mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
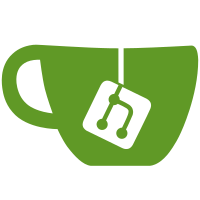
# Which Problems Are Solved Implement a new API service that allows management of OIDC signing web keys. This allows users to manage rotation of the instance level keys. which are currently managed based on expiry. The API accepts the generation of the following key types and parameters: - RSA keys with 2048, 3072 or 4096 bit in size and: - Signing with SHA-256 (RS256) - Signing with SHA-384 (RS384) - Signing with SHA-512 (RS512) - ECDSA keys with - P256 curve - P384 curve - P512 curve - ED25519 keys # How the Problems Are Solved Keys are serialized for storage using the JSON web key format from the `jose` library. This is the format that will be used by OIDC for signing, verification and publication. Each instance can have a number of key pairs. All existing public keys are meant to be used for token verification and publication the keys endpoint. Keys can be activated and the active private key is meant to sign new tokens. There is always exactly 1 active signing key: 1. When the first key for an instance is generated, it is automatically activated. 2. Activation of the next key automatically deactivates the previously active key. 3. Keys cannot be manually deactivated from the API 4. Active keys cannot be deleted # Additional Changes - Query methods that later will be used by the OIDC package are already implemented. Preparation for #8031 - Fix indentation in french translation for instance event - Move user_schema translations to consistent positions in all translation files # Additional Context - Closes #8030 - Part of #7809 --------- Co-authored-by: Elio Bischof <elio@zitadel.com>
137 lines
3.4 KiB
Go
137 lines
3.4 KiB
Go
// Code generated by "enumer -type RSABits -trimprefix RSABits -text -json -linecomment"; DO NOT EDIT.
|
|
|
|
package crypto
|
|
|
|
import (
|
|
"encoding/json"
|
|
"fmt"
|
|
"strings"
|
|
)
|
|
|
|
const (
|
|
_RSABitsName_0 = ""
|
|
_RSABitsLowerName_0 = ""
|
|
_RSABitsName_1 = "2048"
|
|
_RSABitsLowerName_1 = "2048"
|
|
_RSABitsName_2 = "3072"
|
|
_RSABitsLowerName_2 = "3072"
|
|
_RSABitsName_3 = "4096"
|
|
_RSABitsLowerName_3 = "4096"
|
|
)
|
|
|
|
var (
|
|
_RSABitsIndex_0 = [...]uint8{0, 0}
|
|
_RSABitsIndex_1 = [...]uint8{0, 4}
|
|
_RSABitsIndex_2 = [...]uint8{0, 4}
|
|
_RSABitsIndex_3 = [...]uint8{0, 4}
|
|
)
|
|
|
|
func (i RSABits) String() string {
|
|
switch {
|
|
case i == 0:
|
|
return _RSABitsName_0
|
|
case i == 2048:
|
|
return _RSABitsName_1
|
|
case i == 3072:
|
|
return _RSABitsName_2
|
|
case i == 4096:
|
|
return _RSABitsName_3
|
|
default:
|
|
return fmt.Sprintf("RSABits(%d)", i)
|
|
}
|
|
}
|
|
|
|
// An "invalid array index" compiler error signifies that the constant values have changed.
|
|
// Re-run the stringer command to generate them again.
|
|
func _RSABitsNoOp() {
|
|
var x [1]struct{}
|
|
_ = x[RSABitsUnspecified-(0)]
|
|
_ = x[RSABits2048-(2048)]
|
|
_ = x[RSABits3072-(3072)]
|
|
_ = x[RSABits4096-(4096)]
|
|
}
|
|
|
|
var _RSABitsValues = []RSABits{RSABitsUnspecified, RSABits2048, RSABits3072, RSABits4096}
|
|
|
|
var _RSABitsNameToValueMap = map[string]RSABits{
|
|
_RSABitsName_0[0:0]: RSABitsUnspecified,
|
|
_RSABitsLowerName_0[0:0]: RSABitsUnspecified,
|
|
_RSABitsName_1[0:4]: RSABits2048,
|
|
_RSABitsLowerName_1[0:4]: RSABits2048,
|
|
_RSABitsName_2[0:4]: RSABits3072,
|
|
_RSABitsLowerName_2[0:4]: RSABits3072,
|
|
_RSABitsName_3[0:4]: RSABits4096,
|
|
_RSABitsLowerName_3[0:4]: RSABits4096,
|
|
}
|
|
|
|
var _RSABitsNames = []string{
|
|
_RSABitsName_0[0:0],
|
|
_RSABitsName_1[0:4],
|
|
_RSABitsName_2[0:4],
|
|
_RSABitsName_3[0:4],
|
|
}
|
|
|
|
// RSABitsString retrieves an enum value from the enum constants string name.
|
|
// Throws an error if the param is not part of the enum.
|
|
func RSABitsString(s string) (RSABits, error) {
|
|
if val, ok := _RSABitsNameToValueMap[s]; ok {
|
|
return val, nil
|
|
}
|
|
|
|
if val, ok := _RSABitsNameToValueMap[strings.ToLower(s)]; ok {
|
|
return val, nil
|
|
}
|
|
return 0, fmt.Errorf("%s does not belong to RSABits values", s)
|
|
}
|
|
|
|
// RSABitsValues returns all values of the enum
|
|
func RSABitsValues() []RSABits {
|
|
return _RSABitsValues
|
|
}
|
|
|
|
// RSABitsStrings returns a slice of all String values of the enum
|
|
func RSABitsStrings() []string {
|
|
strs := make([]string, len(_RSABitsNames))
|
|
copy(strs, _RSABitsNames)
|
|
return strs
|
|
}
|
|
|
|
// IsARSABits returns "true" if the value is listed in the enum definition. "false" otherwise
|
|
func (i RSABits) IsARSABits() bool {
|
|
for _, v := range _RSABitsValues {
|
|
if i == v {
|
|
return true
|
|
}
|
|
}
|
|
return false
|
|
}
|
|
|
|
// MarshalJSON implements the json.Marshaler interface for RSABits
|
|
func (i RSABits) MarshalJSON() ([]byte, error) {
|
|
return json.Marshal(i.String())
|
|
}
|
|
|
|
// UnmarshalJSON implements the json.Unmarshaler interface for RSABits
|
|
func (i *RSABits) UnmarshalJSON(data []byte) error {
|
|
var s string
|
|
if err := json.Unmarshal(data, &s); err != nil {
|
|
return fmt.Errorf("RSABits should be a string, got %s", data)
|
|
}
|
|
|
|
var err error
|
|
*i, err = RSABitsString(s)
|
|
return err
|
|
}
|
|
|
|
// MarshalText implements the encoding.TextMarshaler interface for RSABits
|
|
func (i RSABits) MarshalText() ([]byte, error) {
|
|
return []byte(i.String()), nil
|
|
}
|
|
|
|
// UnmarshalText implements the encoding.TextUnmarshaler interface for RSABits
|
|
func (i *RSABits) UnmarshalText(text []byte) error {
|
|
var err error
|
|
*i, err = RSABitsString(string(text))
|
|
return err
|
|
}
|