mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
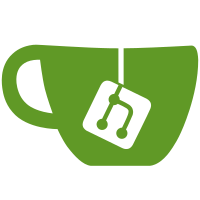
* fix: add setup step to correct creation dates * fix(eventstore): replace now with statement ts * fix(step10): correct number * fix: handle wrong instance domain removed events
54 lines
1000 B
Go
54 lines
1000 B
Go
package setup
|
|
|
|
import (
|
|
"context"
|
|
_ "embed"
|
|
|
|
"github.com/zitadel/logging"
|
|
|
|
"github.com/zitadel/zitadel/internal/database"
|
|
)
|
|
|
|
var (
|
|
//go:embed 10.sql
|
|
correctCreationDate10 string
|
|
)
|
|
|
|
type CorrectCreationDate struct {
|
|
dbClient *database.DB
|
|
}
|
|
|
|
func (mig *CorrectCreationDate) Execute(ctx context.Context) (err error) {
|
|
tx, err := mig.dbClient.Begin()
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if mig.dbClient.Type() == "cockroach" {
|
|
if _, err := tx.Exec("SET experimental_enable_temp_tables=on"); err != nil {
|
|
return err
|
|
}
|
|
}
|
|
defer func() {
|
|
if err != nil {
|
|
logging.OnError(tx.Rollback()).Debug("rollback failed")
|
|
return
|
|
}
|
|
err = tx.Commit()
|
|
}()
|
|
for {
|
|
res, err := tx.ExecContext(ctx, correctCreationDate10)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
affected, _ := res.RowsAffected()
|
|
logging.WithFields("count", affected).Info("creation dates changed")
|
|
if affected == 0 {
|
|
return nil
|
|
}
|
|
}
|
|
}
|
|
|
|
func (mig *CorrectCreationDate) String() string {
|
|
return "10_correct_creation_date"
|
|
}
|