mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 19:14:23 +00:00
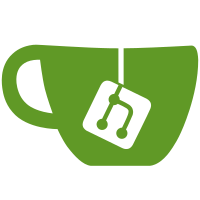
* fix: push events instead of aggregates * fix: tests * try without aggregate methods and with aggregate methods * fix: change push aggregate to push events * fix: change push aggregate to push events * fix: change push aggregate to push events * fix: change push aggregate to push events * fix: change push aggregate to push events * fix: change push aggregate to push events * fix: change push aggregate to push events * fix: change push aggregate to push events * fix: change push aggregate to push events * fix: change push aggregate to push events * fix: client secret * fix: query eventtypes * fix: query eventtypes * fix: eventstore index * fix: index * fix: merge new eventstore * fix: remove unnecessary todos * fix: remove unnecessary todos Co-authored-by: Fabiennne <fabienne.gerschwiler@gmail.com>
130 lines
3.7 KiB
Go
130 lines
3.7 KiB
Go
package command
|
|
|
|
import (
|
|
"context"
|
|
"reflect"
|
|
|
|
"github.com/caos/zitadel/internal/crypto"
|
|
"github.com/caos/zitadel/internal/eventstore/v2"
|
|
"github.com/caos/zitadel/internal/v2/domain"
|
|
"github.com/caos/zitadel/internal/v2/repository/idpconfig"
|
|
"github.com/caos/zitadel/internal/v2/repository/org"
|
|
)
|
|
|
|
type IDPOIDCConfigWriteModel struct {
|
|
OIDCConfigWriteModel
|
|
}
|
|
|
|
func NewOrgIDPOIDCConfigWriteModel(idpConfigID, orgID string) *IDPOIDCConfigWriteModel {
|
|
return &IDPOIDCConfigWriteModel{
|
|
OIDCConfigWriteModel{
|
|
WriteModel: eventstore.WriteModel{
|
|
AggregateID: orgID,
|
|
ResourceOwner: orgID,
|
|
},
|
|
IDPConfigID: idpConfigID,
|
|
},
|
|
}
|
|
}
|
|
|
|
func (wm *IDPOIDCConfigWriteModel) AppendEvents(events ...eventstore.EventReader) {
|
|
for _, event := range events {
|
|
switch e := event.(type) {
|
|
case *org.IDPOIDCConfigAddedEvent:
|
|
if wm.IDPConfigID != e.IDPConfigID {
|
|
continue
|
|
}
|
|
wm.OIDCConfigWriteModel.AppendEvents(&e.OIDCConfigAddedEvent)
|
|
case *org.IDPOIDCConfigChangedEvent:
|
|
if wm.IDPConfigID != e.IDPConfigID {
|
|
continue
|
|
}
|
|
wm.OIDCConfigWriteModel.AppendEvents(&e.OIDCConfigChangedEvent)
|
|
case *org.IDPConfigReactivatedEvent:
|
|
if wm.IDPConfigID != e.ConfigID {
|
|
continue
|
|
}
|
|
wm.OIDCConfigWriteModel.AppendEvents(&e.IDPConfigReactivatedEvent)
|
|
case *org.IDPConfigDeactivatedEvent:
|
|
if wm.IDPConfigID != e.ConfigID {
|
|
continue
|
|
}
|
|
wm.OIDCConfigWriteModel.AppendEvents(&e.IDPConfigDeactivatedEvent)
|
|
case *org.IDPConfigRemovedEvent:
|
|
if wm.IDPConfigID != e.ConfigID {
|
|
continue
|
|
}
|
|
wm.OIDCConfigWriteModel.AppendEvents(&e.IDPConfigRemovedEvent)
|
|
default:
|
|
wm.OIDCConfigWriteModel.AppendEvents(e)
|
|
}
|
|
}
|
|
}
|
|
|
|
func (wm *IDPOIDCConfigWriteModel) Reduce() error {
|
|
if err := wm.OIDCConfigWriteModel.Reduce(); err != nil {
|
|
return err
|
|
}
|
|
return wm.WriteModel.Reduce()
|
|
}
|
|
|
|
func (wm *IDPOIDCConfigWriteModel) Query() *eventstore.SearchQueryBuilder {
|
|
return eventstore.NewSearchQueryBuilder(eventstore.ColumnsEvent, org.AggregateType).
|
|
AggregateIDs(wm.AggregateID).
|
|
ResourceOwner(wm.ResourceOwner).
|
|
EventTypes(
|
|
org.IDPOIDCConfigAddedEventType,
|
|
org.IDPOIDCConfigChangedEventType,
|
|
org.IDPConfigReactivatedEventType,
|
|
org.IDPConfigDeactivatedEventType,
|
|
org.IDPConfigRemovedEventType)
|
|
}
|
|
|
|
func (wm *IDPOIDCConfigWriteModel) NewChangedEvent(
|
|
ctx context.Context,
|
|
aggregate *eventstore.Aggregate,
|
|
idpConfigID,
|
|
clientID,
|
|
issuer,
|
|
clientSecretString string,
|
|
secretCrypto crypto.Crypto,
|
|
idpDisplayNameMapping,
|
|
userNameMapping domain.OIDCMappingField,
|
|
scopes ...string,
|
|
) (*org.IDPOIDCConfigChangedEvent, bool, error) {
|
|
|
|
changes := make([]idpconfig.OIDCConfigChanges, 0)
|
|
var clientSecret *crypto.CryptoValue
|
|
var err error
|
|
if clientSecretString != "" {
|
|
clientSecret, err = crypto.Crypt([]byte(clientSecretString), secretCrypto)
|
|
if err != nil {
|
|
return nil, false, err
|
|
}
|
|
changes = append(changes, idpconfig.ChangeClientSecret(clientSecret))
|
|
}
|
|
if wm.ClientID != clientID {
|
|
changes = append(changes, idpconfig.ChangeClientID(clientID))
|
|
}
|
|
if wm.Issuer != issuer {
|
|
changes = append(changes, idpconfig.ChangeIssuer(issuer))
|
|
}
|
|
if idpDisplayNameMapping.Valid() && wm.IDPDisplayNameMapping != idpDisplayNameMapping {
|
|
changes = append(changes, idpconfig.ChangeIDPDisplayNameMapping(idpDisplayNameMapping))
|
|
}
|
|
if userNameMapping.Valid() && wm.UserNameMapping != userNameMapping {
|
|
changes = append(changes, idpconfig.ChangeUserNameMapping(userNameMapping))
|
|
}
|
|
if reflect.DeepEqual(wm.Scopes, scopes) {
|
|
changes = append(changes, idpconfig.ChangeScopes(scopes))
|
|
}
|
|
if len(changes) == 0 {
|
|
return nil, false, nil
|
|
}
|
|
changeEvent, err := org.NewIDPOIDCConfigChangedEvent(ctx, aggregate, idpConfigID, changes)
|
|
if err != nil {
|
|
return nil, false, err
|
|
}
|
|
return changeEvent, true, nil
|
|
}
|