mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 19:14:23 +00:00
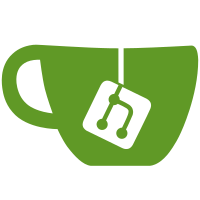
* check uniqueness on create and register user * change user email, reserve release unique email * usergrant unique aggregate * usergrant uniqueness * validate UserGrant * fix tests * domain is set on username in all orgs * domain in admin * org domain sql * zitadel domain org name * org domains * org iam policy * default org iam policy * SETUP * load login names * login by login name * login name * fix: merge master * fix: merge master * Update internal/user/repository/eventsourcing/user.go Co-authored-by: Livio Amstutz <livio.a@gmail.com> * fix: fix unique domains * fix: rename env variable Co-authored-by: adlerhurst <silvan.reusser@gmail.com> Co-authored-by: Livio Amstutz <livio.a@gmail.com>
62 lines
1.5 KiB
Go
62 lines
1.5 KiB
Go
package model
|
|
|
|
import (
|
|
"time"
|
|
|
|
req_model "github.com/caos/zitadel/internal/auth_request/model"
|
|
"github.com/caos/zitadel/internal/model"
|
|
)
|
|
|
|
type UserSessionView struct {
|
|
CreationDate time.Time
|
|
ChangeDate time.Time
|
|
State req_model.UserSessionState
|
|
ResourceOwner string
|
|
UserAgentID string
|
|
UserID string
|
|
UserName string
|
|
PasswordVerification time.Time
|
|
MfaSoftwareVerification time.Time
|
|
MfaSoftwareVerificationType req_model.MfaType
|
|
MfaHardwareVerification time.Time
|
|
MfaHardwareVerificationType req_model.MfaType
|
|
Sequence uint64
|
|
}
|
|
|
|
type UserSessionSearchRequest struct {
|
|
Offset uint64
|
|
Limit uint64
|
|
SortingColumn UserSessionSearchKey
|
|
Asc bool
|
|
Queries []*UserSessionSearchQuery
|
|
}
|
|
|
|
type UserSessionSearchKey int32
|
|
|
|
const (
|
|
USERSESSIONSEARCHKEY_UNSPECIFIED UserSessionSearchKey = iota
|
|
USERSESSIONSEARCHKEY_USER_AGENT_ID
|
|
USERSESSIONSEARCHKEY_USER_ID
|
|
USERSESSIONSEARCHKEY_STATE
|
|
USERSESSIONSEARCHKEY_RESOURCEOWNER
|
|
)
|
|
|
|
type UserSessionSearchQuery struct {
|
|
Key UserSessionSearchKey
|
|
Method model.SearchMethod
|
|
Value interface{}
|
|
}
|
|
|
|
type UserSessionSearchResponse struct {
|
|
Offset uint64
|
|
Limit uint64
|
|
TotalResult uint64
|
|
Result []*UserSessionView
|
|
}
|
|
|
|
func (r *UserSessionSearchRequest) EnsureLimit(limit uint64) {
|
|
if r.Limit == 0 || r.Limit > limit {
|
|
r.Limit = limit
|
|
}
|
|
}
|