mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
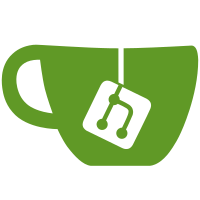
* features * features * features * fix json tags * add features handler to auth * mocks for tests * add setup step * fixes * add featurelist to auth api * grandfather state and typos * typo * merge new-eventstore * fix login policy tests * label policy in features * audit log retention
49 lines
1.6 KiB
Go
49 lines
1.6 KiB
Go
package view
|
|
|
|
import (
|
|
"github.com/jinzhu/gorm"
|
|
|
|
"github.com/caos/zitadel/internal/domain"
|
|
caos_errs "github.com/caos/zitadel/internal/errors"
|
|
"github.com/caos/zitadel/internal/features/model"
|
|
view_model "github.com/caos/zitadel/internal/features/repository/view/model"
|
|
"github.com/caos/zitadel/internal/view/repository"
|
|
)
|
|
|
|
func GetDefaultFeatures(db *gorm.DB, table string) ([]*view_model.FeaturesView, error) {
|
|
features := make([]*view_model.FeaturesView, 0)
|
|
queries := []*model.FeaturesSearchQuery{
|
|
{Key: model.FeaturesSearchKeyDefault, Value: true, Method: domain.SearchMethodEquals},
|
|
}
|
|
query := repository.PrepareSearchQuery(table, view_model.FeaturesSearchRequest{Queries: queries})
|
|
_, err := query(db, &features)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return features, nil
|
|
}
|
|
|
|
func GetFeaturesByAggregateID(db *gorm.DB, table, aggregateID string) (*view_model.FeaturesView, error) {
|
|
features := new(view_model.FeaturesView)
|
|
query := repository.PrepareGetByKey(table, view_model.FeaturesSearchKey(model.FeaturesSearchKeyAggregateID), aggregateID)
|
|
err := query(db, features)
|
|
if caos_errs.IsNotFound(err) {
|
|
return nil, caos_errs.ThrowNotFound(nil, "VIEW-Dbf3h", "Errors.Features.NotFound")
|
|
}
|
|
return features, err
|
|
}
|
|
|
|
func PutFeatures(db *gorm.DB, table string, features *view_model.FeaturesView) error {
|
|
save := repository.PrepareSave(table)
|
|
return save(db, features)
|
|
}
|
|
|
|
func PutFeaturesList(db *gorm.DB, table string, featuresList ...*view_model.FeaturesView) error {
|
|
save := repository.PrepareBulkSave(table)
|
|
f := make([]interface{}, len(featuresList))
|
|
for i, features := range featuresList {
|
|
f[i] = features
|
|
}
|
|
return save(db, f...)
|
|
}
|