mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 19:14:23 +00:00
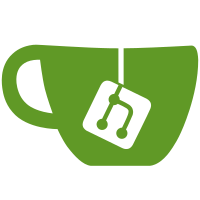
* feat(import): add functionality to import data into an instance * feat(import): move import to admin api and additional checks for nil pointer * fix(export): export implementation with filtered members and grants * fix: export and import implementation * fix: add possibility to export hashed passwords with the user * fix(import): import with structure of v1 and v2 * docs: add v1 proto * fix(import): check im imported user is already existing * fix(import): add otp import function * fix(import): add external idps, domains, custom text and messages * fix(import): correct usage of default values from login policy * fix(export): fix renaming of add project function * fix(import): move checks for unit tests * expect filter * fix(import): move checks for unit tests * fix(import): move checks for unit tests * fix(import): produce prerelease from branch * fix(import): correctly use provided user id for machine user imports * fix(import): corrected otp import and added guide for export and import * fix: import verified and primary domains * fix(import): add reading from gcs, s3 and localfile with tracing * fix(import): gcs and s3, file size correction and error logging * Delete docker-compose.yml * fix(import): progress logging and count of resources * fix(import): progress logging and count of resources * log subscription * fix(import): incorporate review * fix(import): incorporate review * docs: add suggestion for import Co-authored-by: Fabi <38692350+hifabienne@users.noreply.github.com> * fix(import): add verification otp event and handling of deleted but existing users Co-authored-by: Livio Amstutz <livio.a@gmail.com> Co-authored-by: Fabienne <fabienne.gerschwiler@gmail.com> Co-authored-by: Silvan <silvan.reusser@gmail.com> Co-authored-by: Fabi <38692350+hifabienne@users.noreply.github.com>
83 lines
2.1 KiB
Go
83 lines
2.1 KiB
Go
package admin
|
|
|
|
import (
|
|
"context"
|
|
"google.golang.org/grpc"
|
|
|
|
"github.com/zitadel/zitadel/internal/admin/repository"
|
|
"github.com/zitadel/zitadel/internal/admin/repository/eventsourcing"
|
|
"github.com/zitadel/zitadel/internal/api/assets"
|
|
"github.com/zitadel/zitadel/internal/api/authz"
|
|
"github.com/zitadel/zitadel/internal/api/grpc/server"
|
|
"github.com/zitadel/zitadel/internal/command"
|
|
"github.com/zitadel/zitadel/internal/config/systemdefaults"
|
|
"github.com/zitadel/zitadel/internal/crypto"
|
|
"github.com/zitadel/zitadel/internal/query"
|
|
"github.com/zitadel/zitadel/pkg/grpc/admin"
|
|
)
|
|
|
|
const (
|
|
adminName = "Admin-API"
|
|
)
|
|
|
|
var _ admin.AdminServiceServer = (*Server)(nil)
|
|
|
|
type Server struct {
|
|
admin.UnimplementedAdminServiceServer
|
|
database string
|
|
command *command.Commands
|
|
query *query.Queries
|
|
administrator repository.AdministratorRepository
|
|
assetsAPIDomain func(context.Context) string
|
|
userCodeAlg crypto.EncryptionAlgorithm
|
|
passwordHashAlg crypto.HashAlgorithm
|
|
}
|
|
|
|
type Config struct {
|
|
Repository eventsourcing.Config
|
|
}
|
|
|
|
func CreateServer(
|
|
database string,
|
|
command *command.Commands,
|
|
query *query.Queries,
|
|
sd systemdefaults.SystemDefaults,
|
|
repo repository.Repository,
|
|
externalSecure bool,
|
|
userCodeAlg crypto.EncryptionAlgorithm,
|
|
) *Server {
|
|
return &Server{
|
|
database: database,
|
|
command: command,
|
|
query: query,
|
|
administrator: repo,
|
|
assetsAPIDomain: assets.AssetAPI(externalSecure),
|
|
userCodeAlg: userCodeAlg,
|
|
passwordHashAlg: crypto.NewBCrypt(sd.SecretGenerators.PasswordSaltCost),
|
|
}
|
|
}
|
|
|
|
func (s *Server) RegisterServer(grpcServer *grpc.Server) {
|
|
admin.RegisterAdminServiceServer(grpcServer, s)
|
|
}
|
|
|
|
func (s *Server) AppName() string {
|
|
return adminName
|
|
}
|
|
|
|
func (s *Server) MethodPrefix() string {
|
|
return admin.AdminService_MethodPrefix
|
|
}
|
|
|
|
func (s *Server) AuthMethods() authz.MethodMapping {
|
|
return admin.AdminService_AuthMethods
|
|
}
|
|
|
|
func (s *Server) RegisterGateway() server.GatewayFunc {
|
|
return admin.RegisterAdminServiceHandlerFromEndpoint
|
|
}
|
|
|
|
func (s *Server) GatewayPathPrefix() string {
|
|
return "/admin/v1"
|
|
}
|