mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 19:14:23 +00:00
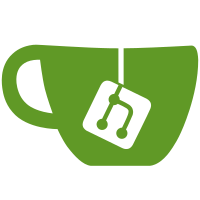
* feat(api): add google provider template * refactor reduce functions * handle removed event * linting * fix projection * feat(api): add generic oauth provider template * feat(api): add github provider templates * feat(api): add github provider templates * fixes * proto comment * fix filtering * requested changes * feat(api): add generic oauth provider template * remove wrongly committed message * increase budget for angular build * fix linting * fixes * fix merge * fix merge * fix projection * fix merge * updates from previous PRs * enable github providers in login * fix merge * fix test and add github styling in login * cleanup * feat(api): add gitlab provider templates * fix: merge * fix display of providers in login * implement gitlab in login and make prompt `select_account` optional since gitlab can't handle it * fix merge * fix merge and add tests for command side * requested changes * requested changes * Update internal/query/idp_template.go Co-authored-by: Silvan <silvan.reusser@gmail.com> * fix merge * requested changes --------- Co-authored-by: Silvan <silvan.reusser@gmail.com>
66 lines
1.6 KiB
Go
66 lines
1.6 KiB
Go
package gitlab
|
|
|
|
import (
|
|
"context"
|
|
"testing"
|
|
|
|
"github.com/stretchr/testify/assert"
|
|
"github.com/stretchr/testify/require"
|
|
|
|
"github.com/zitadel/zitadel/internal/idp"
|
|
"github.com/zitadel/zitadel/internal/idp/providers/oidc"
|
|
)
|
|
|
|
func TestProvider_BeginAuth(t *testing.T) {
|
|
type fields struct {
|
|
clientID string
|
|
clientSecret string
|
|
redirectURI string
|
|
scopes []string
|
|
opts []oidc.ProviderOpts
|
|
}
|
|
tests := []struct {
|
|
name string
|
|
fields fields
|
|
want idp.Session
|
|
}{
|
|
{
|
|
name: "successful auth",
|
|
fields: fields{
|
|
clientID: "clientID",
|
|
clientSecret: "clientSecret",
|
|
redirectURI: "redirectURI",
|
|
scopes: []string{"openid"},
|
|
},
|
|
want: &oidc.Session{
|
|
AuthURL: "https://gitlab.com/oauth/authorize?client_id=clientID&redirect_uri=redirectURI&response_type=code&scope=openid&state=testState",
|
|
},
|
|
},
|
|
{
|
|
name: "successful auth default scopes",
|
|
fields: fields{
|
|
clientID: "clientID",
|
|
clientSecret: "clientSecret",
|
|
redirectURI: "redirectURI",
|
|
},
|
|
want: &oidc.Session{
|
|
AuthURL: "https://gitlab.com/oauth/authorize?client_id=clientID&redirect_uri=redirectURI&response_type=code&scope=openid+profile+email&state=testState",
|
|
},
|
|
},
|
|
}
|
|
for _, tt := range tests {
|
|
t.Run(tt.name, func(t *testing.T) {
|
|
a := assert.New(t)
|
|
r := require.New(t)
|
|
|
|
provider, err := New(tt.fields.clientID, tt.fields.clientSecret, tt.fields.redirectURI, tt.fields.scopes, tt.fields.opts...)
|
|
r.NoError(err)
|
|
|
|
session, err := provider.BeginAuth(context.Background(), "testState")
|
|
r.NoError(err)
|
|
|
|
a.Equal(tt.want.GetAuthURL(), session.GetAuthURL())
|
|
})
|
|
}
|
|
}
|