mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
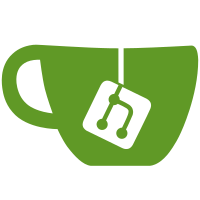
* beginning with postgres statements * try pgx * use pgx * database * init works for postgres * arrays working * init for cockroach * init * start tests * tests * TESTS * ch * ch * chore: use go 1.18 * read stmts * fix typo * tests * connection string * add missing error handler * cleanup * start all apis * go mod tidy * old update * switch back to minute * on conflict * replace string slice with `database.StringArray` in db models * fix tests and start * update go version in dockerfile * setup go * clean up * remove notification migration * update * docs: add deploy guide for postgres * fix: revert sonyflake * use `database.StringArray` for daos * use `database.StringArray` every where * new tables * index naming, metadata primary key, project grant role key type * docs(postgres): change to beta * chore: correct compose * fix(defaults): add empty postgres config * refactor: remove unused code * docs: add postgres to self hosted * fix broken link * so? * change title * add mdx to link * fix stmt * update goreleaser in test-code * docs: improve postgres example * update more projections * fix: add beta log for postgres * revert index name change * prerelease * fix: add sequence to v1 "reduce paniced" * log if nil * add logging * fix: log output * fix(import): check if org exists and user * refactor: imports * fix(user): ignore malformed events * refactor: method naming * fix: test * refactor: correct errors.Is call * ci: don't build dev binaries on main * fix(go releaser): update version to 1.11.0 * fix(user): projection should not break * fix(user): handle error properly * docs: correct config example * Update .releaserc.js * Update .releaserc.js Co-authored-by: Livio Amstutz <livio.a@gmail.com> Co-authored-by: Elio Bischof <eliobischof@gmail.com>
681 lines
20 KiB
Go
681 lines
20 KiB
Go
package projection
|
|
|
|
import (
|
|
"testing"
|
|
|
|
"github.com/zitadel/zitadel/internal/domain"
|
|
"github.com/zitadel/zitadel/internal/errors"
|
|
"github.com/zitadel/zitadel/internal/eventstore"
|
|
"github.com/zitadel/zitadel/internal/eventstore/handler"
|
|
"github.com/zitadel/zitadel/internal/eventstore/repository"
|
|
"github.com/zitadel/zitadel/internal/repository/instance"
|
|
"github.com/zitadel/zitadel/internal/repository/org"
|
|
)
|
|
|
|
func TestMessageTextProjection_reduces(t *testing.T) {
|
|
type args struct {
|
|
event func(t *testing.T) eventstore.Event
|
|
}
|
|
tests := []struct {
|
|
name string
|
|
args args
|
|
reduce func(event eventstore.Event) (*handler.Statement, error)
|
|
want wantReduce
|
|
}{
|
|
{
|
|
name: "org.reduceAdded.Title",
|
|
args: args{
|
|
event: getEvent(testEvent(
|
|
repository.EventType(org.CustomTextSetEventType),
|
|
org.AggregateType,
|
|
[]byte(`{
|
|
"key": "Title",
|
|
"language": "en",
|
|
"template": "InitCode",
|
|
"text": "Test"
|
|
}`),
|
|
), org.CustomTextSetEventMapper),
|
|
},
|
|
reduce: (&messageTextProjection{}).reduceAdded,
|
|
want: wantReduce{
|
|
aggregateType: eventstore.AggregateType("org"),
|
|
sequence: 15,
|
|
previousSequence: 10,
|
|
projection: MessageTextTable,
|
|
executer: &testExecuter{
|
|
executions: []execution{
|
|
{
|
|
expectedStmt: "INSERT INTO projections.message_texts (aggregate_id, instance_id, creation_date, change_date, sequence, state, type, language, title) VALUES ($1, $2, $3, $4, $5, $6, $7, $8, $9) ON CONFLICT (instance_id, aggregate_id, type, language) DO UPDATE SET (creation_date, change_date, sequence, state, title) = (EXCLUDED.creation_date, EXCLUDED.change_date, EXCLUDED.sequence, EXCLUDED.state, EXCLUDED.title)",
|
|
expectedArgs: []interface{}{
|
|
"agg-id",
|
|
"instance-id",
|
|
anyArg{},
|
|
anyArg{},
|
|
uint64(15),
|
|
domain.PolicyStateActive,
|
|
"InitCode",
|
|
"en",
|
|
"Test",
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
{
|
|
name: "org.reduceAdded.PreHeader",
|
|
args: args{
|
|
event: getEvent(testEvent(
|
|
repository.EventType(org.CustomTextSetEventType),
|
|
org.AggregateType,
|
|
[]byte(`{
|
|
"key": "PreHeader",
|
|
"language": "en",
|
|
"template": "InitCode",
|
|
"text": "Test"
|
|
}`),
|
|
), org.CustomTextSetEventMapper),
|
|
},
|
|
reduce: (&messageTextProjection{}).reduceAdded,
|
|
want: wantReduce{
|
|
aggregateType: eventstore.AggregateType("org"),
|
|
sequence: 15,
|
|
previousSequence: 10,
|
|
projection: MessageTextTable,
|
|
executer: &testExecuter{
|
|
executions: []execution{
|
|
{
|
|
expectedStmt: "INSERT INTO projections.message_texts (aggregate_id, instance_id, creation_date, change_date, sequence, state, type, language, pre_header) VALUES ($1, $2, $3, $4, $5, $6, $7, $8, $9) ON CONFLICT (instance_id, aggregate_id, type, language) DO UPDATE SET (creation_date, change_date, sequence, state, pre_header) = (EXCLUDED.creation_date, EXCLUDED.change_date, EXCLUDED.sequence, EXCLUDED.state, EXCLUDED.pre_header)",
|
|
expectedArgs: []interface{}{
|
|
"agg-id",
|
|
"instance-id",
|
|
anyArg{},
|
|
anyArg{},
|
|
uint64(15),
|
|
domain.PolicyStateActive,
|
|
"InitCode",
|
|
"en",
|
|
"Test",
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
{
|
|
name: "org.reduceAdded.Subject",
|
|
args: args{
|
|
event: getEvent(testEvent(
|
|
repository.EventType(org.CustomTextSetEventType),
|
|
org.AggregateType,
|
|
[]byte(`{
|
|
"key": "Subject",
|
|
"language": "en",
|
|
"template": "InitCode",
|
|
"text": "Test"
|
|
}`),
|
|
), org.CustomTextSetEventMapper),
|
|
},
|
|
reduce: (&messageTextProjection{}).reduceAdded,
|
|
want: wantReduce{
|
|
aggregateType: eventstore.AggregateType("org"),
|
|
sequence: 15,
|
|
previousSequence: 10,
|
|
projection: MessageTextTable,
|
|
executer: &testExecuter{
|
|
executions: []execution{
|
|
{
|
|
expectedStmt: "INSERT INTO projections.message_texts (aggregate_id, instance_id, creation_date, change_date, sequence, state, type, language, subject) VALUES ($1, $2, $3, $4, $5, $6, $7, $8, $9) ON CONFLICT (instance_id, aggregate_id, type, language) DO UPDATE SET (creation_date, change_date, sequence, state, subject) = (EXCLUDED.creation_date, EXCLUDED.change_date, EXCLUDED.sequence, EXCLUDED.state, EXCLUDED.subject)",
|
|
expectedArgs: []interface{}{
|
|
"agg-id",
|
|
"instance-id",
|
|
anyArg{},
|
|
anyArg{},
|
|
uint64(15),
|
|
domain.PolicyStateActive,
|
|
"InitCode",
|
|
"en",
|
|
"Test",
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
{
|
|
name: "org.reduceAdded.Greeting",
|
|
args: args{
|
|
event: getEvent(testEvent(
|
|
repository.EventType(org.CustomTextSetEventType),
|
|
org.AggregateType,
|
|
[]byte(`{
|
|
"key": "Greeting",
|
|
"language": "en",
|
|
"template": "InitCode",
|
|
"text": "Test"
|
|
}`),
|
|
), org.CustomTextSetEventMapper),
|
|
},
|
|
reduce: (&messageTextProjection{}).reduceAdded,
|
|
want: wantReduce{
|
|
aggregateType: eventstore.AggregateType("org"),
|
|
sequence: 15,
|
|
previousSequence: 10,
|
|
projection: MessageTextTable,
|
|
executer: &testExecuter{
|
|
executions: []execution{
|
|
{
|
|
expectedStmt: "INSERT INTO projections.message_texts (aggregate_id, instance_id, creation_date, change_date, sequence, state, type, language, greeting) VALUES ($1, $2, $3, $4, $5, $6, $7, $8, $9) ON CONFLICT (instance_id, aggregate_id, type, language) DO UPDATE SET (creation_date, change_date, sequence, state, greeting) = (EXCLUDED.creation_date, EXCLUDED.change_date, EXCLUDED.sequence, EXCLUDED.state, EXCLUDED.greeting)",
|
|
expectedArgs: []interface{}{
|
|
"agg-id",
|
|
"instance-id",
|
|
anyArg{},
|
|
anyArg{},
|
|
uint64(15),
|
|
domain.PolicyStateActive,
|
|
"InitCode",
|
|
"en",
|
|
"Test",
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
{
|
|
name: "org.reduceAdded.Text",
|
|
args: args{
|
|
event: getEvent(testEvent(
|
|
repository.EventType(org.CustomTextSetEventType),
|
|
org.AggregateType,
|
|
[]byte(`{
|
|
"key": "Text",
|
|
"language": "en",
|
|
"template": "InitCode",
|
|
"text": "Test"
|
|
}`),
|
|
), org.CustomTextSetEventMapper),
|
|
},
|
|
reduce: (&messageTextProjection{}).reduceAdded,
|
|
want: wantReduce{
|
|
aggregateType: eventstore.AggregateType("org"),
|
|
sequence: 15,
|
|
previousSequence: 10,
|
|
projection: MessageTextTable,
|
|
executer: &testExecuter{
|
|
executions: []execution{
|
|
{
|
|
expectedStmt: "INSERT INTO projections.message_texts (aggregate_id, instance_id, creation_date, change_date, sequence, state, type, language, text) VALUES ($1, $2, $3, $4, $5, $6, $7, $8, $9) ON CONFLICT (instance_id, aggregate_id, type, language) DO UPDATE SET (creation_date, change_date, sequence, state, text) = (EXCLUDED.creation_date, EXCLUDED.change_date, EXCLUDED.sequence, EXCLUDED.state, EXCLUDED.text)",
|
|
expectedArgs: []interface{}{
|
|
"agg-id",
|
|
"instance-id",
|
|
anyArg{},
|
|
anyArg{},
|
|
uint64(15),
|
|
domain.PolicyStateActive,
|
|
"InitCode",
|
|
"en",
|
|
"Test",
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
{
|
|
name: "org.reduceAdded.ButtonText",
|
|
args: args{
|
|
event: getEvent(testEvent(
|
|
repository.EventType(org.CustomTextSetEventType),
|
|
org.AggregateType,
|
|
[]byte(`{
|
|
"key": "ButtonText",
|
|
"language": "en",
|
|
"template": "InitCode",
|
|
"text": "Test"
|
|
}`),
|
|
), org.CustomTextSetEventMapper),
|
|
},
|
|
reduce: (&messageTextProjection{}).reduceAdded,
|
|
want: wantReduce{
|
|
aggregateType: eventstore.AggregateType("org"),
|
|
sequence: 15,
|
|
previousSequence: 10,
|
|
projection: MessageTextTable,
|
|
executer: &testExecuter{
|
|
executions: []execution{
|
|
{
|
|
expectedStmt: "INSERT INTO projections.message_texts (aggregate_id, instance_id, creation_date, change_date, sequence, state, type, language, button_text) VALUES ($1, $2, $3, $4, $5, $6, $7, $8, $9) ON CONFLICT (instance_id, aggregate_id, type, language) DO UPDATE SET (creation_date, change_date, sequence, state, button_text) = (EXCLUDED.creation_date, EXCLUDED.change_date, EXCLUDED.sequence, EXCLUDED.state, EXCLUDED.button_text)",
|
|
expectedArgs: []interface{}{
|
|
"agg-id",
|
|
"instance-id",
|
|
anyArg{},
|
|
anyArg{},
|
|
uint64(15),
|
|
domain.PolicyStateActive,
|
|
"InitCode",
|
|
"en",
|
|
"Test",
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
{
|
|
name: "org.reduceAdded.Footer",
|
|
args: args{
|
|
event: getEvent(testEvent(
|
|
repository.EventType(org.CustomTextSetEventType),
|
|
org.AggregateType,
|
|
[]byte(`{
|
|
"key": "Footer",
|
|
"language": "en",
|
|
"template": "InitCode",
|
|
"text": "Test"
|
|
}`),
|
|
), org.CustomTextSetEventMapper),
|
|
},
|
|
reduce: (&messageTextProjection{}).reduceAdded,
|
|
want: wantReduce{
|
|
aggregateType: eventstore.AggregateType("org"),
|
|
sequence: 15,
|
|
previousSequence: 10,
|
|
projection: MessageTextTable,
|
|
executer: &testExecuter{
|
|
executions: []execution{
|
|
{
|
|
expectedStmt: "INSERT INTO projections.message_texts (aggregate_id, instance_id, creation_date, change_date, sequence, state, type, language, footer_text) VALUES ($1, $2, $3, $4, $5, $6, $7, $8, $9) ON CONFLICT (instance_id, aggregate_id, type, language) DO UPDATE SET (creation_date, change_date, sequence, state, footer_text) = (EXCLUDED.creation_date, EXCLUDED.change_date, EXCLUDED.sequence, EXCLUDED.state, EXCLUDED.footer_text)",
|
|
expectedArgs: []interface{}{
|
|
"agg-id",
|
|
"instance-id",
|
|
anyArg{},
|
|
anyArg{},
|
|
uint64(15),
|
|
domain.PolicyStateActive,
|
|
"InitCode",
|
|
"en",
|
|
"Test",
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
{
|
|
name: "org.reduceRemoved.Title",
|
|
args: args{
|
|
event: getEvent(testEvent(
|
|
repository.EventType(org.CustomTextRemovedEventType),
|
|
org.AggregateType,
|
|
[]byte(`{
|
|
"key": "Title",
|
|
"language": "en",
|
|
"template": "InitCode"
|
|
}`),
|
|
), org.CustomTextRemovedEventMapper),
|
|
},
|
|
reduce: (&messageTextProjection{}).reduceRemoved,
|
|
want: wantReduce{
|
|
aggregateType: eventstore.AggregateType("org"),
|
|
sequence: 15,
|
|
previousSequence: 10,
|
|
projection: MessageTextTable,
|
|
executer: &testExecuter{
|
|
executions: []execution{
|
|
{
|
|
expectedStmt: "UPDATE projections.message_texts SET (change_date, sequence, title) = ($1, $2, $3) WHERE (aggregate_id = $4) AND (type = $5) AND (language = $6)",
|
|
expectedArgs: []interface{}{
|
|
anyArg{},
|
|
uint64(15),
|
|
"",
|
|
"agg-id",
|
|
"InitCode",
|
|
"en",
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
{
|
|
name: "org.reduceRemoved.PreHeader",
|
|
args: args{
|
|
event: getEvent(testEvent(
|
|
repository.EventType(org.CustomTextRemovedEventType),
|
|
org.AggregateType,
|
|
[]byte(`{
|
|
"key": "PreHeader",
|
|
"language": "en",
|
|
"template": "InitCode"
|
|
}`),
|
|
), org.CustomTextRemovedEventMapper),
|
|
},
|
|
reduce: (&messageTextProjection{}).reduceRemoved,
|
|
want: wantReduce{
|
|
aggregateType: eventstore.AggregateType("org"),
|
|
sequence: 15,
|
|
previousSequence: 10,
|
|
projection: MessageTextTable,
|
|
executer: &testExecuter{
|
|
executions: []execution{
|
|
{
|
|
expectedStmt: "UPDATE projections.message_texts SET (change_date, sequence, pre_header) = ($1, $2, $3) WHERE (aggregate_id = $4) AND (type = $5) AND (language = $6)",
|
|
expectedArgs: []interface{}{
|
|
anyArg{},
|
|
uint64(15),
|
|
"",
|
|
"agg-id",
|
|
"InitCode",
|
|
"en",
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
{
|
|
name: "org.reduceRemoved.Subject",
|
|
args: args{
|
|
event: getEvent(testEvent(
|
|
repository.EventType(org.CustomTextRemovedEventType),
|
|
org.AggregateType,
|
|
[]byte(`{
|
|
"key": "Subject",
|
|
"language": "en",
|
|
"template": "InitCode"
|
|
}`),
|
|
), org.CustomTextRemovedEventMapper),
|
|
},
|
|
reduce: (&messageTextProjection{}).reduceRemoved,
|
|
want: wantReduce{
|
|
aggregateType: eventstore.AggregateType("org"),
|
|
sequence: 15,
|
|
previousSequence: 10,
|
|
projection: MessageTextTable,
|
|
executer: &testExecuter{
|
|
executions: []execution{
|
|
{
|
|
expectedStmt: "UPDATE projections.message_texts SET (change_date, sequence, subject) = ($1, $2, $3) WHERE (aggregate_id = $4) AND (type = $5) AND (language = $6)",
|
|
expectedArgs: []interface{}{
|
|
anyArg{},
|
|
uint64(15),
|
|
"",
|
|
"agg-id",
|
|
"InitCode",
|
|
"en",
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
{
|
|
name: "org.reduceRemoved.Greeting",
|
|
args: args{
|
|
event: getEvent(testEvent(
|
|
repository.EventType(org.CustomTextRemovedEventType),
|
|
org.AggregateType,
|
|
[]byte(`{
|
|
"key": "Greeting",
|
|
"language": "en",
|
|
"template": "InitCode"
|
|
}`),
|
|
), org.CustomTextRemovedEventMapper),
|
|
},
|
|
reduce: (&messageTextProjection{}).reduceRemoved,
|
|
want: wantReduce{
|
|
aggregateType: eventstore.AggregateType("org"),
|
|
sequence: 15,
|
|
previousSequence: 10,
|
|
projection: MessageTextTable,
|
|
executer: &testExecuter{
|
|
executions: []execution{
|
|
{
|
|
expectedStmt: "UPDATE projections.message_texts SET (change_date, sequence, greeting) = ($1, $2, $3) WHERE (aggregate_id = $4) AND (type = $5) AND (language = $6)",
|
|
expectedArgs: []interface{}{
|
|
anyArg{},
|
|
uint64(15),
|
|
"",
|
|
"agg-id",
|
|
"InitCode",
|
|
"en",
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
{
|
|
name: "org.reduceRemoved.Text",
|
|
args: args{
|
|
event: getEvent(testEvent(
|
|
repository.EventType(org.CustomTextRemovedEventType),
|
|
org.AggregateType,
|
|
[]byte(`{
|
|
"key": "Text",
|
|
"language": "en",
|
|
"template": "InitCode"
|
|
}`),
|
|
), org.CustomTextRemovedEventMapper),
|
|
},
|
|
reduce: (&messageTextProjection{}).reduceRemoved,
|
|
want: wantReduce{
|
|
aggregateType: eventstore.AggregateType("org"),
|
|
sequence: 15,
|
|
previousSequence: 10,
|
|
projection: MessageTextTable,
|
|
executer: &testExecuter{
|
|
executions: []execution{
|
|
{
|
|
expectedStmt: "UPDATE projections.message_texts SET (change_date, sequence, text) = ($1, $2, $3) WHERE (aggregate_id = $4) AND (type = $5) AND (language = $6)",
|
|
expectedArgs: []interface{}{
|
|
anyArg{},
|
|
uint64(15),
|
|
"",
|
|
"agg-id",
|
|
"InitCode",
|
|
"en",
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
{
|
|
name: "org.reduceRemoved.ButtonText",
|
|
args: args{
|
|
event: getEvent(testEvent(
|
|
repository.EventType(org.CustomTextRemovedEventType),
|
|
org.AggregateType,
|
|
[]byte(`{
|
|
"key": "ButtonText",
|
|
"language": "en",
|
|
"template": "InitCode"
|
|
}`),
|
|
), org.CustomTextRemovedEventMapper),
|
|
},
|
|
reduce: (&messageTextProjection{}).reduceRemoved,
|
|
want: wantReduce{
|
|
aggregateType: eventstore.AggregateType("org"),
|
|
sequence: 15,
|
|
previousSequence: 10,
|
|
projection: MessageTextTable,
|
|
executer: &testExecuter{
|
|
executions: []execution{
|
|
{
|
|
expectedStmt: "UPDATE projections.message_texts SET (change_date, sequence, button_text) = ($1, $2, $3) WHERE (aggregate_id = $4) AND (type = $5) AND (language = $6)",
|
|
expectedArgs: []interface{}{
|
|
anyArg{},
|
|
uint64(15),
|
|
"",
|
|
"agg-id",
|
|
"InitCode",
|
|
"en",
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
{
|
|
name: "org.reduceRemoved.Footer",
|
|
args: args{
|
|
event: getEvent(testEvent(
|
|
repository.EventType(org.CustomTextRemovedEventType),
|
|
org.AggregateType,
|
|
[]byte(`{
|
|
"key": "Footer",
|
|
"language": "en",
|
|
"template": "InitCode"
|
|
}`),
|
|
), org.CustomTextRemovedEventMapper),
|
|
},
|
|
reduce: (&messageTextProjection{}).reduceRemoved,
|
|
want: wantReduce{
|
|
aggregateType: eventstore.AggregateType("org"),
|
|
sequence: 15,
|
|
previousSequence: 10,
|
|
projection: MessageTextTable,
|
|
executer: &testExecuter{
|
|
executions: []execution{
|
|
{
|
|
expectedStmt: "UPDATE projections.message_texts SET (change_date, sequence, footer_text) = ($1, $2, $3) WHERE (aggregate_id = $4) AND (type = $5) AND (language = $6)",
|
|
expectedArgs: []interface{}{
|
|
anyArg{},
|
|
uint64(15),
|
|
"",
|
|
"agg-id",
|
|
"InitCode",
|
|
"en",
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
{
|
|
name: "org.reduceRemoved",
|
|
reduce: (&messageTextProjection{}).reduceTemplateRemoved,
|
|
args: args{
|
|
event: getEvent(testEvent(
|
|
repository.EventType(org.CustomTextTemplateRemovedEventType),
|
|
org.AggregateType,
|
|
[]byte(`{
|
|
"key": "Title",
|
|
"language": "en",
|
|
"template": "InitCode"
|
|
}`),
|
|
), org.CustomTextTemplateRemovedEventMapper),
|
|
},
|
|
want: wantReduce{
|
|
aggregateType: eventstore.AggregateType("org"),
|
|
sequence: 15,
|
|
previousSequence: 10,
|
|
projection: MessageTextTable,
|
|
executer: &testExecuter{
|
|
executions: []execution{
|
|
{
|
|
expectedStmt: "DELETE FROM projections.message_texts WHERE (aggregate_id = $1) AND (type = $2) AND (language = $3)",
|
|
expectedArgs: []interface{}{
|
|
"agg-id",
|
|
"InitCode",
|
|
"en",
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
{
|
|
name: "instance.reduceAdded",
|
|
reduce: (&messageTextProjection{}).reduceAdded,
|
|
args: args{
|
|
event: getEvent(testEvent(
|
|
repository.EventType(instance.CustomTextSetEventType),
|
|
instance.AggregateType,
|
|
[]byte(`{
|
|
"key": "Title",
|
|
"language": "en",
|
|
"template": "InitCode",
|
|
"text": "Test"
|
|
}`),
|
|
), instance.CustomTextSetEventMapper),
|
|
},
|
|
want: wantReduce{
|
|
aggregateType: eventstore.AggregateType("instance"),
|
|
sequence: 15,
|
|
previousSequence: 10,
|
|
projection: MessageTextTable,
|
|
executer: &testExecuter{
|
|
executions: []execution{
|
|
{
|
|
expectedStmt: "INSERT INTO projections.message_texts (aggregate_id, instance_id, creation_date, change_date, sequence, state, type, language, title) VALUES ($1, $2, $3, $4, $5, $6, $7, $8, $9) ON CONFLICT (instance_id, aggregate_id, type, language) DO UPDATE SET (creation_date, change_date, sequence, state, title) = (EXCLUDED.creation_date, EXCLUDED.change_date, EXCLUDED.sequence, EXCLUDED.state, EXCLUDED.title)",
|
|
expectedArgs: []interface{}{
|
|
"agg-id",
|
|
"instance-id",
|
|
anyArg{},
|
|
anyArg{},
|
|
uint64(15),
|
|
domain.PolicyStateActive,
|
|
"InitCode",
|
|
"en",
|
|
"Test",
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
{
|
|
name: "instance.reduceRemoved.Title",
|
|
args: args{
|
|
event: getEvent(testEvent(
|
|
repository.EventType(instance.CustomTextRemovedEventType),
|
|
instance.AggregateType,
|
|
[]byte(`{
|
|
"key": "Title",
|
|
"language": "en",
|
|
"template": "InitCode"
|
|
}`),
|
|
), instance.CustomTextRemovedEventMapper),
|
|
},
|
|
reduce: (&messageTextProjection{}).reduceRemoved,
|
|
want: wantReduce{
|
|
aggregateType: eventstore.AggregateType("instance"),
|
|
sequence: 15,
|
|
previousSequence: 10,
|
|
projection: MessageTextTable,
|
|
executer: &testExecuter{
|
|
executions: []execution{
|
|
{
|
|
expectedStmt: "UPDATE projections.message_texts SET (change_date, sequence, title) = ($1, $2, $3) WHERE (aggregate_id = $4) AND (type = $5) AND (language = $6)",
|
|
expectedArgs: []interface{}{
|
|
anyArg{},
|
|
uint64(15),
|
|
"",
|
|
"agg-id",
|
|
"InitCode",
|
|
"en",
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
}
|
|
for _, tt := range tests {
|
|
t.Run(tt.name, func(t *testing.T) {
|
|
event := baseEvent(t)
|
|
got, err := tt.reduce(event)
|
|
if _, ok := err.(errors.InvalidArgument); !ok {
|
|
t.Errorf("no wrong event mapping: %v, got: %v", err, got)
|
|
}
|
|
|
|
event = tt.args.event(t)
|
|
got, err = tt.reduce(event)
|
|
assertReduce(t, got, err, tt.want)
|
|
})
|
|
}
|
|
}
|