mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-15 20:38:00 +00:00
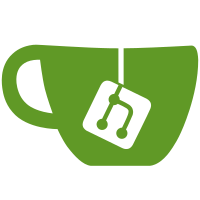
# Which Problems Are Solved Add a cache implementation using Redis single mode. This does not add support for Redis Cluster or sentinel. # How the Problems Are Solved Added the `internal/cache/redis` package. All operations occur atomically, including setting of secondary indexes, using LUA scripts where needed. The [`miniredis`](https://github.com/alicebob/miniredis) package is used to run unit tests. # Additional Changes - Move connector code to `internal/cache/connector/...` and remove duplicate code from `query` and `command` packages. - Fix a missed invalidation on the restrictions projection # Additional Context Closes #8130
28 lines
1.0 KiB
Lua
28 lines
1.0 KiB
Lua
-- KEYS: [1]: object_id; [>1]: index keys.
|
|
local object_id = KEYS[1]
|
|
local object = ARGV[2]
|
|
local usage_lifetime = tonumber(ARGV[3]) -- usage based lifetime in seconds
|
|
local max_age = tonumber(ARGV[4]) -- max age liftime in seconds
|
|
|
|
redis.call("HSET", object_id,"object", object)
|
|
if usage_lifetime > 0 then
|
|
redis.call("HSET", object_id, "usage_lifetime", usage_lifetime)
|
|
-- enable usage based TTL
|
|
redis.call("EXPIRE", object_id, usage_lifetime)
|
|
if max_age > 0 then
|
|
-- set max_age to hash map for expired remove on Get
|
|
local expiry = getTime() + max_age
|
|
redis.call("HSET", object_id, "expiry", expiry)
|
|
end
|
|
elseif max_age > 0 then
|
|
-- enable max_age based TTL
|
|
redis.call("EXPIRE", object_id, max_age)
|
|
end
|
|
|
|
local n = #KEYS
|
|
local setKey = keySetKey(object_id)
|
|
for i = 2, n do -- offset to the second element to skip object_id
|
|
redis.call("SADD", setKey, KEYS[i]) -- set of all keys used for housekeeping
|
|
redis.call("SET", KEYS[i], object_id) -- key to object_id mapping
|
|
end
|