mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-15 12:27:59 +00:00
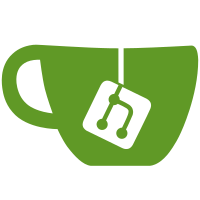
Some checks are pending
ZITADEL CI/CD / core (push) Waiting to run
ZITADEL CI/CD / console (push) Waiting to run
ZITADEL CI/CD / version (push) Waiting to run
ZITADEL CI/CD / compile (push) Blocked by required conditions
ZITADEL CI/CD / core-unit-test (push) Blocked by required conditions
ZITADEL CI/CD / core-integration-test (push) Blocked by required conditions
ZITADEL CI/CD / lint (push) Blocked by required conditions
ZITADEL CI/CD / container (push) Blocked by required conditions
ZITADEL CI/CD / e2e (push) Blocked by required conditions
ZITADEL CI/CD / release (push) Blocked by required conditions
Code Scanning / CodeQL-Build (go) (push) Waiting to run
Code Scanning / CodeQL-Build (javascript) (push) Waiting to run
# Which Problems Are Solved Milestones used existing events from a number of aggregates. OIDC session is one of them. We noticed in load-tests that the reduction of the oidc_session.added event into the milestone projection is a costly business with payload based conditionals. A milestone is reached once, but even then we remain subscribed to the OIDC events. This requires the projections.current_states to be updated continuously. # How the Problems Are Solved The milestone creation is refactored to use dedicated events instead. The command side decides when a milestone is reached and creates the reached event once for each milestone when required. # Additional Changes In order to prevent reached milestones being created twice, a migration script is provided. When the old `projections.milestones` table exist, the state is read from there and `v2` milestone aggregate events are created, with the original reached and pushed dates. # Additional Context - Closes https://github.com/zitadel/zitadel/issues/8800
137 lines
2.7 KiB
Go
137 lines
2.7 KiB
Go
package authz
|
|
|
|
import (
|
|
"context"
|
|
"time"
|
|
|
|
"golang.org/x/text/language"
|
|
|
|
"github.com/zitadel/zitadel/internal/feature"
|
|
)
|
|
|
|
var (
|
|
emptyInstance = &instance{}
|
|
)
|
|
|
|
type Instance interface {
|
|
InstanceID() string
|
|
ProjectID() string
|
|
ConsoleClientID() string
|
|
ConsoleApplicationID() string
|
|
DefaultLanguage() language.Tag
|
|
DefaultOrganisationID() string
|
|
SecurityPolicyAllowedOrigins() []string
|
|
EnableImpersonation() bool
|
|
Block() *bool
|
|
AuditLogRetention() *time.Duration
|
|
Features() feature.Features
|
|
}
|
|
|
|
type InstanceVerifier interface {
|
|
InstanceByHost(ctx context.Context, host, publicDomain string) (Instance, error)
|
|
InstanceByID(ctx context.Context, id string) (Instance, error)
|
|
}
|
|
|
|
type instance struct {
|
|
id string
|
|
domain string
|
|
projectID string
|
|
appID string
|
|
clientID string
|
|
orgID string
|
|
features feature.Features
|
|
}
|
|
|
|
func (i *instance) Block() *bool {
|
|
return nil
|
|
}
|
|
|
|
func (i *instance) AuditLogRetention() *time.Duration {
|
|
return nil
|
|
}
|
|
|
|
func (i *instance) InstanceID() string {
|
|
return i.id
|
|
}
|
|
|
|
func (i *instance) ProjectID() string {
|
|
return i.projectID
|
|
}
|
|
|
|
func (i *instance) ConsoleClientID() string {
|
|
return i.clientID
|
|
}
|
|
|
|
func (i *instance) ConsoleApplicationID() string {
|
|
return i.appID
|
|
}
|
|
|
|
func (i *instance) DefaultLanguage() language.Tag {
|
|
return language.Und
|
|
}
|
|
|
|
func (i *instance) DefaultOrganisationID() string {
|
|
return i.orgID
|
|
}
|
|
|
|
func (i *instance) SecurityPolicyAllowedOrigins() []string {
|
|
return nil
|
|
}
|
|
|
|
func (i *instance) EnableImpersonation() bool {
|
|
return false
|
|
}
|
|
|
|
func (i *instance) Features() feature.Features {
|
|
return i.features
|
|
}
|
|
|
|
func GetInstance(ctx context.Context) Instance {
|
|
instance, ok := ctx.Value(instanceKey).(Instance)
|
|
if !ok {
|
|
return emptyInstance
|
|
}
|
|
return instance
|
|
}
|
|
|
|
func GetFeatures(ctx context.Context) feature.Features {
|
|
return GetInstance(ctx).Features()
|
|
}
|
|
|
|
func WithInstance(ctx context.Context, instance Instance) context.Context {
|
|
return context.WithValue(ctx, instanceKey, instance)
|
|
}
|
|
|
|
func WithInstanceID(ctx context.Context, id string) context.Context {
|
|
return context.WithValue(ctx, instanceKey, &instance{id: id})
|
|
}
|
|
|
|
func WithConsole(ctx context.Context, projectID, appID string) context.Context {
|
|
i, ok := ctx.Value(instanceKey).(*instance)
|
|
if !ok {
|
|
i = new(instance)
|
|
}
|
|
|
|
i.projectID = projectID
|
|
i.appID = appID
|
|
return context.WithValue(ctx, instanceKey, i)
|
|
}
|
|
|
|
func WithConsoleClientID(ctx context.Context, clientID string) context.Context {
|
|
i, ok := ctx.Value(instanceKey).(*instance)
|
|
if !ok {
|
|
i = new(instance)
|
|
}
|
|
i.clientID = clientID
|
|
return context.WithValue(ctx, instanceKey, i)
|
|
}
|
|
|
|
func WithFeatures(ctx context.Context, f feature.Features) context.Context {
|
|
i, ok := ctx.Value(instanceKey).(*instance)
|
|
if !ok {
|
|
i = new(instance)
|
|
}
|
|
i.features = f
|
|
return context.WithValue(ctx, instanceKey, i)
|
|
}
|