mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
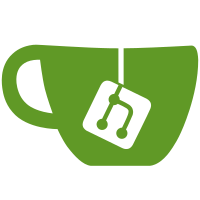
* feat: org remove on admin api and org query with state * docs: change description for admin api remove org Co-authored-by: Livio Spring <livio.a@gmail.com>
153 lines
4.2 KiB
Protocol Buffer
153 lines
4.2 KiB
Protocol Buffer
syntax = "proto3";
|
|
|
|
import "zitadel/object.proto";
|
|
import "validate/validate.proto";
|
|
import "protoc-gen-openapiv2/options/annotations.proto";
|
|
|
|
package zitadel.org.v1;
|
|
|
|
option go_package ="github.com/zitadel/zitadel/pkg/grpc/org";
|
|
|
|
message Org {
|
|
string id = 1 [
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"69629023906488334\""
|
|
}
|
|
];
|
|
zitadel.v1.ObjectDetails details = 2;
|
|
OrgState state = 3 [
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
description: "current state of the organisation";
|
|
}
|
|
];
|
|
string name = 4 [
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"CAOS AG\"";
|
|
}
|
|
];
|
|
string primary_domain = 5 [
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"caos.ch\"";
|
|
}
|
|
];
|
|
}
|
|
|
|
enum OrgState {
|
|
ORG_STATE_UNSPECIFIED = 0;
|
|
ORG_STATE_ACTIVE = 1;
|
|
ORG_STATE_INACTIVE = 2;
|
|
ORG_STATE_REMOVED = 3;
|
|
}
|
|
|
|
message Domain {
|
|
string org_id = 1 [
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"69629023906488334\""
|
|
}
|
|
];
|
|
zitadel.v1.ObjectDetails details = 2;
|
|
string domain_name = 3 [
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"caos.ch\"";
|
|
}
|
|
];
|
|
bool is_verified = 4 [
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
description: "defines if the domain is verified"
|
|
}
|
|
];
|
|
bool is_primary = 5 [
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
description: "defines if the domain is the primary domain"
|
|
}
|
|
];
|
|
DomainValidationType validation_type = 6 [
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
description: "defines the protocol the domain was validated with";
|
|
}
|
|
];
|
|
}
|
|
|
|
enum DomainValidationType {
|
|
DOMAIN_VALIDATION_TYPE_UNSPECIFIED = 0;
|
|
DOMAIN_VALIDATION_TYPE_HTTP = 1;
|
|
DOMAIN_VALIDATION_TYPE_DNS = 2;
|
|
}
|
|
|
|
message OrgQuery {
|
|
oneof query {
|
|
option (validate.required) = true;
|
|
|
|
OrgNameQuery name_query = 1;
|
|
OrgDomainQuery domain_query = 2;
|
|
OrgStateQuery state_query = 3;
|
|
}
|
|
}
|
|
|
|
message OrgNameQuery {
|
|
string name = 1 [
|
|
(validate.rules).string = {max_len: 200},
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"caos ag\"";
|
|
}
|
|
];
|
|
zitadel.v1.TextQueryMethod method = 2 [
|
|
(validate.rules).enum.defined_only = true,
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
description: "defines which text equality method is used";
|
|
}
|
|
];
|
|
}
|
|
|
|
message OrgDomainQuery {
|
|
string domain = 1 [
|
|
(validate.rules).string = {max_len: 200},
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"CAOS.C\"";
|
|
}
|
|
];
|
|
zitadel.v1.TextQueryMethod method = 2 [
|
|
(validate.rules).enum.defined_only = true,
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
description: "defines which text equality method is used";
|
|
}
|
|
];
|
|
}
|
|
|
|
message OrgStateQuery {
|
|
OrgState state = 1 [
|
|
(validate.rules).enum.defined_only = true,
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
description: "current state of the organisation";
|
|
}
|
|
];
|
|
}
|
|
|
|
enum OrgFieldName {
|
|
ORG_FIELD_NAME_UNSPECIFIED = 0;
|
|
ORG_FIELD_NAME_NAME = 1;
|
|
}
|
|
|
|
message DomainSearchQuery {
|
|
oneof query {
|
|
option (validate.required) = true;
|
|
|
|
DomainNameQuery domain_name_query = 1;
|
|
}
|
|
}
|
|
|
|
message DomainNameQuery {
|
|
string name = 1 [
|
|
(validate.rules).string = {max_len: 200},
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"caos.ch\"";
|
|
}
|
|
];
|
|
zitadel.v1.TextQueryMethod method = 2 [
|
|
(validate.rules).enum.defined_only = true,
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
description: "defines which text equality method is used";
|
|
}
|
|
];
|
|
}
|