mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
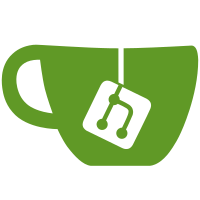
* feat: comprehensive sentry instrumentation * test: pass * fix: only fetch zitadel dsn in zitadel-operator * chore: use dns for sentry environment as soon as parsed * fix: trust ca certs * ci: update orbos * docs: add usage data explanation * fix: dont send validation errors * docs: improve ingestion data explanation * style: rename flag --disable-ingestion to --disable-analytics * fix: pass --disable-analytics flag to self deployments * fix: destroy command for sentry * fix: update orbos * fix: only switch environment if analytics is enabled * fix: ensure SENTRY_DSN is always set * test: test empty sentry dsn * ci: invalidate build caches * chore: use zitadel-dev if no version is passed * chore: combine dev releases in sentry * refactor: only check for semrel if sentry is enabled
88 lines
2.1 KiB
Go
88 lines
2.1 KiB
Go
package cmds
|
|
|
|
import (
|
|
"github.com/caos/orbos/pkg/kubernetes"
|
|
"github.com/caos/zitadel/operator/crtlcrd"
|
|
"github.com/caos/zitadel/operator/crtlgitops"
|
|
"github.com/spf13/cobra"
|
|
)
|
|
|
|
func StartOperator(getRv GetRootValues) *cobra.Command {
|
|
var (
|
|
metricsAddr string
|
|
cmd = &cobra.Command{
|
|
Use: "operator",
|
|
Short: "Launch a ZITADEL operator",
|
|
Long: "Ensures a desired state of ZITADEL",
|
|
}
|
|
)
|
|
flags := cmd.Flags()
|
|
flags.StringVar(&metricsAddr, "metrics-addr", "", "The address the metric endpoint binds to.")
|
|
|
|
cmd.RunE = func(cmd *cobra.Command, args []string) (err error) {
|
|
rv := getRv("operator", nil, "zitadel-operator", "zitadel")
|
|
defer func() {
|
|
err = rv.ErrFunc(err)
|
|
}()
|
|
|
|
monitor := rv.Monitor
|
|
orbConfig := rv.OrbConfig
|
|
version := rv.Version
|
|
|
|
if rv.Gitops {
|
|
k8sClient, err := kubernetes.NewK8sClientWithPath(monitor, rv.Kubeconfig)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
return crtlgitops.Operator(monitor, orbConfig.Path, k8sClient, &version, rv.Gitops)
|
|
} else {
|
|
if err := crtlcrd.Start(monitor, version, metricsAddr, crtlcrd.Zitadel); err != nil {
|
|
return err
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
return cmd
|
|
}
|
|
|
|
func StartDatabase(getRv GetRootValues) *cobra.Command {
|
|
var (
|
|
metricsAddr string
|
|
cmd = &cobra.Command{
|
|
Use: "database",
|
|
Short: "Launch a database operator",
|
|
Long: "Ensures a desired state of the database",
|
|
}
|
|
)
|
|
flags := cmd.Flags()
|
|
flags.StringVar(&metricsAddr, "metrics-addr", "", "The address the metric endpoint binds to.")
|
|
|
|
cmd.RunE = func(cmd *cobra.Command, args []string) (err error) {
|
|
rv := getRv("database", nil, "database-operator")
|
|
defer func() {
|
|
err = rv.ErrFunc(err)
|
|
}()
|
|
|
|
monitor := rv.Monitor
|
|
orbConfig := rv.OrbConfig
|
|
version := rv.Version
|
|
|
|
if rv.Gitops {
|
|
k8sClient, err := kubernetes.NewK8sClientWithPath(monitor, rv.Kubeconfig)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
return crtlgitops.Database(monitor, orbConfig.Path, k8sClient, &version, rv.Gitops)
|
|
} else {
|
|
if err := crtlcrd.Start(monitor, version, metricsAddr, crtlcrd.Database); err != nil {
|
|
return err
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
return cmd
|
|
}
|