mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
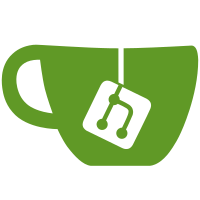
This PR starts the OIDC implementation for the API V2 including the Implicit and Code Flow. Co-authored-by: Livio Spring <livio.a@gmail.com> Co-authored-by: Tim Möhlmann <tim+github@zitadel.com> Co-authored-by: Stefan Benz <46600784+stebenz@users.noreply.github.com>
60 lines
1.3 KiB
Go
60 lines
1.3 KiB
Go
package oidc
|
|
|
|
import (
|
|
"github.com/zitadel/oidc/v2/pkg/op"
|
|
"google.golang.org/grpc"
|
|
|
|
"github.com/zitadel/zitadel/internal/api/authz"
|
|
"github.com/zitadel/zitadel/internal/api/grpc/server"
|
|
"github.com/zitadel/zitadel/internal/command"
|
|
"github.com/zitadel/zitadel/internal/query"
|
|
oidc_pb "github.com/zitadel/zitadel/pkg/grpc/oidc/v2alpha"
|
|
)
|
|
|
|
var _ oidc_pb.OIDCServiceServer = (*Server)(nil)
|
|
|
|
type Server struct {
|
|
oidc_pb.UnimplementedOIDCServiceServer
|
|
command *command.Commands
|
|
query *query.Queries
|
|
|
|
op op.OpenIDProvider
|
|
externalSecure bool
|
|
}
|
|
|
|
type Config struct{}
|
|
|
|
func CreateServer(
|
|
command *command.Commands,
|
|
query *query.Queries,
|
|
op op.OpenIDProvider,
|
|
externalSecure bool,
|
|
) *Server {
|
|
return &Server{
|
|
command: command,
|
|
query: query,
|
|
op: op,
|
|
externalSecure: externalSecure,
|
|
}
|
|
}
|
|
|
|
func (s *Server) RegisterServer(grpcServer *grpc.Server) {
|
|
oidc_pb.RegisterOIDCServiceServer(grpcServer, s)
|
|
}
|
|
|
|
func (s *Server) AppName() string {
|
|
return oidc_pb.OIDCService_ServiceDesc.ServiceName
|
|
}
|
|
|
|
func (s *Server) MethodPrefix() string {
|
|
return oidc_pb.OIDCService_ServiceDesc.ServiceName
|
|
}
|
|
|
|
func (s *Server) AuthMethods() authz.MethodMapping {
|
|
return oidc_pb.OIDCService_AuthMethods
|
|
}
|
|
|
|
func (s *Server) RegisterGateway() server.RegisterGatewayFunc {
|
|
return oidc_pb.RegisterOIDCServiceHandler
|
|
}
|