mirror of
https://github.com/zitadel/zitadel.git
synced 2025-03-04 06:55:14 +00:00
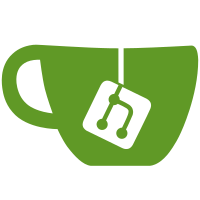
* feat: change mail template to new query side * feat: adminapi message text * feat: adminapi message text * feat: adminapi message text * feat: message texts * feat: admin texts * feat: tests * feat: tests * feat: custom login text on adminapi * feat: custom login text * feat: custom login text * feat: message text prepare test * feat: login text texts * feat: custom login text * merge main * fix go.sum Co-authored-by: Livio Amstutz <livio.a@gmail.com>
5452 lines
176 KiB
Protocol Buffer
5452 lines
176 KiB
Protocol Buffer
syntax = "proto3";
|
|
|
|
import "zitadel/app.proto";
|
|
import "zitadel/idp.proto";
|
|
import "zitadel/user.proto";
|
|
import "zitadel/object.proto";
|
|
import "zitadel/options.proto";
|
|
import "zitadel/org.proto";
|
|
import "zitadel/member.proto";
|
|
import "zitadel/project.proto";
|
|
import "zitadel/policy.proto";
|
|
import "zitadel/text.proto";
|
|
import "zitadel/message.proto";
|
|
import "zitadel/change.proto";
|
|
import "zitadel/auth_n_key.proto";
|
|
import "zitadel/features.proto";
|
|
import "zitadel/metadata.proto";
|
|
import "zitadel/action.proto";
|
|
|
|
import "google/api/annotations.proto";
|
|
import "google/protobuf/timestamp.proto";
|
|
import "google/protobuf/duration.proto";
|
|
import "protoc-gen-openapiv2/options/annotations.proto";
|
|
import "validate/validate.proto";
|
|
|
|
|
|
package zitadel.management.v1;
|
|
|
|
option go_package ="github.com/caos/zitadel/pkg/grpc/management";
|
|
|
|
option (grpc.gateway.protoc_gen_openapiv2.options.openapiv2_swagger) = {
|
|
info: {
|
|
title: "Management API";
|
|
version: "1.0";
|
|
description: "The management API is as the name states the interface where systems can mutate IAM objects like, organisations, projects, clients, users and so on if they have the necessary access rights.";
|
|
contact:{
|
|
name: "CAOS developers of ZITADEL"
|
|
url: "https://zitadel.ch"
|
|
email: "hi@zitadel.ch"
|
|
}
|
|
license: {
|
|
name: "Apache License 2.0",
|
|
url: "https://github.com/caos/zitadel/blob/main/LICENSE"
|
|
};
|
|
};
|
|
|
|
schemes: HTTPS;
|
|
|
|
consumes: "application/json";
|
|
produces: "application/json";
|
|
|
|
consumes: "application/grpc";
|
|
produces: "application/grpc";
|
|
|
|
consumes: "application/grpc-web+proto";
|
|
produces: "application/grpc-web+proto";
|
|
|
|
host: "api.zitadel.ch";
|
|
base_path: "/management/v1";
|
|
|
|
external_docs: {
|
|
description: "Detailed information about ZITADEL",
|
|
url: "https://docs.zitadel.ch"
|
|
}
|
|
|
|
extensions: {
|
|
key: "x-zitadel-orgid";
|
|
value: {
|
|
string_value: "your-org-id";
|
|
}
|
|
}
|
|
};
|
|
|
|
|
|
service ManagementService {
|
|
rpc Healthz(HealthzRequest) returns (HealthzResponse) {
|
|
option (google.api.http) = {
|
|
get: "/healthz"
|
|
};
|
|
}
|
|
|
|
rpc GetOIDCInformation(GetOIDCInformationRequest) returns (GetOIDCInformationResponse) {
|
|
option (google.api.http) = {
|
|
get: "/zitadel/docs"
|
|
};
|
|
}
|
|
|
|
// Returns some needed settings of the IAM (Global Organisation ID, Zitadel Project ID)
|
|
rpc GetIAM(GetIAMRequest) returns (GetIAMResponse) {
|
|
option (google.api.http) = {
|
|
get: "/iam"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "authenticated"
|
|
};
|
|
}
|
|
|
|
// Returns the default languages
|
|
rpc GetSupportedLanguages(GetSupportedLanguagesRequest) returns (GetSupportedLanguagesResponse) {
|
|
option (google.api.http) = {
|
|
get: "/languages";
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "authenticated";
|
|
};
|
|
}
|
|
|
|
// Returns the requested full blown user (human or machine)
|
|
rpc GetUserByID(GetUserByIDRequest) returns (GetUserByIDResponse) {
|
|
option (google.api.http) = {
|
|
get: "/users/{id}"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.read"
|
|
};
|
|
}
|
|
|
|
// Searches a user over all organisations
|
|
// the login name has to match exactly
|
|
rpc GetUserByLoginNameGlobal(GetUserByLoginNameGlobalRequest) returns (GetUserByLoginNameGlobalResponse) {
|
|
option (google.api.http) = {
|
|
get: "/global/users/_by_login_name"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.global.read"
|
|
};
|
|
|
|
option (grpc.gateway.protoc_gen_openapiv2.options.openapiv2_operation) = {
|
|
summary: "Search a user within all organisations by it's loginname";
|
|
description: "The request only returns data if the login name matches exactly."
|
|
tags: "user";
|
|
tags: "global";
|
|
responses: {
|
|
key: "200"
|
|
value: {
|
|
description: "OK";
|
|
}
|
|
//TODO: errors
|
|
};
|
|
};
|
|
}
|
|
|
|
// Return the users matching the query
|
|
// Limit should always be set, there is a default limit set by the service
|
|
rpc ListUsers(ListUsersRequest) returns (ListUsersResponse) {
|
|
option (google.api.http) = {
|
|
post: "/users/_search"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.read"
|
|
};
|
|
}
|
|
|
|
// Returns the history of the user (each event)
|
|
// Limit should always be set, there is a default limit set by the service
|
|
rpc ListUserChanges(ListUserChangesRequest) returns (ListUserChangesResponse) {
|
|
option (google.api.http) = {
|
|
post: "/users/{user_id}/changes/_search"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.read"
|
|
};
|
|
}
|
|
|
|
// Returns if a user with the searched email or username is unique
|
|
rpc IsUserUnique(IsUserUniqueRequest) returns (IsUserUniqueResponse) {
|
|
option (google.api.http) = {
|
|
get: "/users/_is_unique"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.read"
|
|
};
|
|
}
|
|
|
|
// Create a user of the type human
|
|
// A email will be sent to the user if email is not verified or no password is set
|
|
// If a password is given, the user has to change on the next login
|
|
rpc AddHumanUser(AddHumanUserRequest) returns (AddHumanUserResponse) {
|
|
option (google.api.http) = {
|
|
post: "/users/human"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.write"
|
|
};
|
|
}
|
|
|
|
// Create a user of the type human
|
|
// A email will be sent to the user if email is not verified or no password is set
|
|
// If a password is given, the user doesn't have to change on the next login
|
|
rpc ImportHumanUser(ImportHumanUserRequest) returns (ImportHumanUserResponse) {
|
|
option (google.api.http) = {
|
|
post: "/users/human/_import"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.write"
|
|
};
|
|
}
|
|
|
|
// Create a user of the type machine
|
|
rpc AddMachineUser(AddMachineUserRequest) returns (AddMachineUserResponse) {
|
|
option (google.api.http) = {
|
|
post: "/users/machine"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.write"
|
|
};
|
|
}
|
|
|
|
// Changes the user state to deactivated
|
|
// The user will not be able to login
|
|
// returns an error if user state is already deactivated
|
|
rpc DeactivateUser(DeactivateUserRequest) returns (DeactivateUserResponse) {
|
|
option (google.api.http) = {
|
|
post: "/users/{id}/_deactivate"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.write"
|
|
};
|
|
}
|
|
|
|
// Changes the user state to active
|
|
// returns an error if user state is not deactivated
|
|
rpc ReactivateUser(ReactivateUserRequest) returns (ReactivateUserResponse) {
|
|
option (google.api.http) = {
|
|
post: "/users/{id}/_reactivate"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.write"
|
|
};
|
|
}
|
|
|
|
// Changes the user state to deactivated
|
|
// The user will not be able to login
|
|
// returns an error if user state is already locked
|
|
rpc LockUser(LockUserRequest) returns (LockUserResponse) {
|
|
option (google.api.http) = {
|
|
post: "/users/{id}/_lock"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.write"
|
|
};
|
|
}
|
|
|
|
// Changes the user state to active
|
|
// returns an error if user state is not locked
|
|
rpc UnlockUser(UnlockUserRequest) returns (UnlockUserResponse) {
|
|
option (google.api.http) = {
|
|
post: "/users/{id}/_unlock"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.write"
|
|
};
|
|
}
|
|
|
|
// Changes the user state to deleted
|
|
rpc RemoveUser(RemoveUserRequest) returns (RemoveUserResponse) {
|
|
option (google.api.http) = {
|
|
delete: "/users/{id}"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.delete"
|
|
};
|
|
}
|
|
|
|
// Changes the username
|
|
rpc UpdateUserName(UpdateUserNameRequest) returns (UpdateUserNameResponse) {
|
|
option (google.api.http) = {
|
|
put: "/users/{user_id}/username"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.write"
|
|
};
|
|
}
|
|
|
|
// Sets a user metadata by key
|
|
rpc SetUserMetadata(SetUserMetadataRequest) returns (SetUserMetadataResponse) {
|
|
option (google.api.http) = {
|
|
post: "/users/{id}/metadata/{key}"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.write"
|
|
feature: "metadata.user"
|
|
};
|
|
}
|
|
|
|
// Set a list of user metadata
|
|
rpc BulkSetUserMetadata(BulkSetUserMetadataRequest) returns (BulkSetUserMetadataResponse) {
|
|
option (google.api.http) = {
|
|
post: "/users/{id}/metadata/_bulk"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.write"
|
|
feature: "metadata.user"
|
|
};
|
|
}
|
|
|
|
// Returns the user metadata
|
|
rpc ListUserMetadata(ListUserMetadataRequest) returns (ListUserMetadataResponse) {
|
|
option (google.api.http) = {
|
|
post: "/users/{id}/metadata/_search"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.read"
|
|
feature: "metadata.user"
|
|
};
|
|
}
|
|
|
|
// Returns the user metadata by key
|
|
rpc GetUserMetadata(GetUserMetadataRequest) returns (GetUserMetadataResponse) {
|
|
option (google.api.http) = {
|
|
get: "/users/{id}/metadata/{key}"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.read"
|
|
feature: "metadata.user"
|
|
};
|
|
}
|
|
|
|
// Removes a user metadata by key
|
|
rpc RemoveUserMetadata(RemoveUserMetadataRequest) returns (RemoveUserMetadataResponse) {
|
|
option (google.api.http) = {
|
|
delete: "/users/{id}/metadata/{key}"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.write"
|
|
feature: "metadata.user"
|
|
};
|
|
}
|
|
|
|
// Set a list of user metadata
|
|
rpc BulkRemoveUserMetadata(BulkRemoveUserMetadataRequest) returns (BulkRemoveUserMetadataResponse) {
|
|
option (google.api.http) = {
|
|
delete: "/users/{id}/metadata/_bulk"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.write"
|
|
feature: "metadata.user"
|
|
};
|
|
}
|
|
|
|
// Returns the profile of the human
|
|
rpc GetHumanProfile(GetHumanProfileRequest) returns (GetHumanProfileResponse) {
|
|
option (google.api.http) = {
|
|
get: "/users/{user_id}/profile"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.read"
|
|
};
|
|
}
|
|
|
|
// Changes the profile of the human
|
|
rpc UpdateHumanProfile(UpdateHumanProfileRequest) returns (UpdateHumanProfileResponse) {
|
|
option (google.api.http) = {
|
|
put: "/users/{user_id}/profile"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.write"
|
|
};
|
|
}
|
|
|
|
// GetHumanEmail returns the email and verified state of the human
|
|
rpc GetHumanEmail(GetHumanEmailRequest) returns (GetHumanEmailResponse) {
|
|
option (google.api.http) = {
|
|
get: "/users/{user_id}/email"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.read"
|
|
};
|
|
}
|
|
|
|
// Changes the email of the human
|
|
// If state is not verified, the user will get a verification email
|
|
rpc UpdateHumanEmail(UpdateHumanEmailRequest) returns (UpdateHumanEmailResponse) {
|
|
option (google.api.http) = {
|
|
put: "/users/{user_id}/email"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.write"
|
|
};
|
|
}
|
|
|
|
// Resends an email to the given email address to finish the initialization process of the user
|
|
// Changes the email address of the user if it is provided
|
|
rpc ResendHumanInitialization(ResendHumanInitializationRequest) returns (ResendHumanInitializationResponse) {
|
|
option (google.api.http) = {
|
|
post: "/users/{user_id}/_resend_initialization"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.write"
|
|
};
|
|
}
|
|
|
|
// Resends an email to the given email address to finish the email verification process of the user
|
|
rpc ResendHumanEmailVerification(ResendHumanEmailVerificationRequest) returns (ResendHumanEmailVerificationResponse) {
|
|
option (google.api.http) = {
|
|
post: "/users/{user_id}/email/_resend_verification"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.write"
|
|
};
|
|
}
|
|
|
|
// Returns the phone and verified state of the human phone
|
|
rpc GetHumanPhone(GetHumanPhoneRequest) returns (GetHumanPhoneResponse) {
|
|
option (google.api.http) = {
|
|
get: "/users/{user_id}/phone"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.read"
|
|
};
|
|
}
|
|
|
|
// Changes the phone number
|
|
// If verified is not set, the user will get an sms to verify the number
|
|
rpc UpdateHumanPhone(UpdateHumanPhoneRequest) returns (UpdateHumanPhoneResponse) {
|
|
option (google.api.http) = {
|
|
put: "/users/{user_id}/phone"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.write"
|
|
};
|
|
}
|
|
|
|
// Removes the phone number of the human
|
|
rpc RemoveHumanPhone(RemoveHumanPhoneRequest) returns (RemoveHumanPhoneResponse) {
|
|
option (google.api.http) = {
|
|
delete: "/users/{user_id}/phone"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.write"
|
|
};
|
|
}
|
|
|
|
// An sms will be sent to the given phone number to finish the phone verification process of the user
|
|
rpc ResendHumanPhoneVerification(ResendHumanPhoneVerificationRequest) returns (ResendHumanPhoneVerificationResponse) {
|
|
option (google.api.http) = {
|
|
post: "/users/{user_id}/phone/_resend_verification"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.write"
|
|
};
|
|
}
|
|
// Removes the avatar number of the human
|
|
rpc RemoveHumanAvatar(RemoveHumanAvatarRequest) returns (RemoveHumanAvatarResponse) {
|
|
option (google.api.http) = {
|
|
delete: "/users/{user_id}/avatar"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.write"
|
|
};
|
|
}
|
|
|
|
// deprecated: use SetHumanPassword
|
|
rpc SetHumanInitialPassword(SetHumanInitialPasswordRequest) returns (SetHumanInitialPasswordResponse) {
|
|
option (google.api.http) = {
|
|
post: "/users/{user_id}/password/_initialize"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.write"
|
|
};
|
|
}
|
|
|
|
// Set a new password for a user, on default the user has to change the password on the next login
|
|
// Set no_change_required to true if the user does not have to change the password on the next login
|
|
rpc SetHumanPassword(SetHumanPasswordRequest) returns (SetHumanPasswordResponse) {
|
|
option (google.api.http) = {
|
|
post: "/users/{user_id}/password"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.write"
|
|
};
|
|
}
|
|
|
|
// An email will be sent to the given address to reset the password of the user
|
|
rpc SendHumanResetPasswordNotification(SendHumanResetPasswordNotificationRequest) returns (SendHumanResetPasswordNotificationResponse) {
|
|
option (google.api.http) = {
|
|
post: "/users/{user_id}/password/_reset"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.write"
|
|
};
|
|
}
|
|
|
|
// Returns a list of all factors (second and multi) which are configured on the user
|
|
rpc ListHumanAuthFactors(ListHumanAuthFactorsRequest) returns (ListHumanAuthFactorsResponse) {
|
|
option (google.api.http) = {
|
|
post: "/users/{user_id}/auth_factors/_search"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.read"
|
|
};
|
|
}
|
|
|
|
// The otp second factor will be removed from the user
|
|
// Because only one otp can be configured per user, the configured one will be removed
|
|
rpc RemoveHumanAuthFactorOTP(RemoveHumanAuthFactorOTPRequest) returns (RemoveHumanAuthFactorOTPResponse) {
|
|
option (google.api.http) = {
|
|
delete: "/users/{user_id}/auth_factors/otp"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.write"
|
|
};
|
|
}
|
|
|
|
// The u2f (universial second factor) will be removed from the user
|
|
rpc RemoveHumanAuthFactorU2F(RemoveHumanAuthFactorU2FRequest) returns (RemoveHumanAuthFactorU2FResponse) {
|
|
option (google.api.http) = {
|
|
delete: "/users/{user_id}/auth_factors/u2f/{token_id}"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.write"
|
|
};
|
|
}
|
|
|
|
// Returns all configured passwordless authenticators
|
|
rpc ListHumanPasswordless(ListHumanPasswordlessRequest) returns (ListHumanPasswordlessResponse) {
|
|
option (google.api.http) = {
|
|
post: "/users/{user_id}/passwordless/_search"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.read"
|
|
};
|
|
}
|
|
|
|
// Adds a new passwordless authenticator link to the user and returns it directly
|
|
// This link enables the user to register a new device if current passwordless devices are all platform authenticators
|
|
// e.g. User has already registered Windows Hello and wants to register FaceID on the iPhone
|
|
rpc AddPasswordlessRegistration(AddPasswordlessRegistrationRequest) returns (AddPasswordlessRegistrationResponse) {
|
|
option (google.api.http) = {
|
|
post: "/users/{user_id}/passwordless/_link"
|
|
};
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.credential.write"
|
|
};
|
|
}
|
|
|
|
// Adds a new passwordless authenticator link to the user and sends it to the registered email address
|
|
// This link enables the user to register a new device if current passwordless devices are all platform authenticators
|
|
// e.g. User has already registered Windows Hello and wants to register FaceID on the iPhone
|
|
rpc SendPasswordlessRegistration(SendPasswordlessRegistrationRequest) returns (SendPasswordlessRegistrationResponse) {
|
|
option (google.api.http) = {
|
|
post: "/users/{user_id}/passwordless/_send_link"
|
|
body: "*"
|
|
};
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.write"
|
|
};
|
|
}
|
|
|
|
// Removed a configured passwordless authenticator
|
|
rpc RemoveHumanPasswordless(RemoveHumanPasswordlessRequest) returns (RemoveHumanPasswordlessResponse) {
|
|
option (google.api.http) = {
|
|
delete: "/users/{user_id}/passwordless/{token_id}"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.write"
|
|
};
|
|
}
|
|
|
|
// Changes a machine user
|
|
rpc UpdateMachine(UpdateMachineRequest) returns (UpdateMachineResponse) {
|
|
option (google.api.http) = {
|
|
put: "/users/{user_id}/machine"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.write"
|
|
};
|
|
}
|
|
|
|
// Returns a machine key of a (machine) user
|
|
rpc GetMachineKeyByIDs(GetMachineKeyByIDsRequest) returns (GetMachineKeyByIDsResponse) {
|
|
option (google.api.http) = {
|
|
get: "/users/{user_id}/keys/{key_id}"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.read"
|
|
};
|
|
}
|
|
|
|
// Returns all machine keys of a (machine) user which match the query
|
|
// Limit should always be set, there is a default limit set by the service
|
|
rpc ListMachineKeys(ListMachineKeysRequest) returns (ListMachineKeysResponse) {
|
|
option (google.api.http) = {
|
|
post: "/users/{user_id}/keys/_search"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.read"
|
|
};
|
|
}
|
|
|
|
// Generates a new machine key, details should be stored after return
|
|
rpc AddMachineKey(AddMachineKeyRequest) returns (AddMachineKeyResponse) {
|
|
option (google.api.http) = {
|
|
post: "/users/{user_id}/keys"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.write"
|
|
};
|
|
}
|
|
|
|
// Removed a machine key
|
|
rpc RemoveMachineKey(RemoveMachineKeyRequest) returns (RemoveMachineKeyResponse) {
|
|
option (google.api.http) = {
|
|
delete: "/users/{user_id}/keys/{key_id}"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.write"
|
|
};
|
|
}
|
|
|
|
// Lists all identity providers (social logins) which a human has configured (e.g Google, Microsoft, AD, etc..)
|
|
// Limit should always be set, there is a default limit set by the service
|
|
rpc ListHumanLinkedIDPs(ListHumanLinkedIDPsRequest) returns (ListHumanLinkedIDPsResponse) {
|
|
option (google.api.http) = {
|
|
post: "/users/{user_id}/idps/_search"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.read"
|
|
};
|
|
}
|
|
|
|
// Removed a configured identity provider (social login) of a human
|
|
rpc RemoveHumanLinkedIDP(RemoveHumanLinkedIDPRequest) returns (RemoveHumanLinkedIDPResponse) {
|
|
option (google.api.http) = {
|
|
delete: "/users/{user_id}/idps/{idp_id}/{linked_user_id}"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.write"
|
|
};
|
|
}
|
|
|
|
// Show all the permissions a user has iin ZITADEL (ZITADEL Manager)
|
|
// Limit should always be set, there is a default limit set by the service
|
|
rpc ListUserMemberships(ListUserMembershipsRequest) returns (ListUserMembershipsResponse) {
|
|
option (google.api.http) = {
|
|
post: "/users/{user_id}/memberships/_search"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.membership.read"
|
|
};
|
|
}
|
|
|
|
// Returns the org given in the header
|
|
rpc GetMyOrg(GetMyOrgRequest) returns (GetMyOrgResponse) {
|
|
option (google.api.http) = {
|
|
get: "/orgs/me"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "org.read"
|
|
};
|
|
}
|
|
|
|
// Search a org over all organisations
|
|
// Domain must match exactly
|
|
rpc GetOrgByDomainGlobal(GetOrgByDomainGlobalRequest) returns (GetOrgByDomainGlobalResponse) {
|
|
option (google.api.http) = {
|
|
get: "/global/orgs/_by_domain"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "org.global.read"
|
|
};
|
|
}
|
|
|
|
// Returns the history of my organisation (each event)
|
|
// Limit should always be set, there is a default limit set by the service
|
|
rpc ListOrgChanges(ListOrgChangesRequest) returns (ListOrgChangesResponse) {
|
|
option (google.api.http) = {
|
|
post: "/orgs/me/changes/_search"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "org.read"
|
|
};
|
|
}
|
|
|
|
// Creates a new organisation
|
|
rpc AddOrg(AddOrgRequest) returns (AddOrgResponse) {
|
|
option (google.api.http) = {
|
|
post: "/orgs"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "org.create"
|
|
};
|
|
}
|
|
|
|
// Changes my organisation
|
|
rpc UpdateOrg(UpdateOrgRequest) returns (UpdateOrgResponse) {
|
|
option (google.api.http) = {
|
|
put: "/orgs/me"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "org.write"
|
|
};
|
|
}
|
|
|
|
// Sets the state of my organisation to deactivated
|
|
// Users of this organisation will not be able login
|
|
rpc DeactivateOrg(DeactivateOrgRequest) returns (DeactivateOrgResponse) {
|
|
option (google.api.http) = {
|
|
post: "/orgs/me/_deactivate"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "org.write"
|
|
};
|
|
}
|
|
|
|
// Sets the state of my organisation to active
|
|
rpc ReactivateOrg(ReactivateOrgRequest) returns (ReactivateOrgResponse) {
|
|
option (google.api.http) = {
|
|
post: "/orgs/me/_reactivate"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "org.write"
|
|
};
|
|
}
|
|
|
|
// Returns all registered domains of my organisation
|
|
// Limit should always be set, there is a default limit set by the service
|
|
rpc ListOrgDomains(ListOrgDomainsRequest) returns (ListOrgDomainsResponse) {
|
|
option (google.api.http) = {
|
|
post: "/orgs/me/domains/_search"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "org.read"
|
|
};
|
|
}
|
|
|
|
// Adds a new domain to my organisation
|
|
rpc AddOrgDomain(AddOrgDomainRequest) returns (AddOrgDomainResponse) {
|
|
option (google.api.http) = {
|
|
post: "/orgs/me/domains"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "org.write"
|
|
feature: "custom_domain"
|
|
};
|
|
}
|
|
|
|
// Removed the domain from my organisation
|
|
rpc RemoveOrgDomain(RemoveOrgDomainRequest) returns (RemoveOrgDomainResponse) {
|
|
option (google.api.http) = {
|
|
delete: "/orgs/me/domains/{domain}"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "org.write"
|
|
};
|
|
}
|
|
|
|
// Generates a new file to validate you domain
|
|
rpc GenerateOrgDomainValidation(GenerateOrgDomainValidationRequest) returns (GenerateOrgDomainValidationResponse) {
|
|
option (google.api.http) = {
|
|
post: "/orgs/me/domains/{domain}/validation/_generate"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "org.write"
|
|
feature: "custom_domain"
|
|
};
|
|
}
|
|
|
|
// Validates your domain with the choosen method
|
|
// Validated domains must be unique
|
|
rpc ValidateOrgDomain(ValidateOrgDomainRequest) returns (ValidateOrgDomainResponse) {
|
|
option (google.api.http) = {
|
|
post: "/orgs/me/domains/{domain}/validation/_validate"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "org.write"
|
|
feature: "custom_domain"
|
|
};
|
|
}
|
|
|
|
// Sets the domain as primary
|
|
// Primary domain is shown as suffix on the preferred username on the users of the organisation
|
|
rpc SetPrimaryOrgDomain(SetPrimaryOrgDomainRequest) returns (SetPrimaryOrgDomainResponse) {
|
|
option (google.api.http) = {
|
|
post: "/orgs/me/domains/{domain}/_set_primary"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "org.write"
|
|
feature: "custom_domain"
|
|
};
|
|
}
|
|
|
|
// Returns all ZITADEL roles which are for organisation managers
|
|
rpc ListOrgMemberRoles(ListOrgMemberRolesRequest) returns (ListOrgMemberRolesResponse) {
|
|
option (google.api.http) = {
|
|
post: "/orgs/members/roles/_search"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "org.member.read"
|
|
};
|
|
}
|
|
|
|
// Returns all ZITADEL managers of this organisation (Project and Project Grant managers not included)
|
|
// Limit should always be set, there is a default limit set by the service
|
|
rpc ListOrgMembers(ListOrgMembersRequest) returns (ListOrgMembersResponse) {
|
|
option (google.api.http) = {
|
|
post: "/orgs/me/members/_search"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "org.member.read"
|
|
};
|
|
}
|
|
|
|
// Adds a new organisation manager, which is allowed to administrate ZITADEL
|
|
rpc AddOrgMember(AddOrgMemberRequest) returns (AddOrgMemberResponse) {
|
|
option (google.api.http) = {
|
|
post: "/orgs/me/members"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "org.member.write"
|
|
};
|
|
}
|
|
|
|
// Changes the organisation manager
|
|
rpc UpdateOrgMember(UpdateOrgMemberRequest) returns (UpdateOrgMemberResponse) {
|
|
option (google.api.http) = {
|
|
put: "/orgs/me/members/{user_id}"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "org.member.write"
|
|
};
|
|
}
|
|
|
|
// Removes an organisation manager
|
|
rpc RemoveOrgMember(RemoveOrgMemberRequest) returns (RemoveOrgMemberResponse) {
|
|
option (google.api.http) = {
|
|
delete: "/orgs/me/members/{user_id}"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "org.member.delete"
|
|
};
|
|
}
|
|
|
|
// Returns a project from my organisation (no granted projects)
|
|
rpc GetProjectByID(GetProjectByIDRequest) returns (GetProjectByIDResponse) {
|
|
option (google.api.http) = {
|
|
get: "/projects/{id}"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.read"
|
|
check_field_name: "Id"
|
|
};
|
|
}
|
|
|
|
// returns a project my organisation got granted from another organisation
|
|
rpc GetGrantedProjectByID(GetGrantedProjectByIDRequest) returns (GetGrantedProjectByIDResponse) {
|
|
option (google.api.http) = {
|
|
get: "/granted_projects/{project_id}/grants/{grant_id}"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.read"
|
|
check_field_name: "GrantId"
|
|
};
|
|
}
|
|
|
|
// Returns all projects my organisation is the owner (no granted projects)
|
|
// Limit should always be set, there is a default limit set by the service
|
|
rpc ListProjects(ListProjectsRequest) returns (ListProjectsResponse) {
|
|
option (google.api.http) = {
|
|
post: "/projects/_search"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.read"
|
|
};
|
|
}
|
|
|
|
// returns all projects my organisation got granted from another organisation
|
|
// Limit should always be set, there is a default limit set by the service
|
|
rpc ListGrantedProjects(ListGrantedProjectsRequest) returns (ListGrantedProjectsResponse) {
|
|
option (google.api.http) = {
|
|
post: "/granted_projects/_search"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.read"
|
|
};
|
|
}
|
|
|
|
// returns all roles of a project grant
|
|
// Limit should always be set, there is a default limit set by the service
|
|
rpc ListGrantedProjectRoles(ListGrantedProjectRolesRequest) returns (ListGrantedProjectRolesResponse) {
|
|
option (google.api.http) = {
|
|
get: "/granted_projects/{project_id}/grants/{grant_id}/roles/_search"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.role.read"
|
|
check_field_name: "GrantId"
|
|
};
|
|
}
|
|
|
|
// Returns the history of the project (each event)
|
|
// Limit should always be set, there is a default limit set by the service
|
|
rpc ListProjectChanges(ListProjectChangesRequest) returns (ListProjectChangesResponse) {
|
|
option (google.api.http) = {
|
|
post: "/projects/{project_id}/changes/_search"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.read"
|
|
};
|
|
}
|
|
|
|
// Adds an new project to the organisation
|
|
rpc AddProject(AddProjectRequest) returns (AddProjectResponse) {
|
|
option (google.api.http) = {
|
|
post: "/projects"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.create"
|
|
};
|
|
}
|
|
|
|
// Changes a project
|
|
rpc UpdateProject(UpdateProjectRequest) returns (UpdateProjectResponse) {
|
|
option (google.api.http) = {
|
|
put: "/projects/{id}"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.write"
|
|
check_field_name: "Id"
|
|
};
|
|
}
|
|
|
|
// Sets the state of a project to deactivated
|
|
// Returns an error if project is already deactivated
|
|
rpc DeactivateProject(DeactivateProjectRequest) returns (DeactivateProjectResponse) {
|
|
option (google.api.http) = {
|
|
post: "/projects/{id}/_deactivate"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.write"
|
|
check_field_name: "Id"
|
|
};
|
|
}
|
|
|
|
// Sets the state of a project to active
|
|
// Returns an error if project is not deactivated
|
|
rpc ReactivateProject(ReactivateProjectRequest) returns (ReactivateProjectResponse) {
|
|
option (google.api.http) = {
|
|
post: "/projects/{id}/_reactivate"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.write"
|
|
check_field_name: "Id"
|
|
};
|
|
}
|
|
|
|
// Removes a project
|
|
// All project grants, applications and user grants for this project will be removed
|
|
rpc RemoveProject(RemoveProjectRequest) returns (RemoveProjectResponse) {
|
|
option (google.api.http) = {
|
|
delete: "/projects/{id}"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.delete"
|
|
check_field_name: "Id"
|
|
};
|
|
}
|
|
|
|
// Returns all roles of a project matching the search query
|
|
// If no limit is requested, default limit will be set, if the limit is higher then the default an error will be returned
|
|
rpc ListProjectRoles(ListProjectRolesRequest) returns (ListProjectRolesResponse) {
|
|
option (google.api.http) = {
|
|
post: "/projects/{project_id}/roles/_search"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.role.read"
|
|
check_field_name: "ProjectId"
|
|
};
|
|
}
|
|
|
|
// Adds a role to a project, key must be unique in the project
|
|
rpc AddProjectRole(AddProjectRoleRequest) returns (AddProjectRoleResponse) {
|
|
option (google.api.http) = {
|
|
post: "/projects/{project_id}/roles"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.role.write"
|
|
check_field_name: "ProjectId"
|
|
};
|
|
}
|
|
|
|
// add a list of project roles in one request
|
|
rpc BulkAddProjectRoles(BulkAddProjectRolesRequest) returns (BulkAddProjectRolesResponse) {
|
|
option (google.api.http) = {
|
|
post: "/projects/{project_id}/roles/_bulk"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.role.write"
|
|
check_field_name: "ProjectId"
|
|
};
|
|
}
|
|
|
|
// Changes a project role, key is not editable
|
|
// If a key should change, remove the role and create a new
|
|
rpc UpdateProjectRole(UpdateProjectRoleRequest) returns (UpdateProjectRoleResponse) {
|
|
option (google.api.http) = {
|
|
put: "/projects/{project_id}/roles/{role_key}"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.role.write"
|
|
check_field_name: "ProjectId"
|
|
};
|
|
}
|
|
|
|
// Removes role from UserGrants, ProjectGrants and from Project
|
|
rpc RemoveProjectRole(RemoveProjectRoleRequest) returns (RemoveProjectRoleResponse) {
|
|
option (google.api.http) = {
|
|
delete: "/projects/{project_id}/roles/{role_key}"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.role.delete"
|
|
check_field_name: "ProjectId"
|
|
};
|
|
}
|
|
|
|
// Returns all ZITADEL roles which are for project managers
|
|
rpc ListProjectMemberRoles(ListProjectMemberRolesRequest) returns (ListProjectMemberRolesResponse) {
|
|
option (google.api.http) = {
|
|
post: "/projects/members/roles/_search"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.member.read"
|
|
};
|
|
}
|
|
|
|
// Returns all ZITADEL managers of a projects
|
|
// Limit should always be set, there is a default limit set by the service
|
|
rpc ListProjectMembers(ListProjectMembersRequest) returns (ListProjectMembersResponse) {
|
|
option (google.api.http) = {
|
|
post: "/projects/{project_id}/members/_search"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.member.read"
|
|
check_field_name: "ProjectId"
|
|
};
|
|
}
|
|
|
|
// Adds a new project manager, which is allowed to administrate in ZITADEL
|
|
rpc AddProjectMember(AddProjectMemberRequest) returns (AddProjectMemberResponse) {
|
|
option (google.api.http) = {
|
|
post: "/projects/{project_id}/members"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.member.write"
|
|
check_field_name: "ProjectId"
|
|
};
|
|
}
|
|
|
|
// Change project manager, which is allowed to administrate in ZITADEL
|
|
rpc UpdateProjectMember(UpdateProjectMemberRequest) returns (UpdateProjectMemberResponse) {
|
|
option (google.api.http) = {
|
|
put: "/projects/{project_id}/members/{user_id}"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.member.write"
|
|
check_field_name: "ProjectId"
|
|
};
|
|
}
|
|
|
|
// Remove project manager, which is allowed to administrate in ZITADEL
|
|
rpc RemoveProjectMember(RemoveProjectMemberRequest) returns (RemoveProjectMemberResponse) {
|
|
option (google.api.http) = {
|
|
delete: "/projects/{project_id}/members/{user_id}"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.member.delete"
|
|
check_field_name: "ProjectId"
|
|
};
|
|
}
|
|
|
|
// Returns an application (oidc or api)
|
|
rpc GetAppByID(GetAppByIDRequest) returns (GetAppByIDResponse) {
|
|
option (google.api.http) = {
|
|
get: "/projects/{project_id}/apps/{app_id}"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.app.read"
|
|
check_field_name: "ProjectId"
|
|
};
|
|
}
|
|
|
|
// Returns all applications of a project matching the query
|
|
// Limit should always be set, there is a default limit set by the service
|
|
rpc ListApps(ListAppsRequest) returns (ListAppsResponse) {
|
|
option (google.api.http) = {
|
|
post: "/projects/{project_id}/apps/_search"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.app.read"
|
|
check_field_name: "ProjectId"
|
|
};
|
|
}
|
|
|
|
// Returns the history of the application (each event)
|
|
// Limit should always be set, there is a default limit set by the service
|
|
rpc ListAppChanges(ListAppChangesRequest) returns (ListAppChangesResponse) {
|
|
option (google.api.http) = {
|
|
post: "/projects/{project_id}/apps/{app_id}/changes/_search"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.app.read"
|
|
check_field_name: "ProjectId"
|
|
};
|
|
}
|
|
|
|
// Adds a new oidc client
|
|
// Returns a client id
|
|
// Returns a new generated secret if needed (Depending on the configuration)
|
|
rpc AddOIDCApp(AddOIDCAppRequest) returns (AddOIDCAppResponse) {
|
|
option (google.api.http) = {
|
|
post: "/projects/{project_id}/apps/oidc"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.app.write"
|
|
check_field_name: "ProjectId"
|
|
};
|
|
}
|
|
|
|
// Adds a new api application
|
|
// Returns a client id
|
|
// Returns a new generated secret if needed (Depending on the configuration)
|
|
rpc AddAPIApp(AddAPIAppRequest) returns (AddAPIAppResponse) {
|
|
option (google.api.http) = {
|
|
post: "/projects/{project_id}/apps/api"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.app.write"
|
|
check_field_name: "ProjectId"
|
|
};
|
|
}
|
|
|
|
// Changes application
|
|
rpc UpdateApp(UpdateAppRequest) returns (UpdateAppResponse) {
|
|
option (google.api.http) = {
|
|
put: "/projects/{project_id}/apps/{app_id}"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.app.write"
|
|
check_field_name: "ProjectId"
|
|
};
|
|
}
|
|
|
|
// Changes the configuration of the oidc client
|
|
rpc UpdateOIDCAppConfig(UpdateOIDCAppConfigRequest) returns (UpdateOIDCAppConfigResponse) {
|
|
option (google.api.http) = {
|
|
put: "/projects/{project_id}/apps/{app_id}/oidc_config"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.app.write"
|
|
check_field_name: "ProjectId"
|
|
};
|
|
}
|
|
|
|
// Changes the configuration of the api application
|
|
rpc UpdateAPIAppConfig(UpdateAPIAppConfigRequest) returns (UpdateAPIAppConfigResponse) {
|
|
option (google.api.http) = {
|
|
put: "/projects/{project_id}/apps/{app_id}/api_config"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.app.write"
|
|
check_field_name: "ProjectId"
|
|
};
|
|
}
|
|
|
|
// Set the state to deactivated
|
|
// Its not possible to request tokens for deactivated apps
|
|
// Returns an error if already deactivated
|
|
rpc DeactivateApp(DeactivateAppRequest) returns (DeactivateAppResponse) {
|
|
option (google.api.http) = {
|
|
post: "/projects/{project_id}/apps/{app_id}/_deactivate"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.app.write"
|
|
check_field_name: "ProjectId"
|
|
};
|
|
}
|
|
|
|
// Set the state to active
|
|
// Returns an error if not deactivated
|
|
rpc ReactivateApp(ReactivateAppRequest) returns (ReactivateAppResponse) {
|
|
option (google.api.http) = {
|
|
post: "/projects/{project_id}/apps/{app_id}/_reactivate"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.app.write"
|
|
check_field_name: "ProjectId"
|
|
};
|
|
}
|
|
|
|
// Removed the application
|
|
rpc RemoveApp(RemoveAppRequest) returns (RemoveAppResponse) {
|
|
option (google.api.http) = {
|
|
delete: "/projects/{project_id}/apps/{app_id}"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.app.delete"
|
|
check_field_name: "ProjectId"
|
|
};
|
|
}
|
|
|
|
// Generates a new client secret for the oidc client, make sure to save the response
|
|
rpc RegenerateOIDCClientSecret(RegenerateOIDCClientSecretRequest) returns (RegenerateOIDCClientSecretResponse) {
|
|
option (google.api.http) = {
|
|
post: "/projects/{project_id}/apps/{app_id}/oidc_config/_generate_client_secret"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.app.write"
|
|
check_field_name: "ProjectId"
|
|
};
|
|
}
|
|
|
|
// Generates a new client secret for the api application, make sure to save the response
|
|
rpc RegenerateAPIClientSecret(RegenerateAPIClientSecretRequest) returns (RegenerateAPIClientSecretResponse) {
|
|
option (google.api.http) = {
|
|
post: "/projects/{project_id}/apps/{app_id}/api_config/_generate_client_secret"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.app.write"
|
|
check_field_name: "ProjectId"
|
|
};
|
|
}
|
|
|
|
// Returns an application key
|
|
rpc GetAppKey(GetAppKeyRequest) returns (GetAppKeyResponse) {
|
|
option (google.api.http) = {
|
|
get: "/projects/{project_id}/apps/{app_id}/keys/{key_id}"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.app.read"
|
|
check_field_name: "ProjectId"
|
|
};
|
|
}
|
|
|
|
// Returns all application keys matching the result
|
|
// Limit should always be set, there is a default limit set by the service
|
|
rpc ListAppKeys(ListAppKeysRequest) returns (ListAppKeysResponse) {
|
|
option (google.api.http) = {
|
|
post: "/projects/{project_id}/apps/{app_id}/keys/_search"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.app.read"
|
|
check_field_name: "ProjectId"
|
|
};
|
|
}
|
|
|
|
// Creates a new app key
|
|
// Will return key details in result, make sure to save it
|
|
rpc AddAppKey(AddAppKeyRequest) returns (AddAppKeyResponse){
|
|
option (google.api.http) = {
|
|
post: "/projects/{project_id}/apps/{app_id}/keys"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.app.write"
|
|
check_field_name: "ProjectId"
|
|
};
|
|
}
|
|
|
|
// Removes an app key
|
|
rpc RemoveAppKey(RemoveAppKeyRequest) returns (RemoveAppKeyResponse) {
|
|
option (google.api.http) = {
|
|
delete: "/projects/{project_id}/apps/{app_id}/keys/{key_id}"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.app.write"
|
|
check_field_name: "ProjectId"
|
|
};
|
|
}
|
|
|
|
// Returns a project grant (ProjectGrant = Grant another organisation for my project)
|
|
rpc GetProjectGrantByID(GetProjectGrantByIDRequest) returns (GetProjectGrantByIDResponse) {
|
|
option (google.api.http) = {
|
|
get: "/projects/{project_id}/grants/{grant_id}"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.grant.read"
|
|
};
|
|
}
|
|
|
|
// Returns all project grants matching the query, (ProjectGrant = Grant another organisation for my project)
|
|
// Limit should always be set, there is a default limit set by the service
|
|
rpc ListProjectGrants(ListProjectGrantsRequest) returns (ListProjectGrantsResponse) {
|
|
option (google.api.http) = {
|
|
post: "/projects/{project_id}/grants/_search"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.grant.read"
|
|
check_field_name: "ProjectId"
|
|
};
|
|
}
|
|
|
|
// Returns all project grants matching the query, (ProjectGrant = Grant another organisation for my project)
|
|
// Limit should always be set, there is a default limit set by the service
|
|
rpc ListAllProjectGrants(ListAllProjectGrantsRequest) returns (ListAllProjectGrantsResponse) {
|
|
option (google.api.http) = {
|
|
post: "/projectgrants/_search"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.grant.read"
|
|
};
|
|
}
|
|
|
|
// Add a new project grant (ProjectGrant = Grant another organisation for my project)
|
|
// Project Grant will be listed in granted project of the other organisation
|
|
rpc AddProjectGrant(AddProjectGrantRequest) returns (AddProjectGrantResponse) {
|
|
option (google.api.http) = {
|
|
post: "/projects/{project_id}/grants"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.grant.write"
|
|
};
|
|
}
|
|
|
|
// Change project grant (ProjectGrant = Grant another organisation for my project)
|
|
// Project Grant will be listed in granted project of the other organisation
|
|
rpc UpdateProjectGrant(UpdateProjectGrantRequest) returns (UpdateProjectGrantResponse) {
|
|
option (google.api.http) = {
|
|
put: "/projects/{project_id}/grants/{grant_id}"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.grant.write"
|
|
};
|
|
}
|
|
|
|
// Set state of project grant to deactivated (ProjectGrant = Grant another organisation for my project)
|
|
// Returns error if project not active
|
|
rpc DeactivateProjectGrant(DeactivateProjectGrantRequest) returns (DeactivateProjectGrantResponse) {
|
|
option (google.api.http) = {
|
|
post: "/projects/{project_id}/grants/{grant_id}/_deactivate"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.grant.write"
|
|
};
|
|
}
|
|
|
|
// Set state of project grant to active (ProjectGrant = Grant another organisation for my project)
|
|
// Returns error if project not deactivated
|
|
rpc ReactivateProjectGrant(ReactivateProjectGrantRequest) returns (ReactivateProjectGrantResponse) {
|
|
option (google.api.http) = {
|
|
post: "/projects/{project_id}/grants/{grant_id}/_reactivate"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.grant.write"
|
|
};
|
|
}
|
|
|
|
// Removes project grant and all user grants for this project grant
|
|
rpc RemoveProjectGrant(RemoveProjectGrantRequest) returns (RemoveProjectGrantResponse) {
|
|
option (google.api.http) = {
|
|
delete: "/projects/{project_id}/grants/{grant_id}"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.grant.delete"
|
|
};
|
|
}
|
|
|
|
// Returns all ZITADEL roles which are for project grant managers
|
|
rpc ListProjectGrantMemberRoles(ListProjectGrantMemberRolesRequest) returns (ListProjectGrantMemberRolesResponse) {
|
|
option (google.api.http) = {
|
|
post: "/projects/grants/members/roles/_search"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.grant.member.read"
|
|
};
|
|
}
|
|
|
|
// Returns all ZITADEL managers of this project grant
|
|
// Limit should always be set, there is a default limit set by the service
|
|
rpc ListProjectGrantMembers(ListProjectGrantMembersRequest) returns (ListProjectGrantMembersResponse) {
|
|
option (google.api.http) = {
|
|
post: "/projects/{project_id}/grants/{grant_id}/members/_search"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.grant.member.read"
|
|
};
|
|
}
|
|
|
|
// Adds a new project grant manager, which is allowed to administrate in ZITADEL
|
|
rpc AddProjectGrantMember(AddProjectGrantMemberRequest) returns (AddProjectGrantMemberResponse) {
|
|
option (google.api.http) = {
|
|
post: "/projects/{project_id}/grants/{grant_id}/members"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.grant.member.write"
|
|
};
|
|
}
|
|
|
|
// Changes project grant manager, which is allowed to administrate in ZITADEL
|
|
rpc UpdateProjectGrantMember(UpdateProjectGrantMemberRequest) returns (UpdateProjectGrantMemberResponse) {
|
|
option (google.api.http) = {
|
|
put: "/projects/{project_id}/grants/{grant_id}/members/{user_id}"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.grant.member.write"
|
|
};
|
|
}
|
|
|
|
// Removed project grant manager
|
|
rpc RemoveProjectGrantMember(RemoveProjectGrantMemberRequest) returns (RemoveProjectGrantMemberResponse) {
|
|
option (google.api.http) = {
|
|
delete: "/projects/{project_id}/grants/{grant_id}/members/{user_id}"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "project.grant.member.delete"
|
|
};
|
|
}
|
|
|
|
// Returns a user grant (authorization of a user for a project)
|
|
rpc GetUserGrantByID(GetUserGrantByIDRequest) returns (GetUserGrantByIDResponse) {
|
|
option (google.api.http) = {
|
|
get: "/users/{user_id}/grants/{grant_id}"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.grant.read"
|
|
};
|
|
}
|
|
|
|
// Returns al user grant matching the query (authorizations of user for projects)
|
|
// Limit should always be set, there is a default limit set by the service
|
|
rpc ListUserGrants(ListUserGrantRequest) returns (ListUserGrantResponse) {
|
|
option (google.api.http) = {
|
|
post: "/users/grants/_search"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.grant.read"
|
|
};
|
|
}
|
|
|
|
// Creates a new user grant (authorization of a user for a project with specified roles)
|
|
rpc AddUserGrant(AddUserGrantRequest) returns (AddUserGrantResponse) {
|
|
option (google.api.http) = {
|
|
post: "/users/{user_id}/grants"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.grant.write"
|
|
};
|
|
}
|
|
|
|
// Changes a user grant (authorization of a user for a project with specified roles)
|
|
rpc UpdateUserGrant(UpdateUserGrantRequest) returns (UpdateUserGrantResponse) {
|
|
option (google.api.http) = {
|
|
put: "/users/{user_id}/grants/{grant_id}"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.grant.write"
|
|
};
|
|
}
|
|
|
|
// Sets the state of a user grant to deactivated
|
|
// User will not be able to use the granted project anymore
|
|
// Returns an error if user grant is already deactivated
|
|
rpc DeactivateUserGrant(DeactivateUserGrantRequest) returns (DeactivateUserGrantResponse) {
|
|
option (google.api.http) = {
|
|
post: "/users/{user_id}/grants/{grant_id}/_deactivate"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.grant.write"
|
|
};
|
|
}
|
|
|
|
// Sets the state of a user grant to active
|
|
// Returns an error if user grant is not deactivated
|
|
rpc ReactivateUserGrant(ReactivateUserGrantRequest) returns (ReactivateUserGrantResponse) {
|
|
option (google.api.http) = {
|
|
post: "/users/{user_id}/grants/{grant_id}/_reactivate"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.grant.write"
|
|
};
|
|
}
|
|
|
|
// Removes a user grant
|
|
rpc RemoveUserGrant(RemoveUserGrantRequest) returns (RemoveUserGrantResponse) {
|
|
option (google.api.http) = {
|
|
delete: "/users/{user_id}/grants/{grant_id}"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.grant.delete"
|
|
};
|
|
}
|
|
|
|
// remove a list of user grants in one request
|
|
rpc BulkRemoveUserGrant(BulkRemoveUserGrantRequest) returns (BulkRemoveUserGrantResponse) {
|
|
option (google.api.http) = {
|
|
delete: "/user_grants/_bulk"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "user.grant.delete"
|
|
};
|
|
}
|
|
|
|
rpc GetFeatures(GetFeaturesRequest) returns (GetFeaturesResponse) {
|
|
option (google.api.http) = {
|
|
get: "/features"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "features.read"
|
|
};
|
|
}
|
|
|
|
// Returns the org iam policy (this policy is managed by the iam administrator)
|
|
rpc GetOrgIAMPolicy(GetOrgIAMPolicyRequest) returns (GetOrgIAMPolicyResponse) {
|
|
option (google.api.http) = {
|
|
get: "/policies/orgiam"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "authenticated"
|
|
};
|
|
}
|
|
|
|
// Returns the login policy of the organisation
|
|
// With this policy the login gui can be configured
|
|
rpc GetLoginPolicy(GetLoginPolicyRequest) returns (GetLoginPolicyResponse) {
|
|
option (google.api.http) = {
|
|
get: "/policies/login"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.read"
|
|
};
|
|
}
|
|
|
|
// Returns the default login policy configured in the IAM
|
|
rpc GetDefaultLoginPolicy(GetDefaultLoginPolicyRequest) returns (GetDefaultLoginPolicyResponse) {
|
|
option (google.api.http) = {
|
|
get: "/policies/default/login"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.read"
|
|
};
|
|
}
|
|
|
|
// Add a custom login policy for the organisation
|
|
// With this policy the login gui can be configured
|
|
rpc AddCustomLoginPolicy(AddCustomLoginPolicyRequest) returns (AddCustomLoginPolicyResponse) {
|
|
option (google.api.http) = {
|
|
post: "/policies/login"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.write"
|
|
feature: "login_policy"
|
|
};
|
|
}
|
|
|
|
// Change the custom login policy for the organisation
|
|
// With this policy the login gui can be configured
|
|
rpc UpdateCustomLoginPolicy(UpdateCustomLoginPolicyRequest) returns (UpdateCustomLoginPolicyResponse) {
|
|
option (google.api.http) = {
|
|
put: "/policies/login"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.write"
|
|
feature: "login_policy"
|
|
};
|
|
}
|
|
|
|
// Removes the custom login policy of the organisation
|
|
// The default policy of the IAM will trigger after
|
|
rpc ResetLoginPolicyToDefault(ResetLoginPolicyToDefaultRequest) returns (ResetLoginPolicyToDefaultResponse) {
|
|
option (google.api.http) = {
|
|
delete: "/policies/login"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.delete"
|
|
};
|
|
}
|
|
|
|
// Lists all possible identity providers configured on the organisation
|
|
// Limit should always be set, there is a default limit set by the service
|
|
rpc ListLoginPolicyIDPs(ListLoginPolicyIDPsRequest) returns (ListLoginPolicyIDPsResponse) {
|
|
option (google.api.http) = {
|
|
post: "/policies/login/idps/_search"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.read"
|
|
};
|
|
}
|
|
|
|
// Add a (preconfigured) identity provider to the custom login policy
|
|
rpc AddIDPToLoginPolicy(AddIDPToLoginPolicyRequest) returns (AddIDPToLoginPolicyResponse) {
|
|
option (google.api.http) = {
|
|
post: "/policies/login/idps"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.write"
|
|
feature: "login_policy.idp"
|
|
};
|
|
}
|
|
|
|
// Remove a identity provider from the custom login policy
|
|
rpc RemoveIDPFromLoginPolicy(RemoveIDPFromLoginPolicyRequest) returns (RemoveIDPFromLoginPolicyResponse) {
|
|
option (google.api.http) = {
|
|
delete: "/policies/login/idps/{idp_id}"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.write"
|
|
feature: "login_policy.idp"
|
|
};
|
|
}
|
|
|
|
// Returns all configured second factors of the custom login policy
|
|
rpc ListLoginPolicySecondFactors(ListLoginPolicySecondFactorsRequest) returns (ListLoginPolicySecondFactorsResponse) {
|
|
option (google.api.http) = {
|
|
post: "/policies/login/second_factors/_search"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.read"
|
|
};
|
|
}
|
|
|
|
// Adds a new second factor to the custom login policy
|
|
rpc AddSecondFactorToLoginPolicy(AddSecondFactorToLoginPolicyRequest) returns (AddSecondFactorToLoginPolicyResponse) {
|
|
option (google.api.http) = {
|
|
post: "/policies/login/second_factors"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.write"
|
|
feature: "login_policy.factors"
|
|
};
|
|
}
|
|
|
|
// Remove a second factor from the custom login policy
|
|
rpc RemoveSecondFactorFromLoginPolicy(RemoveSecondFactorFromLoginPolicyRequest) returns (RemoveSecondFactorFromLoginPolicyResponse) {
|
|
option (google.api.http) = {
|
|
delete: "/policies/login/second_factors/{type}"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.write"
|
|
feature: "login_policy.factors"
|
|
};
|
|
}
|
|
|
|
// Returns all configured multi factors of the custom login policy
|
|
rpc ListLoginPolicyMultiFactors(ListLoginPolicyMultiFactorsRequest) returns (ListLoginPolicyMultiFactorsResponse) {
|
|
option (google.api.http) = {
|
|
post: "/policies/login/auth_factors/_search"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.read"
|
|
};
|
|
}
|
|
|
|
// Adds a new multi factor to the custom login policy
|
|
rpc AddMultiFactorToLoginPolicy(AddMultiFactorToLoginPolicyRequest) returns (AddMultiFactorToLoginPolicyResponse) {
|
|
option (google.api.http) = {
|
|
post: "/policies/login/multi_factors"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.write"
|
|
feature: "login_policy.factors"
|
|
};
|
|
}
|
|
|
|
// Remove a multi factor from the custom login policy
|
|
rpc RemoveMultiFactorFromLoginPolicy(RemoveMultiFactorFromLoginPolicyRequest) returns (RemoveMultiFactorFromLoginPolicyResponse) {
|
|
option (google.api.http) = {
|
|
delete: "/policies/login/multi_factors/{type}"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.write"
|
|
feature: "login_policy.factors"
|
|
};
|
|
}
|
|
|
|
// Returns the password complexity policy of the organisation
|
|
// With this policy the password strength can be configured
|
|
rpc GetPasswordComplexityPolicy(GetPasswordComplexityPolicyRequest) returns (GetPasswordComplexityPolicyResponse) {
|
|
option (google.api.http) = {
|
|
get: "/policies/password/complexity"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.read"
|
|
};
|
|
}
|
|
|
|
// Returns the default password complexity policy of the IAM
|
|
// With this policy the password strength can be configured
|
|
rpc GetDefaultPasswordComplexityPolicy(GetDefaultPasswordComplexityPolicyRequest) returns (GetDefaultPasswordComplexityPolicyResponse) {
|
|
option (google.api.http) = {
|
|
get: "/policies/default/password/complexity"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.read"
|
|
};
|
|
}
|
|
|
|
// Add a custom password complexity policy for the organisation
|
|
// With this policy the password strength can be configured
|
|
rpc AddCustomPasswordComplexityPolicy(AddCustomPasswordComplexityPolicyRequest) returns (AddCustomPasswordComplexityPolicyResponse) {
|
|
option (google.api.http) = {
|
|
post: "/policies/password/complexity"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.write"
|
|
feature: "password_complexity_policy"
|
|
};
|
|
}
|
|
|
|
// Update the custom password complexity policy for the organisation
|
|
// With this policy the password strength can be configured
|
|
rpc UpdateCustomPasswordComplexityPolicy(UpdateCustomPasswordComplexityPolicyRequest) returns (UpdateCustomPasswordComplexityPolicyResponse) {
|
|
option (google.api.http) = {
|
|
put: "/policies/password/complexity"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.write"
|
|
feature: "password_complexity_policy"
|
|
};
|
|
}
|
|
|
|
// Removes the custom password complexity policy of the organisation
|
|
// The default policy of the IAM will trigger after
|
|
rpc ResetPasswordComplexityPolicyToDefault(ResetPasswordComplexityPolicyToDefaultRequest) returns (ResetPasswordComplexityPolicyToDefaultResponse) {
|
|
option (google.api.http) = {
|
|
delete: "/policies/password/complexity"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.delete"
|
|
};
|
|
}
|
|
|
|
// The password age policy is not used at the moment
|
|
rpc GetPasswordAgePolicy(GetPasswordAgePolicyRequest) returns (GetPasswordAgePolicyResponse) {
|
|
option (google.api.http) = {
|
|
get: "/policies/password/age"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.read"
|
|
};
|
|
}
|
|
|
|
// The password age policy is not used at the moment
|
|
rpc GetDefaultPasswordAgePolicy(GetDefaultPasswordAgePolicyRequest) returns (GetDefaultPasswordAgePolicyResponse) {
|
|
option (google.api.http) = {
|
|
get: "/policies/default/password/age"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.read"
|
|
};
|
|
}
|
|
|
|
// The password age policy is not used at the moment
|
|
rpc AddCustomPasswordAgePolicy(AddCustomPasswordAgePolicyRequest) returns (AddCustomPasswordAgePolicyResponse) {
|
|
option (google.api.http) = {
|
|
post: "/policies/password/age"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.write"
|
|
};
|
|
}
|
|
|
|
// The password age policy is not used at the moment
|
|
rpc UpdateCustomPasswordAgePolicy(UpdateCustomPasswordAgePolicyRequest) returns (UpdateCustomPasswordAgePolicyResponse) {
|
|
option (google.api.http) = {
|
|
put: "/policies/password/age"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.write"
|
|
};
|
|
}
|
|
|
|
// The password age policy is not used at the moment
|
|
rpc ResetPasswordAgePolicyToDefault(ResetPasswordAgePolicyToDefaultRequest) returns (ResetPasswordAgePolicyToDefaultResponse) {
|
|
option (google.api.http) = {
|
|
delete: "/policies/password/age"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.delete"
|
|
};
|
|
}
|
|
|
|
rpc GetLockoutPolicy(GetLockoutPolicyRequest) returns (GetLockoutPolicyResponse) {
|
|
option (google.api.http) = {
|
|
get: "/policies/lockout"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.read"
|
|
};
|
|
}
|
|
|
|
rpc GetDefaultLockoutPolicy(GetDefaultLockoutPolicyRequest) returns (GetDefaultLockoutPolicyResponse) {
|
|
option (google.api.http) = {
|
|
get: "/policies/default/lockout"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.read"
|
|
};
|
|
}
|
|
|
|
rpc AddCustomLockoutPolicy(AddCustomLockoutPolicyRequest) returns (AddCustomLockoutPolicyResponse) {
|
|
option (google.api.http) = {
|
|
post: "/policies/lockout"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.write"
|
|
};
|
|
}
|
|
|
|
rpc UpdateCustomLockoutPolicy(UpdateCustomLockoutPolicyRequest) returns (UpdateCustomLockoutPolicyResponse) {
|
|
option (google.api.http) = {
|
|
put: "/policies/lockout"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.write"
|
|
};
|
|
}
|
|
|
|
rpc ResetLockoutPolicyToDefault(ResetLockoutPolicyToDefaultRequest) returns (ResetLockoutPolicyToDefaultResponse) {
|
|
option (google.api.http) = {
|
|
delete: "/policies/lockout"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.delete"
|
|
};
|
|
}
|
|
|
|
// Returns the privacy policy of the organisation
|
|
// With this policy privacy relevant things can be configured (e.g. tos link)
|
|
rpc GetPrivacyPolicy(GetPrivacyPolicyRequest) returns (GetPrivacyPolicyResponse) {
|
|
option (google.api.http) = {
|
|
get: "/policies/privacy"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.read"
|
|
};
|
|
}
|
|
|
|
// Returns the default privacy policy of the IAM
|
|
// With this policy the privacy relevant things can be configured (e.g tos link)
|
|
rpc GetDefaultPrivacyPolicy(GetDefaultPrivacyPolicyRequest) returns (GetDefaultPrivacyPolicyResponse) {
|
|
option (google.api.http) = {
|
|
get: "/policies/default/privacy"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.read"
|
|
};
|
|
}
|
|
|
|
// Add a custom privacy policy for the organisation
|
|
// With this policy privacy relevant things can be configured (e.g. tos link)
|
|
rpc AddCustomPrivacyPolicy(AddCustomPrivacyPolicyRequest) returns (AddCustomPrivacyPolicyResponse) {
|
|
option (google.api.http) = {
|
|
post: "/policies/privacy"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.write"
|
|
feature: "privacy_policy"
|
|
};
|
|
}
|
|
|
|
// Update the privacy complexity policy for the organisation
|
|
// With this policy privacy relevant things can be configured (e.g. tos link)
|
|
rpc UpdateCustomPrivacyPolicy(UpdateCustomPrivacyPolicyRequest) returns (UpdateCustomPrivacyPolicyResponse) {
|
|
option (google.api.http) = {
|
|
put: "/policies/privacy"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.write"
|
|
feature: "privacy_policy"
|
|
};
|
|
}
|
|
|
|
// Removes the privacy policy of the organisation
|
|
// The default policy of the IAM will trigger after
|
|
rpc ResetPrivacyPolicyToDefault(ResetPrivacyPolicyToDefaultRequest) returns (ResetPrivacyPolicyToDefaultResponse) {
|
|
option (google.api.http) = {
|
|
delete: "/policies/privacy"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.delete"
|
|
};
|
|
}
|
|
|
|
// Returns the active label policy of the organisation
|
|
// With this policy the private labeling can be configured (colors, etc.)
|
|
rpc GetLabelPolicy(GetLabelPolicyRequest) returns (GetLabelPolicyResponse) {
|
|
option (google.api.http) = {
|
|
get: "/policies/label"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.read"
|
|
};
|
|
}
|
|
|
|
// Returns the preview label policy of the organisation
|
|
// With this policy the private labeling can be configured (colors, etc.)
|
|
rpc GetPreviewLabelPolicy(GetPreviewLabelPolicyRequest) returns (GetPreviewLabelPolicyResponse) {
|
|
option (google.api.http) = {
|
|
get: "/policies/label/_preview"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.read"
|
|
};
|
|
}
|
|
|
|
// Returns the default label policy of the IAM
|
|
// With this policy the private labeling can be configured (colors, etc.)
|
|
rpc GetDefaultLabelPolicy(GetDefaultLabelPolicyRequest) returns (GetDefaultLabelPolicyResponse) {
|
|
option (google.api.http) = {
|
|
get: "/policies/default/label"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.read"
|
|
feature: "label_policy"
|
|
};
|
|
}
|
|
|
|
// Add a custom label policy for the organisation
|
|
// With this policy the private labeling can be configured (colors, etc.)
|
|
rpc AddCustomLabelPolicy(AddCustomLabelPolicyRequest) returns (AddCustomLabelPolicyResponse) {
|
|
option (google.api.http) = {
|
|
post: "/policies/label"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.write"
|
|
feature: "label_policy"
|
|
};
|
|
}
|
|
|
|
// Changes the custom label policy for the organisation
|
|
// With this policy the private labeling can be configured (colors, etc.)
|
|
rpc UpdateCustomLabelPolicy(UpdateCustomLabelPolicyRequest) returns (UpdateCustomLabelPolicyResponse) {
|
|
option (google.api.http) = {
|
|
put: "/policies/label"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.write"
|
|
feature: "label_policy"
|
|
};
|
|
}
|
|
|
|
// Activates all changes of the label policy
|
|
rpc ActivateCustomLabelPolicy(ActivateCustomLabelPolicyRequest) returns (ActivateCustomLabelPolicyResponse) {
|
|
option (google.api.http) = {
|
|
post: "/policies/label/_activate"
|
|
body: "*"
|
|
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.write"
|
|
feature: "label_policy"
|
|
};
|
|
}
|
|
|
|
// Removes the logo of the label policy
|
|
rpc RemoveCustomLabelPolicyLogo(RemoveCustomLabelPolicyLogoRequest) returns (RemoveCustomLabelPolicyLogoResponse) {
|
|
option (google.api.http) = {
|
|
delete: "/policies/label/logo"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.write"
|
|
feature: "label_policy"
|
|
};
|
|
}
|
|
|
|
// Removes the logo dark of the label policy
|
|
rpc RemoveCustomLabelPolicyLogoDark(RemoveCustomLabelPolicyLogoDarkRequest) returns (RemoveCustomLabelPolicyLogoDarkResponse) {
|
|
option (google.api.http) = {
|
|
delete: "/policies/label/logo_dark"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.write"
|
|
feature: "label_policy"
|
|
};
|
|
}
|
|
|
|
// Removes the icon of the label policy
|
|
rpc RemoveCustomLabelPolicyIcon(RemoveCustomLabelPolicyIconRequest) returns (RemoveCustomLabelPolicyIconResponse) {
|
|
option (google.api.http) = {
|
|
delete: "/policies/label/icon"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.write"
|
|
feature: "label_policy"
|
|
};
|
|
}
|
|
|
|
// Removes the logo dark of the label policy
|
|
rpc RemoveCustomLabelPolicyIconDark(RemoveCustomLabelPolicyIconDarkRequest) returns (RemoveCustomLabelPolicyIconDarkResponse) {
|
|
option (google.api.http) = {
|
|
delete: "/policies/label/icon_dark"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.write"
|
|
feature: "label_policy"
|
|
};
|
|
}
|
|
|
|
// Removes the font of the label policy
|
|
rpc RemoveCustomLabelPolicyFont(RemoveCustomLabelPolicyFontRequest) returns (RemoveCustomLabelPolicyFontResponse) {
|
|
option (google.api.http) = {
|
|
delete: "/policies/label/font"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.write"
|
|
feature: "label_policy"
|
|
};
|
|
}
|
|
|
|
// Removes the custom label policy of the organisation
|
|
// The default policy of the IAM will trigger after
|
|
rpc ResetLabelPolicyToDefault(ResetLabelPolicyToDefaultRequest) returns (ResetLabelPolicyToDefaultResponse) {
|
|
option (google.api.http) = {
|
|
delete: "/policies/label"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.delete"
|
|
};
|
|
}
|
|
|
|
//Returns the custom text for initial message
|
|
rpc GetCustomInitMessageText(GetCustomInitMessageTextRequest) returns (GetCustomInitMessageTextResponse) {
|
|
option (google.api.http) = {
|
|
get: "/text/message/init/{language}";
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.read";
|
|
};
|
|
}
|
|
|
|
//Returns the default text for initial message
|
|
rpc GetDefaultInitMessageText(GetDefaultInitMessageTextRequest) returns (GetDefaultInitMessageTextResponse) {
|
|
option (google.api.http) = {
|
|
get: "/text/default/message/init/{language}";
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.read";
|
|
};
|
|
}
|
|
|
|
// Sets the custom text for initial message
|
|
// The Following Variables can be used:
|
|
// {{.Code}} {{.UserName}} {{.FirstName}} {{.LastName}} {{.NickName}} {{.DisplayName}} {{.LastEmail}} {{.VerifiedEmail}} {{.LastPhone}} {{.VerifiedPhone}} {{.PreferredLoginName}} {{.LoginNames}} {{.ChangeDate}}
|
|
rpc SetCustomInitMessageText(SetCustomInitMessageTextRequest) returns (SetCustomInitMessageTextResponse) {
|
|
option (google.api.http) = {
|
|
put: "/text/message/init/{language}";
|
|
body: "*";
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.write";
|
|
feature: "custom_text.message"
|
|
};
|
|
}
|
|
|
|
// Removes the custom init message text of the organisation
|
|
// The default text of the IAM will trigger after
|
|
rpc ResetCustomInitMessageTextToDefault(ResetCustomInitMessageTextToDefaultRequest) returns (ResetCustomInitMessageTextToDefaultResponse) {
|
|
option (google.api.http) = {
|
|
delete: "/text/message/init/{language}"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.delete"
|
|
};
|
|
}
|
|
//Returns the custom text for password reset message
|
|
rpc GetCustomPasswordResetMessageText(GetCustomPasswordResetMessageTextRequest) returns (GetCustomPasswordResetMessageTextResponse) {
|
|
option (google.api.http) = {
|
|
get: "/text/message/passwordreset/{language}";
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.read";
|
|
};
|
|
}
|
|
|
|
//Returns the default text for password reset message
|
|
rpc GetDefaultPasswordResetMessageText(GetDefaultPasswordResetMessageTextRequest) returns (GetDefaultPasswordResetMessageTextResponse) {
|
|
option (google.api.http) = {
|
|
get: "/text/default/message/passwordreset/{language}";
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.read";
|
|
};
|
|
}
|
|
|
|
// Sets the custom text for password reset message
|
|
// The Following Variables can be used:
|
|
// {{.Code}} {{.UserName}} {{.FirstName}} {{.LastName}} {{.NickName}} {{.DisplayName}} {{.LastEmail}} {{.VerifiedEmail}} {{.LastPhone}} {{.VerifiedPhone}} {{.PreferredLoginName}} {{.LoginNames}} {{.ChangeDate}}
|
|
rpc SetCustomPasswordResetMessageText(SetCustomPasswordResetMessageTextRequest) returns (SetCustomPasswordResetMessageTextResponse) {
|
|
option (google.api.http) = {
|
|
put: "/text/message/passwordreset/{language}";
|
|
body: "*";
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.write";
|
|
feature: "custom_text.message"
|
|
};
|
|
}
|
|
|
|
// Removes the custom password reset message text of the organisation
|
|
// The default text of the IAM will trigger after
|
|
rpc ResetCustomPasswordResetMessageTextToDefault(ResetCustomPasswordResetMessageTextToDefaultRequest) returns (ResetCustomPasswordResetMessageTextToDefaultResponse) {
|
|
option (google.api.http) = {
|
|
delete: "/text/message/verifyemail/{language}"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.delete"
|
|
};
|
|
}
|
|
|
|
//Returns the custom text for verify email message
|
|
rpc GetCustomVerifyEmailMessageText(GetCustomVerifyEmailMessageTextRequest) returns (GetCustomVerifyEmailMessageTextResponse) {
|
|
option (google.api.http) = {
|
|
get: "/text/message/verifyemail/{language}";
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.read";
|
|
};
|
|
}
|
|
|
|
//Returns the default text for verify email message
|
|
rpc GetDefaultVerifyEmailMessageText(GetDefaultVerifyEmailMessageTextRequest) returns (GetDefaultVerifyEmailMessageTextResponse) {
|
|
option (google.api.http) = {
|
|
get: "/text/default/message/verifyemail/{language}";
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.read";
|
|
};
|
|
}
|
|
|
|
// Sets the custom text for verify email message
|
|
// The Following Variables can be used:
|
|
// {{.Code}} {{.UserName}} {{.FirstName}} {{.LastName}} {{.NickName}} {{.DisplayName}} {{.LastEmail}} {{.VerifiedEmail}} {{.LastPhone}} {{.VerifiedPhone}} {{.PreferredLoginName}} {{.LoginNames}} {{.ChangeDate}}
|
|
rpc SetCustomVerifyEmailMessageText(SetCustomVerifyEmailMessageTextRequest) returns (SetCustomVerifyEmailMessageTextResponse) {
|
|
option (google.api.http) = {
|
|
put: "/text/message/verifyemail/{language}";
|
|
body: "*";
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.write";
|
|
feature: "custom_text.message"
|
|
};
|
|
}
|
|
|
|
// Removes the custom verify email message text of the organisation
|
|
// The default text of the IAM will trigger after
|
|
rpc ResetCustomVerifyEmailMessageTextToDefault(ResetCustomVerifyEmailMessageTextToDefaultRequest) returns (ResetCustomVerifyEmailMessageTextToDefaultResponse) {
|
|
option (google.api.http) = {
|
|
delete: "/text/message/verifyemail/{language}"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.delete"
|
|
};
|
|
}
|
|
|
|
//Returns the custom text for verify email message
|
|
rpc GetCustomVerifyPhoneMessageText(GetCustomVerifyPhoneMessageTextRequest) returns (GetCustomVerifyPhoneMessageTextResponse) {
|
|
option (google.api.http) = {
|
|
get: "/text/message/verifyphone/{language}";
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.read";
|
|
};
|
|
}
|
|
|
|
//Returns the custom text for verify email message
|
|
rpc GetDefaultVerifyPhoneMessageText(GetDefaultVerifyPhoneMessageTextRequest) returns (GetDefaultVerifyPhoneMessageTextResponse) {
|
|
option (google.api.http) = {
|
|
get: "/text/default/message/verifyphone/{language}";
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.read";
|
|
};
|
|
}
|
|
|
|
// Sets the default custom text for verify email message
|
|
// The Following Variables can be used:
|
|
// {{.Code}} {{.UserName}} {{.FirstName}} {{.LastName}} {{.NickName}} {{.DisplayName}} {{.LastEmail}} {{.VerifiedEmail}} {{.LastPhone}} {{.VerifiedPhone}} {{.PreferredLoginName}} {{.LoginNames}} {{.ChangeDate}}
|
|
rpc SetCustomVerifyPhoneMessageText(SetCustomVerifyPhoneMessageTextRequest) returns (SetCustomVerifyPhoneMessageTextResponse) {
|
|
option (google.api.http) = {
|
|
put: "/text/message/verifyphone/{language}";
|
|
body: "*";
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.write";
|
|
feature: "custom_text.message"
|
|
};
|
|
}
|
|
|
|
// Removes the custom verify phone text of the organisation
|
|
// The default text of the IAM will trigger after
|
|
rpc ResetCustomVerifyPhoneMessageTextToDefault(ResetCustomVerifyPhoneMessageTextToDefaultRequest) returns (ResetCustomVerifyPhoneMessageTextToDefaultResponse) {
|
|
option (google.api.http) = {
|
|
delete: "/text/message/verifyphone/{language}"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.delete"
|
|
};
|
|
}
|
|
|
|
//Returns the custom text for domain claimed message
|
|
rpc GetCustomDomainClaimedMessageText(GetCustomDomainClaimedMessageTextRequest) returns (GetCustomDomainClaimedMessageTextResponse) {
|
|
option (google.api.http) = {
|
|
get: "/text/message/domainclaimed/{language}";
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.read";
|
|
};
|
|
}
|
|
|
|
//Returns the custom text for domain claimed message
|
|
rpc GetDefaultDomainClaimedMessageText(GetDefaultDomainClaimedMessageTextRequest) returns (GetDefaultDomainClaimedMessageTextResponse) {
|
|
option (google.api.http) = {
|
|
get: "/text/default/message/domainclaimed/{language}";
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.read";
|
|
};
|
|
}
|
|
|
|
// Sets the custom text for domain claimed message
|
|
// The Following Variables can be used:
|
|
// {{.Domain}} {{.TempUsername}} {{.UserName}} {{.FirstName}} {{.LastName}} {{.NickName}} {{.DisplayName}} {{.LastEmail}} {{.VerifiedEmail}} {{.LastPhone}} {{.VerifiedPhone}} {{.PreferredLoginName}} {{.LoginNames}} {{.ChangeDate}}
|
|
rpc SetCustomDomainClaimedMessageCustomText(SetCustomDomainClaimedMessageTextRequest) returns (SetCustomDomainClaimedMessageTextResponse) {
|
|
option (google.api.http) = {
|
|
put: "/text/message/domainclaimed/{language}";
|
|
body: "*";
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.write";
|
|
feature: "custom_text.message"
|
|
};
|
|
}
|
|
|
|
// Removes the custom domain claimed message text of the organisation
|
|
// The default text of the IAM will trigger after
|
|
rpc ResetCustomDomainClaimedMessageTextToDefault(ResetCustomDomainClaimedMessageTextToDefaultRequest) returns (ResetCustomDomainClaimedMessageTextToDefaultResponse) {
|
|
option (google.api.http) = {
|
|
delete: "/text/message/domainclaimed/{language}"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.delete"
|
|
};
|
|
}
|
|
|
|
//Returns the custom text for passwordless link message
|
|
rpc GetCustomPasswordlessRegistrationMessageText(GetCustomPasswordlessRegistrationMessageTextRequest) returns (GetCustomPasswordlessRegistrationMessageTextResponse) {
|
|
option (google.api.http) = {
|
|
get: "/text/message/passwordless_registration/{language}";
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.read";
|
|
};
|
|
}
|
|
|
|
//Returns the custom text for passwordless link message
|
|
rpc GetDefaultPasswordlessRegistrationMessageText(GetDefaultPasswordlessRegistrationMessageTextRequest) returns (GetDefaultPasswordlessRegistrationMessageTextResponse) {
|
|
option (google.api.http) = {
|
|
get: "/text/default/message/passwordless_registration/{language}";
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.read";
|
|
};
|
|
}
|
|
|
|
// Sets the custom text for passwordless link message
|
|
// The Following Variables can be used:
|
|
// {{.UserName}} {{.FirstName}} {{.LastName}} {{.NickName}} {{.DisplayName}} {{.LastEmail}} {{.VerifiedEmail}} {{.LastPhone}} {{.VerifiedPhone}} {{.PreferredLoginName}} {{.LoginNames}} {{.ChangeDate}}
|
|
rpc SetCustomPasswordlessRegistrationMessageCustomText(SetCustomPasswordlessRegistrationMessageTextRequest) returns (SetCustomPasswordlessRegistrationMessageTextResponse) {
|
|
option (google.api.http) = {
|
|
put: "/text/message/passwordless_registration/{language}";
|
|
body: "*";
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.write";
|
|
feature: "custom_text.message"
|
|
};
|
|
}
|
|
|
|
// Removes the custom passwordless link message text of the organisation
|
|
// The default text of the IAM will trigger after
|
|
rpc ResetCustomPasswordlessRegistrationMessageTextToDefault(ResetCustomPasswordlessRegistrationMessageTextToDefaultRequest) returns (ResetCustomPasswordlessRegistrationMessageTextToDefaultResponse) {
|
|
option (google.api.http) = {
|
|
delete: "/text/message/passwordless_registration/{language}"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.delete"
|
|
};
|
|
}
|
|
|
|
//Returns the custom texts for login ui
|
|
rpc GetCustomLoginTexts(GetCustomLoginTextsRequest) returns (GetCustomLoginTextsResponse) {
|
|
option (google.api.http) = {
|
|
get: "/text/login/{language}";
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.read";
|
|
};
|
|
}
|
|
|
|
//Returns the custom texts for login ui
|
|
rpc GetDefaultLoginTexts(GetDefaultLoginTextsRequest) returns (GetDefaultLoginTextsResponse) {
|
|
option (google.api.http) = {
|
|
get: "/text/default/login/{language}";
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.read";
|
|
};
|
|
}
|
|
|
|
//Sets the default custom text for login ui
|
|
//it impacts all organisations without customized login ui texts
|
|
rpc SetCustomLoginText(SetCustomLoginTextsRequest) returns (SetCustomLoginTextsResponse) {
|
|
option (google.api.http) = {
|
|
put: "/text/login/{language}";
|
|
body: "*";
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.write";
|
|
feature: "custom_text.login"
|
|
};
|
|
}
|
|
|
|
// Removes the custom login text of the organisation
|
|
// The default text of the IAM will trigger after
|
|
rpc ResetCustomLoginTextToDefault(ResetCustomLoginTextsToDefaultRequest) returns (ResetCustomLoginTextsToDefaultResponse) {
|
|
option (google.api.http) = {
|
|
delete: "/text/login/{language}"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "policy.delete"
|
|
};
|
|
}
|
|
|
|
// Returns a identity provider configuration of the organisation
|
|
rpc GetOrgIDPByID(GetOrgIDPByIDRequest) returns (GetOrgIDPByIDResponse) {
|
|
option (google.api.http) = {
|
|
get: "/idps/{id}"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "org.idp.read"
|
|
};
|
|
}
|
|
|
|
// Returns all identity provider configuration in the organisation, which match the query
|
|
// Limit should always be set, there is a default limit set by the service
|
|
rpc ListOrgIDPs(ListOrgIDPsRequest) returns (ListOrgIDPsResponse) {
|
|
option (google.api.http) = {
|
|
post: "/idps/_search"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "org.idp.read"
|
|
};
|
|
}
|
|
|
|
// Add a new identity provider configuration in the organisation
|
|
// Provider must be OIDC compliant
|
|
rpc AddOrgOIDCIDP(AddOrgOIDCIDPRequest) returns (AddOrgOIDCIDPResponse) {
|
|
option (google.api.http) = {
|
|
post: "/idps/oidc"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "org.idp.write"
|
|
feature: "login_policy.idp"
|
|
};
|
|
}
|
|
|
|
// Add a new jwt identity provider configuration in the organisation
|
|
rpc AddOrgJWTIDP(AddOrgJWTIDPRequest) returns (AddOrgJWTIDPResponse) {
|
|
option (google.api.http) = {
|
|
post: "/idps/jwt"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "org.idp.write"
|
|
feature: "login_policy.idp"
|
|
};
|
|
}
|
|
|
|
// Deactivate identity provider configuration
|
|
// Users will not be able to use this provider for login (e.g Google, Microsoft, AD, etc)
|
|
// Returns error if already deactivated
|
|
rpc DeactivateOrgIDP(DeactivateOrgIDPRequest) returns (DeactivateOrgIDPResponse) {
|
|
option (google.api.http) = {
|
|
post: "/idps/{idp_id}/_deactivate"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "org.idp.write"
|
|
feature: "login_policy.idp"
|
|
};
|
|
}
|
|
|
|
// Activate identity provider configuration
|
|
// Returns error if not deactivated
|
|
rpc ReactivateOrgIDP(ReactivateOrgIDPRequest) returns (ReactivateOrgIDPResponse) {
|
|
option (google.api.http) = {
|
|
post: "/idps/{idp_id}/_reactivate"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "org.idp.write"
|
|
feature: "login_policy.idp"
|
|
};
|
|
}
|
|
|
|
// Removes identity provider configuration
|
|
// Will remove all linked providers of this configuration on the users
|
|
rpc RemoveOrgIDP(RemoveOrgIDPRequest) returns (RemoveOrgIDPResponse) {
|
|
option (google.api.http) = {
|
|
delete: "/idps/{idp_id}"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "org.idp.write"
|
|
feature: "login_policy.idp"
|
|
};
|
|
}
|
|
|
|
// Change identity provider configuration of the organisation
|
|
rpc UpdateOrgIDP(UpdateOrgIDPRequest) returns (UpdateOrgIDPResponse) {
|
|
option (google.api.http) = {
|
|
put: "/idps/{idp_id}"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "org.idp.write"
|
|
feature: "login_policy.idp"
|
|
};
|
|
}
|
|
|
|
// Change OIDC identity provider configuration of the organisation
|
|
rpc UpdateOrgIDPOIDCConfig(UpdateOrgIDPOIDCConfigRequest) returns (UpdateOrgIDPOIDCConfigResponse) {
|
|
option (google.api.http) = {
|
|
put: "/idps/{idp_id}/oidc_config"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "org.idp.write"
|
|
feature: "login_policy.idp"
|
|
};
|
|
}
|
|
|
|
// Change JWT identity provider configuration of the organisation
|
|
rpc UpdateOrgIDPJWTConfig(UpdateOrgIDPJWTConfigRequest) returns (UpdateOrgIDPJWTConfigResponse) {
|
|
option (google.api.http) = {
|
|
put: "/idps/{idp_id}/jwt_config"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "org.idp.write"
|
|
feature: "login_policy.idp"
|
|
};
|
|
}
|
|
|
|
rpc ListActions(ListActionsRequest) returns (ListActionsResponse) {
|
|
option (google.api.http) = {
|
|
post: "/actions/_search"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "org.action.read"
|
|
feature: "actions"
|
|
};
|
|
}
|
|
|
|
rpc GetAction(GetActionRequest) returns (GetActionResponse) {
|
|
option (google.api.http) = {
|
|
get: "/actions/{id}"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "org.action.read"
|
|
feature: "actions"
|
|
};
|
|
}
|
|
|
|
rpc CreateAction(CreateActionRequest) returns (CreateActionResponse) {
|
|
option (google.api.http) = {
|
|
post: "/actions"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "org.action.write"
|
|
feature: "actions"
|
|
};
|
|
}
|
|
|
|
rpc UpdateAction(UpdateActionRequest) returns (UpdateActionResponse) {
|
|
option (google.api.http) = {
|
|
put: "/actions/{id}"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "org.action.write"
|
|
feature: "actions"
|
|
};
|
|
}
|
|
|
|
//TODO: enable in next release
|
|
// rpc DeactivateAction(DeactivateActionRequest) returns (DeactivateActionResponse) {
|
|
// option (google.api.http) = {
|
|
// post: "/actions/{id}/_deactivate"
|
|
// body: "*"
|
|
// };
|
|
//
|
|
// option (zitadel.v1.auth_option) = {
|
|
// permission: "org.action.write"
|
|
// feature: "actions"
|
|
// };
|
|
// }
|
|
//
|
|
// rpc ReactivateAction(ReactivateActionRequest) returns (ReactivateActionResponse) {
|
|
// option (google.api.http) = {
|
|
// post: "/actions/{id}/_reactivate"
|
|
// body: "*"
|
|
// };
|
|
//
|
|
// option (zitadel.v1.auth_option) = {
|
|
// permission: "org.action.write"
|
|
// feature: "actions"
|
|
// };
|
|
// }
|
|
|
|
rpc DeleteAction(DeleteActionRequest) returns (DeleteActionResponse) {
|
|
option (google.api.http) = {
|
|
delete: "/actions/{id}"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "org.action.delete"
|
|
feature: "actions"
|
|
};
|
|
}
|
|
|
|
rpc GetFlow(GetFlowRequest) returns (GetFlowResponse) {
|
|
option (google.api.http) = {
|
|
get: "/flows/{type}"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "org.flow.read"
|
|
feature: "actions"
|
|
};
|
|
}
|
|
|
|
rpc ClearFlow(ClearFlowRequest) returns (ClearFlowResponse) {
|
|
option (google.api.http) = {
|
|
post: "/flows/{type}/_clear"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "org.flow.delete"
|
|
feature: "actions"
|
|
};
|
|
}
|
|
|
|
rpc SetTriggerActions(SetTriggerActionsRequest) returns (SetTriggerActionsResponse) {
|
|
option (google.api.http) = {
|
|
post: "/flows/{flow_type}/trigger/{trigger_type}"
|
|
body: "*"
|
|
};
|
|
|
|
option (zitadel.v1.auth_option) = {
|
|
permission: "org.flow.write"
|
|
feature: "actions"
|
|
};
|
|
}
|
|
}
|
|
|
|
//This is an empty request
|
|
message HealthzRequest {}
|
|
|
|
//This is an empty response
|
|
message HealthzResponse {}
|
|
|
|
//This is an empty request
|
|
message GetOIDCInformationRequest {}
|
|
|
|
message GetOIDCInformationResponse {
|
|
string issuer = 1;
|
|
string discovery_endpoint = 2;
|
|
}
|
|
|
|
//This is an empty request
|
|
message GetIAMRequest {}
|
|
|
|
message GetIAMResponse {
|
|
string global_org_id = 1;
|
|
string iam_project_id = 2;
|
|
}
|
|
|
|
//This is an empty request
|
|
message GetSupportedLanguagesRequest {}
|
|
|
|
message GetSupportedLanguagesResponse {
|
|
repeated string languages = 1;
|
|
}
|
|
|
|
message GetUserByIDRequest {
|
|
string id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message GetUserByIDResponse {
|
|
zitadel.user.v1.User user = 1;
|
|
}
|
|
|
|
message GetUserByLoginNameGlobalRequest{
|
|
string login_name = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message GetUserByLoginNameGlobalResponse {
|
|
zitadel.user.v1.User user = 1;
|
|
}
|
|
|
|
message ListUsersRequest {
|
|
//list limitations and ordering
|
|
zitadel.v1.ListQuery query = 1;
|
|
// the field the result is sorted
|
|
zitadel.user.v1.UserFieldName sorting_column = 2;
|
|
//criterias the client is looking for
|
|
repeated zitadel.user.v1.SearchQuery queries = 3;
|
|
}
|
|
|
|
message ListUsersResponse {
|
|
zitadel.v1.ListDetails details = 1;
|
|
zitadel.user.v1.UserFieldName sorting_column = 2;
|
|
repeated zitadel.user.v1.User result = 3;
|
|
}
|
|
|
|
message ListUserChangesRequest {
|
|
//list limitations and ordering
|
|
zitadel.change.v1.ChangeQuery query = 1;
|
|
string user_id = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message ListUserChangesResponse {
|
|
zitadel.v1.ListDetails details = 1;
|
|
repeated zitadel.change.v1.Change result = 2;
|
|
}
|
|
|
|
message IsUserUniqueRequest {
|
|
string user_name = 1 [(validate.rules).string = {max_len: 200}];
|
|
string email = 2 [(validate.rules).string = {max_len: 200}];
|
|
}
|
|
|
|
message IsUserUniqueResponse {
|
|
bool is_unique = 1;
|
|
}
|
|
|
|
message AddHumanUserRequest {
|
|
message Profile {
|
|
string first_name = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string last_name = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string nick_name = 3 [(validate.rules).string = {max_len: 200}];
|
|
string display_name = 4 [(validate.rules).string = {max_len: 200}];
|
|
string preferred_language = 5 [(validate.rules).string = {max_len: 10}];
|
|
zitadel.user.v1.Gender gender = 6;
|
|
}
|
|
message Email {
|
|
string email = 1 [(validate.rules).string.email = true]; //TODO: check if no value is allowed
|
|
bool is_email_verified = 2;
|
|
}
|
|
message Phone {
|
|
// has to be a global number
|
|
string phone = 1 [(validate.rules).string = {min_len: 1, max_len: 50, prefix: "+"}];
|
|
bool is_phone_verified = 2;
|
|
}
|
|
|
|
string user_name = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
|
|
Profile profile = 2 [(validate.rules).message.required = true];
|
|
Email email = 3 [(validate.rules).message.required = true];
|
|
Phone phone = 4;
|
|
string initial_password = 5;
|
|
}
|
|
|
|
message AddHumanUserResponse {
|
|
string user_id = 1;
|
|
zitadel.v1.ObjectDetails details = 2;
|
|
}
|
|
|
|
message ImportHumanUserRequest {
|
|
message Profile {
|
|
string first_name = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string last_name = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string nick_name = 3 [(validate.rules).string = {max_len: 200}];
|
|
string display_name = 4 [(validate.rules).string = {max_len: 200}];
|
|
string preferred_language = 5 [(validate.rules).string = {max_len: 10}];
|
|
zitadel.user.v1.Gender gender = 6;
|
|
}
|
|
message Email {
|
|
string email = 1 [(validate.rules).string.email = true]; //TODO: check if no value is allowed
|
|
bool is_email_verified = 2;
|
|
}
|
|
message Phone {
|
|
// has to be a global number
|
|
string phone = 1 [(validate.rules).string = {min_len: 1, max_len: 50, prefix: "+"}];
|
|
bool is_phone_verified = 2;
|
|
}
|
|
|
|
string user_name = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
|
|
Profile profile = 2 [(validate.rules).message.required = true];
|
|
Email email = 3 [(validate.rules).message.required = true];
|
|
Phone phone = 4;
|
|
string password = 5;
|
|
bool password_change_required = 6;
|
|
bool request_passwordless_registration = 7;
|
|
}
|
|
|
|
message ImportHumanUserResponse {
|
|
message PasswordlessRegistration {
|
|
string link = 1;
|
|
//deprecated: use expiration instead
|
|
google.protobuf.Duration lifetime = 2;
|
|
google.protobuf.Duration expiration = 3;
|
|
}
|
|
|
|
string user_id = 1;
|
|
zitadel.v1.ObjectDetails details = 2;
|
|
PasswordlessRegistration passwordless_registration = 3;
|
|
}
|
|
|
|
message AddMachineUserRequest {
|
|
string user_name = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
|
|
string name = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string description = 3 [(validate.rules).string = {max_len: 500}];
|
|
}
|
|
|
|
message AddMachineUserResponse {
|
|
string user_id = 1;
|
|
zitadel.v1.ObjectDetails details = 2;
|
|
}
|
|
|
|
message DeactivateUserRequest {
|
|
string id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message DeactivateUserResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message ReactivateUserRequest {
|
|
string id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message ReactivateUserResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message LockUserRequest {
|
|
string id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message LockUserResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message UnlockUserRequest {
|
|
string id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message UnlockUserResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message RemoveUserRequest {
|
|
string id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message RemoveUserResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message UpdateUserNameRequest {
|
|
string user_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string user_name = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message UpdateUserNameResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message ListUserMetadataRequest {
|
|
string id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
zitadel.v1.ListQuery query = 2;
|
|
repeated zitadel.metadata.v1.MetadataQuery queries = 3;
|
|
}
|
|
|
|
message ListUserMetadataResponse {
|
|
zitadel.v1.ListDetails details = 1;
|
|
repeated zitadel.metadata.v1.Metadata result = 2;
|
|
}
|
|
|
|
message GetUserMetadataRequest {
|
|
string id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string key = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message GetUserMetadataResponse {
|
|
zitadel.metadata.v1.Metadata metadata = 1;
|
|
}
|
|
|
|
message SetUserMetadataRequest {
|
|
string id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string key = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
bytes value = 3 [(validate.rules).bytes = {min_len: 1, max_len: 500000}];
|
|
}
|
|
|
|
message SetUserMetadataResponse {
|
|
string id = 1;
|
|
zitadel.v1.ObjectDetails details = 2;
|
|
}
|
|
|
|
message BulkSetUserMetadataRequest {
|
|
string id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
message Metadata {
|
|
string key = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
bytes value = 2 [(validate.rules).bytes = {min_len: 1, max_len: 500000}];
|
|
}
|
|
repeated Metadata metadata = 2;
|
|
}
|
|
|
|
message BulkSetUserMetadataResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message RemoveUserMetadataRequest {
|
|
string id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string key = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message RemoveUserMetadataResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message BulkRemoveUserMetadataRequest {
|
|
string id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
repeated string keys = 2 [(validate.rules).repeated.items.string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message BulkRemoveUserMetadataResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message GetHumanProfileRequest {
|
|
string user_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message GetHumanProfileResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
zitadel.user.v1.Profile profile = 2;
|
|
}
|
|
|
|
message UpdateHumanProfileRequest {
|
|
string user_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
|
|
string first_name = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string last_name = 3 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string nick_name = 4 [(validate.rules).string = {max_len: 200}];
|
|
string display_name = 5 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string preferred_language = 6 [(validate.rules).string = {max_len: 10}];
|
|
zitadel.user.v1.Gender gender = 7;
|
|
}
|
|
|
|
message UpdateHumanProfileResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message GetHumanEmailRequest {
|
|
string user_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message GetHumanEmailResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
zitadel.user.v1.Email email = 2;
|
|
}
|
|
|
|
message UpdateHumanEmailRequest {
|
|
string user_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
|
|
string email = 2 [(validate.rules).string.email = true];
|
|
bool is_email_verified = 3;
|
|
}
|
|
|
|
message UpdateHumanEmailResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message ResendHumanInitializationRequest {
|
|
string user_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string email = 2 [(validate.rules).string = {ignore_empty: true, email: true}];
|
|
}
|
|
|
|
message ResendHumanInitializationResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message ResendHumanEmailVerificationRequest {
|
|
string user_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message ResendHumanEmailVerificationResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message GetHumanPhoneRequest {
|
|
string user_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message GetHumanPhoneResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
zitadel.user.v1.Phone phone = 2;
|
|
}
|
|
|
|
message UpdateHumanPhoneRequest {
|
|
string user_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
|
|
|
|
string phone = 2 [(validate.rules).string = {min_len: 1, max_len: 50, prefix: "+"}];
|
|
bool is_phone_verified = 3;
|
|
}
|
|
|
|
message UpdateHumanPhoneResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message RemoveHumanPhoneRequest {
|
|
string user_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message RemoveHumanPhoneResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message ResendHumanPhoneVerificationRequest {
|
|
string user_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message ResendHumanPhoneVerificationResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message RemoveHumanAvatarRequest {
|
|
string user_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message RemoveHumanAvatarResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message SetHumanInitialPasswordRequest {
|
|
string user_id = 1 [(validate.rules).string.min_len = 1];
|
|
string password = 2 [(validate.rules).string = {min_len: 1, max_len: 72}];
|
|
}
|
|
|
|
message SetHumanInitialPasswordResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message SetHumanPasswordRequest {
|
|
string user_id = 1 [(validate.rules).string.min_len = 1];
|
|
string password = 2 [(validate.rules).string = {min_len: 1, max_len: 72}];
|
|
bool no_change_required = 3;
|
|
}
|
|
|
|
message SetHumanPasswordResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message SendHumanResetPasswordNotificationRequest {
|
|
enum Type {
|
|
TYPE_EMAIL = 0;
|
|
TYPE_SMS = 1;
|
|
}
|
|
string user_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
Type type = 2 [(validate.rules).enum.defined_only = true];
|
|
}
|
|
|
|
message SendHumanResetPasswordNotificationResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message ListHumanAuthFactorsRequest {
|
|
string user_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message ListHumanAuthFactorsResponse {
|
|
repeated zitadel.user.v1.AuthFactor result = 1;
|
|
}
|
|
|
|
message RemoveHumanAuthFactorOTPRequest {
|
|
string user_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message RemoveHumanAuthFactorOTPResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message RemoveHumanAuthFactorU2FRequest {
|
|
string user_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string token_id = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message RemoveHumanAuthFactorU2FResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message ListHumanPasswordlessRequest {
|
|
string user_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message ListHumanPasswordlessResponse {
|
|
repeated zitadel.user.v1.WebAuthNToken result = 1;
|
|
}
|
|
|
|
message AddPasswordlessRegistrationRequest {
|
|
string user_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message AddPasswordlessRegistrationResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
string link = 2;
|
|
google.protobuf.Duration expiration = 3;
|
|
}
|
|
|
|
message SendPasswordlessRegistrationRequest {
|
|
string user_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message SendPasswordlessRegistrationResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message RemoveHumanPasswordlessRequest {
|
|
string user_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string token_id = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message RemoveHumanPasswordlessResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message UpdateMachineRequest {
|
|
string user_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string description = 2 [(validate.rules).string.max_len = 500];
|
|
string name = 3 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message UpdateMachineResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message GetMachineKeyByIDsRequest {
|
|
string user_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string key_id = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message GetMachineKeyByIDsResponse {
|
|
zitadel.authn.v1.Key key = 1;
|
|
}
|
|
|
|
message ListMachineKeysRequest {
|
|
string user_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
//list limitations and ordering
|
|
zitadel.v1.ListQuery query = 2;
|
|
}
|
|
|
|
message ListMachineKeysResponse {
|
|
zitadel.v1.ListDetails details = 1;
|
|
repeated zitadel.authn.v1.Key result = 2;
|
|
}
|
|
|
|
message AddMachineKeyRequest {
|
|
string user_id = 1 [(validate.rules).string.min_len = 1];
|
|
zitadel.authn.v1.KeyType type = 2 [(validate.rules).enum = {defined_only: true, not_in: [0]}];
|
|
google.protobuf.Timestamp expiration_date = 3 [
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"2519-04-01T08:45:00.000000Z\"";
|
|
description: "The date the key will expire and no logins will be possible";
|
|
}
|
|
];
|
|
}
|
|
|
|
message AddMachineKeyResponse {
|
|
string key_id = 1;
|
|
bytes key_details = 2;
|
|
zitadel.v1.ObjectDetails details = 3;
|
|
}
|
|
|
|
message RemoveMachineKeyRequest {
|
|
string user_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string key_id = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message RemoveMachineKeyResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message ListHumanLinkedIDPsRequest {
|
|
string user_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
//list limitations and ordering
|
|
zitadel.v1.ListQuery query = 2;
|
|
}
|
|
|
|
message ListHumanLinkedIDPsResponse {
|
|
zitadel.v1.ListDetails details = 1;
|
|
repeated zitadel.idp.v1.IDPUserLink result = 2;
|
|
}
|
|
|
|
message RemoveHumanLinkedIDPRequest {
|
|
string user_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string idp_id = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string linked_user_id = 3 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message RemoveHumanLinkedIDPResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message ListUserMembershipsRequest {
|
|
//list limitations and ordering
|
|
string user_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
//the field the result is sorted
|
|
zitadel.v1.ListQuery query = 2;
|
|
//criterias the client is looking for
|
|
repeated zitadel.user.v1.MembershipQuery queries = 3;
|
|
}
|
|
|
|
message ListUserMembershipsResponse {
|
|
zitadel.v1.ListDetails details = 1;
|
|
repeated zitadel.user.v1.Membership result = 2;
|
|
}
|
|
|
|
//This is an empty request
|
|
message GetMyOrgRequest {}
|
|
|
|
message GetMyOrgResponse {
|
|
zitadel.org.v1.Org org = 1;
|
|
}
|
|
|
|
message GetOrgByDomainGlobalRequest {
|
|
string domain = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message ListOrgChangesRequest {
|
|
//list limitations and ordering
|
|
zitadel.change.v1.ChangeQuery query = 1;
|
|
}
|
|
|
|
message ListOrgChangesResponse {
|
|
zitadel.v1.ListDetails details = 1;
|
|
repeated zitadel.change.v1.Change result = 2;
|
|
}
|
|
|
|
message GetOrgByDomainGlobalResponse {
|
|
zitadel.org.v1.Org org = 1;
|
|
}
|
|
|
|
message AddOrgRequest {
|
|
string name = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message AddOrgResponse {
|
|
string id = 1;
|
|
zitadel.v1.ObjectDetails details = 2;
|
|
}
|
|
|
|
message UpdateOrgRequest {
|
|
string name = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message UpdateOrgResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
//This is an empty request
|
|
message DeactivateOrgRequest {}
|
|
|
|
message DeactivateOrgResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
//This is an empty request
|
|
message ReactivateOrgRequest {}
|
|
|
|
message ReactivateOrgResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message ListOrgDomainsRequest {
|
|
//list limitations and ordering
|
|
zitadel.v1.ListQuery query = 1;
|
|
//criterias the client is looking for
|
|
repeated zitadel.org.v1.DomainSearchQuery queries = 2;
|
|
}
|
|
|
|
message ListOrgDomainsResponse {
|
|
zitadel.v1.ListDetails details = 1;
|
|
repeated zitadel.org.v1.Domain result = 2;
|
|
}
|
|
|
|
message AddOrgDomainRequest {
|
|
string domain = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message AddOrgDomainResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message RemoveOrgDomainRequest {
|
|
string domain = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message RemoveOrgDomainResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message GenerateOrgDomainValidationRequest {
|
|
string domain = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
zitadel.org.v1.DomainValidationType type = 2 [(validate.rules).enum = {defined_only: true, not_in: [0]}];
|
|
}
|
|
|
|
message GenerateOrgDomainValidationResponse {
|
|
string token = 1;
|
|
string url = 2;
|
|
}
|
|
|
|
message ValidateOrgDomainRequest {
|
|
string domain = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message ValidateOrgDomainResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message SetPrimaryOrgDomainRequest {
|
|
string domain = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message SetPrimaryOrgDomainResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
//This is an empty request
|
|
message ListOrgMemberRolesRequest {}
|
|
|
|
message ListOrgMemberRolesResponse {
|
|
repeated string result = 1;
|
|
}
|
|
|
|
message ListOrgMembersRequest {
|
|
//list limitations and ordering
|
|
zitadel.v1.ListQuery query = 1;
|
|
//criterias the client is looking for
|
|
repeated zitadel.member.v1.SearchQuery queries = 2;
|
|
}
|
|
|
|
message ListOrgMembersResponse {
|
|
//list limitations and ordering
|
|
zitadel.v1.ListDetails details = 1;
|
|
//criterias the client is looking for
|
|
repeated zitadel.member.v1.Member result = 2;
|
|
}
|
|
|
|
message AddOrgMemberRequest {
|
|
string user_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
repeated string roles = 2;
|
|
}
|
|
message AddOrgMemberResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message UpdateOrgMemberRequest {
|
|
string user_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
repeated string roles = 2;
|
|
}
|
|
|
|
message UpdateOrgMemberResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message RemoveOrgMemberRequest {
|
|
string user_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message RemoveOrgMemberResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message GetProjectByIDRequest {
|
|
string id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message GetProjectByIDResponse {
|
|
zitadel.project.v1.Project project = 1;
|
|
}
|
|
|
|
message GetGrantedProjectByIDRequest {
|
|
string project_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string grant_id = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message GetGrantedProjectByIDResponse {
|
|
zitadel.project.v1.GrantedProject granted_project = 1;
|
|
}
|
|
|
|
message ListProjectsRequest {
|
|
//list limitations and ordering
|
|
zitadel.v1.ListQuery query = 1;
|
|
//criterias the client is looking for
|
|
repeated zitadel.project.v1.ProjectQuery queries = 2;
|
|
}
|
|
|
|
message ListProjectsResponse {
|
|
zitadel.v1.ListDetails details = 1;
|
|
repeated zitadel.project.v1.Project result = 2;
|
|
}
|
|
|
|
message ListGrantedProjectsRequest {
|
|
//list limitations and ordering
|
|
zitadel.v1.ListQuery query = 1;
|
|
//criterias the client is looking for
|
|
repeated zitadel.project.v1.ProjectQuery queries = 2;
|
|
}
|
|
|
|
message ListGrantedProjectsResponse {
|
|
zitadel.v1.ListDetails details = 1;
|
|
repeated zitadel.project.v1.GrantedProject result = 2;
|
|
}
|
|
|
|
message ListProjectChangesRequest {
|
|
//list limitations and ordering
|
|
zitadel.change.v1.ChangeQuery query = 1;
|
|
string project_id = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message ListProjectChangesResponse {
|
|
zitadel.v1.ListDetails details = 1;
|
|
repeated zitadel.change.v1.Change result = 2;
|
|
}
|
|
|
|
message AddProjectRequest {
|
|
string name = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
bool project_role_assertion = 2;
|
|
bool project_role_check = 3;
|
|
bool has_project_check = 4;
|
|
zitadel.project.v1.PrivateLabelingSetting private_labeling_setting = 5 [(validate.rules).enum = {defined_only: true}];
|
|
}
|
|
|
|
message AddProjectResponse {
|
|
string id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
zitadel.v1.ObjectDetails details = 2;
|
|
}
|
|
|
|
message UpdateProjectRequest {
|
|
string id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string name = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
bool project_role_assertion = 3;
|
|
bool project_role_check = 4;
|
|
bool has_project_check = 5;
|
|
zitadel.project.v1.PrivateLabelingSetting private_labeling_setting = 6 [(validate.rules).enum = {defined_only: true}];
|
|
}
|
|
|
|
message UpdateProjectResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message DeactivateProjectRequest {
|
|
string id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message DeactivateProjectResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message ReactivateProjectRequest {
|
|
string id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message ReactivateProjectResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message RemoveProjectRequest {
|
|
string id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message RemoveProjectResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
//This is an empty request
|
|
message ListProjectMemberRolesRequest {}
|
|
|
|
message ListProjectMemberRolesResponse {
|
|
zitadel.v1.ListDetails details = 1;
|
|
repeated string result = 2;
|
|
}
|
|
|
|
message AddProjectRoleRequest {
|
|
string project_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string role_key = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string display_name = 3 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string group = 4 [(validate.rules).string = {max_len: 200}];
|
|
}
|
|
|
|
message AddProjectRoleResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message BulkAddProjectRolesRequest {
|
|
message Role {
|
|
string key = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string display_name = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string group = 3 [(validate.rules).string = {max_len: 200}];
|
|
}
|
|
|
|
string project_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
repeated Role roles = 2;
|
|
}
|
|
|
|
message BulkAddProjectRolesResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message UpdateProjectRoleRequest {
|
|
string project_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string role_key = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string display_name = 3 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string group = 4 [(validate.rules).string = {max_len: 200}];
|
|
}
|
|
|
|
message UpdateProjectRoleResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message RemoveProjectRoleRequest {
|
|
string project_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string role_key = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message RemoveProjectRoleResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message ListProjectRolesRequest {
|
|
string project_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
//list limitations and ordering
|
|
zitadel.v1.ListQuery query = 2;
|
|
//criterias the client is looking for
|
|
repeated zitadel.project.v1.RoleQuery queries = 3;
|
|
}
|
|
|
|
message ListProjectRolesResponse {
|
|
zitadel.v1.ListDetails details = 1;
|
|
repeated zitadel.project.v1.Role result = 2;
|
|
}
|
|
|
|
message ListGrantedProjectRolesRequest {
|
|
string project_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string grant_id = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
//list limitations and ordering
|
|
zitadel.v1.ListQuery query = 3;
|
|
//criterias the client is looking for
|
|
repeated zitadel.project.v1.RoleQuery queries = 4;
|
|
}
|
|
|
|
message ListGrantedProjectRolesResponse {
|
|
zitadel.v1.ListDetails details = 1;
|
|
repeated zitadel.project.v1.Role result = 2;
|
|
}
|
|
|
|
message ListProjectMembersRequest {
|
|
string project_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
//list limitations and ordering
|
|
zitadel.v1.ListQuery query = 2;
|
|
//criterias the client is looking for
|
|
repeated zitadel.member.v1.SearchQuery queries = 3;
|
|
}
|
|
|
|
message ListProjectMembersResponse {
|
|
zitadel.v1.ListDetails details = 1;
|
|
repeated zitadel.member.v1.Member result = 2;
|
|
}
|
|
|
|
message AddProjectMemberRequest {
|
|
string project_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string user_id = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
repeated string roles = 3;
|
|
}
|
|
|
|
message AddProjectMemberResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message UpdateProjectMemberRequest {
|
|
string project_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string user_id = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
repeated string roles = 3;
|
|
}
|
|
|
|
message UpdateProjectMemberResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message RemoveProjectMemberRequest {
|
|
string project_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string user_id = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message RemoveProjectMemberResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message GetAppByIDRequest {
|
|
string project_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string app_id = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message GetAppByIDResponse {
|
|
zitadel.app.v1.App app = 1;
|
|
}
|
|
|
|
message ListAppsRequest {
|
|
string project_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
//list limitations and ordering
|
|
zitadel.v1.ListQuery query = 2;
|
|
//criterias the client is looking for
|
|
repeated zitadel.app.v1.AppQuery queries = 3;
|
|
}
|
|
|
|
message ListAppsResponse {
|
|
zitadel.v1.ListDetails details = 1;
|
|
repeated zitadel.app.v1.App result = 2;
|
|
}
|
|
|
|
message ListAppChangesRequest {
|
|
//list limitations and ordering
|
|
zitadel.change.v1.ChangeQuery query = 1;
|
|
string project_id = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string app_id = 3 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message ListAppChangesResponse {
|
|
zitadel.v1.ListDetails details = 1;
|
|
repeated zitadel.change.v1.Change result = 2;
|
|
}
|
|
|
|
message AddOIDCAppRequest {
|
|
string project_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string name = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
repeated string redirect_uris = 3;
|
|
repeated zitadel.app.v1.OIDCResponseType response_types = 4;
|
|
repeated zitadel.app.v1.OIDCGrantType grant_types = 5;
|
|
zitadel.app.v1.OIDCAppType app_type = 6 [(validate.rules).enum = {defined_only: true}];
|
|
zitadel.app.v1.OIDCAuthMethodType auth_method_type = 7 [(validate.rules).enum = {defined_only: true}];
|
|
repeated string post_logout_redirect_uris = 8;
|
|
zitadel.app.v1.OIDCVersion version = 9 [(validate.rules).enum = {defined_only: true}];
|
|
bool dev_mode = 10;
|
|
zitadel.app.v1.OIDCTokenType access_token_type = 11 [(validate.rules).enum = {defined_only: true}];
|
|
bool access_token_role_assertion = 12;
|
|
bool id_token_role_assertion = 13;
|
|
bool id_token_userinfo_assertion = 14;
|
|
google.protobuf.Duration clock_skew = 15 [(validate.rules).duration = {gte: {}, lte: {seconds: 5}}];
|
|
repeated string additional_origins = 16;
|
|
}
|
|
|
|
message AddOIDCAppResponse {
|
|
string app_id = 1;
|
|
zitadel.v1.ObjectDetails details = 2;
|
|
string client_id = 3 [
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"gjöq34589uasgh\"";
|
|
description: "generated secret for this config";
|
|
}
|
|
];
|
|
string client_secret = 4 [
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"gjöq34589uasgh\"";
|
|
description: "generated secret for this config";
|
|
}
|
|
];
|
|
bool none_compliant = 5;
|
|
repeated zitadel.v1.LocalizedMessage compliance_problems = 6;
|
|
}
|
|
|
|
message AddAPIAppRequest {
|
|
string project_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string name = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
zitadel.app.v1.APIAuthMethodType auth_method_type = 3 [(validate.rules).enum = {defined_only: true}];
|
|
}
|
|
|
|
message AddAPIAppResponse {
|
|
string app_id = 1;
|
|
zitadel.v1.ObjectDetails details = 2;
|
|
string client_id = 3 [
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"gjöq34589uasgh\"";
|
|
description: "generated secret for this config";
|
|
}
|
|
];
|
|
string client_secret = 4 [
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"gjöq34589uasgh\"";
|
|
description: "generated secret for this config";
|
|
}
|
|
];
|
|
}
|
|
|
|
message UpdateAppRequest {
|
|
string project_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string app_id = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string name = 5 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message UpdateAppResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message UpdateOIDCAppConfigRequest {
|
|
string project_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string app_id = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
|
|
repeated string redirect_uris = 3;
|
|
repeated zitadel.app.v1.OIDCResponseType response_types = 4;
|
|
repeated zitadel.app.v1.OIDCGrantType grant_types = 5;
|
|
zitadel.app.v1.OIDCAppType app_type = 6 [(validate.rules).enum = {defined_only: true}];
|
|
zitadel.app.v1.OIDCAuthMethodType auth_method_type = 7 [(validate.rules).enum = {defined_only: true}];
|
|
repeated string post_logout_redirect_uris = 8;
|
|
bool dev_mode = 9;
|
|
zitadel.app.v1.OIDCTokenType access_token_type = 10 [(validate.rules).enum = {defined_only: true}];
|
|
bool access_token_role_assertion = 11;
|
|
bool id_token_role_assertion = 12;
|
|
bool id_token_userinfo_assertion = 13;
|
|
google.protobuf.Duration clock_skew = 14 [(validate.rules).duration = {gte: {}, lte: {seconds: 5}}];
|
|
repeated string additional_origins = 15;
|
|
}
|
|
|
|
message UpdateOIDCAppConfigResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message UpdateAPIAppConfigRequest {
|
|
string project_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string app_id = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
zitadel.app.v1.APIAuthMethodType auth_method_type = 7 [(validate.rules).enum = {defined_only: true}];
|
|
}
|
|
|
|
message UpdateAPIAppConfigResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message DeactivateAppRequest {
|
|
string project_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string app_id = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message DeactivateAppResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message ReactivateAppRequest {
|
|
string project_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string app_id = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message ReactivateAppResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message RemoveAppRequest {
|
|
string project_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string app_id = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message RemoveAppResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message RegenerateOIDCClientSecretRequest {
|
|
string project_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string app_id = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message RegenerateOIDCClientSecretResponse {
|
|
string client_secret = 1 [
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"gjöq34589uasgh\"";
|
|
description: "generated secret for the client";
|
|
}
|
|
];
|
|
zitadel.v1.ObjectDetails details = 2;
|
|
}
|
|
|
|
message RegenerateAPIClientSecretRequest {
|
|
string project_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string app_id = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message RegenerateAPIClientSecretResponse {
|
|
string client_secret = 1;
|
|
zitadel.v1.ObjectDetails details = 2;
|
|
}
|
|
|
|
message GetAppKeyRequest {
|
|
string project_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string app_id = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string key_id = 3 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message GetAppKeyResponse {
|
|
zitadel.authn.v1.Key key = 1;
|
|
}
|
|
|
|
message ListAppKeysRequest {
|
|
//list limitations and ordering
|
|
zitadel.v1.ListQuery query = 1;
|
|
string app_id = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string project_id = 3 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
message ListAppKeysResponse {
|
|
zitadel.v1.ListDetails details = 1;
|
|
repeated zitadel.authn.v1.Key result = 2;
|
|
}
|
|
|
|
message AddAppKeyRequest {
|
|
string project_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string app_id = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
zitadel.authn.v1.KeyType type = 3 [(validate.rules).enum = {defined_only: true, not_in: [0]}];
|
|
google.protobuf.Timestamp expiration_date = 4 [
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"2519-04-01T08:45:00.000000Z\"";
|
|
description: "The date the key will expire and no logins will be possible";
|
|
}
|
|
];
|
|
}
|
|
|
|
message AddAppKeyResponse {
|
|
string id = 1;
|
|
zitadel.v1.ObjectDetails details = 2;
|
|
bytes key_details = 3;
|
|
}
|
|
|
|
message RemoveAppKeyRequest {
|
|
string project_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string app_id = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string key_id = 3 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message RemoveAppKeyResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message GetProjectGrantByIDRequest {
|
|
string project_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string grant_id = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message GetProjectGrantByIDResponse {
|
|
zitadel.project.v1.GrantedProject project_grant = 1;
|
|
}
|
|
|
|
message ListProjectGrantsRequest {
|
|
string project_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
//list limitations and ordering
|
|
zitadel.v1.ListQuery query = 2;
|
|
//criterias the client is looking for
|
|
repeated zitadel.project.v1.ProjectGrantQuery queries = 3;
|
|
}
|
|
|
|
message ListProjectGrantsResponse {
|
|
zitadel.v1.ListDetails details = 1;
|
|
repeated zitadel.project.v1.GrantedProject result = 2;
|
|
}
|
|
|
|
message ListAllProjectGrantsRequest {
|
|
//list limitations and ordering
|
|
zitadel.v1.ListQuery query = 1;
|
|
//criterias the client is looking for
|
|
repeated zitadel.project.v1.AllProjectGrantQuery queries = 2;
|
|
}
|
|
|
|
message ListAllProjectGrantsResponse {
|
|
zitadel.v1.ListDetails details = 1;
|
|
repeated zitadel.project.v1.GrantedProject result = 2;
|
|
}
|
|
|
|
message AddProjectGrantRequest {
|
|
string project_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string granted_org_id = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
repeated string role_keys = 3;
|
|
}
|
|
|
|
message AddProjectGrantResponse {
|
|
string grant_id = 1;
|
|
zitadel.v1.ObjectDetails details = 2;
|
|
}
|
|
|
|
message UpdateProjectGrantRequest {
|
|
string project_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string grant_id = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
repeated string role_keys = 3;
|
|
}
|
|
|
|
message UpdateProjectGrantResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message DeactivateProjectGrantRequest {
|
|
string project_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string grant_id = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message DeactivateProjectGrantResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message ReactivateProjectGrantRequest {
|
|
string project_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string grant_id = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
message ReactivateProjectGrantResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message RemoveProjectGrantRequest {
|
|
string project_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string grant_id = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
message RemoveProjectGrantResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message ListProjectGrantMemberRolesRequest {
|
|
zitadel.v1.ListQuery query = 1;
|
|
repeated string result = 2;
|
|
}
|
|
|
|
message ListProjectGrantMemberRolesResponse {
|
|
zitadel.v1.ListDetails details = 1;
|
|
repeated string result = 2;
|
|
}
|
|
|
|
message ListProjectGrantMembersRequest {
|
|
string project_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string grant_id = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
//list limitations and ordering
|
|
zitadel.v1.ListQuery query = 3;
|
|
//criterias the client is looking for
|
|
repeated zitadel.member.v1.SearchQuery queries = 4;
|
|
}
|
|
|
|
message ListProjectGrantMembersResponse {
|
|
zitadel.v1.ListDetails details = 1;
|
|
repeated zitadel.member.v1.Member result = 2;
|
|
}
|
|
|
|
message AddProjectGrantMemberRequest {
|
|
string project_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string grant_id = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string user_id = 3 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
repeated string roles = 4;
|
|
}
|
|
|
|
message AddProjectGrantMemberResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message UpdateProjectGrantMemberRequest {
|
|
string project_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string grant_id = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string user_id = 3 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
repeated string roles = 4;
|
|
}
|
|
|
|
message UpdateProjectGrantMemberResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message RemoveProjectGrantMemberRequest {
|
|
string project_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string grant_id = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string user_id = 3 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message RemoveProjectGrantMemberResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message GetUserGrantByIDRequest {
|
|
string user_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string grant_id = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message GetUserGrantByIDResponse {
|
|
zitadel.user.v1.UserGrant user_grant = 1;
|
|
}
|
|
|
|
message ListUserGrantRequest {
|
|
//list limitations and ordering
|
|
zitadel.v1.ListQuery query = 1;
|
|
//criterias the client is looking for
|
|
repeated zitadel.user.v1.UserGrantQuery queries = 2;
|
|
}
|
|
|
|
message ListUserGrantResponse {
|
|
zitadel.v1.ListDetails details = 1;
|
|
repeated zitadel.user.v1.UserGrant result = 2;
|
|
}
|
|
|
|
message AddUserGrantRequest {
|
|
string user_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string project_id = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string project_grant_id = 3 [(validate.rules).string = {max_len: 200}];
|
|
repeated string role_keys = 4;
|
|
}
|
|
|
|
message AddUserGrantResponse {
|
|
string user_grant_id = 1;
|
|
zitadel.v1.ObjectDetails details = 2;
|
|
}
|
|
|
|
message UpdateUserGrantRequest {
|
|
string user_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string grant_id = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
repeated string role_keys = 3;
|
|
}
|
|
|
|
message UpdateUserGrantResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message DeactivateUserGrantRequest {
|
|
string user_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string grant_id = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message DeactivateUserGrantResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message ReactivateUserGrantRequest {
|
|
string user_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string grant_id = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message ReactivateUserGrantResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message RemoveUserGrantRequest {
|
|
string user_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string grant_id = 2 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message RemoveUserGrantResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message BulkRemoveUserGrantRequest {
|
|
repeated string grant_id = 1;
|
|
}
|
|
|
|
message BulkRemoveUserGrantResponse {}
|
|
|
|
message GetFeaturesRequest {}
|
|
|
|
message GetFeaturesResponse {
|
|
zitadel.features.v1.Features features = 1;
|
|
}
|
|
|
|
message GetOrgIAMPolicyRequest {}
|
|
|
|
message GetOrgIAMPolicyResponse {
|
|
zitadel.policy.v1.OrgIAMPolicy policy = 1;
|
|
}
|
|
|
|
message GetLoginPolicyRequest {}
|
|
|
|
message GetLoginPolicyResponse {
|
|
zitadel.policy.v1.LoginPolicy policy = 1;
|
|
//deprecated: is_default is also defined in zitadel.policy.v1.LoginPolicy
|
|
bool is_default = 2;
|
|
}
|
|
|
|
message GetDefaultLoginPolicyRequest {}
|
|
|
|
message GetDefaultLoginPolicyResponse {
|
|
zitadel.policy.v1.LoginPolicy policy = 1;
|
|
}
|
|
|
|
message AddCustomLoginPolicyRequest {
|
|
bool allow_username_password = 1;
|
|
bool allow_register = 2;
|
|
bool allow_external_idp = 3;
|
|
bool force_mfa = 4;
|
|
zitadel.policy.v1.PasswordlessType passwordless_type = 5 [(validate.rules).enum = {defined_only: true}];
|
|
bool hide_password_reset = 6;
|
|
}
|
|
|
|
message AddCustomLoginPolicyResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message UpdateCustomLoginPolicyRequest {
|
|
bool allow_username_password = 1;
|
|
bool allow_register = 2;
|
|
bool allow_external_idp = 3;
|
|
bool force_mfa = 4;
|
|
zitadel.policy.v1.PasswordlessType passwordless_type = 5 [(validate.rules).enum = {defined_only: true}];
|
|
bool hide_password_reset = 6;
|
|
}
|
|
|
|
message UpdateCustomLoginPolicyResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message ResetLoginPolicyToDefaultRequest {}
|
|
|
|
message ResetLoginPolicyToDefaultResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message ListLoginPolicyIDPsRequest {
|
|
zitadel.v1.ListQuery query = 1;
|
|
}
|
|
|
|
message ListLoginPolicyIDPsResponse {
|
|
zitadel.v1.ListDetails details = 1;
|
|
repeated zitadel.idp.v1.IDPLoginPolicyLink result = 2;
|
|
}
|
|
|
|
message AddIDPToLoginPolicyRequest {
|
|
string idp_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
zitadel.idp.v1.IDPOwnerType ownerType = 2 [(validate.rules).enum = {defined_only: true, not_in: [0]}];
|
|
}
|
|
|
|
message AddIDPToLoginPolicyResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message RemoveIDPFromLoginPolicyRequest {
|
|
string idp_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message RemoveIDPFromLoginPolicyResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message ListLoginPolicySecondFactorsRequest {}
|
|
|
|
message ListLoginPolicySecondFactorsResponse {
|
|
zitadel.v1.ListDetails details = 1;
|
|
repeated zitadel.policy.v1.SecondFactorType result = 2;
|
|
}
|
|
|
|
message AddSecondFactorToLoginPolicyRequest {
|
|
zitadel.policy.v1.SecondFactorType type = 1 [(validate.rules).enum = {defined_only: true, not_in: [0]}];
|
|
}
|
|
message AddSecondFactorToLoginPolicyResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message RemoveSecondFactorFromLoginPolicyRequest {
|
|
zitadel.policy.v1.SecondFactorType type = 1 [(validate.rules).enum = {defined_only: true, not_in: [0]}];
|
|
}
|
|
|
|
message RemoveSecondFactorFromLoginPolicyResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message ListLoginPolicyMultiFactorsRequest {}
|
|
|
|
message ListLoginPolicyMultiFactorsResponse {
|
|
zitadel.v1.ListDetails details = 1;
|
|
repeated zitadel.policy.v1.MultiFactorType result = 2;
|
|
}
|
|
|
|
message AddMultiFactorToLoginPolicyRequest {
|
|
zitadel.policy.v1.MultiFactorType type = 1 [(validate.rules).enum = {defined_only: true, not_in: [0]}];
|
|
}
|
|
|
|
message AddMultiFactorToLoginPolicyResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message RemoveMultiFactorFromLoginPolicyRequest {
|
|
zitadel.policy.v1.MultiFactorType type = 1 [(validate.rules).enum = {defined_only: true, not_in: [0]}];
|
|
}
|
|
|
|
message RemoveMultiFactorFromLoginPolicyResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message GetPasswordComplexityPolicyRequest {}
|
|
|
|
message GetPasswordComplexityPolicyResponse {
|
|
zitadel.policy.v1.PasswordComplexityPolicy policy = 1;
|
|
//deprecated: is_default is also defined in zitadel.policy.v1.PasswordComplexityPolicy
|
|
bool is_default = 2;
|
|
}
|
|
|
|
//This is an empty request
|
|
message GetDefaultPasswordComplexityPolicyRequest {}
|
|
|
|
message GetDefaultPasswordComplexityPolicyResponse {
|
|
zitadel.policy.v1.PasswordComplexityPolicy policy = 1;
|
|
}
|
|
|
|
message AddCustomPasswordComplexityPolicyRequest {
|
|
uint64 min_length = 1;
|
|
bool has_uppercase = 2;
|
|
bool has_lowercase = 3;
|
|
bool has_number = 4;
|
|
bool has_symbol = 5;
|
|
}
|
|
|
|
message AddCustomPasswordComplexityPolicyResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message UpdateCustomPasswordComplexityPolicyRequest {
|
|
uint64 min_length = 1;
|
|
bool has_uppercase = 2;
|
|
bool has_lowercase = 3;
|
|
bool has_number = 4;
|
|
bool has_symbol = 5;
|
|
}
|
|
|
|
message UpdateCustomPasswordComplexityPolicyResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
//This is an empty request
|
|
message ResetPasswordComplexityPolicyToDefaultRequest {}
|
|
|
|
message ResetPasswordComplexityPolicyToDefaultResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
//This is an empty request
|
|
message GetPasswordAgePolicyRequest {}
|
|
|
|
message GetPasswordAgePolicyResponse {
|
|
zitadel.policy.v1.PasswordAgePolicy policy = 1;
|
|
//deprecated: is_default is also defined in zitadel.policy.v1.PasswordAgePolicy
|
|
bool is_default = 2;
|
|
}
|
|
|
|
//This is an empty request
|
|
message GetDefaultPasswordAgePolicyRequest {}
|
|
|
|
message GetDefaultPasswordAgePolicyResponse {
|
|
zitadel.policy.v1.PasswordAgePolicy policy = 1;
|
|
}
|
|
|
|
message AddCustomPasswordAgePolicyRequest {
|
|
uint32 max_age_days = 1;
|
|
uint32 expire_warn_days = 2;
|
|
}
|
|
|
|
message AddCustomPasswordAgePolicyResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message UpdateCustomPasswordAgePolicyRequest {
|
|
uint32 max_age_days = 1;
|
|
uint32 expire_warn_days = 2;
|
|
}
|
|
|
|
message UpdateCustomPasswordAgePolicyResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
//This is an empty request
|
|
message ResetPasswordAgePolicyToDefaultRequest {}
|
|
|
|
message ResetPasswordAgePolicyToDefaultResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
//This is an empty request
|
|
message GetLockoutPolicyRequest {}
|
|
|
|
message GetLockoutPolicyResponse {
|
|
zitadel.policy.v1.LockoutPolicy policy = 1;
|
|
//deprecated: is_default is also defined in zitadel.policy.v1.LockoutPolicy
|
|
bool is_default = 2;
|
|
}
|
|
|
|
//This is an empty request
|
|
message GetDefaultLockoutPolicyRequest {}
|
|
|
|
message GetDefaultLockoutPolicyResponse {
|
|
zitadel.policy.v1.LockoutPolicy policy = 1;
|
|
}
|
|
|
|
message AddCustomLockoutPolicyRequest {
|
|
uint32 max_password_attempts = 1;
|
|
}
|
|
|
|
message AddCustomLockoutPolicyResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message UpdateCustomLockoutPolicyRequest {
|
|
uint32 max_password_attempts = 1;
|
|
}
|
|
|
|
message UpdateCustomLockoutPolicyResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
//This is an empty request
|
|
message ResetLockoutPolicyToDefaultRequest {}
|
|
|
|
message ResetLockoutPolicyToDefaultResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
//This is an empty request
|
|
message GetPrivacyPolicyRequest {}
|
|
|
|
message GetPrivacyPolicyResponse {
|
|
zitadel.policy.v1.PrivacyPolicy policy = 1;
|
|
}
|
|
|
|
//This is an empty request
|
|
message GetDefaultPrivacyPolicyRequest {}
|
|
|
|
message GetDefaultPrivacyPolicyResponse {
|
|
zitadel.policy.v1.PrivacyPolicy policy = 1;
|
|
}
|
|
|
|
message AddCustomPrivacyPolicyRequest {
|
|
string tos_link = 1;
|
|
string privacy_link = 2;
|
|
}
|
|
|
|
message AddCustomPrivacyPolicyResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message UpdateCustomPrivacyPolicyRequest {
|
|
string tos_link = 1;
|
|
string privacy_link = 2;
|
|
}
|
|
|
|
message UpdateCustomPrivacyPolicyResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
//This is an empty request
|
|
message ResetPrivacyPolicyToDefaultRequest {}
|
|
|
|
message ResetPrivacyPolicyToDefaultResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
//This is an empty request
|
|
message GetLabelPolicyRequest {}
|
|
|
|
message GetLabelPolicyResponse {
|
|
zitadel.policy.v1.LabelPolicy policy = 1;
|
|
//deprecated: is_default is also defined in zitadel.policy.v1.LabelPolicy
|
|
bool is_default = 2;
|
|
}
|
|
|
|
//This is an empty request
|
|
message GetPreviewLabelPolicyRequest {}
|
|
|
|
message GetPreviewLabelPolicyResponse {
|
|
zitadel.policy.v1.LabelPolicy policy = 1;
|
|
//deprecated: is_default is also defined in zitadel.policy.v1.LabelPolicy
|
|
bool is_default = 2;
|
|
}
|
|
|
|
//This is an empty request
|
|
message GetDefaultLabelPolicyRequest {}
|
|
|
|
message GetDefaultLabelPolicyResponse {
|
|
zitadel.policy.v1.LabelPolicy policy = 1;
|
|
}
|
|
|
|
message AddCustomLabelPolicyRequest {
|
|
string primary_color = 1 [(validate.rules).string = {max_len: 50}];
|
|
// hides the org suffix on the login form if the scope \"urn:zitadel:iam:org:domain:primary:{domainname}\" is set. Details about this scope in https://docs.zitadel.ch/concepts#Reserved_Scopes
|
|
bool hide_login_name_suffix = 3 [
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
description: "hides the org suffix on the login form if the scope \"urn:zitadel:iam:org:domain:primary:{domainname}\" is set. Details about this scope in https://docs.zitadel.ch/concepts#Reserved_Scopes";
|
|
}
|
|
];
|
|
string warn_color = 4 [(validate.rules).string = {max_len: 50}];
|
|
string background_color = 5 [(validate.rules).string = {max_len: 50}];
|
|
string font_color = 6 [(validate.rules).string = {max_len: 50}];
|
|
string primary_color_dark = 7 [(validate.rules).string = {max_len: 50}];
|
|
string background_color_dark = 8 [(validate.rules).string = {max_len: 50}];
|
|
string warn_color_dark = 9 [(validate.rules).string = {max_len: 50}];
|
|
string font_color_dark = 10 [(validate.rules).string = {max_len: 50}];
|
|
bool disable_watermark = 11;
|
|
}
|
|
|
|
message AddCustomLabelPolicyResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message UpdateCustomLabelPolicyRequest {
|
|
string primary_color = 1 [(validate.rules).string = {max_len: 50}];
|
|
bool hide_login_name_suffix = 3 [
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
description: "hides the org suffix on the login form if the scope \"urn:zitadel:iam:org:domain:primary:{domainname}\" is set. Details about this scope in https://docs.zitadel.ch/concepts#Reserved_Scopes";
|
|
}
|
|
];
|
|
string warn_color = 4 [(validate.rules).string = {max_len: 50}];
|
|
string background_color = 5 [(validate.rules).string = {max_len: 50}];
|
|
string font_color = 6 [(validate.rules).string = {max_len: 50}];
|
|
string primary_color_dark = 7 [(validate.rules).string = {max_len: 50}];
|
|
string background_color_dark = 8 [(validate.rules).string = {max_len: 50}];
|
|
string warn_color_dark = 9 [(validate.rules).string = {max_len: 50}];
|
|
string font_color_dark = 10 [(validate.rules).string = {max_len: 50}];
|
|
bool disable_watermark = 11;
|
|
}
|
|
|
|
message UpdateCustomLabelPolicyResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
//This is an empty request
|
|
message ActivateCustomLabelPolicyRequest {}
|
|
|
|
message ActivateCustomLabelPolicyResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
//This is an empty request
|
|
message RemoveCustomLabelPolicyLogoRequest {}
|
|
|
|
message RemoveCustomLabelPolicyLogoResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
//This is an empty request
|
|
message RemoveCustomLabelPolicyLogoDarkRequest {}
|
|
|
|
message RemoveCustomLabelPolicyLogoDarkResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
//This is an empty request
|
|
message RemoveCustomLabelPolicyIconRequest {}
|
|
|
|
message RemoveCustomLabelPolicyIconResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
//This is an empty request
|
|
message RemoveCustomLabelPolicyIconDarkRequest {}
|
|
|
|
message RemoveCustomLabelPolicyIconDarkResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
//This is an empty request
|
|
message RemoveCustomLabelPolicyFontRequest {}
|
|
|
|
message RemoveCustomLabelPolicyFontResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
//This is an empty request
|
|
message ResetLabelPolicyToDefaultRequest {}
|
|
|
|
message ResetLabelPolicyToDefaultResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message GetCustomInitMessageTextRequest {
|
|
string language = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message GetCustomInitMessageTextResponse {
|
|
zitadel.text.v1.MessageCustomText custom_text = 1;
|
|
}
|
|
|
|
message GetDefaultInitMessageTextRequest {
|
|
string language = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message GetDefaultInitMessageTextResponse {
|
|
zitadel.text.v1.MessageCustomText custom_text = 1;
|
|
}
|
|
|
|
message SetCustomInitMessageTextRequest {
|
|
string language = 1 [
|
|
(validate.rules).string = {min_len: 1, max_len: 200},
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"de\""
|
|
}
|
|
];
|
|
string title = 2 [(validate.rules).string = {max_len: 200}];
|
|
string pre_header = 3 [(validate.rules).string = {max_len: 200}];
|
|
string subject = 4 [(validate.rules).string = {max_len: 200}];
|
|
string greeting = 5 [(validate.rules).string = {max_len: 200}];
|
|
string text = 6 [(validate.rules).string = {max_len: 800}];
|
|
string button_text = 7 [(validate.rules).string = {max_len: 200}];
|
|
string footer_text = 8 [(validate.rules).string = {max_len: 200}];
|
|
}
|
|
|
|
message SetCustomInitMessageTextResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message ResetCustomInitMessageTextToDefaultRequest {
|
|
string language = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message ResetCustomInitMessageTextToDefaultResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message GetDefaultLoginTextsRequest {
|
|
string language = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message GetDefaultLoginTextsResponse {
|
|
zitadel.text.v1.LoginCustomText custom_text = 1;
|
|
}
|
|
|
|
message GetCustomLoginTextsRequest {
|
|
string language = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message GetCustomLoginTextsResponse {
|
|
zitadel.text.v1.LoginCustomText custom_text = 1;
|
|
}
|
|
|
|
message SetCustomLoginTextsRequest {
|
|
string language = 1 [
|
|
(validate.rules).string = {min_len: 1, max_len: 200},
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"de\""
|
|
}
|
|
];
|
|
zitadel.text.v1.SelectAccountScreenText select_account_text = 2;
|
|
zitadel.text.v1.LoginScreenText login_text = 3;
|
|
zitadel.text.v1.PasswordScreenText password_text = 4;
|
|
zitadel.text.v1.UsernameChangeScreenText username_change_text = 5;
|
|
zitadel.text.v1.UsernameChangeDoneScreenText username_change_done_text = 6;
|
|
zitadel.text.v1.InitPasswordScreenText init_password_text = 7;
|
|
zitadel.text.v1.InitPasswordDoneScreenText init_password_done_text = 8;
|
|
zitadel.text.v1.EmailVerificationScreenText email_verification_text = 9;
|
|
zitadel.text.v1.EmailVerificationDoneScreenText email_verification_done_text = 10;
|
|
zitadel.text.v1.InitializeUserScreenText initialize_user_text = 11;
|
|
zitadel.text.v1.InitializeUserDoneScreenText initialize_done_text = 12;
|
|
zitadel.text.v1.InitMFAPromptScreenText init_mfa_prompt_text = 13;
|
|
zitadel.text.v1.InitMFAOTPScreenText init_mfa_otp_text = 14;
|
|
zitadel.text.v1.InitMFAU2FScreenText init_mfa_u2f_text = 15;
|
|
zitadel.text.v1.InitMFADoneScreenText init_mfa_done_text = 16;
|
|
zitadel.text.v1.MFAProvidersText mfa_providers_text = 17;
|
|
zitadel.text.v1.VerifyMFAOTPScreenText verify_mfa_otp_text = 18;
|
|
zitadel.text.v1.VerifyMFAU2FScreenText verify_mfa_u2f_text = 19;
|
|
zitadel.text.v1.PasswordlessScreenText passwordless_text = 20;
|
|
zitadel.text.v1.PasswordChangeScreenText password_change_text = 21;
|
|
zitadel.text.v1.PasswordChangeDoneScreenText password_change_done_text = 22;
|
|
zitadel.text.v1.PasswordResetDoneScreenText password_reset_done_text = 23;
|
|
zitadel.text.v1.RegistrationOptionScreenText registration_option_text = 24;
|
|
zitadel.text.v1.RegistrationUserScreenText registration_user_text = 25;
|
|
zitadel.text.v1.RegistrationOrgScreenText registration_org_text = 26;
|
|
zitadel.text.v1.LinkingUserDoneScreenText linking_user_done_text = 27;
|
|
zitadel.text.v1.ExternalUserNotFoundScreenText external_user_not_found_text = 28;
|
|
zitadel.text.v1.SuccessLoginScreenText success_login_text = 29;
|
|
zitadel.text.v1.LogoutDoneScreenText logout_text = 30;
|
|
zitadel.text.v1.FooterText footer_text = 31;
|
|
zitadel.text.v1.PasswordlessPromptScreenText passwordless_prompt_text = 32;
|
|
zitadel.text.v1.PasswordlessRegistrationScreenText passwordless_registration_text = 33;
|
|
zitadel.text.v1.PasswordlessRegistrationDoneScreenText passwordless_registration_done_text = 34;
|
|
zitadel.text.v1.ExternalRegistrationUserOverviewScreenText external_registration_user_overview_text = 35;
|
|
}
|
|
|
|
message SetCustomLoginTextsResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
message ResetCustomLoginTextsToDefaultRequest {
|
|
string language = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message ResetCustomLoginTextsToDefaultResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message GetCustomPasswordResetMessageTextRequest {
|
|
string language = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message GetCustomPasswordResetMessageTextResponse {
|
|
zitadel.text.v1.MessageCustomText custom_text = 1;
|
|
}
|
|
|
|
message GetDefaultPasswordResetMessageTextRequest {
|
|
string language = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message GetDefaultPasswordResetMessageTextResponse {
|
|
zitadel.text.v1.MessageCustomText custom_text = 1;
|
|
}
|
|
|
|
message SetCustomPasswordResetMessageTextRequest {
|
|
string language = 1 [
|
|
(validate.rules).string = {min_len: 1, max_len: 200},
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"de\""
|
|
}
|
|
];
|
|
string title = 2 [(validate.rules).string = {max_len: 200}];
|
|
string pre_header = 3 [(validate.rules).string = {max_len: 200}];
|
|
string subject = 4 [(validate.rules).string = {max_len: 200}];
|
|
string greeting = 5 [(validate.rules).string = {max_len: 200}];
|
|
string text = 6 [(validate.rules).string = {max_len: 800}];
|
|
string button_text = 7 [(validate.rules).string = {max_len: 200}];
|
|
string footer_text = 8 [(validate.rules).string = {max_len: 200}];
|
|
}
|
|
|
|
message SetCustomPasswordResetMessageTextResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message ResetCustomPasswordResetMessageTextToDefaultRequest {
|
|
string language = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message ResetCustomPasswordResetMessageTextToDefaultResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message GetCustomVerifyEmailMessageTextRequest {
|
|
string language = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message GetCustomVerifyEmailMessageTextResponse {
|
|
zitadel.text.v1.MessageCustomText custom_text = 1;
|
|
}
|
|
|
|
message GetDefaultVerifyEmailMessageTextRequest {
|
|
string language = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message GetDefaultVerifyEmailMessageTextResponse {
|
|
zitadel.text.v1.MessageCustomText custom_text = 1;
|
|
}
|
|
|
|
message SetCustomVerifyEmailMessageTextRequest {
|
|
string language = 1 [
|
|
(validate.rules).string = {min_len: 1, max_len: 200},
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"de\""
|
|
}
|
|
];
|
|
string title = 2 [(validate.rules).string = {max_len: 200}];
|
|
string pre_header = 3 [(validate.rules).string = {max_len: 200}];
|
|
string subject = 4 [(validate.rules).string = {max_len: 200}];
|
|
string greeting = 5 [(validate.rules).string = {max_len: 200}];
|
|
string text = 6 [(validate.rules).string = {max_len: 800}];
|
|
string button_text = 7 [(validate.rules).string = {max_len: 200}];
|
|
string footer_text = 8 [(validate.rules).string = {max_len: 200}];
|
|
}
|
|
|
|
message SetCustomVerifyEmailMessageTextResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message ResetCustomVerifyEmailMessageTextToDefaultRequest {
|
|
string language = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message ResetCustomVerifyEmailMessageTextToDefaultResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message GetCustomVerifyPhoneMessageTextRequest {
|
|
string language = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message GetCustomVerifyPhoneMessageTextResponse {
|
|
zitadel.text.v1.MessageCustomText custom_text = 1;
|
|
}
|
|
|
|
message GetDefaultVerifyPhoneMessageTextRequest {
|
|
string language = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message GetDefaultVerifyPhoneMessageTextResponse {
|
|
zitadel.text.v1.MessageCustomText custom_text = 1;
|
|
}
|
|
|
|
message SetCustomVerifyPhoneMessageTextRequest {
|
|
string language = 1 [
|
|
(validate.rules).string = {min_len: 1, max_len: 200},
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"de\""
|
|
}
|
|
];
|
|
string title = 2 [(validate.rules).string = {max_len: 200}];
|
|
string pre_header = 3 [(validate.rules).string = {max_len: 200}];
|
|
string subject = 4 [(validate.rules).string = {max_len: 200}];
|
|
string greeting = 5 [(validate.rules).string = {max_len: 200}];
|
|
string text = 6 [(validate.rules).string = {max_len: 800}];
|
|
string button_text = 7 [(validate.rules).string = {max_len: 200}];
|
|
string footer_text = 8 [(validate.rules).string = {max_len: 200}];
|
|
}
|
|
|
|
message SetCustomVerifyPhoneMessageTextResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message ResetCustomVerifyPhoneMessageTextToDefaultRequest {
|
|
string language = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message ResetCustomVerifyPhoneMessageTextToDefaultResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message GetCustomDomainClaimedMessageTextRequest {
|
|
string language = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message GetCustomDomainClaimedMessageTextResponse {
|
|
zitadel.text.v1.MessageCustomText custom_text = 1;
|
|
}
|
|
|
|
message GetDefaultDomainClaimedMessageTextRequest {
|
|
string language = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message GetDefaultDomainClaimedMessageTextResponse {
|
|
zitadel.text.v1.MessageCustomText custom_text = 1;
|
|
}
|
|
|
|
message SetCustomDomainClaimedMessageTextRequest {
|
|
string language = 1 [
|
|
(validate.rules).string = {min_len: 1, max_len: 200},
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"de\""
|
|
}
|
|
];
|
|
string title = 2 [(validate.rules).string = {max_len: 200}];
|
|
string pre_header = 3 [(validate.rules).string = {max_len: 200}];
|
|
string subject = 4 [(validate.rules).string = {max_len: 200}];
|
|
string greeting = 5 [(validate.rules).string = {max_len: 200}];
|
|
string text = 6 [(validate.rules).string = {max_len: 800}];
|
|
string button_text = 7 [(validate.rules).string = {max_len: 200}];
|
|
string footer_text = 8 [(validate.rules).string = {max_len: 200}];
|
|
}
|
|
|
|
message SetCustomDomainClaimedMessageTextResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
//This is an empty request
|
|
message ResetCustomDomainClaimedMessageTextToDefaultRequest {
|
|
string language = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message ResetCustomDomainClaimedMessageTextToDefaultResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message GetCustomPasswordlessRegistrationMessageTextRequest {
|
|
string language = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message GetCustomPasswordlessRegistrationMessageTextResponse {
|
|
zitadel.text.v1.MessageCustomText custom_text = 1;
|
|
}
|
|
|
|
message GetDefaultPasswordlessRegistrationMessageTextRequest {
|
|
string language = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message GetDefaultPasswordlessRegistrationMessageTextResponse {
|
|
zitadel.text.v1.MessageCustomText custom_text = 1;
|
|
}
|
|
|
|
message SetCustomPasswordlessRegistrationMessageTextRequest {
|
|
string language = 1 [
|
|
(validate.rules).string = {min_len: 1, max_len: 200},
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"de\""
|
|
}
|
|
];
|
|
string title = 2 [(validate.rules).string = {max_len: 200}];
|
|
string pre_header = 3 [(validate.rules).string = {max_len: 200}];
|
|
string subject = 4 [(validate.rules).string = {max_len: 200}];
|
|
string greeting = 5 [(validate.rules).string = {max_len: 200}];
|
|
string text = 6 [(validate.rules).string = {max_len: 800}];
|
|
string button_text = 7 [(validate.rules).string = {max_len: 200}];
|
|
string footer_text = 8 [(validate.rules).string = {max_len: 200}];
|
|
}
|
|
|
|
message SetCustomPasswordlessRegistrationMessageTextResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message ResetCustomPasswordlessRegistrationMessageTextToDefaultRequest {
|
|
string language = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message ResetCustomPasswordlessRegistrationMessageTextToDefaultResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message GetOrgIDPByIDRequest {
|
|
string id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message GetOrgIDPByIDResponse {
|
|
zitadel.idp.v1.IDP idp = 1;
|
|
}
|
|
|
|
message ListOrgIDPsRequest {
|
|
//list limitations and ordering
|
|
zitadel.v1.ListQuery query = 1;
|
|
//the field the result is sorted
|
|
zitadel.idp.v1.IDPFieldName sorting_column = 2;
|
|
//criterias the client is looking for
|
|
repeated IDPQuery queries = 3;
|
|
}
|
|
|
|
message IDPQuery {
|
|
oneof query {
|
|
option (validate.required) = true;
|
|
|
|
zitadel.idp.v1.IDPIDQuery idp_id_query = 1;
|
|
zitadel.idp.v1.IDPNameQuery idp_name_query = 2;
|
|
zitadel.idp.v1.IDPOwnerTypeQuery owner_type_query = 3;
|
|
}
|
|
}
|
|
|
|
message ListOrgIDPsResponse {
|
|
zitadel.v1.ListDetails details = 1;
|
|
zitadel.idp.v1.IDPFieldName sorting_column = 2;
|
|
repeated zitadel.idp.v1.IDP result = 3;
|
|
}
|
|
|
|
message AddOrgOIDCIDPRequest {
|
|
string name = 1 [
|
|
(validate.rules).string = {min_len: 1, max_len: 200},
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"google\"";
|
|
}
|
|
];
|
|
zitadel.idp.v1.IDPStylingType styling_type = 2 [
|
|
(validate.rules).enum = {defined_only: true},
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
description: "some identity providers specify the styling of the button to their login";
|
|
}
|
|
];
|
|
|
|
string client_id = 3 [
|
|
(validate.rules).string = {min_len: 1, max_len: 200},
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
description: "client id generated by the identity provider";
|
|
}
|
|
];
|
|
string client_secret = 4 [
|
|
(validate.rules).string = {min_len: 1, max_len: 200},
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
description: "client secret generated by the identity provider";
|
|
}
|
|
];
|
|
string issuer = 5 [
|
|
(validate.rules).string = {min_len: 1, max_len: 200},
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"https://accounts.google.com\"";
|
|
description: "the oidc issuer of the identity provider";
|
|
}
|
|
];
|
|
repeated string scopes = 6 [
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "[\"openid\", \"profile\", \"email\"]";
|
|
description: "the scopes requested by ZITADEL during the request on the identity provider";
|
|
}
|
|
];
|
|
zitadel.idp.v1.OIDCMappingField display_name_mapping = 7 [
|
|
(validate.rules).enum = {defined_only: true},
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
description: "definition which field is mapped to the display name of the user";
|
|
}
|
|
];
|
|
zitadel.idp.v1.OIDCMappingField username_mapping = 8 [
|
|
(validate.rules).enum = {defined_only: true},
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
description: "definition which field is mapped to the email of the user";
|
|
}
|
|
];
|
|
bool auto_register = 9;
|
|
}
|
|
|
|
message AddOrgOIDCIDPResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
string idp_id = 2;
|
|
}
|
|
|
|
message AddOrgJWTIDPRequest {
|
|
string name = 1 [
|
|
(validate.rules).string = {min_len: 1, max_len: 200},
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"google\"";
|
|
}
|
|
];
|
|
zitadel.idp.v1.IDPStylingType styling_type = 2 [
|
|
(validate.rules).enum = {defined_only: true},
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
description: "some identity providers specify the styling of the button to their login";
|
|
}
|
|
];
|
|
string jwt_endpoint = 3 [
|
|
(validate.rules).string = {min_len: 1, max_len: 200},
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"https://accounts.google.com\"";
|
|
description: "the endpoint where the jwt can be extracted";
|
|
min_length: 1;
|
|
max_length: 200;
|
|
}
|
|
];
|
|
string issuer = 4 [
|
|
(validate.rules).string = {min_len: 1, max_len: 200},
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"https://accounts.google.com\"";
|
|
description: "the issuer of the jwt (for validation)";
|
|
min_length: 1;
|
|
max_length: 200;
|
|
}
|
|
];
|
|
string keys_endpoint = 5 [
|
|
(validate.rules).string = {min_len: 1, max_len: 200},
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"https://accounts.google.com/keys\"";
|
|
description: "the endpoint to the key (JWK) which are used to sign the JWT with";
|
|
min_length: 1;
|
|
max_length: 200;
|
|
}
|
|
];
|
|
string header_name = 6 [
|
|
(validate.rules).string = {min_len: 1, max_len: 200},
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"x-auth-token\"";
|
|
description: "the name of the header where the JWT is sent in, default is authorization";
|
|
max_length: 200;
|
|
}
|
|
];
|
|
bool auto_register = 7;
|
|
}
|
|
|
|
message AddOrgJWTIDPResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
string idp_id = 2;
|
|
}
|
|
|
|
message DeactivateOrgIDPRequest {
|
|
string idp_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message DeactivateOrgIDPResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message ReactivateOrgIDPRequest {
|
|
string idp_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
message ReactivateOrgIDPResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message RemoveOrgIDPRequest {
|
|
string idp_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
}
|
|
|
|
//This is an empty response
|
|
message RemoveOrgIDPResponse {}
|
|
|
|
message UpdateOrgIDPRequest {
|
|
string idp_id = 1 [(validate.rules).string = {min_len: 1, max_len: 200}];
|
|
string name = 2 [
|
|
(validate.rules).string = {min_len: 1, max_len: 200},
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"google\"";
|
|
}
|
|
];
|
|
zitadel.idp.v1.IDPStylingType styling_type = 3 [
|
|
(validate.rules).enum = {defined_only: true},
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
description: "some identity providers specify the styling of the button to their login";
|
|
}
|
|
];
|
|
bool auto_register = 4;
|
|
}
|
|
|
|
message UpdateOrgIDPResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message UpdateOrgIDPOIDCConfigRequest {
|
|
string idp_id = 1 [
|
|
(validate.rules).string = {min_len: 1, max_len: 200},
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"69629023906488334\"";
|
|
}
|
|
];
|
|
|
|
string client_id = 2 [
|
|
(validate.rules).string = {min_len: 1, max_len: 200},
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
description: "client id generated by the identity provider";
|
|
}
|
|
];
|
|
string client_secret = 3 [
|
|
(validate.rules).string = {max_len: 200},
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
description: "client secret generated by the identity provider. If empty the secret is not overwritten";
|
|
}
|
|
];
|
|
string issuer = 4 [
|
|
(validate.rules).string = {min_len: 1, max_len: 200},
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"https://accounts.google.com\"";
|
|
description: "the oidc issuer of the identity provider";
|
|
}
|
|
];
|
|
repeated string scopes = 5 [
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "[\"openid\", \"profile\", \"email\"]";
|
|
description: "the scopes requested by ZITADEL during the request on the identity provider";
|
|
}
|
|
];
|
|
zitadel.idp.v1.OIDCMappingField display_name_mapping = 6 [
|
|
(validate.rules).enum = {defined_only: true},
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
description: "definition which field is mapped to the display name of the user";
|
|
}
|
|
];
|
|
zitadel.idp.v1.OIDCMappingField username_mapping = 7 [
|
|
(validate.rules).enum = {defined_only: true},
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
description: "definition which field is mapped to the email of the user";
|
|
}
|
|
];
|
|
}
|
|
|
|
message UpdateOrgIDPOIDCConfigResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
|
|
message UpdateOrgIDPJWTConfigRequest {
|
|
string idp_id = 1 [
|
|
(validate.rules).string = {min_len: 1, max_len: 200},
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"69629023906488334\"";
|
|
}
|
|
];
|
|
string jwt_endpoint = 2 [
|
|
(validate.rules).string = {min_len: 1, max_len: 200},
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"https://accounts.google.com\"";
|
|
description: "the endpoint where the jwt can be extracted";
|
|
min_length: 1;
|
|
max_length: 200;
|
|
}
|
|
];
|
|
string issuer = 3 [
|
|
(validate.rules).string = {min_len: 1, max_len: 200},
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"https://accounts.google.com\"";
|
|
description: "the issuer of the jwt (for validation)";
|
|
min_length: 1;
|
|
max_length: 200;
|
|
}
|
|
];
|
|
string keys_endpoint = 4 [
|
|
(validate.rules).string = {min_len: 1, max_len: 200},
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"https://accounts.google.com/keys\"";
|
|
description: "the endpoint to the key (JWK) which are used to sign the JWT with";
|
|
min_length: 1;
|
|
max_length: 200;
|
|
}
|
|
];
|
|
string header_name = 5 [
|
|
(validate.rules).string = {min_len: 1, max_len: 200},
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"x-auth-token\"";
|
|
description: "the name of the header where the JWT is sent in, default is authorization";
|
|
max_length: 200;
|
|
}
|
|
];
|
|
}
|
|
|
|
message UpdateOrgIDPJWTConfigResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message ListActionsRequest {
|
|
//list limitations and ordering
|
|
zitadel.v1.ListQuery query = 1;
|
|
//the field the result is sorted
|
|
zitadel.action.v1.ActionFieldName sorting_column = 2;
|
|
//criteria the client is looking for
|
|
repeated ActionQuery queries = 3;
|
|
}
|
|
|
|
message ActionQuery {
|
|
oneof query {
|
|
option (validate.required) = true;
|
|
|
|
zitadel.action.v1.ActionIDQuery action_id_query = 1;
|
|
zitadel.action.v1.ActionNameQuery action_name_query = 2;
|
|
zitadel.action.v1.ActionStateQuery action_state_query = 3;
|
|
}
|
|
}
|
|
|
|
message ListActionsResponse {
|
|
zitadel.v1.ListDetails details = 1;
|
|
zitadel.action.v1.ActionFieldName sorting_column = 2;
|
|
repeated zitadel.action.v1.Action result = 3;
|
|
}
|
|
|
|
message CreateActionRequest {
|
|
string name = 1 [
|
|
(validate.rules).string = {min_len: 1, max_len: 200},
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"log context\"";
|
|
}
|
|
];
|
|
string script = 2 [
|
|
(validate.rules).string = {min_len: 1, max_len: 2000},
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"function log(context, calls){console.log(context)}\"";
|
|
}
|
|
];
|
|
google.protobuf.Duration timeout = 3 [
|
|
(validate.rules).duration = {gte: {}, lte: {seconds: 20}},
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
description: "after which time the action will be terminated if not finished";
|
|
}
|
|
];
|
|
bool allowed_to_fail = 4 [
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
description: "when true, the next action will be called even if this action fails";
|
|
}
|
|
];
|
|
}
|
|
|
|
message CreateActionResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
string id = 2;
|
|
}
|
|
|
|
message GetActionRequest {
|
|
string id = 1;
|
|
}
|
|
|
|
message GetActionResponse {
|
|
zitadel.action.v1.Action action = 1;
|
|
}
|
|
|
|
message UpdateActionRequest {
|
|
string id = 1 [
|
|
(validate.rules).string = {min_len: 1, max_len: 200},
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"69629023906488334\"";
|
|
}
|
|
];
|
|
string name = 2 [
|
|
(validate.rules).string = {min_len: 1, max_len: 200},
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"log context\"";
|
|
}
|
|
];
|
|
string script = 3 [
|
|
(validate.rules).string = {min_len: 1, max_len: 2000},
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"function log(context, calls){console.log(context)}\"";
|
|
}
|
|
];
|
|
google.protobuf.Duration timeout = 4 [
|
|
(validate.rules).duration = {gte: {}, lte: {seconds: 20}},
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
description: "after which time the action will be terminated if not finished";
|
|
}
|
|
];
|
|
bool allowed_to_fail = 5 [
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
description: "when true, the next action will be called even if this action fails";
|
|
}
|
|
];
|
|
}
|
|
|
|
message UpdateActionResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message DeleteActionRequest {
|
|
string id = 1;
|
|
}
|
|
|
|
message DeleteActionResponse {}
|
|
|
|
message DeactivateActionRequest {
|
|
string id = 1;
|
|
}
|
|
|
|
message DeactivateActionResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message ReactivateActionRequest {
|
|
string id = 1;
|
|
}
|
|
|
|
message ReactivateActionResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message GetFlowRequest {
|
|
zitadel.action.v1.FlowType type = 1;
|
|
}
|
|
|
|
message GetFlowResponse {
|
|
zitadel.action.v1.Flow flow = 1;
|
|
}
|
|
|
|
message ClearFlowRequest {
|
|
zitadel.action.v1.FlowType type = 1;
|
|
}
|
|
|
|
message ClearFlowResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|
|
|
|
message SetTriggerActionsRequest {
|
|
zitadel.action.v1.FlowType flow_type = 1;
|
|
zitadel.action.v1.TriggerType trigger_type = 2;
|
|
repeated string action_ids = 3;
|
|
}
|
|
|
|
message SetTriggerActionsResponse {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
}
|