 0x73, 0x74, 0x72, 0x65, 0x65,
|
|
0x74, 0x41, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x22, 0x9c, 0x03, 0x0a, 0x0b, 0x55, 0x73, 0x65,
|
|
0x72, 0x50, 0x72, 0x6f, 0x66, 0x69, 0x6c, 0x65, 0x12, 0x0e, 0x0a, 0x02, 0x69, 0x64, 0x18, 0x01,
|
|
0x20, 0x01, 0x28, 0x09, 0x52, 0x02, 0x69, 0x64, 0x12, 0x1d, 0x0a, 0x0a, 0x66, 0x69, 0x72, 0x73,
|
|
0x74, 0x5f, 0x6e, 0x61, 0x6d, 0x65, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52, 0x09, 0x66, 0x69,
|
|
0x72, 0x73, 0x74, 0x4e, 0x61, 0x6d, 0x65, 0x12, 0x1b, 0x0a, 0x09, 0x6c, 0x61, 0x73, 0x74, 0x5f,
|
|
0x6e, 0x61, 0x6d, 0x65, 0x18, 0x03, 0x20, 0x01, 0x28, 0x09, 0x52, 0x08, 0x6c, 0x61, 0x73, 0x74,
|
|
0x4e, 0x61, 0x6d, 0x65, 0x12, 0x1b, 0x0a, 0x09, 0x6e, 0x69, 0x63, 0x6b, 0x5f, 0x6e, 0x61, 0x6d,
|
|
0x65, 0x18, 0x04, 0x20, 0x01, 0x28, 0x09, 0x52, 0x08, 0x6e, 0x69, 0x63, 0x6b, 0x4e, 0x61, 0x6d,
|
|
0x65, 0x12, 0x21, 0x0a, 0x0c, 0x64, 0x69, 0x73, 0x70, 0x6c, 0x61, 0x79, 0x5f, 0x6e, 0x61, 0x6d,
|
|
0x65, 0x18, 0x05, 0x20, 0x01, 0x28, 0x09, 0x52, 0x0b, 0x64, 0x69, 0x73, 0x70, 0x6c, 0x61, 0x79,
|
|
0x4e, 0x61, 0x6d, 0x65, 0x12, 0x2d, 0x0a, 0x12, 0x70, 0x72, 0x65, 0x66, 0x65, 0x72, 0x72, 0x65,
|
|
0x64, 0x5f, 0x6c, 0x61, 0x6e, 0x67, 0x75, 0x61, 0x67, 0x65, 0x18, 0x06, 0x20, 0x01, 0x28, 0x09,
|
|
0x52, 0x11, 0x70, 0x72, 0x65, 0x66, 0x65, 0x72, 0x72, 0x65, 0x64, 0x4c, 0x61, 0x6e, 0x67, 0x75,
|
|
0x61, 0x67, 0x65, 0x12, 0x38, 0x0a, 0x06, 0x67, 0x65, 0x6e, 0x64, 0x65, 0x72, 0x18, 0x07, 0x20,
|
|
0x01, 0x28, 0x0e, 0x32, 0x20, 0x2e, 0x63, 0x61, 0x6f, 0x73, 0x2e, 0x7a, 0x69, 0x74, 0x61, 0x64,
|
|
0x65, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x61, 0x70, 0x69, 0x2e, 0x76, 0x31, 0x2e, 0x47,
|
|
0x65, 0x6e, 0x64, 0x65, 0x72, 0x52, 0x06, 0x67, 0x65, 0x6e, 0x64, 0x65, 0x72, 0x12, 0x1a, 0x0a,
|
|
0x08, 0x73, 0x65, 0x71, 0x75, 0x65, 0x6e, 0x63, 0x65, 0x18, 0x08, 0x20, 0x01, 0x28, 0x04, 0x52,
|
|
0x08, 0x73, 0x65, 0x71, 0x75, 0x65, 0x6e, 0x63, 0x65, 0x12, 0x3f, 0x0a, 0x0d, 0x63, 0x72, 0x65,
|
|
0x61, 0x74, 0x69, 0x6f, 0x6e, 0x5f, 0x64, 0x61, 0x74, 0x65, 0x18, 0x09, 0x20, 0x01, 0x28, 0x0b,
|
|
0x32, 0x1a, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62,
|
|
0x75, 0x66, 0x2e, 0x54, 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d, 0x70, 0x52, 0x0c, 0x63, 0x72,
|
|
0x65, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x44, 0x61, 0x74, 0x65, 0x12, 0x3b, 0x0a, 0x0b, 0x63, 0x68,
|
|
0x61, 0x6e, 0x67, 0x65, 0x5f, 0x64, 0x61, 0x74, 0x65, 0x18, 0x0a, 0x20, 0x01, 0x28, 0x0b, 0x32,
|
|
0x1a, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75,
|
|
0x66, 0x2e, 0x54, 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d, 0x70, 0x52, 0x0a, 0x63, 0x68, 0x61,
|
|
0x6e, 0x67, 0x65, 0x44, 0x61, 0x74, 0x65, 0x22, 0xf3, 0x03, 0x0a, 0x0f, 0x55, 0x73, 0x65, 0x72,
|
|
0x50, 0x72, 0x6f, 0x66, 0x69, 0x6c, 0x65, 0x56, 0x69, 0x65, 0x77, 0x12, 0x0e, 0x0a, 0x02, 0x69,
|
|
0x64, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x02, 0x69, 0x64, 0x12, 0x1d, 0x0a, 0x0a, 0x66,
|
|
0x69, 0x72, 0x73, 0x74, 0x5f, 0x6e, 0x61, 0x6d, 0x65, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52,
|
|
0x09, 0x66, 0x69, 0x72, 0x73, 0x74, 0x4e, 0x61, 0x6d, 0x65, 0x12, 0x1b, 0x0a, 0x09, 0x6c, 0x61,
|
|
0x73, 0x74, 0x5f, 0x6e, 0x61, 0x6d, 0x65, 0x18, 0x03, 0x20, 0x01, 0x28, 0x09, 0x52, 0x08, 0x6c,
|
|
0x61, 0x73, 0x74, 0x4e, 0x61, 0x6d, 0x65, 0x12, 0x1b, 0x0a, 0x09, 0x6e, 0x69, 0x63, 0x6b, 0x5f,
|
|
0x6e, 0x61, 0x6d, 0x65, 0x18, 0x04, 0x20, 0x01, 0x28, 0x09, 0x52, 0x08, 0x6e, 0x69, 0x63, 0x6b,
|
|
0x4e, 0x61, 0x6d, 0x65, 0x12, 0x21, 0x0a, 0x0c, 0x64, 0x69, 0x73, 0x70, 0x6c, 0x61, 0x79, 0x5f,
|
|
0x6e, 0x61, 0x6d, 0x65, 0x18, 0x05, 0x20, 0x01, 0x28, 0x09, 0x52, 0x0b, 0x64, 0x69, 0x73, 0x70,
|
|
0x6c, 0x61, 0x79, 0x4e, 0x61, 0x6d, 0x65, 0x12, 0x2d, 0x0a, 0x12, 0x70, 0x72, 0x65, 0x66, 0x65,
|
|
0x72, 0x72, 0x65, 0x64, 0x5f, 0x6c, 0x61, 0x6e, 0x67, 0x75, 0x61, 0x67, 0x65, 0x18, 0x06, 0x20,
|
|
0x01, 0x28, 0x09, 0x52, 0x11, 0x70, 0x72, 0x65, 0x66, 0x65, 0x72, 0x72, 0x65, 0x64, 0x4c, 0x61,
|
|
0x6e, 0x67, 0x75, 0x61, 0x67, 0x65, 0x12, 0x38, 0x0a, 0x06, 0x67, 0x65, 0x6e, 0x64, 0x65, 0x72,
|
|
0x18, 0x07, 0x20, 0x01, 0x28, 0x0e, 0x32, 0x20, 0x2e, 0x63, 0x61, 0x6f, 0x73, 0x2e, 0x7a, 0x69,
|
|
0x74, 0x61, 0x64, 0x65, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x61, 0x70, 0x69, 0x2e, 0x76,
|
|
0x31, 0x2e, 0x47, 0x65, 0x6e, 0x64, 0x65, 0x72, 0x52, 0x06, 0x67, 0x65, 0x6e, 0x64, 0x65, 0x72,
|
|
0x12, 0x1a, 0x0a, 0x08, 0x73, 0x65, 0x71, 0x75, 0x65, 0x6e, 0x63, 0x65, 0x18, 0x08, 0x20, 0x01,
|
|
0x28, 0x04, 0x52, 0x08, 0x73, 0x65, 0x71, 0x75, 0x65, 0x6e, 0x63, 0x65, 0x12, 0x3f, 0x0a, 0x0d,
|
|
0x63, 0x72, 0x65, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x5f, 0x64, 0x61, 0x74, 0x65, 0x18, 0x09, 0x20,
|
|
0x01, 0x28, 0x0b, 0x32, 0x1a, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f,
|
|
0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e, 0x54, 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d, 0x70, 0x52,
|
|
0x0c, 0x63, 0x72, 0x65, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x44, 0x61, 0x74, 0x65, 0x12, 0x3b, 0x0a,
|
|
0x0b, 0x63, 0x68, 0x61, 0x6e, 0x67, 0x65, 0x5f, 0x64, 0x61, 0x74, 0x65, 0x18, 0x0a, 0x20, 0x01,
|
|
0x28, 0x0b, 0x32, 0x1a, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74,
|
|
0x6f, 0x62, 0x75, 0x66, 0x2e, 0x54, 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d, 0x70, 0x52, 0x0a,
|
|
0x63, 0x68, 0x61, 0x6e, 0x67, 0x65, 0x44, 0x61, 0x74, 0x65, 0x12, 0x1f, 0x0a, 0x0b, 0x6c, 0x6f,
|
|
0x67, 0x69, 0x6e, 0x5f, 0x6e, 0x61, 0x6d, 0x65, 0x73, 0x18, 0x0b, 0x20, 0x03, 0x28, 0x09, 0x52,
|
|
0x0a, 0x6c, 0x6f, 0x67, 0x69, 0x6e, 0x4e, 0x61, 0x6d, 0x65, 0x73, 0x12, 0x30, 0x0a, 0x14, 0x70,
|
|
0x72, 0x65, 0x66, 0x65, 0x72, 0x72, 0x65, 0x64, 0x5f, 0x6c, 0x6f, 0x67, 0x69, 0x6e, 0x5f, 0x6e,
|
|
0x61, 0x6d, 0x65, 0x18, 0x0c, 0x20, 0x01, 0x28, 0x09, 0x52, 0x12, 0x70, 0x72, 0x65, 0x66, 0x65,
|
|
0x72, 0x72, 0x65, 0x64, 0x4c, 0x6f, 0x67, 0x69, 0x6e, 0x4e, 0x61, 0x6d, 0x65, 0x22, 0x8a, 0x02,
|
|
0x0a, 0x18, 0x55, 0x70, 0x64, 0x61, 0x74, 0x65, 0x55, 0x73, 0x65, 0x72, 0x50, 0x72, 0x6f, 0x66,
|
|
0x69, 0x6c, 0x65, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x12, 0x29, 0x0a, 0x0a, 0x66, 0x69,
|
|
0x72, 0x73, 0x74, 0x5f, 0x6e, 0x61, 0x6d, 0x65, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x42, 0x0a,
|
|
0xfa, 0x42, 0x07, 0x72, 0x05, 0x10, 0x01, 0x18, 0xc8, 0x01, 0x52, 0x09, 0x66, 0x69, 0x72, 0x73,
|
|
0x74, 0x4e, 0x61, 0x6d, 0x65, 0x12, 0x27, 0x0a, 0x09, 0x6c, 0x61, 0x73, 0x74, 0x5f, 0x6e, 0x61,
|
|
0x6d, 0x65, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x42, 0x0a, 0xfa, 0x42, 0x07, 0x72, 0x05, 0x10,
|
|
0x01, 0x18, 0xc8, 0x01, 0x52, 0x08, 0x6c, 0x61, 0x73, 0x74, 0x4e, 0x61, 0x6d, 0x65, 0x12, 0x25,
|
|
0x0a, 0x09, 0x6e, 0x69, 0x63, 0x6b, 0x5f, 0x6e, 0x61, 0x6d, 0x65, 0x18, 0x03, 0x20, 0x01, 0x28,
|
|
0x09, 0x42, 0x08, 0xfa, 0x42, 0x05, 0x72, 0x03, 0x18, 0xc8, 0x01, 0x52, 0x08, 0x6e, 0x69, 0x63,
|
|
0x6b, 0x4e, 0x61, 0x6d, 0x65, 0x12, 0x39, 0x0a, 0x12, 0x70, 0x72, 0x65, 0x66, 0x65, 0x72, 0x72,
|
|
0x65, 0x64, 0x5f, 0x6c, 0x61, 0x6e, 0x67, 0x75, 0x61, 0x67, 0x65, 0x18, 0x04, 0x20, 0x01, 0x28,
|
|
0x09, 0x42, 0x0a, 0xfa, 0x42, 0x07, 0x72, 0x05, 0x10, 0x01, 0x18, 0xc8, 0x01, 0x52, 0x11, 0x70,
|
|
0x72, 0x65, 0x66, 0x65, 0x72, 0x72, 0x65, 0x64, 0x4c, 0x61, 0x6e, 0x67, 0x75, 0x61, 0x67, 0x65,
|
|
0x12, 0x38, 0x0a, 0x06, 0x67, 0x65, 0x6e, 0x64, 0x65, 0x72, 0x18, 0x05, 0x20, 0x01, 0x28, 0x0e,
|
|
0x32, 0x20, 0x2e, 0x63, 0x61, 0x6f, 0x73, 0x2e, 0x7a, 0x69, 0x74, 0x61, 0x64, 0x65, 0x6c, 0x2e,
|
|
0x61, 0x75, 0x74, 0x68, 0x2e, 0x61, 0x70, 0x69, 0x2e, 0x76, 0x31, 0x2e, 0x47, 0x65, 0x6e, 0x64,
|
|
0x65, 0x72, 0x52, 0x06, 0x67, 0x65, 0x6e, 0x64, 0x65, 0x72, 0x22, 0x52, 0x0a, 0x15, 0x43, 0x68,
|
|
0x61, 0x6e, 0x67, 0x65, 0x55, 0x73, 0x65, 0x72, 0x4e, 0x61, 0x6d, 0x65, 0x52, 0x65, 0x71, 0x75,
|
|
0x65, 0x73, 0x74, 0x12, 0x39, 0x0a, 0x09, 0x75, 0x73, 0x65, 0x72, 0x5f, 0x6e, 0x61, 0x6d, 0x65,
|
|
0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x42, 0x1c, 0xfa, 0x42, 0x19, 0x72, 0x17, 0x32, 0x15, 0x5e,
|
|
0x5b, 0x5e, 0x5b, 0x3a, 0x73, 0x70, 0x61, 0x63, 0x65, 0x3a, 0x5d, 0x5d, 0x7b, 0x31, 0x2c, 0x32,
|
|
0x30, 0x30, 0x7d, 0x24, 0x52, 0x08, 0x75, 0x73, 0x65, 0x72, 0x4e, 0x61, 0x6d, 0x65, 0x22, 0xf5,
|
|
0x01, 0x0a, 0x09, 0x55, 0x73, 0x65, 0x72, 0x45, 0x6d, 0x61, 0x69, 0x6c, 0x12, 0x0e, 0x0a, 0x02,
|
|
0x69, 0x64, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x02, 0x69, 0x64, 0x12, 0x14, 0x0a, 0x05,
|
|
0x65, 0x6d, 0x61, 0x69, 0x6c, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52, 0x05, 0x65, 0x6d, 0x61,
|
|
0x69, 0x6c, 0x12, 0x28, 0x0a, 0x0f, 0x69, 0x73, 0x45, 0x6d, 0x61, 0x69, 0x6c, 0x56, 0x65, 0x72,
|
|
0x69, 0x66, 0x69, 0x65, 0x64, 0x18, 0x03, 0x20, 0x01, 0x28, 0x08, 0x52, 0x0f, 0x69, 0x73, 0x45,
|
|
0x6d, 0x61, 0x69, 0x6c, 0x56, 0x65, 0x72, 0x69, 0x66, 0x69, 0x65, 0x64, 0x12, 0x1a, 0x0a, 0x08,
|
|
0x73, 0x65, 0x71, 0x75, 0x65, 0x6e, 0x63, 0x65, 0x18, 0x04, 0x20, 0x01, 0x28, 0x04, 0x52, 0x08,
|
|
0x73, 0x65, 0x71, 0x75, 0x65, 0x6e, 0x63, 0x65, 0x12, 0x3f, 0x0a, 0x0d, 0x63, 0x72, 0x65, 0x61,
|
|
0x74, 0x69, 0x6f, 0x6e, 0x5f, 0x64, 0x61, 0x74, 0x65, 0x18, 0x05, 0x20, 0x01, 0x28, 0x0b, 0x32,
|
|
0x1a, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75,
|
|
0x66, 0x2e, 0x54, 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d, 0x70, 0x52, 0x0c, 0x63, 0x72, 0x65,
|
|
0x61, 0x74, 0x69, 0x6f, 0x6e, 0x44, 0x61, 0x74, 0x65, 0x12, 0x3b, 0x0a, 0x0b, 0x63, 0x68, 0x61,
|
|
0x6e, 0x67, 0x65, 0x5f, 0x64, 0x61, 0x74, 0x65, 0x18, 0x06, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1a,
|
|
0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66,
|
|
0x2e, 0x54, 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d, 0x70, 0x52, 0x0a, 0x63, 0x68, 0x61, 0x6e,
|
|
0x67, 0x65, 0x44, 0x61, 0x74, 0x65, 0x22, 0xf9, 0x01, 0x0a, 0x0d, 0x55, 0x73, 0x65, 0x72, 0x45,
|
|
0x6d, 0x61, 0x69, 0x6c, 0x56, 0x69, 0x65, 0x77, 0x12, 0x0e, 0x0a, 0x02, 0x69, 0x64, 0x18, 0x01,
|
|
0x20, 0x01, 0x28, 0x09, 0x52, 0x02, 0x69, 0x64, 0x12, 0x14, 0x0a, 0x05, 0x65, 0x6d, 0x61, 0x69,
|
|
0x6c, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52, 0x05, 0x65, 0x6d, 0x61, 0x69, 0x6c, 0x12, 0x28,
|
|
0x0a, 0x0f, 0x69, 0x73, 0x45, 0x6d, 0x61, 0x69, 0x6c, 0x56, 0x65, 0x72, 0x69, 0x66, 0x69, 0x65,
|
|
0x64, 0x18, 0x03, 0x20, 0x01, 0x28, 0x08, 0x52, 0x0f, 0x69, 0x73, 0x45, 0x6d, 0x61, 0x69, 0x6c,
|
|
0x56, 0x65, 0x72, 0x69, 0x66, 0x69, 0x65, 0x64, 0x12, 0x1a, 0x0a, 0x08, 0x73, 0x65, 0x71, 0x75,
|
|
0x65, 0x6e, 0x63, 0x65, 0x18, 0x04, 0x20, 0x01, 0x28, 0x04, 0x52, 0x08, 0x73, 0x65, 0x71, 0x75,
|
|
0x65, 0x6e, 0x63, 0x65, 0x12, 0x3f, 0x0a, 0x0d, 0x63, 0x72, 0x65, 0x61, 0x74, 0x69, 0x6f, 0x6e,
|
|
0x5f, 0x64, 0x61, 0x74, 0x65, 0x18, 0x05, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1a, 0x2e, 0x67, 0x6f,
|
|
0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e, 0x54, 0x69,
|
|
0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d, 0x70, 0x52, 0x0c, 0x63, 0x72, 0x65, 0x61, 0x74, 0x69, 0x6f,
|
|
0x6e, 0x44, 0x61, 0x74, 0x65, 0x12, 0x3b, 0x0a, 0x0b, 0x63, 0x68, 0x61, 0x6e, 0x67, 0x65, 0x5f,
|
|
0x64, 0x61, 0x74, 0x65, 0x18, 0x06, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1a, 0x2e, 0x67, 0x6f, 0x6f,
|
|
0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e, 0x54, 0x69, 0x6d,
|
|
0x65, 0x73, 0x74, 0x61, 0x6d, 0x70, 0x52, 0x0a, 0x63, 0x68, 0x61, 0x6e, 0x67, 0x65, 0x44, 0x61,
|
|
0x74, 0x65, 0x22, 0x3a, 0x0a, 0x18, 0x56, 0x65, 0x72, 0x69, 0x66, 0x79, 0x4d, 0x79, 0x55, 0x73,
|
|
0x65, 0x72, 0x45, 0x6d, 0x61, 0x69, 0x6c, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x12, 0x1e,
|
|
0x0a, 0x04, 0x63, 0x6f, 0x64, 0x65, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x42, 0x0a, 0xfa, 0x42,
|
|
0x07, 0x72, 0x05, 0x10, 0x01, 0x18, 0xc8, 0x01, 0x52, 0x04, 0x63, 0x6f, 0x64, 0x65, 0x22, 0x3a,
|
|
0x0a, 0x16, 0x55, 0x70, 0x64, 0x61, 0x74, 0x65, 0x55, 0x73, 0x65, 0x72, 0x45, 0x6d, 0x61, 0x69,
|
|
0x6c, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x12, 0x20, 0x0a, 0x05, 0x65, 0x6d, 0x61, 0x69,
|
|
0x6c, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x42, 0x0a, 0xfa, 0x42, 0x07, 0x72, 0x05, 0x10, 0x01,
|
|
0x18, 0xc8, 0x01, 0x52, 0x05, 0x65, 0x6d, 0x61, 0x69, 0x6c, 0x22, 0xf7, 0x01, 0x0a, 0x09, 0x55,
|
|
0x73, 0x65, 0x72, 0x50, 0x68, 0x6f, 0x6e, 0x65, 0x12, 0x0e, 0x0a, 0x02, 0x69, 0x64, 0x18, 0x01,
|
|
0x20, 0x01, 0x28, 0x09, 0x52, 0x02, 0x69, 0x64, 0x12, 0x14, 0x0a, 0x05, 0x70, 0x68, 0x6f, 0x6e,
|
|
0x65, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52, 0x05, 0x70, 0x68, 0x6f, 0x6e, 0x65, 0x12, 0x2a,
|
|
0x0a, 0x11, 0x69, 0x73, 0x5f, 0x70, 0x68, 0x6f, 0x6e, 0x65, 0x5f, 0x76, 0x65, 0x72, 0x69, 0x66,
|
|
0x69, 0x65, 0x64, 0x18, 0x03, 0x20, 0x01, 0x28, 0x08, 0x52, 0x0f, 0x69, 0x73, 0x50, 0x68, 0x6f,
|
|
0x6e, 0x65, 0x56, 0x65, 0x72, 0x69, 0x66, 0x69, 0x65, 0x64, 0x12, 0x1a, 0x0a, 0x08, 0x73, 0x65,
|
|
0x71, 0x75, 0x65, 0x6e, 0x63, 0x65, 0x18, 0x04, 0x20, 0x01, 0x28, 0x04, 0x52, 0x08, 0x73, 0x65,
|
|
0x71, 0x75, 0x65, 0x6e, 0x63, 0x65, 0x12, 0x3f, 0x0a, 0x0d, 0x63, 0x72, 0x65, 0x61, 0x74, 0x69,
|
|
0x6f, 0x6e, 0x5f, 0x64, 0x61, 0x74, 0x65, 0x18, 0x05, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1a, 0x2e,
|
|
0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e,
|
|
0x54, 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d, 0x70, 0x52, 0x0c, 0x63, 0x72, 0x65, 0x61, 0x74,
|
|
0x69, 0x6f, 0x6e, 0x44, 0x61, 0x74, 0x65, 0x12, 0x3b, 0x0a, 0x0b, 0x63, 0x68, 0x61, 0x6e, 0x67,
|
|
0x65, 0x5f, 0x64, 0x61, 0x74, 0x65, 0x18, 0x06, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1a, 0x2e, 0x67,
|
|
0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e, 0x54,
|
|
0x69, 0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d, 0x70, 0x52, 0x0a, 0x63, 0x68, 0x61, 0x6e, 0x67, 0x65,
|
|
0x44, 0x61, 0x74, 0x65, 0x22, 0xfb, 0x01, 0x0a, 0x0d, 0x55, 0x73, 0x65, 0x72, 0x50, 0x68, 0x6f,
|
|
0x6e, 0x65, 0x56, 0x69, 0x65, 0x77, 0x12, 0x0e, 0x0a, 0x02, 0x69, 0x64, 0x18, 0x01, 0x20, 0x01,
|
|
0x28, 0x09, 0x52, 0x02, 0x69, 0x64, 0x12, 0x14, 0x0a, 0x05, 0x70, 0x68, 0x6f, 0x6e, 0x65, 0x18,
|
|
0x02, 0x20, 0x01, 0x28, 0x09, 0x52, 0x05, 0x70, 0x68, 0x6f, 0x6e, 0x65, 0x12, 0x2a, 0x0a, 0x11,
|
|
0x69, 0x73, 0x5f, 0x70, 0x68, 0x6f, 0x6e, 0x65, 0x5f, 0x76, 0x65, 0x72, 0x69, 0x66, 0x69, 0x65,
|
|
0x64, 0x18, 0x03, 0x20, 0x01, 0x28, 0x08, 0x52, 0x0f, 0x69, 0x73, 0x50, 0x68, 0x6f, 0x6e, 0x65,
|
|
0x56, 0x65, 0x72, 0x69, 0x66, 0x69, 0x65, 0x64, 0x12, 0x1a, 0x0a, 0x08, 0x73, 0x65, 0x71, 0x75,
|
|
0x65, 0x6e, 0x63, 0x65, 0x18, 0x04, 0x20, 0x01, 0x28, 0x04, 0x52, 0x08, 0x73, 0x65, 0x71, 0x75,
|
|
0x65, 0x6e, 0x63, 0x65, 0x12, 0x3f, 0x0a, 0x0d, 0x63, 0x72, 0x65, 0x61, 0x74, 0x69, 0x6f, 0x6e,
|
|
0x5f, 0x64, 0x61, 0x74, 0x65, 0x18, 0x05, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1a, 0x2e, 0x67, 0x6f,
|
|
0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e, 0x54, 0x69,
|
|
0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d, 0x70, 0x52, 0x0c, 0x63, 0x72, 0x65, 0x61, 0x74, 0x69, 0x6f,
|
|
0x6e, 0x44, 0x61, 0x74, 0x65, 0x12, 0x3b, 0x0a, 0x0b, 0x63, 0x68, 0x61, 0x6e, 0x67, 0x65, 0x5f,
|
|
0x64, 0x61, 0x74, 0x65, 0x18, 0x06, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1a, 0x2e, 0x67, 0x6f, 0x6f,
|
|
0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e, 0x54, 0x69, 0x6d,
|
|
0x65, 0x73, 0x74, 0x61, 0x6d, 0x70, 0x52, 0x0a, 0x63, 0x68, 0x61, 0x6e, 0x67, 0x65, 0x44, 0x61,
|
|
0x74, 0x65, 0x22, 0x39, 0x0a, 0x16, 0x55, 0x70, 0x64, 0x61, 0x74, 0x65, 0x55, 0x73, 0x65, 0x72,
|
|
0x50, 0x68, 0x6f, 0x6e, 0x65, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x12, 0x1f, 0x0a, 0x05,
|
|
0x70, 0x68, 0x6f, 0x6e, 0x65, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x42, 0x09, 0xfa, 0x42, 0x06,
|
|
0x72, 0x04, 0x10, 0x01, 0x18, 0x14, 0x52, 0x05, 0x70, 0x68, 0x6f, 0x6e, 0x65, 0x22, 0x38, 0x0a,
|
|
0x16, 0x56, 0x65, 0x72, 0x69, 0x66, 0x79, 0x55, 0x73, 0x65, 0x72, 0x50, 0x68, 0x6f, 0x6e, 0x65,
|
|
0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x12, 0x1e, 0x0a, 0x04, 0x63, 0x6f, 0x64, 0x65, 0x18,
|
|
0x01, 0x20, 0x01, 0x28, 0x09, 0x42, 0x0a, 0xfa, 0x42, 0x07, 0x72, 0x05, 0x10, 0x01, 0x18, 0xc8,
|
|
0x01, 0x52, 0x04, 0x63, 0x6f, 0x64, 0x65, 0x22, 0xcd, 0x02, 0x0a, 0x0b, 0x55, 0x73, 0x65, 0x72,
|
|
0x41, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x12, 0x0e, 0x0a, 0x02, 0x69, 0x64, 0x18, 0x01, 0x20,
|
|
0x01, 0x28, 0x09, 0x52, 0x02, 0x69, 0x64, 0x12, 0x18, 0x0a, 0x07, 0x63, 0x6f, 0x75, 0x6e, 0x74,
|
|
0x72, 0x79, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52, 0x07, 0x63, 0x6f, 0x75, 0x6e, 0x74, 0x72,
|
|
0x79, 0x12, 0x1a, 0x0a, 0x08, 0x6c, 0x6f, 0x63, 0x61, 0x6c, 0x69, 0x74, 0x79, 0x18, 0x03, 0x20,
|
|
0x01, 0x28, 0x09, 0x52, 0x08, 0x6c, 0x6f, 0x63, 0x61, 0x6c, 0x69, 0x74, 0x79, 0x12, 0x1f, 0x0a,
|
|
0x0b, 0x70, 0x6f, 0x73, 0x74, 0x61, 0x6c, 0x5f, 0x63, 0x6f, 0x64, 0x65, 0x18, 0x04, 0x20, 0x01,
|
|
0x28, 0x09, 0x52, 0x0a, 0x70, 0x6f, 0x73, 0x74, 0x61, 0x6c, 0x43, 0x6f, 0x64, 0x65, 0x12, 0x16,
|
|
0x0a, 0x06, 0x72, 0x65, 0x67, 0x69, 0x6f, 0x6e, 0x18, 0x05, 0x20, 0x01, 0x28, 0x09, 0x52, 0x06,
|
|
0x72, 0x65, 0x67, 0x69, 0x6f, 0x6e, 0x12, 0x25, 0x0a, 0x0e, 0x73, 0x74, 0x72, 0x65, 0x65, 0x74,
|
|
0x5f, 0x61, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x18, 0x06, 0x20, 0x01, 0x28, 0x09, 0x52, 0x0d,
|
|
0x73, 0x74, 0x72, 0x65, 0x65, 0x74, 0x41, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x12, 0x1a, 0x0a,
|
|
0x08, 0x73, 0x65, 0x71, 0x75, 0x65, 0x6e, 0x63, 0x65, 0x18, 0x07, 0x20, 0x01, 0x28, 0x04, 0x52,
|
|
0x08, 0x73, 0x65, 0x71, 0x75, 0x65, 0x6e, 0x63, 0x65, 0x12, 0x3f, 0x0a, 0x0d, 0x63, 0x72, 0x65,
|
|
0x61, 0x74, 0x69, 0x6f, 0x6e, 0x5f, 0x64, 0x61, 0x74, 0x65, 0x18, 0x08, 0x20, 0x01, 0x28, 0x0b,
|
|
0x32, 0x1a, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62,
|
|
0x75, 0x66, 0x2e, 0x54, 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d, 0x70, 0x52, 0x0c, 0x63, 0x72,
|
|
0x65, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x44, 0x61, 0x74, 0x65, 0x12, 0x3b, 0x0a, 0x0b, 0x63, 0x68,
|
|
0x61, 0x6e, 0x67, 0x65, 0x5f, 0x64, 0x61, 0x74, 0x65, 0x18, 0x09, 0x20, 0x01, 0x28, 0x0b, 0x32,
|
|
0x1a, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75,
|
|
0x66, 0x2e, 0x54, 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d, 0x70, 0x52, 0x0a, 0x63, 0x68, 0x61,
|
|
0x6e, 0x67, 0x65, 0x44, 0x61, 0x74, 0x65, 0x22, 0xd1, 0x02, 0x0a, 0x0f, 0x55, 0x73, 0x65, 0x72,
|
|
0x41, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x56, 0x69, 0x65, 0x77, 0x12, 0x0e, 0x0a, 0x02, 0x69,
|
|
0x64, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x02, 0x69, 0x64, 0x12, 0x18, 0x0a, 0x07, 0x63,
|
|
0x6f, 0x75, 0x6e, 0x74, 0x72, 0x79, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52, 0x07, 0x63, 0x6f,
|
|
0x75, 0x6e, 0x74, 0x72, 0x79, 0x12, 0x1a, 0x0a, 0x08, 0x6c, 0x6f, 0x63, 0x61, 0x6c, 0x69, 0x74,
|
|
0x79, 0x18, 0x03, 0x20, 0x01, 0x28, 0x09, 0x52, 0x08, 0x6c, 0x6f, 0x63, 0x61, 0x6c, 0x69, 0x74,
|
|
0x79, 0x12, 0x1f, 0x0a, 0x0b, 0x70, 0x6f, 0x73, 0x74, 0x61, 0x6c, 0x5f, 0x63, 0x6f, 0x64, 0x65,
|
|
0x18, 0x04, 0x20, 0x01, 0x28, 0x09, 0x52, 0x0a, 0x70, 0x6f, 0x73, 0x74, 0x61, 0x6c, 0x43, 0x6f,
|
|
0x64, 0x65, 0x12, 0x16, 0x0a, 0x06, 0x72, 0x65, 0x67, 0x69, 0x6f, 0x6e, 0x18, 0x05, 0x20, 0x01,
|
|
0x28, 0x09, 0x52, 0x06, 0x72, 0x65, 0x67, 0x69, 0x6f, 0x6e, 0x12, 0x25, 0x0a, 0x0e, 0x73, 0x74,
|
|
0x72, 0x65, 0x65, 0x74, 0x5f, 0x61, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x18, 0x06, 0x20, 0x01,
|
|
0x28, 0x09, 0x52, 0x0d, 0x73, 0x74, 0x72, 0x65, 0x65, 0x74, 0x41, 0x64, 0x64, 0x72, 0x65, 0x73,
|
|
0x73, 0x12, 0x1a, 0x0a, 0x08, 0x73, 0x65, 0x71, 0x75, 0x65, 0x6e, 0x63, 0x65, 0x18, 0x07, 0x20,
|
|
0x01, 0x28, 0x04, 0x52, 0x08, 0x73, 0x65, 0x71, 0x75, 0x65, 0x6e, 0x63, 0x65, 0x12, 0x3f, 0x0a,
|
|
0x0d, 0x63, 0x72, 0x65, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x5f, 0x64, 0x61, 0x74, 0x65, 0x18, 0x08,
|
|
0x20, 0x01, 0x28, 0x0b, 0x32, 0x1a, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72,
|
|
0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e, 0x54, 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d, 0x70,
|
|
0x52, 0x0c, 0x63, 0x72, 0x65, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x44, 0x61, 0x74, 0x65, 0x12, 0x3b,
|
|
0x0a, 0x0b, 0x63, 0x68, 0x61, 0x6e, 0x67, 0x65, 0x5f, 0x64, 0x61, 0x74, 0x65, 0x18, 0x09, 0x20,
|
|
0x01, 0x28, 0x0b, 0x32, 0x1a, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f,
|
|
0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e, 0x54, 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d, 0x70, 0x52,
|
|
0x0a, 0x63, 0x68, 0x61, 0x6e, 0x67, 0x65, 0x44, 0x61, 0x74, 0x65, 0x22, 0xe2, 0x01, 0x0a, 0x18,
|
|
0x55, 0x70, 0x64, 0x61, 0x74, 0x65, 0x55, 0x73, 0x65, 0x72, 0x41, 0x64, 0x64, 0x72, 0x65, 0x73,
|
|
0x73, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x12, 0x22, 0x0a, 0x07, 0x63, 0x6f, 0x75, 0x6e,
|
|
0x74, 0x72, 0x79, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x42, 0x08, 0xfa, 0x42, 0x05, 0x72, 0x03,
|
|
0x18, 0xc8, 0x01, 0x52, 0x07, 0x63, 0x6f, 0x75, 0x6e, 0x74, 0x72, 0x79, 0x12, 0x24, 0x0a, 0x08,
|
|
0x6c, 0x6f, 0x63, 0x61, 0x6c, 0x69, 0x74, 0x79, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x42, 0x08,
|
|
0xfa, 0x42, 0x05, 0x72, 0x03, 0x18, 0xc8, 0x01, 0x52, 0x08, 0x6c, 0x6f, 0x63, 0x61, 0x6c, 0x69,
|
|
0x74, 0x79, 0x12, 0x29, 0x0a, 0x0b, 0x70, 0x6f, 0x73, 0x74, 0x61, 0x6c, 0x5f, 0x63, 0x6f, 0x64,
|
|
0x65, 0x18, 0x03, 0x20, 0x01, 0x28, 0x09, 0x42, 0x08, 0xfa, 0x42, 0x05, 0x72, 0x03, 0x18, 0xc8,
|
|
0x01, 0x52, 0x0a, 0x70, 0x6f, 0x73, 0x74, 0x61, 0x6c, 0x43, 0x6f, 0x64, 0x65, 0x12, 0x20, 0x0a,
|
|
0x06, 0x72, 0x65, 0x67, 0x69, 0x6f, 0x6e, 0x18, 0x04, 0x20, 0x01, 0x28, 0x09, 0x42, 0x08, 0xfa,
|
|
0x42, 0x05, 0x72, 0x03, 0x18, 0xc8, 0x01, 0x52, 0x06, 0x72, 0x65, 0x67, 0x69, 0x6f, 0x6e, 0x12,
|
|
0x2f, 0x0a, 0x0e, 0x73, 0x74, 0x72, 0x65, 0x65, 0x74, 0x5f, 0x61, 0x64, 0x64, 0x72, 0x65, 0x73,
|
|
0x73, 0x18, 0x05, 0x20, 0x01, 0x28, 0x09, 0x42, 0x08, 0xfa, 0x42, 0x05, 0x72, 0x03, 0x18, 0xc8,
|
|
0x01, 0x52, 0x0d, 0x73, 0x74, 0x72, 0x65, 0x65, 0x74, 0x41, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73,
|
|
0x22, 0x6c, 0x0a, 0x0e, 0x50, 0x61, 0x73, 0x73, 0x77, 0x6f, 0x72, 0x64, 0x43, 0x68, 0x61, 0x6e,
|
|
0x67, 0x65, 0x12, 0x2c, 0x0a, 0x0c, 0x6f, 0x6c, 0x64, 0x5f, 0x70, 0x61, 0x73, 0x73, 0x77, 0x6f,
|
|
0x72, 0x64, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x42, 0x09, 0xfa, 0x42, 0x06, 0x72, 0x04, 0x10,
|
|
0x01, 0x18, 0x48, 0x52, 0x0b, 0x6f, 0x6c, 0x64, 0x50, 0x61, 0x73, 0x73, 0x77, 0x6f, 0x72, 0x64,
|
|
0x12, 0x2c, 0x0a, 0x0c, 0x6e, 0x65, 0x77, 0x5f, 0x70, 0x61, 0x73, 0x73, 0x77, 0x6f, 0x72, 0x64,
|
|
0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x42, 0x09, 0xfa, 0x42, 0x06, 0x72, 0x04, 0x10, 0x01, 0x18,
|
|
0x48, 0x52, 0x0b, 0x6e, 0x65, 0x77, 0x50, 0x61, 0x73, 0x73, 0x77, 0x6f, 0x72, 0x64, 0x22, 0x2b,
|
|
0x0a, 0x0c, 0x56, 0x65, 0x72, 0x69, 0x66, 0x79, 0x4d, 0x66, 0x61, 0x4f, 0x74, 0x70, 0x12, 0x1b,
|
|
0x0a, 0x04, 0x63, 0x6f, 0x64, 0x65, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x42, 0x07, 0xfa, 0x42,
|
|
0x04, 0x72, 0x02, 0x10, 0x01, 0x52, 0x04, 0x63, 0x6f, 0x64, 0x65, 0x22, 0x49, 0x0a, 0x0c, 0x4d,
|
|
0x75, 0x6c, 0x74, 0x69, 0x46, 0x61, 0x63, 0x74, 0x6f, 0x72, 0x73, 0x12, 0x39, 0x0a, 0x04, 0x6d,
|
|
0x66, 0x61, 0x73, 0x18, 0x01, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x25, 0x2e, 0x63, 0x61, 0x6f, 0x73,
|
|
0x2e, 0x7a, 0x69, 0x74, 0x61, 0x64, 0x65, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x61, 0x70,
|
|
0x69, 0x2e, 0x76, 0x31, 0x2e, 0x4d, 0x75, 0x6c, 0x74, 0x69, 0x46, 0x61, 0x63, 0x74, 0x6f, 0x72,
|
|
0x52, 0x04, 0x6d, 0x66, 0x61, 0x73, 0x22, 0x7e, 0x0a, 0x0b, 0x4d, 0x75, 0x6c, 0x74, 0x69, 0x46,
|
|
0x61, 0x63, 0x74, 0x6f, 0x72, 0x12, 0x35, 0x0a, 0x04, 0x74, 0x79, 0x70, 0x65, 0x18, 0x01, 0x20,
|
|
0x01, 0x28, 0x0e, 0x32, 0x21, 0x2e, 0x63, 0x61, 0x6f, 0x73, 0x2e, 0x7a, 0x69, 0x74, 0x61, 0x64,
|
|
0x65, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x61, 0x70, 0x69, 0x2e, 0x76, 0x31, 0x2e, 0x4d,
|
|
0x66, 0x61, 0x54, 0x79, 0x70, 0x65, 0x52, 0x04, 0x74, 0x79, 0x70, 0x65, 0x12, 0x38, 0x0a, 0x05,
|
|
0x73, 0x74, 0x61, 0x74, 0x65, 0x18, 0x02, 0x20, 0x01, 0x28, 0x0e, 0x32, 0x22, 0x2e, 0x63, 0x61,
|
|
0x6f, 0x73, 0x2e, 0x7a, 0x69, 0x74, 0x61, 0x64, 0x65, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e,
|
|
0x61, 0x70, 0x69, 0x2e, 0x76, 0x31, 0x2e, 0x4d, 0x46, 0x41, 0x53, 0x74, 0x61, 0x74, 0x65, 0x52,
|
|
0x05, 0x73, 0x74, 0x61, 0x74, 0x65, 0x22, 0x8d, 0x01, 0x0a, 0x0e, 0x4d, 0x66, 0x61, 0x4f, 0x74,
|
|
0x70, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x12, 0x17, 0x0a, 0x07, 0x75, 0x73, 0x65,
|
|
0x72, 0x5f, 0x69, 0x64, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x06, 0x75, 0x73, 0x65, 0x72,
|
|
0x49, 0x64, 0x12, 0x10, 0x0a, 0x03, 0x75, 0x72, 0x6c, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52,
|
|
0x03, 0x75, 0x72, 0x6c, 0x12, 0x16, 0x0a, 0x06, 0x73, 0x65, 0x63, 0x72, 0x65, 0x74, 0x18, 0x03,
|
|
0x20, 0x01, 0x28, 0x09, 0x52, 0x06, 0x73, 0x65, 0x63, 0x72, 0x65, 0x74, 0x12, 0x38, 0x0a, 0x05,
|
|
0x73, 0x74, 0x61, 0x74, 0x65, 0x18, 0x04, 0x20, 0x01, 0x28, 0x0e, 0x32, 0x22, 0x2e, 0x63, 0x61,
|
|
0x6f, 0x73, 0x2e, 0x7a, 0x69, 0x74, 0x61, 0x64, 0x65, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e,
|
|
0x61, 0x70, 0x69, 0x2e, 0x76, 0x31, 0x2e, 0x4d, 0x46, 0x41, 0x53, 0x74, 0x61, 0x74, 0x65, 0x52,
|
|
0x05, 0x73, 0x74, 0x61, 0x74, 0x65, 0x22, 0x81, 0x02, 0x0a, 0x16, 0x55, 0x73, 0x65, 0x72, 0x47,
|
|
0x72, 0x61, 0x6e, 0x74, 0x53, 0x65, 0x61, 0x72, 0x63, 0x68, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73,
|
|
0x74, 0x12, 0x16, 0x0a, 0x06, 0x6f, 0x66, 0x66, 0x73, 0x65, 0x74, 0x18, 0x01, 0x20, 0x01, 0x28,
|
|
0x04, 0x52, 0x06, 0x6f, 0x66, 0x66, 0x73, 0x65, 0x74, 0x12, 0x14, 0x0a, 0x05, 0x6c, 0x69, 0x6d,
|
|
0x69, 0x74, 0x18, 0x02, 0x20, 0x01, 0x28, 0x04, 0x52, 0x05, 0x6c, 0x69, 0x6d, 0x69, 0x74, 0x12,
|
|
0x5d, 0x0a, 0x0e, 0x73, 0x6f, 0x72, 0x74, 0x69, 0x6e, 0x67, 0x5f, 0x63, 0x6f, 0x6c, 0x75, 0x6d,
|
|
0x6e, 0x18, 0x03, 0x20, 0x01, 0x28, 0x0e, 0x32, 0x2c, 0x2e, 0x63, 0x61, 0x6f, 0x73, 0x2e, 0x7a,
|
|
0x69, 0x74, 0x61, 0x64, 0x65, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x61, 0x70, 0x69, 0x2e,
|
|
0x76, 0x31, 0x2e, 0x55, 0x73, 0x65, 0x72, 0x47, 0x72, 0x61, 0x6e, 0x74, 0x53, 0x65, 0x61, 0x72,
|
|
0x63, 0x68, 0x4b, 0x65, 0x79, 0x42, 0x08, 0xfa, 0x42, 0x05, 0x82, 0x01, 0x02, 0x20, 0x00, 0x52,
|
|
0x0d, 0x73, 0x6f, 0x72, 0x74, 0x69, 0x6e, 0x67, 0x43, 0x6f, 0x6c, 0x75, 0x6d, 0x6e, 0x12, 0x10,
|
|
0x0a, 0x03, 0x61, 0x73, 0x63, 0x18, 0x04, 0x20, 0x01, 0x28, 0x08, 0x52, 0x03, 0x61, 0x73, 0x63,
|
|
0x12, 0x48, 0x0a, 0x07, 0x71, 0x75, 0x65, 0x72, 0x69, 0x65, 0x73, 0x18, 0x05, 0x20, 0x03, 0x28,
|
|
0x0b, 0x32, 0x2e, 0x2e, 0x63, 0x61, 0x6f, 0x73, 0x2e, 0x7a, 0x69, 0x74, 0x61, 0x64, 0x65, 0x6c,
|
|
0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x61, 0x70, 0x69, 0x2e, 0x76, 0x31, 0x2e, 0x55, 0x73, 0x65,
|
|
0x72, 0x47, 0x72, 0x61, 0x6e, 0x74, 0x53, 0x65, 0x61, 0x72, 0x63, 0x68, 0x51, 0x75, 0x65, 0x72,
|
|
0x79, 0x52, 0x07, 0x71, 0x75, 0x65, 0x72, 0x69, 0x65, 0x73, 0x22, 0xb6, 0x01, 0x0a, 0x14, 0x55,
|
|
0x73, 0x65, 0x72, 0x47, 0x72, 0x61, 0x6e, 0x74, 0x53, 0x65, 0x61, 0x72, 0x63, 0x68, 0x51, 0x75,
|
|
0x65, 0x72, 0x79, 0x12, 0x48, 0x0a, 0x03, 0x6b, 0x65, 0x79, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0e,
|
|
0x32, 0x2c, 0x2e, 0x63, 0x61, 0x6f, 0x73, 0x2e, 0x7a, 0x69, 0x74, 0x61, 0x64, 0x65, 0x6c, 0x2e,
|
|
0x61, 0x75, 0x74, 0x68, 0x2e, 0x61, 0x70, 0x69, 0x2e, 0x76, 0x31, 0x2e, 0x55, 0x73, 0x65, 0x72,
|
|
0x47, 0x72, 0x61, 0x6e, 0x74, 0x53, 0x65, 0x61, 0x72, 0x63, 0x68, 0x4b, 0x65, 0x79, 0x42, 0x08,
|
|
0xfa, 0x42, 0x05, 0x82, 0x01, 0x02, 0x20, 0x00, 0x52, 0x03, 0x6b, 0x65, 0x79, 0x12, 0x3e, 0x0a,
|
|
0x06, 0x6d, 0x65, 0x74, 0x68, 0x6f, 0x64, 0x18, 0x02, 0x20, 0x01, 0x28, 0x0e, 0x32, 0x26, 0x2e,
|
|
0x63, 0x61, 0x6f, 0x73, 0x2e, 0x7a, 0x69, 0x74, 0x61, 0x64, 0x65, 0x6c, 0x2e, 0x61, 0x75, 0x74,
|
|
0x68, 0x2e, 0x61, 0x70, 0x69, 0x2e, 0x76, 0x31, 0x2e, 0x53, 0x65, 0x61, 0x72, 0x63, 0x68, 0x4d,
|
|
0x65, 0x74, 0x68, 0x6f, 0x64, 0x52, 0x06, 0x6d, 0x65, 0x74, 0x68, 0x6f, 0x64, 0x12, 0x14, 0x0a,
|
|
0x05, 0x76, 0x61, 0x6c, 0x75, 0x65, 0x18, 0x03, 0x20, 0x01, 0x28, 0x09, 0x52, 0x05, 0x76, 0x61,
|
|
0x6c, 0x75, 0x65, 0x22, 0x9d, 0x02, 0x0a, 0x17, 0x55, 0x73, 0x65, 0x72, 0x47, 0x72, 0x61, 0x6e,
|
|
0x74, 0x53, 0x65, 0x61, 0x72, 0x63, 0x68, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x12,
|
|
0x16, 0x0a, 0x06, 0x6f, 0x66, 0x66, 0x73, 0x65, 0x74, 0x18, 0x01, 0x20, 0x01, 0x28, 0x04, 0x52,
|
|
0x06, 0x6f, 0x66, 0x66, 0x73, 0x65, 0x74, 0x12, 0x14, 0x0a, 0x05, 0x6c, 0x69, 0x6d, 0x69, 0x74,
|
|
0x18, 0x02, 0x20, 0x01, 0x28, 0x04, 0x52, 0x05, 0x6c, 0x69, 0x6d, 0x69, 0x74, 0x12, 0x21, 0x0a,
|
|
0x0c, 0x74, 0x6f, 0x74, 0x61, 0x6c, 0x5f, 0x72, 0x65, 0x73, 0x75, 0x6c, 0x74, 0x18, 0x03, 0x20,
|
|
0x01, 0x28, 0x04, 0x52, 0x0b, 0x74, 0x6f, 0x74, 0x61, 0x6c, 0x52, 0x65, 0x73, 0x75, 0x6c, 0x74,
|
|
0x12, 0x3f, 0x0a, 0x06, 0x72, 0x65, 0x73, 0x75, 0x6c, 0x74, 0x18, 0x04, 0x20, 0x03, 0x28, 0x0b,
|
|
0x32, 0x27, 0x2e, 0x63, 0x61, 0x6f, 0x73, 0x2e, 0x7a, 0x69, 0x74, 0x61, 0x64, 0x65, 0x6c, 0x2e,
|
|
0x61, 0x75, 0x74, 0x68, 0x2e, 0x61, 0x70, 0x69, 0x2e, 0x76, 0x31, 0x2e, 0x55, 0x73, 0x65, 0x72,
|
|
0x47, 0x72, 0x61, 0x6e, 0x74, 0x56, 0x69, 0x65, 0x77, 0x52, 0x06, 0x72, 0x65, 0x73, 0x75, 0x6c,
|
|
0x74, 0x12, 0x2d, 0x0a, 0x12, 0x70, 0x72, 0x6f, 0x63, 0x65, 0x73, 0x73, 0x65, 0x64, 0x5f, 0x73,
|
|
0x65, 0x71, 0x75, 0x65, 0x6e, 0x63, 0x65, 0x18, 0x05, 0x20, 0x01, 0x28, 0x04, 0x52, 0x11, 0x70,
|
|
0x72, 0x6f, 0x63, 0x65, 0x73, 0x73, 0x65, 0x64, 0x53, 0x65, 0x71, 0x75, 0x65, 0x6e, 0x63, 0x65,
|
|
0x12, 0x41, 0x0a, 0x0e, 0x76, 0x69, 0x65, 0x77, 0x5f, 0x74, 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61,
|
|
0x6d, 0x70, 0x18, 0x06, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1a, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c,
|
|
0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e, 0x54, 0x69, 0x6d, 0x65, 0x73,
|
|
0x74, 0x61, 0x6d, 0x70, 0x52, 0x0d, 0x76, 0x69, 0x65, 0x77, 0x54, 0x69, 0x6d, 0x65, 0x73, 0x74,
|
|
0x61, 0x6d, 0x70, 0x22, 0xa5, 0x01, 0x0a, 0x0d, 0x55, 0x73, 0x65, 0x72, 0x47, 0x72, 0x61, 0x6e,
|
|
0x74, 0x56, 0x69, 0x65, 0x77, 0x12, 0x14, 0x0a, 0x05, 0x4f, 0x72, 0x67, 0x49, 0x64, 0x18, 0x01,
|
|
0x20, 0x01, 0x28, 0x09, 0x52, 0x05, 0x4f, 0x72, 0x67, 0x49, 0x64, 0x12, 0x1c, 0x0a, 0x09, 0x50,
|
|
0x72, 0x6f, 0x6a, 0x65, 0x63, 0x74, 0x49, 0x64, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52, 0x09,
|
|
0x50, 0x72, 0x6f, 0x6a, 0x65, 0x63, 0x74, 0x49, 0x64, 0x12, 0x16, 0x0a, 0x06, 0x55, 0x73, 0x65,
|
|
0x72, 0x49, 0x64, 0x18, 0x03, 0x20, 0x01, 0x28, 0x09, 0x52, 0x06, 0x55, 0x73, 0x65, 0x72, 0x49,
|
|
0x64, 0x12, 0x14, 0x0a, 0x05, 0x52, 0x6f, 0x6c, 0x65, 0x73, 0x18, 0x04, 0x20, 0x03, 0x28, 0x09,
|
|
0x52, 0x05, 0x52, 0x6f, 0x6c, 0x65, 0x73, 0x12, 0x18, 0x0a, 0x07, 0x4f, 0x72, 0x67, 0x4e, 0x61,
|
|
0x6d, 0x65, 0x18, 0x05, 0x20, 0x01, 0x28, 0x09, 0x52, 0x07, 0x4f, 0x72, 0x67, 0x4e, 0x61, 0x6d,
|
|
0x65, 0x12, 0x18, 0x0a, 0x07, 0x47, 0x72, 0x61, 0x6e, 0x74, 0x49, 0x64, 0x18, 0x06, 0x20, 0x01,
|
|
0x28, 0x09, 0x52, 0x07, 0x47, 0x72, 0x61, 0x6e, 0x74, 0x49, 0x64, 0x22, 0xa8, 0x01, 0x0a, 0x19,
|
|
0x4d, 0x79, 0x50, 0x72, 0x6f, 0x6a, 0x65, 0x63, 0x74, 0x4f, 0x72, 0x67, 0x53, 0x65, 0x61, 0x72,
|
|
0x63, 0x68, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x12, 0x16, 0x0a, 0x06, 0x6f, 0x66, 0x66,
|
|
0x73, 0x65, 0x74, 0x18, 0x01, 0x20, 0x01, 0x28, 0x04, 0x52, 0x06, 0x6f, 0x66, 0x66, 0x73, 0x65,
|
|
0x74, 0x12, 0x14, 0x0a, 0x05, 0x6c, 0x69, 0x6d, 0x69, 0x74, 0x18, 0x02, 0x20, 0x01, 0x28, 0x04,
|
|
0x52, 0x05, 0x6c, 0x69, 0x6d, 0x69, 0x74, 0x12, 0x10, 0x0a, 0x03, 0x61, 0x73, 0x63, 0x18, 0x04,
|
|
0x20, 0x01, 0x28, 0x08, 0x52, 0x03, 0x61, 0x73, 0x63, 0x12, 0x4b, 0x0a, 0x07, 0x71, 0x75, 0x65,
|
|
0x72, 0x69, 0x65, 0x73, 0x18, 0x05, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x31, 0x2e, 0x63, 0x61, 0x6f,
|
|
0x73, 0x2e, 0x7a, 0x69, 0x74, 0x61, 0x64, 0x65, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x61,
|
|
0x70, 0x69, 0x2e, 0x76, 0x31, 0x2e, 0x4d, 0x79, 0x50, 0x72, 0x6f, 0x6a, 0x65, 0x63, 0x74, 0x4f,
|
|
0x72, 0x67, 0x53, 0x65, 0x61, 0x72, 0x63, 0x68, 0x51, 0x75, 0x65, 0x72, 0x79, 0x52, 0x07, 0x71,
|
|
0x75, 0x65, 0x72, 0x69, 0x65, 0x73, 0x22, 0xbc, 0x01, 0x0a, 0x17, 0x4d, 0x79, 0x50, 0x72, 0x6f,
|
|
0x6a, 0x65, 0x63, 0x74, 0x4f, 0x72, 0x67, 0x53, 0x65, 0x61, 0x72, 0x63, 0x68, 0x51, 0x75, 0x65,
|
|
0x72, 0x79, 0x12, 0x4b, 0x0a, 0x03, 0x6b, 0x65, 0x79, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0e, 0x32,
|
|
0x2f, 0x2e, 0x63, 0x61, 0x6f, 0x73, 0x2e, 0x7a, 0x69, 0x74, 0x61, 0x64, 0x65, 0x6c, 0x2e, 0x61,
|
|
0x75, 0x74, 0x68, 0x2e, 0x61, 0x70, 0x69, 0x2e, 0x76, 0x31, 0x2e, 0x4d, 0x79, 0x50, 0x72, 0x6f,
|
|
0x6a, 0x65, 0x63, 0x74, 0x4f, 0x72, 0x67, 0x53, 0x65, 0x61, 0x72, 0x63, 0x68, 0x4b, 0x65, 0x79,
|
|
0x42, 0x08, 0xfa, 0x42, 0x05, 0x82, 0x01, 0x02, 0x20, 0x00, 0x52, 0x03, 0x6b, 0x65, 0x79, 0x12,
|
|
0x3e, 0x0a, 0x06, 0x6d, 0x65, 0x74, 0x68, 0x6f, 0x64, 0x18, 0x02, 0x20, 0x01, 0x28, 0x0e, 0x32,
|
|
0x26, 0x2e, 0x63, 0x61, 0x6f, 0x73, 0x2e, 0x7a, 0x69, 0x74, 0x61, 0x64, 0x65, 0x6c, 0x2e, 0x61,
|
|
0x75, 0x74, 0x68, 0x2e, 0x61, 0x70, 0x69, 0x2e, 0x76, 0x31, 0x2e, 0x53, 0x65, 0x61, 0x72, 0x63,
|
|
0x68, 0x4d, 0x65, 0x74, 0x68, 0x6f, 0x64, 0x52, 0x06, 0x6d, 0x65, 0x74, 0x68, 0x6f, 0x64, 0x12,
|
|
0x14, 0x0a, 0x05, 0x76, 0x61, 0x6c, 0x75, 0x65, 0x18, 0x03, 0x20, 0x01, 0x28, 0x09, 0x52, 0x05,
|
|
0x76, 0x61, 0x6c, 0x75, 0x65, 0x22, 0xa4, 0x01, 0x0a, 0x1a, 0x4d, 0x79, 0x50, 0x72, 0x6f, 0x6a,
|
|
0x65, 0x63, 0x74, 0x4f, 0x72, 0x67, 0x53, 0x65, 0x61, 0x72, 0x63, 0x68, 0x52, 0x65, 0x73, 0x70,
|
|
0x6f, 0x6e, 0x73, 0x65, 0x12, 0x16, 0x0a, 0x06, 0x6f, 0x66, 0x66, 0x73, 0x65, 0x74, 0x18, 0x01,
|
|
0x20, 0x01, 0x28, 0x04, 0x52, 0x06, 0x6f, 0x66, 0x66, 0x73, 0x65, 0x74, 0x12, 0x14, 0x0a, 0x05,
|
|
0x6c, 0x69, 0x6d, 0x69, 0x74, 0x18, 0x02, 0x20, 0x01, 0x28, 0x04, 0x52, 0x05, 0x6c, 0x69, 0x6d,
|
|
0x69, 0x74, 0x12, 0x21, 0x0a, 0x0c, 0x74, 0x6f, 0x74, 0x61, 0x6c, 0x5f, 0x72, 0x65, 0x73, 0x75,
|
|
0x6c, 0x74, 0x18, 0x03, 0x20, 0x01, 0x28, 0x04, 0x52, 0x0b, 0x74, 0x6f, 0x74, 0x61, 0x6c, 0x52,
|
|
0x65, 0x73, 0x75, 0x6c, 0x74, 0x12, 0x35, 0x0a, 0x06, 0x72, 0x65, 0x73, 0x75, 0x6c, 0x74, 0x18,
|
|
0x04, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x1d, 0x2e, 0x63, 0x61, 0x6f, 0x73, 0x2e, 0x7a, 0x69, 0x74,
|
|
0x61, 0x64, 0x65, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x61, 0x70, 0x69, 0x2e, 0x76, 0x31,
|
|
0x2e, 0x4f, 0x72, 0x67, 0x52, 0x06, 0x72, 0x65, 0x73, 0x75, 0x6c, 0x74, 0x22, 0x29, 0x0a, 0x03,
|
|
0x4f, 0x72, 0x67, 0x12, 0x0e, 0x0a, 0x02, 0x69, 0x64, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52,
|
|
0x02, 0x69, 0x64, 0x12, 0x12, 0x0a, 0x04, 0x6e, 0x61, 0x6d, 0x65, 0x18, 0x02, 0x20, 0x01, 0x28,
|
|
0x09, 0x52, 0x04, 0x6e, 0x61, 0x6d, 0x65, 0x22, 0x31, 0x0a, 0x0d, 0x4d, 0x79, 0x50, 0x65, 0x72,
|
|
0x6d, 0x69, 0x73, 0x73, 0x69, 0x6f, 0x6e, 0x73, 0x12, 0x20, 0x0a, 0x0b, 0x70, 0x65, 0x72, 0x6d,
|
|
0x69, 0x73, 0x73, 0x69, 0x6f, 0x6e, 0x73, 0x18, 0x01, 0x20, 0x03, 0x28, 0x09, 0x52, 0x0b, 0x70,
|
|
0x65, 0x72, 0x6d, 0x69, 0x73, 0x73, 0x69, 0x6f, 0x6e, 0x73, 0x22, 0x61, 0x0a, 0x0e, 0x43, 0x68,
|
|
0x61, 0x6e, 0x67, 0x65, 0x73, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x12, 0x14, 0x0a, 0x05,
|
|
0x6c, 0x69, 0x6d, 0x69, 0x74, 0x18, 0x01, 0x20, 0x01, 0x28, 0x04, 0x52, 0x05, 0x6c, 0x69, 0x6d,
|
|
0x69, 0x74, 0x12, 0x27, 0x0a, 0x0f, 0x73, 0x65, 0x71, 0x75, 0x65, 0x6e, 0x63, 0x65, 0x5f, 0x6f,
|
|
0x66, 0x66, 0x73, 0x65, 0x74, 0x18, 0x02, 0x20, 0x01, 0x28, 0x04, 0x52, 0x0e, 0x73, 0x65, 0x71,
|
|
0x75, 0x65, 0x6e, 0x63, 0x65, 0x4f, 0x66, 0x66, 0x73, 0x65, 0x74, 0x12, 0x10, 0x0a, 0x03, 0x61,
|
|
0x73, 0x63, 0x18, 0x03, 0x20, 0x01, 0x28, 0x08, 0x52, 0x03, 0x61, 0x73, 0x63, 0x22, 0x73, 0x0a,
|
|
0x07, 0x43, 0x68, 0x61, 0x6e, 0x67, 0x65, 0x73, 0x12, 0x3a, 0x0a, 0x07, 0x63, 0x68, 0x61, 0x6e,
|
|
0x67, 0x65, 0x73, 0x18, 0x01, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x20, 0x2e, 0x63, 0x61, 0x6f, 0x73,
|
|
0x2e, 0x7a, 0x69, 0x74, 0x61, 0x64, 0x65, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x61, 0x70,
|
|
0x69, 0x2e, 0x76, 0x31, 0x2e, 0x43, 0x68, 0x61, 0x6e, 0x67, 0x65, 0x52, 0x07, 0x63, 0x68, 0x61,
|
|
0x6e, 0x67, 0x65, 0x73, 0x12, 0x16, 0x0a, 0x06, 0x6f, 0x66, 0x66, 0x73, 0x65, 0x74, 0x18, 0x02,
|
|
0x20, 0x01, 0x28, 0x04, 0x52, 0x06, 0x6f, 0x66, 0x66, 0x73, 0x65, 0x74, 0x12, 0x14, 0x0a, 0x05,
|
|
0x6c, 0x69, 0x6d, 0x69, 0x74, 0x18, 0x03, 0x20, 0x01, 0x28, 0x04, 0x52, 0x05, 0x6c, 0x69, 0x6d,
|
|
0x69, 0x74, 0x22, 0x89, 0x02, 0x0a, 0x06, 0x43, 0x68, 0x61, 0x6e, 0x67, 0x65, 0x12, 0x3b, 0x0a,
|
|
0x0b, 0x63, 0x68, 0x61, 0x6e, 0x67, 0x65, 0x5f, 0x64, 0x61, 0x74, 0x65, 0x18, 0x01, 0x20, 0x01,
|
|
0x28, 0x0b, 0x32, 0x1a, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74,
|
|
0x6f, 0x62, 0x75, 0x66, 0x2e, 0x54, 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d, 0x70, 0x52, 0x0a,
|
|
0x63, 0x68, 0x61, 0x6e, 0x67, 0x65, 0x44, 0x61, 0x74, 0x65, 0x12, 0x44, 0x0a, 0x0a, 0x65, 0x76,
|
|
0x65, 0x6e, 0x74, 0x5f, 0x74, 0x79, 0x70, 0x65, 0x18, 0x02, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x25,
|
|
0x2e, 0x63, 0x61, 0x6f, 0x73, 0x2e, 0x7a, 0x69, 0x74, 0x61, 0x64, 0x65, 0x6c, 0x2e, 0x61, 0x70,
|
|
0x69, 0x2e, 0x76, 0x31, 0x2e, 0x4c, 0x6f, 0x63, 0x61, 0x6c, 0x69, 0x7a, 0x65, 0x64, 0x4d, 0x65,
|
|
0x73, 0x73, 0x61, 0x67, 0x65, 0x52, 0x09, 0x65, 0x76, 0x65, 0x6e, 0x74, 0x54, 0x79, 0x70, 0x65,
|
|
0x12, 0x1a, 0x0a, 0x08, 0x73, 0x65, 0x71, 0x75, 0x65, 0x6e, 0x63, 0x65, 0x18, 0x03, 0x20, 0x01,
|
|
0x28, 0x04, 0x52, 0x08, 0x73, 0x65, 0x71, 0x75, 0x65, 0x6e, 0x63, 0x65, 0x12, 0x1b, 0x0a, 0x09,
|
|
0x65, 0x64, 0x69, 0x74, 0x6f, 0x72, 0x5f, 0x69, 0x64, 0x18, 0x04, 0x20, 0x01, 0x28, 0x09, 0x52,
|
|
0x08, 0x65, 0x64, 0x69, 0x74, 0x6f, 0x72, 0x49, 0x64, 0x12, 0x16, 0x0a, 0x06, 0x65, 0x64, 0x69,
|
|
0x74, 0x6f, 0x72, 0x18, 0x05, 0x20, 0x01, 0x28, 0x09, 0x52, 0x06, 0x65, 0x64, 0x69, 0x74, 0x6f,
|
|
0x72, 0x12, 0x2b, 0x0a, 0x04, 0x64, 0x61, 0x74, 0x61, 0x18, 0x06, 0x20, 0x01, 0x28, 0x0b, 0x32,
|
|
0x17, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75,
|
|
0x66, 0x2e, 0x53, 0x74, 0x72, 0x75, 0x63, 0x74, 0x52, 0x04, 0x64, 0x61, 0x74, 0x61, 0x22, 0xac,
|
|
0x03, 0x0a, 0x18, 0x50, 0x61, 0x73, 0x73, 0x77, 0x6f, 0x72, 0x64, 0x43, 0x6f, 0x6d, 0x70, 0x6c,
|
|
0x65, 0x78, 0x69, 0x74, 0x79, 0x50, 0x6f, 0x6c, 0x69, 0x63, 0x79, 0x12, 0x0e, 0x0a, 0x02, 0x69,
|
|
0x64, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x02, 0x69, 0x64, 0x12, 0x20, 0x0a, 0x0b, 0x64,
|
|
0x65, 0x73, 0x63, 0x72, 0x69, 0x70, 0x74, 0x69, 0x6f, 0x6e, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09,
|
|
0x52, 0x0b, 0x64, 0x65, 0x73, 0x63, 0x72, 0x69, 0x70, 0x74, 0x69, 0x6f, 0x6e, 0x12, 0x3f, 0x0a,
|
|
0x0d, 0x63, 0x72, 0x65, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x5f, 0x64, 0x61, 0x74, 0x65, 0x18, 0x03,
|
|
0x20, 0x01, 0x28, 0x0b, 0x32, 0x1a, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72,
|
|
0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e, 0x54, 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d, 0x70,
|
|
0x52, 0x0c, 0x63, 0x72, 0x65, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x44, 0x61, 0x74, 0x65, 0x12, 0x3b,
|
|
0x0a, 0x0b, 0x63, 0x68, 0x61, 0x6e, 0x67, 0x65, 0x5f, 0x64, 0x61, 0x74, 0x65, 0x18, 0x04, 0x20,
|
|
0x01, 0x28, 0x0b, 0x32, 0x1a, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f,
|
|
0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e, 0x54, 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d, 0x70, 0x52,
|
|
0x0a, 0x63, 0x68, 0x61, 0x6e, 0x67, 0x65, 0x44, 0x61, 0x74, 0x65, 0x12, 0x1d, 0x0a, 0x0a, 0x6d,
|
|
0x69, 0x6e, 0x5f, 0x6c, 0x65, 0x6e, 0x67, 0x74, 0x68, 0x18, 0x05, 0x20, 0x01, 0x28, 0x04, 0x52,
|
|
0x09, 0x6d, 0x69, 0x6e, 0x4c, 0x65, 0x6e, 0x67, 0x74, 0x68, 0x12, 0x23, 0x0a, 0x0d, 0x68, 0x61,
|
|
0x73, 0x5f, 0x6c, 0x6f, 0x77, 0x65, 0x72, 0x63, 0x61, 0x73, 0x65, 0x18, 0x06, 0x20, 0x01, 0x28,
|
|
0x08, 0x52, 0x0c, 0x68, 0x61, 0x73, 0x4c, 0x6f, 0x77, 0x65, 0x72, 0x63, 0x61, 0x73, 0x65, 0x12,
|
|
0x23, 0x0a, 0x0d, 0x68, 0x61, 0x73, 0x5f, 0x75, 0x70, 0x70, 0x65, 0x72, 0x63, 0x61, 0x73, 0x65,
|
|
0x18, 0x07, 0x20, 0x01, 0x28, 0x08, 0x52, 0x0c, 0x68, 0x61, 0x73, 0x55, 0x70, 0x70, 0x65, 0x72,
|
|
0x63, 0x61, 0x73, 0x65, 0x12, 0x1d, 0x0a, 0x0a, 0x68, 0x61, 0x73, 0x5f, 0x6e, 0x75, 0x6d, 0x62,
|
|
0x65, 0x72, 0x18, 0x08, 0x20, 0x01, 0x28, 0x08, 0x52, 0x09, 0x68, 0x61, 0x73, 0x4e, 0x75, 0x6d,
|
|
0x62, 0x65, 0x72, 0x12, 0x1d, 0x0a, 0x0a, 0x68, 0x61, 0x73, 0x5f, 0x73, 0x79, 0x6d, 0x62, 0x6f,
|
|
0x6c, 0x18, 0x09, 0x20, 0x01, 0x28, 0x08, 0x52, 0x09, 0x68, 0x61, 0x73, 0x53, 0x79, 0x6d, 0x62,
|
|
0x6f, 0x6c, 0x12, 0x1a, 0x0a, 0x08, 0x73, 0x65, 0x71, 0x75, 0x65, 0x6e, 0x63, 0x65, 0x18, 0x0a,
|
|
0x20, 0x01, 0x28, 0x04, 0x52, 0x08, 0x73, 0x65, 0x71, 0x75, 0x65, 0x6e, 0x63, 0x65, 0x12, 0x1d,
|
|
0x0a, 0x0a, 0x69, 0x73, 0x5f, 0x64, 0x65, 0x66, 0x61, 0x75, 0x6c, 0x74, 0x18, 0x0b, 0x20, 0x01,
|
|
0x28, 0x08, 0x52, 0x09, 0x69, 0x73, 0x44, 0x65, 0x66, 0x61, 0x75, 0x6c, 0x74, 0x22, 0x75, 0x0a,
|
|
0x13, 0x45, 0x78, 0x74, 0x65, 0x72, 0x6e, 0x61, 0x6c, 0x49, 0x44, 0x50, 0x52, 0x65, 0x73, 0x70,
|
|
0x6f, 0x6e, 0x73, 0x65, 0x12, 0x22, 0x0a, 0x0d, 0x69, 0x64, 0x70, 0x5f, 0x63, 0x6f, 0x6e, 0x66,
|
|
0x69, 0x67, 0x5f, 0x69, 0x64, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x0b, 0x69, 0x64, 0x70,
|
|
0x43, 0x6f, 0x6e, 0x66, 0x69, 0x67, 0x49, 0x64, 0x12, 0x17, 0x0a, 0x07, 0x75, 0x73, 0x65, 0x72,
|
|
0x5f, 0x69, 0x64, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52, 0x06, 0x75, 0x73, 0x65, 0x72, 0x49,
|
|
0x64, 0x12, 0x21, 0x0a, 0x0c, 0x64, 0x69, 0x73, 0x70, 0x6c, 0x61, 0x79, 0x5f, 0x6e, 0x61, 0x6d,
|
|
0x65, 0x18, 0x03, 0x20, 0x01, 0x28, 0x09, 0x52, 0x0b, 0x64, 0x69, 0x73, 0x70, 0x6c, 0x61, 0x79,
|
|
0x4e, 0x61, 0x6d, 0x65, 0x22, 0x68, 0x0a, 0x18, 0x45, 0x78, 0x74, 0x65, 0x72, 0x6e, 0x61, 0x6c,
|
|
0x49, 0x44, 0x50, 0x52, 0x65, 0x6d, 0x6f, 0x76, 0x65, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74,
|
|
0x12, 0x22, 0x0a, 0x0d, 0x69, 0x64, 0x70, 0x5f, 0x63, 0x6f, 0x6e, 0x66, 0x69, 0x67, 0x5f, 0x69,
|
|
0x64, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x0b, 0x69, 0x64, 0x70, 0x43, 0x6f, 0x6e, 0x66,
|
|
0x69, 0x67, 0x49, 0x64, 0x12, 0x28, 0x0a, 0x10, 0x65, 0x78, 0x74, 0x65, 0x72, 0x6e, 0x61, 0x6c,
|
|
0x5f, 0x75, 0x73, 0x65, 0x72, 0x5f, 0x69, 0x64, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52, 0x0e,
|
|
0x65, 0x78, 0x74, 0x65, 0x72, 0x6e, 0x61, 0x6c, 0x55, 0x73, 0x65, 0x72, 0x49, 0x64, 0x22, 0x48,
|
|
0x0a, 0x18, 0x45, 0x78, 0x74, 0x65, 0x72, 0x6e, 0x61, 0x6c, 0x49, 0x44, 0x50, 0x53, 0x65, 0x61,
|
|
0x72, 0x63, 0x68, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x12, 0x16, 0x0a, 0x06, 0x6f, 0x66,
|
|
0x66, 0x73, 0x65, 0x74, 0x18, 0x01, 0x20, 0x01, 0x28, 0x04, 0x52, 0x06, 0x6f, 0x66, 0x66, 0x73,
|
|
0x65, 0x74, 0x12, 0x14, 0x0a, 0x05, 0x6c, 0x69, 0x6d, 0x69, 0x74, 0x18, 0x02, 0x20, 0x01, 0x28,
|
|
0x04, 0x52, 0x05, 0x6c, 0x69, 0x6d, 0x69, 0x74, 0x22, 0xa1, 0x02, 0x0a, 0x19, 0x45, 0x78, 0x74,
|
|
0x65, 0x72, 0x6e, 0x61, 0x6c, 0x49, 0x44, 0x50, 0x53, 0x65, 0x61, 0x72, 0x63, 0x68, 0x52, 0x65,
|
|
0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x12, 0x16, 0x0a, 0x06, 0x6f, 0x66, 0x66, 0x73, 0x65, 0x74,
|
|
0x18, 0x01, 0x20, 0x01, 0x28, 0x04, 0x52, 0x06, 0x6f, 0x66, 0x66, 0x73, 0x65, 0x74, 0x12, 0x14,
|
|
0x0a, 0x05, 0x6c, 0x69, 0x6d, 0x69, 0x74, 0x18, 0x02, 0x20, 0x01, 0x28, 0x04, 0x52, 0x05, 0x6c,
|
|
0x69, 0x6d, 0x69, 0x74, 0x12, 0x21, 0x0a, 0x0c, 0x74, 0x6f, 0x74, 0x61, 0x6c, 0x5f, 0x72, 0x65,
|
|
0x73, 0x75, 0x6c, 0x74, 0x18, 0x03, 0x20, 0x01, 0x28, 0x04, 0x52, 0x0b, 0x74, 0x6f, 0x74, 0x61,
|
|
0x6c, 0x52, 0x65, 0x73, 0x75, 0x6c, 0x74, 0x12, 0x41, 0x0a, 0x06, 0x72, 0x65, 0x73, 0x75, 0x6c,
|
|
0x74, 0x18, 0x04, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x29, 0x2e, 0x63, 0x61, 0x6f, 0x73, 0x2e, 0x7a,
|
|
0x69, 0x74, 0x61, 0x64, 0x65, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x61, 0x70, 0x69, 0x2e,
|
|
0x76, 0x31, 0x2e, 0x45, 0x78, 0x74, 0x65, 0x72, 0x6e, 0x61, 0x6c, 0x49, 0x44, 0x50, 0x56, 0x69,
|
|
0x65, 0x77, 0x52, 0x06, 0x72, 0x65, 0x73, 0x75, 0x6c, 0x74, 0x12, 0x2d, 0x0a, 0x12, 0x70, 0x72,
|
|
0x6f, 0x63, 0x65, 0x73, 0x73, 0x65, 0x64, 0x5f, 0x73, 0x65, 0x71, 0x75, 0x65, 0x6e, 0x63, 0x65,
|
|
0x18, 0x05, 0x20, 0x01, 0x28, 0x04, 0x52, 0x11, 0x70, 0x72, 0x6f, 0x63, 0x65, 0x73, 0x73, 0x65,
|
|
0x64, 0x53, 0x65, 0x71, 0x75, 0x65, 0x6e, 0x63, 0x65, 0x12, 0x41, 0x0a, 0x0e, 0x76, 0x69, 0x65,
|
|
0x77, 0x5f, 0x74, 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d, 0x70, 0x18, 0x06, 0x20, 0x01, 0x28,
|
|
0x0b, 0x32, 0x1a, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f,
|
|
0x62, 0x75, 0x66, 0x2e, 0x54, 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d, 0x70, 0x52, 0x0d, 0x76,
|
|
0x69, 0x65, 0x77, 0x54, 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d, 0x70, 0x22, 0xce, 0x02, 0x0a,
|
|
0x0f, 0x45, 0x78, 0x74, 0x65, 0x72, 0x6e, 0x61, 0x6c, 0x49, 0x44, 0x50, 0x56, 0x69, 0x65, 0x77,
|
|
0x12, 0x17, 0x0a, 0x07, 0x75, 0x73, 0x65, 0x72, 0x5f, 0x69, 0x64, 0x18, 0x01, 0x20, 0x01, 0x28,
|
|
0x09, 0x52, 0x06, 0x75, 0x73, 0x65, 0x72, 0x49, 0x64, 0x12, 0x22, 0x0a, 0x0d, 0x69, 0x64, 0x70,
|
|
0x5f, 0x63, 0x6f, 0x6e, 0x66, 0x69, 0x67, 0x5f, 0x69, 0x64, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09,
|
|
0x52, 0x0b, 0x69, 0x64, 0x70, 0x43, 0x6f, 0x6e, 0x66, 0x69, 0x67, 0x49, 0x64, 0x12, 0x28, 0x0a,
|
|
0x10, 0x65, 0x78, 0x74, 0x65, 0x72, 0x6e, 0x61, 0x6c, 0x5f, 0x75, 0x73, 0x65, 0x72, 0x5f, 0x69,
|
|
0x64, 0x18, 0x03, 0x20, 0x01, 0x28, 0x09, 0x52, 0x0e, 0x65, 0x78, 0x74, 0x65, 0x72, 0x6e, 0x61,
|
|
0x6c, 0x55, 0x73, 0x65, 0x72, 0x49, 0x64, 0x12, 0x19, 0x0a, 0x08, 0x69, 0x64, 0x70, 0x5f, 0x6e,
|
|
0x61, 0x6d, 0x65, 0x18, 0x04, 0x20, 0x01, 0x28, 0x09, 0x52, 0x07, 0x69, 0x64, 0x70, 0x4e, 0x61,
|
|
0x6d, 0x65, 0x12, 0x3b, 0x0a, 0x1a, 0x65, 0x78, 0x74, 0x65, 0x72, 0x6e, 0x61, 0x6c, 0x5f, 0x75,
|
|
0x73, 0x65, 0x72, 0x5f, 0x64, 0x69, 0x73, 0x70, 0x6c, 0x61, 0x79, 0x5f, 0x6e, 0x61, 0x6d, 0x65,
|
|
0x18, 0x05, 0x20, 0x01, 0x28, 0x09, 0x52, 0x17, 0x65, 0x78, 0x74, 0x65, 0x72, 0x6e, 0x61, 0x6c,
|
|
0x55, 0x73, 0x65, 0x72, 0x44, 0x69, 0x73, 0x70, 0x6c, 0x61, 0x79, 0x4e, 0x61, 0x6d, 0x65, 0x12,
|
|
0x3f, 0x0a, 0x0d, 0x63, 0x72, 0x65, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x5f, 0x64, 0x61, 0x74, 0x65,
|
|
0x18, 0x06, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1a, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e,
|
|
0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e, 0x54, 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61,
|
|
0x6d, 0x70, 0x52, 0x0c, 0x63, 0x72, 0x65, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x44, 0x61, 0x74, 0x65,
|
|
0x12, 0x3b, 0x0a, 0x0b, 0x63, 0x68, 0x61, 0x6e, 0x67, 0x65, 0x5f, 0x64, 0x61, 0x74, 0x65, 0x18,
|
|
0x07, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1a, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70,
|
|
0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e, 0x54, 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d,
|
|
0x70, 0x52, 0x0a, 0x63, 0x68, 0x61, 0x6e, 0x67, 0x65, 0x44, 0x61, 0x74, 0x65, 0x2a, 0x72, 0x0a,
|
|
0x10, 0x55, 0x73, 0x65, 0x72, 0x53, 0x65, 0x73, 0x73, 0x69, 0x6f, 0x6e, 0x53, 0x74, 0x61, 0x74,
|
|
0x65, 0x12, 0x20, 0x0a, 0x1c, 0x55, 0x53, 0x45, 0x52, 0x53, 0x45, 0x53, 0x53, 0x49, 0x4f, 0x4e,
|
|
0x53, 0x54, 0x41, 0x54, 0x45, 0x5f, 0x55, 0x4e, 0x53, 0x50, 0x45, 0x43, 0x49, 0x46, 0x49, 0x45,
|
|
0x44, 0x10, 0x00, 0x12, 0x1b, 0x0a, 0x17, 0x55, 0x53, 0x45, 0x52, 0x53, 0x45, 0x53, 0x53, 0x49,
|
|
0x4f, 0x4e, 0x53, 0x54, 0x41, 0x54, 0x45, 0x5f, 0x41, 0x43, 0x54, 0x49, 0x56, 0x45, 0x10, 0x01,
|
|
0x12, 0x1f, 0x0a, 0x1b, 0x55, 0x53, 0x45, 0x52, 0x53, 0x45, 0x53, 0x53, 0x49, 0x4f, 0x4e, 0x53,
|
|
0x54, 0x41, 0x54, 0x45, 0x5f, 0x54, 0x45, 0x52, 0x4d, 0x49, 0x4e, 0x41, 0x54, 0x45, 0x44, 0x10,
|
|
0x02, 0x2a, 0x41, 0x0a, 0x0e, 0x4d, 0x61, 0x63, 0x68, 0x69, 0x6e, 0x65, 0x4b, 0x65, 0x79, 0x54,
|
|
0x79, 0x70, 0x65, 0x12, 0x1a, 0x0a, 0x16, 0x4d, 0x41, 0x43, 0x48, 0x49, 0x4e, 0x45, 0x4b, 0x45,
|
|
0x59, 0x5f, 0x55, 0x4e, 0x53, 0x50, 0x45, 0x43, 0x49, 0x46, 0x49, 0x45, 0x44, 0x10, 0x00, 0x12,
|
|
0x13, 0x0a, 0x0f, 0x4d, 0x41, 0x43, 0x48, 0x49, 0x4e, 0x45, 0x4b, 0x45, 0x59, 0x5f, 0x4a, 0x53,
|
|
0x4f, 0x4e, 0x10, 0x01, 0x2a, 0xaf, 0x01, 0x0a, 0x09, 0x55, 0x73, 0x65, 0x72, 0x53, 0x74, 0x61,
|
|
0x74, 0x65, 0x12, 0x19, 0x0a, 0x15, 0x55, 0x53, 0x45, 0x52, 0x53, 0x54, 0x41, 0x54, 0x45, 0x5f,
|
|
0x55, 0x4e, 0x53, 0x50, 0x45, 0x43, 0x49, 0x46, 0x49, 0x45, 0x44, 0x10, 0x00, 0x12, 0x14, 0x0a,
|
|
0x10, 0x55, 0x53, 0x45, 0x52, 0x53, 0x54, 0x41, 0x54, 0x45, 0x5f, 0x41, 0x43, 0x54, 0x49, 0x56,
|
|
0x45, 0x10, 0x01, 0x12, 0x16, 0x0a, 0x12, 0x55, 0x53, 0x45, 0x52, 0x53, 0x54, 0x41, 0x54, 0x45,
|
|
0x5f, 0x49, 0x4e, 0x41, 0x43, 0x54, 0x49, 0x56, 0x45, 0x10, 0x02, 0x12, 0x15, 0x0a, 0x11, 0x55,
|
|
0x53, 0x45, 0x52, 0x53, 0x54, 0x41, 0x54, 0x45, 0x5f, 0x44, 0x45, 0x4c, 0x45, 0x54, 0x45, 0x44,
|
|
0x10, 0x03, 0x12, 0x14, 0x0a, 0x10, 0x55, 0x53, 0x45, 0x52, 0x53, 0x54, 0x41, 0x54, 0x45, 0x5f,
|
|
0x4c, 0x4f, 0x43, 0x4b, 0x45, 0x44, 0x10, 0x04, 0x12, 0x15, 0x0a, 0x11, 0x55, 0x53, 0x45, 0x52,
|
|
0x53, 0x54, 0x41, 0x54, 0x45, 0x5f, 0x53, 0x55, 0x53, 0x50, 0x45, 0x4e, 0x44, 0x10, 0x05, 0x12,
|
|
0x15, 0x0a, 0x11, 0x55, 0x53, 0x45, 0x52, 0x53, 0x54, 0x41, 0x54, 0x45, 0x5f, 0x49, 0x4e, 0x49,
|
|
0x54, 0x49, 0x41, 0x4c, 0x10, 0x06, 0x2a, 0x58, 0x0a, 0x06, 0x47, 0x65, 0x6e, 0x64, 0x65, 0x72,
|
|
0x12, 0x16, 0x0a, 0x12, 0x47, 0x45, 0x4e, 0x44, 0x45, 0x52, 0x5f, 0x55, 0x4e, 0x53, 0x50, 0x45,
|
|
0x43, 0x49, 0x46, 0x49, 0x45, 0x44, 0x10, 0x00, 0x12, 0x11, 0x0a, 0x0d, 0x47, 0x45, 0x4e, 0x44,
|
|
0x45, 0x52, 0x5f, 0x46, 0x45, 0x4d, 0x41, 0x4c, 0x45, 0x10, 0x01, 0x12, 0x0f, 0x0a, 0x0b, 0x47,
|
|
0x45, 0x4e, 0x44, 0x45, 0x52, 0x5f, 0x4d, 0x41, 0x4c, 0x45, 0x10, 0x02, 0x12, 0x12, 0x0a, 0x0e,
|
|
0x47, 0x45, 0x4e, 0x44, 0x45, 0x52, 0x5f, 0x44, 0x49, 0x56, 0x45, 0x52, 0x53, 0x45, 0x10, 0x03,
|
|
0x2a, 0x44, 0x0a, 0x07, 0x4d, 0x66, 0x61, 0x54, 0x79, 0x70, 0x65, 0x12, 0x17, 0x0a, 0x13, 0x4d,
|
|
0x46, 0x41, 0x54, 0x59, 0x50, 0x45, 0x5f, 0x55, 0x4e, 0x53, 0x50, 0x45, 0x43, 0x49, 0x46, 0x49,
|
|
0x45, 0x44, 0x10, 0x00, 0x12, 0x0f, 0x0a, 0x0b, 0x4d, 0x46, 0x41, 0x54, 0x59, 0x50, 0x45, 0x5f,
|
|
0x53, 0x4d, 0x53, 0x10, 0x01, 0x12, 0x0f, 0x0a, 0x0b, 0x4d, 0x46, 0x41, 0x54, 0x59, 0x50, 0x45,
|
|
0x5f, 0x4f, 0x54, 0x50, 0x10, 0x02, 0x2a, 0x66, 0x0a, 0x08, 0x4d, 0x46, 0x41, 0x53, 0x74, 0x61,
|
|
0x74, 0x65, 0x12, 0x18, 0x0a, 0x14, 0x4d, 0x46, 0x41, 0x53, 0x54, 0x41, 0x54, 0x45, 0x5f, 0x55,
|
|
0x4e, 0x53, 0x50, 0x45, 0x43, 0x49, 0x46, 0x49, 0x45, 0x44, 0x10, 0x00, 0x12, 0x16, 0x0a, 0x12,
|
|
0x4d, 0x46, 0x41, 0x53, 0x54, 0x41, 0x54, 0x45, 0x5f, 0x4e, 0x4f, 0x54, 0x5f, 0x52, 0x45, 0x41,
|
|
0x44, 0x59, 0x10, 0x01, 0x12, 0x12, 0x0a, 0x0e, 0x4d, 0x46, 0x41, 0x53, 0x54, 0x41, 0x54, 0x45,
|
|
0x5f, 0x52, 0x45, 0x41, 0x44, 0x59, 0x10, 0x02, 0x12, 0x14, 0x0a, 0x10, 0x4d, 0x46, 0x41, 0x53,
|
|
0x54, 0x41, 0x54, 0x45, 0x5f, 0x52, 0x45, 0x4d, 0x4f, 0x56, 0x45, 0x44, 0x10, 0x03, 0x2a, 0x76,
|
|
0x0a, 0x12, 0x55, 0x73, 0x65, 0x72, 0x47, 0x72, 0x61, 0x6e, 0x74, 0x53, 0x65, 0x61, 0x72, 0x63,
|
|
0x68, 0x4b, 0x65, 0x79, 0x12, 0x1e, 0x0a, 0x1a, 0x55, 0x73, 0x65, 0x72, 0x47, 0x72, 0x61, 0x6e,
|
|
0x74, 0x53, 0x65, 0x61, 0x72, 0x63, 0x68, 0x4b, 0x65, 0x79, 0x5f, 0x55, 0x4e, 0x4b, 0x4e, 0x4f,
|
|
0x57, 0x4e, 0x10, 0x00, 0x12, 0x1d, 0x0a, 0x19, 0x55, 0x73, 0x65, 0x72, 0x47, 0x72, 0x61, 0x6e,
|
|
0x74, 0x53, 0x65, 0x61, 0x72, 0x63, 0x68, 0x4b, 0x65, 0x79, 0x5f, 0x4f, 0x52, 0x47, 0x5f, 0x49,
|
|
0x44, 0x10, 0x01, 0x12, 0x21, 0x0a, 0x1d, 0x55, 0x73, 0x65, 0x72, 0x47, 0x72, 0x61, 0x6e, 0x74,
|
|
0x53, 0x65, 0x61, 0x72, 0x63, 0x68, 0x4b, 0x65, 0x79, 0x5f, 0x50, 0x52, 0x4f, 0x4a, 0x45, 0x43,
|
|
0x54, 0x5f, 0x49, 0x44, 0x10, 0x02, 0x2a, 0x62, 0x0a, 0x15, 0x4d, 0x79, 0x50, 0x72, 0x6f, 0x6a,
|
|
0x65, 0x63, 0x74, 0x4f, 0x72, 0x67, 0x53, 0x65, 0x61, 0x72, 0x63, 0x68, 0x4b, 0x65, 0x79, 0x12,
|
|
0x25, 0x0a, 0x21, 0x4d, 0x59, 0x50, 0x52, 0x4f, 0x4a, 0x45, 0x43, 0x54, 0x4f, 0x52, 0x47, 0x53,
|
|
0x45, 0x41, 0x52, 0x43, 0x48, 0x4b, 0x45, 0x59, 0x5f, 0x55, 0x4e, 0x53, 0x50, 0x45, 0x43, 0x49,
|
|
0x46, 0x49, 0x45, 0x44, 0x10, 0x00, 0x12, 0x22, 0x0a, 0x1e, 0x4d, 0x59, 0x50, 0x52, 0x4f, 0x4a,
|
|
0x45, 0x43, 0x54, 0x4f, 0x52, 0x47, 0x53, 0x45, 0x41, 0x52, 0x43, 0x48, 0x4b, 0x45, 0x59, 0x5f,
|
|
0x4f, 0x52, 0x47, 0x5f, 0x4e, 0x41, 0x4d, 0x45, 0x10, 0x01, 0x2a, 0xd6, 0x01, 0x0a, 0x0c, 0x53,
|
|
0x65, 0x61, 0x72, 0x63, 0x68, 0x4d, 0x65, 0x74, 0x68, 0x6f, 0x64, 0x12, 0x17, 0x0a, 0x13, 0x53,
|
|
0x45, 0x41, 0x52, 0x43, 0x48, 0x4d, 0x45, 0x54, 0x48, 0x4f, 0x44, 0x5f, 0x45, 0x51, 0x55, 0x41,
|
|
0x4c, 0x53, 0x10, 0x00, 0x12, 0x1c, 0x0a, 0x18, 0x53, 0x45, 0x41, 0x52, 0x43, 0x48, 0x4d, 0x45,
|
|
0x54, 0x48, 0x4f, 0x44, 0x5f, 0x53, 0x54, 0x41, 0x52, 0x54, 0x53, 0x5f, 0x57, 0x49, 0x54, 0x48,
|
|
0x10, 0x01, 0x12, 0x19, 0x0a, 0x15, 0x53, 0x45, 0x41, 0x52, 0x43, 0x48, 0x4d, 0x45, 0x54, 0x48,
|
|
0x4f, 0x44, 0x5f, 0x43, 0x4f, 0x4e, 0x54, 0x41, 0x49, 0x4e, 0x53, 0x10, 0x02, 0x12, 0x23, 0x0a,
|
|
0x1f, 0x53, 0x45, 0x41, 0x52, 0x43, 0x48, 0x4d, 0x45, 0x54, 0x48, 0x4f, 0x44, 0x5f, 0x45, 0x51,
|
|
0x55, 0x41, 0x4c, 0x53, 0x5f, 0x49, 0x47, 0x4e, 0x4f, 0x52, 0x45, 0x5f, 0x43, 0x41, 0x53, 0x45,
|
|
0x10, 0x03, 0x12, 0x28, 0x0a, 0x24, 0x53, 0x45, 0x41, 0x52, 0x43, 0x48, 0x4d, 0x45, 0x54, 0x48,
|
|
0x4f, 0x44, 0x5f, 0x53, 0x54, 0x41, 0x52, 0x54, 0x53, 0x5f, 0x57, 0x49, 0x54, 0x48, 0x5f, 0x49,
|
|
0x47, 0x4e, 0x4f, 0x52, 0x45, 0x5f, 0x43, 0x41, 0x53, 0x45, 0x10, 0x04, 0x12, 0x25, 0x0a, 0x21,
|
|
0x53, 0x45, 0x41, 0x52, 0x43, 0x48, 0x4d, 0x45, 0x54, 0x48, 0x4f, 0x44, 0x5f, 0x43, 0x4f, 0x4e,
|
|
0x54, 0x41, 0x49, 0x4e, 0x53, 0x5f, 0x49, 0x47, 0x4e, 0x4f, 0x52, 0x45, 0x5f, 0x43, 0x41, 0x53,
|
|
0x45, 0x10, 0x05, 0x32, 0x91, 0x23, 0x0a, 0x0b, 0x41, 0x75, 0x74, 0x68, 0x53, 0x65, 0x72, 0x76,
|
|
0x69, 0x63, 0x65, 0x12, 0x4b, 0x0a, 0x07, 0x48, 0x65, 0x61, 0x6c, 0x74, 0x68, 0x7a, 0x12, 0x16,
|
|
0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66,
|
|
0x2e, 0x45, 0x6d, 0x70, 0x74, 0x79, 0x1a, 0x16, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e,
|
|
0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e, 0x45, 0x6d, 0x70, 0x74, 0x79, 0x22, 0x10,
|
|
0x82, 0xd3, 0xe4, 0x93, 0x02, 0x0a, 0x12, 0x08, 0x2f, 0x68, 0x65, 0x61, 0x6c, 0x74, 0x68, 0x7a,
|
|
0x12, 0x47, 0x0a, 0x05, 0x52, 0x65, 0x61, 0x64, 0x79, 0x12, 0x16, 0x2e, 0x67, 0x6f, 0x6f, 0x67,
|
|
0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e, 0x45, 0x6d, 0x70, 0x74,
|
|
0x79, 0x1a, 0x16, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f,
|
|
0x62, 0x75, 0x66, 0x2e, 0x45, 0x6d, 0x70, 0x74, 0x79, 0x22, 0x0e, 0x82, 0xd3, 0xe4, 0x93, 0x02,
|
|
0x08, 0x12, 0x06, 0x2f, 0x72, 0x65, 0x61, 0x64, 0x79, 0x12, 0x4e, 0x0a, 0x08, 0x56, 0x61, 0x6c,
|
|
0x69, 0x64, 0x61, 0x74, 0x65, 0x12, 0x16, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70,
|
|
0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e, 0x45, 0x6d, 0x70, 0x74, 0x79, 0x1a, 0x17, 0x2e,
|
|
0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e,
|
|
0x53, 0x74, 0x72, 0x75, 0x63, 0x74, 0x22, 0x11, 0x82, 0xd3, 0xe4, 0x93, 0x02, 0x0b, 0x12, 0x09,
|
|
0x2f, 0x76, 0x61, 0x6c, 0x69, 0x64, 0x61, 0x74, 0x65, 0x12, 0x86, 0x01, 0x0a, 0x11, 0x47, 0x65,
|
|
0x74, 0x4d, 0x79, 0x55, 0x73, 0x65, 0x72, 0x53, 0x65, 0x73, 0x73, 0x69, 0x6f, 0x6e, 0x73, 0x12,
|
|
0x16, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75,
|
|
0x66, 0x2e, 0x45, 0x6d, 0x70, 0x74, 0x79, 0x1a, 0x2a, 0x2e, 0x63, 0x61, 0x6f, 0x73, 0x2e, 0x7a,
|
|
0x69, 0x74, 0x61, 0x64, 0x65, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x61, 0x70, 0x69, 0x2e,
|
|
0x76, 0x31, 0x2e, 0x55, 0x73, 0x65, 0x72, 0x53, 0x65, 0x73, 0x73, 0x69, 0x6f, 0x6e, 0x56, 0x69,
|
|
0x65, 0x77, 0x73, 0x22, 0x2d, 0x82, 0xd3, 0xe4, 0x93, 0x02, 0x14, 0x12, 0x12, 0x2f, 0x75, 0x73,
|
|
0x65, 0x72, 0x73, 0x2f, 0x6d, 0x65, 0x2f, 0x73, 0x65, 0x73, 0x73, 0x69, 0x6f, 0x6e, 0x73, 0x82,
|
|
0xb5, 0x18, 0x0f, 0x0a, 0x0d, 0x61, 0x75, 0x74, 0x68, 0x65, 0x6e, 0x74, 0x69, 0x63, 0x61, 0x74,
|
|
0x65, 0x64, 0x12, 0x6d, 0x0a, 0x09, 0x47, 0x65, 0x74, 0x4d, 0x79, 0x55, 0x73, 0x65, 0x72, 0x12,
|
|
0x16, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75,
|
|
0x66, 0x2e, 0x45, 0x6d, 0x70, 0x74, 0x79, 0x1a, 0x22, 0x2e, 0x63, 0x61, 0x6f, 0x73, 0x2e, 0x7a,
|
|
0x69, 0x74, 0x61, 0x64, 0x65, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x61, 0x70, 0x69, 0x2e,
|
|
0x76, 0x31, 0x2e, 0x55, 0x73, 0x65, 0x72, 0x56, 0x69, 0x65, 0x77, 0x22, 0x24, 0x82, 0xd3, 0xe4,
|
|
0x93, 0x02, 0x0b, 0x12, 0x09, 0x2f, 0x75, 0x73, 0x65, 0x72, 0x73, 0x2f, 0x6d, 0x65, 0x82, 0xb5,
|
|
0x18, 0x0f, 0x0a, 0x0d, 0x61, 0x75, 0x74, 0x68, 0x65, 0x6e, 0x74, 0x69, 0x63, 0x61, 0x74, 0x65,
|
|
0x64, 0x12, 0x83, 0x01, 0x0a, 0x10, 0x47, 0x65, 0x74, 0x4d, 0x79, 0x55, 0x73, 0x65, 0x72, 0x50,
|
|
0x72, 0x6f, 0x66, 0x69, 0x6c, 0x65, 0x12, 0x16, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e,
|
|
0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e, 0x45, 0x6d, 0x70, 0x74, 0x79, 0x1a, 0x29,
|
|
0x2e, 0x63, 0x61, 0x6f, 0x73, 0x2e, 0x7a, 0x69, 0x74, 0x61, 0x64, 0x65, 0x6c, 0x2e, 0x61, 0x75,
|
|
0x74, 0x68, 0x2e, 0x61, 0x70, 0x69, 0x2e, 0x76, 0x31, 0x2e, 0x55, 0x73, 0x65, 0x72, 0x50, 0x72,
|
|
0x6f, 0x66, 0x69, 0x6c, 0x65, 0x56, 0x69, 0x65, 0x77, 0x22, 0x2c, 0x82, 0xd3, 0xe4, 0x93, 0x02,
|
|
0x13, 0x12, 0x11, 0x2f, 0x75, 0x73, 0x65, 0x72, 0x73, 0x2f, 0x6d, 0x65, 0x2f, 0x70, 0x72, 0x6f,
|
|
0x66, 0x69, 0x6c, 0x65, 0x82, 0xb5, 0x18, 0x0f, 0x0a, 0x0d, 0x61, 0x75, 0x74, 0x68, 0x65, 0x6e,
|
|
0x74, 0x69, 0x63, 0x61, 0x74, 0x65, 0x64, 0x12, 0xa1, 0x01, 0x0a, 0x13, 0x55, 0x70, 0x64, 0x61,
|
|
0x74, 0x65, 0x4d, 0x79, 0x55, 0x73, 0x65, 0x72, 0x50, 0x72, 0x6f, 0x66, 0x69, 0x6c, 0x65, 0x12,
|
|
0x32, 0x2e, 0x63, 0x61, 0x6f, 0x73, 0x2e, 0x7a, 0x69, 0x74, 0x61, 0x64, 0x65, 0x6c, 0x2e, 0x61,
|
|
0x75, 0x74, 0x68, 0x2e, 0x61, 0x70, 0x69, 0x2e, 0x76, 0x31, 0x2e, 0x55, 0x70, 0x64, 0x61, 0x74,
|
|
0x65, 0x55, 0x73, 0x65, 0x72, 0x50, 0x72, 0x6f, 0x66, 0x69, 0x6c, 0x65, 0x52, 0x65, 0x71, 0x75,
|
|
0x65, 0x73, 0x74, 0x1a, 0x25, 0x2e, 0x63, 0x61, 0x6f, 0x73, 0x2e, 0x7a, 0x69, 0x74, 0x61, 0x64,
|
|
0x65, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x61, 0x70, 0x69, 0x2e, 0x76, 0x31, 0x2e, 0x55,
|
|
0x73, 0x65, 0x72, 0x50, 0x72, 0x6f, 0x66, 0x69, 0x6c, 0x65, 0x22, 0x2f, 0x82, 0xd3, 0xe4, 0x93,
|
|
0x02, 0x16, 0x1a, 0x11, 0x2f, 0x75, 0x73, 0x65, 0x72, 0x73, 0x2f, 0x6d, 0x65, 0x2f, 0x70, 0x72,
|
|
0x6f, 0x66, 0x69, 0x6c, 0x65, 0x3a, 0x01, 0x2a, 0x82, 0xb5, 0x18, 0x0f, 0x0a, 0x0d, 0x61, 0x75,
|
|
0x74, 0x68, 0x65, 0x6e, 0x74, 0x69, 0x63, 0x61, 0x74, 0x65, 0x64, 0x12, 0x8d, 0x01, 0x0a, 0x10,
|
|
0x43, 0x68, 0x61, 0x6e, 0x67, 0x65, 0x4d, 0x79, 0x55, 0x73, 0x65, 0x72, 0x4e, 0x61, 0x6d, 0x65,
|
|
0x12, 0x2f, 0x2e, 0x63, 0x61, 0x6f, 0x73, 0x2e, 0x7a, 0x69, 0x74, 0x61, 0x64, 0x65, 0x6c, 0x2e,
|
|
0x61, 0x75, 0x74, 0x68, 0x2e, 0x61, 0x70, 0x69, 0x2e, 0x76, 0x31, 0x2e, 0x43, 0x68, 0x61, 0x6e,
|
|
0x67, 0x65, 0x55, 0x73, 0x65, 0x72, 0x4e, 0x61, 0x6d, 0x65, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73,
|
|
0x74, 0x1a, 0x16, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f,
|
|
0x62, 0x75, 0x66, 0x2e, 0x45, 0x6d, 0x70, 0x74, 0x79, 0x22, 0x30, 0x82, 0xd3, 0xe4, 0x93, 0x02,
|
|
0x17, 0x1a, 0x12, 0x2f, 0x75, 0x73, 0x65, 0x72, 0x73, 0x2f, 0x6d, 0x65, 0x2f, 0x75, 0x73, 0x65,
|
|
0x72, 0x6e, 0x61, 0x6d, 0x65, 0x3a, 0x01, 0x2a, 0x82, 0xb5, 0x18, 0x0f, 0x0a, 0x0d, 0x61, 0x75,
|
|
0x74, 0x68, 0x65, 0x6e, 0x74, 0x69, 0x63, 0x61, 0x74, 0x65, 0x64, 0x12, 0x7d, 0x0a, 0x0e, 0x47,
|
|
0x65, 0x74, 0x4d, 0x79, 0x55, 0x73, 0x65, 0x72, 0x45, 0x6d, 0x61, 0x69, 0x6c, 0x12, 0x16, 0x2e,
|
|
0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e,
|
|
0x45, 0x6d, 0x70, 0x74, 0x79, 0x1a, 0x27, 0x2e, 0x63, 0x61, 0x6f, 0x73, 0x2e, 0x7a, 0x69, 0x74,
|
|
0x61, 0x64, 0x65, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x61, 0x70, 0x69, 0x2e, 0x76, 0x31,
|
|
0x2e, 0x55, 0x73, 0x65, 0x72, 0x45, 0x6d, 0x61, 0x69, 0x6c, 0x56, 0x69, 0x65, 0x77, 0x22, 0x2a,
|
|
0x82, 0xd3, 0xe4, 0x93, 0x02, 0x11, 0x12, 0x0f, 0x2f, 0x75, 0x73, 0x65, 0x72, 0x73, 0x2f, 0x6d,
|
|
0x65, 0x2f, 0x65, 0x6d, 0x61, 0x69, 0x6c, 0x82, 0xb5, 0x18, 0x0f, 0x0a, 0x0d, 0x61, 0x75, 0x74,
|
|
0x68, 0x65, 0x6e, 0x74, 0x69, 0x63, 0x61, 0x74, 0x65, 0x64, 0x12, 0x99, 0x01, 0x0a, 0x11, 0x43,
|
|
0x68, 0x61, 0x6e, 0x67, 0x65, 0x4d, 0x79, 0x55, 0x73, 0x65, 0x72, 0x45, 0x6d, 0x61, 0x69, 0x6c,
|
|
0x12, 0x30, 0x2e, 0x63, 0x61, 0x6f, 0x73, 0x2e, 0x7a, 0x69, 0x74, 0x61, 0x64, 0x65, 0x6c, 0x2e,
|
|
0x61, 0x75, 0x74, 0x68, 0x2e, 0x61, 0x70, 0x69, 0x2e, 0x76, 0x31, 0x2e, 0x55, 0x70, 0x64, 0x61,
|
|
0x74, 0x65, 0x55, 0x73, 0x65, 0x72, 0x45, 0x6d, 0x61, 0x69, 0x6c, 0x52, 0x65, 0x71, 0x75, 0x65,
|
|
0x73, 0x74, 0x1a, 0x23, 0x2e, 0x63, 0x61, 0x6f, 0x73, 0x2e, 0x7a, 0x69, 0x74, 0x61, 0x64, 0x65,
|
|
0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x61, 0x70, 0x69, 0x2e, 0x76, 0x31, 0x2e, 0x55, 0x73,
|
|
0x65, 0x72, 0x45, 0x6d, 0x61, 0x69, 0x6c, 0x22, 0x2d, 0x82, 0xd3, 0xe4, 0x93, 0x02, 0x14, 0x1a,
|
|
0x0f, 0x2f, 0x75, 0x73, 0x65, 0x72, 0x73, 0x2f, 0x6d, 0x65, 0x2f, 0x65, 0x6d, 0x61, 0x69, 0x6c,
|
|
0x3a, 0x01, 0x2a, 0x82, 0xb5, 0x18, 0x0f, 0x0a, 0x0d, 0x61, 0x75, 0x74, 0x68, 0x65, 0x6e, 0x74,
|
|
0x69, 0x63, 0x61, 0x74, 0x65, 0x64, 0x12, 0x96, 0x01, 0x0a, 0x11, 0x56, 0x65, 0x72, 0x69, 0x66,
|
|
0x79, 0x4d, 0x79, 0x55, 0x73, 0x65, 0x72, 0x45, 0x6d, 0x61, 0x69, 0x6c, 0x12, 0x32, 0x2e, 0x63,
|
|
0x61, 0x6f, 0x73, 0x2e, 0x7a, 0x69, 0x74, 0x61, 0x64, 0x65, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68,
|
|
0x2e, 0x61, 0x70, 0x69, 0x2e, 0x76, 0x31, 0x2e, 0x56, 0x65, 0x72, 0x69, 0x66, 0x79, 0x4d, 0x79,
|
|
0x55, 0x73, 0x65, 0x72, 0x45, 0x6d, 0x61, 0x69, 0x6c, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74,
|
|
0x1a, 0x16, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62,
|
|
0x75, 0x66, 0x2e, 0x45, 0x6d, 0x70, 0x74, 0x79, 0x22, 0x35, 0x82, 0xd3, 0xe4, 0x93, 0x02, 0x1c,
|
|
0x22, 0x17, 0x2f, 0x75, 0x73, 0x65, 0x72, 0x73, 0x2f, 0x6d, 0x65, 0x2f, 0x65, 0x6d, 0x61, 0x69,
|
|
0x6c, 0x2f, 0x5f, 0x76, 0x65, 0x72, 0x69, 0x66, 0x79, 0x3a, 0x01, 0x2a, 0x82, 0xb5, 0x18, 0x0f,
|
|
0x0a, 0x0d, 0x61, 0x75, 0x74, 0x68, 0x65, 0x6e, 0x74, 0x69, 0x63, 0x61, 0x74, 0x65, 0x64, 0x12,
|
|
0x92, 0x01, 0x0a, 0x1d, 0x52, 0x65, 0x73, 0x65, 0x6e, 0x64, 0x4d, 0x79, 0x45, 0x6d, 0x61, 0x69,
|
|
0x6c, 0x56, 0x65, 0x72, 0x69, 0x66, 0x69, 0x63, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x4d, 0x61, 0x69,
|
|
0x6c, 0x12, 0x16, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f,
|
|
0x62, 0x75, 0x66, 0x2e, 0x45, 0x6d, 0x70, 0x74, 0x79, 0x1a, 0x16, 0x2e, 0x67, 0x6f, 0x6f, 0x67,
|
|
0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e, 0x45, 0x6d, 0x70, 0x74,
|
|
0x79, 0x22, 0x41, 0x82, 0xd3, 0xe4, 0x93, 0x02, 0x28, 0x22, 0x23, 0x2f, 0x75, 0x73, 0x65, 0x72,
|
|
0x73, 0x2f, 0x6d, 0x65, 0x2f, 0x65, 0x6d, 0x61, 0x69, 0x6c, 0x2f, 0x5f, 0x72, 0x65, 0x73, 0x65,
|
|
0x6e, 0x64, 0x76, 0x65, 0x72, 0x69, 0x66, 0x69, 0x63, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x3a, 0x01,
|
|
0x2a, 0x82, 0xb5, 0x18, 0x0f, 0x0a, 0x0d, 0x61, 0x75, 0x74, 0x68, 0x65, 0x6e, 0x74, 0x69, 0x63,
|
|
0x61, 0x74, 0x65, 0x64, 0x12, 0x7d, 0x0a, 0x0e, 0x47, 0x65, 0x74, 0x4d, 0x79, 0x55, 0x73, 0x65,
|
|
0x72, 0x50, 0x68, 0x6f, 0x6e, 0x65, 0x12, 0x16, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e,
|
|
0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e, 0x45, 0x6d, 0x70, 0x74, 0x79, 0x1a, 0x27,
|
|
0x2e, 0x63, 0x61, 0x6f, 0x73, 0x2e, 0x7a, 0x69, 0x74, 0x61, 0x64, 0x65, 0x6c, 0x2e, 0x61, 0x75,
|
|
0x74, 0x68, 0x2e, 0x61, 0x70, 0x69, 0x2e, 0x76, 0x31, 0x2e, 0x55, 0x73, 0x65, 0x72, 0x50, 0x68,
|
|
0x6f, 0x6e, 0x65, 0x56, 0x69, 0x65, 0x77, 0x22, 0x2a, 0x82, 0xd3, 0xe4, 0x93, 0x02, 0x11, 0x12,
|
|
0x0f, 0x2f, 0x75, 0x73, 0x65, 0x72, 0x73, 0x2f, 0x6d, 0x65, 0x2f, 0x70, 0x68, 0x6f, 0x6e, 0x65,
|
|
0x82, 0xb5, 0x18, 0x0f, 0x0a, 0x0d, 0x61, 0x75, 0x74, 0x68, 0x65, 0x6e, 0x74, 0x69, 0x63, 0x61,
|
|
0x74, 0x65, 0x64, 0x12, 0x99, 0x01, 0x0a, 0x11, 0x43, 0x68, 0x61, 0x6e, 0x67, 0x65, 0x4d, 0x79,
|
|
0x55, 0x73, 0x65, 0x72, 0x50, 0x68, 0x6f, 0x6e, 0x65, 0x12, 0x30, 0x2e, 0x63, 0x61, 0x6f, 0x73,
|
|
0x2e, 0x7a, 0x69, 0x74, 0x61, 0x64, 0x65, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x61, 0x70,
|
|
0x69, 0x2e, 0x76, 0x31, 0x2e, 0x55, 0x70, 0x64, 0x61, 0x74, 0x65, 0x55, 0x73, 0x65, 0x72, 0x50,
|
|
0x68, 0x6f, 0x6e, 0x65, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x1a, 0x23, 0x2e, 0x63, 0x61,
|
|
0x6f, 0x73, 0x2e, 0x7a, 0x69, 0x74, 0x61, 0x64, 0x65, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e,
|
|
0x61, 0x70, 0x69, 0x2e, 0x76, 0x31, 0x2e, 0x55, 0x73, 0x65, 0x72, 0x50, 0x68, 0x6f, 0x6e, 0x65,
|
|
0x22, 0x2d, 0x82, 0xd3, 0xe4, 0x93, 0x02, 0x14, 0x1a, 0x0f, 0x2f, 0x75, 0x73, 0x65, 0x72, 0x73,
|
|
0x2f, 0x6d, 0x65, 0x2f, 0x70, 0x68, 0x6f, 0x6e, 0x65, 0x3a, 0x01, 0x2a, 0x82, 0xb5, 0x18, 0x0f,
|
|
0x0a, 0x0d, 0x61, 0x75, 0x74, 0x68, 0x65, 0x6e, 0x74, 0x69, 0x63, 0x61, 0x74, 0x65, 0x64, 0x12,
|
|
0x6f, 0x0a, 0x11, 0x52, 0x65, 0x6d, 0x6f, 0x76, 0x65, 0x4d, 0x79, 0x55, 0x73, 0x65, 0x72, 0x50,
|
|
0x68, 0x6f, 0x6e, 0x65, 0x12, 0x16, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72,
|
|
0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e, 0x45, 0x6d, 0x70, 0x74, 0x79, 0x1a, 0x16, 0x2e, 0x67,
|
|
0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e, 0x45,
|
|
0x6d, 0x70, 0x74, 0x79, 0x22, 0x2a, 0x82, 0xd3, 0xe4, 0x93, 0x02, 0x11, 0x2a, 0x0f, 0x2f, 0x75,
|
|
0x73, 0x65, 0x72, 0x73, 0x2f, 0x6d, 0x65, 0x2f, 0x70, 0x68, 0x6f, 0x6e, 0x65, 0x82, 0xb5, 0x18,
|
|
0x0f, 0x0a, 0x0d, 0x61, 0x75, 0x74, 0x68, 0x65, 0x6e, 0x74, 0x69, 0x63, 0x61, 0x74, 0x65, 0x64,
|
|
0x12, 0x94, 0x01, 0x0a, 0x11, 0x56, 0x65, 0x72, 0x69, 0x66, 0x79, 0x4d, 0x79, 0x55, 0x73, 0x65,
|
|
0x72, 0x50, 0x68, 0x6f, 0x6e, 0x65, 0x12, 0x30, 0x2e, 0x63, 0x61, 0x6f, 0x73, 0x2e, 0x7a, 0x69,
|
|
0x74, 0x61, 0x64, 0x65, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x61, 0x70, 0x69, 0x2e, 0x76,
|
|
0x31, 0x2e, 0x56, 0x65, 0x72, 0x69, 0x66, 0x79, 0x55, 0x73, 0x65, 0x72, 0x50, 0x68, 0x6f, 0x6e,
|
|
0x65, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x1a, 0x16, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c,
|
|
0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e, 0x45, 0x6d, 0x70, 0x74, 0x79,
|
|
0x22, 0x35, 0x82, 0xd3, 0xe4, 0x93, 0x02, 0x1c, 0x22, 0x17, 0x2f, 0x75, 0x73, 0x65, 0x72, 0x73,
|
|
0x2f, 0x6d, 0x65, 0x2f, 0x70, 0x68, 0x6f, 0x6e, 0x65, 0x2f, 0x5f, 0x76, 0x65, 0x72, 0x69, 0x66,
|
|
0x79, 0x3a, 0x01, 0x2a, 0x82, 0xb5, 0x18, 0x0f, 0x0a, 0x0d, 0x61, 0x75, 0x74, 0x68, 0x65, 0x6e,
|
|
0x74, 0x69, 0x63, 0x61, 0x74, 0x65, 0x64, 0x12, 0x92, 0x01, 0x0a, 0x1d, 0x52, 0x65, 0x73, 0x65,
|
|
0x6e, 0x64, 0x4d, 0x79, 0x50, 0x68, 0x6f, 0x6e, 0x65, 0x56, 0x65, 0x72, 0x69, 0x66, 0x69, 0x63,
|
|
0x61, 0x74, 0x69, 0x6f, 0x6e, 0x43, 0x6f, 0x64, 0x65, 0x12, 0x16, 0x2e, 0x67, 0x6f, 0x6f, 0x67,
|
|
0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e, 0x45, 0x6d, 0x70, 0x74,
|
|
0x79, 0x1a, 0x16, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f,
|
|
0x62, 0x75, 0x66, 0x2e, 0x45, 0x6d, 0x70, 0x74, 0x79, 0x22, 0x41, 0x82, 0xd3, 0xe4, 0x93, 0x02,
|
|
0x28, 0x22, 0x23, 0x2f, 0x75, 0x73, 0x65, 0x72, 0x73, 0x2f, 0x6d, 0x65, 0x2f, 0x70, 0x68, 0x6f,
|
|
0x6e, 0x65, 0x2f, 0x5f, 0x72, 0x65, 0x73, 0x65, 0x6e, 0x64, 0x76, 0x65, 0x72, 0x69, 0x66, 0x69,
|
|
0x63, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x3a, 0x01, 0x2a, 0x82, 0xb5, 0x18, 0x0f, 0x0a, 0x0d, 0x61,
|
|
0x75, 0x74, 0x68, 0x65, 0x6e, 0x74, 0x69, 0x63, 0x61, 0x74, 0x65, 0x64, 0x12, 0x83, 0x01, 0x0a,
|
|
0x10, 0x47, 0x65, 0x74, 0x4d, 0x79, 0x55, 0x73, 0x65, 0x72, 0x41, 0x64, 0x64, 0x72, 0x65, 0x73,
|
|
0x73, 0x12, 0x16, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f,
|
|
0x62, 0x75, 0x66, 0x2e, 0x45, 0x6d, 0x70, 0x74, 0x79, 0x1a, 0x29, 0x2e, 0x63, 0x61, 0x6f, 0x73,
|
|
0x2e, 0x7a, 0x69, 0x74, 0x61, 0x64, 0x65, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x61, 0x70,
|
|
0x69, 0x2e, 0x76, 0x31, 0x2e, 0x55, 0x73, 0x65, 0x72, 0x41, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73,
|
|
0x56, 0x69, 0x65, 0x77, 0x22, 0x2c, 0x82, 0xd3, 0xe4, 0x93, 0x02, 0x13, 0x12, 0x11, 0x2f, 0x75,
|
|
0x73, 0x65, 0x72, 0x73, 0x2f, 0x6d, 0x65, 0x2f, 0x61, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x82,
|
|
0xb5, 0x18, 0x0f, 0x0a, 0x0d, 0x61, 0x75, 0x74, 0x68, 0x65, 0x6e, 0x74, 0x69, 0x63, 0x61, 0x74,
|
|
0x65, 0x64, 0x12, 0x8d, 0x01, 0x0a, 0x10, 0x47, 0x65, 0x74, 0x4d, 0x79, 0x55, 0x73, 0x65, 0x72,
|
|
0x43, 0x68, 0x61, 0x6e, 0x67, 0x65, 0x73, 0x12, 0x28, 0x2e, 0x63, 0x61, 0x6f, 0x73, 0x2e, 0x7a,
|
|
0x69, 0x74, 0x61, 0x64, 0x65, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x61, 0x70, 0x69, 0x2e,
|
|
0x76, 0x31, 0x2e, 0x43, 0x68, 0x61, 0x6e, 0x67, 0x65, 0x73, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73,
|
|
0x74, 0x1a, 0x21, 0x2e, 0x63, 0x61, 0x6f, 0x73, 0x2e, 0x7a, 0x69, 0x74, 0x61, 0x64, 0x65, 0x6c,
|
|
0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x61, 0x70, 0x69, 0x2e, 0x76, 0x31, 0x2e, 0x43, 0x68, 0x61,
|
|
0x6e, 0x67, 0x65, 0x73, 0x22, 0x2c, 0x82, 0xd3, 0xe4, 0x93, 0x02, 0x13, 0x12, 0x11, 0x2f, 0x75,
|
|
0x73, 0x65, 0x72, 0x73, 0x2f, 0x6d, 0x65, 0x2f, 0x63, 0x68, 0x61, 0x6e, 0x67, 0x65, 0x73, 0x82,
|
|
0xb5, 0x18, 0x0f, 0x0a, 0x0d, 0x61, 0x75, 0x74, 0x68, 0x65, 0x6e, 0x74, 0x69, 0x63, 0x61, 0x74,
|
|
0x65, 0x64, 0x12, 0xa1, 0x01, 0x0a, 0x13, 0x55, 0x70, 0x64, 0x61, 0x74, 0x65, 0x4d, 0x79, 0x55,
|
|
0x73, 0x65, 0x72, 0x41, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x12, 0x32, 0x2e, 0x63, 0x61, 0x6f,
|
|
0x73, 0x2e, 0x7a, 0x69, 0x74, 0x61, 0x64, 0x65, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x61,
|
|
0x70, 0x69, 0x2e, 0x76, 0x31, 0x2e, 0x55, 0x70, 0x64, 0x61, 0x74, 0x65, 0x55, 0x73, 0x65, 0x72,
|
|
0x41, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x1a, 0x25,
|
|
0x2e, 0x63, 0x61, 0x6f, 0x73, 0x2e, 0x7a, 0x69, 0x74, 0x61, 0x64, 0x65, 0x6c, 0x2e, 0x61, 0x75,
|
|
0x74, 0x68, 0x2e, 0x61, 0x70, 0x69, 0x2e, 0x76, 0x31, 0x2e, 0x55, 0x73, 0x65, 0x72, 0x41, 0x64,
|
|
0x64, 0x72, 0x65, 0x73, 0x73, 0x22, 0x2f, 0x82, 0xd3, 0xe4, 0x93, 0x02, 0x16, 0x1a, 0x11, 0x2f,
|
|
0x75, 0x73, 0x65, 0x72, 0x73, 0x2f, 0x6d, 0x65, 0x2f, 0x61, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73,
|
|
0x3a, 0x01, 0x2a, 0x82, 0xb5, 0x18, 0x0f, 0x0a, 0x0d, 0x61, 0x75, 0x74, 0x68, 0x65, 0x6e, 0x74,
|
|
0x69, 0x63, 0x61, 0x74, 0x65, 0x64, 0x12, 0x76, 0x0a, 0x09, 0x47, 0x65, 0x74, 0x4d, 0x79, 0x4d,
|
|
0x66, 0x61, 0x73, 0x12, 0x16, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f,
|
|
0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e, 0x45, 0x6d, 0x70, 0x74, 0x79, 0x1a, 0x26, 0x2e, 0x63, 0x61,
|
|
0x6f, 0x73, 0x2e, 0x7a, 0x69, 0x74, 0x61, 0x64, 0x65, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e,
|
|
0x61, 0x70, 0x69, 0x2e, 0x76, 0x31, 0x2e, 0x4d, 0x75, 0x6c, 0x74, 0x69, 0x46, 0x61, 0x63, 0x74,
|
|
0x6f, 0x72, 0x73, 0x22, 0x29, 0x82, 0xd3, 0xe4, 0x93, 0x02, 0x10, 0x12, 0x0e, 0x2f, 0x75, 0x73,
|
|
0x65, 0x72, 0x73, 0x2f, 0x6d, 0x65, 0x2f, 0x6d, 0x66, 0x61, 0x73, 0x82, 0xb5, 0x18, 0x0f, 0x0a,
|
|
0x0d, 0x61, 0x75, 0x74, 0x68, 0x65, 0x6e, 0x74, 0x69, 0x63, 0x61, 0x74, 0x65, 0x64, 0x12, 0x8f,
|
|
0x01, 0x0a, 0x10, 0x43, 0x68, 0x61, 0x6e, 0x67, 0x65, 0x4d, 0x79, 0x50, 0x61, 0x73, 0x73, 0x77,
|
|
0x6f, 0x72, 0x64, 0x12, 0x28, 0x2e, 0x63, 0x61, 0x6f, 0x73, 0x2e, 0x7a, 0x69, 0x74, 0x61, 0x64,
|
|
0x65, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x61, 0x70, 0x69, 0x2e, 0x76, 0x31, 0x2e, 0x50,
|
|
0x61, 0x73, 0x73, 0x77, 0x6f, 0x72, 0x64, 0x43, 0x68, 0x61, 0x6e, 0x67, 0x65, 0x1a, 0x16, 0x2e,
|
|
0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e,
|
|
0x45, 0x6d, 0x70, 0x74, 0x79, 0x22, 0x39, 0x82, 0xd3, 0xe4, 0x93, 0x02, 0x20, 0x1a, 0x1b, 0x2f,
|
|
0x75, 0x73, 0x65, 0x72, 0x73, 0x2f, 0x6d, 0x65, 0x2f, 0x70, 0x61, 0x73, 0x73, 0x77, 0x6f, 0x72,
|
|
0x64, 0x73, 0x2f, 0x5f, 0x63, 0x68, 0x61, 0x6e, 0x67, 0x65, 0x3a, 0x01, 0x2a, 0x82, 0xb5, 0x18,
|
|
0x0f, 0x0a, 0x0d, 0x61, 0x75, 0x74, 0x68, 0x65, 0x6e, 0x74, 0x69, 0x63, 0x61, 0x74, 0x65, 0x64,
|
|
0x12, 0xa6, 0x01, 0x0a, 0x1d, 0x47, 0x65, 0x74, 0x4d, 0x79, 0x50, 0x61, 0x73, 0x73, 0x77, 0x6f,
|
|
0x72, 0x64, 0x43, 0x6f, 0x6d, 0x70, 0x6c, 0x65, 0x78, 0x69, 0x74, 0x79, 0x50, 0x6f, 0x6c, 0x69,
|
|
0x63, 0x79, 0x12, 0x16, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74,
|
|
0x6f, 0x62, 0x75, 0x66, 0x2e, 0x45, 0x6d, 0x70, 0x74, 0x79, 0x1a, 0x32, 0x2e, 0x63, 0x61, 0x6f,
|
|
0x73, 0x2e, 0x7a, 0x69, 0x74, 0x61, 0x64, 0x65, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x61,
|
|
0x70, 0x69, 0x2e, 0x76, 0x31, 0x2e, 0x50, 0x61, 0x73, 0x73, 0x77, 0x6f, 0x72, 0x64, 0x43, 0x6f,
|
|
0x6d, 0x70, 0x6c, 0x65, 0x78, 0x69, 0x74, 0x79, 0x50, 0x6f, 0x6c, 0x69, 0x63, 0x79, 0x22, 0x39,
|
|
0x82, 0xd3, 0xe4, 0x93, 0x02, 0x20, 0x12, 0x1e, 0x2f, 0x70, 0x6f, 0x6c, 0x69, 0x63, 0x69, 0x65,
|
|
0x73, 0x2f, 0x70, 0x61, 0x73, 0x73, 0x77, 0x6f, 0x72, 0x64, 0x73, 0x2f, 0x63, 0x6f, 0x6d, 0x70,
|
|
0x6c, 0x65, 0x78, 0x69, 0x74, 0x79, 0x82, 0xb5, 0x18, 0x0f, 0x0a, 0x0d, 0x61, 0x75, 0x74, 0x68,
|
|
0x65, 0x6e, 0x74, 0x69, 0x63, 0x61, 0x74, 0x65, 0x64, 0x12, 0xbd, 0x01, 0x0a, 0x14, 0x53, 0x65,
|
|
0x61, 0x72, 0x63, 0x68, 0x4d, 0x79, 0x45, 0x78, 0x74, 0x65, 0x72, 0x6e, 0x61, 0x6c, 0x49, 0x44,
|
|
0x50, 0x73, 0x12, 0x32, 0x2e, 0x63, 0x61, 0x6f, 0x73, 0x2e, 0x7a, 0x69, 0x74, 0x61, 0x64, 0x65,
|
|
0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x61, 0x70, 0x69, 0x2e, 0x76, 0x31, 0x2e, 0x45, 0x78,
|
|
0x74, 0x65, 0x72, 0x6e, 0x61, 0x6c, 0x49, 0x44, 0x50, 0x53, 0x65, 0x61, 0x72, 0x63, 0x68, 0x52,
|
|
0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x1a, 0x33, 0x2e, 0x63, 0x61, 0x6f, 0x73, 0x2e, 0x7a, 0x69,
|
|
0x74, 0x61, 0x64, 0x65, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x61, 0x70, 0x69, 0x2e, 0x76,
|
|
0x31, 0x2e, 0x45, 0x78, 0x74, 0x65, 0x72, 0x6e, 0x61, 0x6c, 0x49, 0x44, 0x50, 0x53, 0x65, 0x61,
|
|
0x72, 0x63, 0x68, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x22, 0x3c, 0x82, 0xd3, 0xe4,
|
|
0x93, 0x02, 0x23, 0x22, 0x1e, 0x2f, 0x75, 0x73, 0x65, 0x72, 0x73, 0x2f, 0x6d, 0x65, 0x2f, 0x65,
|
|
0x78, 0x74, 0x65, 0x72, 0x6e, 0x61, 0x6c, 0x69, 0x64, 0x70, 0x73, 0x2f, 0x5f, 0x73, 0x65, 0x61,
|
|
0x72, 0x63, 0x68, 0x3a, 0x01, 0x2a, 0x82, 0xb5, 0x18, 0x0f, 0x0a, 0x0d, 0x61, 0x75, 0x74, 0x68,
|
|
0x65, 0x6e, 0x74, 0x69, 0x63, 0x61, 0x74, 0x65, 0x64, 0x12, 0xb7, 0x01, 0x0a, 0x13, 0x52, 0x65,
|
|
0x6d, 0x6f, 0x76, 0x65, 0x4d, 0x79, 0x45, 0x78, 0x74, 0x65, 0x72, 0x6e, 0x61, 0x6c, 0x49, 0x44,
|
|
0x50, 0x12, 0x32, 0x2e, 0x63, 0x61, 0x6f, 0x73, 0x2e, 0x7a, 0x69, 0x74, 0x61, 0x64, 0x65, 0x6c,
|
|
0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x61, 0x70, 0x69, 0x2e, 0x76, 0x31, 0x2e, 0x45, 0x78, 0x74,
|
|
0x65, 0x72, 0x6e, 0x61, 0x6c, 0x49, 0x44, 0x50, 0x52, 0x65, 0x6d, 0x6f, 0x76, 0x65, 0x52, 0x65,
|
|
0x71, 0x75, 0x65, 0x73, 0x74, 0x1a, 0x16, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70,
|
|
0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e, 0x45, 0x6d, 0x70, 0x74, 0x79, 0x22, 0x54, 0x82,
|
|
0xd3, 0xe4, 0x93, 0x02, 0x3b, 0x2a, 0x39, 0x2f, 0x75, 0x73, 0x65, 0x72, 0x73, 0x2f, 0x6d, 0x65,
|
|
0x2f, 0x65, 0x78, 0x74, 0x65, 0x72, 0x6e, 0x61, 0x6c, 0x69, 0x64, 0x70, 0x73, 0x2f, 0x7b, 0x69,
|
|
0x64, 0x70, 0x5f, 0x63, 0x6f, 0x6e, 0x66, 0x69, 0x67, 0x5f, 0x69, 0x64, 0x7d, 0x2f, 0x7b, 0x65,
|
|
0x78, 0x74, 0x65, 0x72, 0x6e, 0x61, 0x6c, 0x5f, 0x75, 0x73, 0x65, 0x72, 0x5f, 0x69, 0x64, 0x7d,
|
|
0x82, 0xb5, 0x18, 0x0f, 0x0a, 0x0d, 0x61, 0x75, 0x74, 0x68, 0x65, 0x6e, 0x74, 0x69, 0x63, 0x61,
|
|
0x74, 0x65, 0x64, 0x12, 0x7f, 0x0a, 0x09, 0x41, 0x64, 0x64, 0x4d, 0x66, 0x61, 0x4f, 0x54, 0x50,
|
|
0x12, 0x16, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62,
|
|
0x75, 0x66, 0x2e, 0x45, 0x6d, 0x70, 0x74, 0x79, 0x1a, 0x28, 0x2e, 0x63, 0x61, 0x6f, 0x73, 0x2e,
|
|
0x7a, 0x69, 0x74, 0x61, 0x64, 0x65, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x61, 0x70, 0x69,
|
|
0x2e, 0x76, 0x31, 0x2e, 0x4d, 0x66, 0x61, 0x4f, 0x74, 0x70, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e,
|
|
0x73, 0x65, 0x22, 0x30, 0x82, 0xd3, 0xe4, 0x93, 0x02, 0x17, 0x22, 0x12, 0x2f, 0x75, 0x73, 0x65,
|
|
0x72, 0x73, 0x2f, 0x6d, 0x65, 0x2f, 0x6d, 0x66, 0x61, 0x73, 0x2f, 0x6f, 0x74, 0x70, 0x3a, 0x01,
|
|
0x2a, 0x82, 0xb5, 0x18, 0x0f, 0x0a, 0x0d, 0x61, 0x75, 0x74, 0x68, 0x65, 0x6e, 0x74, 0x69, 0x63,
|
|
0x61, 0x74, 0x65, 0x64, 0x12, 0x88, 0x01, 0x0a, 0x0c, 0x56, 0x65, 0x72, 0x69, 0x66, 0x79, 0x4d,
|
|
0x66, 0x61, 0x4f, 0x54, 0x50, 0x12, 0x26, 0x2e, 0x63, 0x61, 0x6f, 0x73, 0x2e, 0x7a, 0x69, 0x74,
|
|
0x61, 0x64, 0x65, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x61, 0x70, 0x69, 0x2e, 0x76, 0x31,
|
|
0x2e, 0x56, 0x65, 0x72, 0x69, 0x66, 0x79, 0x4d, 0x66, 0x61, 0x4f, 0x74, 0x70, 0x1a, 0x16, 0x2e,
|
|
0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e,
|
|
0x45, 0x6d, 0x70, 0x74, 0x79, 0x22, 0x38, 0x82, 0xd3, 0xe4, 0x93, 0x02, 0x1f, 0x1a, 0x1a, 0x2f,
|
|
0x75, 0x73, 0x65, 0x72, 0x73, 0x2f, 0x6d, 0x65, 0x2f, 0x6d, 0x66, 0x61, 0x73, 0x2f, 0x6f, 0x74,
|
|
0x70, 0x2f, 0x5f, 0x76, 0x65, 0x72, 0x69, 0x66, 0x79, 0x3a, 0x01, 0x2a, 0x82, 0xb5, 0x18, 0x0f,
|
|
0x0a, 0x0d, 0x61, 0x75, 0x74, 0x68, 0x65, 0x6e, 0x74, 0x69, 0x63, 0x61, 0x74, 0x65, 0x64, 0x12,
|
|
0x6d, 0x0a, 0x0c, 0x52, 0x65, 0x6d, 0x6f, 0x76, 0x65, 0x4d, 0x66, 0x61, 0x4f, 0x54, 0x50, 0x12,
|
|
0x16, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75,
|
|
0x66, 0x2e, 0x45, 0x6d, 0x70, 0x74, 0x79, 0x1a, 0x16, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65,
|
|
0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e, 0x45, 0x6d, 0x70, 0x74, 0x79, 0x22,
|
|
0x2d, 0x82, 0xd3, 0xe4, 0x93, 0x02, 0x14, 0x2a, 0x12, 0x2f, 0x75, 0x73, 0x65, 0x72, 0x73, 0x2f,
|
|
0x6d, 0x65, 0x2f, 0x6d, 0x66, 0x61, 0x73, 0x2f, 0x6f, 0x74, 0x70, 0x82, 0xb5, 0x18, 0x0f, 0x0a,
|
|
0x0d, 0x61, 0x75, 0x74, 0x68, 0x65, 0x6e, 0x74, 0x69, 0x63, 0x61, 0x74, 0x65, 0x64, 0x12, 0xae,
|
|
0x01, 0x0a, 0x11, 0x53, 0x65, 0x61, 0x72, 0x63, 0x68, 0x4d, 0x79, 0x55, 0x73, 0x65, 0x72, 0x47,
|
|
0x72, 0x61, 0x6e, 0x74, 0x12, 0x30, 0x2e, 0x63, 0x61, 0x6f, 0x73, 0x2e, 0x7a, 0x69, 0x74, 0x61,
|
|
0x64, 0x65, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x61, 0x70, 0x69, 0x2e, 0x76, 0x31, 0x2e,
|
|
0x55, 0x73, 0x65, 0x72, 0x47, 0x72, 0x61, 0x6e, 0x74, 0x53, 0x65, 0x61, 0x72, 0x63, 0x68, 0x52,
|
|
0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x1a, 0x31, 0x2e, 0x63, 0x61, 0x6f, 0x73, 0x2e, 0x7a, 0x69,
|
|
0x74, 0x61, 0x64, 0x65, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x61, 0x70, 0x69, 0x2e, 0x76,
|
|
0x31, 0x2e, 0x55, 0x73, 0x65, 0x72, 0x47, 0x72, 0x61, 0x6e, 0x74, 0x53, 0x65, 0x61, 0x72, 0x63,
|
|
0x68, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x22, 0x34, 0x82, 0xd3, 0xe4, 0x93, 0x02,
|
|
0x1b, 0x22, 0x16, 0x2f, 0x75, 0x73, 0x65, 0x72, 0x67, 0x72, 0x61, 0x6e, 0x74, 0x73, 0x2f, 0x6d,
|
|
0x65, 0x2f, 0x5f, 0x73, 0x65, 0x61, 0x72, 0x63, 0x68, 0x3a, 0x01, 0x2a, 0x82, 0xb5, 0x18, 0x0f,
|
|
0x0a, 0x0d, 0x61, 0x75, 0x74, 0x68, 0x65, 0x6e, 0x74, 0x69, 0x63, 0x61, 0x74, 0x65, 0x64, 0x12,
|
|
0xbb, 0x01, 0x0a, 0x13, 0x53, 0x65, 0x61, 0x72, 0x63, 0x68, 0x4d, 0x79, 0x50, 0x72, 0x6f, 0x6a,
|
|
0x65, 0x63, 0x74, 0x4f, 0x72, 0x67, 0x73, 0x12, 0x33, 0x2e, 0x63, 0x61, 0x6f, 0x73, 0x2e, 0x7a,
|
|
0x69, 0x74, 0x61, 0x64, 0x65, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x61, 0x70, 0x69, 0x2e,
|
|
0x76, 0x31, 0x2e, 0x4d, 0x79, 0x50, 0x72, 0x6f, 0x6a, 0x65, 0x63, 0x74, 0x4f, 0x72, 0x67, 0x53,
|
|
0x65, 0x61, 0x72, 0x63, 0x68, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x1a, 0x34, 0x2e, 0x63,
|
|
0x61, 0x6f, 0x73, 0x2e, 0x7a, 0x69, 0x74, 0x61, 0x64, 0x65, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68,
|
|
0x2e, 0x61, 0x70, 0x69, 0x2e, 0x76, 0x31, 0x2e, 0x4d, 0x79, 0x50, 0x72, 0x6f, 0x6a, 0x65, 0x63,
|
|
0x74, 0x4f, 0x72, 0x67, 0x53, 0x65, 0x61, 0x72, 0x63, 0x68, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e,
|
|
0x73, 0x65, 0x22, 0x39, 0x82, 0xd3, 0xe4, 0x93, 0x02, 0x20, 0x22, 0x1b, 0x2f, 0x67, 0x6c, 0x6f,
|
|
0x62, 0x61, 0x6c, 0x2f, 0x70, 0x72, 0x6f, 0x6a, 0x65, 0x63, 0x74, 0x6f, 0x72, 0x67, 0x73, 0x2f,
|
|
0x5f, 0x73, 0x65, 0x61, 0x72, 0x63, 0x68, 0x3a, 0x01, 0x2a, 0x82, 0xb5, 0x18, 0x0f, 0x0a, 0x0d,
|
|
0x61, 0x75, 0x74, 0x68, 0x65, 0x6e, 0x74, 0x69, 0x63, 0x61, 0x74, 0x65, 0x64, 0x12, 0x8e, 0x01,
|
|
0x0a, 0x17, 0x47, 0x65, 0x74, 0x4d, 0x79, 0x5a, 0x69, 0x74, 0x61, 0x64, 0x65, 0x6c, 0x50, 0x65,
|
|
0x72, 0x6d, 0x69, 0x73, 0x73, 0x69, 0x6f, 0x6e, 0x73, 0x12, 0x16, 0x2e, 0x67, 0x6f, 0x6f, 0x67,
|
|
0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e, 0x45, 0x6d, 0x70, 0x74,
|
|
0x79, 0x1a, 0x27, 0x2e, 0x63, 0x61, 0x6f, 0x73, 0x2e, 0x7a, 0x69, 0x74, 0x61, 0x64, 0x65, 0x6c,
|
|
0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x61, 0x70, 0x69, 0x2e, 0x76, 0x31, 0x2e, 0x4d, 0x79, 0x50,
|
|
0x65, 0x72, 0x6d, 0x69, 0x73, 0x73, 0x69, 0x6f, 0x6e, 0x73, 0x22, 0x32, 0x82, 0xd3, 0xe4, 0x93,
|
|
0x02, 0x19, 0x12, 0x17, 0x2f, 0x70, 0x65, 0x72, 0x6d, 0x69, 0x73, 0x73, 0x69, 0x6f, 0x6e, 0x73,
|
|
0x2f, 0x7a, 0x69, 0x74, 0x61, 0x64, 0x65, 0x6c, 0x2f, 0x6d, 0x65, 0x82, 0xb5, 0x18, 0x0f, 0x0a,
|
|
0x0d, 0x61, 0x75, 0x74, 0x68, 0x65, 0x6e, 0x74, 0x69, 0x63, 0x61, 0x74, 0x65, 0x64, 0x12, 0x86,
|
|
0x01, 0x0a, 0x17, 0x47, 0x65, 0x74, 0x4d, 0x79, 0x50, 0x72, 0x6f, 0x6a, 0x65, 0x63, 0x74, 0x50,
|
|
0x65, 0x72, 0x6d, 0x69, 0x73, 0x73, 0x69, 0x6f, 0x6e, 0x73, 0x12, 0x16, 0x2e, 0x67, 0x6f, 0x6f,
|
|
0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e, 0x45, 0x6d, 0x70,
|
|
0x74, 0x79, 0x1a, 0x27, 0x2e, 0x63, 0x61, 0x6f, 0x73, 0x2e, 0x7a, 0x69, 0x74, 0x61, 0x64, 0x65,
|
|
0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x61, 0x70, 0x69, 0x2e, 0x76, 0x31, 0x2e, 0x4d, 0x79,
|
|
0x50, 0x65, 0x72, 0x6d, 0x69, 0x73, 0x73, 0x69, 0x6f, 0x6e, 0x73, 0x22, 0x2a, 0x82, 0xd3, 0xe4,
|
|
0x93, 0x02, 0x11, 0x12, 0x0f, 0x2f, 0x70, 0x65, 0x72, 0x6d, 0x69, 0x73, 0x73, 0x69, 0x6f, 0x6e,
|
|
0x73, 0x2f, 0x6d, 0x65, 0x82, 0xb5, 0x18, 0x0f, 0x0a, 0x0d, 0x61, 0x75, 0x74, 0x68, 0x65, 0x6e,
|
|
0x74, 0x69, 0x63, 0x61, 0x74, 0x65, 0x64, 0x42, 0xb3, 0x01, 0x5a, 0x25, 0x67, 0x69, 0x74, 0x68,
|
|
0x75, 0x62, 0x2e, 0x63, 0x6f, 0x6d, 0x2f, 0x63, 0x61, 0x6f, 0x73, 0x2f, 0x7a, 0x69, 0x74, 0x61,
|
|
0x64, 0x65, 0x6c, 0x2f, 0x70, 0x6b, 0x67, 0x2f, 0x67, 0x72, 0x70, 0x63, 0x2f, 0x61, 0x75, 0x74,
|
|
0x68, 0x92, 0x41, 0x88, 0x01, 0x12, 0x3b, 0x0a, 0x08, 0x41, 0x75, 0x74, 0x68, 0x20, 0x41, 0x50,
|
|
0x49, 0x22, 0x2a, 0x12, 0x28, 0x68, 0x74, 0x74, 0x70, 0x73, 0x3a, 0x2f, 0x2f, 0x67, 0x69, 0x74,
|
|
0x68, 0x75, 0x62, 0x2e, 0x63, 0x6f, 0x6d, 0x2f, 0x63, 0x61, 0x6f, 0x73, 0x2f, 0x7a, 0x69, 0x74,
|
|
0x61, 0x64, 0x65, 0x6c, 0x2f, 0x70, 0x6b, 0x67, 0x2f, 0x61, 0x75, 0x74, 0x68, 0x32, 0x03, 0x30,
|
|
0x2e, 0x31, 0x2a, 0x01, 0x02, 0x32, 0x10, 0x61, 0x70, 0x70, 0x6c, 0x69, 0x63, 0x61, 0x74, 0x69,
|
|
0x6f, 0x6e, 0x2f, 0x6a, 0x73, 0x6f, 0x6e, 0x32, 0x10, 0x61, 0x70, 0x70, 0x6c, 0x69, 0x63, 0x61,
|
|
0x74, 0x69, 0x6f, 0x6e, 0x2f, 0x67, 0x72, 0x70, 0x63, 0x3a, 0x10, 0x61, 0x70, 0x70, 0x6c, 0x69,
|
|
0x63, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x2f, 0x6a, 0x73, 0x6f, 0x6e, 0x3a, 0x10, 0x61, 0x70, 0x70,
|
|
0x6c, 0x69, 0x63, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x2f, 0x67, 0x72, 0x70, 0x63, 0x62, 0x06, 0x70,
|
|
0x72, 0x6f, 0x74, 0x6f, 0x33,
|
|
}
|
|
|
|
var (
|
|
file_auth_proto_rawDescOnce sync.Once
|
|
file_auth_proto_rawDescData = file_auth_proto_rawDesc
|
|
)
|
|
|
|
func file_auth_proto_rawDescGZIP() []byte {
|
|
file_auth_proto_rawDescOnce.Do(func() {
|
|
file_auth_proto_rawDescData = protoimpl.X.CompressGZIP(file_auth_proto_rawDescData)
|
|
})
|
|
return file_auth_proto_rawDescData
|
|
}
|
|
|
|
var file_auth_proto_enumTypes = make([]protoimpl.EnumInfo, 9)
|
|
var file_auth_proto_msgTypes = make([]protoimpl.MessageInfo, 44)
|
|
var file_auth_proto_goTypes = []interface{}{
|
|
(UserSessionState)(0), // 0: caos.zitadel.auth.api.v1.UserSessionState
|
|
(MachineKeyType)(0), // 1: caos.zitadel.auth.api.v1.MachineKeyType
|
|
(UserState)(0), // 2: caos.zitadel.auth.api.v1.UserState
|
|
(Gender)(0), // 3: caos.zitadel.auth.api.v1.Gender
|
|
(MfaType)(0), // 4: caos.zitadel.auth.api.v1.MfaType
|
|
(MFAState)(0), // 5: caos.zitadel.auth.api.v1.MFAState
|
|
(UserGrantSearchKey)(0), // 6: caos.zitadel.auth.api.v1.UserGrantSearchKey
|
|
(MyProjectOrgSearchKey)(0), // 7: caos.zitadel.auth.api.v1.MyProjectOrgSearchKey
|
|
(SearchMethod)(0), // 8: caos.zitadel.auth.api.v1.SearchMethod
|
|
(*UserSessionViews)(nil), // 9: caos.zitadel.auth.api.v1.UserSessionViews
|
|
(*UserSessionView)(nil), // 10: caos.zitadel.auth.api.v1.UserSessionView
|
|
(*UserView)(nil), // 11: caos.zitadel.auth.api.v1.UserView
|
|
(*MachineView)(nil), // 12: caos.zitadel.auth.api.v1.MachineView
|
|
(*MachineKeyView)(nil), // 13: caos.zitadel.auth.api.v1.MachineKeyView
|
|
(*HumanView)(nil), // 14: caos.zitadel.auth.api.v1.HumanView
|
|
(*UserProfile)(nil), // 15: caos.zitadel.auth.api.v1.UserProfile
|
|
(*UserProfileView)(nil), // 16: caos.zitadel.auth.api.v1.UserProfileView
|
|
(*UpdateUserProfileRequest)(nil), // 17: caos.zitadel.auth.api.v1.UpdateUserProfileRequest
|
|
(*ChangeUserNameRequest)(nil), // 18: caos.zitadel.auth.api.v1.ChangeUserNameRequest
|
|
(*UserEmail)(nil), // 19: caos.zitadel.auth.api.v1.UserEmail
|
|
(*UserEmailView)(nil), // 20: caos.zitadel.auth.api.v1.UserEmailView
|
|
(*VerifyMyUserEmailRequest)(nil), // 21: caos.zitadel.auth.api.v1.VerifyMyUserEmailRequest
|
|
(*UpdateUserEmailRequest)(nil), // 22: caos.zitadel.auth.api.v1.UpdateUserEmailRequest
|
|
(*UserPhone)(nil), // 23: caos.zitadel.auth.api.v1.UserPhone
|
|
(*UserPhoneView)(nil), // 24: caos.zitadel.auth.api.v1.UserPhoneView
|
|
(*UpdateUserPhoneRequest)(nil), // 25: caos.zitadel.auth.api.v1.UpdateUserPhoneRequest
|
|
(*VerifyUserPhoneRequest)(nil), // 26: caos.zitadel.auth.api.v1.VerifyUserPhoneRequest
|
|
(*UserAddress)(nil), // 27: caos.zitadel.auth.api.v1.UserAddress
|
|
(*UserAddressView)(nil), // 28: caos.zitadel.auth.api.v1.UserAddressView
|
|
(*UpdateUserAddressRequest)(nil), // 29: caos.zitadel.auth.api.v1.UpdateUserAddressRequest
|
|
(*PasswordChange)(nil), // 30: caos.zitadel.auth.api.v1.PasswordChange
|
|
(*VerifyMfaOtp)(nil), // 31: caos.zitadel.auth.api.v1.VerifyMfaOtp
|
|
(*MultiFactors)(nil), // 32: caos.zitadel.auth.api.v1.MultiFactors
|
|
(*MultiFactor)(nil), // 33: caos.zitadel.auth.api.v1.MultiFactor
|
|
(*MfaOtpResponse)(nil), // 34: caos.zitadel.auth.api.v1.MfaOtpResponse
|
|
(*UserGrantSearchRequest)(nil), // 35: caos.zitadel.auth.api.v1.UserGrantSearchRequest
|
|
(*UserGrantSearchQuery)(nil), // 36: caos.zitadel.auth.api.v1.UserGrantSearchQuery
|
|
(*UserGrantSearchResponse)(nil), // 37: caos.zitadel.auth.api.v1.UserGrantSearchResponse
|
|
(*UserGrantView)(nil), // 38: caos.zitadel.auth.api.v1.UserGrantView
|
|
(*MyProjectOrgSearchRequest)(nil), // 39: caos.zitadel.auth.api.v1.MyProjectOrgSearchRequest
|
|
(*MyProjectOrgSearchQuery)(nil), // 40: caos.zitadel.auth.api.v1.MyProjectOrgSearchQuery
|
|
(*MyProjectOrgSearchResponse)(nil), // 41: caos.zitadel.auth.api.v1.MyProjectOrgSearchResponse
|
|
(*Org)(nil), // 42: caos.zitadel.auth.api.v1.Org
|
|
(*MyPermissions)(nil), // 43: caos.zitadel.auth.api.v1.MyPermissions
|
|
(*ChangesRequest)(nil), // 44: caos.zitadel.auth.api.v1.ChangesRequest
|
|
(*Changes)(nil), // 45: caos.zitadel.auth.api.v1.Changes
|
|
(*Change)(nil), // 46: caos.zitadel.auth.api.v1.Change
|
|
(*PasswordComplexityPolicy)(nil), // 47: caos.zitadel.auth.api.v1.PasswordComplexityPolicy
|
|
(*ExternalIDPResponse)(nil), // 48: caos.zitadel.auth.api.v1.ExternalIDPResponse
|
|
(*ExternalIDPRemoveRequest)(nil), // 49: caos.zitadel.auth.api.v1.ExternalIDPRemoveRequest
|
|
(*ExternalIDPSearchRequest)(nil), // 50: caos.zitadel.auth.api.v1.ExternalIDPSearchRequest
|
|
(*ExternalIDPSearchResponse)(nil), // 51: caos.zitadel.auth.api.v1.ExternalIDPSearchResponse
|
|
(*ExternalIDPView)(nil), // 52: caos.zitadel.auth.api.v1.ExternalIDPView
|
|
(*timestamp.Timestamp)(nil), // 53: google.protobuf.Timestamp
|
|
(*message.LocalizedMessage)(nil), // 54: caos.zitadel.api.v1.LocalizedMessage
|
|
(*_struct.Struct)(nil), // 55: google.protobuf.Struct
|
|
(*empty.Empty)(nil), // 56: google.protobuf.Empty
|
|
}
|
|
var file_auth_proto_depIdxs = []int32{
|
|
10, // 0: caos.zitadel.auth.api.v1.UserSessionViews.user_sessions:type_name -> caos.zitadel.auth.api.v1.UserSessionView
|
|
0, // 1: caos.zitadel.auth.api.v1.UserSessionView.auth_state:type_name -> caos.zitadel.auth.api.v1.UserSessionState
|
|
2, // 2: caos.zitadel.auth.api.v1.UserView.state:type_name -> caos.zitadel.auth.api.v1.UserState
|
|
53, // 3: caos.zitadel.auth.api.v1.UserView.creation_date:type_name -> google.protobuf.Timestamp
|
|
53, // 4: caos.zitadel.auth.api.v1.UserView.change_date:type_name -> google.protobuf.Timestamp
|
|
53, // 5: caos.zitadel.auth.api.v1.UserView.last_login:type_name -> google.protobuf.Timestamp
|
|
14, // 6: caos.zitadel.auth.api.v1.UserView.human:type_name -> caos.zitadel.auth.api.v1.HumanView
|
|
12, // 7: caos.zitadel.auth.api.v1.UserView.machine:type_name -> caos.zitadel.auth.api.v1.MachineView
|
|
53, // 8: caos.zitadel.auth.api.v1.MachineView.last_key_added:type_name -> google.protobuf.Timestamp
|
|
1, // 9: caos.zitadel.auth.api.v1.MachineKeyView.type:type_name -> caos.zitadel.auth.api.v1.MachineKeyType
|
|
53, // 10: caos.zitadel.auth.api.v1.MachineKeyView.creation_date:type_name -> google.protobuf.Timestamp
|
|
53, // 11: caos.zitadel.auth.api.v1.MachineKeyView.expiration_date:type_name -> google.protobuf.Timestamp
|
|
53, // 12: caos.zitadel.auth.api.v1.HumanView.password_changed:type_name -> google.protobuf.Timestamp
|
|
3, // 13: caos.zitadel.auth.api.v1.HumanView.gender:type_name -> caos.zitadel.auth.api.v1.Gender
|
|
3, // 14: caos.zitadel.auth.api.v1.UserProfile.gender:type_name -> caos.zitadel.auth.api.v1.Gender
|
|
53, // 15: caos.zitadel.auth.api.v1.UserProfile.creation_date:type_name -> google.protobuf.Timestamp
|
|
53, // 16: caos.zitadel.auth.api.v1.UserProfile.change_date:type_name -> google.protobuf.Timestamp
|
|
3, // 17: caos.zitadel.auth.api.v1.UserProfileView.gender:type_name -> caos.zitadel.auth.api.v1.Gender
|
|
53, // 18: caos.zitadel.auth.api.v1.UserProfileView.creation_date:type_name -> google.protobuf.Timestamp
|
|
53, // 19: caos.zitadel.auth.api.v1.UserProfileView.change_date:type_name -> google.protobuf.Timestamp
|
|
3, // 20: caos.zitadel.auth.api.v1.UpdateUserProfileRequest.gender:type_name -> caos.zitadel.auth.api.v1.Gender
|
|
53, // 21: caos.zitadel.auth.api.v1.UserEmail.creation_date:type_name -> google.protobuf.Timestamp
|
|
53, // 22: caos.zitadel.auth.api.v1.UserEmail.change_date:type_name -> google.protobuf.Timestamp
|
|
53, // 23: caos.zitadel.auth.api.v1.UserEmailView.creation_date:type_name -> google.protobuf.Timestamp
|
|
53, // 24: caos.zitadel.auth.api.v1.UserEmailView.change_date:type_name -> google.protobuf.Timestamp
|
|
53, // 25: caos.zitadel.auth.api.v1.UserPhone.creation_date:type_name -> google.protobuf.Timestamp
|
|
53, // 26: caos.zitadel.auth.api.v1.UserPhone.change_date:type_name -> google.protobuf.Timestamp
|
|
53, // 27: caos.zitadel.auth.api.v1.UserPhoneView.creation_date:type_name -> google.protobuf.Timestamp
|
|
53, // 28: caos.zitadel.auth.api.v1.UserPhoneView.change_date:type_name -> google.protobuf.Timestamp
|
|
53, // 29: caos.zitadel.auth.api.v1.UserAddress.creation_date:type_name -> google.protobuf.Timestamp
|
|
53, // 30: caos.zitadel.auth.api.v1.UserAddress.change_date:type_name -> google.protobuf.Timestamp
|
|
53, // 31: caos.zitadel.auth.api.v1.UserAddressView.creation_date:type_name -> google.protobuf.Timestamp
|
|
53, // 32: caos.zitadel.auth.api.v1.UserAddressView.change_date:type_name -> google.protobuf.Timestamp
|
|
33, // 33: caos.zitadel.auth.api.v1.MultiFactors.mfas:type_name -> caos.zitadel.auth.api.v1.MultiFactor
|
|
4, // 34: caos.zitadel.auth.api.v1.MultiFactor.type:type_name -> caos.zitadel.auth.api.v1.MfaType
|
|
5, // 35: caos.zitadel.auth.api.v1.MultiFactor.state:type_name -> caos.zitadel.auth.api.v1.MFAState
|
|
5, // 36: caos.zitadel.auth.api.v1.MfaOtpResponse.state:type_name -> caos.zitadel.auth.api.v1.MFAState
|
|
6, // 37: caos.zitadel.auth.api.v1.UserGrantSearchRequest.sorting_column:type_name -> caos.zitadel.auth.api.v1.UserGrantSearchKey
|
|
36, // 38: caos.zitadel.auth.api.v1.UserGrantSearchRequest.queries:type_name -> caos.zitadel.auth.api.v1.UserGrantSearchQuery
|
|
6, // 39: caos.zitadel.auth.api.v1.UserGrantSearchQuery.key:type_name -> caos.zitadel.auth.api.v1.UserGrantSearchKey
|
|
8, // 40: caos.zitadel.auth.api.v1.UserGrantSearchQuery.method:type_name -> caos.zitadel.auth.api.v1.SearchMethod
|
|
38, // 41: caos.zitadel.auth.api.v1.UserGrantSearchResponse.result:type_name -> caos.zitadel.auth.api.v1.UserGrantView
|
|
53, // 42: caos.zitadel.auth.api.v1.UserGrantSearchResponse.view_timestamp:type_name -> google.protobuf.Timestamp
|
|
40, // 43: caos.zitadel.auth.api.v1.MyProjectOrgSearchRequest.queries:type_name -> caos.zitadel.auth.api.v1.MyProjectOrgSearchQuery
|
|
7, // 44: caos.zitadel.auth.api.v1.MyProjectOrgSearchQuery.key:type_name -> caos.zitadel.auth.api.v1.MyProjectOrgSearchKey
|
|
8, // 45: caos.zitadel.auth.api.v1.MyProjectOrgSearchQuery.method:type_name -> caos.zitadel.auth.api.v1.SearchMethod
|
|
42, // 46: caos.zitadel.auth.api.v1.MyProjectOrgSearchResponse.result:type_name -> caos.zitadel.auth.api.v1.Org
|
|
46, // 47: caos.zitadel.auth.api.v1.Changes.changes:type_name -> caos.zitadel.auth.api.v1.Change
|
|
53, // 48: caos.zitadel.auth.api.v1.Change.change_date:type_name -> google.protobuf.Timestamp
|
|
54, // 49: caos.zitadel.auth.api.v1.Change.event_type:type_name -> caos.zitadel.api.v1.LocalizedMessage
|
|
55, // 50: caos.zitadel.auth.api.v1.Change.data:type_name -> google.protobuf.Struct
|
|
53, // 51: caos.zitadel.auth.api.v1.PasswordComplexityPolicy.creation_date:type_name -> google.protobuf.Timestamp
|
|
53, // 52: caos.zitadel.auth.api.v1.PasswordComplexityPolicy.change_date:type_name -> google.protobuf.Timestamp
|
|
52, // 53: caos.zitadel.auth.api.v1.ExternalIDPSearchResponse.result:type_name -> caos.zitadel.auth.api.v1.ExternalIDPView
|
|
53, // 54: caos.zitadel.auth.api.v1.ExternalIDPSearchResponse.view_timestamp:type_name -> google.protobuf.Timestamp
|
|
53, // 55: caos.zitadel.auth.api.v1.ExternalIDPView.creation_date:type_name -> google.protobuf.Timestamp
|
|
53, // 56: caos.zitadel.auth.api.v1.ExternalIDPView.change_date:type_name -> google.protobuf.Timestamp
|
|
56, // 57: caos.zitadel.auth.api.v1.AuthService.Healthz:input_type -> google.protobuf.Empty
|
|
56, // 58: caos.zitadel.auth.api.v1.AuthService.Ready:input_type -> google.protobuf.Empty
|
|
56, // 59: caos.zitadel.auth.api.v1.AuthService.Validate:input_type -> google.protobuf.Empty
|
|
56, // 60: caos.zitadel.auth.api.v1.AuthService.GetMyUserSessions:input_type -> google.protobuf.Empty
|
|
56, // 61: caos.zitadel.auth.api.v1.AuthService.GetMyUser:input_type -> google.protobuf.Empty
|
|
56, // 62: caos.zitadel.auth.api.v1.AuthService.GetMyUserProfile:input_type -> google.protobuf.Empty
|
|
17, // 63: caos.zitadel.auth.api.v1.AuthService.UpdateMyUserProfile:input_type -> caos.zitadel.auth.api.v1.UpdateUserProfileRequest
|
|
18, // 64: caos.zitadel.auth.api.v1.AuthService.ChangeMyUserName:input_type -> caos.zitadel.auth.api.v1.ChangeUserNameRequest
|
|
56, // 65: caos.zitadel.auth.api.v1.AuthService.GetMyUserEmail:input_type -> google.protobuf.Empty
|
|
22, // 66: caos.zitadel.auth.api.v1.AuthService.ChangeMyUserEmail:input_type -> caos.zitadel.auth.api.v1.UpdateUserEmailRequest
|
|
21, // 67: caos.zitadel.auth.api.v1.AuthService.VerifyMyUserEmail:input_type -> caos.zitadel.auth.api.v1.VerifyMyUserEmailRequest
|
|
56, // 68: caos.zitadel.auth.api.v1.AuthService.ResendMyEmailVerificationMail:input_type -> google.protobuf.Empty
|
|
56, // 69: caos.zitadel.auth.api.v1.AuthService.GetMyUserPhone:input_type -> google.protobuf.Empty
|
|
25, // 70: caos.zitadel.auth.api.v1.AuthService.ChangeMyUserPhone:input_type -> caos.zitadel.auth.api.v1.UpdateUserPhoneRequest
|
|
56, // 71: caos.zitadel.auth.api.v1.AuthService.RemoveMyUserPhone:input_type -> google.protobuf.Empty
|
|
26, // 72: caos.zitadel.auth.api.v1.AuthService.VerifyMyUserPhone:input_type -> caos.zitadel.auth.api.v1.VerifyUserPhoneRequest
|
|
56, // 73: caos.zitadel.auth.api.v1.AuthService.ResendMyPhoneVerificationCode:input_type -> google.protobuf.Empty
|
|
56, // 74: caos.zitadel.auth.api.v1.AuthService.GetMyUserAddress:input_type -> google.protobuf.Empty
|
|
44, // 75: caos.zitadel.auth.api.v1.AuthService.GetMyUserChanges:input_type -> caos.zitadel.auth.api.v1.ChangesRequest
|
|
29, // 76: caos.zitadel.auth.api.v1.AuthService.UpdateMyUserAddress:input_type -> caos.zitadel.auth.api.v1.UpdateUserAddressRequest
|
|
56, // 77: caos.zitadel.auth.api.v1.AuthService.GetMyMfas:input_type -> google.protobuf.Empty
|
|
30, // 78: caos.zitadel.auth.api.v1.AuthService.ChangeMyPassword:input_type -> caos.zitadel.auth.api.v1.PasswordChange
|
|
56, // 79: caos.zitadel.auth.api.v1.AuthService.GetMyPasswordComplexityPolicy:input_type -> google.protobuf.Empty
|
|
50, // 80: caos.zitadel.auth.api.v1.AuthService.SearchMyExternalIDPs:input_type -> caos.zitadel.auth.api.v1.ExternalIDPSearchRequest
|
|
49, // 81: caos.zitadel.auth.api.v1.AuthService.RemoveMyExternalIDP:input_type -> caos.zitadel.auth.api.v1.ExternalIDPRemoveRequest
|
|
56, // 82: caos.zitadel.auth.api.v1.AuthService.AddMfaOTP:input_type -> google.protobuf.Empty
|
|
31, // 83: caos.zitadel.auth.api.v1.AuthService.VerifyMfaOTP:input_type -> caos.zitadel.auth.api.v1.VerifyMfaOtp
|
|
56, // 84: caos.zitadel.auth.api.v1.AuthService.RemoveMfaOTP:input_type -> google.protobuf.Empty
|
|
35, // 85: caos.zitadel.auth.api.v1.AuthService.SearchMyUserGrant:input_type -> caos.zitadel.auth.api.v1.UserGrantSearchRequest
|
|
39, // 86: caos.zitadel.auth.api.v1.AuthService.SearchMyProjectOrgs:input_type -> caos.zitadel.auth.api.v1.MyProjectOrgSearchRequest
|
|
56, // 87: caos.zitadel.auth.api.v1.AuthService.GetMyZitadelPermissions:input_type -> google.protobuf.Empty
|
|
56, // 88: caos.zitadel.auth.api.v1.AuthService.GetMyProjectPermissions:input_type -> google.protobuf.Empty
|
|
56, // 89: caos.zitadel.auth.api.v1.AuthService.Healthz:output_type -> google.protobuf.Empty
|
|
56, // 90: caos.zitadel.auth.api.v1.AuthService.Ready:output_type -> google.protobuf.Empty
|
|
55, // 91: caos.zitadel.auth.api.v1.AuthService.Validate:output_type -> google.protobuf.Struct
|
|
9, // 92: caos.zitadel.auth.api.v1.AuthService.GetMyUserSessions:output_type -> caos.zitadel.auth.api.v1.UserSessionViews
|
|
11, // 93: caos.zitadel.auth.api.v1.AuthService.GetMyUser:output_type -> caos.zitadel.auth.api.v1.UserView
|
|
16, // 94: caos.zitadel.auth.api.v1.AuthService.GetMyUserProfile:output_type -> caos.zitadel.auth.api.v1.UserProfileView
|
|
15, // 95: caos.zitadel.auth.api.v1.AuthService.UpdateMyUserProfile:output_type -> caos.zitadel.auth.api.v1.UserProfile
|
|
56, // 96: caos.zitadel.auth.api.v1.AuthService.ChangeMyUserName:output_type -> google.protobuf.Empty
|
|
20, // 97: caos.zitadel.auth.api.v1.AuthService.GetMyUserEmail:output_type -> caos.zitadel.auth.api.v1.UserEmailView
|
|
19, // 98: caos.zitadel.auth.api.v1.AuthService.ChangeMyUserEmail:output_type -> caos.zitadel.auth.api.v1.UserEmail
|
|
56, // 99: caos.zitadel.auth.api.v1.AuthService.VerifyMyUserEmail:output_type -> google.protobuf.Empty
|
|
56, // 100: caos.zitadel.auth.api.v1.AuthService.ResendMyEmailVerificationMail:output_type -> google.protobuf.Empty
|
|
24, // 101: caos.zitadel.auth.api.v1.AuthService.GetMyUserPhone:output_type -> caos.zitadel.auth.api.v1.UserPhoneView
|
|
23, // 102: caos.zitadel.auth.api.v1.AuthService.ChangeMyUserPhone:output_type -> caos.zitadel.auth.api.v1.UserPhone
|
|
56, // 103: caos.zitadel.auth.api.v1.AuthService.RemoveMyUserPhone:output_type -> google.protobuf.Empty
|
|
56, // 104: caos.zitadel.auth.api.v1.AuthService.VerifyMyUserPhone:output_type -> google.protobuf.Empty
|
|
56, // 105: caos.zitadel.auth.api.v1.AuthService.ResendMyPhoneVerificationCode:output_type -> google.protobuf.Empty
|
|
28, // 106: caos.zitadel.auth.api.v1.AuthService.GetMyUserAddress:output_type -> caos.zitadel.auth.api.v1.UserAddressView
|
|
45, // 107: caos.zitadel.auth.api.v1.AuthService.GetMyUserChanges:output_type -> caos.zitadel.auth.api.v1.Changes
|
|
27, // 108: caos.zitadel.auth.api.v1.AuthService.UpdateMyUserAddress:output_type -> caos.zitadel.auth.api.v1.UserAddress
|
|
32, // 109: caos.zitadel.auth.api.v1.AuthService.GetMyMfas:output_type -> caos.zitadel.auth.api.v1.MultiFactors
|
|
56, // 110: caos.zitadel.auth.api.v1.AuthService.ChangeMyPassword:output_type -> google.protobuf.Empty
|
|
47, // 111: caos.zitadel.auth.api.v1.AuthService.GetMyPasswordComplexityPolicy:output_type -> caos.zitadel.auth.api.v1.PasswordComplexityPolicy
|
|
51, // 112: caos.zitadel.auth.api.v1.AuthService.SearchMyExternalIDPs:output_type -> caos.zitadel.auth.api.v1.ExternalIDPSearchResponse
|
|
56, // 113: caos.zitadel.auth.api.v1.AuthService.RemoveMyExternalIDP:output_type -> google.protobuf.Empty
|
|
34, // 114: caos.zitadel.auth.api.v1.AuthService.AddMfaOTP:output_type -> caos.zitadel.auth.api.v1.MfaOtpResponse
|
|
56, // 115: caos.zitadel.auth.api.v1.AuthService.VerifyMfaOTP:output_type -> google.protobuf.Empty
|
|
56, // 116: caos.zitadel.auth.api.v1.AuthService.RemoveMfaOTP:output_type -> google.protobuf.Empty
|
|
37, // 117: caos.zitadel.auth.api.v1.AuthService.SearchMyUserGrant:output_type -> caos.zitadel.auth.api.v1.UserGrantSearchResponse
|
|
41, // 118: caos.zitadel.auth.api.v1.AuthService.SearchMyProjectOrgs:output_type -> caos.zitadel.auth.api.v1.MyProjectOrgSearchResponse
|
|
43, // 119: caos.zitadel.auth.api.v1.AuthService.GetMyZitadelPermissions:output_type -> caos.zitadel.auth.api.v1.MyPermissions
|
|
43, // 120: caos.zitadel.auth.api.v1.AuthService.GetMyProjectPermissions:output_type -> caos.zitadel.auth.api.v1.MyPermissions
|
|
89, // [89:121] is the sub-list for method output_type
|
|
57, // [57:89] is the sub-list for method input_type
|
|
57, // [57:57] is the sub-list for extension type_name
|
|
57, // [57:57] is the sub-list for extension extendee
|
|
0, // [0:57] is the sub-list for field type_name
|
|
}
|
|
|
|
func init() { file_auth_proto_init() }
|
|
func file_auth_proto_init() {
|
|
if File_auth_proto != nil {
|
|
return
|
|
}
|
|
if !protoimpl.UnsafeEnabled {
|
|
file_auth_proto_msgTypes[0].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*UserSessionViews); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_proto_msgTypes[1].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*UserSessionView); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_proto_msgTypes[2].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*UserView); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_proto_msgTypes[3].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*MachineView); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_proto_msgTypes[4].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*MachineKeyView); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_proto_msgTypes[5].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*HumanView); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_proto_msgTypes[6].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*UserProfile); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_proto_msgTypes[7].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*UserProfileView); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_proto_msgTypes[8].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*UpdateUserProfileRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_proto_msgTypes[9].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*ChangeUserNameRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_proto_msgTypes[10].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*UserEmail); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_proto_msgTypes[11].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*UserEmailView); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_proto_msgTypes[12].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*VerifyMyUserEmailRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_proto_msgTypes[13].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*UpdateUserEmailRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_proto_msgTypes[14].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*UserPhone); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_proto_msgTypes[15].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*UserPhoneView); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_proto_msgTypes[16].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*UpdateUserPhoneRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_proto_msgTypes[17].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*VerifyUserPhoneRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_proto_msgTypes[18].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*UserAddress); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_proto_msgTypes[19].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*UserAddressView); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_proto_msgTypes[20].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*UpdateUserAddressRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_proto_msgTypes[21].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*PasswordChange); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_proto_msgTypes[22].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*VerifyMfaOtp); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_proto_msgTypes[23].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*MultiFactors); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_proto_msgTypes[24].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*MultiFactor); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_proto_msgTypes[25].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*MfaOtpResponse); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_proto_msgTypes[26].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*UserGrantSearchRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_proto_msgTypes[27].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*UserGrantSearchQuery); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_proto_msgTypes[28].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*UserGrantSearchResponse); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_proto_msgTypes[29].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*UserGrantView); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_proto_msgTypes[30].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*MyProjectOrgSearchRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_proto_msgTypes[31].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*MyProjectOrgSearchQuery); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_proto_msgTypes[32].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*MyProjectOrgSearchResponse); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_proto_msgTypes[33].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*Org); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_proto_msgTypes[34].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*MyPermissions); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_proto_msgTypes[35].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*ChangesRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_proto_msgTypes[36].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*Changes); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_proto_msgTypes[37].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*Change); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_proto_msgTypes[38].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*PasswordComplexityPolicy); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_proto_msgTypes[39].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*ExternalIDPResponse); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_proto_msgTypes[40].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*ExternalIDPRemoveRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_proto_msgTypes[41].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*ExternalIDPSearchRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_proto_msgTypes[42].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*ExternalIDPSearchResponse); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_proto_msgTypes[43].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*ExternalIDPView); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
}
|
|
file_auth_proto_msgTypes[2].OneofWrappers = []interface{}{
|
|
(*UserView_Human)(nil),
|
|
(*UserView_Machine)(nil),
|
|
}
|
|
type x struct{}
|
|
out := protoimpl.TypeBuilder{
|
|
File: protoimpl.DescBuilder{
|
|
GoPackagePath: reflect.TypeOf(x{}).PkgPath(),
|
|
RawDescriptor: file_auth_proto_rawDesc,
|
|
NumEnums: 9,
|
|
NumMessages: 44,
|
|
NumExtensions: 0,
|
|
NumServices: 1,
|
|
},
|
|
GoTypes: file_auth_proto_goTypes,
|
|
DependencyIndexes: file_auth_proto_depIdxs,
|
|
EnumInfos: file_auth_proto_enumTypes,
|
|
MessageInfos: file_auth_proto_msgTypes,
|
|
}.Build()
|
|
File_auth_proto = out.File
|
|
file_auth_proto_rawDesc = nil
|
|
file_auth_proto_goTypes = nil
|
|
file_auth_proto_depIdxs = nil
|
|
}
|
|
|
|
// Reference imports to suppress errors if they are not otherwise used.
|
|
var _ context.Context
|
|
var _ grpc.ClientConnInterface
|
|
|
|
// This is a compile-time assertion to ensure that this generated file
|
|
// is compatible with the grpc package it is being compiled against.
|
|
const _ = grpc.SupportPackageIsVersion6
|
|
|
|
// AuthServiceClient is the client API for AuthService service.
|
|
//
|
|
// For semantics around ctx use and closing/ending streaming RPCs, please refer to https://godoc.org/google.golang.org/grpc#ClientConn.NewStream.
|
|
type AuthServiceClient interface {
|
|
// Readiness
|
|
Healthz(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*empty.Empty, error)
|
|
Ready(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*empty.Empty, error)
|
|
Validate(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*_struct.Struct, error)
|
|
// Authorization
|
|
GetMyUserSessions(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*UserSessionViews, error)
|
|
//User
|
|
GetMyUser(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*UserView, error)
|
|
GetMyUserProfile(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*UserProfileView, error)
|
|
UpdateMyUserProfile(ctx context.Context, in *UpdateUserProfileRequest, opts ...grpc.CallOption) (*UserProfile, error)
|
|
ChangeMyUserName(ctx context.Context, in *ChangeUserNameRequest, opts ...grpc.CallOption) (*empty.Empty, error)
|
|
GetMyUserEmail(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*UserEmailView, error)
|
|
ChangeMyUserEmail(ctx context.Context, in *UpdateUserEmailRequest, opts ...grpc.CallOption) (*UserEmail, error)
|
|
VerifyMyUserEmail(ctx context.Context, in *VerifyMyUserEmailRequest, opts ...grpc.CallOption) (*empty.Empty, error)
|
|
ResendMyEmailVerificationMail(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*empty.Empty, error)
|
|
GetMyUserPhone(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*UserPhoneView, error)
|
|
ChangeMyUserPhone(ctx context.Context, in *UpdateUserPhoneRequest, opts ...grpc.CallOption) (*UserPhone, error)
|
|
RemoveMyUserPhone(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*empty.Empty, error)
|
|
VerifyMyUserPhone(ctx context.Context, in *VerifyUserPhoneRequest, opts ...grpc.CallOption) (*empty.Empty, error)
|
|
ResendMyPhoneVerificationCode(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*empty.Empty, error)
|
|
GetMyUserAddress(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*UserAddressView, error)
|
|
GetMyUserChanges(ctx context.Context, in *ChangesRequest, opts ...grpc.CallOption) (*Changes, error)
|
|
UpdateMyUserAddress(ctx context.Context, in *UpdateUserAddressRequest, opts ...grpc.CallOption) (*UserAddress, error)
|
|
GetMyMfas(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*MultiFactors, error)
|
|
//Password
|
|
ChangeMyPassword(ctx context.Context, in *PasswordChange, opts ...grpc.CallOption) (*empty.Empty, error)
|
|
GetMyPasswordComplexityPolicy(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*PasswordComplexityPolicy, error)
|
|
//ExternalIDP
|
|
SearchMyExternalIDPs(ctx context.Context, in *ExternalIDPSearchRequest, opts ...grpc.CallOption) (*ExternalIDPSearchResponse, error)
|
|
RemoveMyExternalIDP(ctx context.Context, in *ExternalIDPRemoveRequest, opts ...grpc.CallOption) (*empty.Empty, error)
|
|
// MFA
|
|
AddMfaOTP(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*MfaOtpResponse, error)
|
|
VerifyMfaOTP(ctx context.Context, in *VerifyMfaOtp, opts ...grpc.CallOption) (*empty.Empty, error)
|
|
RemoveMfaOTP(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*empty.Empty, error)
|
|
SearchMyUserGrant(ctx context.Context, in *UserGrantSearchRequest, opts ...grpc.CallOption) (*UserGrantSearchResponse, error)
|
|
SearchMyProjectOrgs(ctx context.Context, in *MyProjectOrgSearchRequest, opts ...grpc.CallOption) (*MyProjectOrgSearchResponse, error)
|
|
//Permission
|
|
GetMyZitadelPermissions(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*MyPermissions, error)
|
|
GetMyProjectPermissions(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*MyPermissions, error)
|
|
}
|
|
|
|
type authServiceClient struct {
|
|
cc grpc.ClientConnInterface
|
|
}
|
|
|
|
func NewAuthServiceClient(cc grpc.ClientConnInterface) AuthServiceClient {
|
|
return &authServiceClient{cc}
|
|
}
|
|
|
|
func (c *authServiceClient) Healthz(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*empty.Empty, error) {
|
|
out := new(empty.Empty)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/Healthz", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) Ready(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*empty.Empty, error) {
|
|
out := new(empty.Empty)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/Ready", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) Validate(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*_struct.Struct, error) {
|
|
out := new(_struct.Struct)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/Validate", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) GetMyUserSessions(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*UserSessionViews, error) {
|
|
out := new(UserSessionViews)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/GetMyUserSessions", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) GetMyUser(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*UserView, error) {
|
|
out := new(UserView)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/GetMyUser", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) GetMyUserProfile(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*UserProfileView, error) {
|
|
out := new(UserProfileView)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/GetMyUserProfile", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) UpdateMyUserProfile(ctx context.Context, in *UpdateUserProfileRequest, opts ...grpc.CallOption) (*UserProfile, error) {
|
|
out := new(UserProfile)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/UpdateMyUserProfile", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) ChangeMyUserName(ctx context.Context, in *ChangeUserNameRequest, opts ...grpc.CallOption) (*empty.Empty, error) {
|
|
out := new(empty.Empty)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/ChangeMyUserName", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) GetMyUserEmail(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*UserEmailView, error) {
|
|
out := new(UserEmailView)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/GetMyUserEmail", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) ChangeMyUserEmail(ctx context.Context, in *UpdateUserEmailRequest, opts ...grpc.CallOption) (*UserEmail, error) {
|
|
out := new(UserEmail)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/ChangeMyUserEmail", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) VerifyMyUserEmail(ctx context.Context, in *VerifyMyUserEmailRequest, opts ...grpc.CallOption) (*empty.Empty, error) {
|
|
out := new(empty.Empty)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/VerifyMyUserEmail", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) ResendMyEmailVerificationMail(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*empty.Empty, error) {
|
|
out := new(empty.Empty)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/ResendMyEmailVerificationMail", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) GetMyUserPhone(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*UserPhoneView, error) {
|
|
out := new(UserPhoneView)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/GetMyUserPhone", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) ChangeMyUserPhone(ctx context.Context, in *UpdateUserPhoneRequest, opts ...grpc.CallOption) (*UserPhone, error) {
|
|
out := new(UserPhone)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/ChangeMyUserPhone", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) RemoveMyUserPhone(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*empty.Empty, error) {
|
|
out := new(empty.Empty)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/RemoveMyUserPhone", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) VerifyMyUserPhone(ctx context.Context, in *VerifyUserPhoneRequest, opts ...grpc.CallOption) (*empty.Empty, error) {
|
|
out := new(empty.Empty)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/VerifyMyUserPhone", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) ResendMyPhoneVerificationCode(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*empty.Empty, error) {
|
|
out := new(empty.Empty)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/ResendMyPhoneVerificationCode", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) GetMyUserAddress(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*UserAddressView, error) {
|
|
out := new(UserAddressView)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/GetMyUserAddress", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) GetMyUserChanges(ctx context.Context, in *ChangesRequest, opts ...grpc.CallOption) (*Changes, error) {
|
|
out := new(Changes)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/GetMyUserChanges", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) UpdateMyUserAddress(ctx context.Context, in *UpdateUserAddressRequest, opts ...grpc.CallOption) (*UserAddress, error) {
|
|
out := new(UserAddress)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/UpdateMyUserAddress", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) GetMyMfas(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*MultiFactors, error) {
|
|
out := new(MultiFactors)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/GetMyMfas", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) ChangeMyPassword(ctx context.Context, in *PasswordChange, opts ...grpc.CallOption) (*empty.Empty, error) {
|
|
out := new(empty.Empty)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/ChangeMyPassword", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) GetMyPasswordComplexityPolicy(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*PasswordComplexityPolicy, error) {
|
|
out := new(PasswordComplexityPolicy)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/GetMyPasswordComplexityPolicy", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) SearchMyExternalIDPs(ctx context.Context, in *ExternalIDPSearchRequest, opts ...grpc.CallOption) (*ExternalIDPSearchResponse, error) {
|
|
out := new(ExternalIDPSearchResponse)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/SearchMyExternalIDPs", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) RemoveMyExternalIDP(ctx context.Context, in *ExternalIDPRemoveRequest, opts ...grpc.CallOption) (*empty.Empty, error) {
|
|
out := new(empty.Empty)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/RemoveMyExternalIDP", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) AddMfaOTP(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*MfaOtpResponse, error) {
|
|
out := new(MfaOtpResponse)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/AddMfaOTP", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) VerifyMfaOTP(ctx context.Context, in *VerifyMfaOtp, opts ...grpc.CallOption) (*empty.Empty, error) {
|
|
out := new(empty.Empty)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/VerifyMfaOTP", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) RemoveMfaOTP(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*empty.Empty, error) {
|
|
out := new(empty.Empty)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/RemoveMfaOTP", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) SearchMyUserGrant(ctx context.Context, in *UserGrantSearchRequest, opts ...grpc.CallOption) (*UserGrantSearchResponse, error) {
|
|
out := new(UserGrantSearchResponse)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/SearchMyUserGrant", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) SearchMyProjectOrgs(ctx context.Context, in *MyProjectOrgSearchRequest, opts ...grpc.CallOption) (*MyProjectOrgSearchResponse, error) {
|
|
out := new(MyProjectOrgSearchResponse)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/SearchMyProjectOrgs", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) GetMyZitadelPermissions(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*MyPermissions, error) {
|
|
out := new(MyPermissions)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/GetMyZitadelPermissions", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *authServiceClient) GetMyProjectPermissions(ctx context.Context, in *empty.Empty, opts ...grpc.CallOption) (*MyPermissions, error) {
|
|
out := new(MyPermissions)
|
|
err := c.cc.Invoke(ctx, "/caos.zitadel.auth.api.v1.AuthService/GetMyProjectPermissions", in, out, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
// AuthServiceServer is the server API for AuthService service.
|
|
type AuthServiceServer interface {
|
|
// Readiness
|
|
Healthz(context.Context, *empty.Empty) (*empty.Empty, error)
|
|
Ready(context.Context, *empty.Empty) (*empty.Empty, error)
|
|
Validate(context.Context, *empty.Empty) (*_struct.Struct, error)
|
|
// Authorization
|
|
GetMyUserSessions(context.Context, *empty.Empty) (*UserSessionViews, error)
|
|
//User
|
|
GetMyUser(context.Context, *empty.Empty) (*UserView, error)
|
|
GetMyUserProfile(context.Context, *empty.Empty) (*UserProfileView, error)
|
|
UpdateMyUserProfile(context.Context, *UpdateUserProfileRequest) (*UserProfile, error)
|
|
ChangeMyUserName(context.Context, *ChangeUserNameRequest) (*empty.Empty, error)
|
|
GetMyUserEmail(context.Context, *empty.Empty) (*UserEmailView, error)
|
|
ChangeMyUserEmail(context.Context, *UpdateUserEmailRequest) (*UserEmail, error)
|
|
VerifyMyUserEmail(context.Context, *VerifyMyUserEmailRequest) (*empty.Empty, error)
|
|
ResendMyEmailVerificationMail(context.Context, *empty.Empty) (*empty.Empty, error)
|
|
GetMyUserPhone(context.Context, *empty.Empty) (*UserPhoneView, error)
|
|
ChangeMyUserPhone(context.Context, *UpdateUserPhoneRequest) (*UserPhone, error)
|
|
RemoveMyUserPhone(context.Context, *empty.Empty) (*empty.Empty, error)
|
|
VerifyMyUserPhone(context.Context, *VerifyUserPhoneRequest) (*empty.Empty, error)
|
|
ResendMyPhoneVerificationCode(context.Context, *empty.Empty) (*empty.Empty, error)
|
|
GetMyUserAddress(context.Context, *empty.Empty) (*UserAddressView, error)
|
|
GetMyUserChanges(context.Context, *ChangesRequest) (*Changes, error)
|
|
UpdateMyUserAddress(context.Context, *UpdateUserAddressRequest) (*UserAddress, error)
|
|
GetMyMfas(context.Context, *empty.Empty) (*MultiFactors, error)
|
|
//Password
|
|
ChangeMyPassword(context.Context, *PasswordChange) (*empty.Empty, error)
|
|
GetMyPasswordComplexityPolicy(context.Context, *empty.Empty) (*PasswordComplexityPolicy, error)
|
|
//ExternalIDP
|
|
SearchMyExternalIDPs(context.Context, *ExternalIDPSearchRequest) (*ExternalIDPSearchResponse, error)
|
|
RemoveMyExternalIDP(context.Context, *ExternalIDPRemoveRequest) (*empty.Empty, error)
|
|
// MFA
|
|
AddMfaOTP(context.Context, *empty.Empty) (*MfaOtpResponse, error)
|
|
VerifyMfaOTP(context.Context, *VerifyMfaOtp) (*empty.Empty, error)
|
|
RemoveMfaOTP(context.Context, *empty.Empty) (*empty.Empty, error)
|
|
SearchMyUserGrant(context.Context, *UserGrantSearchRequest) (*UserGrantSearchResponse, error)
|
|
SearchMyProjectOrgs(context.Context, *MyProjectOrgSearchRequest) (*MyProjectOrgSearchResponse, error)
|
|
//Permission
|
|
GetMyZitadelPermissions(context.Context, *empty.Empty) (*MyPermissions, error)
|
|
GetMyProjectPermissions(context.Context, *empty.Empty) (*MyPermissions, error)
|
|
}
|
|
|
|
// UnimplementedAuthServiceServer can be embedded to have forward compatible implementations.
|
|
type UnimplementedAuthServiceServer struct {
|
|
}
|
|
|
|
func (*UnimplementedAuthServiceServer) Healthz(context.Context, *empty.Empty) (*empty.Empty, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method Healthz not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) Ready(context.Context, *empty.Empty) (*empty.Empty, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method Ready not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) Validate(context.Context, *empty.Empty) (*_struct.Struct, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method Validate not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) GetMyUserSessions(context.Context, *empty.Empty) (*UserSessionViews, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method GetMyUserSessions not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) GetMyUser(context.Context, *empty.Empty) (*UserView, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method GetMyUser not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) GetMyUserProfile(context.Context, *empty.Empty) (*UserProfileView, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method GetMyUserProfile not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) UpdateMyUserProfile(context.Context, *UpdateUserProfileRequest) (*UserProfile, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method UpdateMyUserProfile not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) ChangeMyUserName(context.Context, *ChangeUserNameRequest) (*empty.Empty, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method ChangeMyUserName not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) GetMyUserEmail(context.Context, *empty.Empty) (*UserEmailView, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method GetMyUserEmail not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) ChangeMyUserEmail(context.Context, *UpdateUserEmailRequest) (*UserEmail, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method ChangeMyUserEmail not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) VerifyMyUserEmail(context.Context, *VerifyMyUserEmailRequest) (*empty.Empty, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method VerifyMyUserEmail not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) ResendMyEmailVerificationMail(context.Context, *empty.Empty) (*empty.Empty, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method ResendMyEmailVerificationMail not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) GetMyUserPhone(context.Context, *empty.Empty) (*UserPhoneView, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method GetMyUserPhone not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) ChangeMyUserPhone(context.Context, *UpdateUserPhoneRequest) (*UserPhone, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method ChangeMyUserPhone not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) RemoveMyUserPhone(context.Context, *empty.Empty) (*empty.Empty, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method RemoveMyUserPhone not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) VerifyMyUserPhone(context.Context, *VerifyUserPhoneRequest) (*empty.Empty, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method VerifyMyUserPhone not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) ResendMyPhoneVerificationCode(context.Context, *empty.Empty) (*empty.Empty, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method ResendMyPhoneVerificationCode not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) GetMyUserAddress(context.Context, *empty.Empty) (*UserAddressView, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method GetMyUserAddress not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) GetMyUserChanges(context.Context, *ChangesRequest) (*Changes, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method GetMyUserChanges not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) UpdateMyUserAddress(context.Context, *UpdateUserAddressRequest) (*UserAddress, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method UpdateMyUserAddress not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) GetMyMfas(context.Context, *empty.Empty) (*MultiFactors, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method GetMyMfas not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) ChangeMyPassword(context.Context, *PasswordChange) (*empty.Empty, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method ChangeMyPassword not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) GetMyPasswordComplexityPolicy(context.Context, *empty.Empty) (*PasswordComplexityPolicy, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method GetMyPasswordComplexityPolicy not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) SearchMyExternalIDPs(context.Context, *ExternalIDPSearchRequest) (*ExternalIDPSearchResponse, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method SearchMyExternalIDPs not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) RemoveMyExternalIDP(context.Context, *ExternalIDPRemoveRequest) (*empty.Empty, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method RemoveMyExternalIDP not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) AddMfaOTP(context.Context, *empty.Empty) (*MfaOtpResponse, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method AddMfaOTP not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) VerifyMfaOTP(context.Context, *VerifyMfaOtp) (*empty.Empty, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method VerifyMfaOTP not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) RemoveMfaOTP(context.Context, *empty.Empty) (*empty.Empty, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method RemoveMfaOTP not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) SearchMyUserGrant(context.Context, *UserGrantSearchRequest) (*UserGrantSearchResponse, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method SearchMyUserGrant not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) SearchMyProjectOrgs(context.Context, *MyProjectOrgSearchRequest) (*MyProjectOrgSearchResponse, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method SearchMyProjectOrgs not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) GetMyZitadelPermissions(context.Context, *empty.Empty) (*MyPermissions, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method GetMyZitadelPermissions not implemented")
|
|
}
|
|
func (*UnimplementedAuthServiceServer) GetMyProjectPermissions(context.Context, *empty.Empty) (*MyPermissions, error) {
|
|
return nil, status.Errorf(codes.Unimplemented, "method GetMyProjectPermissions not implemented")
|
|
}
|
|
|
|
func RegisterAuthServiceServer(s *grpc.Server, srv AuthServiceServer) {
|
|
s.RegisterService(&_AuthService_serviceDesc, srv)
|
|
}
|
|
|
|
func _AuthService_Healthz_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(empty.Empty)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).Healthz(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/Healthz",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).Healthz(ctx, req.(*empty.Empty))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_Ready_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(empty.Empty)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).Ready(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/Ready",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).Ready(ctx, req.(*empty.Empty))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_Validate_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(empty.Empty)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).Validate(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/Validate",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).Validate(ctx, req.(*empty.Empty))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_GetMyUserSessions_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(empty.Empty)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).GetMyUserSessions(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/GetMyUserSessions",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).GetMyUserSessions(ctx, req.(*empty.Empty))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_GetMyUser_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(empty.Empty)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).GetMyUser(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/GetMyUser",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).GetMyUser(ctx, req.(*empty.Empty))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_GetMyUserProfile_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(empty.Empty)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).GetMyUserProfile(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/GetMyUserProfile",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).GetMyUserProfile(ctx, req.(*empty.Empty))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_UpdateMyUserProfile_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(UpdateUserProfileRequest)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).UpdateMyUserProfile(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/UpdateMyUserProfile",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).UpdateMyUserProfile(ctx, req.(*UpdateUserProfileRequest))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_ChangeMyUserName_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(ChangeUserNameRequest)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).ChangeMyUserName(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/ChangeMyUserName",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).ChangeMyUserName(ctx, req.(*ChangeUserNameRequest))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_GetMyUserEmail_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(empty.Empty)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).GetMyUserEmail(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/GetMyUserEmail",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).GetMyUserEmail(ctx, req.(*empty.Empty))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_ChangeMyUserEmail_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(UpdateUserEmailRequest)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).ChangeMyUserEmail(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/ChangeMyUserEmail",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).ChangeMyUserEmail(ctx, req.(*UpdateUserEmailRequest))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_VerifyMyUserEmail_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(VerifyMyUserEmailRequest)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).VerifyMyUserEmail(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/VerifyMyUserEmail",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).VerifyMyUserEmail(ctx, req.(*VerifyMyUserEmailRequest))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_ResendMyEmailVerificationMail_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(empty.Empty)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).ResendMyEmailVerificationMail(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/ResendMyEmailVerificationMail",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).ResendMyEmailVerificationMail(ctx, req.(*empty.Empty))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_GetMyUserPhone_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(empty.Empty)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).GetMyUserPhone(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/GetMyUserPhone",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).GetMyUserPhone(ctx, req.(*empty.Empty))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_ChangeMyUserPhone_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(UpdateUserPhoneRequest)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).ChangeMyUserPhone(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/ChangeMyUserPhone",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).ChangeMyUserPhone(ctx, req.(*UpdateUserPhoneRequest))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_RemoveMyUserPhone_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(empty.Empty)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).RemoveMyUserPhone(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/RemoveMyUserPhone",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).RemoveMyUserPhone(ctx, req.(*empty.Empty))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_VerifyMyUserPhone_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(VerifyUserPhoneRequest)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).VerifyMyUserPhone(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/VerifyMyUserPhone",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).VerifyMyUserPhone(ctx, req.(*VerifyUserPhoneRequest))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_ResendMyPhoneVerificationCode_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(empty.Empty)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).ResendMyPhoneVerificationCode(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/ResendMyPhoneVerificationCode",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).ResendMyPhoneVerificationCode(ctx, req.(*empty.Empty))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_GetMyUserAddress_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(empty.Empty)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).GetMyUserAddress(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/GetMyUserAddress",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).GetMyUserAddress(ctx, req.(*empty.Empty))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_GetMyUserChanges_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(ChangesRequest)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).GetMyUserChanges(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/GetMyUserChanges",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).GetMyUserChanges(ctx, req.(*ChangesRequest))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_UpdateMyUserAddress_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(UpdateUserAddressRequest)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).UpdateMyUserAddress(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/UpdateMyUserAddress",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).UpdateMyUserAddress(ctx, req.(*UpdateUserAddressRequest))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_GetMyMfas_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(empty.Empty)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).GetMyMfas(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/GetMyMfas",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).GetMyMfas(ctx, req.(*empty.Empty))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_ChangeMyPassword_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(PasswordChange)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).ChangeMyPassword(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/ChangeMyPassword",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).ChangeMyPassword(ctx, req.(*PasswordChange))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_GetMyPasswordComplexityPolicy_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(empty.Empty)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).GetMyPasswordComplexityPolicy(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/GetMyPasswordComplexityPolicy",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).GetMyPasswordComplexityPolicy(ctx, req.(*empty.Empty))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_SearchMyExternalIDPs_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(ExternalIDPSearchRequest)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).SearchMyExternalIDPs(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/SearchMyExternalIDPs",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).SearchMyExternalIDPs(ctx, req.(*ExternalIDPSearchRequest))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_RemoveMyExternalIDP_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(ExternalIDPRemoveRequest)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).RemoveMyExternalIDP(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/RemoveMyExternalIDP",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).RemoveMyExternalIDP(ctx, req.(*ExternalIDPRemoveRequest))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_AddMfaOTP_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(empty.Empty)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).AddMfaOTP(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/AddMfaOTP",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).AddMfaOTP(ctx, req.(*empty.Empty))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_VerifyMfaOTP_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(VerifyMfaOtp)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).VerifyMfaOTP(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/VerifyMfaOTP",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).VerifyMfaOTP(ctx, req.(*VerifyMfaOtp))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_RemoveMfaOTP_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(empty.Empty)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).RemoveMfaOTP(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/RemoveMfaOTP",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).RemoveMfaOTP(ctx, req.(*empty.Empty))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_SearchMyUserGrant_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(UserGrantSearchRequest)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).SearchMyUserGrant(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/SearchMyUserGrant",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).SearchMyUserGrant(ctx, req.(*UserGrantSearchRequest))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_SearchMyProjectOrgs_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(MyProjectOrgSearchRequest)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).SearchMyProjectOrgs(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/SearchMyProjectOrgs",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).SearchMyProjectOrgs(ctx, req.(*MyProjectOrgSearchRequest))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_GetMyZitadelPermissions_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(empty.Empty)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).GetMyZitadelPermissions(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/GetMyZitadelPermissions",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).GetMyZitadelPermissions(ctx, req.(*empty.Empty))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _AuthService_GetMyProjectPermissions_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(empty.Empty)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(AuthServiceServer).GetMyProjectPermissions(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/caos.zitadel.auth.api.v1.AuthService/GetMyProjectPermissions",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(AuthServiceServer).GetMyProjectPermissions(ctx, req.(*empty.Empty))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
var _AuthService_serviceDesc = grpc.ServiceDesc{
|
|
ServiceName: "caos.zitadel.auth.api.v1.AuthService",
|
|
HandlerType: (*AuthServiceServer)(nil),
|
|
Methods: []grpc.MethodDesc{
|
|
{
|
|
MethodName: "Healthz",
|
|
Handler: _AuthService_Healthz_Handler,
|
|
},
|
|
{
|
|
MethodName: "Ready",
|
|
Handler: _AuthService_Ready_Handler,
|
|
},
|
|
{
|
|
MethodName: "Validate",
|
|
Handler: _AuthService_Validate_Handler,
|
|
},
|
|
{
|
|
MethodName: "GetMyUserSessions",
|
|
Handler: _AuthService_GetMyUserSessions_Handler,
|
|
},
|
|
{
|
|
MethodName: "GetMyUser",
|
|
Handler: _AuthService_GetMyUser_Handler,
|
|
},
|
|
{
|
|
MethodName: "GetMyUserProfile",
|
|
Handler: _AuthService_GetMyUserProfile_Handler,
|
|
},
|
|
{
|
|
MethodName: "UpdateMyUserProfile",
|
|
Handler: _AuthService_UpdateMyUserProfile_Handler,
|
|
},
|
|
{
|
|
MethodName: "ChangeMyUserName",
|
|
Handler: _AuthService_ChangeMyUserName_Handler,
|
|
},
|
|
{
|
|
MethodName: "GetMyUserEmail",
|
|
Handler: _AuthService_GetMyUserEmail_Handler,
|
|
},
|
|
{
|
|
MethodName: "ChangeMyUserEmail",
|
|
Handler: _AuthService_ChangeMyUserEmail_Handler,
|
|
},
|
|
{
|
|
MethodName: "VerifyMyUserEmail",
|
|
Handler: _AuthService_VerifyMyUserEmail_Handler,
|
|
},
|
|
{
|
|
MethodName: "ResendMyEmailVerificationMail",
|
|
Handler: _AuthService_ResendMyEmailVerificationMail_Handler,
|
|
},
|
|
{
|
|
MethodName: "GetMyUserPhone",
|
|
Handler: _AuthService_GetMyUserPhone_Handler,
|
|
},
|
|
{
|
|
MethodName: "ChangeMyUserPhone",
|
|
Handler: _AuthService_ChangeMyUserPhone_Handler,
|
|
},
|
|
{
|
|
MethodName: "RemoveMyUserPhone",
|
|
Handler: _AuthService_RemoveMyUserPhone_Handler,
|
|
},
|
|
{
|
|
MethodName: "VerifyMyUserPhone",
|
|
Handler: _AuthService_VerifyMyUserPhone_Handler,
|
|
},
|
|
{
|
|
MethodName: "ResendMyPhoneVerificationCode",
|
|
Handler: _AuthService_ResendMyPhoneVerificationCode_Handler,
|
|
},
|
|
{
|
|
MethodName: "GetMyUserAddress",
|
|
Handler: _AuthService_GetMyUserAddress_Handler,
|
|
},
|
|
{
|
|
MethodName: "GetMyUserChanges",
|
|
Handler: _AuthService_GetMyUserChanges_Handler,
|
|
},
|
|
{
|
|
MethodName: "UpdateMyUserAddress",
|
|
Handler: _AuthService_UpdateMyUserAddress_Handler,
|
|
},
|
|
{
|
|
MethodName: "GetMyMfas",
|
|
Handler: _AuthService_GetMyMfas_Handler,
|
|
},
|
|
{
|
|
MethodName: "ChangeMyPassword",
|
|
Handler: _AuthService_ChangeMyPassword_Handler,
|
|
},
|
|
{
|
|
MethodName: "GetMyPasswordComplexityPolicy",
|
|
Handler: _AuthService_GetMyPasswordComplexityPolicy_Handler,
|
|
},
|
|
{
|
|
MethodName: "SearchMyExternalIDPs",
|
|
Handler: _AuthService_SearchMyExternalIDPs_Handler,
|
|
},
|
|
{
|
|
MethodName: "RemoveMyExternalIDP",
|
|
Handler: _AuthService_RemoveMyExternalIDP_Handler,
|
|
},
|
|
{
|
|
MethodName: "AddMfaOTP",
|
|
Handler: _AuthService_AddMfaOTP_Handler,
|
|
},
|
|
{
|
|
MethodName: "VerifyMfaOTP",
|
|
Handler: _AuthService_VerifyMfaOTP_Handler,
|
|
},
|
|
{
|
|
MethodName: "RemoveMfaOTP",
|
|
Handler: _AuthService_RemoveMfaOTP_Handler,
|
|
},
|
|
{
|
|
MethodName: "SearchMyUserGrant",
|
|
Handler: _AuthService_SearchMyUserGrant_Handler,
|
|
},
|
|
{
|
|
MethodName: "SearchMyProjectOrgs",
|
|
Handler: _AuthService_SearchMyProjectOrgs_Handler,
|
|
},
|
|
{
|
|
MethodName: "GetMyZitadelPermissions",
|
|
Handler: _AuthService_GetMyZitadelPermissions_Handler,
|
|
},
|
|
{
|
|
MethodName: "GetMyProjectPermissions",
|
|
Handler: _AuthService_GetMyProjectPermissions_Handler,
|
|
},
|
|
},
|
|
Streams: []grpc.StreamDesc{},
|
|
Metadata: "auth.proto",
|
|
}
|