mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
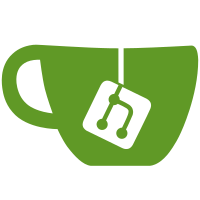
* feat(api): feature API proto definitions * update proto based on discussion with @livio-a * cleanup old feature flag stuff * authz instance queries * align defaults * projection definitions * define commands and event reducers * implement system and instance setter APIs * api getter implementation * unit test repository package * command unit tests * unit test Get queries * grpc converter unit tests * migrate the V1 features * migrate oidc to dynamic features * projection unit test * fix instance by host * fix instance by id data type in sql * fix linting errors * add system projection test * fix behavior inversion * resolve proto file comments * rename SystemDefaultLoginInstanceEventType to SystemLoginDefaultOrgEventType so it's consistent with the instance level event * use write models and conditional set events * system features integration tests * instance features integration tests * error on empty request * documentation entry * typo in feature.proto * fix start unit tests * solve linting error on key case switch * remove system defaults after discussion with @eliobischof * fix system feature projection * resolve comments in defaults.yaml --------- Co-authored-by: Livio Spring <livio.a@gmail.com>
50 lines
1.4 KiB
Go
50 lines
1.4 KiB
Go
package eventstore
|
|
|
|
import "time"
|
|
|
|
// ReadModel is the minimum representation of a read model.
|
|
// It implements a basic reducer
|
|
// it might be saved in a database or in memory
|
|
type ReadModel struct {
|
|
AggregateID string `json:"-"`
|
|
ProcessedSequence uint64 `json:"-"`
|
|
CreationDate time.Time `json:"-"`
|
|
ChangeDate time.Time `json:"-"`
|
|
Events []Event `json:"-"`
|
|
ResourceOwner string `json:"-"`
|
|
InstanceID string `json:"-"`
|
|
}
|
|
|
|
// AppendEvents adds all the events to the read model.
|
|
// The function doesn't compute the new state of the read model
|
|
func (rm *ReadModel) AppendEvents(events ...Event) {
|
|
rm.Events = append(rm.Events, events...)
|
|
}
|
|
|
|
// Reduce is the basic implementation of reducer
|
|
// If this function is extended the extending function should be the last step
|
|
func (rm *ReadModel) Reduce() error {
|
|
if len(rm.Events) == 0 {
|
|
return nil
|
|
}
|
|
|
|
if rm.AggregateID == "" {
|
|
rm.AggregateID = rm.Events[0].Aggregate().ID
|
|
}
|
|
if rm.ResourceOwner == "" {
|
|
rm.ResourceOwner = rm.Events[0].Aggregate().ResourceOwner
|
|
}
|
|
if rm.InstanceID == "" {
|
|
rm.InstanceID = rm.Events[0].Aggregate().InstanceID
|
|
}
|
|
|
|
if rm.CreationDate.IsZero() {
|
|
rm.CreationDate = rm.Events[0].CreatedAt()
|
|
}
|
|
rm.ChangeDate = rm.Events[len(rm.Events)-1].CreatedAt()
|
|
rm.ProcessedSequence = rm.Events[len(rm.Events)-1].Sequence()
|
|
// all events processed and not needed anymore
|
|
rm.Events = rm.Events[0:0]
|
|
return nil
|
|
}
|