mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
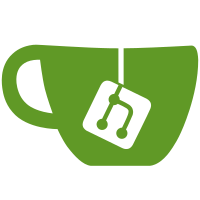
* feat(instance): implement create instance with direct machine user and credentials
* fix: deprecated add endpoint and variable declaration
* fix(instance): update logic for pats and machinekeys
* fix(instance): unit test corrections and additional unit test for pats and machinekeys
* fix(instance-create): include review changes
* fix(instance-create): linter fixes
* move iframe usage to solution scenarios configurations
* Revert "move iframe usage to solution scenarios configurations"
This reverts commit 9db31f3808
.
* fix merge
* fix: add review suggestions
Co-authored-by: Livio Spring <livio.a@gmail.com>
* fix: add review changes
* fix: add review changes for default definitions
* fix: add review changes for machinekey details
* fix: add machinekey output when setup with machineuser
* fix: add changes from review
* fix instance converter for machine and allow overwriting of further machine fields
Co-authored-by: Livio Spring <livio.a@gmail.com>
61 lines
1.3 KiB
Go
61 lines
1.3 KiB
Go
package domain
|
|
|
|
import (
|
|
"encoding/json"
|
|
"time"
|
|
|
|
"github.com/zitadel/zitadel/internal/errors"
|
|
"github.com/zitadel/zitadel/internal/eventstore/v1/models"
|
|
)
|
|
|
|
type ApplicationKey struct {
|
|
models.ObjectRoot
|
|
|
|
ApplicationID string
|
|
ClientID string
|
|
KeyID string
|
|
Type AuthNKeyType
|
|
ExpirationDate time.Time
|
|
PrivateKey []byte
|
|
PublicKey []byte
|
|
}
|
|
|
|
func (k *ApplicationKey) SetPublicKey(publicKey []byte) {
|
|
k.PublicKey = publicKey
|
|
}
|
|
|
|
func (k *ApplicationKey) SetPrivateKey(privateKey []byte) {
|
|
k.PrivateKey = privateKey
|
|
}
|
|
|
|
func (k *ApplicationKey) GetExpirationDate() time.Time {
|
|
return k.ExpirationDate
|
|
}
|
|
|
|
func (k *ApplicationKey) SetExpirationDate(expiration time.Time) {
|
|
k.ExpirationDate = expiration
|
|
}
|
|
|
|
func (k *ApplicationKey) Detail() ([]byte, error) {
|
|
if k.Type == AuthNKeyTypeJSON {
|
|
return k.MarshalJSON()
|
|
}
|
|
return nil, errors.ThrowPreconditionFailed(nil, "KEY-dsg52", "Errors.Internal")
|
|
}
|
|
|
|
func (k *ApplicationKey) MarshalJSON() ([]byte, error) {
|
|
return json.Marshal(struct {
|
|
Type string `json:"type"`
|
|
KeyID string `json:"keyId"`
|
|
Key string `json:"key"`
|
|
AppID string `json:"appId"`
|
|
ClientID string `json:"clientId"`
|
|
}{
|
|
Type: "application",
|
|
KeyID: k.KeyID,
|
|
Key: string(k.PrivateKey),
|
|
AppID: k.ApplicationID,
|
|
ClientID: k.ClientID,
|
|
})
|
|
}
|