mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-15 12:27:59 +00:00
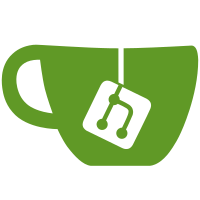
# Which Problems Are Solved Currently ZITADEL supports RP-initiated logout for clients. Back-channel logout ensures that user sessions are terminated across all connected applications, even if the user closes their browser or loses connectivity providing a more secure alternative for certain use cases. # How the Problems Are Solved If the feature is activated and the client used for the authentication has a back_channel_logout_uri configured, a `session_logout.back_channel` will be registered. Once a user terminates their session, a (notification) handler will send a SET (form POST) to the registered uri containing a logout_token (with the user's ID and session ID). - A new feature "back_channel_logout" is added on system and instance level - A `back_channel_logout_uri` can be managed on OIDC applications - Added a `session_logout` aggregate to register and inform about sent `back_channel` notifications - Added a `SecurityEventToken` channel and `Form`message type in the notification handlers - Added `TriggeredAtOrigin` fields to `HumanSignedOut` and `TerminateSession` events for notification handling - Exported various functions and types in the `oidc` package to be able to reuse for token signing in the back_channel notifier. - To prevent that current existing session termination events will be handled, a setup step is added to set the `current_states` for the `projections.notifications_back_channel_logout` to the current position - [x] requires https://github.com/zitadel/oidc/pull/671 # Additional Changes - Updated all OTEL dependencies to v1.29.0, since OIDC already updated some of them to that version. - Single Session Termination feature is correctly checked (fixed feature mapping) # Additional Context - closes https://github.com/zitadel/zitadel/issues/8467 - TODO: - Documentation - UI to be done: https://github.com/zitadel/zitadel/issues/8469 --------- Co-authored-by: Hidde Wieringa <hidde@hiddewieringa.nl>
172 lines
4.5 KiB
Go
172 lines
4.5 KiB
Go
package view
|
|
|
|
import (
|
|
"context"
|
|
"database/sql"
|
|
_ "embed"
|
|
"errors"
|
|
|
|
"github.com/zitadel/zitadel/internal/database"
|
|
"github.com/zitadel/zitadel/internal/user/repository/view/model"
|
|
"github.com/zitadel/zitadel/internal/zerrors"
|
|
)
|
|
|
|
//go:embed user_session_by_id.sql
|
|
var userSessionByIDQuery string
|
|
|
|
//go:embed user_sessions_by_user_agent.sql
|
|
var userSessionsByUserAgentQuery string
|
|
|
|
//go:embed user_agent_by_user_session_id.sql
|
|
var userAgentByUserSessionIDQuery string
|
|
|
|
//go:embed active_user_sessions_by_session_id.sql
|
|
var activeUserSessionsBySessionIDQuery string
|
|
|
|
func UserSessionByIDs(ctx context.Context, db *database.DB, agentID, userID, instanceID string) (userSession *model.UserSessionView, err error) {
|
|
err = db.QueryRowContext(
|
|
ctx,
|
|
func(row *sql.Row) error {
|
|
userSession, err = scanUserSession(row)
|
|
return err
|
|
},
|
|
userSessionByIDQuery,
|
|
agentID,
|
|
userID,
|
|
instanceID,
|
|
)
|
|
return userSession, err
|
|
}
|
|
|
|
func UserSessionsByAgentID(ctx context.Context, db *database.DB, agentID, instanceID string) (userSessions []*model.UserSessionView, err error) {
|
|
err = db.QueryContext(
|
|
ctx,
|
|
func(rows *sql.Rows) error {
|
|
userSessions, err = scanUserSessions(rows)
|
|
return err
|
|
},
|
|
userSessionsByUserAgentQuery,
|
|
agentID,
|
|
instanceID,
|
|
)
|
|
return userSessions, err
|
|
}
|
|
|
|
func UserAgentIDBySessionID(ctx context.Context, db *database.DB, sessionID, instanceID string) (userAgentID string, err error) {
|
|
err = db.QueryRowContext(
|
|
ctx,
|
|
func(row *sql.Row) error {
|
|
return row.Scan(&userAgentID)
|
|
},
|
|
userAgentByUserSessionIDQuery,
|
|
sessionID,
|
|
instanceID,
|
|
)
|
|
return userAgentID, err
|
|
}
|
|
|
|
// ActiveUserSessionsBySessionID returns all sessions (sessionID:userID map) with an active session on the same user agent (its id is also returned) based on a sessionID
|
|
func ActiveUserSessionsBySessionID(ctx context.Context, db *database.DB, sessionID, instanceID string) (userAgentID string, sessions map[string]string, err error) {
|
|
err = db.QueryContext(
|
|
ctx,
|
|
func(rows *sql.Rows) error {
|
|
userAgentID, sessions, err = scanActiveUserAgentUserIDs(rows)
|
|
return err
|
|
},
|
|
activeUserSessionsBySessionIDQuery,
|
|
sessionID,
|
|
instanceID,
|
|
)
|
|
return userAgentID, sessions, err
|
|
}
|
|
|
|
func scanActiveUserAgentUserIDs(rows *sql.Rows) (userAgentID string, sessions map[string]string, err error) {
|
|
sessions = make(map[string]string)
|
|
for rows.Next() {
|
|
var userID, sessionID string
|
|
err := rows.Scan(
|
|
&userAgentID,
|
|
&userID,
|
|
&sessionID,
|
|
)
|
|
if err != nil {
|
|
return "", nil, err
|
|
}
|
|
sessions[sessionID] = userID
|
|
}
|
|
if err := rows.Close(); err != nil {
|
|
return "", nil, zerrors.ThrowInternal(err, "VIEW-Sbrws", "Errors.Query.CloseRows")
|
|
}
|
|
return userAgentID, sessions, nil
|
|
}
|
|
|
|
func scanUserSession(row *sql.Row) (*model.UserSessionView, error) {
|
|
session := new(model.UserSessionView)
|
|
err := row.Scan(
|
|
&session.CreationDate,
|
|
&session.ChangeDate,
|
|
&session.ResourceOwner,
|
|
&session.State,
|
|
&session.UserAgentID,
|
|
&session.UserID,
|
|
&session.UserName,
|
|
&session.LoginName,
|
|
&session.DisplayName,
|
|
&session.AvatarKey,
|
|
&session.SelectedIDPConfigID,
|
|
&session.PasswordVerification,
|
|
&session.PasswordlessVerification,
|
|
&session.ExternalLoginVerification,
|
|
&session.SecondFactorVerification,
|
|
&session.SecondFactorVerificationType,
|
|
&session.MultiFactorVerification,
|
|
&session.MultiFactorVerificationType,
|
|
&session.Sequence,
|
|
&session.InstanceID,
|
|
&session.ID,
|
|
)
|
|
if errors.Is(err, sql.ErrNoRows) {
|
|
return nil, zerrors.ThrowNotFound(nil, "VIEW-NGBs1", "Errors.UserSession.NotFound")
|
|
}
|
|
return session, err
|
|
}
|
|
|
|
func scanUserSessions(rows *sql.Rows) ([]*model.UserSessionView, error) {
|
|
sessions := make([]*model.UserSessionView, 0)
|
|
for rows.Next() {
|
|
session := new(model.UserSessionView)
|
|
err := rows.Scan(
|
|
&session.CreationDate,
|
|
&session.ChangeDate,
|
|
&session.ResourceOwner,
|
|
&session.State,
|
|
&session.UserAgentID,
|
|
&session.UserID,
|
|
&session.UserName,
|
|
&session.LoginName,
|
|
&session.DisplayName,
|
|
&session.AvatarKey,
|
|
&session.SelectedIDPConfigID,
|
|
&session.PasswordVerification,
|
|
&session.PasswordlessVerification,
|
|
&session.ExternalLoginVerification,
|
|
&session.SecondFactorVerification,
|
|
&session.SecondFactorVerificationType,
|
|
&session.MultiFactorVerification,
|
|
&session.MultiFactorVerificationType,
|
|
&session.Sequence,
|
|
&session.InstanceID,
|
|
&session.ID,
|
|
)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
sessions = append(sessions, session)
|
|
}
|
|
|
|
if err := rows.Close(); err != nil {
|
|
return nil, zerrors.ThrowInternal(err, "VIEW-FSF3g", "Errors.Query.CloseRows")
|
|
}
|
|
return sessions, nil
|
|
}
|