mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
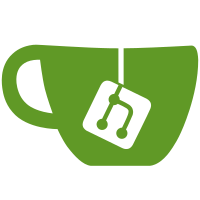
* project quota added * project quota removed * add periods table * make log record generic * accumulate usage * query usage * count action run seconds * fix filter in ReportQuotaUsage * fix existing tests * fix logstore tests * fix typo * fix: add quota unit tests command side * fix: add quota unit tests command side * fix: add quota unit tests command side * move notifications into debouncer and improve limit querying * cleanup * comment * fix: add quota unit tests command side * fix remaining quota usage query * implement InmemLogStorage * cleanup and linting * improve test * fix: add quota unit tests command side * fix: add quota unit tests command side * fix: add quota unit tests command side * fix: add quota unit tests command side * action notifications and fixes for notifications query * revert console prefix * fix: add quota unit tests command side * fix: add quota integration tests * improve accountable requests * improve accountable requests * fix: add quota integration tests * fix: add quota integration tests * fix: add quota integration tests * comment * remove ability to store logs in db and other changes requested from review * changes requested from review * changes requested from review * Update internal/api/http/middleware/access_interceptor.go Co-authored-by: Silvan <silvan.reusser@gmail.com> * tests: fix quotas integration tests * improve incrementUsageStatement * linting * fix: delete e2e tests as intergation tests cover functionality * Update internal/api/http/middleware/access_interceptor.go Co-authored-by: Silvan <silvan.reusser@gmail.com> * backup * fix conflict * create rc * create prerelease * remove issue release labeling * fix tracing --------- Co-authored-by: Livio Spring <livio.a@gmail.com> Co-authored-by: Stefan Benz <stefan@caos.ch> Co-authored-by: adlerhurst <silvan.reusser@gmail.com>
128 lines
2.5 KiB
Go
128 lines
2.5 KiB
Go
package query
|
|
|
|
import (
|
|
"database/sql"
|
|
"database/sql/driver"
|
|
"errors"
|
|
"fmt"
|
|
"regexp"
|
|
"testing"
|
|
"time"
|
|
|
|
"github.com/jackc/pgtype"
|
|
|
|
errs "github.com/zitadel/zitadel/internal/errors"
|
|
)
|
|
|
|
var (
|
|
expectedQuotaQuery = regexp.QuoteMeta(`SELECT projections.quotas.id,` +
|
|
` projections.quotas.from_anchor,` +
|
|
` projections.quotas.interval,` +
|
|
` projections.quotas.amount,` +
|
|
` projections.quotas.limit_usage,` +
|
|
` now()` +
|
|
` FROM projections.quotas`)
|
|
|
|
quotaCols = []string{
|
|
"id",
|
|
"from_anchor",
|
|
"interval",
|
|
"amount",
|
|
"limit_usage",
|
|
"now",
|
|
}
|
|
)
|
|
|
|
func dayNow() time.Time {
|
|
return time.Now().Truncate(24 * time.Hour)
|
|
}
|
|
|
|
func interval(t *testing.T, src time.Duration) pgtype.Interval {
|
|
interval := pgtype.Interval{}
|
|
err := interval.Set(src)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
return interval
|
|
}
|
|
|
|
func Test_QuotaPrepare(t *testing.T) {
|
|
type want struct {
|
|
sqlExpectations sqlExpectation
|
|
err checkErr
|
|
}
|
|
tests := []struct {
|
|
name string
|
|
prepare interface{}
|
|
want want
|
|
object interface{}
|
|
}{
|
|
{
|
|
name: "prepareQuotaQuery no result",
|
|
prepare: prepareQuotaQuery,
|
|
want: want{
|
|
sqlExpectations: mockQueriesScanErr(
|
|
expectedQuotaQuery,
|
|
nil,
|
|
nil,
|
|
),
|
|
err: func(err error) (error, bool) {
|
|
if !errs.IsNotFound(err) {
|
|
return fmt.Errorf("err should be zitadel.NotFoundError got: %w", err), false
|
|
}
|
|
return nil, true
|
|
},
|
|
},
|
|
object: (*Quota)(nil),
|
|
},
|
|
{
|
|
name: "prepareQuotaQuery",
|
|
prepare: prepareQuotaQuery,
|
|
want: want{
|
|
sqlExpectations: mockQuery(
|
|
expectedQuotaQuery,
|
|
quotaCols,
|
|
[]driver.Value{
|
|
"quota-id",
|
|
dayNow(),
|
|
interval(t, time.Hour*24),
|
|
uint64(1000),
|
|
true,
|
|
testNow,
|
|
},
|
|
),
|
|
},
|
|
object: &Quota{
|
|
ID: "quota-id",
|
|
From: dayNow(),
|
|
ResetInterval: time.Hour * 24,
|
|
CurrentPeriodStart: dayNow(),
|
|
Amount: 1000,
|
|
Limit: true,
|
|
},
|
|
},
|
|
{
|
|
name: "prepareQuotaQuery sql err",
|
|
prepare: prepareQuotaQuery,
|
|
want: want{
|
|
sqlExpectations: mockQueryErr(
|
|
expectedQuotaQuery,
|
|
sql.ErrConnDone,
|
|
),
|
|
err: func(err error) (error, bool) {
|
|
if !errors.Is(err, sql.ErrConnDone) {
|
|
return fmt.Errorf("err should be sql.ErrConnDone got: %w", err), false
|
|
}
|
|
return nil, true
|
|
},
|
|
},
|
|
object: (*Quota)(nil),
|
|
},
|
|
}
|
|
for _, tt := range tests {
|
|
t.Run(tt.name, func(t *testing.T) {
|
|
assertPrepare(t, tt.prepare, tt.object, tt.want.sqlExpectations, tt.want.err, defaultPrepareArgs...)
|
|
})
|
|
}
|
|
}
|