mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
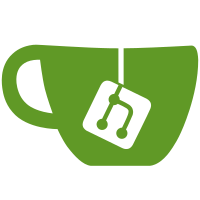
* start v2 * start * run * some cleanup * remove v2 pkg again * simplify * webauthn * remove unused config * fix login path in Dockerfile * fix asset_generator.go * health handler * fix grpc web * refactor * merge * build new main.go * run new main.go * update logging pkg * fix error msg * update logging * cleanup * cleanup * go mod tidy * change localDevMode * fix customEndpoints * update logging * comments * change local flag to external configs * fix location generated go code * fix Co-authored-by: fforootd <florian@caos.ch>
61 lines
1.3 KiB
Go
61 lines
1.3 KiB
Go
package handler
|
|
|
|
import (
|
|
"time"
|
|
|
|
"github.com/caos/zitadel/internal/admin/repository/eventsourcing/view"
|
|
v1 "github.com/caos/zitadel/internal/eventstore/v1"
|
|
"github.com/caos/zitadel/internal/eventstore/v1/query"
|
|
"github.com/caos/zitadel/internal/static"
|
|
)
|
|
|
|
type Configs map[string]*Config
|
|
|
|
type Config struct {
|
|
MinimumCycleDuration time.Duration
|
|
}
|
|
|
|
type handler struct {
|
|
view *view.View
|
|
bulkLimit uint64
|
|
cycleDuration time.Duration
|
|
errorCountUntilSkip uint64
|
|
|
|
es v1.Eventstore
|
|
}
|
|
|
|
func (h *handler) Eventstore() v1.Eventstore {
|
|
return h.es
|
|
}
|
|
|
|
func Register(configs Configs, bulkLimit, errorCount uint64, view *view.View, es v1.Eventstore, static static.Storage, loginPrefix string) []query.Handler {
|
|
handlers := []query.Handler{}
|
|
if static != nil {
|
|
handlers = append(handlers, newStyling(
|
|
handler{view, bulkLimit, configs.cycleDuration("Styling"), errorCount, es},
|
|
static,
|
|
loginPrefix))
|
|
}
|
|
return handlers
|
|
}
|
|
|
|
func (configs Configs) cycleDuration(viewModel string) time.Duration {
|
|
c, ok := configs[viewModel]
|
|
if !ok {
|
|
return 3 * time.Minute
|
|
}
|
|
return c.MinimumCycleDuration
|
|
}
|
|
|
|
func (h *handler) MinimumCycleDuration() time.Duration {
|
|
return h.cycleDuration
|
|
}
|
|
|
|
func (h *handler) LockDuration() time.Duration {
|
|
return h.cycleDuration / 3
|
|
}
|
|
|
|
func (h *handler) QueryLimit() uint64 {
|
|
return h.bulkLimit
|
|
}
|