mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 19:14:23 +00:00
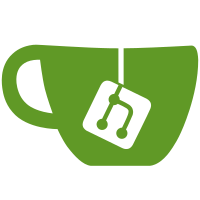
# Which Problems Are Solved ZITADEL currently always uses `urn:oasis:names:tc:SAML:2.0:nameid-format:persistent` in SAML requests, relying on the IdP to respect that flag and always return a peristent nameid in order to be able to map the external user with an existing user (idp link) in ZITADEL. In case the IdP however returns a `urn:oasis:names:tc:SAML:2.0:nameid-format:transient` (transient) nameid, the attribute will differ between each request and it will not be possible to match existing users. # How the Problems Are Solved This PR adds the following two options on SAML IdP: - **nameIDFormat**: allows to set the nameid-format used in the SAML Request - **transientMappingAttributeName**: allows to set an attribute name, which will be used instead of the nameid itself in case the returned nameid-format is transient # Additional Changes To reduce impact on current installations, the `idp_templates6_saml` table is altered with the two added columns by a setup job. New installations will automatically get the table with the two columns directly. All idp unit tests are updated to use `expectEventstore` instead of the deprecated `eventstoreExpect`. # Additional Context Closes #7483 Closes #7743 --------- Co-authored-by: peintnermax <max@caos.ch> Co-authored-by: Stefan Benz <46600784+stebenz@users.noreply.github.com>
183 lines
5.4 KiB
Go
183 lines
5.4 KiB
Go
package idp
|
|
|
|
import (
|
|
"github.com/zitadel/zitadel/internal/crypto"
|
|
"github.com/zitadel/zitadel/internal/domain"
|
|
"github.com/zitadel/zitadel/internal/eventstore"
|
|
"github.com/zitadel/zitadel/internal/zerrors"
|
|
)
|
|
|
|
type SAMLIDPAddedEvent struct {
|
|
eventstore.BaseEvent `json:"-"`
|
|
|
|
ID string `json:"id"`
|
|
Name string `json:"name,omitempty"`
|
|
Metadata []byte `json:"metadata,omitempty"`
|
|
Key *crypto.CryptoValue `json:"key,omitempty"`
|
|
Certificate []byte `json:"certificate,omitempty"`
|
|
Binding string `json:"binding,omitempty"`
|
|
WithSignedRequest bool `json:"withSignedRequest,omitempty"`
|
|
NameIDFormat *domain.SAMLNameIDFormat `json:"nameIDFormat,omitempty"`
|
|
TransientMappingAttributeName string `json:"transientMappingAttributeName,omitempty"`
|
|
Options
|
|
}
|
|
|
|
func NewSAMLIDPAddedEvent(
|
|
base *eventstore.BaseEvent,
|
|
id,
|
|
name string,
|
|
metadata []byte,
|
|
key *crypto.CryptoValue,
|
|
certificate []byte,
|
|
binding string,
|
|
withSignedRequest bool,
|
|
nameIDFormat *domain.SAMLNameIDFormat,
|
|
transientMappingAttributeName string,
|
|
options Options,
|
|
) *SAMLIDPAddedEvent {
|
|
return &SAMLIDPAddedEvent{
|
|
BaseEvent: *base,
|
|
ID: id,
|
|
Name: name,
|
|
Metadata: metadata,
|
|
Key: key,
|
|
Certificate: certificate,
|
|
Binding: binding,
|
|
WithSignedRequest: withSignedRequest,
|
|
NameIDFormat: nameIDFormat,
|
|
TransientMappingAttributeName: transientMappingAttributeName,
|
|
Options: options,
|
|
}
|
|
}
|
|
|
|
func (e *SAMLIDPAddedEvent) Payload() interface{} {
|
|
return e
|
|
}
|
|
|
|
func (e *SAMLIDPAddedEvent) UniqueConstraints() []*eventstore.UniqueConstraint {
|
|
return nil
|
|
}
|
|
|
|
func SAMLIDPAddedEventMapper(event eventstore.Event) (eventstore.Event, error) {
|
|
e := &SAMLIDPAddedEvent{
|
|
BaseEvent: *eventstore.BaseEventFromRepo(event),
|
|
}
|
|
|
|
err := event.Unmarshal(e)
|
|
if err != nil {
|
|
return nil, zerrors.ThrowInternal(err, "IDP-v9uajo3k71", "unable to unmarshal event")
|
|
}
|
|
|
|
return e, nil
|
|
}
|
|
|
|
type SAMLIDPChangedEvent struct {
|
|
eventstore.BaseEvent `json:"-"`
|
|
|
|
ID string `json:"id"`
|
|
Name *string `json:"name,omitempty"`
|
|
Metadata []byte `json:"metadata,omitempty"`
|
|
Key *crypto.CryptoValue `json:"key,omitempty"`
|
|
Certificate []byte `json:"certificate,omitempty"`
|
|
Binding *string `json:"binding,omitempty"`
|
|
WithSignedRequest *bool `json:"withSignedRequest,omitempty"`
|
|
NameIDFormat *domain.SAMLNameIDFormat `json:"nameIDFormat,omitempty"`
|
|
TransientMappingAttributeName *string `json:"transientMappingAttributeName,omitempty"`
|
|
OptionChanges
|
|
}
|
|
|
|
func NewSAMLIDPChangedEvent(
|
|
base *eventstore.BaseEvent,
|
|
id string,
|
|
changes []SAMLIDPChanges,
|
|
) (*SAMLIDPChangedEvent, error) {
|
|
if len(changes) == 0 {
|
|
return nil, zerrors.ThrowPreconditionFailed(nil, "IDP-cz6mnf860t", "Errors.NoChangesFound")
|
|
}
|
|
changedEvent := &SAMLIDPChangedEvent{
|
|
BaseEvent: *base,
|
|
ID: id,
|
|
}
|
|
for _, change := range changes {
|
|
change(changedEvent)
|
|
}
|
|
return changedEvent, nil
|
|
}
|
|
|
|
type SAMLIDPChanges func(*SAMLIDPChangedEvent)
|
|
|
|
func ChangeSAMLName(name string) func(*SAMLIDPChangedEvent) {
|
|
return func(e *SAMLIDPChangedEvent) {
|
|
e.Name = &name
|
|
}
|
|
}
|
|
|
|
func ChangeSAMLMetadata(metadata []byte) func(*SAMLIDPChangedEvent) {
|
|
return func(e *SAMLIDPChangedEvent) {
|
|
e.Metadata = metadata
|
|
}
|
|
}
|
|
|
|
func ChangeSAMLKey(key *crypto.CryptoValue) func(*SAMLIDPChangedEvent) {
|
|
return func(e *SAMLIDPChangedEvent) {
|
|
e.Key = key
|
|
}
|
|
}
|
|
|
|
func ChangeSAMLCertificate(certificate []byte) func(*SAMLIDPChangedEvent) {
|
|
return func(e *SAMLIDPChangedEvent) {
|
|
e.Certificate = certificate
|
|
}
|
|
}
|
|
|
|
func ChangeSAMLBinding(binding string) func(*SAMLIDPChangedEvent) {
|
|
return func(e *SAMLIDPChangedEvent) {
|
|
e.Binding = &binding
|
|
}
|
|
}
|
|
|
|
func ChangeSAMLWithSignedRequest(withSignedRequest bool) func(*SAMLIDPChangedEvent) {
|
|
return func(e *SAMLIDPChangedEvent) {
|
|
e.WithSignedRequest = &withSignedRequest
|
|
}
|
|
}
|
|
|
|
func ChangeSAMLNameIDFormat(nameIDFormat *domain.SAMLNameIDFormat) func(*SAMLIDPChangedEvent) {
|
|
return func(e *SAMLIDPChangedEvent) {
|
|
e.NameIDFormat = nameIDFormat
|
|
}
|
|
}
|
|
|
|
func ChangeSAMLTransientMappingAttributeName(name string) func(*SAMLIDPChangedEvent) {
|
|
return func(e *SAMLIDPChangedEvent) {
|
|
e.TransientMappingAttributeName = &name
|
|
}
|
|
}
|
|
|
|
func ChangeSAMLOptions(options OptionChanges) func(*SAMLIDPChangedEvent) {
|
|
return func(e *SAMLIDPChangedEvent) {
|
|
e.OptionChanges = options
|
|
}
|
|
}
|
|
|
|
func (e *SAMLIDPChangedEvent) Payload() interface{} {
|
|
return e
|
|
}
|
|
|
|
func (e *SAMLIDPChangedEvent) UniqueConstraints() []*eventstore.UniqueConstraint {
|
|
return nil
|
|
}
|
|
|
|
func SAMLIDPChangedEventMapper(event eventstore.Event) (eventstore.Event, error) {
|
|
e := &SAMLIDPChangedEvent{
|
|
BaseEvent: *eventstore.BaseEventFromRepo(event),
|
|
}
|
|
|
|
err := event.Unmarshal(e)
|
|
if err != nil {
|
|
return nil, zerrors.ThrowInternal(err, "IDP-w1t1824tw5", "unable to unmarshal event")
|
|
}
|
|
|
|
return e, nil
|
|
}
|