mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
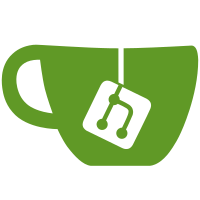
* feat: return 404 or 409 if org reg disallowed * fix: system limit permissions * feat: add iam limits api * feat: disallow public org registrations on default instance * add integration test * test: integration * fix test * docs: describe public org registrations * avoid updating docs deps * fix system limits integration test * silence integration tests * fix linting * ignore strange linter complaints * review * improve reset properties naming * redefine the api * use restrictions aggregate * test query * simplify and test projection * test commands * fix unit tests * move integration test * support restrictions on default instance * also test GetRestrictions * self review * lint * abstract away resource owner * fix tests * configure supported languages * fix allowed languages * fix tests * default lang must not be restricted * preferred language must be allowed * change preferred languages * check languages everywhere * lint * test command side * lint * add integration test * add integration test * restrict supported ui locales * lint * lint * cleanup * lint * allow undefined preferred language * fix integration tests * update main * fix env var * ignore linter * ignore linter * improve integration test config * reduce cognitive complexity * compile * check for duplicates * remove useless restriction checks * review * revert restriction renaming * fix language restrictions * lint * generate * allow custom texts for supported langs for now * fix tests * cleanup * cleanup * cleanup * lint * unsupported preferred lang is allowed * fix integration test * finish reverting to old property name * finish reverting to old property name * load languages * refactor(i18n): centralize translators and fs * lint * amplify no validations on preferred languages * fix integration test * lint * fix resetting allowed languages * test unchanged restrictions
102 lines
2.3 KiB
Go
102 lines
2.3 KiB
Go
package eventstore
|
|
|
|
import (
|
|
"context"
|
|
|
|
"github.com/zitadel/zitadel/internal/api/authz"
|
|
)
|
|
|
|
type aggregateOpt func(*Aggregate)
|
|
|
|
// NewAggregate is the default constructor of an aggregate
|
|
// opts overwrite values calculated by given parameters
|
|
func NewAggregate(
|
|
ctx context.Context,
|
|
id string,
|
|
typ AggregateType,
|
|
version Version,
|
|
opts ...aggregateOpt,
|
|
) *Aggregate {
|
|
a := &Aggregate{
|
|
ID: id,
|
|
Type: typ,
|
|
ResourceOwner: authz.GetCtxData(ctx).OrgID,
|
|
InstanceID: authz.GetInstance(ctx).InstanceID(),
|
|
Version: version,
|
|
}
|
|
|
|
for _, opt := range opts {
|
|
opt(a)
|
|
}
|
|
|
|
return a
|
|
}
|
|
|
|
// WithResourceOwner overwrites the resource owner of the aggregate
|
|
// by default the resource owner is set by the context
|
|
func WithResourceOwner(resourceOwner string) aggregateOpt {
|
|
return func(aggregate *Aggregate) {
|
|
aggregate.ResourceOwner = resourceOwner
|
|
}
|
|
}
|
|
|
|
// WithInstanceID overwrites the instance id of the aggregate
|
|
// by default the instance is set by the context
|
|
func WithInstanceID(id string) aggregateOpt {
|
|
return func(aggregate *Aggregate) {
|
|
aggregate.InstanceID = id
|
|
}
|
|
}
|
|
|
|
// AggregateFromWriteModel maps the given WriteModel to an Aggregate
|
|
func AggregateFromWriteModel(
|
|
wm *WriteModel,
|
|
typ AggregateType,
|
|
version Version,
|
|
) *Aggregate {
|
|
return NewAggregate(
|
|
// TODO: the linter complains if this function is called without passing a context
|
|
context.Background(),
|
|
wm.AggregateID,
|
|
typ,
|
|
version,
|
|
WithResourceOwner(wm.ResourceOwner),
|
|
WithInstanceID(wm.InstanceID),
|
|
)
|
|
}
|
|
|
|
// Aggregate is the basic implementation of Aggregater
|
|
type Aggregate struct {
|
|
// ID is the unique identitfier of this aggregate
|
|
ID string `json:"-"`
|
|
// Type is the name of the aggregate.
|
|
Type AggregateType `json:"-"`
|
|
// ResourceOwner is the org this aggregates belongs to
|
|
ResourceOwner string `json:"-"`
|
|
// InstanceID is the instance this aggregate belongs to
|
|
InstanceID string `json:"-"`
|
|
// Version is the semver this aggregate represents
|
|
Version Version `json:"-"`
|
|
}
|
|
|
|
// AggregateType is the object name
|
|
type AggregateType string
|
|
|
|
func isAggregateTypes(a *Aggregate, types ...AggregateType) bool {
|
|
for _, typ := range types {
|
|
if a.Type == typ {
|
|
return true
|
|
}
|
|
}
|
|
return false
|
|
}
|
|
|
|
func isAggregateIDs(a *Aggregate, ids ...string) bool {
|
|
for _, id := range ids {
|
|
if a.ID == id {
|
|
return true
|
|
}
|
|
}
|
|
return false
|
|
}
|