mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
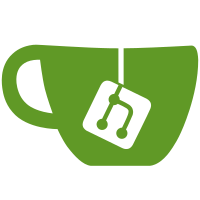
* project quota added * project quota removed * add periods table * make log record generic * accumulate usage * query usage * count action run seconds * fix filter in ReportQuotaUsage * fix existing tests * fix logstore tests * fix typo * fix: add quota unit tests command side * fix: add quota unit tests command side * fix: add quota unit tests command side * move notifications into debouncer and improve limit querying * cleanup * comment * fix: add quota unit tests command side * fix remaining quota usage query * implement InmemLogStorage * cleanup and linting * improve test * fix: add quota unit tests command side * fix: add quota unit tests command side * fix: add quota unit tests command side * fix: add quota unit tests command side * action notifications and fixes for notifications query * revert console prefix * fix: add quota unit tests command side * fix: add quota integration tests * improve accountable requests * improve accountable requests * fix: add quota integration tests * fix: add quota integration tests * fix: add quota integration tests * comment * remove ability to store logs in db and other changes requested from review * changes requested from review * changes requested from review * Update internal/api/http/middleware/access_interceptor.go Co-authored-by: Silvan <silvan.reusser@gmail.com> * tests: fix quotas integration tests * improve incrementUsageStatement * linting * fix: delete e2e tests as intergation tests cover functionality * Update internal/api/http/middleware/access_interceptor.go Co-authored-by: Silvan <silvan.reusser@gmail.com> * backup * fix conflict * create rc * create prerelease * remove issue release labeling * fix tracing --------- Co-authored-by: Livio Spring <livio.a@gmail.com> Co-authored-by: Stefan Benz <stefan@caos.ch> Co-authored-by: adlerhurst <silvan.reusser@gmail.com>
74 lines
1.5 KiB
Go
74 lines
1.5 KiB
Go
package actions
|
|
|
|
import (
|
|
"context"
|
|
"errors"
|
|
"testing"
|
|
"time"
|
|
|
|
"github.com/dop251/goja"
|
|
|
|
"github.com/zitadel/zitadel/internal/logstore"
|
|
"github.com/zitadel/zitadel/internal/logstore/record"
|
|
)
|
|
|
|
func TestRun(t *testing.T) {
|
|
SetLogstoreService(logstore.New[*record.ExecutionLog](nil, nil))
|
|
type args struct {
|
|
timeout time.Duration
|
|
api apiFields
|
|
ctx contextFields
|
|
script string
|
|
name string
|
|
opts []Option
|
|
}
|
|
tests := []struct {
|
|
name string
|
|
args args
|
|
wantErr func(error) bool
|
|
}{
|
|
{
|
|
name: "simple script",
|
|
args: args{
|
|
api: nil,
|
|
script: `
|
|
function testFunc() {
|
|
for (i = 0; i < 10; i++) {}
|
|
}`,
|
|
name: "testFunc",
|
|
opts: []Option{},
|
|
},
|
|
wantErr: func(err error) bool { return err == nil },
|
|
},
|
|
{
|
|
name: "throw error",
|
|
args: args{
|
|
api: nil,
|
|
script: "function testFunc() {throw 'some error'}",
|
|
name: "testFunc",
|
|
opts: []Option{},
|
|
},
|
|
wantErr: func(err error) bool {
|
|
gojaErr := new(goja.Exception)
|
|
if errors.As(err, &gojaErr) {
|
|
return gojaErr.Value().String() == "some error"
|
|
}
|
|
return false
|
|
},
|
|
},
|
|
}
|
|
|
|
for _, tt := range tests {
|
|
t.Run(tt.name, func(t *testing.T) {
|
|
if tt.args.timeout == 0 {
|
|
tt.args.timeout = 10 * time.Second
|
|
}
|
|
ctx, cancel := context.WithTimeout(context.Background(), tt.args.timeout)
|
|
if err := Run(ctx, tt.args.ctx, tt.args.api, tt.args.script, tt.args.name, tt.args.opts...); !tt.wantErr(err) {
|
|
t.Errorf("Run() unexpected error = (%[1]T) %[1]v", err)
|
|
}
|
|
cancel()
|
|
})
|
|
}
|
|
}
|