mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 19:14:23 +00:00
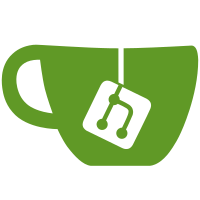
* feat(import): add functionality to import data into an instance * feat(import): move import to admin api and additional checks for nil pointer * fix(export): export implementation with filtered members and grants * fix: export and import implementation * fix: add possibility to export hashed passwords with the user * fix(import): import with structure of v1 and v2 * docs: add v1 proto * fix(import): check im imported user is already existing * fix(import): add otp import function * fix(import): add external idps, domains, custom text and messages * fix(import): correct usage of default values from login policy * fix(export): fix renaming of add project function * fix(import): move checks for unit tests * expect filter * fix(import): move checks for unit tests * fix(import): move checks for unit tests * fix(import): produce prerelease from branch * fix(import): correctly use provided user id for machine user imports * fix(import): corrected otp import and added guide for export and import * fix: import verified and primary domains * fix(import): add reading from gcs, s3 and localfile with tracing * fix(import): gcs and s3, file size correction and error logging * Delete docker-compose.yml * fix(import): progress logging and count of resources * fix(import): progress logging and count of resources * log subscription * fix(import): incorporate review * fix(import): incorporate review * docs: add suggestion for import Co-authored-by: Fabi <38692350+hifabienne@users.noreply.github.com> * fix(import): add verification otp event and handling of deleted but existing users Co-authored-by: Livio Amstutz <livio.a@gmail.com> Co-authored-by: Fabienne <fabienne.gerschwiler@gmail.com> Co-authored-by: Silvan <silvan.reusser@gmail.com> Co-authored-by: Fabi <38692350+hifabienne@users.noreply.github.com>
157 lines
5.5 KiB
Go
157 lines
5.5 KiB
Go
package management
|
|
|
|
import (
|
|
"context"
|
|
|
|
"github.com/zitadel/zitadel/internal/api/authz"
|
|
"github.com/zitadel/zitadel/internal/api/grpc/object"
|
|
policy_grpc "github.com/zitadel/zitadel/internal/api/grpc/policy"
|
|
mgmt_pb "github.com/zitadel/zitadel/pkg/grpc/management"
|
|
)
|
|
|
|
func (s *Server) GetLabelPolicy(ctx context.Context, req *mgmt_pb.GetLabelPolicyRequest) (*mgmt_pb.GetLabelPolicyResponse, error) {
|
|
policy, err := s.query.ActiveLabelPolicyByOrg(ctx, authz.GetCtxData(ctx).OrgID)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return &mgmt_pb.GetLabelPolicyResponse{Policy: policy_grpc.ModelLabelPolicyToPb(policy, s.assetAPIPrefix(ctx)), IsDefault: policy.IsDefault}, nil
|
|
}
|
|
|
|
func (s *Server) GetPreviewLabelPolicy(ctx context.Context, req *mgmt_pb.GetPreviewLabelPolicyRequest) (*mgmt_pb.GetPreviewLabelPolicyResponse, error) {
|
|
policy, err := s.query.PreviewLabelPolicyByOrg(ctx, authz.GetCtxData(ctx).OrgID)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return &mgmt_pb.GetPreviewLabelPolicyResponse{Policy: policy_grpc.ModelLabelPolicyToPb(policy, s.assetAPIPrefix(ctx)), IsDefault: policy.IsDefault}, nil
|
|
}
|
|
|
|
func (s *Server) GetDefaultLabelPolicy(ctx context.Context, req *mgmt_pb.GetDefaultLabelPolicyRequest) (*mgmt_pb.GetDefaultLabelPolicyResponse, error) {
|
|
policy, err := s.query.DefaultActiveLabelPolicy(ctx)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return &mgmt_pb.GetDefaultLabelPolicyResponse{Policy: policy_grpc.ModelLabelPolicyToPb(policy, s.assetAPIPrefix(ctx))}, nil
|
|
}
|
|
|
|
func (s *Server) AddCustomLabelPolicy(ctx context.Context, req *mgmt_pb.AddCustomLabelPolicyRequest) (*mgmt_pb.AddCustomLabelPolicyResponse, error) {
|
|
policy, err := s.command.AddLabelPolicy(ctx, authz.GetCtxData(ctx).OrgID, AddLabelPolicyToDomain(req))
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return &mgmt_pb.AddCustomLabelPolicyResponse{
|
|
Details: object.AddToDetailsPb(
|
|
policy.Sequence,
|
|
policy.ChangeDate,
|
|
policy.ResourceOwner,
|
|
),
|
|
}, nil
|
|
}
|
|
|
|
func (s *Server) UpdateCustomLabelPolicy(ctx context.Context, req *mgmt_pb.UpdateCustomLabelPolicyRequest) (*mgmt_pb.UpdateCustomLabelPolicyResponse, error) {
|
|
policy, err := s.command.ChangeLabelPolicy(ctx, authz.GetCtxData(ctx).OrgID, updateLabelPolicyToDomain(req))
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return &mgmt_pb.UpdateCustomLabelPolicyResponse{
|
|
Details: object.ChangeToDetailsPb(
|
|
policy.Sequence,
|
|
policy.ChangeDate,
|
|
policy.ResourceOwner,
|
|
),
|
|
}, nil
|
|
}
|
|
|
|
func (s *Server) ActivateCustomLabelPolicy(ctx context.Context, req *mgmt_pb.ActivateCustomLabelPolicyRequest) (*mgmt_pb.ActivateCustomLabelPolicyResponse, error) {
|
|
policy, err := s.command.ActivateLabelPolicy(ctx, authz.GetCtxData(ctx).OrgID)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return &mgmt_pb.ActivateCustomLabelPolicyResponse{
|
|
Details: object.ChangeToDetailsPb(
|
|
policy.Sequence,
|
|
policy.EventDate,
|
|
policy.ResourceOwner,
|
|
),
|
|
}, nil
|
|
}
|
|
|
|
func (s *Server) ResetLabelPolicyToDefault(ctx context.Context, req *mgmt_pb.ResetLabelPolicyToDefaultRequest) (*mgmt_pb.ResetLabelPolicyToDefaultResponse, error) {
|
|
objectDetails, err := s.command.RemoveLabelPolicy(ctx, authz.GetCtxData(ctx).OrgID)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return &mgmt_pb.ResetLabelPolicyToDefaultResponse{
|
|
Details: object.DomainToChangeDetailsPb(objectDetails),
|
|
}, nil
|
|
}
|
|
|
|
func (s *Server) RemoveCustomLabelPolicyLogo(ctx context.Context, req *mgmt_pb.RemoveCustomLabelPolicyLogoRequest) (*mgmt_pb.RemoveCustomLabelPolicyLogoResponse, error) {
|
|
policy, err := s.command.RemoveLogoLabelPolicy(ctx, authz.GetCtxData(ctx).OrgID)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return &mgmt_pb.RemoveCustomLabelPolicyLogoResponse{
|
|
Details: object.ChangeToDetailsPb(
|
|
policy.Sequence,
|
|
policy.EventDate,
|
|
policy.ResourceOwner,
|
|
),
|
|
}, nil
|
|
}
|
|
|
|
func (s *Server) RemoveCustomLabelPolicyLogoDark(ctx context.Context, req *mgmt_pb.RemoveCustomLabelPolicyLogoDarkRequest) (*mgmt_pb.RemoveCustomLabelPolicyLogoDarkResponse, error) {
|
|
policy, err := s.command.RemoveLogoDarkLabelPolicy(ctx, authz.GetCtxData(ctx).OrgID)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return &mgmt_pb.RemoveCustomLabelPolicyLogoDarkResponse{
|
|
Details: object.ChangeToDetailsPb(
|
|
policy.Sequence,
|
|
policy.EventDate,
|
|
policy.ResourceOwner,
|
|
),
|
|
}, nil
|
|
}
|
|
|
|
func (s *Server) RemoveCustomLabelPolicyIcon(ctx context.Context, req *mgmt_pb.RemoveCustomLabelPolicyIconRequest) (*mgmt_pb.RemoveCustomLabelPolicyIconResponse, error) {
|
|
policy, err := s.command.RemoveIconLabelPolicy(ctx, authz.GetCtxData(ctx).OrgID)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return &mgmt_pb.RemoveCustomLabelPolicyIconResponse{
|
|
Details: object.ChangeToDetailsPb(
|
|
policy.Sequence,
|
|
policy.EventDate,
|
|
policy.ResourceOwner,
|
|
),
|
|
}, nil
|
|
}
|
|
|
|
func (s *Server) RemoveCustomLabelPolicyIconDark(ctx context.Context, req *mgmt_pb.RemoveCustomLabelPolicyIconDarkRequest) (*mgmt_pb.RemoveCustomLabelPolicyIconDarkResponse, error) {
|
|
policy, err := s.command.RemoveIconDarkLabelPolicy(ctx, authz.GetCtxData(ctx).OrgID)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return &mgmt_pb.RemoveCustomLabelPolicyIconDarkResponse{
|
|
Details: object.ChangeToDetailsPb(
|
|
policy.Sequence,
|
|
policy.EventDate,
|
|
policy.ResourceOwner,
|
|
),
|
|
}, nil
|
|
}
|
|
|
|
func (s *Server) RemoveCustomLabelPolicyFont(ctx context.Context, req *mgmt_pb.RemoveCustomLabelPolicyFontRequest) (*mgmt_pb.RemoveCustomLabelPolicyFontResponse, error) {
|
|
policy, err := s.command.RemoveFontLabelPolicy(ctx, authz.GetCtxData(ctx).OrgID)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return &mgmt_pb.RemoveCustomLabelPolicyFontResponse{
|
|
Details: object.ChangeToDetailsPb(
|
|
policy.Sequence,
|
|
policy.EventDate,
|
|
policy.ResourceOwner,
|
|
),
|
|
}, nil
|
|
}
|