mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
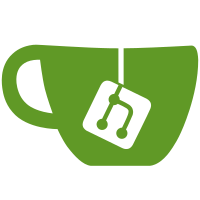
* chore(proto): update versions
* change protoc plugin
* some cleanups
* define api for setting emails in new api
* implement user.SetEmail
* move SetEmail buisiness logic into command
* resuse newCryptoCode
* command: add ChangeEmail unit tests
Not complete, was not able to mock the generator.
* Revert "resuse newCryptoCode"
This reverts commit c89e90ae35
.
* undo change to crypto code generators
* command: use a generator so we can test properly
* command: reorganise ChangeEmail
improve test coverage
* implement VerifyEmail
including unit tests
* add URL template tests
* begin user creation
* change protos
* implement metadata and move context
* merge commands
* proto: change context to object
* remove old auth option
* remove old auth option
* fix linting errors
run gci on modified files
* add permission checks and fix some errors
* comments
* comments
* update email requests
* rename proto requests
* cleanup and docs
* simplify
* simplify
* fix setup
* remove unused proto messages / fields
---------
Co-authored-by: adlerhurst <silvan.reusser@gmail.com>
Co-authored-by: Tim Möhlmann <tim+github@zitadel.com>
77 lines
2.3 KiB
Go
77 lines
2.3 KiB
Go
package command
|
|
|
|
import (
|
|
"context"
|
|
"time"
|
|
|
|
"github.com/zitadel/zitadel/internal/command/preparation"
|
|
"github.com/zitadel/zitadel/internal/crypto"
|
|
"github.com/zitadel/zitadel/internal/domain"
|
|
"github.com/zitadel/zitadel/internal/errors"
|
|
)
|
|
|
|
type CryptoCodeWithExpiry struct {
|
|
Crypted *crypto.CryptoValue
|
|
Plain string
|
|
Expiry time.Duration
|
|
}
|
|
|
|
func newCryptoCodeWithExpiry(ctx context.Context, filter preparation.FilterToQueryReducer, typ domain.SecretGeneratorType, alg crypto.Crypto) (*CryptoCodeWithExpiry, error) {
|
|
config, err := secretGeneratorConfig(ctx, filter, typ)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
code := new(CryptoCodeWithExpiry)
|
|
switch a := alg.(type) {
|
|
case crypto.HashAlgorithm:
|
|
code.Crypted, code.Plain, err = crypto.NewCode(crypto.NewHashGenerator(*config, a))
|
|
case crypto.EncryptionAlgorithm:
|
|
code.Crypted, code.Plain, err = crypto.NewCode(crypto.NewEncryptionGenerator(*config, a))
|
|
default:
|
|
return nil, errors.ThrowInternal(nil, "COMMA-RreV6", "Errors.Internal")
|
|
}
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
code.Expiry = config.Expiry
|
|
return code, nil
|
|
}
|
|
|
|
func newCryptoCodeWithPlain(ctx context.Context, filter preparation.FilterToQueryReducer, typ domain.SecretGeneratorType, alg crypto.Crypto) (value *crypto.CryptoValue, plain string, err error) {
|
|
config, err := secretGeneratorConfig(ctx, filter, typ)
|
|
if err != nil {
|
|
return nil, "", err
|
|
}
|
|
|
|
switch a := alg.(type) {
|
|
case crypto.HashAlgorithm:
|
|
return crypto.NewCode(crypto.NewHashGenerator(*config, a))
|
|
case crypto.EncryptionAlgorithm:
|
|
return crypto.NewCode(crypto.NewEncryptionGenerator(*config, a))
|
|
}
|
|
|
|
return nil, "", errors.ThrowInvalidArgument(nil, "V2-NGESt", "Errors.Internal")
|
|
}
|
|
|
|
func secretGeneratorConfig(ctx context.Context, filter preparation.FilterToQueryReducer, typ domain.SecretGeneratorType) (*crypto.GeneratorConfig, error) {
|
|
wm := NewInstanceSecretGeneratorConfigWriteModel(ctx, typ)
|
|
events, err := filter(ctx, wm.Query())
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
wm.AppendEvents(events...)
|
|
if err := wm.Reduce(); err != nil {
|
|
return nil, err
|
|
}
|
|
return &crypto.GeneratorConfig{
|
|
Length: wm.Length,
|
|
Expiry: wm.Expiry,
|
|
IncludeLowerLetters: wm.IncludeLowerLetters,
|
|
IncludeUpperLetters: wm.IncludeUpperLetters,
|
|
IncludeDigits: wm.IncludeDigits,
|
|
IncludeSymbols: wm.IncludeSymbols,
|
|
}, nil
|
|
}
|