mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 19:14:23 +00:00
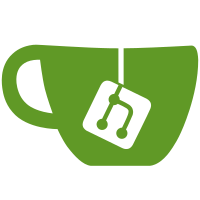
* chore: move to new org * logging * fix: org rename caos -> zitadel Co-authored-by: adlerhurst <silvan.reusser@gmail.com>
69 lines
1.6 KiB
Go
69 lines
1.6 KiB
Go
package model
|
|
|
|
import (
|
|
"github.com/zitadel/zitadel/internal/domain"
|
|
caos_errors "github.com/zitadel/zitadel/internal/errors"
|
|
|
|
"time"
|
|
)
|
|
|
|
type ExternalIDPView struct {
|
|
UserID string
|
|
IDPConfigID string
|
|
ExternalUserID string
|
|
IDPName string
|
|
UserDisplayName string
|
|
CreationDate time.Time
|
|
ChangeDate time.Time
|
|
ResourceOwner string
|
|
Sequence uint64
|
|
}
|
|
|
|
type ExternalIDPSearchRequest struct {
|
|
Offset uint64
|
|
Limit uint64
|
|
SortingColumn ExternalIDPSearchKey
|
|
Asc bool
|
|
Queries []*ExternalIDPSearchQuery
|
|
}
|
|
|
|
type ExternalIDPSearchKey int32
|
|
|
|
const (
|
|
ExternalIDPSearchKeyUnspecified ExternalIDPSearchKey = iota
|
|
ExternalIDPSearchKeyExternalUserID
|
|
ExternalIDPSearchKeyUserID
|
|
ExternalIDPSearchKeyIdpConfigID
|
|
ExternalIDPSearchKeyResourceOwner
|
|
ExternalIDPSearchKeyInstanceID
|
|
)
|
|
|
|
type ExternalIDPSearchQuery struct {
|
|
Key ExternalIDPSearchKey
|
|
Method domain.SearchMethod
|
|
Value interface{}
|
|
}
|
|
|
|
type ExternalIDPSearchResponse struct {
|
|
Offset uint64
|
|
Limit uint64
|
|
TotalResult uint64
|
|
Result []*ExternalIDPView
|
|
Sequence uint64
|
|
Timestamp time.Time
|
|
}
|
|
|
|
func (r *ExternalIDPSearchRequest) EnsureLimit(limit uint64) error {
|
|
if r.Limit > limit {
|
|
return caos_errors.ThrowInvalidArgument(nil, "SEARCH-3n8fM", "Errors.Limit.ExceedsDefault")
|
|
}
|
|
if r.Limit == 0 {
|
|
r.Limit = limit
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (r *ExternalIDPSearchRequest) AppendUserQuery(userID string) {
|
|
r.Queries = append(r.Queries, &ExternalIDPSearchQuery{Key: ExternalIDPSearchKeyUserID, Method: domain.SearchMethodEquals, Value: userID})
|
|
}
|